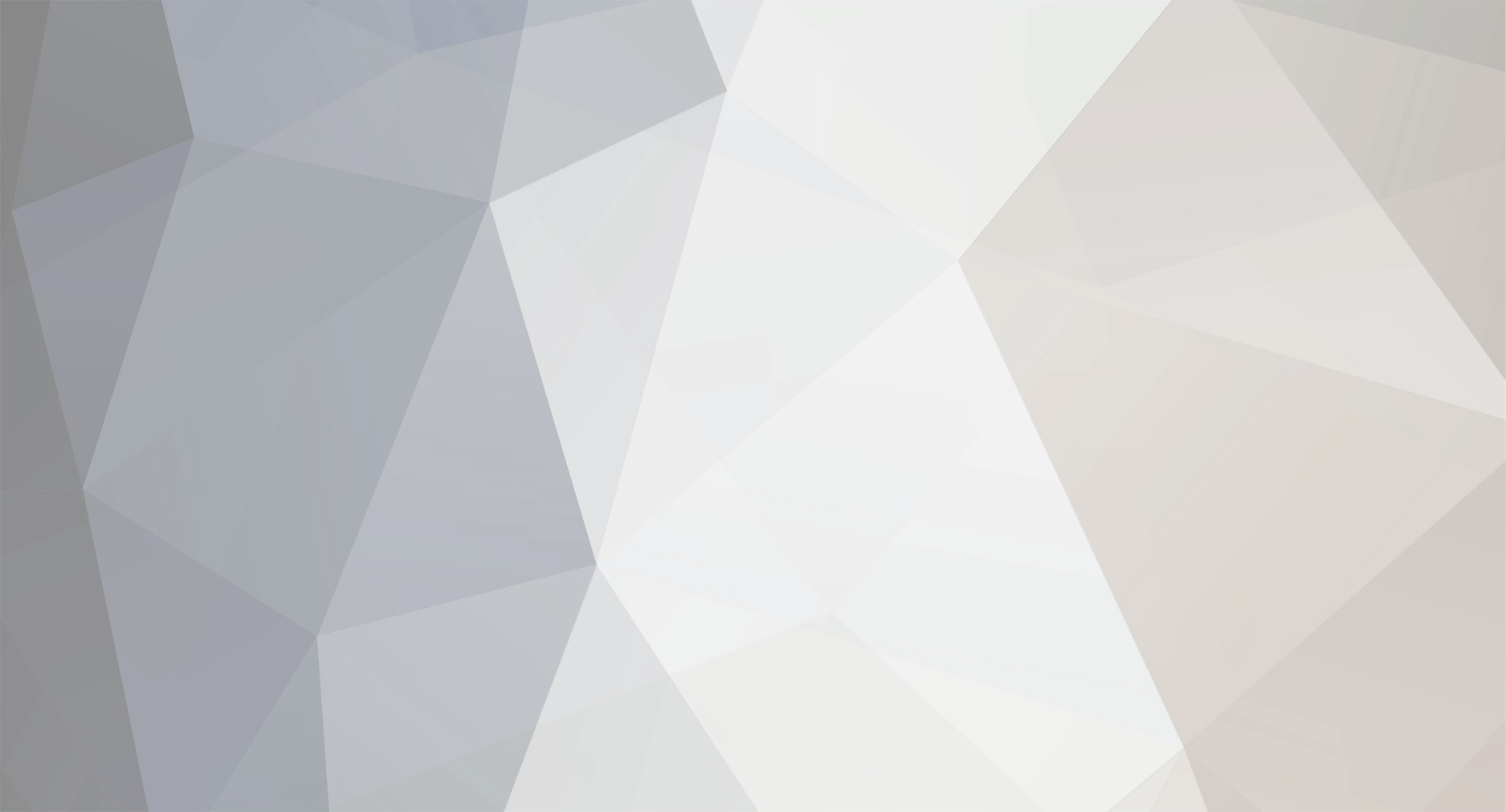
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
I've found the vanilla json to be most useful: YourMod/eclipse/assets/sounds.json Pretty much everything you need is right there, and you then you just play the sound with player.playSound, world.playSoundAtEntity, etc. The parameters for the sound-playing methods are all pretty well documented.
-
Ranged Attribute Error - What is causing this?
coolAlias replied to HappleAcks's topic in Modder Support
If you posted the full log, we could see where the error was happening - it is probably after the world has already finished generating, but the player is trying to join. Did you change or add any attributes for the player, such as in IExtendedEntityProperties? -
Try "gradlew --refresh-dependencies eclipse" or "gradlew --refresh-dependencies setupDecompWorkspace" (or setupDevWorkspace if you don't want the source readable...).
-
Ranged Attribute Error - What is causing this?
coolAlias replied to HappleAcks's topic in Modder Support
java.lang.IllegalArgumentException: Default value cannot be lower than minimum value! That pretty much says it all right there; whatever ranged attribute you added / changed, you have either an invalid default value or you set the minimum value incorrectly. Ranged attributes are things like attackDamage, movementSpeed, maxHealth, etc. -
[1.7.2][Solved]Checking what a thrown entity has collided with
coolAlias replied to Taji34's topic in Modder Support
MovingObjectPosition is not a Block, so of course it's not an instance of Blocks.leaves.getClass(). MovingObjectPosition has a MovingObjectType field 'typeOfHit' that tells you if it struck an ENTITY, BLOCK, or was a MISS. If the type is BLOCK, then you can use your moving object position's blockX, blockY, and blockZ fields to get the block that was struck from the world. Thrown items are Entities, after all, so they have a worldObj field you can use: protected void onImpact(MovingObjectPosition mop) { Block block = this.worldObj.getBlock(mop.blockX, mop.blockY, mop.blockZ); // now you have the block, you can check if it is a leaf block with instanceof if (block instanceof BlockLeaves) { // it's a leaf block } } -
Tile Entity NBT not syncing properly?
coolAlias replied to Alix_The_Alicorn's topic in Modder Support
If you are going to send the entire NBT data, the easiest way is to use the vanilla TileEntity methods: @Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new S35PacketUpdateTileEntity(xCoord, yCoord, zCoord, 1, tag); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity packet) { readFromNBT(packet.func_148857_g()); } That will make sure your tile entity is updated on the client when the block is first placed or the world loads, as well as whenever the block is forced to update. Then, whenever you need to update your tile entity manually, you can simply mark the block for an update and it will do the work for you: worldObj.markBlockForUpdate(xCoord, yCoord, zCoord); -
You can have a tool material just fine, see my sword class for a working example.
-
[1.7.2] Add slots to player's survival inventory
coolAlias replied to ben7's topic in Modder Support
See my reply to this post, which contains a link to a tutorial about adding extra inventory slots. -
A workaround for additional survival inventory slots is to use IExtendedEntityProperties to add them, with an accompanying GUI / Container, etc., and bind a key to open your custom inventory; players can re-map the key to 'E' if they want, effectively making your inventory the new 'vanilla inventory'. This circumvents both the issue of inter-mod compatibility and having to manipulate byte-code; it's easy and clean, imo. If you want to see an example / tutorial on it, check here.
-
It would be helpful if you linked directly to the Container and IInventory in question... your repo has quite a lot of folders and different stuff in it, making it difficult to determine which one you are having troubles with.
-
That's the correct method, but you say it's not working? I've used practically that exact same code just fine plenty of times; did you put the method in your Item class? There is a version of the method added by Forge that takes an ItemStack parameter - this is what you'll need if NBT is to affect damage, but first you need to get the method to work.
-
[1.7.x] How to add custom Drops to vanilla mobs
coolAlias replied to SuperHB's topic in Modder Support
You register your event handling class, not an actual Event class: public class MyEventHandler { @SubscribeEvent public void onDrop(LivingDropsEvent event) { // code } } // registration: MinecraftForge.EVENT_BUS.register(new MyEventHandler()); Please see here for more information about events and how to use them. -
[1.7.x] How to add custom Drops to vanilla mobs
coolAlias replied to SuperHB's topic in Modder Support
Change it to 'event.entity.worldObj' then - I was responding without Eclipse open, and some classes in 1.7.2 no longer provide public access to their World object (Tile Entities, I believe, for one). I added one. Doesn't do much ._. While one could argue that, it is absolutely necessary for your Event to be called, just as proper syntax "doesn't do much", but your code certainly won't work without it. Java can do pretty much anything, yes. An easy way to filter mob types is "event.entity instanceof IMob", which returns true if the entity is any type of aggressive mob (for vanilla at least, and 99.9% of modded entities will follow this rule as well). -
[1.7.x] How to add custom Drops to vanilla mobs
coolAlias replied to SuperHB's topic in Modder Support
It's much better to only subscribe to the event once and map the entity class to Item drops, or even just chain else-ifs within the one event if you are not comfortable with Maps, Lists, etc. Example of chaining else-ifs: -
[1.7.2]Tick Handler is not being Initialized?
coolAlias replied to Mecblader's topic in Modder Support
TickEvents are not registered to the MinecraftForge.EVENT_BUS, but to the FML bus: FMLCommonHandler.instance().bus().register(new YourTickHandler()); -
You have a null pointer exception occurring in GuiListExtended for whatever reason: Caused by: java.lang.NullPointerException at net.minecraft.client.gui.GuiListExtended.func_148181_b(GuiListExtended.java:70) ~[GuiListExtended.class:?] at net.minecraft.client.gui.GuiControls.mouseMovedOrUp(GuiControls.java:111) ~[GuiControls.class:?] at net.minecraft.client.gui.GuiScreen.handleMouseInput(GuiScreen.java:349) ~[GuiScreen.class:?] at net.minecraft.client.gui.GuiSlot.drawScreen(GuiSlot.java:314) ~[GuiSlot.class:?] at net.minecraft.client.gui.GuiControls.drawScreen(GuiControls.java:148) ~[GuiControls.class:?] at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1143) ~[EntityRenderer.class:?] I don't have MC code open at the moment, so I need ask, is GuiListExtended your class, or a vanilla class? If it's your class, we'll need to see the code for it; if not, then whatever you are doing to access func_148181_b is causing a null pointer.
-
[1.7.2] Dynamically Adding Slots to a Inventory/Container/Gui
coolAlias replied to chbachman's topic in Modder Support
A possible solution would be to not hard-code the number of slots in your TileEntity, but assign and re-assign it as needed when you add or remove slots, but this seems pretty unwieldy to me. Another option would be to handle the dynamic slots separately, as in each one could be its own IInventory / InventoryBasic, which you could then add or remove as needed. Btw, TinkersConstruct is open source, so you could check out how they do it. -
2 different swords. One inherits a method.
coolAlias replied to cheezytacos's topic in Modder Support
Could you show us the code where you declare and instantiate the items? I suspect you may have accidentally mixed them up, as in "waterSword = new ItemFireDiremondSword". -
Sure (you could send all the data in one packet without a HashMap, too), but the disadvantage is you then have to send all of the data every time any value needs updating, so you are wasting a lot of bandwidth and processing time handling your packets this way, depending of course on how many different values you plan on storing in your maps. You are trading performance for convenience, which of course is your decision to make, but it's something to consider, at least.
-
beacos if player just reconects - what will be? It will load that default values..Or actually it will be worse - it will load from NBT value 0.Beacos getInteger returns 0 , if its cant find that tag. Or why dont do both of that? 1.If there is no that tag , what we a searching in NBT , dont modify HashMap. 2. If there is that value in NBT , load it from NBT. So if you do default values , it will load them , beacos player dont have that tag. If you are saving and loading NBT properly, the value you load will be correct; loading from NBT is not the place to set a default value. When the player first starts a world, the constructor is called without reading from NBT afterward, so the default value you set there will then be saved and loaded just fine.
-
You will still need to add the attributes to the player somehow, possibly with IExtendedEntityProperties or during EntityConstructing event. As for checking the attributes, just look at any of the vanilla Entity classes and you will see plenty of examples of adding and checking attributes.
-
Why would you check during loading from NBT? If you want a default value, set it in the constructor - that's what constructors are for. If you want the maximum value to change, you can do so just fine without a HashMap, and if you only have a few pre-defined values (such as 'mana', 'xp', etc.), you aren't gaining anything by using a HashMap versus several local fields or even an array or List.
-
I'm not sure I really understand what you mean, but you can get an appropriate ItemBlock using Item.getItemFromBlock(block), and then get the id from that using your previous method. ItemBlocks, however, already contain information about the Block that they store, and any Blocks with subtypes use ItemBlockWithMetadata, which also stores the metadata information; you can add tooltip information yourself from a Forge event, or in your own custom ItemBlock classes directly... Are you sure you really need this BlockCompound class?