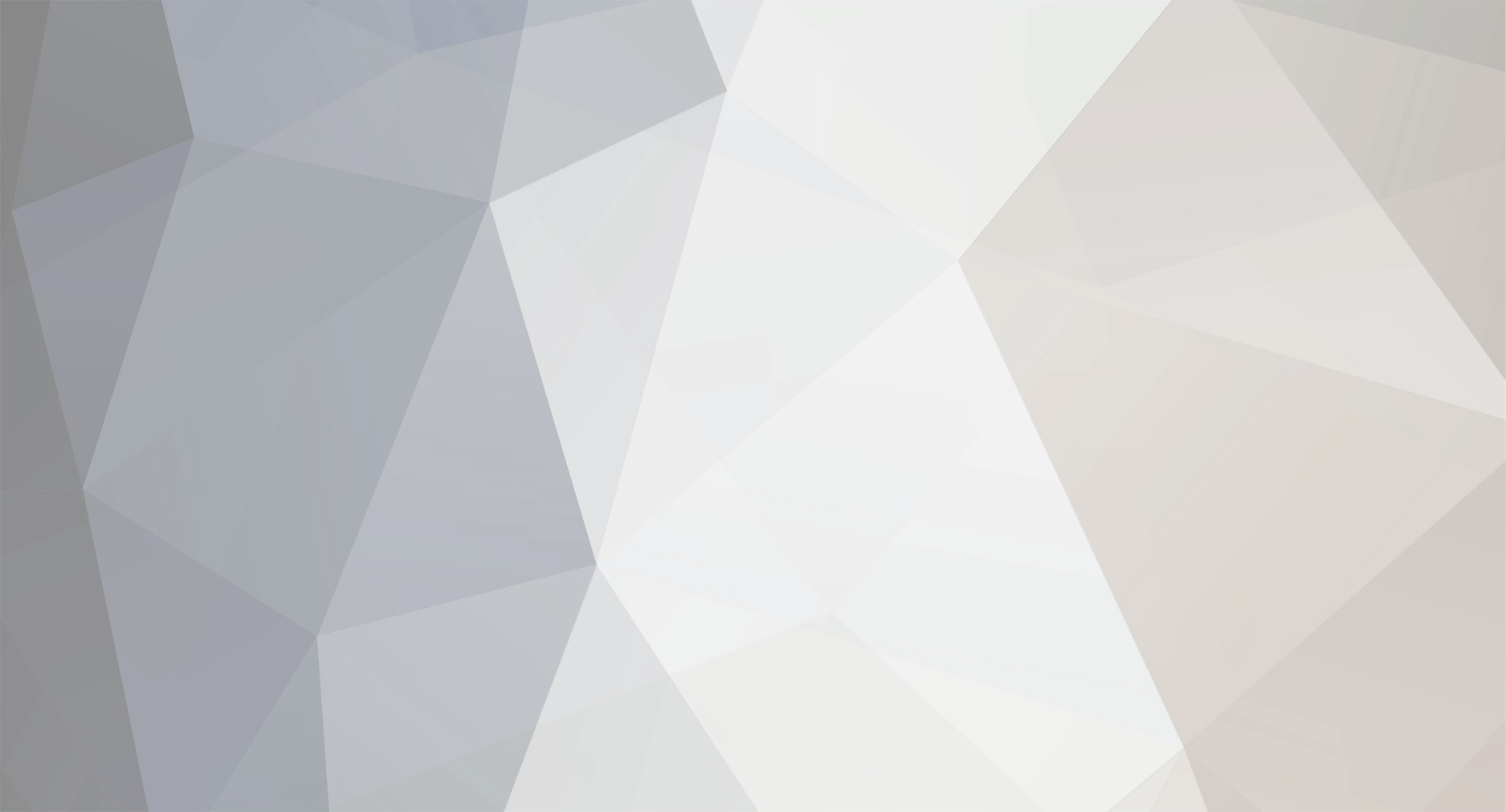
killerjdog51
Members-
Posts
56 -
Joined
-
Last visited
Everything posted by killerjdog51
-
[1.14.4/1.15.2] [Solved] Custom Biome/Structure/Feature Help
killerjdog51 replied to killerjdog51's topic in Modder Support
Is it possible to create another feature from a feature? Because I'd like palm trees and flowers to spawn around the lake/oasis. -
So I've successfully created my biome and have it spawn. The issue is that the biome is too large. But whatever value I set the scale variable to the biome never grows smaller (I even tried setting a negative number and the results were horrifying). The other issue is my biome spawns on the outskirts of deserts rather than the insides. I'm thinking of making my idea into a feature that spawns randomly in deserts instead, but is there any way to make a feature linked to a specific biome? I'd like to hear some feedback/sugesstions on this. goal: - Small mini feature/biome that spawns within deserts - 20 to 60 blocks in diameter - small to medium lake (depending on diameter) in center with grass blocks and vegetation surrounding it (palm trees, melons, flowers, etc) - vegetation to be a vibrant green similar to what is found in jungles code: public class OasisBiome extends Biome { public OasisBiome() { super((new Biome.Builder()).surfaceBuilder(SurfaceBuilder.DEFAULT, SurfaceBuilder.GRASS_DIRT_GRAVEL_CONFIG).precipitation(Biome.RainType.NONE).category(Biome.Category.DESERT).depth(-0.1F).scale(0.00F).temperature(0.95F).downfall(0.9F).waterColor(4159204).waterFogColor(329011).parent("desert")); this.addStructure(Feature.MINESHAFT, new MineshaftConfig(0.004D, MineshaftStructure.Type.NORMAL)); this.addStructure(Feature.STRONGHOLD, IFeatureConfig.NO_FEATURE_CONFIG); this.addStructure(Feature.VILLAGE, new VillageConfig("village/desert/town_centers", 6)); this.addFeature(GenerationStage.Decoration.LOCAL_MODIFICATIONS, Biome.createDecoratedFeature(Feature.LAKE, new LakesConfig(Blocks.WATER.getDefaultState()), Placement.WATER_LAKE, new LakeChanceConfig(4))); this.addFeature(Decoration.VEGETAL_DECORATION, Biome.createDecoratedFeature(WorldFeatures.PALM_TREE, IFeatureConfig.NO_FEATURE_CONFIG, Placement.COUNT_EXTRA_HEIGHTMAP, new AtSurfaceWithExtraConfig(1, 0.1F, 1))); DefaultBiomeFeatures.addCarvers(this); DefaultBiomeFeatures.addMonsterRooms(this); DefaultBiomeFeatures.addStoneVariants(this); DefaultBiomeFeatures.addOres(this); DefaultBiomeFeatures.addSedimentDisks(this); DefaultBiomeFeatures.addDefaultFlowers(this); DefaultBiomeFeatures.func_222348_W(this); DefaultBiomeFeatures.addExtraReedsPumpkinsCactus(this); DefaultBiomeFeatures.addSprings(this); DefaultBiomeFeatures.addDesertFeatures(this); this.addSpawn(EntityClassification.CREATURE, new Biome.SpawnListEntry(EntityType.RABBIT, 4, 2, 3)); this.addSpawn(EntityClassification.AMBIENT, new Biome.SpawnListEntry(EntityType.BAT, 10, 8, 8)); this.addSpawn(EntityClassification.MONSTER, new Biome.SpawnListEntry(EntityType.SPIDER, 100, 4, 4)); this.addSpawn(EntityClassification.MONSTER, new Biome.SpawnListEntry(EntityType.SKELETON, 100, 4, 4)); this.addSpawn(EntityClassification.MONSTER, new Biome.SpawnListEntry(EntityType.ZOMBIE, 19, 4, 4)); this.addSpawn(EntityClassification.MONSTER, new Biome.SpawnListEntry(EntityType.ZOMBIE_VILLAGER, 1, 1, 1)); } } public class WorldGeneration { public static Biome OASIS = new OasisBiome(); public static void registerBiome(Biome biome, int weight, BiomeType type, Type... types) { BiomeDictionary.addTypes(biome, types); BiomeManager.addBiome(type, new BiomeEntry(biome, weight)); BiomeManager.addSpawnBiome(biome); BiomeProvider.BIOMES_TO_SPAWN_IN.add(biome); } } public static final DeferredRegister<Biome> BIOMES = new DeferredRegister<>(ForgeRegistries.BIOMES, Main.MOD_ID); public static void setup() { BIOMES.register(FMLJavaModLoadingContext.get().getModEventBus()); WorldGeneration.registerBiome(WorldGeneration.OASIS, 5, BiomeType.DESERT, Type.DRY); } public static final RegistryObject<Biome> OASIS_BIOME = BIOMES.register("oasis", () -> WorldGeneration.OASIS); }
-
I need to prevent the Minecraft Swamp trees feature from generating in the world. In the past I used a Decorate event to cancel the event/feature from generating. But when I went to look for this in the Forge terrain_gen event folder it didn't exist (probably because Minecraft switched to using features). So I'm curious if there is a way to prevent Minecraft Swamp trees from generating in 1.14/1.15 (since I'm replacing them with Mangroves).
-
[1.14.4/1.15.2] [Solved] Custom Leaf Help
killerjdog51 replied to killerjdog51's topic in Modder Support
Thank you, this worked as well! Now none of the leaves despawn from the tree. -
[1.14.4/1.15.2] [Solved] Custom Leaf Help
killerjdog51 replied to killerjdog51's topic in Modder Support
Thank you, that solved it! I had no idea that the color handler events were moved to the mod event bus since in 1.12 and 1.13 they were in the Forge event bus. Now to tackle Vemerion's suggestion -
[1.14.4/1.15.2] [Solved] Custom Leaf Help
killerjdog51 replied to killerjdog51's topic in Modder Support
I set up a breakpoint at the beginning of each event, since neither of the breakpoints were triggered I'm guessing the events just aren't called. -
[1.14.4/1.15.2] [Solved] Custom Leaf Help
killerjdog51 replied to killerjdog51's topic in Modder Support
public class BlockColorHandler { @OnlyIn(Dist.CLIENT) @SubscribeEvent public void registerBlockColourHandlers(final ColorHandlerEvent.Block event) { // Use the colour of the biome or the default colour final IBlockColor grassColourHandler = (state, blockAccess, pos, tintIndex) -> { if (blockAccess != null && pos != null) { return BiomeColors.getFoliageColor(blockAccess, pos); } return GrassColors.get(0.5D, 1.0D); }; event.getBlockColors().register(grassColourHandler, ModBlocks.BAOBAB_LEAVES, ModBlocks.MANGROVE_LEAVES, ModBlocks.PALM_LEAVES); } @OnlyIn(Dist.CLIENT) @SubscribeEvent public void registerItemColourHandlers(final ColorHandlerEvent.Item event) { // Use the Block's colour handler for an ItemBlock final IItemColor itemBlockColourHandler = (stack, tintIndex) -> { BlockState iblockstate = ((BlockItem) stack.getItem()).getBlock().getDefaultState(); return event.getBlockColors().getColor(iblockstate, null, null, tintIndex); }; event.getItemColors().register(itemBlockColourHandler, ModBlocks.BAOBAB_LEAVES, ModBlocks.MANGROVE_LEAVES, ModBlocks.PALM_LEAVES); } } MinecraftForge.EVENT_BUS.register(new BlockColorHandler()); So here are my events, but they never get called. Initially i used: Minecraft.GetInstance().getBlockColors().register(); like in the Coloring Textures section in the Forge Documentation, but that results in a nullPointerException error -
Hello, I'm trying to make a custom leaf block in my mod (for both 1.14 and 1.15 [and probably 1.16 now that that's out]) and have run into two problems. The first issue is the farthest leaves despawn, so to fix that I tried creating my own leaf class and extending the LeavesBlock class as shown, but upon loading the game I get this error: Cannot set property IntegerProperty{name=distance, clazz=class java.lang.Integer, values=[1, 2, 3, 4, 5, 6, 7]} as it does not exist in Block{null} package killerjdog51.biomeEnhancementsMod.blocks; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.block.BlockState; import net.minecraft.block.LeavesBlock; import net.minecraft.item.BlockItemUseContext; import net.minecraft.state.IntegerProperty; import net.minecraft.state.StateContainer; import net.minecraft.state.properties.BlockStateProperties; import net.minecraft.tags.BlockTags; import net.minecraft.util.Direction; import net.minecraft.util.math.BlockPos; import net.minecraft.world.IWorld; import net.minecraft.world.World; public class PalmLeavesBlock extends LeavesBlock { public static final IntegerProperty DISTANCE = IntegerProperty.create("distance", 1, 11); public PalmLeavesBlock(Block.Properties properties) { super(properties); this.setDefaultState(this.stateContainer.getBaseState().with(DISTANCE, Integer.valueOf(11)).with(PERSISTENT, Boolean.valueOf(false))); } @Override public boolean ticksRandomly(BlockState state) { return state.get(DISTANCE) == 11 && !state.get(PERSISTENT); } @Override public void randomTick(BlockState state, World worldIn, BlockPos pos, Random random) { if (!state.get(PERSISTENT) && state.get(DISTANCE) == 11) { spawnDrops(state, worldIn, pos); worldIn.removeBlock(pos, false); } } @Override public void tick(BlockState state, World worldIn, BlockPos pos, Random random) { worldIn.setBlockState(pos, updateDistance(state, worldIn, pos), 3); } @Override public BlockState updatePostPlacement(BlockState stateIn, Direction facing, BlockState facingState, IWorld worldIn, BlockPos currentPos, BlockPos facingPos) { int i = getDistance(facingState) + 1; if (i != 1 || stateIn.get(DISTANCE) != i) { worldIn.getPendingBlockTicks().scheduleTick(currentPos, this, 1); } return stateIn; } private static BlockState updateDistance(BlockState p_208493_0_, IWorld p_208493_1_, BlockPos p_208493_2_) { int i = 11; try (BlockPos.PooledMutableBlockPos blockpos$pooledmutableblockpos = BlockPos.PooledMutableBlockPos.retain()) { for(Direction direction : Direction.values()) { blockpos$pooledmutableblockpos.setPos(p_208493_2_).move(direction); i = Math.min(i, getDistance(p_208493_1_.getBlockState(blockpos$pooledmutableblockpos)) + 1); if (i == 1) { break; } } } return p_208493_0_.with(DISTANCE, Integer.valueOf(i)); } private static int getDistance(BlockState neighbor) { if (BlockTags.LOGS.contains(neighbor.getBlock())) { return 0; } else { return neighbor.getBlock() instanceof PalmLeavesBlock ? neighbor.get(DISTANCE) : 11; } } @Override protected void fillStateContainer(StateContainer.Builder<Block, BlockState> builder) { builder.add(DISTANCE, PERSISTENT); } @Override public BlockState getStateForPlacement(BlockItemUseContext context) { return updateDistance(this.getDefaultState().with(PERSISTENT, Boolean.valueOf(true)), context.getWorld(), context.getPos()); } } So far the best solution I could think of was just to copy the entire LeavesBlock class and replace the necessary values since it appears that there's some sort of conflict between my distance property and LeavesBlock's distance property (I tried changing all the values to newdistance and that didn't work). I want to try avoiding this if possible and just find a solution that involves extending the LeavesBlock class and overriding the necessary functions to increase the distance. The second issue is that my leaves are all grayed out. I saw that in 1.12.2 people created a color manager class for this, I was wondering if that was still the case in 1.14/1.15? Thanks for the feedback, I'll really appreciate it.
-
[1.12.2] How to disable specific villager trades?
killerjdog51 replied to Jiro7's topic in Modder Support
Was a solution ever found for this? I'm also trying to remove a vanilla Villager trade. I'm looking into the concept of reflection, but it's kinda complicated. -
I tried to simplify my code by having all of my slabs run from a single class file, and they work, except that they all drop the same slab. Do you know why that is? half slab class package com.chocolatemod.blocks; import net.minecraft.block.Block; import net.minecraft.block.material.Material; public class BlockHalfSlab extends Blockslab { public BlockHalfSlab(Material materialIn, String string) { super(materialIn, string); this.setHarvestLevel("pickaxe", 2); this.setHardness(2.0F); this.setResistance(10.0F); this.setStepSound(soundTypePiston); } @Override public final boolean isDouble() { return false; } } slab class package com.chocolatemod.blocks; import com.chocolatemod.main.GameUtility; import com.chocolatemod.main.MainRegistry; import com.chocolatemod.init.ChocolateBlocks; import com.chocolatemod.main.CommonProxy; import net.minecraft.block.Block; import net.minecraft.block.BlockSlab; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyBool; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public abstract class Blockslab extends BlockSlab { private static final int HALF_META_BIT = 8; private static String ID; private static final PropertyBool VARIANT_PROPERTY = PropertyBool.create("variant"); public Blockslab(Material materialIn, String string) { super(materialIn); ID = string; // TODO Auto-generated constructor stub if (!this.isDouble()) { setCreativeTab(MainRegistry.tabChocolateBlocks); } this.useNeighborBrightness = !this.isDouble(); IBlockState blockState = this.blockState.getBaseState(); blockState = blockState.withProperty(VARIANT_PROPERTY, false); if (!this.isDouble()) { blockState = blockState.withProperty(HALF, EnumBlockHalf.BOTTOM); } setDefaultState(blockState); } public final String getId() { return this.innerGetId(this.isDouble()); } @Override public final String getUnlocalizedName(final int metadata) { return this.getUnlocalizedName(); } @Override public final Object getVariant(final ItemStack itemStack) { return false; } @Override public final IProperty getVariantProperty() { return VARIANT_PROPERTY; } @Override public final IBlockState getStateFromMeta(final int meta) { IBlockState blockState = this.getDefaultState(); blockState = blockState.withProperty(VARIANT_PROPERTY, false); if (!this.isDouble()) { EnumBlockHalf value = EnumBlockHalf.BOTTOM; if ((meta & HALF_META_BIT) != 0) { value = EnumBlockHalf.TOP; } blockState = blockState.withProperty(HALF, value); } return blockState; } @Override public final int getMetaFromState(final IBlockState state) { if (this.isDouble()) { return 0; } if ((EnumBlockHalf) state.getValue(HALF) == EnumBlockHalf.TOP) { return HALF_META_BIT; } else { return 0; } } @Override public final int damageDropped(final IBlockState state) { return 0; } @Override public final Item getItemDropped( final IBlockState blockState, final java.util.Random random, final int unused) { String blockId = this.innerGetId(false); return GameUtility.getItemFromBlock(blockId); } @SideOnly(Side.CLIENT) @Override public final net.minecraft.item.Item getItem( final net.minecraft.world.World world, final net.minecraft.util.BlockPos blockPos) { String blockId = this.innerGetId(false); return GameUtility.getItemFromBlock(blockId); } @Override protected final BlockState createBlockState() { if (this.isDouble()) { return new BlockState(this, new IProperty[] {VARIANT_PROPERTY}); } else { return new BlockState( this, new IProperty[] {VARIANT_PROPERTY, HALF}); } } public static void registerInventoryModel( final Item item, final String id, final int metadata) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register( item, metadata, new ModelResourceLocation( MainRegistry.MODID + id, "inventory")); } private String innerGetId(final boolean isDoubleStacked) { return ID; } }
-
Theres no errors in the console pertaining to the growthcraft api I have. If you want to check here it is: And here is my repository: https://github.com/killerjdog51/chocolate-mod-1.7.10
-
Alright, so I'm trying to use growthcraft 2.7.0 Api to integrate my chocolate mod with growthcraft in order to allow my mod to access growthcraft's cellar registry so that growthcraft's brew kettle could use my mod's fluid for brewing. However, when I used the gradlew.bat build command it stopped and spat out a bunch of errors. I would really appreciate the help. Error report MainRegistry Class
-
Thanks for the tutorial, its really helped me understand 1.8. Although my question is the index color, what do we do if we don't have or don't want an index color? because when I delete that portion it freaks out and creates a method. But on the other hand, it asking for me to give it a variable, but I don't have a variable to give it.
-
Im not the smartest, so im just trying to clarify something. but for this part of the code you input your .json file locations, correct? So you might replace "different" with "ModID:Cobblestone.json" and "variant" with "ModID:Cobblestone_mossy.json". I just want to make sure so im not missing anything.
-
Im sorry for being a noob. but i still dont understand 1.8 one bit (1.7 was simple compared to 1.8 for me) i mean, i have my metadata block made, but it wont render the texture. please help me. package com.stonemod.Main; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.client.Minecraft; import net.minecraft.client.resources.model.ModelBakery; import net.minecraft.client.resources.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.registry.GameRegistry; @Mod(modid = MainRegistry.MODID, version = MainRegistry.VERSION) public class MainRegistry { public static final String MODID = "stonemod"; public static final String VERSION = "1.0"; public static Block Andesite; public static Block Diorite; public static Block Granite; @EventHandler public void init(FMLInitializationEvent event) { Andesite = new BlockAndesite(Material.rock).setHardness(1.5F).setResistance(10.0F).setStepSound(Block.soundTypePiston).setUnlocalizedName("andesite"); GameRegistry.registerBlock(Andesite, "andesite"); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(Andesite, 0, new ModelResourceLocation("stonemod:chiseled_andesite", "inventory")); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(Andesite, 1, new ModelResourceLocation("stonemod:andesite_column", "inventory")); ModelBakery.addVariantName(Andesite, new String[]{"stonemod:chiseled_andesite", "stonemod:andesite_column"}); } }
-
Well if you look in the examplemod.class file i've provided you would know that i am indeed registering the fluid and block before the bucket or bucket registry. Because i create, define, and register everything in this order: fluid, fluid block, fluid container (bucket).
-
Ok, so i wanted to see if it was possible to make a fluid in 1.7.10 and i used this forge tutorial to create it http://www.minecraftforge.net/wiki/Create_a_Fluid. Now i know the tutorial is for 1.7.2 and im using for 1.7.10, but i dont think thats the problem because i dont thing a whole lot has changed between the two. Now, my fluid works well, and im able to pick it up in a bucket. But the problem lies with placing the fluid from the bucket, im still able to place it from its "block" form but not from its "item/bucket" form. Here is my code and my crash report, usually i can understand what the crash report is saying if it errored at the forge code but this is erroring at mojang's code so im not sure what to do and thats why im posting here. I would really appreciate the help, sincerelly killerjdog51. ExampleMod.class BlockYourFluid ItemYourBucket BucketHandler Crash Report
-
Yea... im having a hard time finding the source of the problem. the links you sent are a little difficult to understand.
-
Its nulling something here: IIcon icon = par1EntityLiving.getItemIcon(par2ItemStack, par3); if (icon == null) i think icon may be linked to ItemStack
-
The title hopefully explains it. Basically my bow works, but then when i implemented a bow renderer for it, it crashed the game when the player holds it in his hand. I was going to test if the renderer worked, apparently it didnt. I dont really know alot about java and sometimes i dont understand it, thats why im trying to learn it as i go along with my mod. I would really appreciate the help, sincerely killerjdog51. crash report Bow Render client proxy The error specifies these two lines from the Bow Renderer but i dont understand what they mean. this.renderItem(entity, item, 0); texturemanager.bindTexture(texturemanager.getResourceLocation(par2ItemStack.getItemSpriteNumber()));
-
alright, if you say so.
-
well, i was planning on updating my mod to 1.7.2 first, and then to 1.7.10 afterwards. why should i update it to 1.7.10 when im in the middle of updating it to 1.7.2?
-
Alright, so i got a new computer and just happened to install java 8 and when i tried to launch minecraft in eclipse it wouldnt work. eventually i learned that minecraft and java 8 dont like eachother. so i uninstalled java 8 and instead installed java 7(jdk and jre). So ive installed forge gradle using java 7 and open my mod in eclipse, so far no errors. I try to launch it in eclipse but it doesnt work, I ended up getting this error. Ive searched on the forums to see if there are any similar errors without much luck. so now im making my own thread. I would really appreciate the help, sincerely killerjdog51. [06:53:39] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLTweaker [06:53:39] [main/INFO] [LaunchWrapper]: Using primary tweak class name cpw.mods.fml.common.launcher.FMLTweaker [06:53:39] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLTweaker [06:53:39] [main/INFO] [FML]: Forge Mod Loader version 7.2.211.1121 for Minecraft 1.7.2 loading [06:53:39] [main/INFO] [FML]: Java is Java HotSpot 64-Bit Server VM, version 1.7.0_71, running on Windows 8.1:amd64:6.3, installed at C:\Program Files\Java\jre7 [06:53:39] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [06:53:39] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [06:53:39] [main/INFO] [LaunchWrapper]: Loading tweak class name cpw.mods.fml.common.launcher.FMLDeobfTweaker [06:53:39] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [06:53:39] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLInjectionAndSortingTweaker [06:53:39] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper [06:53:39] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [06:53:40] [main/ERROR] [FML]: The minecraft jar file:/C:/Users/Jordan/.gradle/caches/minecraft/net/minecraftforge/forge/1.7.2-10.12.2.1121/forgeSrc-1.7.2-10.12.2.1121.jar!/net/minecraft/client/ClientBrandRetriever.class appears to be corrupt! There has been CRITICAL TAMPERING WITH MINECRAFT, it is highly unlikely minecraft will work! STOP NOW, get a clean copy and try again! [06:53:40] [main/ERROR] [FML]: FML has been ordered to ignore the invalid or missing minecraft certificate. This is very likely to cause a problem! [06:53:40] [main/ERROR] [FML]: Technical information: ClientBrandRetriever was at jar:file:/C:/Users/Jordan/.gradle/caches/minecraft/net/minecraftforge/forge/1.7.2-10.12.2.1121/forgeSrc-1.7.2-10.12.2.1121.jar!/net/minecraft/client/ClientBrandRetriever.class, there were 0 certificates for it [06:53:40] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [06:53:40] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.relauncher.CoreModManager$FMLPluginWrapper [06:53:40] [main/INFO] [LaunchWrapper]: Calling tweak class cpw.mods.fml.common.launcher.FMLDeobfTweaker [06:53:40] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} [06:53:41] [main/ERROR] [LaunchWrapper]: Unable to launch java.lang.reflect.InvocationTargetException at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_71] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_71] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_71] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_71] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] Caused by: java.lang.UnsatisfiedLinkError: no lwjgl in java.library.path at java.lang.ClassLoader.loadLibrary(Unknown Source) ~[?:1.7.0_71] at java.lang.Runtime.loadLibrary0(Unknown Source) ~[?:1.7.0_71] at java.lang.System.loadLibrary(Unknown Source) ~[?:1.7.0_71] at org.lwjgl.Sys$1.run(Sys.java:73) ~[lwjgl-2.9.0.jar:?] at java.security.AccessController.doPrivileged(Native Method) ~[?:1.7.0_71] at org.lwjgl.Sys.doLoadLibrary(Sys.java:66) ~[lwjgl-2.9.0.jar:?] at org.lwjgl.Sys.loadLibrary(Sys.java:95) ~[lwjgl-2.9.0.jar:?] at org.lwjgl.Sys.<clinit>(Sys.java:112) ~[lwjgl-2.9.0.jar:?] at net.minecraft.client.Minecraft.getSystemTime(Minecraft.java:2674) ~[Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:40) ~[Main.class:?] ... 6 more
-
Not exactly. i want to add in a recipe for the resistance potions.