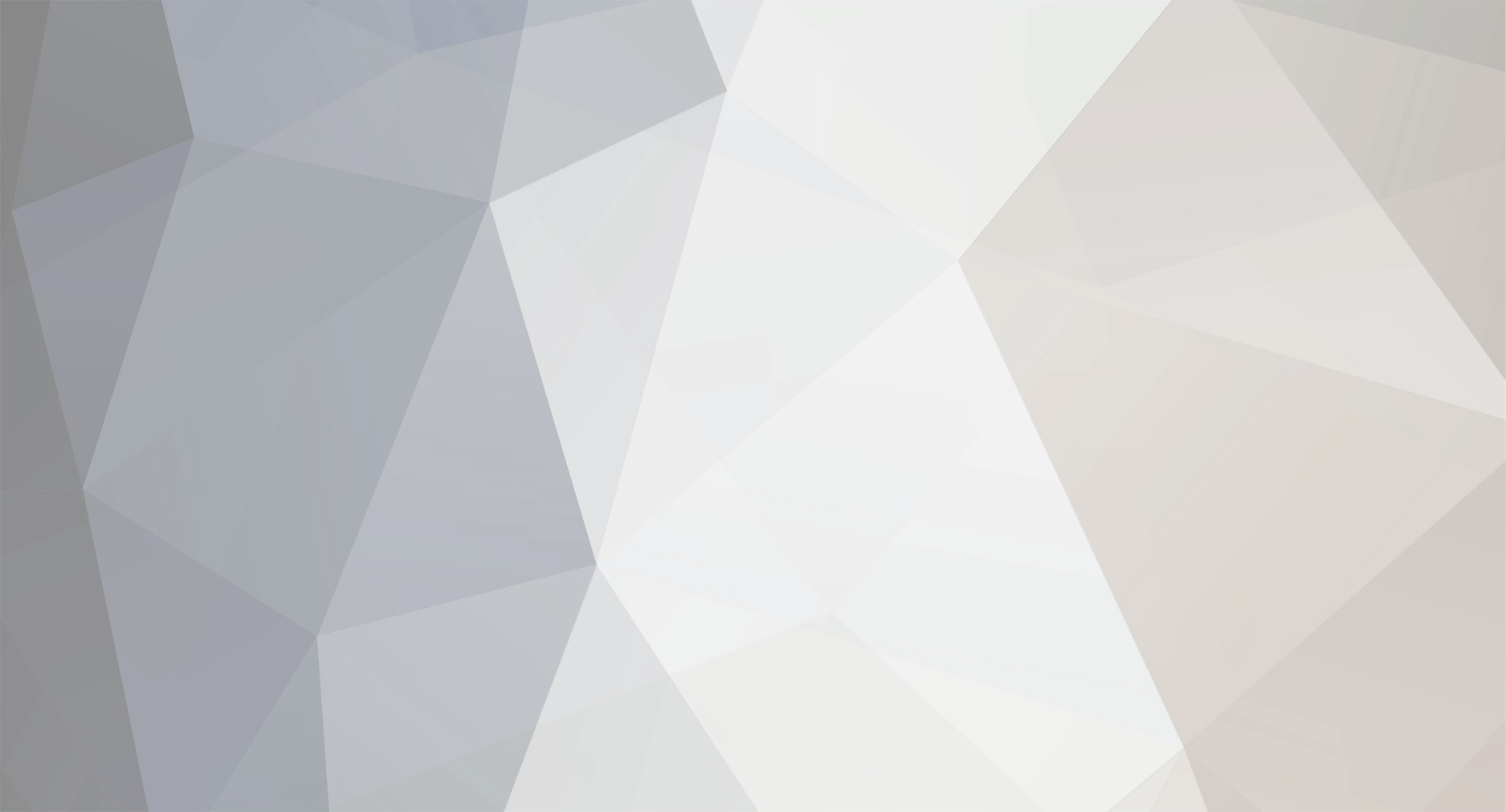
sequituri
-
Posts
669 -
Joined
-
Last visited
Posts posted by sequituri
-
-
Like diesieben07 said, your static initializer is called before the constructor even executes - In a procedure call <init>. That is way to early to register things. Use PreInitialization event handler for creating everything and registering them.
Then, use Init for adding recipes and everything (because other mods will have registered their blocks and items already.)
In PostInitialization, you can do things like check and alter other's recipes, and do late stage functions.
Hope that helps.
-
I know I can't be of much help, but I've been reading through and trying to understand the whole Loader to ModContainer to Mod system for a few days now. Suffice it to say, I cannot figure out how to select the specific ModContainer (factory method or otherwise) that will wrap your mod. At least you now have a mod container. That is farther than I got. I really wish the mcmod.info or something would let you specify a specific ModContainer class.
-
You will need to get the sounds from somewhere. Know how to create ogg-vorbis files? Sounds are assets like anything else. You create the asset, save it in your folder, activate it in the code.
-
Unlike NP++, Eclipse can check your code on the fly and even prompt you with method and class name and arguments. Eclipse practically holds your hand and leads you all the way. NP++? Nah, not so much. It's a dead scintilla wrapper.
-
Quoth "I know some java."
This is about like saying, I am a rocket scientist, because I have blown up a balloon and let it go. Java is not a language you learn through trial and error, unless you're a super-genius (Mozart even had to take classes in music).
-
Kinniken,
My point was, not everyone knows that your mod is found in a graphic link in your signature area. You might have included a normal text link in the body of your request to find the source / GitRepo (For those of us who haven't helped you out many times before, that is). A lot of people link their mod (however, not the source code). I'll know next time, but you may get this kind of response from other newbs.
Anyways, glad you got it figured out.
-
I can tell you one thing.
This:
this>>> at net.minecraft.network.packet.Packet24MobSpawn.func_73279_a(SourceFile:87)
Indicates that the class was modified. The normal call location is something like: Packet24MobSpawn.java
So, someone edited (or used ASM rewrite on) the base class and created a bug. I dunno who and I do not see a full log.
-
See amended reply. ^
-
Did you look at net.minecraft.item.ItemTool.class?
That may give you some hints.
By the way, if you call the Item class's setHarvestLevel("hoshovaxe",1) method, your hoshovaxe will be added to toolClasses automagically. Be sure that you return true for isDamagable() and set the stacklimit to 1. If your class subclasses ItemTool, the getToolClasses only returns your tool class. Called from Item, it returns all tool classes.
-
Interesting that you decided to register on the MinecraftForge eventbus, rather than the LoadController eventbus that was passed to your register function. Also, the registration code for event busses has to determine the modid to use for events (in order to properly set the thread context). Yet, it still works you say?
-
That second leg of your outer if will never send the message. Your local variable (f1) is always false in that leg. It only gets set true (sometimes) in the other leg. Get rid of the "} else {" and just let the code fall through to the "if (f1) ..." part.
-
Does the crash occur without MFR installed?
-
You would have to change the net.minecraft.world.chunk.storage.AnvilChunkLoader class methods that read and write to NBT.
-
If your item is a block, then you have to specify your ItemBlock when you register the block, otherwise the system will create an ItemBlock for you and render that with default rendering.
Register as an Item if you want to use a custom item renderer. Render as a block if you want the block rendering as an item. I create a new ItemBlock subclass for every block on which I want to get rendering control over.
Then call the GameRegistry.registerBlock(myblock, MyItemBlock.class, "my_ore", myMod.MODID, (new Object[]{}));
Then in my ItemBlock class I can handle rendering and everything as the Item.
-
public Electrocuted() //<-- this is your constructor for you main mod { //Registry GameRegistry.registerItem(BonePlate, "BonePlate"); GameRegistry.registerItem(BoneIngot, "BoneIngot"); GameRegistry.registerItem(CoalMotor, "CoalMotor"); GameRegistry.registerItem(ElectricMotor, "ElectricMotor"); GameRegistry.registerItem(Generator, "Generator"); GameRegistry.registerBlock(CompressionFurnaceIdle, "CompressionFurnaceIdle"); GameRegistry.registerBlock(CompressionFurnaceActive, "CompressionFurnaceActive"); GameRegistry.registerItem(BoneSword, "BoneSword"); GameRegistry.registerItem(BoneSpade, "BoneSpade"); GameRegistry.registerItem(BonePickaxe, "BonePickaxe"); GameRegistry.registerItem(BoneAxe, "BoneAxe"); GameRegistry.registerItem(BoneHoe, "BoneHoe"); GameRegistry.registerItem(BoneHelmet, "BoneHelmet"); GameRegistry.registerItem(BoneChestPlate, "BoneChestPlate"); GameRegistry.registerItem(BoneLeggings, "BoneLeggings"); GameRegistry.registerItem(BoneBoots, "BoneBoots"); LanguageRegistry.instance().addStringLocalization("container.CompressionFurnace", "Compression Furnace"); GuiHandler GuiHandler = new GuiHandler(); //Recipe //Items GameRegistry.addRecipe(new ItemStack(BonePlate, 2), new Object [] { "XX ", "XX ", 'X', Items.bone }); GameRegistry.addRecipe(new ItemStack(BoneIngot, 3), new Object [] { "XXX", "XYX", "XXX", 'X', Items.bone, 'Y', Items.iron_ingot }); //Tools GameRegistry.addRecipe(new ItemStack(BoneSword, 1), new Object [] { " X ", " X ", " Y ", 'X', BoneIngot, 'Y', Items.stick }); GameRegistry.addRecipe(new ItemStack(BoneSpade, 1), new Object [] { " X ", " Y ", " Y ", 'X', BoneIngot, 'Y', Items.stick }); GameRegistry.addRecipe(new ItemStack(BonePickaxe, 1), new Object [] { "XXX", " Y ", " Y ", 'X', BoneIngot, 'Y', Items.stick }); GameRegistry.addRecipe(new ItemStack(BoneAxe, 1), new Object [] { "XX ", "XY ", " Y ", 'X', BoneIngot, 'Y', Items.stick }); GameRegistry.addRecipe(new ItemStack(BoneHoe, 1), new Object [] { " XX", " Y ", " Y ", 'X', BoneIngot, 'Y', Items.stick }); //Armor GameRegistry.addRecipe(new ItemStack(BoneHelmet, 1), new Object [] { "XXX", "X X", 'X', BonePlate }); GameRegistry.addRecipe(new ItemStack(BoneChestPlate, 1), new Object [] { "X X", "XXX", "XXX", 'X', BonePlate }); GameRegistry.addRecipe(new ItemStack(BoneLeggings, 1), new Object [] { "XXX", "X X", "X X", 'X', BonePlate }); GameRegistry.addRecipe(new ItemStack(BoneBoots, 1), new Object [] { "X X", "X X", 'X', BonePlate }); }
See your problem?
-
Darn, I just took my swami mentalist cap to the cleaners. It won't be back for a few days, so my skill in clairvoyant code reading is null right now. It you wait a few days, I may be able to discern what you did to your code to make it not work. Sorry.
-
The argument as the generator is only used for sorting the generator. The problem with your code is not that. My mod uses 1000 as in
GameRegistry.registerWorldGenerator(new MetalOreGen(), 1000);
And my ores generator everywhere. I'd suggest actually checking what the generator is doing.
Look closely at your WorldGenMinable constructor call. The called constructor seems to take arguments that disagree with what you are sending.
Minecraft code:
public WorldGenMinable(Block block, int meta, int number, Block target) { this(block, number, target); this.mineableBlockMeta = meta; }
You are sending "WorldGenMinable(blocks.ore, 2048, 7, Blocks.stone)". I don't think 2048 is a good meta.
-
*Head-desk*
For the java retarded, here is the difference:
Simple Class called MyClass
Class MyClass { private boolean field; // <--- this is a field (of type boolean and private to the class) public MyClass() { // <--- this is a constructor!! Notice the name and the fact that there is no return type field = codeInTheConstructor(new StuffToMake()); // <--- code in constructor } int getANumber() { // <--- this is a method (a property getter) return 15; // <--- code in a method } }
This shows most of the simple layout of classes.
-
Should this be moved into support & Bug reports?
I suppose no one else has run into this problem. Am I on my own?
-
here is a pretty good tutorial for a custom furnace:
Thats the series i watched...
But, I still don't understand where i'm steeing the metadata again...
OnBlockPlacedBy() and setDefaultDirection() are both setting the metadata for the block. One function sets direction based on furnace surroundings (so it doesn't face a block). The other bases it on the facing of the player (like a door or stairs). They should agree?
-
This is all done in FMLModContainer$processFieldAnnotations(...).
-
I'm gonna make a guess here for you. It looks like Tinker's is looking at MFR API that is out of date (in relation to TiCon). Or, MFR itself is out of date with the API TiCon is using. MFR seems to be using the 1.6.2 version still, but they have had a few updates. Try getting the latest MFR or remove MFR and try again.
-
Personally, I think it is an oversight, rather than a "bug". When I'd like to see happen is the field should have another setting. FAIL, IGNORE, and ASK. The latter option would gui prompt the user to ignore or fail.
-
Don't do that. The ModContainer initializes the instance field automatically when the mod is instantialized. If you put some there with static initialized it will be overwritten.
Can't get FMLModIdMappingEvent
in Modder Support
Posted
Those events are not allowed to be sent, since they are not included in the list maintained by FMLModContainer.
This is the class that seems to fire most of the FML events.