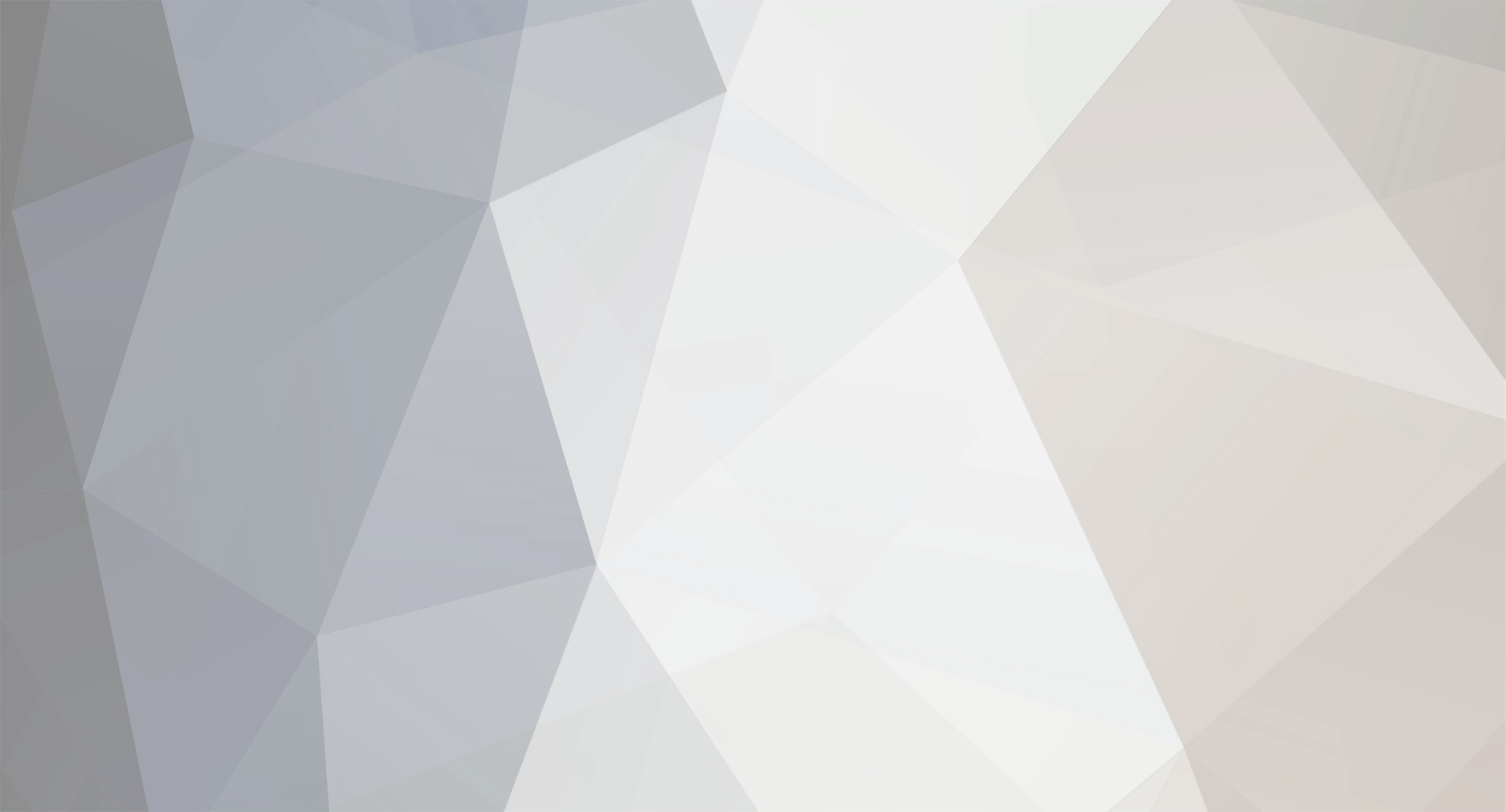
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
wierd sound glitch in smp (not present in ssp or idea)
jabelar replied to mrgreaper's topic in Modder Support
Where is the old field initialized? What is the console saying? Which of the sounds is involved in the problem? Also you can clean up your logic a bit -- you check if you're on server side before the block of checking for items, so you don't need to check again for being on server side when you check for each item. Why are you adding the player display name to the item name? Since you're getting the itemstack from the player passed with the event, the itemstack should already be unique to that one player. Why do you bother creating an onEntityPlay() method if you can just use the almost exactly the same playSoundAtEntity() method?' Why are you using atWorldPlace() for one sound and onEntityPlay() for another -- what's the difference? -
Yeah, it is interesting but if you don't register an entity it can do some funky stuff. Surprisingly you can still spawn entities without registering them, so sometimes it is hard to realize you made the mistake of not spawning them.
-
Why do you think it can only be called once?
-
[1.7.10] How to Force Obfuscation for my custom markDirty function..?
jabelar replied to Abastro's topic in Modder Support
Not sure but I suspect it would be something that would be specified in the build process, maybe in the build.gradle file. What does your build.gradle look like? Also by the way, what exactly are you doing with the ItemEntity concept? Sounds interesting, but I wonder if it is something that is solved in some other way. TileEntity makes sense because blocks are physically placed in the world so you have an idea of all "instances" (not actual Java instances but at least known locations for the blocks). Items aren't really placed in the world in the same way. They can be in containers, in your hand, in inventories, etc. And when there is an item on the ground, it is already an EntityItem. Anyway, just curious on what you're trying to do exactly, -
Well that tells you that my suspicion is correct -- something is killing the entity. I have no idea what though. What is your current code for the entity? did you have some special condition coded to kill it?
-
It should alternate because both client and server run the onUpdate() method in the class. But it is very weird that server stops (then client does). I still think it is dying, but maybe the isDead is being updated between updates or something. Let's try to override the setDead() method directly to see if we can catch it actually happening. In your class put the following: @Override public void setDead() { System.out.println("setDead() called"); this.isDead = true; } Capture the console output again and post it here.
-
That proxy code doesn't show the registration actually happening, just methods that could register the renderer. Where do you actually call the RegisterInformation() method?
-
I usually use IExtendedEntityProperties frequently. But when I was going through Entity class recently I noticed the getEntityData() method which is described as: /** * Returns a NBTTagCompound that can be used to store custom data for this entity. * It will be written, and read from disc, so it persists over world saves. * @return A NBTTagCompound */ That seems very similar idea. However, looking through the code that calls this method, it seems to have little use in the rest of the code -- only used in clone player or something. So probably sort of an orphan functionality. Anyway, is the getEntityData() useful or should I stick with IExtendedEntityProperties?
-
[1.7.2] [SOLVED]Problem with giving items on first join
jabelar replied to RedEnergy's topic in Modder Support
You should try to trace the code to see if it is behaving as expected. For example, put some console statements at certain points. Like you should print out the value retrieved for the entityData.getBoolean("firstJoin") to see if it is actually true. Now I think it won't work because you don't show any code that creates the firstJoin tag. Therefore, the first time it tests for it, there will not be any tag and in the case of getBoolean() that will return a false. So it is going to return false always as far as I can tell. I think you really want to flip the logic because first join will return false. Instead maybe try this way: @SubscribeEvent public void firstJoin(PlayerLoggedInEvent event) { EntityPlayer player = event.player; NBTTagCompound entityData = player.getEntityData(); if(!entityData.getBoolean("joinedBefore")) { entityData.setBoolean("joinedBefore", true); player.inventory.addItemStackToInventory(new ItemStack(Items.compass)); } } -
1) did you register the renderer in your ClientProxy? can you show that code? 2) do you see any console errors complaining about not finding the texture? 3) are your texture offsets correct in your model class? can you show the model class code?
-
[1.7.2][SOLVED] Generate structures in two Dimensions at the same time
jabelar replied to Jacky2611's topic in Modder Support
Well, it tells you exactly where the error is -- line 23 in your class. It seems that maybe the dimension ID you're passing is not good. Maybe you should use console print statement to print out the ID so you can check if it makes sense at that point in the code. -
At the end of the day, it is your own code. While it is more "professional" coding style to use indexes, loops, etc. to process similar things, it isn't that bad (if you're more comfortable with it) to separate them. Especially if there are just a few variations. For example, let's say you need to cycle through four things in an array. While it is natural for experienced coders to use a loop, it is actually about the same amount of lines of code to simply process each element with its own line of code. And often that is more readable, especially if you use enumeration or constants to represent the index. Anyway, it sounds to me that you'd be more comfortable separating variations into separate classes, and I think that is fine. It may in some cases mean more lines of code, but the code may be more readable and less prone to error. I think for directional blocks, metadata is still preferred because otherwise each direction will be different -- for example in the creative tab you'd have to have all four directions, and in inventory it would be different, etc. But for things like colors, or different behavior, it doesn't matter that much. By the way, if you're feeling limited with the metadata due to small number of bits to work with you can create your own sort of extended metadata if you want. In your main class you can just make a public byte array with index being position of the block for example and just make sure to update it when blocks are placed or destroyed.
-
Do the coordinates make sense? Like are they where you expect them? It is good to confirm the side that the instance is running on. Furthermore, if the printouts stop after a few times, then the entity must be "dying" because the onUpdate() should fire. You need to figure out why that is happening. But to confirm that it is dying, maybe also print out the public isDead field. So try this and post what the console says. System.out.println("X: " + this.posX + ",Y:" + this.posY + ",Z:" + this.posZ+", client side? ="+worldObj.isRemote)+", isDead ="+this.isDead);
-
Well, as long as the amount you need is in a public field or method somewhere, you can just add another drawString() method to your foreground layer method. Of course you can also draw things other than strings. For example if you wanted it as a percentage filled bar you could draw a rectangle and then draw a gradient rectangle on top where the gradient rectangle length show percentage of the steam amount versus max steam amount possible.
-
I didn't know animals could climb ladders. Anyway you might be able to block the pathfinding by messing with the bounding box. It is possible to make the bounding box change for each entity type. Not sure it would work with climbable block but it might. See my tutorial on this here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-modding-quick-tips.html?m=1
-
I have a tutorial on custom entities that might help. It uses animal entities as main examples, but a lot of the information should help for hostile mobs as well. http://jabelarminecraft.blogspot.com/p/creating-custom-entities.html Also, specifically for animation I have: http://jabelarminecraft.blogspot.com/p/complex-entity-models-including.html And for AI I have: http://jabelarminecraft.blogspot.com/p/complex-entity-models-including.html Anyway, maybe useful to you.
-
Well, the error is pretty clear: Unable to parse metadata from itemsmod:textures/blocks/corn_0.png java.lang.RuntimeException: broken aspect ratio and not an animation It gives that error for most of you graphics. It seems to think that the image should have animation metadata -- for animated blocks like fluids I think there is supposed to be some .mcmeta file that goes with the image. However, for the crop the growing animation isn't done with an animated image, but instead swaps in separate images. So it should not be trying to do animated texture... Can you post the code for your ItemBlockCrops class? You may want to post separate help thread asking about the specific error message -- others may know better how to fix that.
-
Well think of a centralized storage system where you can add items to and retrieve them again - not like a chest more like Applied Energistics. There the number of different items you have to match can easily go over a few hundred (especially with enchanted stuff and other items with an NBT tag) - if every retrieval process has to lookup the required item storage location with the trivial method it means for every available item in the storage a comparison required in the worst case. So you only do the lookup when you're taking from or adding to this inventory? I wouldn't worry much about perf as you'll be in a GUI and user can tolerate some delay.
-
What's your code look like now? Are you getting any errors on the console? What do you mean by no texture? Nothing at all? Or the pink and black default texture?
-
You also have to declare the proper size of the array. Change the line: iIcon = new IIcon[4]; to iIcon = new IIcon[8]; In both classes.
-
Okay, one problem is that a crop has 8 growth stages so needs 8 icons. Right now if your growth stage is more than 4 it won't have a texture. Not sure if that is your problem, but it would be a problem so you should fix it. So I think you should correct the registerBlockIcon() methods to have 8 icons registered. Try this in your corn class: @Override @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister parIIconRegister) { iIcon = new IIcon[4]; iIcon[0] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_0"); iIcon[1] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_0"); iIcon[2] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_1"); iIcon[3] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_1"); iIcon[4] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_2"); iIcon[5] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_2"); iIcon[6] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_3"); iIcon[7] = parIIconRegister.registerIcon(MainClass.MODID + ":corn_3"); } And your tomato like this: @Override @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister parIIconRegister) { iIcon = new IIcon[4]; // seems that crops like to have 8 growth icons, but okay to repeat actual texture if you want iIcon[0] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_0"); iIcon[1] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_0"); iIcon[2] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_1"); iIcon[3] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_1"); iIcon[4] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_2"); iIcon[5] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_2"); iIcon[6] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_3"); iIcon[7] = parIIconRegister.registerIcon(MainClass.MODID + ":tomato_3"); } Does that help at all?
-
Sure, but you still need to look at it in perspective of whether that still gets to point of being a problem. There are lots of similar sorts of algorithms going on in a video game, for example multiple entities in same area checking for collisions with each other, blocks checking for changes in neighbors, and so forth. Anyway, while it is good to make the basic code efficient where you have a choice, I still think short-circuiting is the bigger band for your buck if you can find such opportunities. I don't quite understand how an "item storage system" would create such a problem. Maybe you can be more specific about the problem you're trying to solve.
-
[1.7.2] Best way to approcah making a power system
jabelar replied to KeeganDeathman's topic in Modder Support
I don't think power systems are THAT difficult. The main idea is that you have a bunch of different custom blocks (generators, conduits/cables, and machines that use the power). Each block would have a couple pieces of data needed, possibly small enough to be kept in metadata but if not you can add some tile entities (or if that is too much of performance issue you can have one common class with some arrays that keeps track of info for all placed custom blocks). For example, in a generator you might have a boolean value to indicate if it is on or off, and an int value to indicate how much power it produces. In a cable you might have an int that indicates how far it is from the power source (if you want an effect like redstone that dies out over distance). In a machine you might just have a boolean for on and off, and int to indicate direction. Then, all you need to do in each block is monitor neighbors. The generator doesn't actually need to do much. A cable will check to see first to see if it is next to a generator and if it is it will set the distance variable to 1, otherwise it will check to see if it is next to any other powered cables (cables that have non-zero distance variable) and if it is it will set its distance to 1 more than the least of any neighboring cables, and if it isn't next to any it will set distance to 0 (not powered). The machines would check if it is next to any generator or next to any powered cable and set it's on / off state accordingly. That's pretty much all the power system does. You can of course get fancy with textures to show power levels and stuff. But mostly it is just a matter of each block "inspecting" surrounding blocks and picking up on anything that is powered around it. Hope that makes sense. -
While it is good to be worried about efficiency, do you already know you have a problem with perf or are you just worrying about it? A few things to think about: You should organize any series of tests by how likely they are to happen to stop the tests as early as possible. Basically test the least likely first. Imagine you have code that needs to check if you're walking on grass and holding a "grass staff" item. Walking on grass happens a lot, but presumably holding a grass staff would only be occasional. So it would be much faster to go if (holding grass staff) { if (walking on grass) { // do something cool here} } than the other way around because most of the time it would only do the first test then quit. The same thing happens with expressions using &&. Java will "short circuit" (meaning quit as fast as possible) when it finds the first false part of the expression. So holding grass staff && walking on grass is faster than the other way around. Instead of equals you may also want to consider instanceof. I'm not actually sure which is faster, but it is quite possible that instanceof is fast. Lastly, in most languages switch statements can be quite efficient. In Java though you can only switch on certain types of values, so that might limit your particular case. Ideally you'd just switch based on the item itself, but instead you'll need to resolve it to some sort of int or similar. But anyway, only optimize when you know you need to. Otherwise you should just write well organized and straight-forward code. Profile your code and then tackle the parts that are actually causing trouble.
-
[1.7.10][Solved] EntityItem (Dropped Item) destroyed by explosion.
jabelar replied to Scribblon's topic in Modder Support
Good work. Yeah, when modding it is interesting but you can run into dead ends, at least in the hopes of finding a logically straight forward approach. Regarding the private health variable, you can use Java reflection to check it. In general programming, Java reflection is often sign of some bad code architecture, but in modding it is quite acceptable since you don't have the ability to really affect changes in the scope of the actual code otherwise. Anyway, just mentioning this in case you have to do something "deep" like this again. I think it is also worth issuing an enhancement request to the Forge github to ask for events related to EntityItem hurt and death.