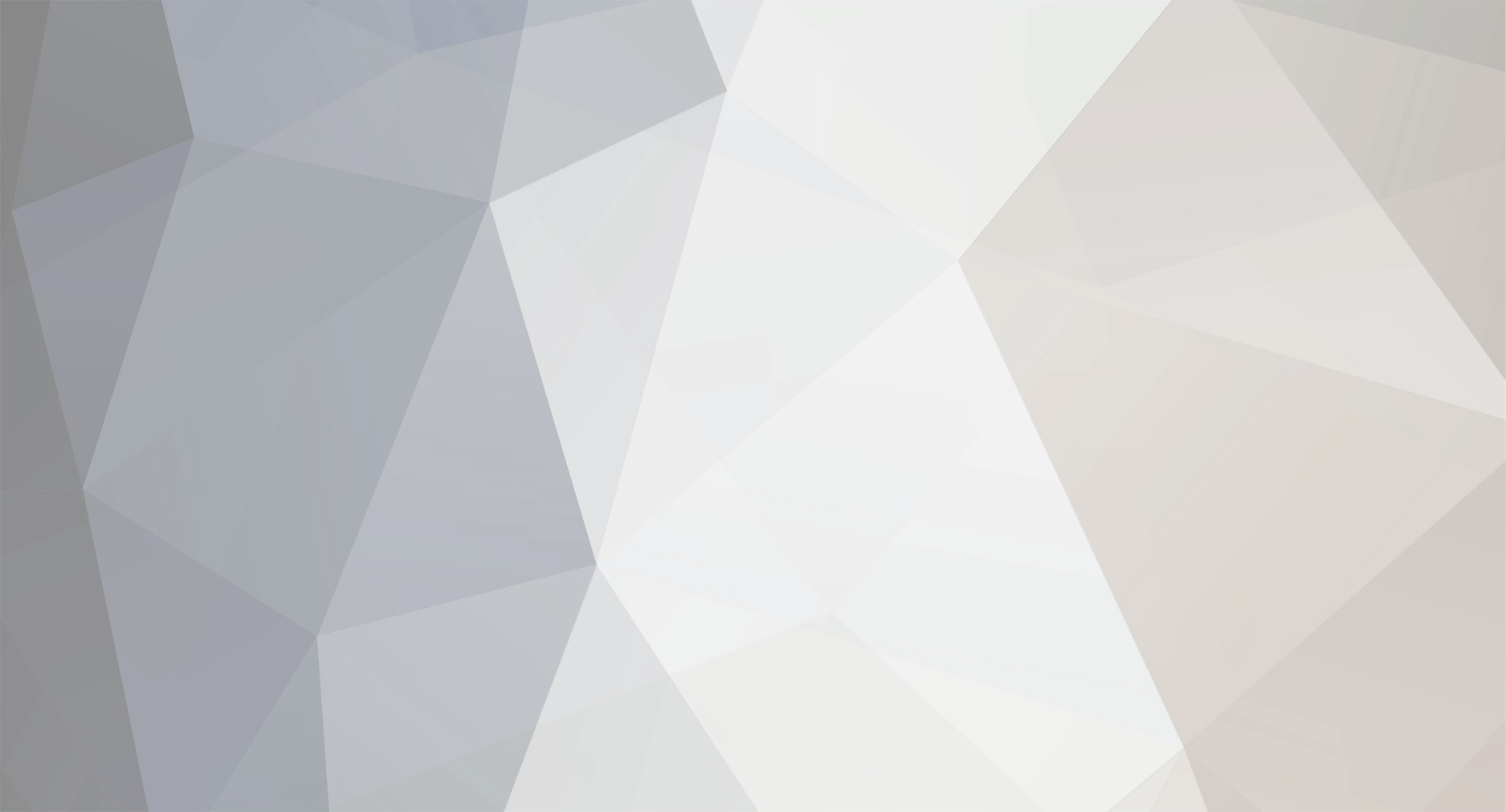
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
For the two methods mentioned by TGG you should copy the code from another tameable class like EntityWolf.
-
Okay, the best way to solve this is to associate the entity to the block when created using the entity's lang files. This would avoid creating a block lang file. So you could @Override the getLocalizedName() in your block that return the block name with field that captures the entity name when you create the block. Does that make sense as an approach for your needs? Basically read all the entities in the registry and create block instances for each for a block class that has field you set to localized name of the entity, and your custom block code overrides the method to return that name.
-
Okay, in none of that code is the model and renderer associated with your mob. How is Minecraft Forge supposed to know that that model goes with that renderer goes with that mob class? You need to register the renderer (not just register the mob). You need code in your ClientProxy that does something like this: RenderingRegistry.registerEntityRenderingHandler(woolyMob.class, new Renderwooly(new wooly(), 0.5F)); By the way, you're not naming your classes properly. First of all they should be properly capitalized: WoolyMob not woolyMob, Wooly instead of wooly, and RenderWooly instead of Renderwooly. Also you should probably name the model class better, like ModelWooly.
-
I don't understand from your description why you'd want to change the lang files. It sounds like you just want to read them, and are worried that there are multiple mods that may have additional entities? All entities, including from multiple mods are in the registry. So you can read back all the names from there (either unlocalized, which would include info on which mod it came from, or localized which would have the human-location-friendly names). Or maybe you can explain why you feel you need to change anything in lang files to do this.
-
in your items init() use console statement System.out.println() to print out a test of whether the block is null just before you register it to the seed item. If it is null, then it means you still have issue with order of initialization.
-
http://tutorials.jenkov.com/java-reflection/private-fields-and-methods.html It is actually quite easy.
-
In that code you don't show where you registerer the renderer. Somewhere you need to associate the model with the renderer (which binds the texture), with the mob class. Please show that code.
-
How would I - Right clicking block yields a message in the chatbox
jabelar replied to Forabor's topic in Modder Support
This is your own custom block, right? In that case I think you can @Override the onBlockActivated() method which should be called when your block is right-clicked. In that method, you should check that you're on the server side bychecking if the world parameter (the first parameter passed to the onBlockActivated() method is the world) is not remote (not remote means it is server side). Then you should send the chat message. You can use player.sendChatToPlayer(message) method. The player is one of the parameters passed into the onBlockActivated() as well. I think you get the idea.... -
Sorry I don't have a suggestion for your actual problem (I suspect CoolAlias will continue to help). but I wanted to point out something that is useful to remember -- instanceof handles the null case so you don't have to check for null && instanceof. So in your common proxy where you have: entity != null && entity instanceof TileEntityRepairBox You just need: entity instanceof TileEntityRepairBox In other words, null is not an instance of and it also will not fail with null pointer exception. Just a tip.
-
Okay, I think the next step would be to intercept what the client code regarding names. Name format is funny because it isn't really cancelable anyway but instead you just change the field value to what you want. Like for a funny example in my event tutorial I suggested putting the following in the NameEvent to change the way names are displayed: if (event.username == "myUserName") // put your user name in the string { event.displayname = event.username+" the Great and Powerful"; } else if (event.username == "myFriendsUserName") // put your friend's user name in the string { event.displayname = event.username+" the Wise"; } else if (event.username == "myGirlFriendsUserName") // put your significant other's user name in the string { event.displayname = event.username+" the Beautiful"; } else { event.displayname = event.username+" the Ugly"; // for everyone else } The comments say the following about the NameFormat event: If you follow the call hierarchy, all of this just updates a field in EntityPlayer called displayName. It is private, but you can change it with Java reflection I think. So what if you did a ClientTickHandler and used Java reflection to inspect and modify (if necessary) the displayName field? I think this would still be limited to Client, so if you want other users to see the effect they'd have to use the mod too probably. If that doesn't work, I think you'd have to try to intercept the chat and the player rendering where they display names.
-
By "multiplayer" do you mean this is a client-only mod? Like it isn't installed on the server? If that's what you mean, then I guess I'm not sure whether this particular event is fired. It should be though I think because the call hierarchy for the event shows that it is used in the EntityPlayer class. This should make it available to EntityPlayerMP because that extends EntityPlayer. EDIT] Maybe one way to figure this out is to print out what side it is firing on. You can print out event.entityPlayer.worldObj.isRemote() and then run this in the single player case and see what it says. If it always shows "false" then it is only firing on the server.
-
Okay, you're still a little confused. This is Minecraft-specific stuff so I understand it takes a little time to wrap your head around it. But you have several issues going on here. First of all, your FrameMod class is your "main" class. You shouldn't be creating any instances of it anywhere because there should only be one -- but when you registered it as the event handler you created two new instances. Instead, for the event handler class to register you should make a separate event handler class, put all your methods in that and register that instead. You should read my tutorial on events. It is a bit long, but covers a lot of what you're getting wrong here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html Anyway, so basically: 1) create a class called MyEventHandler 2) change the registrations in the CommonProxy init() method to register MyEventHandler (instead of FrameMod) 3) move the getName() method (including the @SubscribeEvent) from the FrameMod class into the MyEventHandler class you just created. 4) Actually I would consider moving the getName() method out of the ClientProxy. The NameFormat event doesn't have to be client only (or if it is the event would only fire on that side, so no problem). You only need to put code into the ClientProxy if it *calls* code that is SideOnly for client side. I think that might work. At least make those changes, read my tutorial, and let's see how far you get.
-
It is easy to think that because DamageSource#getEntity() method always returns null. What you need to consider though is that there are other classes that extend DamageSource, such as EntityDamageSource, that can be passed as the event.source (because these extended classes are valid DamageSource classes). EntityDamageSource returns the entity in the getEntity() method. It is an important thing to remember in Java (and similar languages) -- when a method parameter has a type, you need to consider that any of the subtypes of that type are also valid to pass in.
-
Yeah, boundingbox is good idea, but I was worried about your lack of -1 ... I know I've been immensely frustrated before trying to debug why code wasn't returning the block until I realized the -1 was missing... so I got in the habit of just copying the vanilla onEntityWalking(). But now that you mention it, for custom entities the yOffset is likely not very trustworthy while boundingbox is. Good idea. (Note this thread is becoming one of those where we all have fun showing off our expertise, but I'm not sure the person who started the thread is really making progress...)
-
The event parameter has a source field which is type DamageSource. DamageSource is a bit funny because of course there are all sorts of different things that can cause damage besides the player -- like lava, falling, explosions, hunger, and so on. But there is a getEntity() method as well (it will return null if it wasn't an entity). Now if you just inspect the code of DamageSource you might think it will always return null (because that is what the method does in that class), however there is an EntityDamageSource class that extends DamageSource that may be passed as the source (and of course EntityDamageSource will return an entity). I think that if you check if event.source.getEntity() instanceof EntityPlayer then you'll know it is player.
-
[Solved]getEntityData() versus IExtendedEntityProperties
jabelar replied to jabelar's topic in Modder Support
While I think it is good to be aware of performance and "waste", the reality is that modern computers and modern networks can push a heck of a lot of data. For something that occurs infrequently I don't think it is that bad to use NBT even if it is overkill. Sending it every tick for a lot of entities might start to be a problem, but if it is only sent on changes to the entities and if there are only a few, it is probably just fine. In coding it is common wisdom that as long as you're not stupidly wasting perf, if there is advantage in terms of clean coding logic, coding maintainability, or code reuse, it is okay to not be fully perf optimized. You should only perf optimize those things that are proven to cause perf problems. This is because the Pareto rule usually applies, most of the perf problems will be dominated by a couple inefficient parts of the code. It can be a waste of time as well as bad coding result to try to make new, possibly more contorted code, in your entire code base because you're overly obsessed with performance. Remember also that most performance bottlenecks are usually solved by the next year's computer technology. In fact computer performance is probably increasing faster than most of our ability to code more efficiently. Just don't do obviously stupidly wasteful stuff. So in this case, if NBT has advantage of being fully consistent with the save data and already easy to pack into packet payload, it is worth considering. -
For finding the block under an entity, you need to be bit careful with the y value as entities have a yOffset. if you follow the code in the build-in method onEntityWalking() I think you need code like this: public Block findBlockUnderEntity() { return worldObj.getBlock(MathHelper.floor_double(posX), MathHelper.floor_double(posY - 0.20000000298023224D - (double)yOffset), MathHelper.floor_double(posZ)); }
-
[SOLVED] [1.7.10] PlayerInteractEvent - Strange behaviour
jabelar replied to Nicnl's topic in Modder Support
Why not send a packet to client? Also, it does seem a bit weird that these events aren't consistent in their firing. I mean it kinda makes sense based on the type of click, but it wouldn't really have hurt to fire on both sides...like what if you wanted to add visual effect on left clicks or something? -
[Solved]getEntityData() versus IExtendedEntityProperties
jabelar replied to jabelar's topic in Modder Support
Got it. Thanks for the detailed answer. (It's funny because as I learn modding and crawl into new areas of code, I almost always find you've been there before me!) -
You should make separate posts if you have multiple questions. Anyway, for the @ForgeSubscribe problem, in 1.7.2 and later it changed to just @Subscribe. If you're working with events you might want to check out my tutorial: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html
-
Thanks for the reply (here and on the other thread). I just replied on that other thread. On this issue, I'll need to check in the morning. But I suppose I may have been sloppy on the imports. I usually have Eclipse set to automatically add/delete imports and if there are multiple options it usually gives me a choice. I don't remember it giving a choice. But I think what you're saying is I might have imported an IMessage from some other package. I'm not quite sure that would cause this problem because I think it would consider the parameter to be from that package as well (they would technically match), but I'll check. I suspect that I'm doing the standard mistake of copying code without properly editing it to suit. However, the examples (e.g. diesienben07s here http://www.minecraftforge.net/forum/index.php/topic,20135.0.html and CatDany here https://gist.github.com/CatDany/4a3df7fcb3c8270cf70b) seem to have complete example message. By the way, are you still set against the SimpleNetworkWrapper approach? I was reading through your tutorial again today and it was a valiant attempt at making it fully useable.
-
[Solved]getEntityData() versus IExtendedEntityProperties
jabelar replied to jabelar's topic in Modder Support
I missed your answer until you pointed it out in another thread. Thanks for the detailed reply. You said: but since you have no hook for the player save I guess that's true because I couldn't find any. The comment for the getEntityData() seems a bit misleading though because it says "It will be written, and read from disc, so it persists over world saves." Are you making a distinction between world saves and player saves? -
Find Block Anywhere in World (or a workaround)
jabelar replied to TricliniumAlegorium's topic in Modder Support
Try to print out the name of the block that you do get at that position. Also, if you have it in onEntityCollidedWithBlock why would you check the entity position? Wouldn't the entity position be same as the block that it just collided with? (Aside: do you really want all entities to be teleported? If not you might want to check that the entity is a player entity as well.) -
You should contact TheGreyGhost. He's helped some others with rendering problems. He has a utility as well that will dump all the G11 state so you can find anything that might not be set as expected.
-
This is different than a missing method. Eclipse is properly seeing that I'm implementing an interface, for which it knows the onMessage() method is needed, but it isn't liking the implementation -- it doesn't seem to like the generic parameters part of it. So even though I'm creating a method that seems to have the right parameter types, it doesn't consider it an override. If I wrote this myself I'd figure it was just me being dumb, but I copied this code from diesieben07s tutorial and copied a similar code from CatDany example, and both had exactly the same complaint.