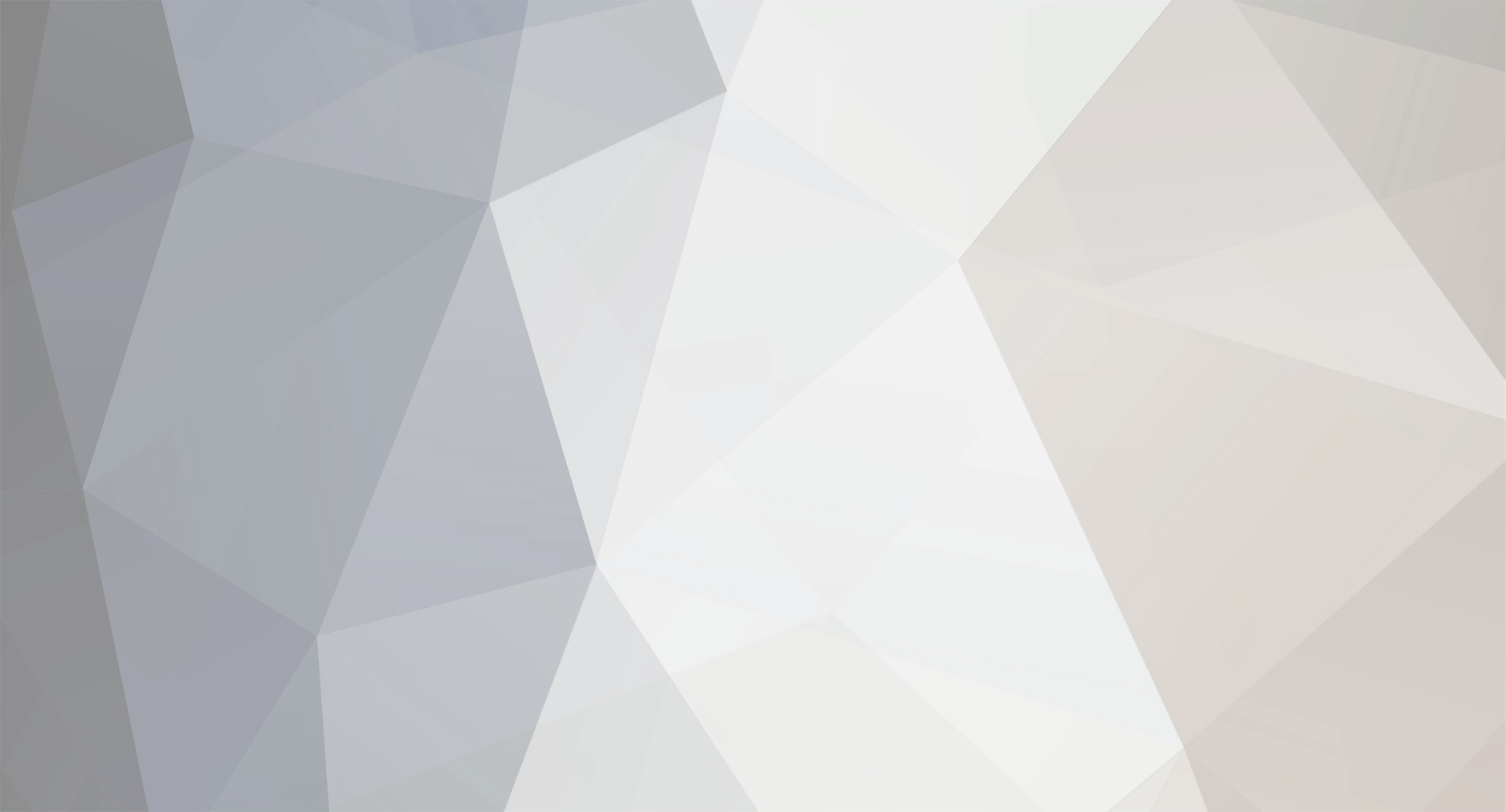
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
In the server version you would set cancelled. That should work. You mentioned handling the event in multiple places in your code? That is a bad idea because you don't know the order they will fire.
-
Okay, then it should be: ItemStack(Item.getItemFromBlock(event.getBlockState().getBlock()), 1, this.damageDropped(event.getBlockState())); I guess you're right the damageDropped can be different than the blocks meta.
-
No, wait it should work. This is the way you should do it. In your proxy class create a method called something like showMyGui(). In the ClientProxy version of that method you would actually make the GUI. In the server version you would do nothing. In your event handler you just call that proxy method and it will behave differently on each side. Server would not put up a GUI.
-
The easier fix is just to use the right constructor. In your code you have ItemStack(event.getBlockState().getBlock) But instead you should replace it with something like: ItemStack(event.getBlockState().getBlock(), 1, event.getBlockState().getBlock().getMetaFromBlockState(event.getBlockState()))
-
Do you really mean an "inventory" menu? Or do you just mean a server selection GUI? Those are different things. You said you wanted to select the server, and at the point you're selecting a server I don't think the player would even have an inventory. So it doesn't make sense to want an inventory menu to select a server. What exactly are you trying to do?
-
It all sounds pretty good .... except the fact that it means major rewrites for any mods you want to port forward. I'm really, really hoping that the interfaces settle down so that the maintenance of existing mods becomes less of a chore. Anyone that has more than a couple mods literally has to spend weeks, even months (if you include thorough testing and assume that hobbyists can only spend a couple hours per day working on it) to bring them up to each upgrade. For example, the flattening makes a lot of sense but I can imagine a person with a mod with dozens of sophisticated blocks will have significant work in front of them. My dream is that someday any big updates to Minecraft or Forge are simply content and not the API mechanisms. Then we would truly have a proper API. Thanks to everyone on the Forge team though doing the work to keep up with all this!
-
LivingJumpEvent issues: Firing twice, not finding capability/NBT
jabelar replied to Alekseyev's topic in Modder Support
The event is supposed to fire twice, client and server side. Any capability data may not be present on the client (this is normal) unless you are properly syncing that yourself using custom packets. Player movement is a bit strange because (in order to ensure smooth player movement despite network lag) the client has a lot of control of it with the server just verifying and occasionally affecting it. Since this affects player movement I suspect you do want to process the event on both sides. However, it sounds like your player data isn't synced and available on client. You need to debug that. -
He's not actually trying to send additional data though, he's trying to get the client-side entity to spawn in the first place. For the vanilla fish hook there is a dedicated packet type for this SServerSpawnObject that is distinct from other entities. Now ultimately I asume he can rely on a regular registered entity type of spawn mechanism, but just that if the approach is to copy the fishhook approach it might be a bit different..
-
Why don't you use the "target" instance? You've already established it is a righteous penguin type and presumably the penguin you right-click on is the one you want to interact with.
-
Looking at it briefly, the fishhook along with other "entity objects" like minecart are spawned on the client side based on a packet called SPacketSpawnObject. You can see the processing of that received packet in NetHandlerPlayClient#handleSpawnObject() method. Basically the vanilla objects are hard-coded to a number of values (fishhook is 90). So you won't be able to use the same packet, but you may have to try to replicate the same system. At the time you spawn the fishhook on the server side you probably need to send a custom packet that then spawns on client similarly. However, it may be possible to be simpler than that. In your onItemRightClick() you're specifically checking for server side before spawning. What happens if you just allow it to spawn on both sides? I think the problem though is for other players -- even if this works for singleplayer I'm not sure that other people would see it without packets. I also think that doing a registered entity approach could work, but not entirely sure what modifications would be needed. In any case, the most obvious way of copying the fishhook is to send a spawn packet from server. So check out how the SPacketSpawnObject is sent for fishhook and then send a similar custom packet for your claw..
-
This is to support the concept of server-side "resource packs" right? Thanks for the summary!
-
Actually, I can even make different colors for different enchantments (my son's idea). Here the bow has flame and is read while the others are all defaulting to green: https://imgur.com/a/pHmqVrZ
-
It's not that hard with a bit of reflection. I just got home from work and figured it out pretty quickly. Basically, you use reflection to put your own extended of RenderItem into the Minecraft class, with control over the color. There is a little bit of trickiness because the RenderItem instance actually gets passed to other renderers as well and so you have to reflect into all the copies. Here's example of green: https://imgur.com/a/Sg1wxBL and blue: https://imgur.com/a/kOubNyx I'll write up a tutorial on this over next few days.
-
By "random" I think he means that it is not specified as a dependency, so from the point of view of including it is is unrelated to your dependencies and thus "random".
-
ItemStack class has several methods specifically for checking different amounts of equivalence. You can just check for the same item, but can also check for same item and quantity, or even everything the same including NBT.
-
Yeah, the "problem" with the JSON asset system is that you have to get everything exactly right and if you don't there isn't always a lot of good warning on exactly what is wrong. For actual Java code, your IDE (like Eclipse) will warn you instantly of references to non-recognizable things, misspellings, wrongly formatted code. But for JSON you have to usually do a lot more manual checking -- is path exactly right, is filename exactly right, is the file a properly formed JSON, are the values in the JSON exactly right. Once you're used to the amount of detail required though you'll pay attention and also have some common ideas on where to look to fix problems that arise.
-
Oops I meant the unlocalized name. I tend to always make my registry name and unlocalized the same (of course not necessary).
-
It has to match the registry name for your item, and I think it needs to be all lower-case. So if you registered your item it should have registry name "bit" not "Bit" and you need to match that with "item.bit.name=Bit". Also the name of the lang file depends on the format version in pack.mcmeta file. If you have "pack_format": 3 then it will be en_us.lang but otherwise it will be the older en_US.lang.
-
1.12.2 How to make Vanilla Ai attack custom mobs
jabelar replied to solidarityK's topic in Modder Support
Yes, that should work. -
I agree except ... people need to understand what is going on underneath. It is great if there is already a method for flattening collections, but I don't think it really helps understand the underlying construction of the data. The question posted indicated he didn't really even know what a map is.
-
Although diesieben07s flatmap approach should work, it is good to understand what you're doing. This is really just basic Java. You have a map and it has keys of DimensionType and values of IntSortedSet. If you think about what that means, there is a set of ints (i.e. dimension ids) for each dimension type. So the logical way to turn this into an array of ints is to iterate through the map (i.e. loop through each DimensionType) and then take the value int set and iterate through that, putting each element into your array.
-
I don't think you need to feel guilty about the performance impact. With a modern computer, the GPU is capable of probably billions of such calculations. I assume that your item will be reasonably rare (a lot of the time probably not even rendering the item at all), and remember that only the items being rendered (i.e. in field of view) would even have an impact. So at most you're probably going to have a couple itemstacks in an inventory (and when viewing inventory frame rate isn't really as much of an issue even if performance dips), or maybe a couple items thrown on the ground. With modern programming (assuming you're using a full computer and not some resource-strapped embedded processor) it is best to use the proper logic and only deal with perf issues when they actually are an issue. I.e. profile the code and see if there is an actual problem. Of course you should not do stupid things like big loops, recursion, and such unless necessary. But otherwise you should just code for clean, well-structured code that uses the intended interfaces. Bug-free, maintainable code is the first priority.
-
Pretty much. In MyEntityFallingBlock you'll just want to get rid of part of the fall() method where it updates damage to the anvil. I think you can pretty much just delete this part: if (flag && (double)this.rand.nextFloat() < 0.05000000074505806D + (double)i * 0.05D) { int j = ((Integer)this.fallTile.getValue(BlockAnvil.DAMAGE)).intValue(); ++j; if (j > 2) { this.dontSetBlock = true; } else { this.fallTile = this.fallTile.withProperty(BlockAnvil.DAMAGE, Integer.valueOf(j)); } }
-
Why not just call the same methods for animation and sound, or copy the code in your code?
-
Yeah that is the general idea, although if you use the recommended @ObjectHolder annotation for populating the instances to the constants the order is a bit different. The recommended way is to: 1) create a constant field (like I have a class called ModItems to contain these) that is initialized to null but annotated with @ObjectHolder. 2) handle the related registry event where you instantiate (using Java new operator) each item. 3) register each item. 4) The @ObjectHolders will automatically be updated after the event is finished firing. Here is my ModItems class showing that sort of thing: https://github.com/jabelar/ExampleMod-1.12/blob/master/src/main/java/com/blogspot/jabelarminecraft/examplemod/init/ModItems.java Note that I don't use the registerAll() method. Instead I just register each one by one.