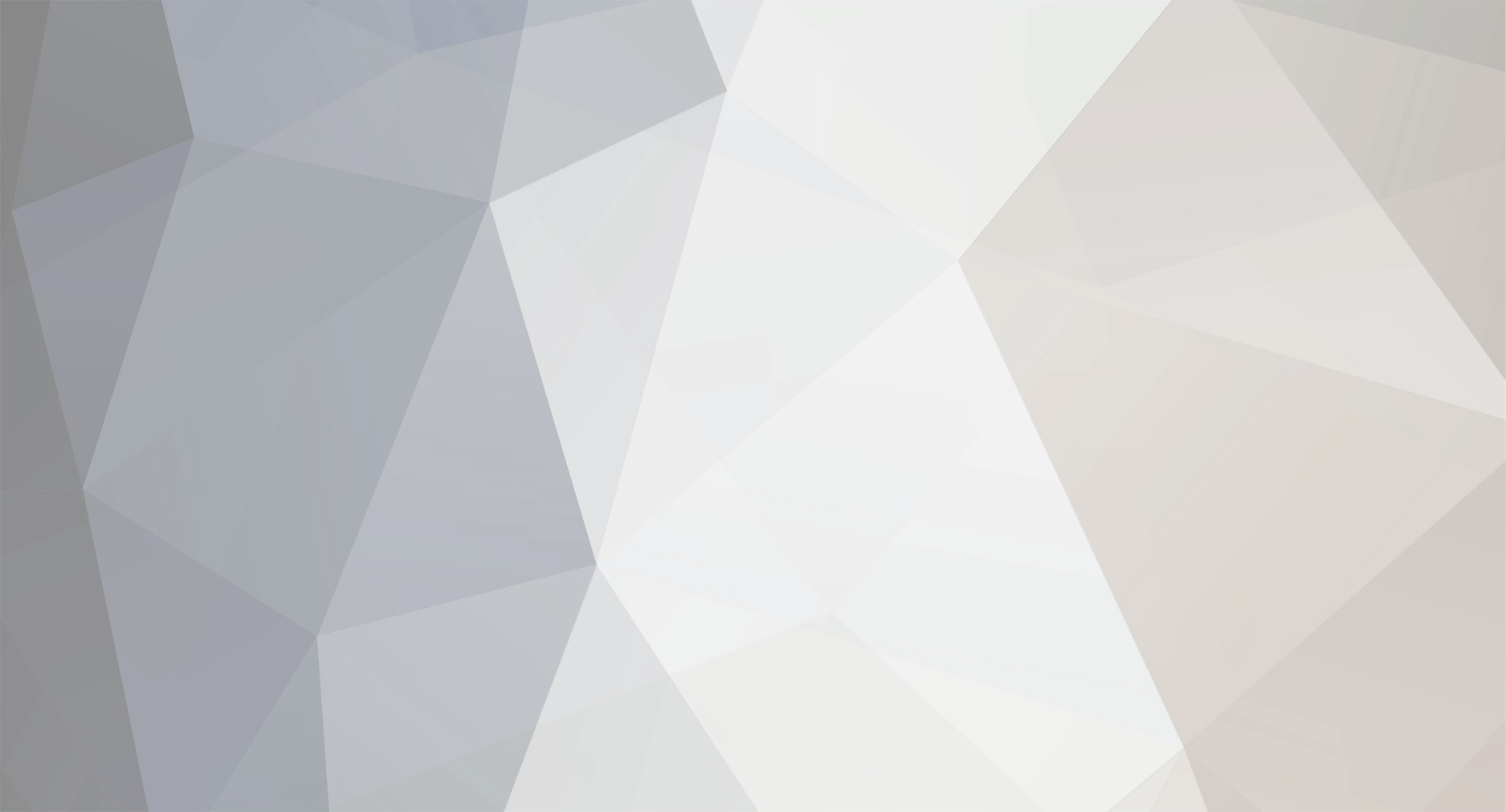
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
In Eclipse you can use the Type Hierarchy to find all classes that extend a class, or all classes that implement an interface. So if you find the base Event class (in net.minecraftforge.fml.common.eventhandler package) and select the "Event" name of the class and right-click you can choose Type Hierarchy from the pop-up menu. It will then show you all classes that are considered children of the Event class. Note that you will need to expand those classes to look for any additional subclasses that are contained in those classes. For example, the "Pre" and "Post" events are included within the same class as the main event in many cases.
-
How to Create Custom Model Armor Without Programs
jabelar replied to Ragnar's topic in Modder Support
We already told you where the sample code is. It is in the LayerBipedArmor class, that extends LayerArmorBase which also has code that might be useful for you. -
How do objects like "PathNavigate" and many others persist across saves?
jabelar replied to MrChoke's topic in Modder Support
Unless there is a writeNBT() type method in the class, or some other class that grabs data from the class, then it probably isn't actually saved. -
How to Create Custom Model Armor Without Programs
jabelar replied to Ragnar's topic in Modder Support
Vanilla armor is done by LayerBipedArmor and related model classes. This is a render layer of the player (and other biped mobs that wear armor). For your custom armor you should copy those classes and modify the way you want for your own layer, then use events to add the layer during rendering. How "crazy" is your custom armor? Are you just modifying it a bit, or really different from vanilla? In any case, most Minecraft models are simply made up of "block" like shapes. So you don't really need a program like Techne. You can just draw it on a piece of paper and put in all the coordinates of the blocks directly into your code. And if you're just modifying the vanilla armor you already have that code to reference so should give you a great starting point. -
With most events you have a choice, you can add to the the vanilla behavior or you can fully replace it. It depends on the event but some have the ability to "cancel" the vanilla behavior (and execute the code you put in the event handler), some events allow you to directly replace the contents of a field passed in the event, and some events use the Results return value (like Result.DENY). It is usually described a bit in the comments for the event, and you also trace the call hierarchy to see how the result is used.
-
How to Create Custom Model Armor Without Programs
jabelar replied to Ragnar's topic in Modder Support
Okay, in that case you just need to make your own layer model then intercept the vanilla layers with the rendering events. For making your own layer model, look at how LayerBipedArmor works and see if you can copy/modify that to your needs. -
[1.12.2]3D items models rendering differently in different slots
jabelar replied to jabelar's topic in Modder Support
It's weird because my mod basically copies the RenderItem code into my own extended class and calls exactly the same logic with the exception of changing the enchantment glint textures/color. I call the TESR rendering stuff exactly the same way. I suspect the problem has to do with the other mod's Render classes referencing a RenderManager that references an entity map that that maybe I have overwritten or something. Basically, I'm injecting (through Reflection) my own RenderItem, but like many things in Minecraft the use of the vanilla RenderItem isn't very clean -- instead of always grabbing it from the Minecraft class, a lot of classes make their local own copy/reference to it. So I need to go through a bunch of classes to make sure they all get converted. Anyway, looks like I've got some serious debugging to do... -
[1.12.2]3D items models rendering differently in different slots
jabelar replied to jabelar's topic in Modder Support
Okay, yeah it looks like it is following a TESR path. Let me see why their TESR isn't rendering. -
[1.12.2]3D items models rendering differently in different slots
jabelar replied to jabelar's topic in Modder Support
Oh, and if I dump the quads from the itemmesher for the non working items they don't have anything, but in the working items they have a bunch of quads. So it seems I'm onto something. Gotta figure out why these entity parented ones end up with no quads in the mesher... -
[1.12.2]3D items models rendering differently in different slots
jabelar replied to jabelar's topic in Modder Support
Okay, I'm still working on debugging this. One thing I noticed that in the mod that is causing trouble, the items that don't work have model json that has parent = builtin/entity. The items that do work have model json that has parent = builtin/generated. I can't find much information on the "builtin/entity" parent model. It seems to be used for blocks like chests and such, but not really that many things. Anyone know more about builtin/entity? And any idea it wouldn't render in a slot or the world, but will render in the hotbar? -
One thing you'll find when modding is that, unfortunately, Minecraft original code isn't written well in many respects including the use of type hierarchy and inheritance. There are two schools of thought about inheritance: (a) parent classes should embody all functionality and child classes restrict it, (b) parent classes embody the common functionality and child classes expand it. But Minecraft mixes it all up -- sometimes they fully override functions in ways which simply change the functionality without specifically restricting or expanding it. In some cases they use abstract classes for common stuff, sometimes not. And to top it all off it is not well encapsulated so there is a mix of private, protected and public fields and methods. So, with that being said, while it would be nice if they used interfaces to group common functionalities, they don't do that much and it is likely also inconsistent. I think it would be easiest to handle vanilla things by simply hardcoding the list, and handle other mods by hoping modders use the type hierarchy better or alternatively providing an API that they can hook into to identify themselves.
-
I have a weird bug in one of my mods. The mod intercepts the RenderItem methods for renderEffect() in order to configurably change the color and/or texture of enchantment glint effects. This works perfectly for vanilla items and the rendering is fine when item is in inventory, on ground, wielded by player, used as tab icon for creative tab, wielded by mob, and so forth. However, there have been a couple other mods where an incompatibility has been logged as an issue. In this case, items will render in the player hotbar but not (nothing is shown) in the inventory slot. In other words, something that won't show in inventory shows when it is dragged to the hotbar. The item does not show when wielded either, but does when it is worn (it is added as a layer to the player model). These items have 3d model (they are things like broomstick). So I think it is an issue with the ItemModelMesher registration or maybe the refresh of the resources. So I'm tracking that down. I'm just surprised though that it behaves differently in the hotbar slots than the other inventory slots. Does anyone know why items (especially those with 3D model) would render differently in the hotbar slot than in other gui slots?
-
Is a mod that is made for 1.12.2 also compatible with 1.12.1 and 1.12.0? And same with 1.11.2 with 1.11.1 and 1.11.0? And so forth for each version? Also, when working on older versions my mappings setting in my build.gradle often doesn't match the version. Is there any way to know what the last version of mappings was relevant for say 1.10.x or 1.11.x? Right now I'm just sort of guessing based on Forge release dates but it still takes a bit to get it right. Ideally I'd like the latest mapping that was intended for a given version. Is that listed anywhere?
-
Okay, so is your problem solved then?
-
Of course all code calling it also has to have @Optional annotation, I didn't say otherwise. The proposal is to make an @Optional-annotated method that is a pre-init handler, that in turn registers the event handling class (which is also @Optional-annotated since it will refer to custom events and probably other stuff dependent on the mod). Both the method that registers and the handling class itself would be stripped from the code if the mod isn't present. I think it would work, right? Anyway, I only recommended it because he seemed to be giving up on our first (both me and VoidWalker) recommendation which was the same as yours: i.e. that conditional (based on isModLoaded() check) registration should work. We have even already discussed that for the most part you can assume that the JVM and ClassLoader won't have trouble with code that references other unloaded mods unless you invoke something from the mod, and also discussed that if he's worried about trusting that he can do additional steps to protect from alternate JVM behavior. So I think we've given him all the same advice -- checkIsModLoaded() and conditionally register the event handler. @Cadiboo Can you clarify what problem you're actually having with all this? I think the advice so far has been pretty clear.
-
If you're already working on it, or if you're making new stuff, the best way to "future proof" for 1.13 is to perform the flattening yourself. Basically make all variants of your blocks a separate block. This should generally be compatible and you can move to such a scheme now in 1.12.2. However, I agree with VoidWalker that otherwise I wouldn't worry about it until we see how 1.13 works and what provisions it already has for data fixing.
-
Can you explain more specifically what you're trying to do, like with an example? For any code executing within an event handling method, the proxy approach (or similar approach also discussed in this thread) should work fine. Then i also think it is possible to conditionally register event. The key to all this is to instantiate classes with mod-dependent code dynamically. So I think you can do the same for the event handler class itself. There is also the @Optional annotation system which can control class loading based on mod dependencies: If you use another mod's API and make calls to its methods in your code, your mod will fail to execute if the other mod isn't loaded. Although you can check if the mod is loaded with the mod loader class, that still cause failures if you've included code that calls the other mod. It is therefore highly recommended, where possible, to use the @Optional annotation specifying the modid for the other mod. Any code marked with that annotation will only be considered for execution if a mod with that modid is loaded. Furthermore, if the code is run once during mod loading you can organize the code by creating a secondary pre-init (or whichever FML phase you need) method in your main @Mod class and annotate it with @Optional specifying the modid of the mod you depend on. Then inside that method (and any classes that are only referenced by that method) you can freely interact with that mod and the method will only run if that mod is present.
-
What exactly do you mean? Do you mean the other mod has created custom events (i.e. extended the Event class) and is posting them?
-
Look at the other methods in the class you're extending (CommandBase). There are methods there regarding permissions you need to override.
-
Yep, pretty much what I was suggesting, since I've seen you suggest it in the past.
-
Why don't you want a TileEntity? It doesn't hurt and is the obvious way to implement it. Any block that needs to have more than basic properties should have a TileEntity. That is the point.
-
Did you register the block model? I see you have a method that is named to indicate that the item model is registered, but not clear if you registered the block model itself.
-
It is a bit weird that there are no errors in the console. Missing models or textures usually cause an error. I guess maybe just try a simpler model and then build it back up to try to isolate the problem.
-
Yeah, I use a plugin just called "JSON Editor". But yeah best way is through a plugin in Eclipse. Doing it in external editor can cause trouble like UTF-8 incompatibilities and such.
-
I see some errors when I do it. It may be a problem with the way you're editing your file, or maybe the way you're pasting it. For example, if I take the code from your post and cut and paste directly I get some errors like hidden characters and stuff. Sometimes there are invisible characters and formatting that can mess things up. Like it is telling me that one line has a hidden /udeff character whatever that means. On the other hand, it may be that your code is good and the way I'm cutting and pasting doesn't represent.