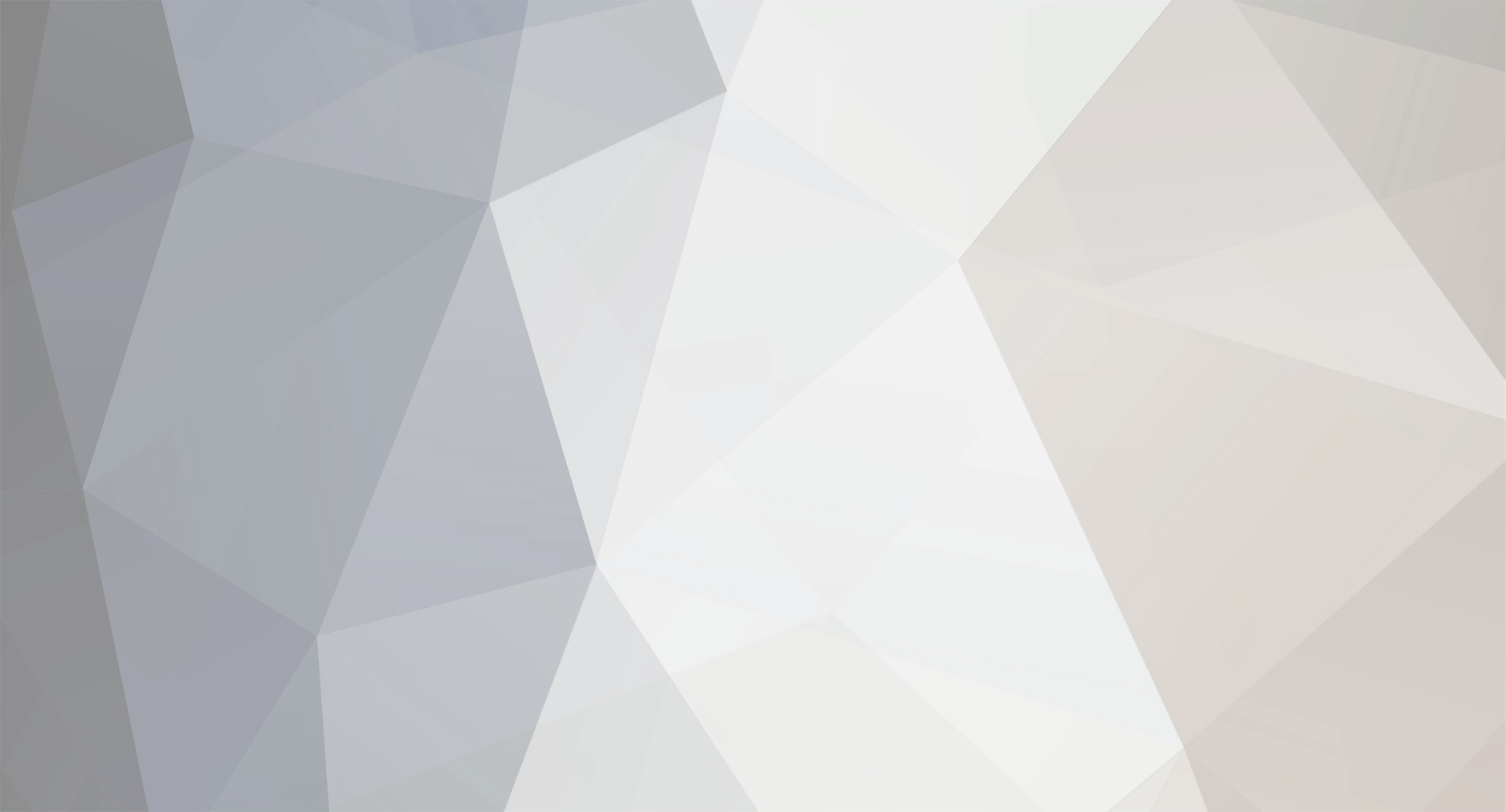
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
[1.7.10] Adding a horse entity to a custom entity's fields
jabelar replied to shultzy's topic in Modder Support
You made a typo in your bounding box: AxisAlignedBB.getBoundingBox((double) this.posX - minMaxAABB, (double) this.posY - minMaxAABB, (double) this.posZ - minMaxAABB, (double) this.posX - minMaxAABB, (double) this.posY + minMaxAABB, (double) this.posZ + minMaxAABB)); The second posX should be +minMaxAABB not -minMaxAABB. -
Is this a custom mob or the vanilla mobs. The problem will come from the pathfinding. Most vanilla entities, and many that people make custom, have an AI list that includes pathfinding using the navigator. The pathfinding specifically prevents collisions since its normal function is to find a path where the entity doesn't collide. I think, not entirely sure, that you can set the position of an entity inside a block's space, but you can't assume that a mob using normal walking AI would ever do that.
-
Worth trying. The key is to find an event that takes place after the position is updated but before it is rendered, so that might work. I also think there may be an issue with client-server sync. The client is able to move entities a bit between server syncs, so it may be related to that.
-
[1.7.10] How to change the texture of buttons in a GUI
jabelar replied to Thornack's topic in Modder Support
You mean GuiButton, right? He is already extending GuiScreen, but the drawScreen() method there actually usually isn't used for the buttons since they have their own drawButton() method that is called automatically if the button is in the GuiScreen's button list. Here is an example of the method from one of my mods.: /** * Draws this button to the screen. */ @Override public void drawButton(Minecraft mc, int parX, int parY) { if (visible) { boolean isButtonPressed = (parX >= xPosition && parY >= yPosition && parX < xPosition + width && parY < yPosition + height); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(bookPageTextures[1]); int textureX = 0; int textureY = 192; if (isButtonPressed) { textureX += 23; } if (!isNextButton) { textureY += 13; } drawTexturedModalRect(xPosition, yPosition, textureX, textureY, 23, 13); } } } The key is the drawTexturedModalRect() method which is taking part of a texture. In this case I take a different part of the texture based on whether the button is pressed or not. -
Yes, I tried the resetting thing and it pretty much works with following code (event handler for LivingUpdateEvent): @SubscribeEvent(priority=EventPriority.NORMAL, receiveCanceled=true) public void onEvent(LivingUpdateEvent event) { // This event has an Entity variable, access it like this: event.entity; // and can check if for player with if (event.entity instanceof EntityPlayer) if (event.entityLiving instanceof EntityPlayer) { EntityPlayer thePlayer = (EntityPlayer)event.entityLiving; if (thePlayer.ticksExisted > 5) // make sure there is a valid prev position, don't run on first tick { // You should also check to see if your paralysis effect is active here! thePlayer.setPosition(thePlayer.prevPosX, thePlayer.prevPosY, thePlayer.prevPosZ); } } } The problem though is that it doesn't entirely prevent the movement, rather it shows the player attempting to move. But it is kinda cool, maybe you'll like it.
-
Why are you wanting to do that?
-
I agree that people should mostly figure things out on their own, so my "tutorials" never give full code but rather just point the direction. But the proxy concept takes a while to understand and it is one of the first things a new modder will encounter -- no point in scaring off new modders with one of the more obtuse aspects of modding.
-
In terms of organizing and instantiating your proxy, you might want to check out my tutorial here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-organizing-your-proxy.html There is a section there about an example annotation and instantiation of the proxy. In terms of your problem, if the entity rendering is only registered in your client proxy then it shouldn't be invoked on server side. In the link above I explain where I recommend you do things like registering renderers.
-
Can you give me a little example? For learning how to handle events (this is worth learning as it is a powerful modding approach) I have a tutorial here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html For the random part, that is just Java. Always remember that in Minecraft modding that you can do anything that Java can do. For example, Java has the Random class: http://docs.oracle.com/javase/7/docs/api/java/util/Random.html Use the random class to generate a number, use an if statement to compare that number to some chance, and if the chance happens play the sound. Note that the client tick handler is fired 20 times per second. So if you want 6 minutes on average between playing the sound, you need the chance to play to be less than 1/3600.
-
For the conversion, after setting up the general system according to diesieben07's link, based on your code you need to: 1. Make your own "custom payload" message packet. In that packet you would send the first byte as a unique identifier of your mod's packets -- like maybe a 0 represents some data for an entity, 1 some data for a GUI, or whatever packet types you need. 2. Instead of byte arrays for the data use ByteBuf. Then similar to your byte array there are methods for packing data together into and out of the buffer. 3. Based on the information read out of the ByteBuf you can do whatever you want (in your code looks like you create particles and such).
-
[1.8][CLOSED] Is IExtendedEntityPorperties disallowing using SideOnly?
jabelar replied to Ernio's topic in Modder Support
You don't need to go overboard with SideOnly. You only need SideOnly if the code in the method calls on something that is SideOnly. The whole SideOnly thing is kinda inconsistent even in the Minecraft code. Generally it shouldn't hurt to have a class compiled that never gets run, so I think the savings (i.e. in memory) they get by annotating some things as SideOnly isn't worth the hassle of it all. But generally don't annotate as SideOnly unless you're forced to -- because you're calling code that is SideOnly. Even diesieben07 says: (from thread at http://www.minecraftforge.net/forum/index.php/topic,18608.msg94192.html#msg94192) -
It is possible to do it in different ways. Some things need to be done in order though -- like you need to register recipes after the items and blocks if the recipes use those items or blocks. Also as I mentioned above, there are certain methods that are unique to each FML life cycle event -- for example only the pre-init gives a recommended config file path and only the server starting event gives a server command registration hook. The way I do it seems to work. See my explanation here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-organizing-your-proxy.html
-
One of the points of preinit, init and postinit is to allow you to order things with respect to other mods. For example, if people generally register their items and blocks in preinit then you can feel confident that in your init you could register a recipe that included something from their mod. Also, each of the events passes some fields and methods which may be useful. For example, the FMLPreInitializationEvent has the getSuggestedConfigurationFile() method so it makes sense that you might initialize your Configuration in pre-init using that file path (although you could also presumably save the path for later). You should check out the methods for each of the events to see things that might be useful. Note also that besides the common preinit, init and postinit there are a couple other "FML Life Cycle" events such as a server starting event (this is good place to register any custom server commands). Here is how I've organized mine, maybe it can give you some ideas: http://jabelarminecraft.blogspot.com/p/minecraft-modding-organizing-your-proxy.html Note that the sphere stuff in my client proxy is an example that if you have methods that you only need on (or that will only compile on) the client side you can put them in the client proxy as well. In this case my method calls the Minecraft.getMinecraft() which is only valid on client side.
-
That is cool. Good find.
-
Yes, if you're going to need the mod on the client side then using regular .lang localization would be easiest way.
-
I think you'd need at least something on the client side to do this. In Java I think you can use the Locale class to get information on the system's local, but that would have to be run on the client.
-
[1.7.10] How to render a gui over another gui?
jabelar replied to HappyKiller1O1's topic in Modder Support
You can use events for this. You can intercept the opening, init, button behavior and drawing of any GuiScreen with: GuiOpenEvent GuiScreenEvent.ActionPerformedEvent GuiScreenEvent.DrawScreenEvent GuiScreenEvent.InitGuiEvent -
[1.8] Create custom fluid without forge fluid stuff
jabelar replied to MacDue's topic in Modder Support
I'm not sure, but I think you can create classes that extend BlockDynamicLiquid and BlockStaticLiquid and modify those so that the flowing part works in the updateTick() method. If you look at how lava and water are made, it is simply through this registration: new BlockDynamicLiquid(Material.water)).setHardness(100.0F).setLightOpacity(3).setUnlocalizedName("water").disableStats()); new BlockDynamicLiquid(Material.lava)).setHardness(100.0F).setLightLevel(1.0F).setUnlocalizedName("lava").disableStats()); And of course you can create your own materials if it makes sense. You'll note that the parent class BlockLiquid has a property called LEVEL which is the fluid level within the block from 0 to 15. You could use that to control the model used to display the liquid. Anyway, I think that could work. -
[1.7.10] How to add a slider gui option?
jabelar replied to HappyKiller1O1's topic in Modder Support
I'm not saying to use them exactly, but rather copy the code to make your own version. So if you copied the GuiOptionsSlider class then instead of passing a GameSettings.Options enum you can make your own enum (or if you only have one slider, maybe none at all). But GuiSlider can also be a reference. The first thing to do when trying to copy similar code is to change the field names to something meaningful -- you'll have to do some detective work to figure that out sometimes. For example, in GuiSlider the constuctor parameters have names like p_i45536_2_ but if you match things to the call with the super constructor to GuiButton you'll see that these parameters represent the button id, x, y, width, height, name, etc. So rename all the fields in your class so that they are easy to read and understand. For example, I think field_175227_p represents the slider positions (from 0.0 to 1.0). This slider needs to be put on a GUI, so you'll need a custom GuiScreen where you add the button. Then, in the actionPerformed() method of that GUIScreen you would react when your slider button is acted on. When the slider is acted on, you're going to want to change a value somewhere that the rest of your code can process. Since most processing needs to happen on the server, you generally should send a packet. So if someone selected 10 on the slider and you wanted that to mean buy 10 diamonds, then you would send a custom packet that when received by the server would put 10 diamonds into the player inventory. -
[1.7.10] How to add a slider gui option?
jabelar replied to HappyKiller1O1's topic in Modder Support
If you look in the initGui() method of the GuiOptions class you can see how it creates a slider (by creating a new GuiOptionsSlider instance). -
I think diesieben07's point is that until a block update happens it may appear to work temporarily. If you saw it working before, it was probably due to no block update being triggered.
-
i'm not certain, but you can get these errors if you keep creating buffers and never closing them. In you "channel handler" class you have methods encodeInto() and decodeInto() and both create a packet buffer that they don't close the buffers. So I think that means it will keep using up memory more and more (a memory "leak"). So try closing those buffers before the end of the methods. Also, the overall packet code you've got seems too complicated. Using the most recent recommended packet system from diesieben07, you should be able to get by with just creating a message class that extends IMessage and registering the messages. Check out his tutorial here: http://www.minecraftforge.net/forum/index.php/topic,20135.0.html
-
That is a complicated model so it is hard to easily see what might be wrong. One thing I think may be wrong is that you might have the array indexes reversed. Since you put the angles for the cycle all in one row, I think that where you have: LeftFemur.rotateAngleX = degToRad(undulationCycle[cycleIndex][0]) ; RightFemur.rotateAngleX = degToRad(undulationCycle[cycleIndex][1]) ; LeftHumerus.rotateAngleX = degToRad(undulationCycle[cycleIndex][2]) ; RightHumerus.rotateAngleX = degToRad(undulationCycle[cycleIndex][3]) ; maybe it should be: LeftFemur.rotateAngleX = degToRad(undulationCycle[0][cycleIndex]) ; RightFemur.rotateAngleX = degToRad(undulationCycle[1][cycleIndex]) ; LeftHumerus.rotateAngleX = degToRad(undulationCycle[2][cycleIndex]) ; RightHumerus.rotateAngleX = degToRad(undulationCycle[3][cycleIndex]) ; See I switched around the indexes. If that doesn't help, then I think you have to add more console statements to see if the values are progressing through the array as you expect.
-
[SOLVED][1.8]Changing block state from tile entity is causing problems
jabelar replied to jabelar's topic in Modder Support
Thanks. I think the reason I'm encountering this now is that previously if I had blocks with tile entities, I didn't tend to use metadata but rather used fields in the tile entity. Now since the 1.8 block properties are so directly tied to models through the JSON, it seemed more appropriate to go the route of having the state kept in the block state rather than as a tile entity field. -
Okay, so I'm making a simple "machine" block that is a tanning rack and converts hides (a custom item) into leather. I am pretty confident in making container blocks with tile entities, but this time I thought I'd try to be fancy with the 1.8 models and have it so that the texture changes based on the progress of the tanning. So I created a boolean property for the block called TANNING_COMPLETE and I was hoping that I could simply have my tile entity update the property value depending on what is happening in the associated inventory. So I thought I could just set the block state from the tile entity's update method. The problem is that when I try to update the block state, it seems that it is actually replacing the whole block (and associated tile entity) since it (a) exits the GUI, (b) drops everything that was in the inventory. So I guess I'm using the wrong method to set the state of the block. I think I need a way to change the state without trigging a block replacement. The code I have been using: public static void changeBlockBasedOnTanningStatus(boolean parTanningComplete, World parWorld, BlockPos parBlockPos) { parWorld.setBlockState(parBlockPos, BlockSmith.blockTanningRack.getDefaultState().withProperty(TANNING_COMPLETE, parTanningComplete)); } What is the proper way to just change a block property value without disrupting the block or associated tile entity? EDIT: Looking at BlockFurnace's func_176446_a() it seems that they actually have to re-associate the tile entity to the position. Is that the way to do it?