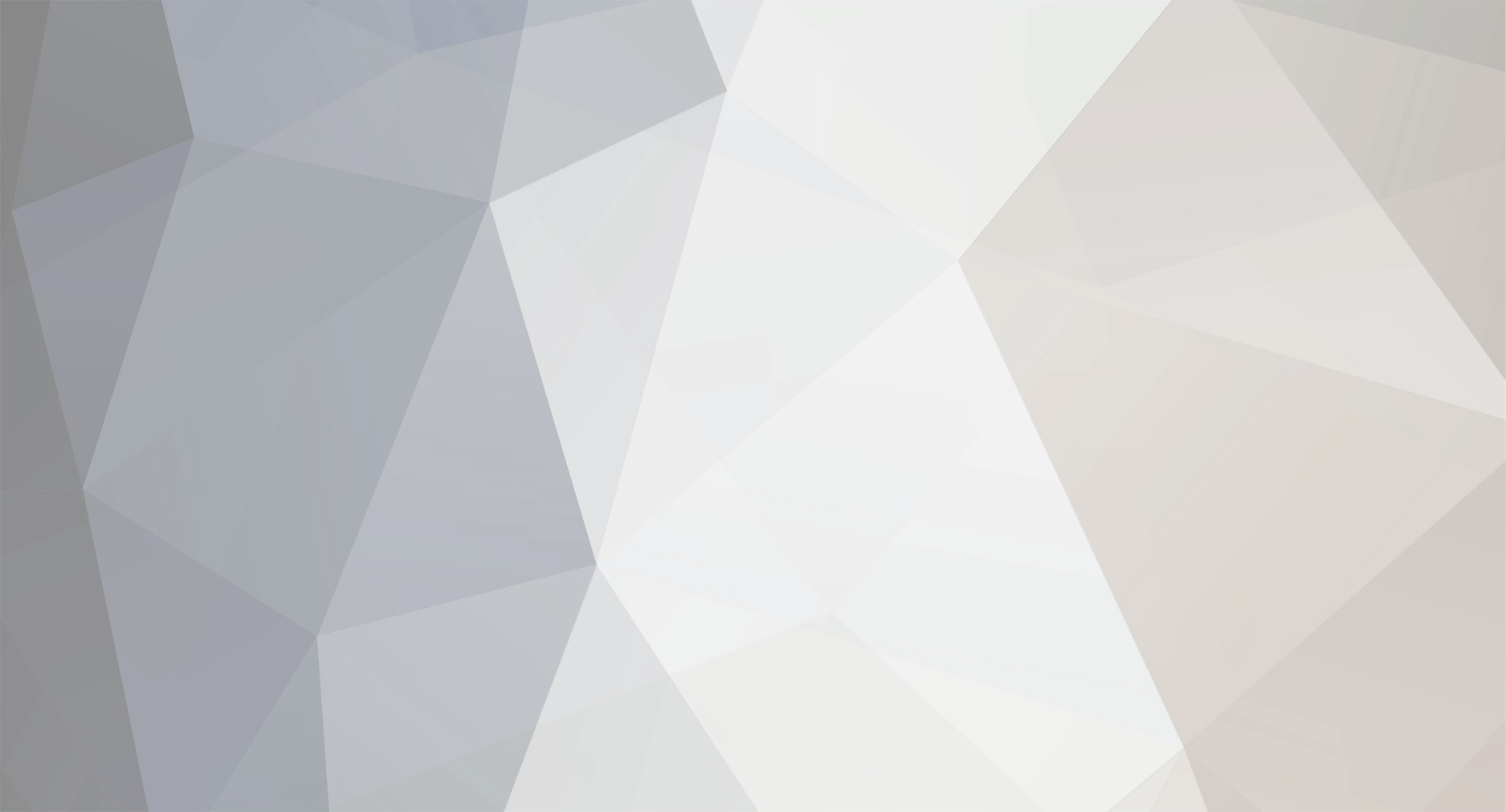
SnowyEgret
Members-
Posts
112 -
Joined
-
Last visited
Everything posted by SnowyEgret
-
NullPointerException when spawning entity
SnowyEgret replied to UntouchedWagons's topic in Modder Support
Some object, somewhere, has tried used reflection to find a constructor for your FakePlayer class taking a single parameter of type World. Since your constructor takes 4 parameters, it could not be found and the object threw the exception. You need to look at the exception's stack trace to find where the problem is. -
[1.7.10] Issue with updating from 1.7.2 to 1.7.10
SnowyEgret replied to mariosio's topic in Modder Support
Mariosio, There is a child board to Modder Support called ForgeGradle. You will find more of an audience for this sort of problem there. -
If you have a reference to a player, you can get an ItemStack in, for example, the fourth slot like this: ItemStack stack = player.inventory.getStackInSlot(3); If you want the item: Item item = stack.getItem();
-
Item disappears when dragged from first slot in container
SnowyEgret replied to SnowyEgret's topic in Modder Support
I solved the doubling up problem by testing for world.isRemote() in MyItem.onItemUse(), however, the GUIs where no longer synchronized when two players open the container in multiplayer, and items were still disappearing when moving them around in the inventory (in both GUIs). On reflection, I think this has to do with the inventory being added to an Item. Here is my test mod again. MyItem (the test for isRemote is commented out because this way it works perfectly in multiplayer): public class MyItem extends Item { InventoryBasic inventory = new InventoryBasic("", false, 9); @Override public boolean onItemUse(ItemStack s, EntityPlayer p, World w, int x, int y, int z, int side, float sx, float sy, float sz) { // if (w.isRemote) { p.openGui(MyMod.instance, 0, w, x, y, z); return true; // } // return false; } } MyContainer: public class MyContainer extends Container { public MyContainer(InventoryPlayer playerInventory, IInventory inventory) { for (int i = 0; i < inventory.getSizeInventory(); i++) { addSlotToContainer(new Slot(inventory, i, 8 + i * 18, 18)); } for (int i = 0; i < 3; i++) { for (int j = 0; j < 9; j++) { addSlotToContainer(new Slot(playerInventory, j + i * 9 + 9, 8 + j * 18, 49 + i * 18)); } } for (int i = 0; i < 9; i++) { addSlotToContainer(new Slot(playerInventory, i, 8 + i * 18, 107)); } } @Override public boolean canInteractWith(EntityPlayer player) { return true; } } MyGui: public class MyGui extends GuiContainer { public MyGui(MyContainer container) { super(container); } @Override protected void drawGuiContainerBackgroundLayer(float p1, int p2, int p3) { int x = (width - xSize) / 2; int y = (height - ySize) / 2; mc.getTextureManager().bindTexture(new ResourceLocation("textures/gui/container/generic_54.png")); drawTexturedModalRect(x, y, 0, 0, xSize, 35); drawTexturedModalRect(x, y + 35, 0, 126, xSize, 96); } } MyGuiHandler: public class MyGuiHandler implements IGuiHandler { IInventory inventory; public MyGuiHandler(IInventory inventory) { this.inventory = inventory; } @Override public Object getServerGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { if (id == 0) { return new MyContainer(player.inventory, inventory); } return null; } @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { if (id == 0) { return new MyGui(new MyContainer(player.inventory, inventory)); } return null; } } MyMod: @Mod(modid = "myMod", name = "MyMod", version = "") public class MyMod { @Instance("myMod") public static MyMod instance; @EventHandler public void preInit(FMLPreInitializationEvent event) { MyItem myItem = new MyItem(); myItem.setCreativeTab(CreativeTabs.tabMisc); myItem.setUnlocalizedName(myItem.getClass().getSimpleName()); GameRegistry.registerItem(myItem, "myMod"); NetworkRegistry.INSTANCE.registerGuiHandler(this, new MyGuiHandler(myItem.inventory)); } } I can't believe something so fundamental to the game is broken. I must be either doing something wrong or leaving something out. Has anyone succeeded in doing this? -
Item disappears when dragged from first slot in container
SnowyEgret replied to SnowyEgret's topic in Modder Support
Ewe, Where you trying to give an inventory to an Item, like me? Containers seem to work well enough for blocks. -
Item disappears when dragged from first slot in container
SnowyEgret replied to SnowyEgret's topic in Modder Support
Yes, the doubling up suggests things are being done on both sides when they should be done only on one. I am registering myItem and an instance of MyGuiHandler in MyMod.preInit: @EventHandler public void preInit(FMLPreInitializationEvent event) { MyItem myItem = new MyItem(); myItem.setCreativeTab(CreativeTabs.tabMisc); myItem.setUnlocalizedName(myItem.getClass().getSimpleName()); GameRegistry.registerItem(myItem, "myMod"); NetworkRegistry.INSTANCE.registerGuiHandler(this, new MyGuiHandler(myItem)); } Is this doing anything on the client side that shouldn't be? Apart from that, I'm not really doing anything else except opening the gui from MyItem.onItemRightClick() For the sake of clarity, I have moved the gui handling to it's own class: public class MyGuiHandler implements IGuiHandler { MyItem myItem; public MyGuiHandler(MyItem myItem) { this.myItem = myItem; } @Override public Object getServerGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { System.out.println("[MyGuiHandler.getServerGuiElement] id=" + id); if (id == 0) { return new MyContainer(player.inventory, myItem.inventory); } return null; } @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { System.out.println("[MyGuiHandler.getClientGuiElement] id=" + id); new Throwable().printStackTrace(); if (id == 0) { return new MyGui(player.inventory, myItem.inventory); } return null; } } MyGuiHandler.getClientGuiElement() is being called twice. I have printed a stack trace from getClientGuiElement(). Only one call to EntityPlayer.openGui() originates from MyItem.onItemRightClick(). Is this normal behavior? [MyGuiHandler.getClientGuiElement] java.lang.Throwable at container.test.MyGuiHandler.getClientGuiElement(MyGuiHandler.java:24) at cpw.mods.fml.common.network.NetworkRegistry.getLocalGuiContainer(NetworkRegistry.java:263) at cpw.mods.fml.common.network.internal.FMLNetworkHandler.openGui(FMLNetworkHandler.java:93) at net.minecraft.entity.player.EntityPlayer.openGui(EntityPlayer.java:2514) at container.test.MyItem.onItemRightClick(MyItem.java:21) at net.minecraft.item.ItemStack.useItemRightClick(ItemStack.java:166) at net.minecraft.client.multiplayer.PlayerControllerMP.sendUseItem(PlayerControllerMP.java:434) at net.minecraft.client.Minecraft.func_147121_ag(Minecraft.java:1557) at net.minecraft.client.Minecraft.runTick(Minecraft.java:2044) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1039) at net.minecraft.client.Minecraft.run(Minecraft.java:961) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:606) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [MyGuiHandler.getServerGuiElement] [MyGuiHandler.getClientGuiElement] java.lang.Throwable at container.test.MyGuiHandler.getClientGuiElement(MyGuiHandler.java:24) at cpw.mods.fml.common.network.NetworkRegistry.getLocalGuiContainer(NetworkRegistry.java:263) at cpw.mods.fml.common.network.internal.FMLNetworkHandler.openGui(FMLNetworkHandler.java:93) at net.minecraft.entity.player.EntityPlayer.openGui(EntityPlayer.java:2514) at cpw.mods.fml.common.network.internal.OpenGuiHandler.channelRead0(OpenGuiHandler.java:16) at cpw.mods.fml.common.network.internal.OpenGuiHandler.channelRead0(OpenGuiHandler.java:11) at io.netty.channel.SimpleChannelInboundHandler.channelRead(SimpleChannelInboundHandler.java:98) at io.netty.channel.DefaultChannelHandlerContext.invokeChannelRead(DefaultChannelHandlerContext.java:337) at io.netty.channel.DefaultChannelHandlerContext.fireChannelRead(DefaultChannelHandlerContext.java:323) at io.netty.channel.SimpleChannelInboundHandler.channelRead(SimpleChannelInboundHandler.java:101) at io.netty.channel.DefaultChannelHandlerContext.invokeChannelRead(DefaultChannelHandlerContext.java:337) at io.netty.channel.DefaultChannelHandlerContext.fireChannelRead(DefaultChannelHandlerContext.java:323) at io.netty.handler.codec.MessageToMessageDecoder.channelRead(MessageToMessageDecoder.java:103) at io.netty.handler.codec.MessageToMessageCodec.channelRead(MessageToMessageCodec.java:111) at io.netty.channel.DefaultChannelHandlerContext.invokeChannelRead(DefaultChannelHandlerContext.java:337) at io.netty.channel.DefaultChannelHandlerContext.fireChannelRead(DefaultChannelHandlerContext.java:323) at io.netty.channel.DefaultChannelPipeline.fireChannelRead(DefaultChannelPipeline.java:785) at io.netty.channel.embedded.EmbeddedChannel.writeInbound(EmbeddedChannel.java:169) at cpw.mods.fml.common.network.internal.FMLProxyPacket.processPacket(FMLProxyPacket.java:86) at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:247) at net.minecraft.client.multiplayer.PlayerControllerMP.updateController(PlayerControllerMP.java:321) at net.minecraft.client.Minecraft.runTick(Minecraft.java:1693) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1039) at net.minecraft.client.Minecraft.run(Minecraft.java:961) at net.minecraft.client.main.Main.main(Main.java:164) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:606) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) -
Item disappears when dragged from first slot in container
SnowyEgret replied to SnowyEgret's topic in Modder Support
Ok, I have implemented getServerGuiElement having it return MyContainer. If I start an singleplayer game and open it to the lan, connect to the game from another machine, put MyItem in each player's inventory, and on MyItem's gui so that MyItem's inventory is visible on both machines, I get this on behavior: On the remote client, things are perfect. I can put items in the inventory, move them around as much as I want, and MyItem's inventory is updated on the other client (the one that has the integrated server). On the client which started the game (the one with the integrated server), when I put a single item in MyItem's inventory, two show up, then when I click on it to drag it, it disappears. The view is synchronized on the other machine (in other words, the items double up then disappear on both machines). Either this is a bug, or I am still not doing all that is necessary to set this up properly. Incidentally, I tried this: public MyItem() { inventory = new InventoryBasic("", false, 9); inventory.func_110134_a(this); } @Override public void onInventoryChanged(InventoryBasic inventory) { System.out.println("[MyItem.onInventoryChanged] this.inventory=" + this.inventory); System.out.println("[MyItem.onInventoryChanged] inventory=" + inventory); } where MyItem implements IInvBasic. This doesn't have any effect on the behavior except that onInventoryChanged is called. I am not sure what I would do with this anyway. -
Item disappears when dragged from first slot in container
SnowyEgret replied to SnowyEgret's topic in Modder Support
If I understand correctly, I return the container? @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return new MyContainer(player.inventory, item.inventory); } Things are even worse now. When I place a single item in a slot, two show up. So far I only having considered the case where the item (the game's instance of MyItem) is in one player's inventory. I left the server side out, thinking it is only needed to synchronize the two GUIs if two players are modifying the inventory at the same time. -
I have given an item an inventory and created a GUI to manage it. It runs as expected, except that when I place a single in item the first slot, it returns to the player inventory instead of sicking to the pointer when dragging it to a new slot. Here is a simple test mod that recreates the problem, isolated from any side effects. My item: package container.test; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInvBasic; import net.minecraft.inventory.InventoryBasic; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public class MyItem extends Item { InventoryBasic inventory; public MyItem() { inventory = new InventoryBasic("", false, 9); } @Override public ItemStack onItemRightClick(ItemStack stack, World w, EntityPlayer player) { player.openGui(MyMod.instance, 0, w, 0, 0, 0); return stack; } } My GUI: package container.test; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; public class MyGui extends GuiContainer { public MyGui(InventoryPlayer playerInventory, IInventory inventory) { super(new MyContainer(playerInventory, inventory)); } @Override protected void drawGuiContainerBackgroundLayer(float p1, int p2, int p3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); int x = (width - xSize) / 2; int y = (height - ySize) / 2; mc.getTextureManager().bindTexture(new ResourceLocation("textures/gui/container/generic_54.png")); drawTexturedModalRect(x, y, 0, 0, xSize, 35); drawTexturedModalRect(x, y + 35, 0, 126, xSize, 96); } } My container: package container.test; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.Slot; public class MyContainer extends Container { IInventory inventory; public MyContainer(InventoryPlayer playerInventory, IInventory inventory) { this.inventory = inventory; for (int i = 0; i < inventory.getSizeInventory(); i++) { addSlotToContainer(new Slot(inventory, i, 8 + i * 18, 18)); } for (int i = 0; i < 3; i++) { for (int j = 0; j < 9; j++) { addSlotToContainer(new Slot(playerInventory, j + i * 9 + 9, 8 + j * 18, 49 + i * 18)); } } for (int i = 0; i < 9; i++) { addSlotToContainer(new Slot(playerInventory, i, 8 + i * 18, 107)); } } @Override public boolean canInteractWith(EntityPlayer player) { return inventory.isUseableByPlayer(player); } } and a simple test mod implementing IGuiHandler: package container.test; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.IGuiHandler; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.registry.GameRegistry; @Mod(modid = "myMod", name = "myMod", version = "") public class MyMod implements IGuiHandler { MyItem item; @Instance("myMod") public static MyMod instance; @EventHandler public void preInit(FMLPreInitializationEvent event) { item = new MyItem(); item.setCreativeTab(CreativeTabs.tabMisc); GameRegistry.registerItem(item, "myMod"); NetworkRegistry.INSTANCE.registerGuiHandler(this, this); } @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { // TODO Auto-generated method stub return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { return new MyGui(player.inventory, item.inventory); } } I have tried implementing IInventory instead of using InventoryBasic - same result. I am running on Forge 1180. Any help appreciated!