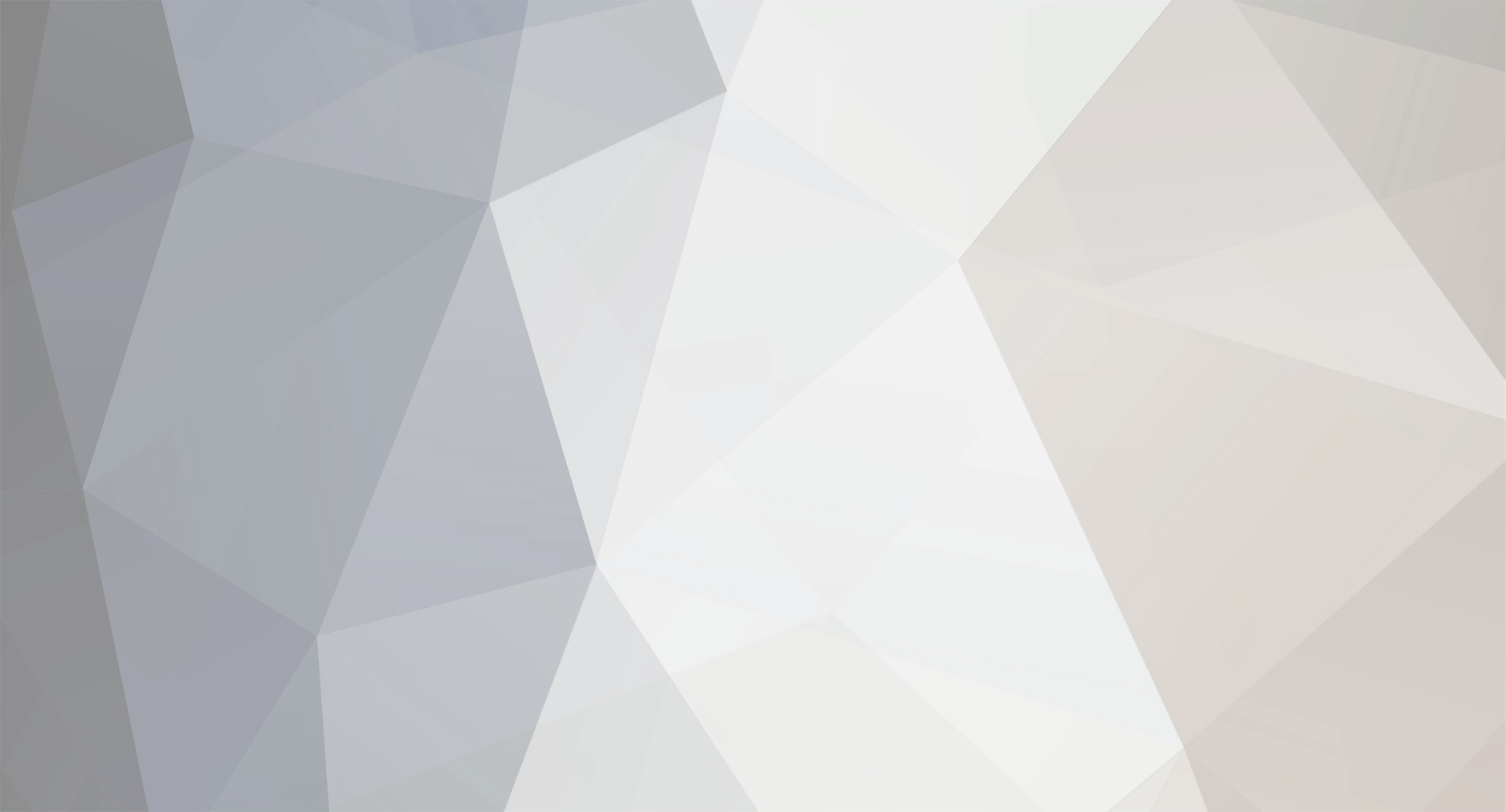
Roboguy99
Members-
Posts
230 -
Joined
-
Last visited
Everything posted by Roboguy99
-
Help with rendering depending on block state
Roboguy99 replied to Roboguy99's topic in Modder Support
Wow, NBT? This is more complicated than I expected. Thanks for the help though. I'll go away and have a go, and report back here if I don't succeed. How do I check on block updates if I'm working in the tile entity though (or do I just call the method from the block update method in the block class)? -
Help with rendering depending on block state
Roboguy99 replied to Roboguy99's topic in Modder Support
Ok I can sorta see what you're saying, but I'm slightly confused on how to migrate it. Could you point me in the right direction with that please? -
Sounds like what you need is 3 separate mods - a core mod with the shared content, a magic mod and a science mod. You'll be able to access content across mods by importing their packages. Have a look at this for help: http://www.minecraftforge.net/wiki/Developing_Addons_for_Existing_Mods
-
I've created a wire block, custom rendered in Techne. What I want for happen is for parts of the wire to show/hide depending on whether or not another wire is connected. This is working in theory and worked when I manually inputted connectivity states. Now I'm actually trying to get the data the game crashes on loading the world due to an uninitialized boolean array containing the connectivity states. If anyone could help me with getting the data from the block class to the rendering class that would be great. Block class package foodTech.blocks; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; import foodTech.tileEntities.TileEntityCable; public class BlockCable extends BlockContainer { /** * Contains 6 values for each face of a cable block * up, down, north, south, east, west */ public BlockCable(Material material) { super(material); float pixel = 1F/16F; this.setBlockBounds(12*pixel/2, 12*pixel/2, 12*pixel/2, 1-12*pixel/2, 1-12*pixel/2, 1-12*pixel/2); this.useNeighborBrightness = true; } public int getRenderType() { return -1; } public boolean isOpaqueCube() { return false; } public boolean renderAsNormalBlock() { return false; } public TileEntity createNewTileEntity(World world, int var2) { return new TileEntityCable(); } public void onNeighborBlockChange(World world, int x, int y, int z, Block block) { TileEntityCable.getConnectedWires(x, y, z, world); } public void updateTick(World world, int x, int y, int z, Random rand) { TileEntityCable.getConnectedWires(x, y, z, world); } public void onBlockAdded(World world, int x, int y, int z) { TileEntityCable.getConnectedWires(x, y, z, world); } } Rendering class package foodTech.tileEntities.render; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.entity.Entity; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; import foodTech.tileEntities.TileEntityCable; import foodTech.tileEntities.models.ModelCable; public class RenderCable extends TileEntitySpecialRenderer { ResourceLocation textureOff = (new ResourceLocation("roboguy99:textures/models/cableOff.png")); ResourceLocation textureOn = (new ResourceLocation("roboguy99:textures/models/cableOn.png")); public ModelCable modelCable; private boolean[] neighbourBlockWires = {false, false, false, false, false, false}; public RenderCable() { this.modelCable = new ModelCable(this.neighbourBlockWires); } public void renderTileEntityAt(TileEntity tileEntity, double x, double y, double z, float scale) { GL11.glPushMatrix(); GL11.glTranslatef((float) x + 0.5F, (float) y - 0.5F, (float) z + 0.5F); Minecraft.getMinecraft().renderEngine.bindTexture(textureOff); this.neighbourBlockWires = TileEntityCable.getAllConectionStates(); this.modelCable = new ModelCable(this.neighbourBlockWires); //Crashes here this.modelCable.render((Entity)null, 0.0F, 0.0F, -0.1F, 0.0F, 0.0F, 0.0625F); GL11.glTranslatef((float) x - 0.5F, (float) y + 0.5F, (float) z - 0.5F); GL11.glPopMatrix(); } } TileEntity class package foodTech.tileEntities; import foodTech.blocks.CreateBlocks; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; public class TileEntityCable extends TileEntity { private static boolean isWireAbove; private static boolean isWireBelow; private static boolean isWireNorth; private static boolean isWireSouth; private static boolean isWireEast; private static boolean isWireWest; private static boolean[] allConnectionStates; public static void getConnectedWires(int x, int y, int z, World world) { if (world.getBlock(x, y+1, z).equals(CreateBlocks.blockCable)) TileEntityCable.isWireAbove = true; if (world.getBlock(x, y-1, z).equals(CreateBlocks.blockCable)) TileEntityCable.isWireBelow = true; if (world.getBlock(x+1, y, z).equals(CreateBlocks.blockCable)) TileEntityCable.isWireNorth = true; if (world.getBlock(x-1, y, z).equals(CreateBlocks.blockCable)) TileEntityCable.isWireSouth = true; if (world.getBlock(x, y, z+1).equals(CreateBlocks.blockCable)) TileEntityCable.isWireEast = true; if (world.getBlock(x, y, z-1).equals(CreateBlocks.blockCable)) TileEntityCable.isWireWest = true; TileEntityCable.allConnectionStates[0] = TileEntityCable.isWireAbove; TileEntityCable.allConnectionStates[1] = TileEntityCable.isWireBelow; TileEntityCable.allConnectionStates[2] = TileEntityCable.isWireNorth; TileEntityCable.allConnectionStates[3] = TileEntityCable.isWireSouth; TileEntityCable.allConnectionStates[4] = TileEntityCable.isWireEast; TileEntityCable.allConnectionStates[5] = TileEntityCable.isWireWest; } public void readFromNBT(NBTTagCompound nbt) { super.readFromNBT(nbt); TileEntityCable.isWireAbove = nbt.getBoolean("isWireAbove"); TileEntityCable.isWireBelow = nbt.getBoolean("isWireBelow"); TileEntityCable.isWireNorth = nbt.getBoolean("isWireNorth"); TileEntityCable.isWireSouth = nbt.getBoolean("isWireSouth"); TileEntityCable.isWireEast = nbt.getBoolean("isWireEast"); TileEntityCable.isWireWest = nbt.getBoolean("isWireWest"); } public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); nbt.setBoolean("isWireAbove", isWireAbove); nbt.setBoolean("isWireBelow", isWireBelow); nbt.setBoolean("isWireNorth", isWireNorth); nbt.setBoolean("isWireSouth", isWireSouth); nbt.setBoolean("isWireEast", isWireEast); nbt.setBoolean("isWireWest", isWireWest); } public static boolean[] getAllConectionStates() { return TileEntityCable.allConnectionStates; } } If you need to see any more of my code I can upload it.
-
Editing a custom rendered block instance live?
Roboguy99 replied to Roboguy99's topic in Modder Support
Thanks. I'll test this out tomorrow and get back to you, as it's late now. -
net.minecraft.world.gen.structure.MapGenVillage.class
-
So I'm trying to make an electric cable system, which obviously requires an ability to connect/detach from other cables. Currently I am only working on the aesthetics and so want to change whether or not each part of a wire is shown. My wire model is made in Techne and consists of 7 parts (a core and up, down, north, south, east, west). They can be toggled currently when the model is first instanced using a boolean for each direction (I don't need to toggle the core): public RenderCable() { this.modelCable = new ModelCableAll(true, true, true, false, false, false); } The problem with this is that the state can only be changed by restarting the game, so how can I live update the state/edit the existing instance/remake the instance of the model? Here is the full class: package foodTech.tileEntities.render; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.entity.Entity; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ResourceLocation; import org.lwjgl.opengl.GL11; import foodTech.tileEntities.models.cable.ModelCableAll; public class RenderCable extends TileEntitySpecialRenderer { ResourceLocation textureOff = (new ResourceLocation("roboguy99:textures/models/cableOff.png")); ResourceLocation textureOn = (new ResourceLocation("roboguy99:textures/models/cableOn.png")); private final ModelCableAll modelCable; public RenderCable() { this.modelCable = new ModelCableAll(false, false, false, false, false, false); } public void renderTileEntityAt(TileEntity tileEntity, double x, double y, double z, float scale) { GL11.glTranslatef((float) x + 0.5F, (float) y - 0.5F, (float) z + 0.5F); setConnections(); GL11.glTranslatef((float) x - 0.5F, (float) y + 0.5F, (float) z - 0.5F); } public void setConnections() { GL11.glPushMatrix(); Minecraft.getMinecraft().renderEngine.bindTexture(textureOff); this.modelCable.render((Entity)null, 0.0F, 0.0F, -0.1F, 0.0F, 0.0F, 0.0625F); GL11.glPopMatrix(); } }
-
I would also like to know if anyone out there can help.
-
[SOLVED] Custom animations on Techne models?
Roboguy99 replied to Roboguy99's topic in Modder Support
Well that explains the stack overflow warnings it was producing. Thanks. -
[SOLVED] Custom animations on Techne models?
Roboguy99 replied to Roboguy99's topic in Modder Support
-
[SOLVED] Custom animations on Techne models?
Roboguy99 replied to Roboguy99's topic in Modder Support
It works, thanks! Perhaps you'd like to explain how my code was written very poorly so that I can improve in the future and correct my mistakes however? -
[SOLVED] Custom animations on Techne models?
Roboguy99 replied to Roboguy99's topic in Modder Support
Well, providing the rest of your sources that are concerned with the block so that i can actually run the mod and debug it will be a good idea. Ok sorry. I'm going to give you the link to the entire mod on dropbox because I'm in the middle of sorting out a lot of things and a huge amount of classes are currently needed for the VERY broken base upon which the windmill must sit. https://www.dropbox.com/sh/nzd6s6usysjri56/hhx8kivoWd To place the turbine you need to assemble a 3*3 area of turbine base blocks and you'll see it turn into a very brokenly rendered multiblock (I'm having troubles with this too). Hopefully you'll find everything you need. A quick pointer: I think the most important class here is tileEntities/render/RenderWindTurbine. Sorry again about the mess and thanks for the help. -
[SOLVED] Custom animations on Techne models?
Roboguy99 replied to Roboguy99's topic in Modder Support
I've been playing around with this code for a little bit, because as you can see in picture below it's not rendering at all correctly. I tried printing out time * mult and the number is indeed changing. The rotation does not however change more than once however. I tried changing the multiplier in debug mode which caused the turbine to move once and then stop again. Please help! It's probably worth noting that the top block of the turbine is rendered entirely wrong (textures are all over the place) -
[SOLVED] Custom animations on Techne models?
Roboguy99 replied to Roboguy99's topic in Modder Support
I've got the blade rendering in a seperate model alright, but I'm kinda stuck on the actual rotation part. I've tried to copy what you've done with no success. Here is my rendering class: -
Hi there, I've been working on a wind turbine and recently changed the way I was rendering it to a Techne model. I want to make the blades constantly spin on the wind turbine so that it looks significantly better. How would I animate it to constantly rotate? I assume it's a method somewhere. If anybody would be kind enough to help that would be very nice as any tutorials I found were two years old. If this helps, here is my wind turbine in game:
-
Hi there, I'm trying to make a machine which has 3 slots - 2 inputs and an output. One of the inputs is for anything, and the other is for cobblestone only. What I want is that when you place cobblestone into the bottom slot, it increases a variable and in turn increases a meter on the screen. How can I check for cobblestone in the slot? I have tried public void updateEntity() { if(this.getStackInSlot(2).equals(Blocks.cobblestone)); { System.out.println("I am awesome!"); } } with no luck. When I attempt to load a world I get a null pointer exception. P.S I know this is a bit off topic, but will help other issues I'm having with the GUI. How can I only let stone be placed in the slot, and how can I only allow items to be taken from the output slot and not placed?
-
(Solved)GUI handling causing game to crash with no crash report?
Roboguy99 replied to Roboguy99's topic in Modder Support
Sorry I'm sure I'm being an idiot here. Could you explain how please? I copied that piece of code from a tutorial video, and theirs worked fine. Okay, there are two kinds of loops that use while . One is: do BLOCK while (boolean-expression); (note the ';' after the keyword. The other is: while (boolean-expression) BLOCK; (notice the ';' after the body of the loop). In both cases BLOCK is usually an actualy block (' { statement; ... } '), but it may also be a single statement. Your while loop appears to be executing a null statement (';' alone is a null statement), and that is why the loop repeats forever. Oh my whatever the supreme being(s) may or may not be. In other words, I misplaced a semicolon on a block like I do so often. Thanks! -
(Solved)GUI handling causing game to crash with no crash report?
Roboguy99 replied to Roboguy99's topic in Modder Support
I thought that, but the buttons were disabled. I will make sure to look into the help for them, thanks. -
(Solved)GUI handling causing game to crash with no crash report?
Roboguy99 replied to Roboguy99's topic in Modder Support
Sorry I'm sure I'm being an idiot here. Could you explain how please? I copied that piece of code from a tutorial video, and theirs worked fine. -
(Solved)GUI handling causing game to crash with no crash report?
Roboguy99 replied to Roboguy99's topic in Modder Support
Anybody? Please? -
(Solved)GUI handling causing game to crash with no crash report?
Roboguy99 replied to Roboguy99's topic in Modder Support
Ok I tried that and all it did was paste AL lib: (EE) alc_cleanup: 1 device not closed to the console. What might be worth noting is that the game no longer freezes. In the method below, it prints "before", but "after" is never printed. public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int side, float hitX, float hitY, float hitZ) { if(!world.isRemote) { Main.print("before"); //Just a custom print method to put my mod name on there FMLNetworkHandler.openGui(player, Main.instance, GuiHandler.guiIDWindmill, world, x, y, z); Main.print("after"); return true; } return false; } -
(Solved)GUI handling causing game to crash with no crash report?
Roboguy99 replied to Roboguy99's topic in Modder Support
Could you explain a little further please? How would I stop it using the debugger (yes, I'm using Eclipse) -
After a little bit of testing (perhaps not enough) I've discovered that my server-side GUI handler is causing the game to freeze indefinitely. I have successfully managed to print to the console all the way through my class ContainerWindmilll and also in my class BlockWindmill, giving me the feeling that something somewhere else is affected. If anybody can locate the cause of this crash (no errors are shown, it's only when I right-click it crashes) I would be very grateful. EDIT: I've edited my code around a bit and now the FMLNetworkHandler.openGUI line from the BlockWindmill class seems to not freeze the game but freezes console output (perhaps freezes the thread?) Gui Handler: ContainerWindmill: TileEntityWindmill: BlockWindmill:
-
aup, you really need to downgrade. I did the same thing only to realise the same thing today. Because Java 8 is only just out, nothing has had time to update (like mods when a new version of Minecraft comes out). Make sure you have both JRE and JDK 7 and you set your path environment variable to point to it (there are a million guides of this, just search "JDK path environment variable" and repeat for JRE). Happy coding!