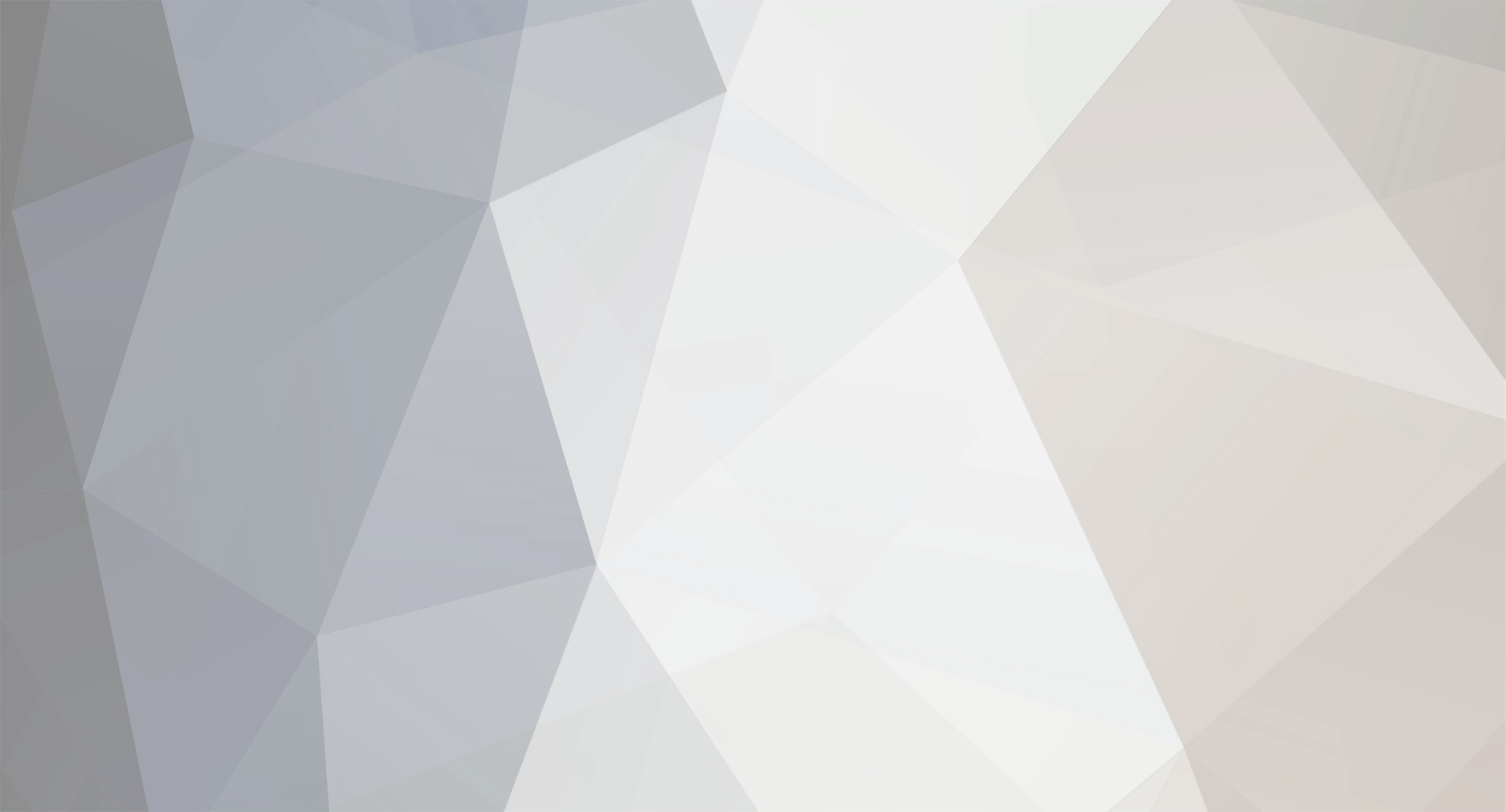
LazerBeans
-
Posts
48 -
Joined
-
Last visited
Posts posted by LazerBeans
-
-
Never mind. I was referencing registerRenderers() in CommonProxy, which did not have the static modifier. I added all static modifiers, and now errors. Now to test... The model renders. I need to look over the texture and model itself, as neither are as they should be (It should be easy enough).
-
No, you should call the method in the main class. This is basic java.
ClientProxy.registerRenderers();
Put it after you register the entity in preInit().
I've done so, but now ClientProxy and ArctiCraft are quarreling over .registerRenderers() being static or not. In ArctiCraft, I get an error that ClientProxy.registerRenderers() is not static, and even if I make it so in ClientProxy, I get an error in there that is telling me to remove the static modifier.
-
You don't call registerRenderers.
Should the code be :
public class ClientProxy extends CommonProxy { RenderingRegistry.registerEntityRenderingHandler(EntityPenguin.class, new RenderPenguin(new ModelPenguin(), 0.8F)); }
Instead of :
public class ClientProxy extends CommonProxy { public void registerRenderers() { RenderingRegistry.registerEntityRenderingHandler(EntityPenguin.class, new RenderPenguin(new ModelPenguin(), 0.8F)); } }
-
Check to see what's going on with the .dropItem() function. For each coin.
-
Hm... I seem to be just one step ahead of you. I used different methods and got no errors, but my Penguin only renders as a textureless white rectangular prism. Feel free to check out mine to see how to fix your errors. (It's the post right below!)
-
Hello all,
As I have been working on my Ores++ furnace for a while, and getting stuck on the TileEntity, I decided to create a side project to divert myself. It will be another module for my MC++(To Be Renamed) mod, Exploration++. Enough of the back story.
I used Techne to create a model for a penguin, and created the texture and ModelPenguin java files. As I am new to mobs, I did a bunch of copy/paste, simply to make my penguin more chicken-like.
The penguin spawns naturally, plays the correct sound, and can create a baby, but when it renders, it's just a vertical, white rectangular prism. Neither the model nor textures work.
You can look through what I have so far for mob-related code below. What did I do wrong?
EDIT: Might as well just Copy/Paste the code here! And not have you go through the hassle of .java files...
Main Mod
package com.LazerBeanz.LazerCraft.ArctiCraft; import ArctiCraftMob.EntityPenguin; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityList; import net.minecraft.entity.EnumCreatureType; import net.minecraft.item.Item; import net.minecraft.world.biome.BiomeGenBase; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.registry.EntityRegistry; @Mod(modid = "ArctiCraft Addon", version = "1.7.10-1.0.0") public class ArctiCraft { @Instance(value = "ArctiCraftModID") public static ArctiCraft instance; @SidedProxy(clientSide="com.LazerBeanz.LazerCraft.ArctiCraft.client.ClientProxy", serverSide="com.LazerBeanz.LazerCraft.ArctiCraft.CommonProxy") public static CommonProxy proxy; public static Item penguinBeak = new BeakPenguin().setUnlocalizedName("penguinbeak").setTextureName("ArctiCraft:beakPenguin"); @EventHandler public void preInit(FMLPreInitializationEvent event) { EntityRegistry.registerGlobalEntityID(EntityPenguin.class, "arcticpenguin", EntityRegistry.findGlobalUniqueEntityId(), 0x000000, 0xFFFFFF); EntityRegistry.addSpawn(EntityPenguin.class, 10, 3, 6, EnumCreatureType.creature, BiomeGenBase.icePlains); } @EventHandler public void load(FMLInitializationEvent event) { } @EventHandler public void postInit(FMLPostInitializationEvent event) { } }
EntityPenguin:
package ArctiCraftMob; import com.LazerBeanz.LazerCraft.ArctiCraft.ArctiCraft; import net.minecraft.block.Block; import net.minecraft.entity.EntityAgeable; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.EntityAIFollowParent; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAIMate; import net.minecraft.entity.ai.EntityAIPanic; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAITempt; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.passive.EntityAnimal; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemFishFood; import net.minecraft.item.ItemSeeds; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class EntityPenguin extends EntityAnimal { public float field_70886_e; public float destPos; public float field_70884_g; public float field_70888_h; public float field_70889_i = 1.0F; public boolean field_152118_bv; private static final String __OBFID = "CL_00001639"; public EntityPenguin(World p_i1682_1_) { super(p_i1682_1_); this.setSize(0.3F, 0.7F); this.tasks.addTask(0, new EntityAISwimming(this)); this.tasks.addTask(1, new EntityAIPanic(this, 1.4D)); this.tasks.addTask(2, new EntityAIMate(this, 1.0D)); this.tasks.addTask(3, new EntityAITempt(this, 1.0D, Items.wheat_seeds, false)); this.tasks.addTask(4, new EntityAIFollowParent(this, 1.1D)); this.tasks.addTask(5, new EntityAIWander(this, 1.0D)); this.tasks.addTask(6, new EntityAIWatchClosest(this, EntityPlayer.class, 6.0F)); this.tasks.addTask(7, new EntityAILookIdle(this)); } /** * Returns true if the newer Entity AI code should be run */ public boolean isAIEnabled() { return true; } protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(4.0D); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.25D); } /** * Called frequently so the entity can update its state every tick as required. For example, zombies and skeletons * use this to react to sunlight and start to burn. */ public void onLivingUpdate() { super.onLivingUpdate(); this.field_70888_h = this.field_70886_e; this.field_70884_g = this.destPos; this.destPos = (float)((double)this.destPos + (double)(this.onGround ? -1 : 4) * 0.3D); if (this.destPos < 0.0F) { this.destPos = 0.0F; } if (this.destPos > 1.0F) { this.destPos = 1.0F; } if (!this.onGround && this.field_70889_i < 1.0F) { this.field_70889_i = 1.0F; } this.field_70889_i = (float)((double)this.field_70889_i * 0.9D); if (!this.onGround && this.motionY < 0.0D) { this.motionY *= 0.6D; } this.field_70886_e += this.field_70889_i * 2.0F; } /** * Returns the sound this mob makes while it's alive. */ protected String getLivingSound() { return "mob.chicken.say"; } /** * Returns the sound this mob makes when it is hurt. */ protected String getHurtSound() { return "mob.chicken.hurt"; } /** * Returns the sound this mob makes on death. */ protected String getDeathSound() { return "mob.chicken.hurt"; } protected void func_145780_a(int p_145780_1_, int p_145780_2_, int p_145780_3_, Block p_145780_4_) { this.playSound("mob.chicken.step", 0.15F, 1.0F); } protected Item getDropItem() { return Items.feather; } /** * Drop 0-2 items of this living's type. @param par1 - Whether this entity has recently been hit by a player. @param * par2 - Level of Looting used to kill this mob. */ protected void dropFewItems(boolean p_70628_1_, int p_70628_2_) { int j = this.rand.nextInt(3) + this.rand.nextInt(1 + p_70628_2_); for (int k = 0; k < j; ++k) { this.dropItem(Items.feather, 1); } if (this.isBurning()) { this.dropItem(Items.cooked_chicken, 1); } else { this.dropItem(ArctiCraft.penguinBeak, 1); } } public EntityPenguin createChild(EntityAgeable p_90011_1_) { return new EntityPenguin(this.worldObj); } /** * Checks if the parameter is an item which this animal can be fed to breed it (wheat, carrots or seeds depending on * the animal type) */ public boolean isBreedingItem(ItemStack p_70877_1_) { return p_70877_1_ != null && p_70877_1_.getItem() instanceof ItemFishFood; } /** * Get the experience points the entity currently has. */ protected int getExperiencePoints(EntityPlayer p_70693_1_) { return this.func_152116_bZ() ? 10 : super.getExperiencePoints(p_70693_1_); } /** * Determines if an entity can be despawned, used on idle far away entities */ protected boolean canDespawn() { return this.func_152116_bZ() && this.riddenByEntity == null; } public boolean func_152116_bZ() { return this.field_152118_bv; } public void func_152117_i(boolean p_152117_1_) { this.field_152118_bv = p_152117_1_; } }
ModelPenguin:
package ArctiCraftMob; import org.lwjgl.opengl.GL11; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; import net.minecraft.util.MathHelper; public class ModelPenguin extends ModelBase { //fields ModelRenderer Leg1; ModelRenderer Leg2; ModelRenderer Body; ModelRenderer Arm1; ModelRenderer Arm2; ModelRenderer Head; ModelRenderer Foot1; ModelRenderer Foot2; ModelRenderer Shape1; public ModelPenguin() { textureWidth = 64; textureHeight = 32; Leg1 = new ModelRenderer(this, 42, 12); Leg1.addBox(0F, 0F, 0F, 1, 7, 1); Leg1.setRotationPoint(0F, 17F, -2F); Leg1.setTextureSize(64, 32); Leg1.mirror = true; setRotation(Leg1, 0F, 0F, 0F); Leg2 = new ModelRenderer(this, 42, 12); Leg2.addBox(0F, 0F, 0F, 1, 7, 1); Leg2.setRotationPoint(0F, 17F, 1F); Leg2.setTextureSize(64, 32); Leg2.mirror = true; setRotation(Leg2, 0F, 0F, 0F); Body = new ModelRenderer(this, 46, 12); Body.addBox(0F, 0F, 0F, 3, 11, 6); Body.setRotationPoint(-1F, 6F, -3F); Body.setTextureSize(64, 32); Body.mirror = true; setRotation(Body, 0F, 0F, 0F); Arm1 = new ModelRenderer(this, 32, 0); Arm1.addBox(0F, 0F, 0F, 3, 11, 1); Arm1.setRotationPoint(2F, 6F, -3F); Arm1.setTextureSize(64, 32); Arm1.mirror = true; setRotation(Arm1, 0.2617994F, 3.141593F, 0F); Arm2 = new ModelRenderer(this, 32, 0); Arm2.addBox(0F, 0F, 0F, 3, 11, 1); Arm2.setRotationPoint(-1F, 6F, 3F); Arm2.setTextureSize(64, 32); Arm2.mirror = true; setRotation(Arm2, 0.2617994F, 0F, 0F); Head = new ModelRenderer(this, 42, 0); Head.addBox(0F, -2F, -3F, 5, 6, 6); Head.setTextureOffset(24, 0).addBox(3F, 0F, 0F, 2, 1, 2); //beak Head.setRotationPoint(-2F, 2F, 0F); Head.setTextureSize(64, 32); Head.mirror = true; setRotation(Head, 0F, 0F, 0F); Foot1 = new ModelRenderer(this, 36, 26); Foot1.addBox(0F, 0F, -3F, 2, 0, 3); Foot1.setRotationPoint(0F, 24F, 0F); Foot1.setTextureSize(64, 32); Foot1.mirror = true; setRotation(Foot1, 0F, 0F, 0F); Foot2 = new ModelRenderer(this, 36, 26); Foot2.addBox(0F, 0F, 0F, 2, 0, 3); Foot2.setRotationPoint(0F, 24F, 0F); Foot2.setTextureSize(64, 32); Foot2.mirror = true; setRotation(Foot2, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); if (this.isChild) { float f6 = 2.0F; GL11.glPushMatrix(); GL11.glTranslatef(0.0F, 5.0F * f5, 2.0F * f5); this.Head.renderWithRotation(f5); GL11.glPopMatrix(); GL11.glPushMatrix(); GL11.glScalef(1.0F / f6, 1.0F / f6, 1.0F / f6); GL11.glTranslatef(0.0F, 24.0F * f5, 0.0F); this.Body.render(f5); this.Leg1.render(f5); this.Leg2.render(f5); this.Arm1.render(f5); this.Arm2.render(f5); this.Foot1.render(f5); this.Foot2.render(f5); GL11.glPopMatrix(); } else { this.Leg1.render(f5); this.Leg2.render(f5); this.Body.render(f5); this.Arm1.render(f5); this.Arm2.render(f5); this.Head.renderWithRotation(f5); this.Foot1.render(f5); this.Foot2.render(f5); } } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float par1, float par2, float par3, float par4, float par5, float par6, Entity par7) { float f6 = (180F / (float)Math.PI); this.Head.rotateAngleX = par5 / (180F / (float)Math.PI); this.Head.rotateAngleY = par4 / (180F / (float)Math.PI); this.Body.rotateAngleX = ((float)Math.PI / 2F); this.Leg1.rotateAngleX = MathHelper.cos(par1 * 0.6662F) * 1.4F * par2; this.Leg2.rotateAngleX = MathHelper.cos(par1 * 0.6662F + (float)Math.PI) * 1.4F * par2; this.Arm1.rotateAngleX = MathHelper.cos(par1 * 0.6662F) * 1.4F *par2; this.Arm2.rotateAngleX = MathHelper.cos(par1 * 0.6662F + (float)Math.PI) * 1.4F * par2; } }
RenderPenguin:
package ArctiCraftMob; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.client.model.ModelBase; import net.minecraft.client.renderer.entity.RenderLiving; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EntityLivingBase; import ArctiCraftMob.EntityPenguin; import net.minecraft.util.MathHelper; import net.minecraft.util.ResourceLocation; @SideOnly(Side.CLIENT) public class RenderPenguin extends RenderLiving { private static final ResourceLocation penguinTextures = new ResourceLocation("textures/entity/arcticpenguin.png"); private static final String __OBFID = "CL_00000983"; public RenderPenguin(ModelBase p_i1252_1_, float p_i1252_2_) { super(p_i1252_1_, p_i1252_2_); } /** * Actually renders the given argument. This is a synthetic bridge method, always casting down its argument and then * handing it off to a worker function which does the actual work. In all probability, the class Render is generic * (Render<T extends Entity) and this method has signature public void func_76986_a(T entity, double d, double d1, * double d2, float f, float f1). But JAD is pre 1.5 so doesn't do that. */ public void doRender(EntityPenguin p_76986_1_, double p_76986_2_, double p_76986_4_, double p_76986_6_, float p_76986_8_, float p_76986_9_) { super.doRender((EntityLiving)p_76986_1_, p_76986_2_, p_76986_4_, p_76986_6_, p_76986_8_, p_76986_9_); } /** * Returns the location of an entity's texture. Doesn't seem to be called unless you call Render.bindEntityTexture. */ protected ResourceLocation getEntityTexture(EntityPenguin p_110775_1_) { return penguinTextures; } /** * Defines what float the third param in setRotationAngles of ModelBase is */ protected float handleRotationFloat(EntityPenguin p_77044_1_, float p_77044_2_) { float f1 = p_77044_1_.field_70888_h + (p_77044_1_.field_70886_e - p_77044_1_.field_70888_h) * p_77044_2_; float f2 = p_77044_1_.field_70884_g + (p_77044_1_.destPos - p_77044_1_.field_70884_g) * p_77044_2_; return (MathHelper.sin(f1) + 1.0F) * f2; } /** * Actually renders the given argument. This is a synthetic bridge method, always casting down its argument and then * handing it off to a worker function which does the actual work. In all probabilty, the class Render is generic * (Render<T extends Entity) and this method has signature public void func_76986_a(T entity, double d, double d1, * double d2, float f, float f1). But JAD is pre 1.5 so doesn't do that. */ public void doRender(EntityLiving p_76986_1_, double p_76986_2_, double p_76986_4_, double p_76986_6_, float p_76986_8_, float p_76986_9_) { this.doRender((EntityPenguin)p_76986_1_, p_76986_2_, p_76986_4_, p_76986_6_, p_76986_8_, p_76986_9_); } /** * Defines what float the third param in setRotationAngles of ModelBase is */ protected float handleRotationFloat(EntityLivingBase p_77044_1_, float p_77044_2_) { return this.handleRotationFloat((EntityPenguin)p_77044_1_, p_77044_2_); } /** * Actually renders the given argument. This is a synthetic bridge method, always casting down its argument and then * handing it off to a worker function which does the actual work. In all probabilty, the class Render is generic * (Render<T extends Entity) and this method has signature public void func_76986_a(T entity, double d, double d1, * double d2, float f, float f1). But JAD is pre 1.5 so doesn't do that. */ public void doRender(EntityLivingBase p_76986_1_, double p_76986_2_, double p_76986_4_, double p_76986_6_, float p_76986_8_, float p_76986_9_) { this.doRender((EntityPenguin)p_76986_1_, p_76986_2_, p_76986_4_, p_76986_6_, p_76986_8_, p_76986_9_); } /** * Returns the location of an entity's texture. Doesn't seem to be called unless you call Render.bindEntityTexture. */ protected ResourceLocation getEntityTexture(Entity p_110775_1_) { return this.getEntityTexture((EntityPenguin)p_110775_1_); } /** * Actually renders the given argument. This is a synthetic bridge method, always casting down its argument and then * handing it off to a worker function which does the actual work. In all probabilty, the class Render is generic * (Render<T extends Entity) and this method has signature public void func_76986_a(T entity, double d, double d1, * double d2, float f, float f1). But JAD is pre 1.5 so doesn't do that. */ public void doRender(Entity p_76986_1_, double p_76986_2_, double p_76986_4_, double p_76986_6_, float p_76986_8_, float p_76986_9_) { this.doRender((EntityPenguin)p_76986_1_, p_76986_2_, p_76986_4_, p_76986_6_, p_76986_8_, p_76986_9_); } }
ClientProxy:
package com.LazerBeanz.LazerCraft.ArctiCraft.client; import net.minecraftforge.client.MinecraftForgeClient; import ArctiCraftMob.EntityPenguin; import ArctiCraftMob.ModelPenguin; import ArctiCraftMob.RenderPenguin; import com.LazerBeanz.LazerCraft.ArctiCraft.CommonProxy; import cpw.mods.fml.client.registry.RenderingRegistry; public class ClientProxy extends CommonProxy { public void registerRenderers() { RenderingRegistry.registerEntityRenderingHandler(EntityPenguin.class, new RenderPenguin(new ModelPenguin(), 0.8F)); } }
Apologies for copy/paste, I'm new to this!
-
Is the block name set to .setBlockName(oreCopper), ore .setBlockName("oreCopper")?
Make sure that the parameter is a string.
-
What is your .setBlockName(String) set to?
If it's set to "copperOre", then the name should work. If it's set to "oreCopper", it won't. Your .setBlockName(String) needs to be equivalent to what is in the lang file. Check that to make sure it works (or not).
I was able to use '§' to change the coloring of an Item, Block, and Creative Tab, so it isn't a problem with the '§'.
-
How might one access the weights for the biome?
-
I don't know how, but the wrong assets were in the resources. I rebuilt the jar and installed, runs perfectly. Thanks!
-
When I was reading tutorials, I was instructed to put the assets in src/main/java. Not a good idea?
-
-
Okay, there were some folders in my mods folder (not sure why, they were supposed to be moved out). The game no longer crashes, but now the latest jar doesn't have ANY assets loaded.
All of the assets are in src/main/resources...
-
The jar was in build/libs. I pasted into my ~/Library/Application Support/minecraft/mods folder. There are multiple mods because I created a test mod for getting to know Eclipse and the project setup. These assets actually DID work, as they were in the correct folder(s). And what do you mean by loaded multiple times? I
-
The reason I used a command was to repack the jar, although I found a way to edit the jar without unarchiving/recompressing (but it stills crashes).
I see. I didn't have the assets in the src/main/resources folder, but in the src/main/java...
When I test run Minecraft through eclipse, it had always loaded fine.
I just rebuilt the jar and pasted it in my mods folder. The crash report is different, although it still crashes. The previous time it would crash during preinitialization. This time, it crashed during initialization, providing me with this:
Time: 8/3/14 12:12 PM Description: Initializing game java.lang.NullPointerException: Initializing game at cpw.mods.fml.common.Loader.preinitializeMods(Loader.java:506) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:239) at net.minecraft.client.Minecraft.func_71384_a(Minecraft.java:467) at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:815) at net.minecraft.client.main.Main.main(SourceFile:103) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) at java.lang.reflect.Method.invoke(Method.java:597) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at cpw.mods.fml.common.Loader.preinitializeMods(Loader.java:506) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:239) at net.minecraft.client.Minecraft.func_71384_a(Minecraft.java:467) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:815) at net.minecraft.client.main.Main.main(SourceFile:103) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) at java.lang.reflect.Method.invoke(Method.java:597) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) -- System Details -- Details: Minecraft Version: 1.7.2 Operating System: Mac OS X (x86_64) version 10.9.4 Java Version: 1.6.0_65, Apple Inc. Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Apple Inc. Memory: 139972888 bytes (133 MB) / 232198144 bytes (221 MB) up to 1060372480 bytes (1011 MB) JVM Flags: 5 total; -Xmx1G -XX:+UseConcMarkSweepGC -XX:+CMSIncrementalMode -XX:-UseAdaptiveSizePolicy -Xmn128M AABB Pool Size: 0 (0 bytes; 0 MB) allocated, 0 (0 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v9.03 FML v7.2.211.1121 Minecraft Forge 10.12.2.1121 9 mods loaded, 0 mods active mcp{9.03} [Minecraft Coder Pack] (minecraft.jar) FML{7.2.211.1121} [Forge Mod Loader] (forge-1.7.2-10.12.2.1121.jar) Forge{10.12.2.1121} [Minecraft Forge] (forge-1.7.2-10.12.2.1121.jar) examplemod{1.0} [Example Mod] (Ores+-1.0.jar) Emeralds+{1.7.2} [Emeralds+] (Ores+-1.0.jar) basic{1.0.2} [basic] (Ores+-1.0.jar) Emeralds+{1.7.2} [Emeralds+] (OresPlus Stuff) examplemod{1.0} [examplemod] (OresPlus Stuff) basic{1.0.2} [basic] (OresPlus Stuff) Launched Version: 1.7.2-Forge10.12.2.1121 LWJGL: 2.9.1 OpenGL: ATI Radeon HD 4670 OpenGL Engine GL version 2.1 ATI-8.24.15, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [Arcanumcraft] Current Language: English (UK) Profiler Position: N/A (disabled) Vec3 Pool Size: ~~ERROR~~ NullPointerException: null Anisotropic Filtering: Off (1)
-
To export my jar file from my Forge environment: ./gradlew build
To export my jar file from folder to .jar:
jar cf OresPlus-1.7.2-1.0 OresPlus-1.0.jar
Perhaps the name change was what tripped up?
My project setup (In the Jar):
|assets
|emeraldsplus
|lang
-en_US.lang
|textures
|blocks
|items
-flimsyEmeraldAxe.png
-flimsyEmeraldHoe.png
-flimsyEmeraldPickaxe.png
-flimsyEmeraldShovel.png
-flimsyEmeraldSword.png
-sturdyEmerald.png
-sturdyEmeraldAxe.png
-sturdyEmeraldHoe.png
-sturdyEmeraldPickaxe.png
-sturdyEmeraldShovel.png
-sturdyEmeraldSword.png
|com
|LazerBeans
|EmeraldsPlus
|EmeraldsPlus.class
|*.class files for each of the above textured items*
-mcmod.info
|META-INF
-MANIFEST.MF
My Forge environment is the same regarding the assets.
-
Running Mac OS X 10.9.4
(Take it easy, I'm a beginner!)
When I exported my mod, I got the jar file as expected. I pasted it into my game, and both the names and textures were missing! I unarchived the .jar and opened up the folder, only to find out that for some reason none of the assets exported. I pasted the correct assets and the folder hierarchies, then I renamed the folder to be a .jar (just as a test), and Minecraft Forge ran it perfectly (regardless of 1.7.2 or 1.7.10), the textures working. I then used Terminal to turn the .jar folder into a compressed jar, but when I did this, it crashed Minecraft each and every time I tried, with naming conventions or otherwise.
Can anybody explain why the assets didn't register in the first place, or why the compressed jar isn't working?
-
-
Should I replace the version with the version of Forge?
-
First of all: "Using MCP"? No, you don't use MCP directly anymore.
Post your build.gradle
Here's the build.gradle:
buildscript { repositories { mavenCentral() maven { name = "forge" url = "http://files.minecraftforge.net/maven" } maven { name = "sonatype" url = "https://oss.sonatype.org/content/repositories/snapshots/" } } dependencies { classpath 'net.minecraftforge.gradle:ForgeGradle:1.2-SNAPSHOT' } } apply plugin: 'forge' version = "1.0" group= "com.LazerBeans.EmeraldsPlus" // http://maven.apache.org/guides/mini/guide-naming-conventions.html archivesBaseName = "Ores+" minecraft { version = “1.7.2-1.0” assetDir = "eclipse/assets" } dependencies { // you may put jars on which you depend on in ./libs // or you may define them like so.. //compile "some.group:artifact:version:classifier" //compile "some.group:artifact:version" // real examples //compile 'com.mod-buildcraft:buildcraft:6.0.8:dev' // adds buildcraft to the dev env //compile 'com.googlecode.efficient-java-matrix-library:ejml:0.24' // adds ejml to the dev env // for more info... // http://www.gradle.org/docs/current/userguide/artifact_dependencies_tutorial.html // http://www.gradle.org/docs/current/userguide/dependency_management.html } processResources { // this will ensure that this task is redone when the versions change. inputs.property "version", project.version inputs.property "mcversion", project.minecraft.version // replace stuff in mcmod.info, nothing else from(sourceSets.main.resources.srcDirs) { include 'mcmod.info' // replace version and mcversion expand 'version':project.version, 'mcversion':project.minecraft.version } // copy everything else, thats not the mcmod.info from(sourceSets.main.resources.srcDirs) { exclude 'mcmod.info' } }
And what do you mean we don't use MCP anymore? What should I be using?
-
--SOLVED--SOLVED--SOLVED--
Before I get into anything, I hope this info helps:
Running Mac OS X 10.9.4
Using MCP for 1.7.2
(Go easy on me, I'm a beginner!)
When I attempt to use './gradlew build' to compile my mod, I get the following error:
The assetDir is deprecated! Use runDir instead! runDir set to eclipse/assets/.. **************************** Powered By MCP: http://mcp.ocean-labs.de/ Searge, ProfMobius, Fesh0r, R4wk, ZeuX, IngisKahn MCP Data version : unknown **************************** FAILURE: Build failed with an exception. * What went wrong: A problem occurred configuring root project 'forge'. > ForgeGradle 1.2 only works for Forge versions 10.12.0.1048+ * Try: Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output. BUILD FAILED
I went into build.gradle and changed the classpath to 'net.minecraftforge.gradle:ForgeGradle:1.1-SNAPSHOT', and got this error:
FAILURE: Build failed with an exception. * Where: Build file '/Users/Noah/Desktop/Test For Curriculum/MCP/forge/build.gradle' line: 25 * What went wrong: A problem occurred evaluating root project 'forge'. > ForgeGradle 1.1 will only work for Minecraft 1.7.2 * Try: Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output. BUILD FAILED
I can't seem to find anything that helps.
If you need any additional information, I can provide it.
Thanks!
[1.7.10] Penguin walking sideways?
in Modder Support
Posted
First of all, a huge thank you to Eternaldoom for helping out with the rendering. Unfortunately, my penguin doesn't quite walk properly, and I can't figure out why. I built the model in Techne, but for some reason I cannot find it and reopen it. http://minecraftforge.net/forum/Smileys/default/undecided.gif My penguin animates properly (aside from the head, I need to fix that), but it walks sideways... Can anyone help?
Here is the ModelPenguin file, if you need more I can help.
Thanks in advance.