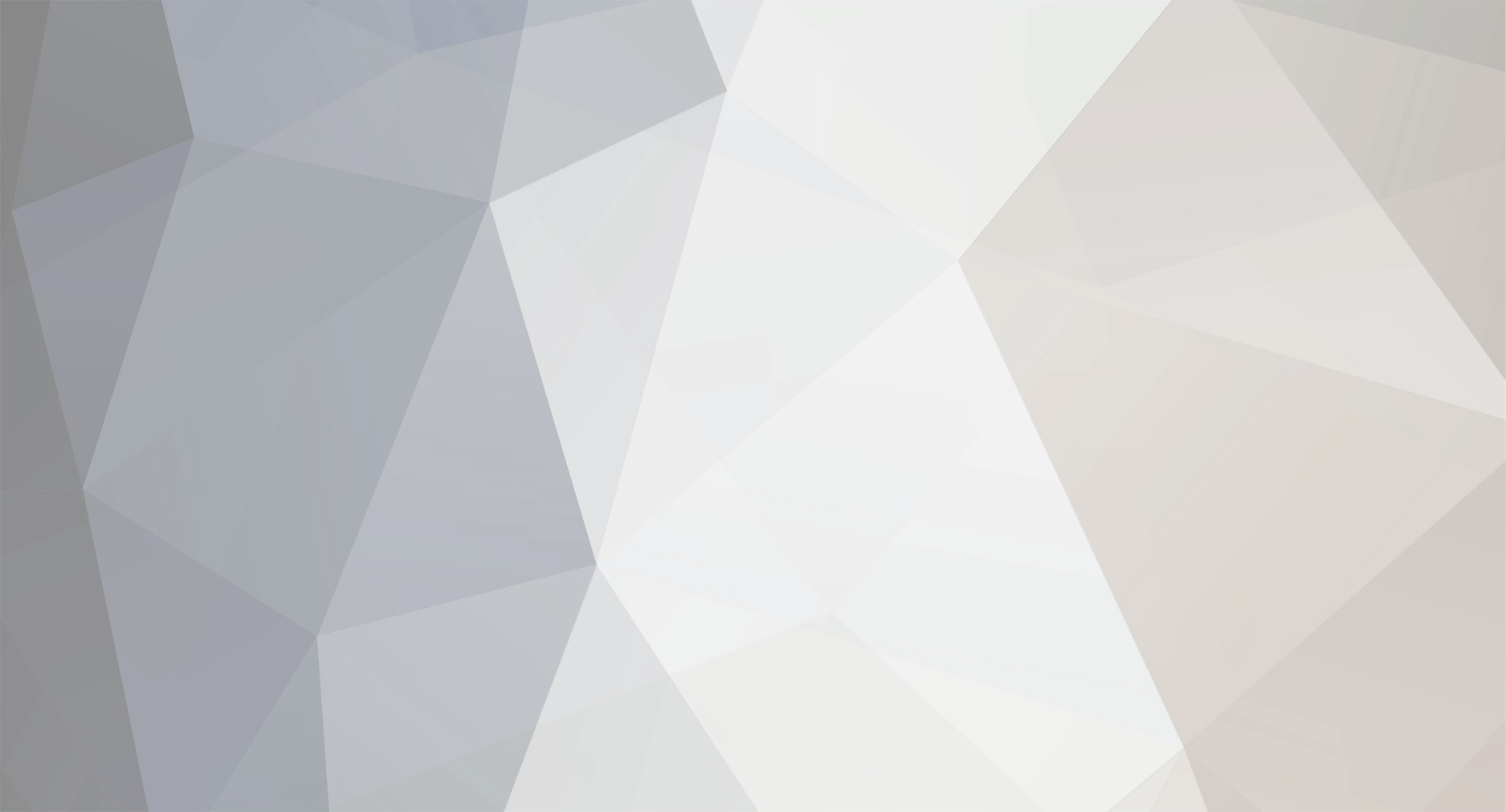
Cerandior
Members-
Posts
385 -
Joined
-
Last visited
Everything posted by Cerandior
-
I can't seem to find a way to do this. I wanted to spawn a zombie when you right click the item, but I don't know how can I get an instance of a ZombieEntity to spawn in world I tried this: ZombieEntity zombie = new ZombieEntity(worldIn); But my IDE won't let me use this. I checked the ZombieEntity class and all it takes in its constructor is the world, so I am not quite sure why I can't do this. Thank you.
-
The closest thing that I have found about offset is: @Override public double getYOffset() { return super.getYOffset(); } I am not quite sure what that does though, but it doesn't fix my problem. Tried several values but my entity still got his feet inside the ground. EDIT: Solved by manually adding offset to each element of my model, wish there was another way to offset the whole thing in a certain direction but oh well.
-
Ok, getting somewhere. The bounding box can be changed by using getSize().scale @Override public EntitySize getSize(Pose poseIn) { return super.getSize(poseIn).scale(1.5F); } Checked in-game and the bounding box's size is correct, however my Entity's legs are still below ground. The entire model needs to be offset by my scaling amount in the Y direction. How can I do this?
-
I tried it using getBoundingBox() and returning a new AxisAlignedBB, but it still despawns my entity. @Override public AxisAlignedBB getBoundingBox() { return new AxisAlignedBB(0.9, 2.985, 0.9, 0.9, 2.985, 0.9); } Any idea why .setSize is no longer there in 1.14? It was convenient and it has been available in the past versions of forge. EDIT: Found this in MobEntity Class: public boolean canSpawn(IWorld worldIn, SpawnReason spawnReasonIn) { return this.getBlockPathWeight(new BlockPos(this.posX, this.getBoundingBox().minY, this.posZ), worldIn) >= 0.0F; } It is probably blocking my entity for spawning because the bounding box is entering the block below (?). Can there be an offset applied for this?
-
Ok, I did what I said above and it is working now. I am also having trouble with something else. I tried to make the SkeletonKing a little bigger than the other skeletons. I tried doing this using the scale variable in the render method of the Model class, and the model is indeed scaled correctly. But the entity size does not correspond to that of the model so his feet go below the ground. I tried looking for something related to size or height and width, but I did not find anything. Is there a way to change my entity's bounding box?
-
Thank you for your answer. I should have looked a little more before wasting other people's time (sorry). I tried creating the custom bow and made my entity equip it in his main hand, but unfortunately the entity is no longer able to shoot arrows. I am almost certain it has to do with the goal. I saw this piece of code: public boolean shouldExecute() { return this.entity.getAttackTarget() == null ? false : this.isBowInMainhand(); } And isBowInMainHand(): protected boolean isBowInMainhand() { net.minecraft.item.ItemStack main = this.entity.getHeldItemMainhand(); net.minecraft.item.ItemStack off = this.entity.getHeldItemOffhand(); return main.getItem() instanceof BowItem || off.getItem() instanceof BowItem; } It is hard-coded to execute the goal only if the entity is carrying an instance of BowItem in his hand. However my custom bow does not extend BowItem because I wanted to add some custom behaviors to it. Is my only option here to create my own EntityGoal extending RangedBowAttackGoal and overriding shouldExecute to work for my custom bow? Thank you again.
-
I looked at the vanilla Skeleton entity and I got something to work, but I can't seem to make it shoot as fast as I want to. Even if I set the attackCooldown to 0 there is still a significant pause between attacks. This is what I tried: https://github.com/Cerandior/VanillaExtended/blob/master/src/main/java/teabx/vanillaextended/entities/SkeletonKing.java Is there a way to make the guy shoot a little faster? Also, I am not sure if this is the most efficient way since I am adding a new goal to the entity every tick. Thank you.
-
I have created a monster that is quite like a skeleton, except that it has some special abilities. I wanted him to have a "frenzy mode" so that if his hp drops below 20%, it starts shooting arrows crazy fast. As far as I am aware, the attack time of a ranged mob is handled by the maxAttackTime (40 in this case) value of this goal: this.goalSelector.addGoal(1, new RangedAttackGoal(this, (double)0.3F, 40, 20.0F)); Is it possible to change this value after the goal has been registered? I tried re-applying the goal on the livingTick method once the health had dropped, but it had no effect.
-
No, if you are going to register it using the EVENT_BUS, there is no need for the annotation.
-
I tried doing something with events lately myself and for some reason my Events Class was not properly registered when using the annotation. Try registering it in your main mod class's constructor (the one with @Mod annotation) using: MinecraftForge.EVENT_BUS.register(YourEventClassName.class); Please keep in mind that if you pass the class itself in the code above (like I have done), all your @SubscribeEvent methods must be static.
-
I am trying to make my own bow, but I don't want it to handle like the Vanilla bow would. I tried doing it by extending ShootableItem in my item class and did some quick stuff to see if the bow would shoot arrows and it worked. However, I am confused on how can I make my bow use the pulling stages textures. Checked the Vanilla BowItem json file and copied it for my own and as far as I understand the change of textures is based on two variables. "pulling" and "pull" However I am not quite sure how do I set those variables in my item class. I tried looking at the Vanilla BowItem class for "inspiration', but I don't quite understand what is going on because a lot of variables are obfuscated. Perhaps something to do with the version of forge I am using. To be fair, even if they weren't obfuscated, I probably still wouldn't understand how it works. Could someone please explain to me what is going on? I never worked with such things before. Here is the Vanilla Bow (just the constructor in case it is copyrighted): https://gist.github.com/Cerandior/525f0bbcd99961ceaf0c2539b117bc44 Thank you for your help.
-
[1.14.4] Multiple problems with custom entity
Cerandior replied to Cerandior's topic in Modder Support
Thank you for your answer. It seems I had underestimated the value I had set to the "speed" variable, I tried something smaller and the entity is behaving correctly. About the second point. This may pose a problem to me for another thing. I was intending to create a new boss which would spawn when the player entered a certain structure. You said that it seems impossible to do custom spawning mechanics at the moment. Is that true only for "natural' world spawns, or includes this scenario as well? On an unrelated note. I was trying to create some custom Dispenser Behaviours for some certain items and I am not quite sure where I am supposed to register my Behaviours. I registered them under the FMLClientSetupEvent, but I am not sure if it is the right place to do this. It caused no problems when I tested it, but maybe it's in the wrong spot in the hierarchy of registrations and may cause problems with other mods. -
[1.14.4] Multiple problems with custom entity
Cerandior replied to Cerandior's topic in Modder Support
I tried using the onInitialSpawn method and killed the entity if it is spawned on a higher level from Y=40, but that hardly looks like a permanent solution. I am sure canSpawn should be able to do something here, but I believe I am not using it right. What exactly is "SpawnReason"? Perhaps I can use that to achieve what I want. -
I was working on a custom monster. I wanted it to spawn on any light level, but below Y=40. So I don't want it to spawn naturally on the surface. I looked on some methods on my entity class and I came upon the *canSpawn* method, and I thought that would do the trick, but it is not working. Perhaps I am using it wrong? I also have another problem where my entity has random "spasms" and it goes crazy spinning around fast even though the movement speed is not set that high. It is also completely ignoring me even though I believe, according to the goals I have set for it, it should attack players when it sees one. The last issue that I have has to do with adding my entity to the spawn list of all the biomes. I want it to spawn pretty much everywhere except for the ocean biomes. I tried doing something, but it doesn't seem that efficient because I have to type out all the biomes myself. Perhaps there is a better way to do this, but I cannot think of something else. Any help would be appreciated. Thank you for your time. The problem with the spinning: My EntityRegistry Class: https://gist.github.com/Cerandior/60b1ac925525f7b5374af9d45e2ee3bf My Entity Class: https://gist.github.com/Cerandior/71230de0484594007d24cf52dd694e2e
-
Okay, thank you for your time man, appreciate it. I will look into some other mods for examples too.
-
It might be simple, I am not sure. I just don't think it is convenient for the player. It limits choice as for example he wouldn't be able to play with my mod if he wants to have biomes o plenty in it as well. I was hoping there was some sort of event that it is fired when the world is generated. I didn't want any big changes in the system anyway. Minor editing like for example the frequency of the generation of caves would probably do the trick for what I want. If there is no other way, I might as well just leave it be. Also, do you know how structure generation works in the newer versions of forge? I used to make a couple of mods a while back (when blockstates were introduced) and I think you could generate structures in the world using schematics back then. Not sure how you would do that now. Would you be able to link me to some forge docs or some existing project that explains what I want to do? Thank you.
-
Resolved by using another version of jdk. Apparently the jdk 8.0.221 doesn't work with gradle.
-
I did that right before you answered. It got rid of the little "x" icons in the run command, however I still cannot run the game (gives the same error: https://imgur.com/35qZLxo). And there are a lot of errors in the example mod file as well (https://imgur.com/a/3Pinslq zoom into the first image). The project has not been built correctly for some reason. It took 7-8 minutes for it to finish when I opened it for the first time, and that seemed a reasonable time to me. And there were no errors displayed during the build phase as well, so I am not sure what's going on. I tried other forge versions (1.14 versions), but the same thing happens. Do you think it has something to do with the jdk? I downloaded the latest version which was 1.12 (I think), but that was not supported by gradle. So I let the thing run using the old jdk version that was installed in my computer (1.8) Do I need a more recent version of jdk?
-
Hello everyone. I decided to give the new minecraft versions a go, but for some reason, I am not able to get the workspace to work. I opened the build.gradle file as a project in IntelliJ (As the instructions say you must do) and I let the process finish: https://imgur.com/a/3Pinslq I then run the "genIntelliJRuns" command and the run config completed successfully because they are imported in the IDE. However they have a little red "x" on their icon, and they don't work at all, displaying this error: https://imgur.com/35qZLxo Can someone help me, because I do not know what have I done wrong. I think I followed all the instructions provided in the README file. Thank you in advance.
-
By new world type, you mean as in "Amplified" or "Superflat"? Is there no other way to do this so it works in any world-type the player chooses?
-
I am working on a "fun" project, changing the game by adding things that in my opinion do not hinder the vanilla experience but make the game better. One thing about the game that I find kind of dull, are the caves. Is there any way to edit the way minecraft generates caves? I guess disabling the cave generation completely and creating a brand new one would work as well, but I do not posses the experience to do such a thing. Minor editing could do the trick. Also, while we are at it. Is there a way to disable an existing structure generation? For example, I want to disable the generation of dungeons and replace them with my own design. How do you generate structures in the world? Thank you for your answers.
-
Okay, thank you guys. It's time to get to work now
-
Well, thank you so much for your help guys, I will surely try this out now. I am also sorry for the late response, I was busy last day and didn't have time to get into Forge. EDIT: After having a quick look into @jabelar tutorial, I have one question to ask. In my case, If i were to add more than one "profession", (e.g: Mining, Enchanting), can I do this in one single interface, or do I need to create different interfaces and capabilities for each and every profession.
-
Hello. Lately I have been experimenting with all sort of things. After getting an idea about adding "abilities" I wanted to start working for it right away. What I want to do, is, to give each player an "ability" or more like a "profession". For example: Each player starts at level 0 at Alchemy. And the level progresses as the player completes different tasks and unlocks new things.And of course it will be more than one ability. So how I would go about adding a variable which holds the level of a specific "profession" to every player who connects in the world? I think with the old way you had to do something with ExtendedEntityProperties (Something along the lines of that), but forge has changed so much in the last couple of MC versions, I don't even know where to start. Someone can point me out the right way, or maybe can link me to an example, I would greatly appreciate it. Thank you for your time!