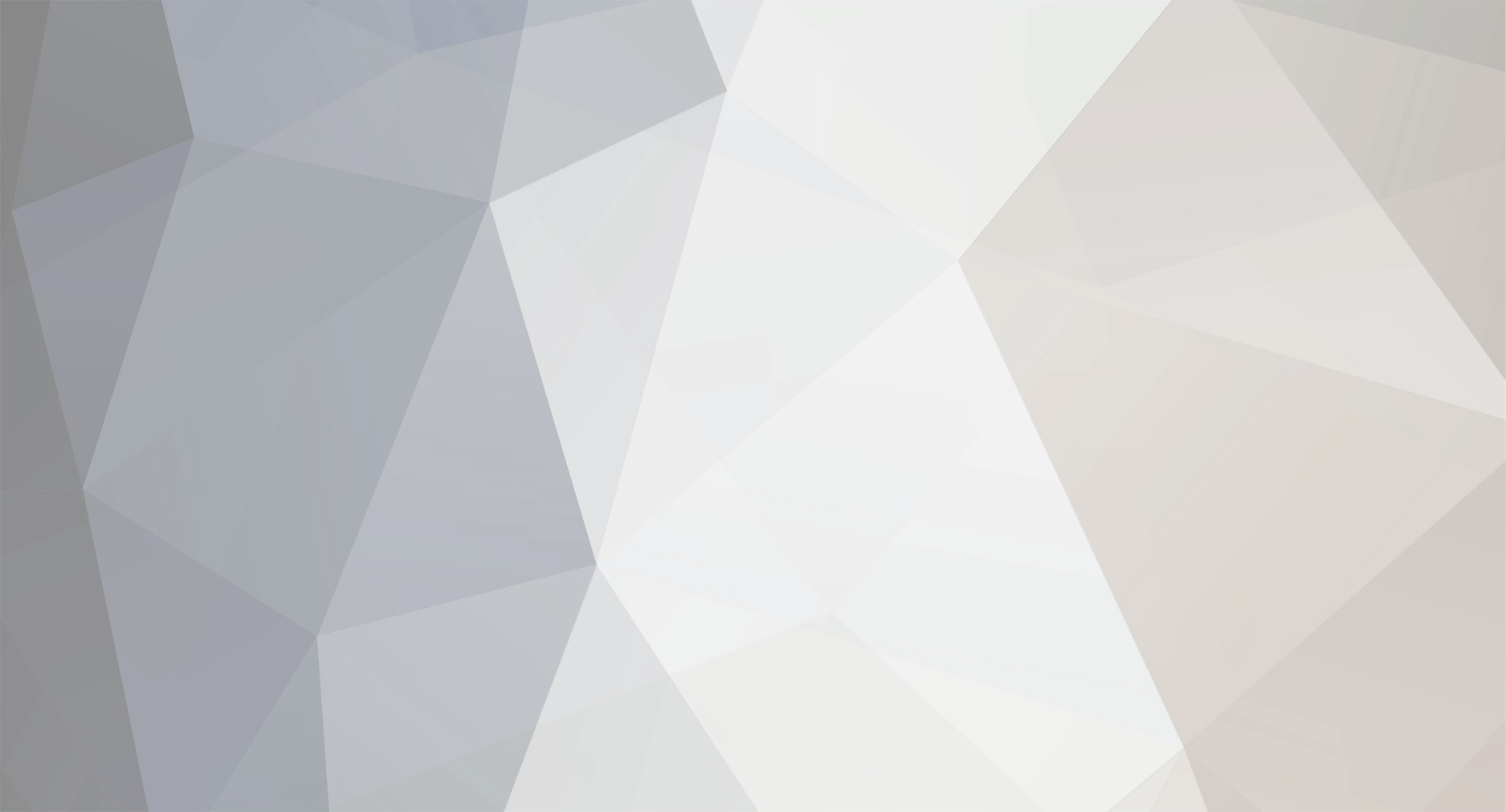
Cerandior
Members-
Posts
385 -
Joined
-
Last visited
Everything posted by Cerandior
-
[1.14.4] Error while trying to update data in the client.
Cerandior replied to Cerandior's topic in Modder Support
This whole thing started because I couldn't think of any other way to the save the list of offers of the entity in the NBT Data. Even the Trade Class itself is sort of a mess if you look at it. If you don't mind me asking. Why is the Java Serialization terrible? So do I just pass an instance of my entity to the packet and update its offer list in the handle() method? -
[1.14.4] Error while trying to update data in the client.
Cerandior posted a topic in Modder Support
I am trying to make a merchant-like entity who will sell the player weapons in return for gold. I am trying to render a list of his offers in the gui using the itemRenderer in my screen to render the items based on a list of the offers that the merchant has. There is a problem though. I randomly generate a list of item offers when the entity is first spawned on the world and all the data of his offers is on the server. The client doesn't know anything so I had to use a packet to notify the client. I am trying to update an arraylist field that is located in the container of the entity, which holds all the offers of this merchant, however I am hitting a NullPointerException at the getSender() of the context in the handle() method of the packet. My guess is that I have done something wrong in the registration of the packet, but I am not quite sure what because everything looks fine. I have never worked with packets before, so any help is appreciated. Also, I am sending the information to the client right after I open the GUI (Look at the entity class). Main Class Entity Class Packet Handler Class Packet Class The Trade Class (if requested for some reason) Thank you in advance. -
I am trying to make a merchant-like entity who will sell stuff to the player. I got the gui displaying correctly and I was about to add the slots to the container. I don't really need the entity to have an inventory of his own because I am checking only one slot content and based on that I update the other. So it is a very simple interface: https://prnt.sc/rgpua6 Anyway, because I don't want the entity to have an inventory of his own, I created an Inventory field in the entity class passing 2 as the number of slots in the constructor. So when I am adding the slots to the container I pass in this inventory field in the entity. However, I am getting this error: Judging by the size of the arraylist that is throwing this error (36), I am guessing it is the inventorySlots arraylist of the container. Its size would be 36 after adding all the player inventory slots. But why is it throwing an IndexOutOfBoundsException ? The addSlot() method doesn't even use #.get(index) anywhere, so where is this happening? Container Class: https://github.com/arjolpanci/VanillaExtended/blob/master/src/main/java/teabx/vanillaextended/container/WanderingAssassinContainer.java Entity Class: https://github.com/arjolpanci/VanillaExtended/blob/master/src/main/java/teabx/vanillaextended/entities/WanderingAssassin.java Note: It occurs to me as I am posting this, that the way that I am handling things to get the entity instance in the container (I am using two constructors) is pointless since I have the entity Id passed as the blockpos coordinates when I send a gui request to the client, and I can use that data to get the entity from the world. I will change that later.
-
I am an idiot. All my variables were int so the division was not accurate. I was essentially passing 0 to all arguments. Sorry for that. One final thing. How can I change the particles that show when I break the pipe block, because it just shows a purple mess. I am guessing it's because the pipe block itself doesn't have a json file (one with its name), instead the blockstate determines which models to render in-game. Can I solve the issue by just adding a default block json for the pipe with a specified texture? Would it override the blockstate model?
-
Okay, I fixed the update thing by using updatePostPlacement and checked there. Probably there are better ways of doing this because at the moment I am checking for every block around it just like in the initial placement but anyway, I am leaving it as it is for the moment. The only problem that remains now is the block bounds. Not sure why that isn't working. Updated block class: https://github.com/arjolpanci/VanillaExtended/blob/master/src/main/java/teabx/vanillaextended/blocks/TransportPipe.java
-
Ignoring the main problem for now, will look at it again. It seems like the block that is already placed doesn't receive updates on its blockstate when I try to connect other pipes to it. So if I place a pipe on the ground, and then another next to it, the one that was just placed adds the connection to it, but I need to break and replace the initial one to make it connect too. Can I use onNeighborChange to update the state of the block? Or is there something else, because I can't find out how to change the block state there.
-
Okay, so I changed the VoxelShape of the block, however it still doesn't work, now with the bonus feature of the blockbound being gone entirely. I can walk through the blocks. Also getShape was deprecated for me, but I used it anyway since I couldn't find any alternative. Updated Block Class: https://github.com/arjolpanci/VanillaExtended/blob/master/src/main/java/teabx/vanillaextended/blocks/TransportPipe.java
-
I also can't find out for what the Voxel Shapes are used for. My initial assumption was that they were used to create the different models, however it seems like those are created in the json file. And the blockstate file is what controls which models to add to the block based on its state. I am guessing the VoxelShapes control the blockbounds / hitbox? Could my problem be related to that?
-
Thank you for the help. I looked a little more carefully and as far as I understand, blockstates are used to determine whether the fence is connected or not and based on that you apply a model to the block. So I looked at the docs a bit for more information related to blockstates and all I need to do is create IProperty fields in my block class and then add them to the state container with fillStateContainer. I create BooleanProperties properties for all directions using BlockStateProperties fields and I used getStateForPlacement to determine at which direction should the block connect to. Also at the blockstate json file I return different models based on the state of connection of the block (Almost exactly the same as fence here, with the two extra values of up and down). However in-game I see no difference. I get no errors however it seems like I have done something wrong somewhere either in the blockstate file, or in the way I am handling the property fields. Block Class Here Block State File Here I am not used to blockstates. This is the first time I do anything with them, so please excuse any blatantly stupid mistake that I may have made.
-
onItemRightClick return an item [1.15.1]
Cerandior replied to Dr.Nickenstein's topic in Modder Support
I have no clue what the hell are you doing there. Don't mean to insult you, but based on that I am assuming you don't have much experience with programming in general. I'll help you this time and write it for you, but you will not get far if you don't know much programming. Item item = player.getHeldItem(handIn).getItem(); if(item instanceof ItemInit.RUBBER_EXTRACTOR){ //Do stuff } What you did wrong before: 1) itemstack.getItem() returns an item and does nothing else. Simply calling it and not assigning the returned value anywhere means that line of code is pretty much useless. 2) That is not how you use if statements. The syntax is: if(condition){ statement } -
onItemRightClick return an item [1.15.1]
Cerandior replied to Dr.Nickenstein's topic in Modder Support
That will return an itemstack I believe. itemstack.getItem() will give you the item and then you use instanceof to check whether that item is the same with what you want or not. -
onItemRightClick return an item [1.15.1]
Cerandior replied to Dr.Nickenstein's topic in Modder Support
Then go to your block class, override onBlockActivated and check there if the player is holding your item. If it is, do stuff. onBlockActivated provides you with the player that right clicked the block and the hand he used, so you have pretty much all you need. Alternatively, you can subscribe to PlayerInteractEvent.RightClickBlock and check there if the player is holding your item. -
onItemRightClick return an item [1.15.1]
Cerandior replied to Dr.Nickenstein's topic in Modder Support
This certain block, is it one of your own blocks or is it a vanilla block? -
onItemRightClick return an item [1.15.1]
Cerandior replied to Dr.Nickenstein's topic in Modder Support
Don't do it on the item, do it on the block. If it is your block override onBlockActivated and check if the player is holding your item. If it is a vanilla block use events to achieve the same thing. -
[1.14.4] How to update the slots in the gui for the client?
Cerandior replied to Cerandior's topic in Modder Support
God damn, thanks man. I resumed my work on this 4 days ago, and although I haven't had too much time to experiment with stuff, it still took me way too long to find any problem with it. Never would have guessed about the slotNumber being important, perhaps would have just given up on it if somebody didn't point that out. Would love to dive deeper into the source code sometime to try and get a better idea of what's going on, but unfortunately I have been too busy with uni stuff lately. -
[1.14.4] How to update the slots in the gui for the client?
Cerandior replied to Cerandior's topic in Modder Support
This is what I am currently doing (There are 2 separate lists for items and empty slots for troubleshooting reasons, there was initially one list): https://gist.github.com/arjolpanci/5e1aa52909d8cb62494d08167e85d634 So instead you suggest adding all the slots in the beginning and then when I scroll I should change the slots xpos and ypos? I also checked how it is done in the creative screen, however vanilla uses setInventorySlotContents which I don't think can be used for what I want to do right? -
So, I have been stuck on something for a while now. I am trying to make a multi-block which detects any inventories connected to it, and then you can manage all these inventories in a main block. I have managed to do pretty much everything at this point. The multi-block detects all inventories connected to it, and I can view the items in the main block. However, a problem arises in the GUI of the main block when I have more than 54 slots worth of inventory connected (Basically more than 2 normal chests or 1 double chest). Because It would be stupid to make a humongous GUI to fit all the slots of all the inventories connected to the multi-block, instead the gui holds only 54 slots, and through a slider I can navigate through each of the inventories. So okay, I did the slider. The way I am handling the "sliding mechanism" is by getting the position of the slider and scaling that to a number that tells me at which position I should start loading the slots. I hold a list of all the slots available in the multi-block. With all the empty slots being at the end, so I don't have random blank spaces showing up in the gui (just for aesthetic purposes). So by getting the offset from the slider, I simply clear the inventory slots of the container, and re-add the slots to the container but instead of starting from index 0 of the list of the slots, I start at the offset given by the slider. (There are also some shenanigans that make it so you scroll row by row and not slot by slot, but you get the idea). The scrolling initially looks fine, however there is a problem. I checked (through some rather silly printlns) and it seems to me like the server has the correct slot data, however the client is completely clueless. Since the client is the one rendering the GUI, the slots that show up after scrolling are essentially useless. They are "copies" of previous slots with incorrect data and unusable to store anything else. How can I give the client the correct slot information? Obviously, the first thing I thought of were packets, but what information should I be sending to the client exactly? If I send the whole container through the packet, then I would need to serialize and deserialize that into a byte array because I can't store it in any other way using the packet buffer. And after I get that container in the client, do I just execute the method that I use for updating the slots there? I am not quite sure what I am supposed to do here, any help would be appreciated. Thank you in advance.
-
How the hell did you even find this post. It's from 2014.