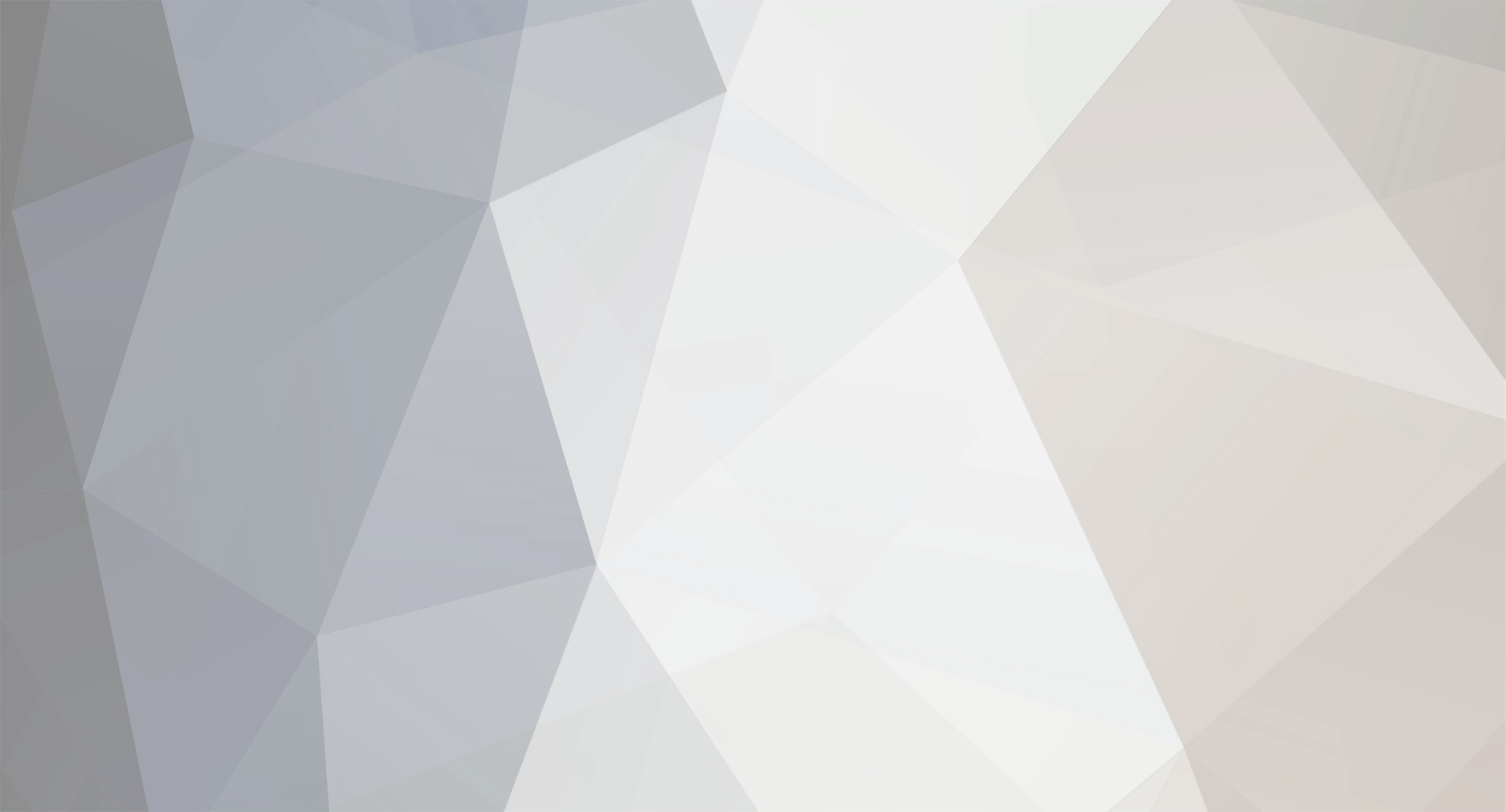
WitherBoss2000
Members-
Posts
25 -
Joined
-
Last visited
Everything posted by WitherBoss2000
-
For making a TARDIS
-
Hi! I am attempting to make a Dr. Who mod, and I want to make it like the TV show itself. I was thinking that I could borrow code from the dimensional doorway mod and hook it up to an entity instead of a door, so it is easier to move. Does anyone know where I can look at some code or if they actually know how to do it? Please help me.
-
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
Sorry it took me so long to respond, here is my most recent updated code. Also, I am not asking you to code my entire mod, this is only MY FIRST MOD!!! Please, just tell me how to do it and then I will know. Here it is... package com.camp.entity; import net.minecraft.entity.Entity; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; public class EntityTruck extends EntityMob // this to make mob hostile { public boolean stationary; public EntityTruck(World par1World) { super(par1World); isImmuneToFire = false; } protected boolean canDespawn() { return false; } public boolean interact(EntityPlayer entityplayer) { if (riddenByEntity == null || riddenByEntity == entityplayer) { entityplayer.mountEntity(this); return true; } else { return false; } } protected boolean isMovementCeased() { return stationary; } public void moveEntity(double d, double d1, double d2) { if (riddenByEntity != null) { EntityPlayer player = (EntityPlayer) this.riddenByEntity; this.prevRotationYaw = this.rotationYaw = this.riddenByEntity.rotationYaw; this.rotationPitch = this.riddenByEntity.rotationPitch * 0.5F; this.setRotation(this.rotationYaw, this.rotationPitch); this.rotationYawHead = player.rotationYawHead; stationary = false; motionX += riddenByEntity.motionX * 10; // * 0.20000000000000001D; motionZ += riddenByEntity.motionZ * 10; // * 0.20000000000000001D; //this.moveStrafing = player.moveStrafing; //this.moveForward = player.moveForward; if (isCollidedHorizontally) { isJumping = true; } else { isJumping = false; } super.moveEntity(motionX, motionY, motionZ); } else { stationary = false; super.moveEntity(d, d1, d2); } } public void onUpdate() { super.onUpdate(); if (riddenByEntity != null) //check if there is a rider { //currentTarget = this; this.randomYawVelocity = 0; //try not to let the horse control where to look. this.rotationYaw = riddenByEntity.rotationYaw; } } protected boolean isAIEnabled() //Allow your AI task to work? { return true; } } -
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
Sorry, but still it refuses to move. -
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
Wow, I have to say you've got some of the best explanations. Very thorough, tells you where to look, and at the same time isn't spoonfeeding. Not sure you can get any better than that. Dude, this isnt a praise area. -
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
Ok, but there is no moveForward field in the riding entity. Or a moveStrafing. -
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
Anyone? -
When you calculate your attributes for the entity, do you store them as a variable? If so,you can write after the fact that the stats are calculated: protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(<whatever you used as your variable>); } The code above will make the stats the same as calculated. Repeat the line beginning with this. for each attribute. Also, look at the give command whenever you make a sword that increases your speed. Then, for your morphing, I think that is one of the ways to do it, but maybe instead of doing that, try: public static <name of mob class> mobOne; //Put this after the class declaration line. int mobMaxHealth = mobOne.getEntityAttribute(SharedMonsterAttributes.maxHealth); //put the following in the if statement player.setHealth(mobMaxHealth); Try that, if the second part doesnt work, I have never tried to change the Player health, but I know it is stored as NBT data. Try it, you dont have to use it, but hopefully that works.
-
[1.7.10] @SideOnly(Side.CLIENT) Not use it anymore?
WitherBoss2000 replied to American2050's topic in Modder Support
I am pretty sure you cant have 2 annotations with the @SideOnly, you dont need the overide. Maybe put the override below the @sideonly for some strange reason. This happens to me too. Trust me, it still works in 1.8 -
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
[glow=red,20,200] I tried the keybinding, and it doesnt work. Also, I couldnt find it in the EntityBoat class. I have no idea what I am doing. If anyone knows how to help me, please tell me! [/glow] -
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
[glow=red,20,200]Oh... Well I dont know any other way of making it move, I have never made one before. If I put a solid number there, it will move, but only in one direction and non-controllable. I was thinking that I could use keybindings, but I have no idea how to do that.[/glow] -
How do I make my Truck work? Help Me!!!
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
[glow=red,20,200] Anyone out there that can help me? Please? [/glow] -
[shadow=red,left][font=arial][glow=red,4,400]Ok, so I am wondering how I can make a truck. So, I made code for it and it works, but it doesnt move. It rotates and everything, but it stays stationary. Why does it do that? How do I fix it? Please help. [/glow] Here is my code for the Entity: package com.camp.entity; [/font][/shadow] import net.minecraft.entity.Entity; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; public class EntityTruck extends EntityMob // this to make mob hostile { public boolean stationary; public EntityTruck(World par1World) { super(par1World); isImmuneToFire = false; } protected void applEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(20000.0d); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.4f); this.getEntityAttribute(SharedMonsterAttributes.knockbackResistance).setBaseValue(1.0f); this.getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(100f); this.getEntityAttribute(SharedMonsterAttributes.attackDamage).setBaseValue(10.0f); } protected boolean canDespawn() { return false; } public boolean interact(EntityPlayer entityplayer) { if (riddenByEntity == null || riddenByEntity == entityplayer) { entityplayer.mountEntity(this); return true; } else { return false; } } protected boolean isMovementCeased() { return stationary; } public void moveEntity(double d, double d1, double d2) { if (riddenByEntity != null) { this.prevRotationYaw = this.rotationYaw = this.riddenByEntity.rotationYaw; this.rotationPitch = this.riddenByEntity.rotationPitch * 0.5F; this.setRotation(this.rotationYaw, this.rotationPitch); this.rotationYawHead = this.renderYawOffset = this.rotationYaw; stationary = true; motionX += riddenByEntity.motionX * 2F; // * 0.20000000000000001D; motionZ += riddenByEntity.motionZ * 2F; // * 0.20000000000000001D; if (isCollidedHorizontally) { isJumping = true; } else { isJumping = false; } super.moveEntity(motionX, motionY, motionZ); } else { stationary = false; super.moveEntity(d, d1, d2); } } public void onUpdate() { super.onUpdate(); if (riddenByEntity != null) //check if there is a rider { //currentTarget = this; this.randomYawVelocity = 0; //try not to let the horse control where to look. this.rotationYaw = riddenByEntity.rotationYaw; } } protected boolean isAIEnabled() //Allow your AI task to work? { return true; } } [shadow=red,left][/shadow]
-
How do I make a custom crafting bench [UNSOLVED]
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
So, you expect someone to just provide you with a fully fledged tutorial in here instead of just linking you to one of the dozens that already exist out there? Yeah no. Well, that was no h. None work. -
[glow=red,2,300]Idea![/glow] Maybe it is a glitch with mac/OSX because I dont have the problem on windows, but my mac it does that.
-
How do I make a custom crafting bench [UNSOLVED]
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
Thanks! I will try it, but could I be able to extend/implement the crafting table block with a override to the on block activated? -
How do I make a custom crafting bench [UNSOLVED]
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
[move][shadow=red,left][glow=red,2,300]and, they don't work for 1.8[/glow][/shadow][/move] -
How do I make a custom crafting bench [UNSOLVED]
WitherBoss2000 replied to WitherBoss2000's topic in Modder Support
OK, all that meant was all I see are "Common Proxy" crap, and it does never work for me, so all i wanted was for someone either to tell me or link it up to another tutorial. -
Help! I want to make a custom block that I can right click on and it will open the crafting GUI, so I can make a custom model fir it (i know hiw to do that.) Anyway, the block is called CoolBlock, and can anybody tell me how to do this for 1.8? No proxy, just strait up tell me.