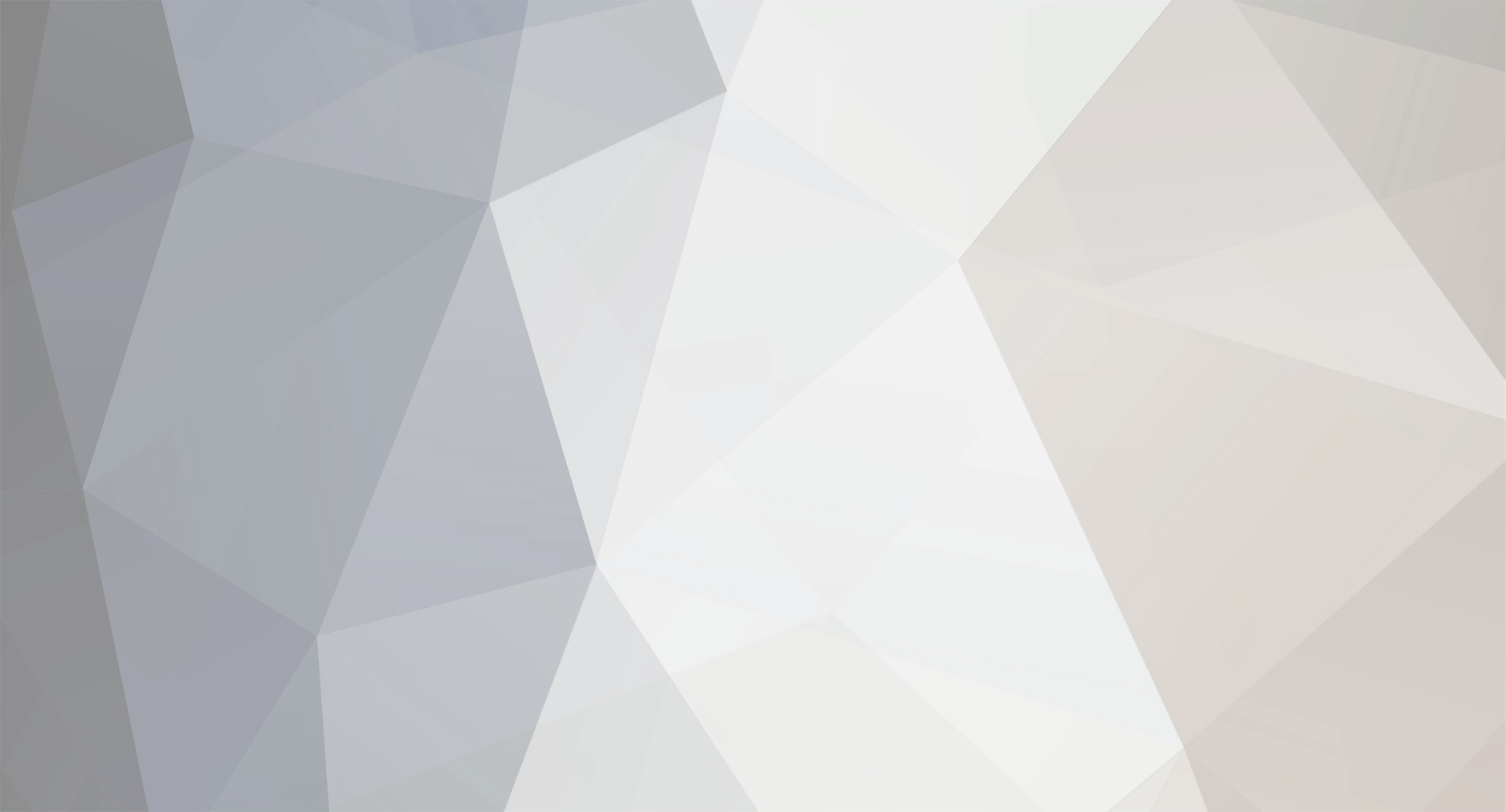
Differentiation
Members-
Posts
606 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Differentiation
-
(SOLVED) [1.12.2] Problems with custom item and NBT
Differentiation replied to MythicalChromosome's topic in Modder Support
Wait, wait, wait, wait!! Are you trying to make it so that you can use the item once PER PLAYER?? Or every player can use that item once. So for example, if I use the item and I give it to another player, should they be able to use it? -
(SOLVED) [1.12.2] Problems with custom item and NBT
Differentiation replied to MythicalChromosome's topic in Modder Support
I tried playing with it myself and it just doesn't seem to work. You can do it my way by just adding to player capability. I do it this way: public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand hand) { DinocraftPlayer player = DinocraftPlayer.getEntityPlayer(playerIn); if (!player.getActions().hasUsedItem1()) { player.setHasUsedItem1(true); return new ActionResult<ItemStack>(EnumActionResult.SUCCESS, stack); } else { return new ActionResult<ItemStack>(EnumActionResult.FAIL, stack); } } Now the player can't use this item until they die. And then the process repeats. For your case, it is best to use player capabilities since the item usage data is unique to the player itself and not the item. -
LOOK FOR COLLABORATORS TO HELP YOU CREATE THIS MOD
Differentiation replied to HomixHD's topic in Mods
No, sorry. -
LOOK FOR COLLABORATORS TO HELP YOU CREATE THIS MOD
Differentiation replied to HomixHD's topic in Mods
I actually can program and I'm a good texturer for items and blocks only. But I am working on my own project, the Dinocraft mod, sorry, I can't participate -
(SOLVED) [1.12.2] Problems with custom item and NBT
Differentiation replied to MythicalChromosome's topic in Modder Support
@Override @SideOnly(Side.CLIENT) public void addInformation(ItemStack stack, World worldIn, List<String> tooltip, ITooltipFlag flag) { EntityPlayer playerIn = Minecraft.getMinecraft().player; super.addInformation(stack, worldIn, tooltip, flag); if (stack.getTagCompound() != null) { NBTTagCompound nbt = stack.getTagCompound(); if (nbt.getTag("usedOn") != null && nbt.hasKey("usedOn")) { NBTTagList list = (NBTTagList) nbt.getTag("usedOn"); for (int i = 0; i < list.tagCount(); ++i) { if (list.getStringTagAt(i) == playerIn.getUniqueID().toString()) { tooltip.add("Already used by " + playerIn.getDisplayName()); } } } } } Sorry, that's better. -
LOOK FOR COLLABORATORS TO HELP YOU CREATE THIS MOD
Differentiation replied to HomixHD's topic in Mods
I'm not too good at animations. But very cool models! Good luck! -
What version are you using?
-
Subscribe to ItemTooltipEvent to add information to the tooltip of items. To change the color of item names, simply include "§" + any formatting codes before the name of the item in the lang file.
-
Does that mean that World::rayTraceBlocks(...) is server-side? So I can do this?: @Override public void execute(MinecraftServer server, ICommandSender sender, String[] args) throws CommandException { EntityPlayer playerIn = getCommandSenderAsPlayer(sender); Vec3d posVec = new Vec3d(playerIn.posX, playerIn.posY + playerIn.getEyeHeight(), playerIn.posZ); Vec3d lookVec = playerIn.getLookVec(); World worldIn = playerIn.worldObj; RayTraceResult trace = worldIn.rayTraceBlocks(posVec, posVec.add(lookVec.scale(100))); // or is this client-side? BlockPos pos = trace.getBlockPos(); }
-
(SOLVED) [1.12.2] Problems with custom item and NBT
Differentiation replied to MythicalChromosome's topic in Modder Support
@Override @SideOnly(Side.CLIENT) public void addInformation(ItemStack stack, World worldIn, List<String> tooltip, ITooltipFlag flagIn) { EntityPlayer playerIn = Minecraft.getMinecraft().player; super.addInformation(stack, worldIn, tooltip, flagIn); if (stack.getTagCompound() != null && nbt.hasKey("usedOn")) { NBTTagCompound nbt = stack.getTagCompound(); if (nbt.getTag("usedOn") != null { NBTTagList list = (NBTTagList) nbt.getTag("usedOn"); for (int i = 0; i < list.tagCount(); ++i) { if (list.getStringTagAt(i) == playerIn.getUniqueID().toString()) { tooltip.add("Already used by " + playerIn.getDisplayName()); } } } } } There, that's better. You have to add null checks, not everything is present all the time. -
(SOLVED) [1.12.2] Problems with custom item and NBT
Differentiation replied to MythicalChromosome's topic in Modder Support
What if it doesn't have "usedOn"? "nbttaglist" is null. That's a NullPointerException. -
(SOLVED) [1.12.2] Problems with custom item and NBT
Differentiation replied to MythicalChromosome's topic in Modder Support
Post your FML Log(s)? -
[1.11]Exploded Block Spaces Stop Player
Differentiation replied to admiralmattbar's topic in Modder Support
You have to send a packet to the server to create an explosion. You can't create an explosion with World::isRemote or else the server will not know that an explosion happened and update the world assuming nothing happened (that's why you get stuck in a block, because the client tries to mimic the server's role). But onImpact() is common method, I believe, so I don't know what the problem is... -
[1.11]Exploded Block Spaces Stop Player
Differentiation replied to admiralmattbar's topic in Modder Support
When you create the explosion with !World::isRemote, does the explosion happen? If not, that means you must send a packet to the server. -
But if I use it in execute(MinecraftServer server, ICommandSender sender, String args[]) throws CommandException, which is a logical server method, will I need to send packets or will World::rayTrace be server-side? In other words, is World::rayTrace client or server-side? Because I need to then get the BlockPos and then make that block air, like so: public void execute(MinecraftServer server, ICommandSender sender, String args[]) throws CommandException { EntityPlayer playerIn = getCommandSenderAsPlayer(sender); World worldIn = playerIn.worldObj; RayTraceResult trace = worldIn.rayTrace(...) // this is client or server side? BlockPos pos = trace.getBlockPos(); worldIn.setBlockToAir(pos); } Thanks!
-
Hey! So there is a method called EntityPlayer::rayTrace(...) and it contains the annotation @SideOnly(Side.CLIENT), does that mean that I cannot use this method when running physical server? And if so, what is an alternative that can get the player's RayTraceResult and BlockPos (block's position that the player is looking at). The reason being is that I want to make a command that can teleport the player to the position of the block they are looking at. Is there any alternative way to accomplish this? Any suggestions and help are welcome. Thanks!
-
1. Make a private int in the class. 2. 1 Minecraft day = 20 minutes (day and night - 10 minutes day, 10 minutes night). 3. 6 Minecraft days = 20 * 6 = 120 minutes = 2 hours. 5. 120 minutes = 3600 seconds. 4. 7200 * 40 = 288000 Java update ticks. 5. Code: public class Test { private int time; public void onTime() { ++time; if (time == 288000 /* 2 hours (6 Minecraft days) */) { time = 0; // do things } } } However, if you log out, I believe the time resets to 0. I hope I helped.
-
[1.10.2] [SOLVED] Side threads running.
Differentiation replied to Differentiation's topic in Modder Support
I mean, yeah, I was expecting it to run on the same thread. Thanks! -
STATEMENTS | REASONS 1. Distance from player to entity is diagonal | 1. Diagonal is a line segment that extends from one angle of the | quadrilateral to its opposite angle | | How do you want the distance demonstrated by diagonal? Are the entities on opposite angles of a quadrilateral? Please clarify. And P.S. don't use 1.8, update to 1.10.2 + please, believe me, it's way more worth it.
-
[1.10.2] [SOLVED] Side threads running.
Differentiation replied to Differentiation's topic in Modder Support
But then if I run a method in a method that runs on the logical server such as execute(...), will the method that I run inside run on the logical server, the logical client or both, in that case? Ex. public void onCommand(EntityPlayer playerIn) { World worldIn = playerIn.worldObj; worldIn.playSound(null, playerIn.getPosition(), SoundEvents.BLOCK_CLOTH_BREAK, SoundCategory.PLAYERS, 1.0F, 1.0F); DinocraftPlayer player = DinocraftPlayer.getEntityPlayer(playerIn); player.setMaxHealth(playerIn.getMaxHealth() + 1.0F); } @Override public void execute(MinecraftServer server, ICommandSender sender, String[] args) throws CommandException { EntityPlayer playerIn = getCommandSenderAsPlayer(sender); this.onCommand(playerIn); // when I call this method, will the contents inside the method run on the server-thread, client-thread, or common (client and server-side)? } Thanks! -
[1.10.2] [SOLVED] Side threads running.
Differentiation replied to Differentiation's topic in Modder Support
Thank you! You cleared up many things for me. I finally fully understand why Minecraft.getMinecraft(), for example, doesn't work when on a server, and things like that. Thanks again! Happy modding! -
Ah, I see. So sorry, I never really fully understand client and server-side, it is very ambiguous and confusing at times. I created a new thread with a question about sides and method calling. I didn't get an answer yet D: Would you mind helping out? it's called [1.10.2] Side threads running. I basically ask: if I call a method in a server-side method, will the contents in that method run on the server-side, client-side, or both?
-
Is there a built-in link for this kind of style: /* Event fired when an entity dies */ @SubscribeEvent(priority = EventPriority.HIGHEST) public void onDeath(LivingDeathEvent event) { if (event.getEntityLiving() instanceof EntityLiving) { EntityLiving entityliving = (EntityLiving) event.getEntityLiving(); entityliving.playSound(SoundEvents.BLOCK_METAL_BREAK, 1.0F, 1.0F); World worldIn = entity.worldObj; for (int i = 0; i < 50; ++i) { worldIn.spawnParticle(EnumParticleTypes.BLOCK_CRACK, entityliving.posX, entityliving.posY + (entityliving.height - 0.25D), entityliving.posZ, Math.random() * 0.2D - 0.1D, Math.random() * 0.25D, Math.random() * 0.2D - 0.1D, Block.getIdFromBlock(Blocks.REDSTONE_BLOCK) ); } } } (The Minecraft built - in style)?