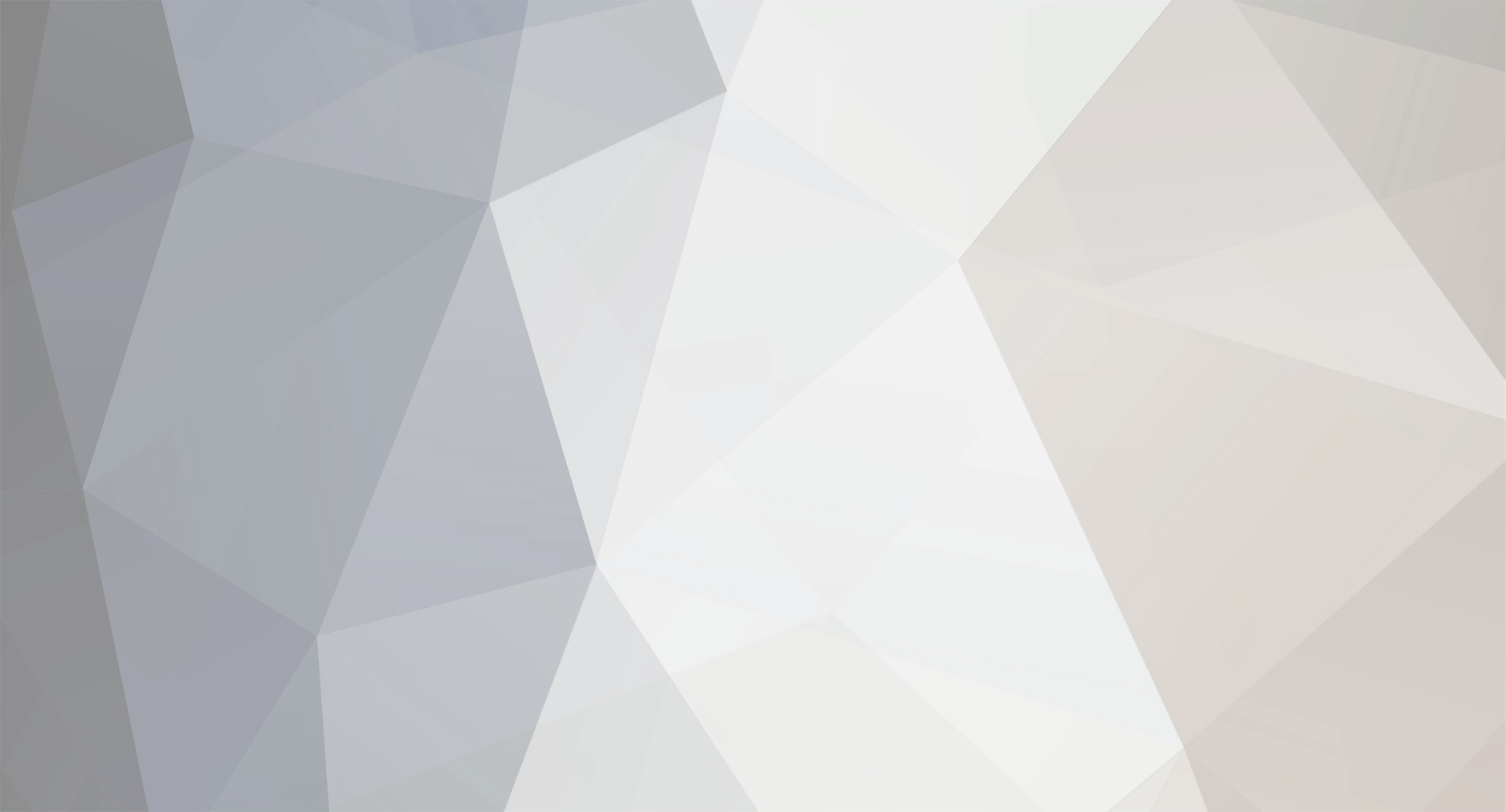
Differentiation
Members-
Posts
606 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Differentiation
-
public class ItemRayGun extends Item { public ItemRayGun(String name) { this.setUnlocalizedName(name); this.setMaxStackSize(1); this.setMaxDamage(1000); this.setRegistryName(new ResourceLocation(Reference.MODID, name)); } @Override public int getMaxItemUseDuration(ItemStack stack) { return 1; } @Override public void onUsingTick(ItemStack stack, EntityLivingBase entityliving, int count) { EntityPlayer player = (EntityPlayer) entityliving; DinocraftEntity dinoEntity = DinocraftEntity.getEntity(player); if (player.isCreative() || dinoEntity.hasAmmo(DinocraftItems.RAY_BULLET)) { if (!player.isCreative()) { dinoEntity.consumeAmmo(DinocraftItems.RAY_BULLET, 1); stack.damageItem(1, player); } if (!player.world.isRemote) { EntityRayBullet ball = new EntityRayBullet(player, 0.001F); ball.shoot(player, player.rotationPitch, player.rotationYaw, 0.0F, 15.0F, 0.0F); ball.setRotationYawHead(player.rotationYawHead); Vec3d vector = player.getLookVec(); double x = vector.x; double y = vector.y; double z = vector.z; ball.motionX = x * 3.33D; ball.motionZ = z * 3.33D; ball.motionY = y * 3.33D; ball.setPositionAndUpdate(player.posX - (x * 0.75D), player.posY + player.eyeHeight, player.posZ - (z * 0.75D)); player.world.spawnEntity(ball); player.world.playSound(null, player.getPosition(), DinocraftSoundEvents.RAY_GUN_SHOT, SoundCategory.NEUTRAL, 3.0F, player.world.rand.nextFloat() + 0.5F); } DinocraftEntity.getEntity(player).recoil(0.1F, 1.25F, true); } super.onUsingTick(stack, player, count); } @Override public void onUpdate(ItemStack stack, World world, Entity entity, int itemSlot, boolean isSelected) { if (isSelected) { EntityPlayer player = (EntityPlayer) entity; DinocraftEntity dinoEntity = DinocraftEntity.getEntity(player); if (player.isCreative() || dinoEntity.hasAmmo(DinocraftItems.RAY_BULLET)) { Item mainhand = player.getHeldItemMainhand().getItem(); if (mainhand != null && mainhand == this) { player.setActiveHand(EnumHand.MAIN_HAND); } else { player.setActiveHand(EnumHand.OFF_HAND); } } else if (!world.isRemote) { dinoEntity.sendActionbarMessage(TextFormatting.RED + "Out of ammo!"); world.playSound(null, player.getPosition(), SoundEvents.BLOCK_DISPENSER_DISPENSE, SoundCategory.NEUTRAL, 0.5F, 5.0F); } super.onUpdate(stack, world, entity, itemSlot, isSelected); } } } I noticed that onUsingTick only fires every tick if I explicitly call setActiveHand in onUpdate every tick... onUsingTick doesn't have anything to do with me right-clicking or using the item. That's what is so confusing to me.
-
package dinocraft.item; import dinocraft.Reference; import dinocraft.capabilities.entity.DinocraftEntity; import dinocraft.entity.EntityRayBullet; import dinocraft.init.DinocraftItems; import dinocraft.init.DinocraftSoundEvents; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.SoundEvents; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ActionResult; import net.minecraft.util.EnumActionResult; import net.minecraft.util.EnumHand; import net.minecraft.util.ResourceLocation; import net.minecraft.util.SoundCategory; import net.minecraft.util.math.Vec3d; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; public class ItemRayGun extends Item { public ItemRayGun(String name) { this.setUnlocalizedName(name); this.setMaxStackSize(1); this.setMaxDamage(1000); this.setRegistryName(new ResourceLocation(Reference.MODID, name)); } @Override public void onUsingTick(ItemStack stack, EntityLivingBase entityliving, int count) { EntityPlayer player = (EntityPlayer) entityliving; DinocraftEntity dinoEntity = DinocraftEntity.getEntity(player); if (player.isCreative() || dinoEntity.hasAmmo(DinocraftItems.RAY_BULLET)) { if (!player.isCreative()) { dinoEntity.consumeAmmo(DinocraftItems.RAY_BULLET, 1); stack.damageItem(1, player); } if (!player.world.isRemote) { EntityRayBullet ball = new EntityRayBullet(player, 0.001F); ball.shoot(player, player.rotationPitch, player.rotationYaw, 0.0F, 15.0F, 0.0F); ball.setRotationYawHead(player.rotationYawHead); Vec3d vector = player.getLookVec(); double x = vector.x; double y = vector.y; double z = vector.z; ball.motionX = x * 3.33D; ball.motionZ = z * 3.33D; ball.motionY = y * 3.33D; ball.setPositionAndUpdate(player.posX - (x * 0.75D), player.posY + player.eyeHeight, player.posZ - (z * 0.75D)); player.world.spawnEntity(ball); player.world.playSound(null, player.getPosition(), DinocraftSoundEvents.RAY_GUN_SHOT, SoundCategory.NEUTRAL, 3.0F, player.world.rand.nextFloat() + 0.5F); } DinocraftEntity.getEntity(player).recoil(0.1F, 1.25F, true); } super.onUsingTick(stack, player, count); } @Override public ActionResult<ItemStack> onItemRightClick(World world, EntityPlayer player, EnumHand hand) { ItemStack stack = player.getHeldItem(hand); DinocraftEntity dinoEntity = DinocraftEntity.getEntity(player); if (player.isCreative() || dinoEntity.hasAmmo(DinocraftItems.RAY_BULLET)) { player.setActiveHand(hand); return ActionResult.newResult(EnumActionResult.SUCCESS, stack); } else if (!world.isRemote) { dinoEntity.sendActionbarMessage(TextFormatting.RED + "Out of ammo!"); world.playSound(null, player.getPosition(), SoundEvents.BLOCK_DISPENSER_DISPENSE, SoundCategory.NEUTRAL, 0.5F, 5.0F); return ActionResult.newResult(EnumActionResult.FAIL, stack); } return ActionResult.newResult(EnumActionResult.FAIL, stack); } } The onUsingTick method doesn't call at all when I right-click.
-
Show the code, initialization events, etc. Waraxe would just be an axe with a different texture and different attack speed (if you want) by adding an attribute modifier.
-
[SOLVED] [1.14.4] Smoke render distance like Campfire, how?
Differentiation replied to Overstand's topic in Modder Support
Set ignoreRange to true in World::spawnParticle(...). -
Subscribe to BreakEvent.Then check if the block is the block you want to get the item out of. Lastly, use World::spawnEntity(...) to spawn an item.
-
[1.14.4] player capability side questions
Differentiation replied to andGarrett's topic in Modder Support
You can attach them to both by subscribing to AttachCapabilitiesEvent<Entity> and attaching to PlayerEntity. You'll need to send a packet for when the player clones to save and load the capability data on Clone event since this event is only called on server thread. -
[SOLVED] [1.12.2] NBT writing/reading for List
Differentiation replied to Differentiation's topic in Modder Support
@Animefan8888 I figured out the problem: readSavedFile() is @SideOnly(Side.SERVER), and all my testing was on the clients with an Integrated Server thread, so readSavedFile() wasn't actually being called. Gonna make this list (and command) Dedicated Server thread-friendly only since it makes more sense that way anyways. Thanks for all the help! -
[SOLVED] [1.12.2] NBT writing/reading for List
Differentiation replied to Differentiation's topic in Modder Support
I mean, I don't know much about this File writing/reading. -
[SOLVED] [1.12.2] NBT writing/reading for List
Differentiation replied to Differentiation's topic in Modder Support
It inevitably creates a new instance of UserListMutes every time the mod is loaded. That's my issue. I could make the class abstract, but then I can't really instantiate it. I have no idea how to resolve this. -
[SOLVED] [1.12.2] NBT writing/reading for List
Differentiation replied to Differentiation's topic in Modder Support
File::createNewfile(); only creates the file if it does not already exist, I thought. I'll have to double check -
[SOLVED] [1.12.2] NBT writing/reading for List
Differentiation replied to Differentiation's topic in Modder Support
https://github.com/TheWoodenWizard/DC-mod/tree/master/src/main/java/dinocraft/handlers