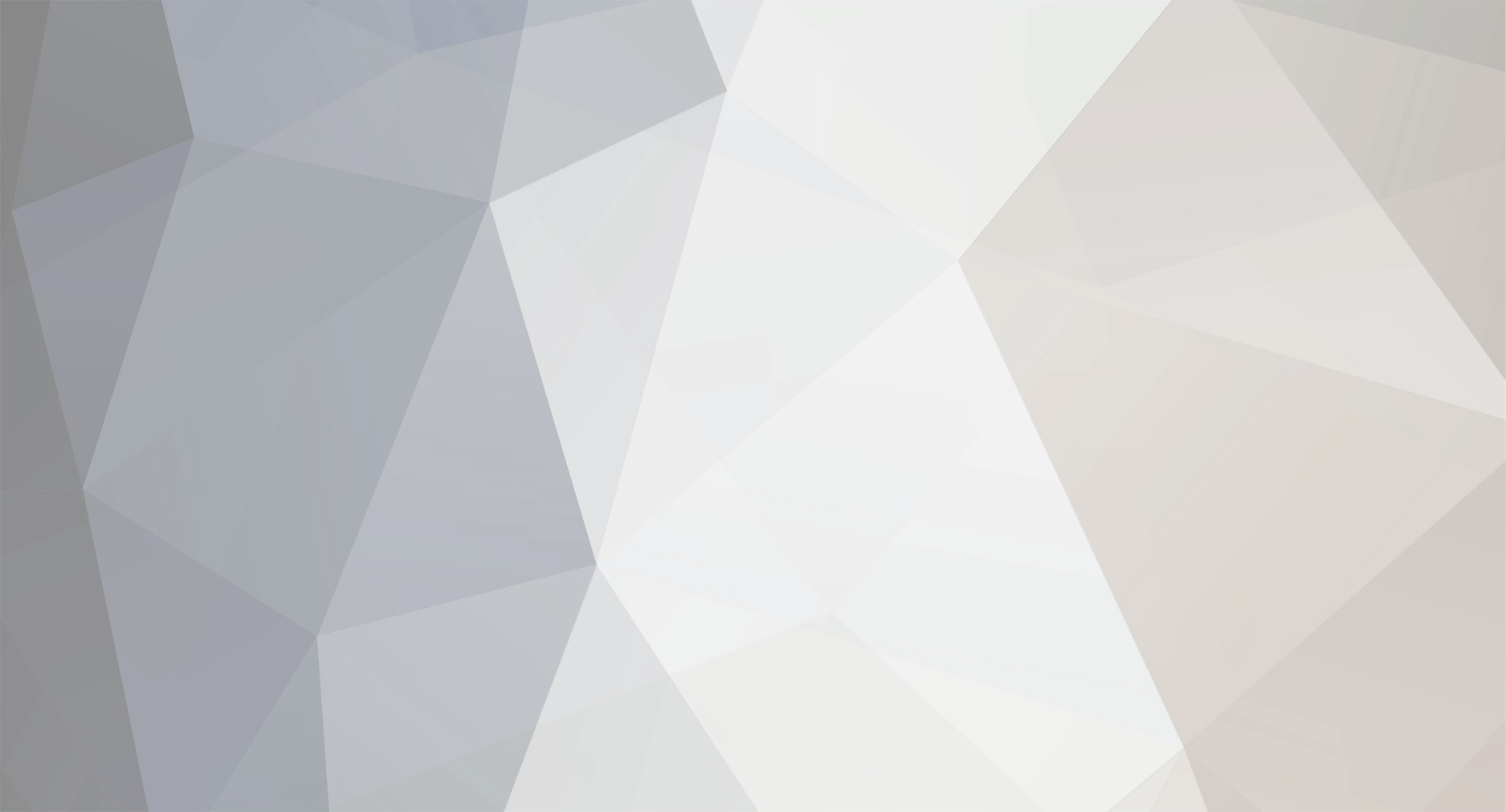
Differentiation
Members-
Posts
606 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Differentiation
-
public class DinocraftConfig { private static Configuration config = null; public static final String COMMANDS = "commands"; public static final String ENTITIES = "entities"; public static final String MISCELLANEOUS = "miscellaneous"; public static final String MUTES = "mutes"; public static final String PLAYER = "player"; public static boolean COMMANDS_ENABLED; public static int PERMISSION_LEVEL_ASPLAYER; public static int PERMISSION_LEVEL_ASSERVER; public static int PERMISSION_LEVEL_FORBID; public static int PERMISSION_LEVEL_FORBIDDENLIST; public static int PERMISSION_LEVEL_MUTELIST; public static int PERMISSION_LEVEL_OPLIST; public static int PERMISSION_LEVEL_MUTE; public static int PERMISSION_LEVEL_MUTE_FULL; public static int PERMISSION_LEVEL_OPLEVEL; public static int PERMISSION_LEVEL_RANK; public static int PERMISSION_LEVEL_SHUTDOWN; public static int PERMISSION_LEVEL_UNFORBID; public static int PERMISSION_LEVEL_UNMUTE; public static int PERMISSION_LEVEL_UNMUTE_FULL; public static int PERMISSION_LEVEL_ADD; public static int PERMISSION_LEVEL_BACK; public static int PERMISSION_LEVEL_BOUNCE; public static int PERMISSION_LEVEL_BREAK; public static int PERMISSION_LEVEL_BURN; public static int PERMISSION_LEVEL_CLEARCHAT; public static int PERMISSION_LEVEL_DURABILITY; public static int PERMISSION_LEVEL_FEED; public static int PERMISSION_LEVEL_FLY; public static int PERMISSION_LEVEL_FREEZE; public static int PERMISSION_LEVEL_GOD; public static int PERMISSION_LEVEL_HEAL; public static int PERMISSION_LEVEL_HEALTH; public static int PERMISSION_LEVEL_HUNGER; public static int PERMISSION_LEVEL_JUMP; public static int PERMISSION_LEVEL_KABOOM; public static int PERMISSION_LEVEL_MAXHEALTH; public static int PERMISSION_LEVEL_PING; public static int PERMISSION_LEVEL_REACH; public static int PERMISSION_LEVEL_REGENERATE; public static int PERMISSION_LEVEL_SPEED; public static int PERMISSION_LEVEL_STRIKE; public static int PERMISSION_LEVEL_TPBACK; public static int PERMISSION_LEVEL_TPHERE; public static int PERMISSION_LEVEL_TPTO; public static int PERMISSION_LEVEL_UNFREEZE; public static int PERMISSION_LEVEL_UNVANISH; public static int PERMISSION_LEVEL_VANISH; public static boolean WORLD_SAVING; public static int WORLD_SAVING_INTERVAL; public static boolean WEAPON_RECOIL; public static String[] FORBIDDEN_ITEMS; public static String CHAT_WORD1; public static String CHAT_REPLACEMENT1; public static String CHAT_WORD2; public static String CHAT_REPLACEMENT2; public static String CHAT_WORD3; public static String CHAT_REPLACEMENT3; public static String CHAT_WORD4; public static String CHAT_REPLACEMENT4; public static String CHAT_WORD5; public static String CHAT_REPLACEMENT5; public static String CHAT_WORD6; public static String CHAT_REPLACEMENT6; public static String CHAT_WORD7; public static String CHAT_REPLACEMENT7; public static String CHAT_WORD8; public static String CHAT_REPLACEMENT8; public static String CHAT_WORD9; public static String CHAT_REPLACEMENT9; public static String CHAT_WORD10; public static String CHAT_REPLACEMENT10; public static String CHAT_WORD11; public static String CHAT_REPLACEMENT11; public static String CHAT_WORD12; public static String CHAT_REPLACEMENT12; public static int MUTE_LEVEL_MAX; public static boolean ENTITY_ITEMS; public static boolean ENTITY_BLOOD_EFFECTS; public static boolean PLAYER_BLOOD_EFFECTS; public static void preInit() { File saveFile = new File(Loader.instance().getConfigDir(), "Dinocraft.cfg"); config = new Configuration(saveFile); syncFromFiles(); } public static Configuration getConfig() { return config; } public static void syncFromFiles() { syncConfig(true, true); } public static void syncFromGUI() { syncConfig(false, true); } public static void syncFromFields() { syncConfig(false, false); } private static void syncConfig(boolean load, boolean read) { if (load) { config.load(); } COMMANDS_ENABLED = config.getBoolean("Commands Enabled", COMMANDS, true, "Whether mod commands are enabled or not."); config.setCategoryComment(COMMANDS, "For each command, specify the permission level necessary to run the command. [range: 0 ~ 4]"); Property asPlayer = config.get(COMMANDS, "'asPlayer' Command Permission Level", 4, "", 0, 4); Property asServer = config.get(COMMANDS, "'asServer' Command Permission Level", 4, "", 0, 4); Property forbid = config.get(COMMANDS, "'forbid' Command Permission Level", 3, "", 0, 4); Property forbiddenlist = config.get(COMMANDS, "'forbiddenlist' Command Permission Level", 3, "", 0, 4); Property mutelist = config.get(COMMANDS, "'mutelist' Command Permission Level", 2, "", 0, 4); Property oplist = config.get(COMMANDS, "'oplist' Command Permission Level", 3, "", 0, 4); Property mute = config.get(COMMANDS, "'mute' Command Permission Level", 2, "", 0, 4); Property muteFull = config.get(COMMANDS, "'mute' Full Command Permission Level", 4, "", 0, 4); Property oplevel = config.get(COMMANDS, "'oplevel' Command Permission Level", 4, "", 0, 4); Property rank = config.get(COMMANDS, "'rank' Command Permission Level", 3, "", 0, 4); Property shutdown = config.get(COMMANDS, "'shutdown' Command Permission Level", 4, "", 0, 4); Property unforbid = config.get(COMMANDS, "'unforbid' Command Permission Level", 3, "", 0, 4); Property unmute = config.get(COMMANDS, "'unmute' Command Permission Level", 4, "", 0, 4); Property unmuteFull = config.get(COMMANDS, "'unmute' Full Command Permission Level", 4, "", 0, 4); Property add = config.get(COMMANDS, "'add' Command Permission Level", 3, "", 0, 4); Property back = config.get(COMMANDS, "'back' Command Permission Level", 2, "", 0, 4); Property bounce = config.get(COMMANDS, "'bounce' Command Permission Level", 3, "", 0, 4); Property break1 = config.get(COMMANDS, "'break' Command Permission Level", 3, "", 0, 4); Property burn = config.get(COMMANDS, "'burn' Command Permission Level", 3, "", 0, 4); Property clearchat = config.get(COMMANDS, "'clearchat' Command Permission Level", 3, "", 0, 4); Property durability = config.get(COMMANDS, "'durability' Command Permission Level", 3, "", 0, 4); Property feed = config.get(COMMANDS, "'feed' Command Permission Level", 3, "", 0, 4); Property fly = config.get(COMMANDS, "'fly' Command Permission Level", 3, "", 0, 4); Property freeze = config.get(COMMANDS, "'freeze' Command Permission Level", 2, "", 0, 4); Property god = config.get(COMMANDS, "'god' Command Permission Level", 3, "", 0, 4); Property heal = config.get(COMMANDS, "'heal' Command Permission Level", 3, "", 0, 4); Property health = config.get(COMMANDS, "'health' Command Permission Level", 3, "", 0, 4); Property hunger = config.get(COMMANDS, "'hunger' Command Permission Level", 3, "", 0, 4); Property jump = config.get(COMMANDS, "'jump' Command Permission Level", 2, "", 0, 4); Property kaboom = config.get(COMMANDS, "'kaboom' Command Permission Level", 3, "", 0, 4); Property maxhealth = config.get(COMMANDS, "'maxhealth' Command Permission Level", 3, "", 0, 4); Property ping = config.get(COMMANDS, "'ping' Command Permission Level", 2, "", 0, 4); Property reach = config.get(COMMANDS, "'reach' Command Permission Level", 4, "", 0, 4); Property regenerate = config.get(COMMANDS, "'regenerate' Command Permission Level", 3, "", 0, 4); Property speed = config.get(COMMANDS, "'speed' Command Permission Level", 4, "", 0, 4); Property strike = config.get(COMMANDS, "'strike' Command Permission Level", 3, "", 0, 4); Property tpback = config.get(COMMANDS, "'tpback' Command Permission Level", 2, "", 0, 4); Property tphere = config.get(COMMANDS, "'tphere' Command Permission Level", 2, "", 0, 4); Property tpto = config.get(COMMANDS, "'tpto' Command Permission Level", 2, "", 0, 4); Property unfreeze = config.get(COMMANDS, "'unfreeze' Command Permission Level", 2, "", 0, 4); Property unvanish = config.get(COMMANDS, "'unvanish' Command Permission Level", 2, "", 0, 4); Property vanish = config.get(COMMANDS, "'vanish' Command Permission Level", 2, "", 0, 4); FORBIDDEN_ITEMS = config.getStringList("Pebbeloneum Forbidden Items", MISCELLANEOUS, new String[] {"minecraft:nether_star", "minecraft:dragon_egg", "minecraft:end_portal_frame", "minecraft:end_portal_frame", "minecraft:end_crystal", "minecraft:spawn_egg", "minecraft:barrier", "minecraft:air", "minecraft:mob_spawner", "minecraft:structure_block", "minecraft:structure_void", "minecraft:command_block", "minecraft:chain_command_block", "minecraft:repeating_command_block", "minecraft:command_block_minecart", "minecraft:bedrock"}, "Items that cannot come out of Pebbeloneum"); ENTITY_ITEMS = config.getBoolean("Entities With Mod Items", ENTITIES, true, "Whether mobs are able to spawn with mod items and armor."); ENTITY_BLOOD_EFFECTS = config.getBoolean("Entity Blood Effects", ENTITIES, true, "Whether entities should shed blood when they die."); PLAYER_BLOOD_EFFECTS = config.getBoolean("Player Blood Effects", ENTITIES, true, "Whether players should shed blood when hit."); WORLD_SAVING = config.getBoolean("World Saving", MISCELLANEOUS, true, "Whether the server saves the world. [only works on a server]"); WORLD_SAVING_INTERVAL = config.getInt("World Saving Interval", MISCELLANEOUS, 600, 1, 10000000, "How often the server will save the world (seconds). [only works on a server]"); WEAPON_RECOIL = config.getBoolean("Weapon Recoil", MISCELLANEOUS, true, "Whether weapons inflict recoil when used."); config.setCategoryComment(PLAYER, "Place a word to be replaced by its replacement"); CHAT_WORD1 = config.getString("Chat Word 1", PLAYER, "", ""); CHAT_REPLACEMENT1 = config.getString("Chat Replacement 1", PLAYER, "", ""); CHAT_WORD2 = config.getString("Chat Word 2", PLAYER, "", ""); CHAT_REPLACEMENT2 = config.getString("Chat Replacement 2", PLAYER, "", ""); CHAT_WORD3 = config.getString("Chat Word 3", PLAYER, "", ""); CHAT_REPLACEMENT3 = config.getString("Chat Replacement 3", PLAYER, "", ""); CHAT_WORD4 = config.getString("Chat Word 4", PLAYER, "", ""); CHAT_REPLACEMENT4 = config.getString("Chat Replacement 4", PLAYER, "", ""); CHAT_WORD5 = config.getString("Chat Word 5", PLAYER, "", ""); CHAT_REPLACEMENT5 = config.getString("Chat Replacement 5", PLAYER, "", ""); CHAT_WORD6 = config.getString("Chat Word 6", PLAYER, "", ""); CHAT_REPLACEMENT6 = config.getString("Chat Replacement 6", PLAYER, "", ""); CHAT_WORD7 = config.getString("Chat Word 7", PLAYER, "", ""); CHAT_REPLACEMENT7 = config.getString("Chat Replacement 7", PLAYER, "", ""); CHAT_WORD8 = config.getString("Chat Word 8", PLAYER, "", ""); CHAT_REPLACEMENT8 = config.getString("Chat Replacement 8", PLAYER, "", ""); CHAT_WORD9 = config.getString("Chat Word 9", PLAYER, "", ""); CHAT_REPLACEMENT9 = config.getString("Chat Replacement 9", PLAYER, "", ""); CHAT_WORD10 = config.getString("Chat Word 10", PLAYER, "", ""); CHAT_REPLACEMENT10 = config.getString("Chat Replacement 10", PLAYER, "", ""); CHAT_WORD11 = config.getString("Chat Word 11", PLAYER, "", ""); CHAT_REPLACEMENT11 = config.getString("Chat Replacement 11", PLAYER, "", ""); CHAT_WORD12 = config.getString("Chat Word 12", PLAYER, "", ""); CHAT_REPLACEMENT12 = config.getString("Chat Replacement 12", PLAYER, "", ""); List<String> order = new ArrayList<String>(); order.add(CHAT_WORD1); order.add(CHAT_REPLACEMENT1); order.add(CHAT_WORD2); order.add(CHAT_REPLACEMENT2); order.add(CHAT_WORD3); order.add(CHAT_REPLACEMENT3); order.add(CHAT_WORD4); order.add(CHAT_REPLACEMENT4); order.add(CHAT_WORD5); order.add(CHAT_REPLACEMENT5); order.add(CHAT_WORD6); order.add(CHAT_REPLACEMENT6); order.add(CHAT_WORD7); order.add(CHAT_REPLACEMENT7); order.add(CHAT_WORD8); order.add(CHAT_REPLACEMENT8); order.add(CHAT_WORD9); order.add(CHAT_REPLACEMENT9); order.add(CHAT_WORD10); order.add(CHAT_REPLACEMENT10); order.add(CHAT_WORD11); order.add(CHAT_REPLACEMENT11); order.add(CHAT_WORD12); order.add(CHAT_REPLACEMENT12); config.setCategoryPropertyOrder(PLAYER, order); if (read) { PERMISSION_LEVEL_ASSERVER = asServer.getInt(); PERMISSION_LEVEL_ASPLAYER = asPlayer.getInt(); PERMISSION_LEVEL_FORBID = forbid.getInt(); PERMISSION_LEVEL_FORBIDDENLIST = forbiddenlist.getInt(); PERMISSION_LEVEL_MUTELIST = mutelist.getInt(); PERMISSION_LEVEL_OPLIST = oplist.getInt(); PERMISSION_LEVEL_MUTE = mute.getInt(); PERMISSION_LEVEL_MUTE_FULL = muteFull.getInt(); PERMISSION_LEVEL_OPLEVEL = oplevel.getInt(); PERMISSION_LEVEL_RANK = rank.getInt(); PERMISSION_LEVEL_SHUTDOWN = shutdown.getInt(); PERMISSION_LEVEL_UNFORBID = unforbid.getInt(); PERMISSION_LEVEL_UNMUTE = unmute.getInt(); PERMISSION_LEVEL_UNMUTE_FULL = unmuteFull.getInt(); PERMISSION_LEVEL_ADD = add.getInt(); PERMISSION_LEVEL_BACK = back.getInt(); PERMISSION_LEVEL_BOUNCE = bounce.getInt(); PERMISSION_LEVEL_BREAK = break1.getInt(); PERMISSION_LEVEL_BURN = burn.getInt(); PERMISSION_LEVEL_CLEARCHAT = clearchat.getInt(); PERMISSION_LEVEL_DURABILITY = durability.getInt(); PERMISSION_LEVEL_FEED = feed.getInt(); PERMISSION_LEVEL_FLY = fly.getInt(); PERMISSION_LEVEL_FREEZE = freeze.getInt(); PERMISSION_LEVEL_GOD = god.getInt(); PERMISSION_LEVEL_HEAL = heal.getInt(); PERMISSION_LEVEL_HEALTH = health.getInt(); PERMISSION_LEVEL_HUNGER = hunger.getInt(); PERMISSION_LEVEL_JUMP = jump.getInt(); PERMISSION_LEVEL_KABOOM = kaboom.getInt(); PERMISSION_LEVEL_MAXHEALTH = maxhealth.getInt(); PERMISSION_LEVEL_PING = ping.getInt(); PERMISSION_LEVEL_REACH = reach.getInt(); PERMISSION_LEVEL_REGENERATE = regenerate.getInt(); PERMISSION_LEVEL_SPEED = speed.getInt(); PERMISSION_LEVEL_STRIKE = strike.getInt(); PERMISSION_LEVEL_TPBACK = tpback.getInt(); PERMISSION_LEVEL_TPHERE = tphere.getInt(); PERMISSION_LEVEL_TPTO = tpto.getInt(); PERMISSION_LEVEL_UNFREEZE = unfreeze.getInt(); PERMISSION_LEVEL_UNVANISH = unvanish.getInt(); PERMISSION_LEVEL_VANISH = vanish.getInt(); } if (config.hasChanged()) { config.save(); } } @EventBusSubscriber public static class ConfigEventHandler { @SubscribeEvent public static void onConfigChanged(OnConfigChangedEvent event) { if (event.getModID().equals(Reference.MODID)) { syncFromGUI(); } } } }
-
Hey! I made a bunch of new settings in the config file, but they organize themselves in alphabetical order and it's quite interrupting with what I'm trying to do. Is there any way to specify the order or have it be ordered according to the way the code appears? Thanks EDIT: I tried using Configuration::setCategoryPropertyOrder(category, propOrder); but it still orders it lexicographically...
-
[1.12.2] Check if player is online?
Differentiation replied to Differentiation's topic in Modder Support
I mean I don't expect some beginner modder to be like "ohhh shiiiit!! I know what I gotta do, I gotta get the MinecraftServer instance, then get the PlayerList instance, then call some random method and check if the object is not null!" Ya feel me? If there is no other simpler way then heck, I'll go with this one, but just out of curiousity ya know -
[1.12.2] Check if player is online?
Differentiation replied to Differentiation's topic in Modder Support
Is there a better way though? I'm sure they created some sort of method to make this easier to figure out -
Hey, I wanna check if a player is online. Currently I use FMLCommonHandler.instance().getMinecraftServerInstance().getPlayerList().getPlayerByUUID(uuid) != null. Is there a better way without calling so many methods? Any help is appreciated! Thanks! :)
-
I mean, it isn't necessarily capabilities that I'm trying to store, it's just raw data (some boolean, integer variables, etc.) and that's what I meant by using JSON. So the way I currently do it is make a temporary entry in my JSON file for offline player. Then when they log on, I move the values to NBT (the player capability class) and remove the JSON entry.
-
[1.14.4] How override a existing minecraft item
Differentiation replied to Brbcode's topic in Modder Support
And keep the event priority at NORMAL or LOW not to be too selfish when it comes to compatibility with other mods that want to do something with that item. -
Checking for EntityPlayer on LivingHurtEvent
Differentiation replied to nil's topic in Modder Support
if (LivingHurtEvent###getEntityLiving instanceof EntityPlayer) Since EntityPlayer extends EntityLivingBase. -
[1.14.4] [SOLVED] Side of the BreakSpeed event?
Differentiation replied to Atila1091's topic in Modder Support
Just test: if world is remote print something. If world is not remote print something else. It's simple. -
[1.14.4] How override vanilla command.
Differentiation replied to MairwunNx's topic in Modder Support
I don't know how to do this, but if I were you I'd make the command name the same and register it. Then, on CommandEvent, cancel the event if the command is the one you want to override. -
There's no real way to actually delete one chat message or modify it after it had been sent. The is a way to delete every chat message, but that won't solve your problem.
-
[1.14.4] How to kick player from server
Differentiation replied to Maciej916's topic in Modder Support
I know that in MC 1.12.2 it's EntityPlayerMP#connection#disconnect, but I'm not so sure on 1.14, I'm guessing it should be the same. -
[SOLVED][1.14.4] Persist Player attributes on respawn
Differentiation replied to JimiIT92's topic in Modder Support
Clone, not PlayerRespawnEvent. -
Simply cancel the event after running all the code will cancel damaging the player. HOWEVER, make sure to set event priority to low or lowest not to conflict with aspects/effects of other mods.
-
Okay, motions and velocity have to be handled on the client side as well. The reason why nothing happens is because you have !World#isRemote check; only the server is notified of the velocity change, the client is not, so you won't witness anything happening. Also, use PlayerEntity###getItemStackFromSlot to check if the boots are equipped. Lastly, you don't need to include your Mod ID in the EventBusSubscriber annotation. P.S. was the Entity#world field removed? If not, just use PlayerEntity#world instead of PlayerEntity###getEntityWorld.