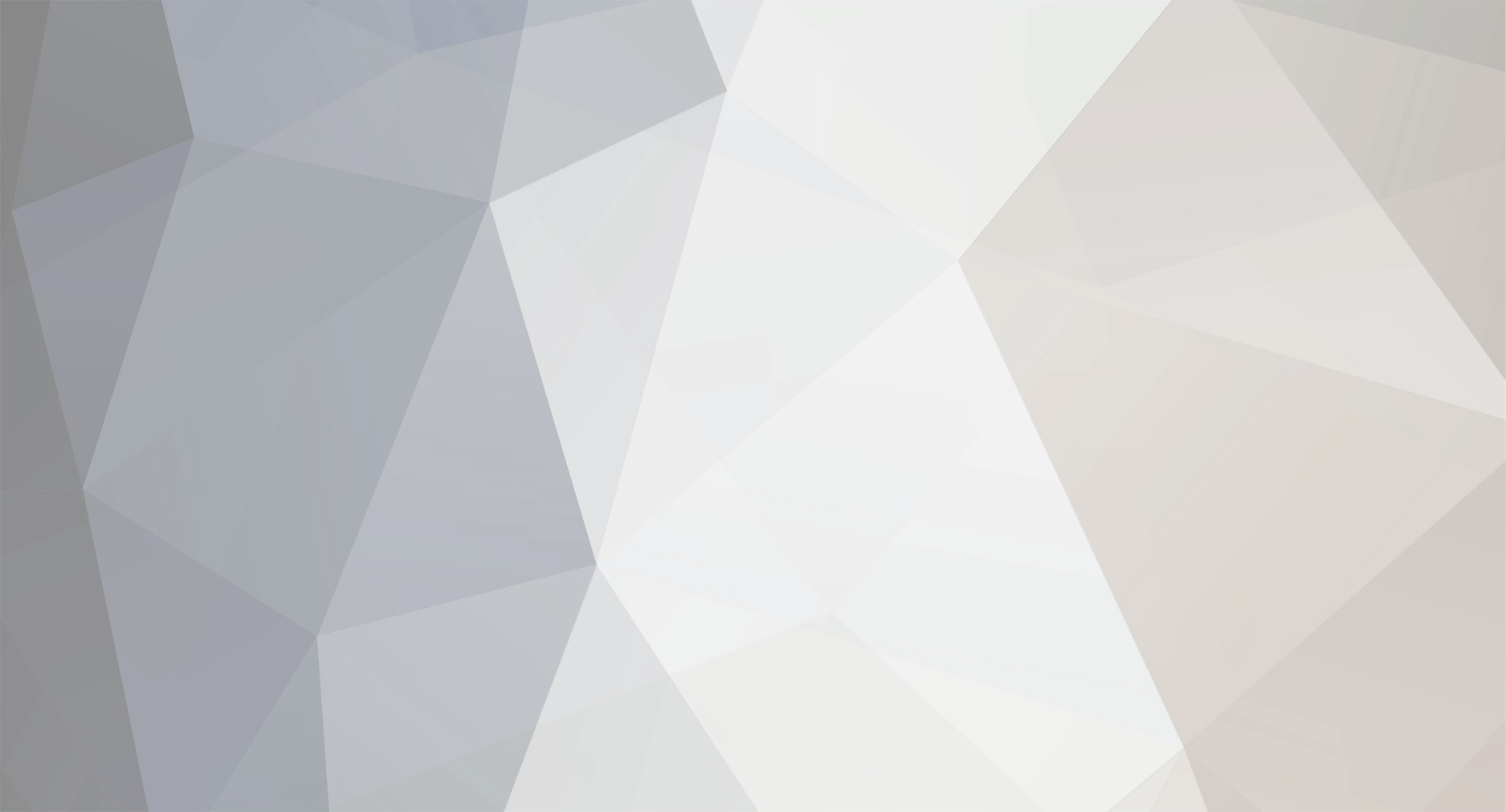
redria7
Members-
Posts
120 -
Joined
-
Last visited
Everything posted by redria7
-
You are completely right. That makes sense. Well, alternatively you could use 2 textures, one for fully connected and one for fully independent, then use the appropriate parts of each texture when drawing textures in your renderer. It would mean doing extra work selecting out the bits from each texture to draw from, but it wouldn't be too awful either. The only problem I might foresee is if there are texture stitching lines, where there is a faint white line where the 2 textures are meeting that would point out where the textures meet.
-
onBlockActivated not working (Changing from one block to another)
redria7 replied to dude22072's topic in Modder Support
It crashes when you have an empty hand because you aren't checking to make sure there is an item in your hand first. Add: if(par5EntityPlayer.getHeldItem() == null) { return super.onBlockActivated(par1World, par2, par3, par4, par5EntityPlayer, par6, par7, par8, par9); } to the very beginning of your method before you do anything else in your method. As far as the block not changing correctly, maybe try just returning true if the correct item is used instead of calling the super onBlockActivated regardless of what item is used. No guarantees but it might be worth a shot. -
You would only need 16 textures. 1 for no connections, 4 for one connection on each of the 4 sides of a block, 6 for 2 connections, 4 for 3 connections, and 1 for 4 connections. I kind of want to do this now just to see how well it would work. I don't imagine I'm missing anything.
-
You would need to make a custom renderer for your glass block that draws each face of the block itself. It will get passed world data and can use this to decide which textures to use. While this would not be obscenely difficult and you could probably do it in a day if you knew hot to make a renderer already etc, Draco is right. Not easily.
-
You need this method in your Grave1Block.java class. This tells the block which direction you were facing when you placed the block. Next, you need to change your renderAModelAt method in your RenderGrave1.java class to this. All I did was add couple lines that rotate it depending on the meta data. I don't know which way it it supposed to rotate, so you will have to fiddle a little bit to make it work correctly. To that end, you should just be able to add 90 to the start variable until it works correctly. (or if it continues not working, change to (start + meta * 90)F ) These are the same things mnn said, just more specific.
-
What is the file path for your texture? For example, my items have a texture at minecraft/mods/%myMod%/textures/items/%myItem%. What is the actual file path to your textures?
-
You are using the wrong method to set your meta data. you need to use: For that method I literally took it straight from the pumpkin class. That sets the meta data depending on the direction you are facing when you place the block. It does not take into account if you are looking up or down, just N, S, E, W. Then getIcon will be something like... Of course you would have to set each of your topIcon variables along with blockIcon. This would give you a block where you rotate the top icon and the other sides all have the same texture. You can, of course, change this. The bottom is side == 0, top is side == 1, and the 4 sides are 2-5.
-
The way I am doing this is just to have different textures for each of the ways a block may face, then depending on your meta data getIcon can just return the appropriate icon. This of course means rotating and saving your top texture into multiple images and storing them using registerIcons, and possibly there is a better way to do this, but this way does work and it is very easy to do.
-
Sorry, I have been mostly unable to use this website recently for whatever reason, so no promises that I will have timely responses at all... If you want it to behave similarly to the workbench, you might want to try looking at the workbench code. I'm sure it could probably be combined with the furnace code to get what you want. However... What I would do as a preliminary test to get things working, followed by fixing and streamlining etc later, would be to have 3 recipe classes. These classes represent having 1 ingredient, 2 ingredients, and 3 ingredients. Each would have a variable similar to mine: private int recipes[][] = new int[M][N]; Where N is the number of ingredients that class covers, and M is maybe the number of recipes you plan on having. Each class also has their own array of results. The index of the recipe is the index of the results array. Lots of code can be copied over from mine. Doing it this way will almost guarantee you a few errors the first time you run it simply because you will have to look through my code, understand what it is doing, see where you are changing something, and make that change work. This process makes this all a lot of work for something that might work slowly or be buggy that you will have to fix, but it is really gratifying when it works the first time and all you have left is making everything beautiful. In the (I think) container class, or wherever checks are made on what recipes do what etc, you will have to check each of your recipe classes. Check how many ingredients are currently in the furnace and use the corresponding recipe class. Since it is shapeless, you will need to either have every variation of the recipe in your recipe class, or when you query your recipe class, you need to run through every variation of the ingredients currently in the furnace. It will probably be easier to code in every recipe and just make one query. Does this make sense? When you make your recipe class, go to your container class and remove the vanilla recipes import. This will highlight every reference to the vanilla recipes and point out what methods are important and need to be understood.
-
I haven't figured out hashmaps yet (somehow I never learned them even though they were covered in a class I took) so I made arrays for my 2 input furnace. You would have yours behave similarly. Note- this is not a good way to do things! Every 5 recipes I add, I have to go through and expand my array. This is just a temporary thing until I can figure out hash-maps independently. Also, my furnace requires a certain order for the items in the slots. If there were no particular order you could just add the recipe with each order possibility. For you, I would actually recommend making 3 recipe classes, one for one input, one for 2 inputs, and one for 3 inputs. If you would like me to any part of this to you just ask.
-
If you dare to try to make your model through code, know enough about coding to survive, and you don't plan on making your model move (because I have no idea how that works yet)... First go to: net.minecraft.client.renderer And open the class: RenderBlocks.java There is a method not too far down called renderBlockByRenderType This method lists lots of different render types used by different normal blocks. Hold control and click on of the method names (or just scroll down to it) to jump to the actual method. It will show you how that specific block is rendered. Fire was particularly interesting for me the past couple days and taught me a lot about how to use the tessellator. If you want to make a new block with your own renderer that renders the same as a vanilla block (for science), copy the code for it and it will be a new method in your renderer class. Go to: http://www.minecraftforge.net/wiki/Multiple_Pass_Render_Blocks Scroll down to How can we get the block to render on both passes? and read. The tutorial is very good. It does not go into how to draw the item in your inventory, which I am still struggling with, but instead of the drawDiamond method, you would use your copied code from the vanilla renderer. A key note here is that the vanilla renderer calls on itself a lot to do things. In the method: renderWorldBlock a renderer is already given to you. Pass this to your drawing method and use that passed renderer instead of this.doSomethingVanillaRendererWasDoing. If you are still reading, expect this to take a little bit of time. Experiment and try changing some of the numbers used by the tessellator to draw a vertice if your code uses the tessellator. It will teach you a lot about what does what. Refer back to this and the linked tutorial as much as necessary. Every error has a very good reason and you will find it.
-
Separate questions: 1. Is there a way to specify the type of lighting a block produces? Say I wanted a block to produce the same type of light as moonlight, so a cool, blue light instead of the warm orange light. Has anyone seen a way to do so? 2. Is there a way to render non-cubic shapes in the inventory? I have a block that uses fire as part of its rendering and I would like to have the fire render in my inventory as part of the block, meaning it would be shaped like fire is on the ground: slanted with intersections etc. At the moment all I can find is a way to draw the fire vertically which does not look as nice. If you have a suggestion or need clarification just let me know. Thank you!
-
The method I need to change is getRenderType(). Currently the ladders are set to render as ladders, and wherever the rendering is happening, the meta data of the block is being pulled and used. Then the image is being rendered separately from the hit-box so the image and hit-box are displayed in 2 different places. I just changed my rendering type to 1, or flowers. It makes a really cool looking X pattern with my texture. So I need to find the ladder renderer and make my own, possibly involving making a new model depending on if I need to make a new model. The inner workings of a game are fascinating.
-
So yeah, when I change the meta values to 0-3, all of my hit boxes are in the right place, but 2 of the ladders have no texture and the other 2 ladders (presumably the ones that overlap with 2-5) show the texture but the texture is floating on nothing on the wrong side of the ladder block. I'll do some research on how to fix this unless someone gets to it first and lets me know.
-
That's what I thought, but it seems like when I try that, my ladder is invisible. Maybe I should try again now that I'm a little farther along with my project but it looks like if the meta values aren't within 2-5 it renders wrong. And I'm pretty sure I changed all the proper methods in my custom ladder class. I'm not sure. I'll try changing things again and post again later if it doesn't work.
-
The meta data of ladders is stored, as far as I know, solely to determine which adjacent block the ladder has been placed on. However, it uses the meta data values of 2, 3, 4, and 5. Does anyone know why this is/why the meta data isn't stored as 0-3? I want to give my custom ladders a directional meta value that would need all 16 meta values. I could just make more custom ladders as alternate blocks but I'd rather handle it with meta.
-
getIcon is definitely the method to use. I don't know which version of forge/minecraft you are using Draco, but in 1.5.1/1.5.2 getIcon is the method to use to put different textures on different sides. vandy-Read carefully through your getIcon code (or post it so we can see). You are probably trying to add code after you have returned a value. If you look through your code and at any point it says "return ***" where *** is some icon, then if your code reaches that point it will never reach anything beyond that point. So if you want to have multiple return possibilities, you need to put your return statements in conditionals, like an if statement. return thisIcon; //code that will never be reached return thatIcon; can never return thatIcon. However, if (butts == true) { return thisIcon; } return thatIcon; //more code that will never be reached if your boolean value butts is set to true, then thisIcon will be returned. If butts is set to false, then thatIcon will be returned. Does this make sense?
-
http://www.minecraftforge.net/forum/index.php/topic,8408.0.html Scroll down to my post. I explain how to figure out what side is where in the world and give an example of how I did it.
-
You need to alter the getIcon method. Mine acts to place a single texture facing the player when the block is placed and the texture of cobblestone on every other side. blockIcon is a variable in my parent class and faceIcon is a variable in my block's class. What you need to do is go through and replace all of my getIcon method with if (side == 0) return faceIcon; return blockIcon; And start the game and see what side your texture shows up on. Make a note of which side it is on your block so you can map out where everything is. Then run it again for side == 1, 2, 3, 4, and 5, then meta 0-3. meta, or the second parameter, is the direction a player is facing when the block is placed. I don't know what happens when the world generates it. Play around with that and you'll figure it out I'm sure. That answer your question? Just add more icons to have more textures on your block.
-
The name isn't final yet. I just wanted something there. This summer I will be spending some time working on a mod with 2 main things that I have found that I really enjoy in other mods: pipes and the energy condenser. However, I will be going about these, and anything else I add to the mod, a little bit differently from most of the mods I have seen. Spoiler is me talking about why I want a less intrusive mod. My energy condenser function will be 2 machines, both powered by coal/charcoal. One will break down materials, probably 64/coal, and give you the value of those items in some form of matter/energy item. This can then be moved to the other machine which will reconstruct the matter/energy into a new resource according to a recipe. Recipes will be crafted using some sort of valuable mineral along with probably paper and the resource to be created. Recipes will have a durability and eventually break. Only certain things will be capable of being converted. Cobblestone will be one, along with valuable minerals. However, organic objects like wood, food, passive mob drops, etc will be nonconvertible. You still need to make your farms. I want my machines to give you the power and convenience of the energy condenser without destroying the purpose and play-style of Minecraft. I have no ideas for pipes yet. I just love them. There will not be any changes to world generation. There will not be tons of new mid-stage items for crafting. No recipe will be more complex than any vanilla recipe. I am posting this here looking for suggestions. Things you like in other mods that don't change the game. Things you would like to see. Possible values for resources to be converted to. Please feel free to give me any advice you have. I would love to make a mod that strikes the perfect balance between modded and vanilla Minecraft and you are the first step.
-
[1.5.2][SOLVED]Multitextured block like a Pumpkin!
redria7 replied to Minemeister01's topic in Modder Support
If you want the texture to change as you move around the block so one side is always facing you, I'm not sure. Maybe set it to update the block on tick depending on the player's location? If you want it like the furnace/pumpkin where it faces you when you place it, keep reading. For the pumpkin feature, I mimicked it on my own a little more clearly than the pumpkin's code. Most important is the getIcon method. The second parameter is the meta data for the block, specifically which direction the player is facing when they place it. This code sets the block facing you when you place it. You can go through and just have single if statements setting one side depending on side or meta so you can figure out the orientation of your block relative to the world and the values of side and meta. Hope this helps! -
You made 2 different knapping stones: private final static Item ItemKnappingStone = new ItemKnappingStone(7500); private final static Item ItemPickaxeheadStone = new ItemKnappingStone(7501); In case you aren't kicking yourself right now because you can't believe you missed it, the proper code would be: private final static Item ItemKnappingStone = new ItemKnappingStone(7500); private final static Item ItemPickaxeheadStone = new ItemPickaxeheadStone(7501); Enjoy and good luck. -edit Also, you may want to (from a coding perspective) start a habit of giving your variables names that start with a lowercase letter: private final static Item itemKnappingStone = new ItemKnappingStone(7500); instead of private final static Item ItemKnappingStone = new ItemKnappingStone(7500); It is small, but there may come a time where you are confused if you are referring to a variable or a class and the distinction will help. Variables start with lowercase and classes start with uppercase. Just being ocd. Take it or leave it.
-
I'm interested in making a block with custom rendering without using a tile entity to avoid adding to lag if a player places lots of it in the world. I know it isn't a big problem, but tile entities do require more space and memory and so slow the game down. If I can make a custom rendered block without it being a tile entity, I'll be happy in a month or so when I start trying to tackle a new block I want. So there is a reason, even if it isn't shucke's reason. Very relevant night for me in the forums...
-
No, but there is a darkness buff that can be applied somehow somewhere. Beacons let you apply buffs to anyone in an area so I could copy over the code from that and have a block cast darkness on anyone nearby. However, it wouldn't be the same as actually changing light levels. Does that make sense?
-
Questions about - Coremods(Still need help), Hooks(Done), and asm(done)
redria7 replied to M1kep's topic in Modder Support
Since I was curious about core mods myself, my interpretation is that core mods alter the source code of minecraft itself. Normal mods just add to it using extra classes. So a normal mod would let you add a new block identical to dirt that emits light. A core mod would let you make dirt itself emit light (which I'm pretty sure normal mods cannot do... but I could be wrong). This is why forge is a core mod. It changes minecraft to allow new classes to be added that register with the existing code. Is that sort of what the difference is?