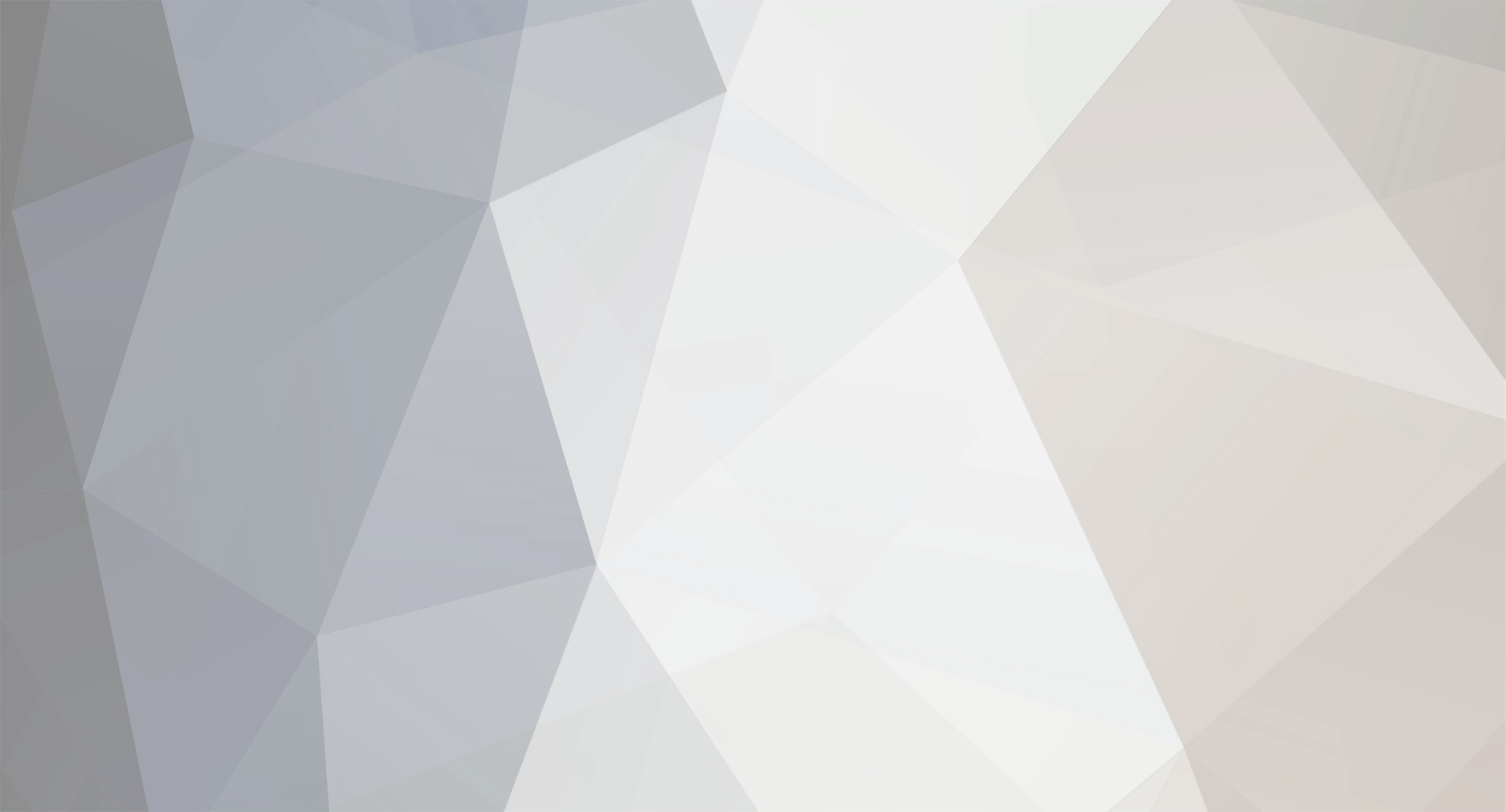
redria7
Members-
Posts
120 -
Joined
-
Last visited
Everything posted by redria7
-
You would have to have coordination between all of your classes of course. This could not be done using vanilla furnaces. You will need to store both the input ID (the id of an iron ore) along with an input quantity (in your case 9) in another hashmap. Then either make a note of when you are adding an iron ore recipe and make it check the quantity in your extra hashmap, or make all of your recipes check the quantity required. Then when you check if an item can be smelted, you have to pass in the itemstack, and if the quantity in that itemstack is larger than the quantity required, it can be smelted. Then when you are actually smelting you need to make a request to your recipe class asking how many of your item you need to perform the smelting operation, then subtract that amount from your input itemstack instead of just subtracting one. Now I'm sure that didn't make any sense. I hope it did, but if not, I can try to explain better.
-
[SOLVED][SLOTS][1.5.1] limitations to only accept blocks
redria7 replied to rasmustof's topic in Modder Support
If Block.blocksList[stack.getItem().itemID] is returning null, that means that the item is not a block, ergo if its not null it must be a block. Ergo allow it (return true). Actually, I feel like this would give array out of bounds errors if you were looking at an item. The blocksList array only has 4096 spaces and item id's go far higher than that, so wouldn't that end up giving you out of bound errors if it isn't a block? -
[SOLVED][SLOTS][1.5.1] limitations to only accept blocks
redria7 replied to rasmustof's topic in Modder Support
@Override public boolean isItemvalid(ItemStack par1ItemStack) { if(par1ItemStack !=null) { if(par1ItemStack.getItem() instanceof Block) { return true; } } return false; } -Fixed. It didn't work because of the return statement. I haven't actually tried to do this, but it should work. -
I'm facing a similar problem. I didn't want monsters to spawn, but I did want to make the world darker. I haven't done anything yet But... My only idea was to have that block force blindness on nearby players, similar to throwing a potion at the players, in whatever way it is that a beacon can do so. To take care of monsters you may want to look into the mob-spawner code and just have monsters randomly spawn using that.
-
Dear god, please please please learn how to use loops. I don't know what shape you are trying to make, but I guarantee that you can do in a couple loops whatever it is this mess is trying to do. Otherwise, don't hard-code in that ID. If, say, you are trying to make a frame using your block, let's call it modBlock in your base mod class called MyMod, even if you declare the ID to be 181, instead compare the world.getBlockID to MyMod.modBlock.blockID, similar to how you reference Block.glass.blockID. This ensures that you are comparing to the right thing.
-
Thank goodness it works this way. I have a block that would be impossible if it worked otherwise. As it is I can't have more than 2 or 3 before it starts freezing the game when they update... partly because of my computer and partly because I still need to work on my optimizations...
-
Changing the color of an item programmatically
redria7 replied to Boxtop5000's topic in Modder Support
Lisp is a functional language, not an imperative language. While you obviously cant only have one way of doing things for everything, I'm sure it is one of the reasons why the parenthesis trick usually doesn't work. I'm sure it could be implemented without too much trouble, but is avoided for other reasons. -
Changing the color of an item programmatically
redria7 replied to Boxtop5000's topic in Modder Support
As someone who has made a parser for a defined language that my teacher wanted us to implement, I can say that there are very good reasons why we cant program using 2(x+1) instead of 2*(x+1). Parsing code is not a simple thing, especially when the language needs to be deterministic. Additionally, a language should ideally have orthogonality. Basically, one way to do something, and one way only, because that makes it easy to read and write. How frustrated would you be to read through someone's code and see them switching between every possible way of writing 2*(x+1) every time they use it? By minimizing the ways that it works, a language becomes more readable. Also, 2(x+1) reminds me more of a method call than a multiplication. Not a good thing. -
MathHelper.floor_double(x), MathHelper.floor_double(y), MathHelper.floor_double(z)?
-
I'm not sure why you are trying to pass in an object array thing when you make your recipe. The recipe format will probably be more like: GameRegistry.addRecipe(new ItemStack(iredantblock), "aaa", "aaa", "aaa", 'a', new ItemStack(YourModItemsClass.iredantcrystal)); Assuming irredant crystal is a variable that has been made somewhere else that you can access.
-
Look at the furnace gui code. It binds the furnace gui texture, but that texture has both the normal gui along with overlays. You simply specify the area you want to be the normal gui, then specify the areas that are overlays and draw them on. The furnace gui code is fairly simple to understand.
-
Instead of using the code from the item renderer, go to your block renderer, get the icon for a sword (you may have to put one in your block textures file? I'm not sure that you can access item textures for a block, but there should be a way), and use a tessellator to add 4 vertices using the sword to draw one image of the sword, then do the same facing the opposite direction. This gives you a sword that can be seen from the sides. There might be a good way to deal with viewing the sword edge-on, but I can only think of drawing in lots of squares to connect the 2 sides. That may not be the best method, but I know you could make it work. Make sense?
-
I'm fighting this at the moment too. I have a block that can render as a 3d model in my inventory (chests can do it!), but I haven't figured out what to do about just a regular item.
-
Packets? I am having trouble with one of my tile entities where I cant update the rendering because no matter what I do to the tile entity, the renderer is accessing an old version of my TE. As in, my TE prints one value for one of my variables and my renderer will say the same variable has a different value. I'm working on learning packets so I can get my client updated. Your problem sounds similar to mine.
-
I'm overriding updateEntity for my purposes. It seems to work well enough.
-
I gave my tile entity a constructor and use the xCoord, yCoord, and zCoord variables int it and all of them just returned as 0. Is there a certain point where they get set, and where I can start using them?
-
What is the best way to tell a tile entity where it is upon placement? Every time minecraft starts, it creates tile entities using the block class that the tile entity comes from. So I can't set up variables that are set on the block's placement, because they would not be initialized when minecraft started, or they would all start the same and all my tile entities of that type would think they have the same location. So how would I tell a tile entity where it is when I place it? My only idea so far is to use my renderer to tell it where it is, which just seems like awful etiquette to me.
-
You have to make sure there is something in kegItemStacks[0] before you access what is inside it: if (this.kegItemStacks[0] != null) { if (this.kegItemStacks[0].isItemEqual(new ItemStack(EatMe.yeast))) { System.out.println("yeast"); return false; } } else { //whatever you want to do if that slot is empty }
-
this.smeltingList.put(Integer.valueOf(par1), new ItemStack[] {par2ItemStack, par3ItemStack, par4ItemStack, par5ItemStack, par6ItemStack, par7ItemStack});
-
I went through, took out the meta data sensitive versions, and I should have handled all the errors you will get from the recipes class. getSmeltingResult will return an array of itemstacks, which can be accessed by using: ItemStack stackNumX = returnedVariable[X]; Where X is a number 0-5.
-
Post the current code for your recipe class, and I'll see if I can walk you through this. Do you understand the doNothing method example I described?
-
Believe me, I can render blocks in the world. I have a blast playing with that and I have some models that I specifically designed to make a viewer uncomfortable and I have a hard time looking at them myself even without finalized textures - which is exactly what I wanted. My problem is item rendering, in the hand and in the inventory. I just want a 2d panel like most items have where the texture is mixed. So I probably need to know how to set up a custom rendering for an item (I know how to do one for a block, which includes holding it hand, but I don't know how for an item) or I need to find a different way of layering the texture. I don't know if it would be a bigger hit on overall performance to just have an extra 150 textures, or to go through the extra processing to draw in custom rendering of a couple textures
-
Go to each of the errors and look at what it tells you logically. I don't want to be mean to you, but you have to be able to look at what the error is telling you and understand what the problem is if you are going to be coding in anything particularly interesting. Likely your problem is that all of your add recipe and get result methods have errors because... Your hashmap variable has changed! Everywhere that variable is used, you have to go and make sure that your changes didn't make your usage of it stop working. For example, if I have a method in one of my classes: public void doNothing(int par1) { //this does nothing } And I use my method doNothing somewhere in that same class, that usage would look something like this: this.doNothing(0); This does nothing, but there is nothing wrong with it either. Then I realize, hey, the integer "par1" serves no purpose in the method doNothing. Why not remove it? public void doNothing() { //this does nothing } Great, doNothing has no errors, everything looks fine. But... my usage of it: this.doNothing(0); is giving me an error. Well, obviously I am trying to give it an integer that it can't accept. I should change my usage to: this.doNothing(); In your case, your put and get methods in your hashmap are probably not working out. This is not because your hashmap is bad, it's because you need to make sure that you are giving it the correct variables. Keep in mind, we also changed the type of output you can expect to get from your hashmap. You should be getting a list now. You have to account for this and pull the item stacks back out of the list that it returns. All the errors should give you a good indicator of what has gone wrong. You have to learn how to read them and solve them independently or you'll never be able to stay off this site and just mod, you will just go back and forth looking for answers right in front of you. Runtime errors, ones that crash your game, will still give you output in the console, but they tend to make less sense, so you will more often want to ask questions here about how to fix those errors.
-
I am currently working on a new item that will be related to a vanilla item. I want to make around 50 of my new item for about 50 vanilla blocks, then I want to have 3 levels of each of my item, making around 150 total new items that I am adding. With this, I want to have a frame and a background relating to my item, then fill in the rest of the texture with the vanilla item texture that it is related to. So for the one relating to cobblestone, it would have my frame, and inside the frame the texture would be filled with that portion of the cobblestone texture. One way to make these textures work is to make 150 textures, one for each of my items. The way I would like to do it is to make 3 frame textures: one for each level I plan to have, then cut out the appropriate filling from the related vanilla block and layer it over top of my textures. So with the cobblestone example, I would have my frame texture, then this process I want would take the center portion of the cobblestone texture and visually paste it on top of my own texture. This would greatly cut down on the amount of icons I would need to make and store to have this item display the way I want. Has anyone done something like this or know how I might try to approach it?
-
Hold on, I actually just realized how hashmaps work in minecraft. If you look at the vanilla furnace recipes class, and look at the meta smelting list, you will notice that the hashmap is created with 2 variables, a list of integers and an item stack. When a list of integers is placed in, it is linked to a corresponding item stack. That list of integers may be searched and it returns the item stack. You want to give the hashmap 2 variables: an integer (the block ID) and a list of item stacks (the outputs). First you have to declare your hashmap to accept an integer and a list: private HashMap<Integer, List<ItemStack>> smeltingList = new HashMap<Integer, List<ItemStack>>(); Then you have to correctly add an integer and a list: this.smeltingList.put(Integer.valueOf(par1), Arrays.asList(par2ItemStack, par3ItemStack, par4ItemStack, par5ItemStack, par6ItemStack, par7ItemStack)); Now you should be able to retrieve that list by giving the hashmap the correct integer. I did not actually try this, but it should work. Does this make sense?