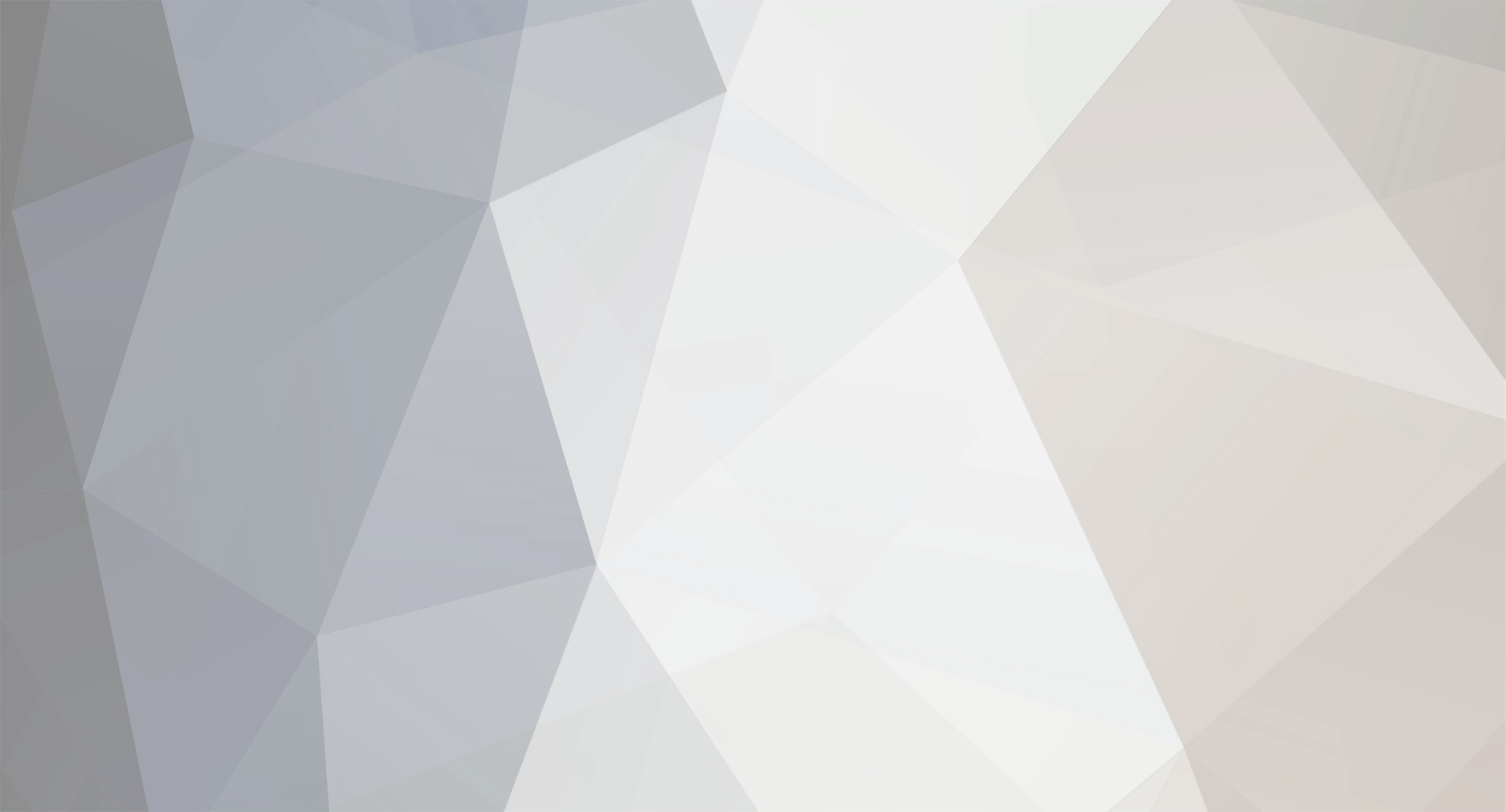
redria7
Members-
Posts
120 -
Joined
-
Last visited
Everything posted by redria7
-
Did you read all of my post? You can't just put in the code I posted. You have to use hashmaps correctly. The put method only takes 2 variables. You are trying to give it 7. You need to give it 2 variables. I just showed you one way where that second variable might contain the 6 variables you actually want to keep: using an array of item stacks.
-
Post the new code in your recipe class here.
-
The problem is exactly what the error is telling you. Your variables smeltingList and experienceList are both hashmaps that take an integer, the input item ID, and give a related output: the resulting item stack for smeltingList and a float number as experience from experienceList. The method put gives the hashmap the variables it needs to make those connections, namely an integer and an item stack or an integer and a float. Instead, you are trying to put in 6 item stacks and 6 float values. Your hashmaps are not set up to recognize this. You need to change the declaration for your hashmaps to something that will store the information you need and utilize the put method accordingly. I'm not too sure how hashmaps work, but I think you should be able to declare your hashmaps using lists or arrays as the output. So instead of having: private HashMap<List<Integer>, ItemStack> metaSmeltingList = new HashMap<List<Integer>, ItemStack>(); private HashMap<List<Integer>, Float> metaExperience = new HashMap<List<Integer>, Float>(); You could maybe instead use: private HashMap<List<Integer>, ItemStack[]> metaSmeltingList = new HashMap<List<Integer>, ItemStack[]>(); private HashMap<List<Integer>, Floa[]t> metaExperience = new HashMap<List<Integer>, Float[]>(); Then when you utilize the put method, you would pass in the item ID of the ingredient, then an array of the 6 outputs, or an array of the 6 experience values. There is probably a better way to do this, but that is what your problem is.
-
Awesome! My vision is coming together... Haha. I don't think there are any extra blocks, there are just 4 block wide rows that are being mirrored. Which means that normally that mirroring happens in code that I removed, or something else is going wrong. Like I said, it just needs lots of testing, which I can do. Those 2 lines of code simply fill empty space with water.
-
That is part of the replaceBlocksForBiome method. I commented out the point where that method is actually used since it changes a lot of block in ways I don't want them changed. It looks like that specific section first checks the height and block data of a block, and if it is below 63 and an air block, it is replaced with either water or ice depending on the temperature. It normally would be used just after terrain is generated, before caves and ravines etc are generated, so it doesn't fill in caverns, it just fills in any low areas with ice or water. I will probably clean out that method so I can use it to generate normal bedrock, but have that be the only thing it does. It currently has no effect on generation. I am curious about what the problem is, since the same code seems to generate normally in the overworld but goes all funky in my dimension. There's no solution like trial and error, so if I figure it out, I will come back here and post again. In the mean time, do you know if there is a way to mute out sounds? like, as soon as you enter this dimension, all noises are silenced until the player leaves the dimension?
-
I think he made another thread for just himself. I accidentally hijacked this one... As far as setting hasNoSky to true, I considered it, and I am considering it more now as I just made it so my base stone has its own low light level, but doesn't that just prevent all light that previously came from the sky? Also, I think I may have seen a place where it halved the world height to have no sky, but if that's the case I would just override it of course. My Chunk Provider gen code, since I do not have a github, is: It is almost exactly the same as net.minecraft.world.gen.ChunkProviderGenerate.java. The only things that have been changed is filling the world with my stone, preventing the game from placing water, and preventing the game from building and sort of structure or adding any of the normal decorations. I wonder if maybe the problem is related to my just commenting out the one line that adds water, but that doesn't make much sense. I wish there was an easy way to determine if all sections below level 64 have an equal chance of being flipped or if it is just water.
-
Fair enough... The error in a river. Another place without the text. A river basin. The right side is good but the left side clearly isn't. An ocean floor. Interestingly, the top portion that is clearly not broken ends at level 64. This would explain why it only happens around water: nowhere else is under level 64. And of course an ocean generated ravine that got slightly mangled. Looking again, it seems that 4 block sections are just being mirrored, giving striations to the generation.
-
Mostly? I just changed my filler block id to 255, so the dimension is created properly with that, and most of the biomes generate correctly. However, any biome that includes water, like the ocean biome or a river etc, seem to have a strange issue. If you took every 4th block and squished them all together, then the next set, then the next, etc in one chunk, that is how it would look, except mirrored. So what would be a hill (left to right) has (still left to right) a high, high-middle, low-middle, low block, then repeated. I could post a picture but I should realistically be able to figure it out myself. Or I might not and leave it because it makes cool patterns.
-
I am using a chunk provider. Vanilla chunks have a constructor that allows them to be built using a short array instead of a byte array, which allows you to use block ids over 255 when creating the chunk. I must have just not changed enough when I tried it on mine.
-
Hahaha I like you. Thank you tons for looking into your code for me. I like this community. At the moment I am thinking of just preventing natural spawns of all mobs. I'll check your solution and get back to you. -edit- It works- and I should have noticed that method way earlier. Haha. Now, I said I figured it out on my own, and I may yet do so, but did you use your chunk provider to fill the world using a custom block? Because I thought I found the solution: making a chunk using a short array instead of a byte array, but there is obviously fancy math that I need to fix for that to work because the wrong block spawned and layers of the world spawned in sideways, very far-lands-esque.
-
Go to your portal block class. Look at your onEntityCollidedWithBlock method. In there is a bunch of code that is used to teleport the player to your dimension. If you want to have the player be teleported via a different activity, move all the code from that method into a new method. In your instance, you probably want to override onBlockActivated and put the code in there. Keep in mind, this still builds your portal the same way when you enter your dimension. If you want to change the way that is built, look at the custom teleporter class you made. And cry. Then if you still want to try to change it, start working on understanding what everything does. And cry. And give up and comment out everything already done and make some silly code that does what you want.
-
His update to 1.5.1 post helps a lot, or in the class where you have the error, open up the class that yours extends (ie, for your custom teleporter class, open up the vanilla Teleporter class) and compare the vanilla methods to yours and change yours to match the vanilla one where you have errors. Or just copy them over. They do the same thing. Ooh, that looks helpful. Yeah, I'm using all the vanilla biomes. My world generates *almost* identically to the normal world. Though in some places, sections of the world are mirrored. It's really strange.
-
I actually could use some help. I'm not really sure about several things. I think I just solved my own problem regarding block id's greater than 255, but I am having trouble in other areas. How should I go about preventing any mob spawns in my new dimension? I have tried using: provider.setAllowedSpawnTypes(false, false); in several places and that doesn't seem to change anything, but it is the only thing I can find to indicate whether a mob spawns. I am not using any custom biomes and would prefer to do my changes to the world instead of the biomes. Also, Do you know of a way to set a light level that is constant through day and night, or even just a way to set the skyblock light level? I'm sure I can figure out how to texture my sky how I want etc, but I'm having trouble with the light level. If you remember any of this from when you made your dimension, thanks would be given freely.
-
CJ, you are trying to be helpful, but new dimensions unfortunately aren't simple enough to just look up (at least not to me). Delta, if you are serious about making this mod, you will want to look up wuppy's tutorial on dimensions. All of wuppy's tutorials are listed here: http://wuppy29.blogspot.com/2012/10/forge-modding-142.html and if you scroll down into the text tutorials, there is a heading called "Dimension". Start with the first tutorial and follow it through all 7 tutorials to the end. The tutorial itself doesn't take too long since there is a lot of copy-paste. Once you finish that, there are 2 more headings under dimension: dimension customization and other. Look at dimension customization because he shows you a couple neat tricks to use, then go to other and look at updating to 1.5.1. His dimension tutorial is old so if you can't figure out how to fix the outdated methods and names he uses, it's a good place to start. This is the most up to date tutorial I've been able to find, and since it isn't a short process it will probably stay that way. Dimensions don't look too easy to me at the moment, so be aware that it takes patience and you can do it.
-
Hahahaha computer science. There's actually a well hidden bug that looks at your code, overrides a portion, and tries to make you feel dumb until you change nothing then lets you be about your business. Enjoy. Glad it works now.
-
That's not the problem: mine does that too. Someone else on here told me what it is and I forgot, but it isn't our problem. I honestly don't know what the problem is. You seem to be telling it where to look and I can't think of any reason it wouldn't be listening. Try putting in a print statement to make sure the registerIcons method is being used, and maybe try splitting up your path name into 2 strings: blockIcon = iconRegister.registerIcon("DennisMod:" + "manganeseore"); 2 strings shouldn't make a difference but you can never be too sure. The print statement would be a good indicator of a different kind of bug.
-
Actually you need to return -1 in the idDropped method if you don't want to drop anything. BUT my method would work (right?). It is harder, slower, and less logical, but you should get the same result. Unless the random variables are identically seeded in which case it would fail catastrophically. Just return -1.
-
Exactly. I just bugged mine up and it spit out: As you can see, mine went into my mods folder, into my mod, etc looking for my texture. Yours did not. We need to figure out why. Are there any other warnings? I'm not really sure why it isn't looking down the full path. Is your mod folder called DennisMod exactly?
-
That's strange if everything else is working fine. There should be a warning being printed out in your console. It will tell you the file path it tried to find your texture files in. Compare that to your actual path. Also, items will need to be stored in textures/items, and blocks will need to be stored in textures/blocks. That may not be your problem but double checking your file path and the names of your textures themselves are a good first step if you haven't done so already. If you have, I don't know where to start...
-
The de-compiled mod clearly should not work because I don't know of any instances in which k will ever equal ... Often times it is very easy to use code already made in Minecraft and back-track using that. For instance, I've never messed with drops in my mod, but I know that lapis lazuli drops a random number of ores. So I went to the Block class, found where lapis lazuli was instantiated, and followed that to the BlockOre class and looked at the quantityDropped method to see how that was done. You are completely right to use the idDropped method. Here you want to use the random passed to you to generate a random integer ranging 0-5. A small framework for you... Unfortunately, I haven't played with this at all and I'm not sure what you want to do to make it drop nothing. Maybe make it always drop one of those 5 items and override the quantityDropped method and use the random variable passed in to make it have a 1 in 6 chance of dropping 0, and drop 1 the other 5 times, similar to what we did in idDropped. If you want help with that let me know but I bet you can figure it out.
-
I don't think the problem is your path anymore. I'm not sure how much I can help without seeing your model class. When you pasted your model class you actually just pasted your ItemParticle class again. What catches my eye in your renderer class is particleModel.render((Entity)data[1], 0.0F, 0.0F, 0.0F, 0.0F, 0.0F, 0.0625F); You have lots of zeros in there and without seeing what the method does in your model class I'm not sure if those zeros aren't making your model come up as a single point instead of a cube. Assuming you did that right, go into your renderer class and go to one of your switch cases where you actually render your item. Add in some sort of print statement (if you can figure out where it prints: I haven't yet and it bugs me to no end) or add a set of statements that will result in an error: I make a small array and intentionally get an out of bounds error. If your statement prints/your game crashes when you have the item in the case you trapped, then you do know that it is being used, just not correctly. If it doesn't trigger, then you know that it isn't being used which would explain it not showing up. Let us know if all of your rendering code is being run and send us your model class.
-
Try drawing in the opposite direction. The direction you start drawing (clockwise or counter clockwise) makes your texture face a certain direction. Your sun is probably just facing away from you so you can't see it. So: tessellator.addVertexWithUV(-size, 100.0D, size, 0.0D, 1.0D); tessellator.addVertexWithUV(size, 100.0D, size, 1.0D, 1.0D); tessellator.addVertexWithUV(size, 100.0D, -size, 1.0D, 0.0D); tessellator.addVertexWithUV(-size, 100.0D, -size, 0.0D, 0.0D); This should fix your problem.
-
I'm not sure why you made this a new post when I'm pretty sure it's the same problem you had in http://www.minecraftforge.net/forum/index.php?topic=8862.0 but... You seem to have taken the route of using one texture that you rotate using the renderer by telling the game to render your block as a piston base. This is baffling to me. I'm not even sure how you got your block working as well as it is. You are making this way more complicated than it needs to be. Go look at the BlockBasePressurePlate class. The important method is the setBlockBoundsBasedOnState method. Following that to its helper method you will see that it changes the size of the block based on a set of data you can specify. Specifically, the method setBlockBounds is used. Call this in the constructor of your block and use it to set appropriate bounds for your block. This will can you that nice flat block you seem to be trying to make. Now go back to your old topic and look at the last post I made. When you set your meta data you should use that onBlockPlacedBy because it sets your blocks to face you. This means they face you in your hand, inventory, etc. The meta data ranges from 0-3 depending on which direction you are looking. Now compare your getIcon method to mine. Mine uses 4 different top textures. You should be able to go into your image editor and just rotate the image by 90 degrees at a time so you have 4 images that face 4 directions. Now, your method uses a lot of checks that all return the same icon. That defeats the purpose of making the checks when they all return the same thing. I can (visually) make your block in 30 minutes. You can do it: I believe in you. Stop making it so difficult! EDIT I looked again and you do set block bounds already. But I don't understand them either, since they describe a block that is almost full height instead of a block that is super thin. I really don't understand how you have your block working as it is right now.
-
onBlockActivated not working (Changing from one block to another)
redria7 replied to dude22072's topic in Modder Support
@Override public boolean onBlockActivated(World par1World, int par2, int par3, int par4, EntityPlayer par5EntityPlayer, int par6, float par7, float par8, float par9) { if(par5EntityPlayer.getHeldItem() == null) { return super.onBlockActivated(par1World, par2, par3, par4, par5EntityPlayer, par6, par7, par8, par9); } if(par5EntityPlayer.getHeldItem().itemID == LOZmod.swordMaster.itemID) { par5EntityPlayer.getHeldItem().stackSize -= 1; //only if you want to use the item when you do this. if(par1World.isRemote) { par1World.setBlock(par2, par3, par4, LOZmod.pedistalSword.blockID); } return true; } return false; } I don't know why your block isn't changing, so unfortunately I can't help any further in that regard.