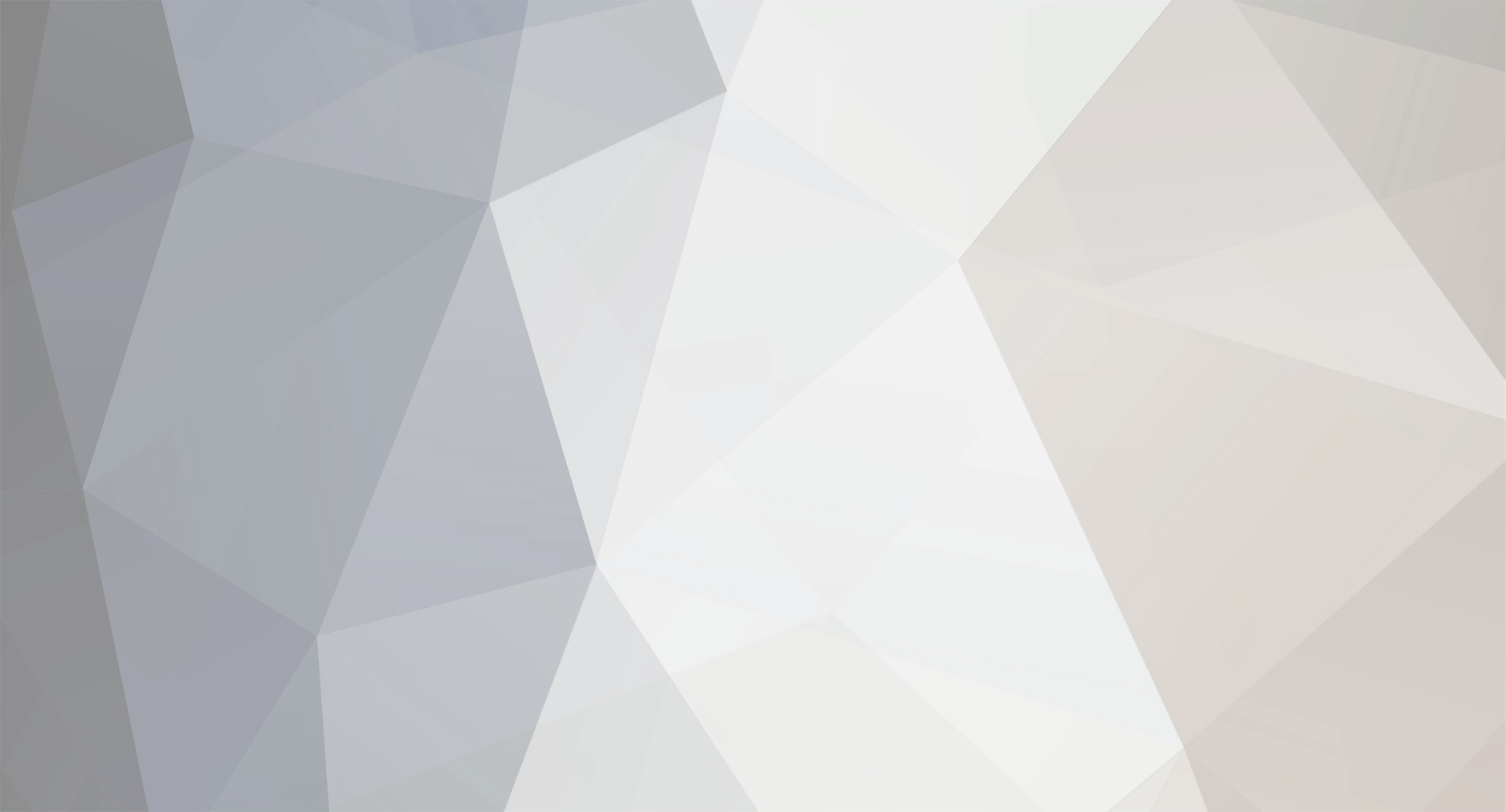
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi The basic problem is that it takes time for the Mob to get where it's going and you have to let the game run while you wait for it to get there. For some background info see here: http://greyminecraftcoder.blogspot.com/2013/09/the-minecraft-main-game-loop.html I would suggest a couple of ways: the .onUpdate is called every tick, so you could put your code in there to see whether the mob is within range of the target position (or if too much time has elapsed, give up). ForgeHooks.onLivingUpdate(this) might be a good spot. Probably better: create your own AI task to do it. See EntityAIMoveTowardsTarget for inspiration, also EntityMoveHelper. A good tutorial to start with is http://wuppy29.blogspot.com.au/2012/11/modding-142-basic-mob-part-3-ai.html -TGG
-
How to make an item change on right click
TheGreyGhost replied to SkylordJoel's topic in Modder Support
Hi I'd suggest: Override Block.onBlockClicked or Block.onBlockActivated to change the block metadata. You'll also need to call World.markBlockForUpdate to make sure that the new texture is drawn. Override BLock.getBlockTexture to return a different texture based on the metadata. If you're not familiar with metadata, this might help http://greyminecraftcoder.blogspot.com.au/2013/07/blocks.html and -TGG -
Hi Try putting a breakpoint into SimpleReloadableResourceManager.getResource The problem seems to be that the root you have specified doesn't match the ones that Forge has registered. ResourceManager resourcemanager = (ResourceManager)this.resourceManagers.get(par1ResourceLocation.getroot()); par1ResourceLocation.getroot() is yours, the valid ones are in the resourceManagers Map. If you look through the map you might find a clue why your root isn't matching any. -TGG
-
Hi Do you mean World.getBlockID? The basic algorithm would look something like for z = -10 to 10 for y = -10 to 10 for x= -10 to 10 if (x*x + y*y + z*z <= radius*radius) if World.getBlockID(x+mobx, y+moby, z+mobz) == myBlockID {etc} Is there any reason you need to return an array of all the block locations, i.e. can't you just pick one (eg the closest?) -TGG
-
Hi The direct answer to your question is that the TileEntity knows its [x,y,z], so you can access the block at [x,y,z] using World.getBlockID() and World.getBlockMetadata() But I think that this probably won't help you much, because every block of that type has the same bounding box. I think what you're asking is backwards - i.e. the Block should be asking its TileEntity what the bounding box is, since the TileEntity stores that information, not the block. Why do you want to change the bounding box, i.e. what effect are you trying to achieve? Your TileEntity ticks every time so you can just tick the block from the TileEntity tick-? Lots of vanilla code uses scheduleUpdate, but I'm not sure it's really what you need. -TGG
-
Hi If your Item's custom renderer uses an icon from the block texture map, make sure that Item.getSpriteNumber() returns 0, otherwise the tessellator will render using the item texture map, i.e. the wrong icons will be used. See also http://greyminecraftcoder.blogspot.com.au/2013/09/custom-item-rendering-using.html -TGG
-
Private Variable To each block of same type
TheGreyGhost replied to pollitoyeye's topic in Modder Support
Hi The important to remember is that there is only one of each Block. You don't get a block2 for every block [x,y,z] location in the world, there is only one block2. There are two ways to store unique information for every block location: (1) If you only need to store up to 16 different numbers, you can use block metadata. (2) If you need more than 16 different numbers, use TileEntity. A quick search on the forge tutorials will show you examples of how to use each one. http://www.minecraftforge.net/wiki/Tutorials -TGG -
Hi I suspect this might be a bit harder than it seems at first, because TileEntities are loaded in and out of memory as the player moves around. Luckily, I reckon it's probably unnecessary to store this information in the TileEntities. I think your best bet is to write some code which searches all the chunks within a certain radius of the player for Teleportation TileEntitys. You would call this code when the user opens the "receiver selection" GUI. -TGG
-
[SOLVED] Current Item Change & Chat Send Event
TheGreyGhost replied to Zelnehlun's topic in Modder Support
Hi I think it might be rather difficult to be honest. I don't know of a way to intercept the call to Keyboard.getKeyDown to stop 0..8 from changing theplayer.inventory.currentItem. You might be able to put some code into FMLCommonHandler.instance().onPostClientTick(); to check whether the currentItem has changed, and somehow figure out whether 0..8 caused it, and if so undo it. I doubt this would be robust unfortunately. There seem to be more opportunities to intercept a chat message to the server, in each case it would mean extending one of the base classes and replacing references to it in the code; for example class MyEntityClientPlayerMP extends EntityClientPlayerMP { @override public void sendChatMessage(String par1Str) { if (par1Str == "mycommand") { // do my command here } else { this.sendQueue.addToSendQueue(new Packet3Chat(par1Str)); } } } and somewhere in your code you would need to set Minecraft.getMinecraft().thePlayer = new MyEntityClientPlayerMP(Minecraft.getMinecraft().thePlayer); where you write the copy constructor to copy the EntityClientPlayerMP into your MyEntityClientPlayerMP. Disclaimer: it looks like this should work but I've never tried it. -TGG -
Hi Here is some background information that might be useful... http://greyminecraftcoder.blogspot.com.au/2013/10/user-input.htm Three choices occur to me.. either change the keybinding, replace the KeyBinding class with your own to swap the settings before they get passed to vanilla code (http://gist.github.com/TheGreyGhost/7033821), or overwrite EntityPlayerSP.movementInput with your own class. -TGG
-
Hi I'm not sure why, but it should be pretty straightforward to figure it out if you put breakpoints or println into the various BlockSand methods, and see if you can figure out what is happening differently to the vanilla sand. -TGG
-
Hi Try looking in EntityPLayer.attackTargetEntityWithCurrentItem(). It suggests a few possibilities - for example the Forge event AttackEntityEvent SharedMonsterAttributes.attackDamage EnchantmentHelper.getEnchantmentModifierLiving -TGG
-
Hi you could try if (par5EntityPlayer instanceof EntityPlayer) { par5EntityPlayer.motionX *= -10D; par5EntityPlayer.motionZ *= -10D; } see here for more background information on Entity inheritance hierarchy... http://greyminecraftcoder.blogspot.com.au/2013/10/the-most-important-minecraft-classes_9.html -TGG
-
Hi This link gives some information about how Minecraft reads user input and turns it into actions, might be useful perhaps. http://greyminecraftcoder.blogspot.com.au/2013/10/user-input.html As for the API - if you want to ship the API as part of your mod, I would put it inside your mod package, but I reckon you could make it work either way. -TGG
-
Hi I don't know why, but I suggest: add a breakpoint in Block.harvestBlock() and step through until you find out where the metadata is being discarded. It should be pretty easy to track? -TGG
-
[Partially Solved] HELP: Strange PlayerEvent Phenomenon
TheGreyGhost replied to MrArcane111's topic in Modder Support
Hi So you are saying that in all cases it executes both of the if statements in playerFallen, and adds 0.069 to the player.motionY. But adding 0.069 has no effect on the actual jump height unless you are breaking a block while jumping? -TGG -
Hi Wow that looks pretty impressive even if it wasn't the effect you were looking for!! Looks like a tricky problem. I think the issue is that you're trying to interpolate across four dimensions (R,G,B, skylight) in something that's only represented in two dimensions. I'm not familiar with OpenGL at all, but your idea of multitexturing with a total of three lightmaps (R * skylight, G * skylight, B * skylight) sounds like a good idea to try. I also notice that textures can have up to four dimensions which might be another way to get the GFX card to do linear interpolation across four dimensions for you. Don't ask me how because I'm just guessing! Interesting challenge... -TGG
-
[1.6.4] Problem adding a String to the NBTTag
TheGreyGhost replied to MagicHaze's topic in Modder Support
Hi Here is some more information about Packet Handling in vanilla, which might be useful for background information. http://greyminecraftcoder.blogspot.com/2013/10/client-server-communication-using.html I think your best bet is to use the custom packet as shown in the tutorial you already looked at. On the client, send the new code to the server along with the [x,y,z] of the TileEntity using Packet250 On the server, find the TileEntity with that [x,y,z] and set the new code, then send the TileEntity information back to all the Clients using Packet132 (for example see TileEntityMobSpawner.getDescriptionPacket(). (The method name is misleading.)) -TGG -
Awesome :-) Good luck with the AO calcs, they did my head in. -TGG
-
Hi Unfortunately I don't understand at all what you're asking. Could you add some more explanation and perhaps the code that you're talking about? -TGG
-
[SOLVED] Change block properties in an if statement.
TheGreyGhost replied to Flayr's topic in Modder Support
Hi It would help if you showed us the crash error message... :-) If it's only just started when you added the renderer code, it's probably a Null Pointer Exception on the item, try ItemStack heldItem = Minecraft.getMinecraft().thePlayer.getHeldItem(); if (heldItem != null && heldItem.getItem().itemID == myLightStaffID) { -TGG -
Hi Here's a little bit more background information, although it sounds like you might know it already. http://greyminecraftcoder.blogspot.com.au/2013/08/lighting.html The lightmap texture is set up in EntityRenderer.enableLightmap() The size is 256x256 (since the blocklight and skylight values are multiplied by 16 when they are stored in the "mixed brightness" number), i.e. each of the 16 blocklight x 16 skylight combinations has a 16x16 texels patch which is all the same colour. ( float f = 0.00390625F; --> 1/f = 256, i.e. the texels are scaled to each be 1/256 wide x 1/256 high) I suspect (but I'm not sure) that the 16x16 texels patches is necessary because the block textures are also 16x16 texels in size. Not sure how all this will help you, but if you figure it out I'm keenly interested... -TGG
-
Hi So I gather your entity is that big black shape on the left? When the entity disappears, do you know for certain that the renderer has drawn it? I.e. perhaps it is being culled by the frustrum code and it never gets to the renderer? (I suggest adding some println to check) I can't see anything wrong with the render code but then I'm no expert. I've previously had rather strange bugs where my render varied depending on what else had previously been rendered in the same scene, because I wasn't setting all the necessary settings. Fresh out of other ideas, sorry... -TGG
-
[SOLVED] Change block properties in an if statement.
TheGreyGhost replied to Flayr's topic in Modder Support
Hi oops! This time I'll type it into my code and make sure it compiles!.... if (Minecraft.getMinecraft().thePlayer.getHeldItem().getItem().itemID == myLightStaffID) { // staff is held so render it } -TGG