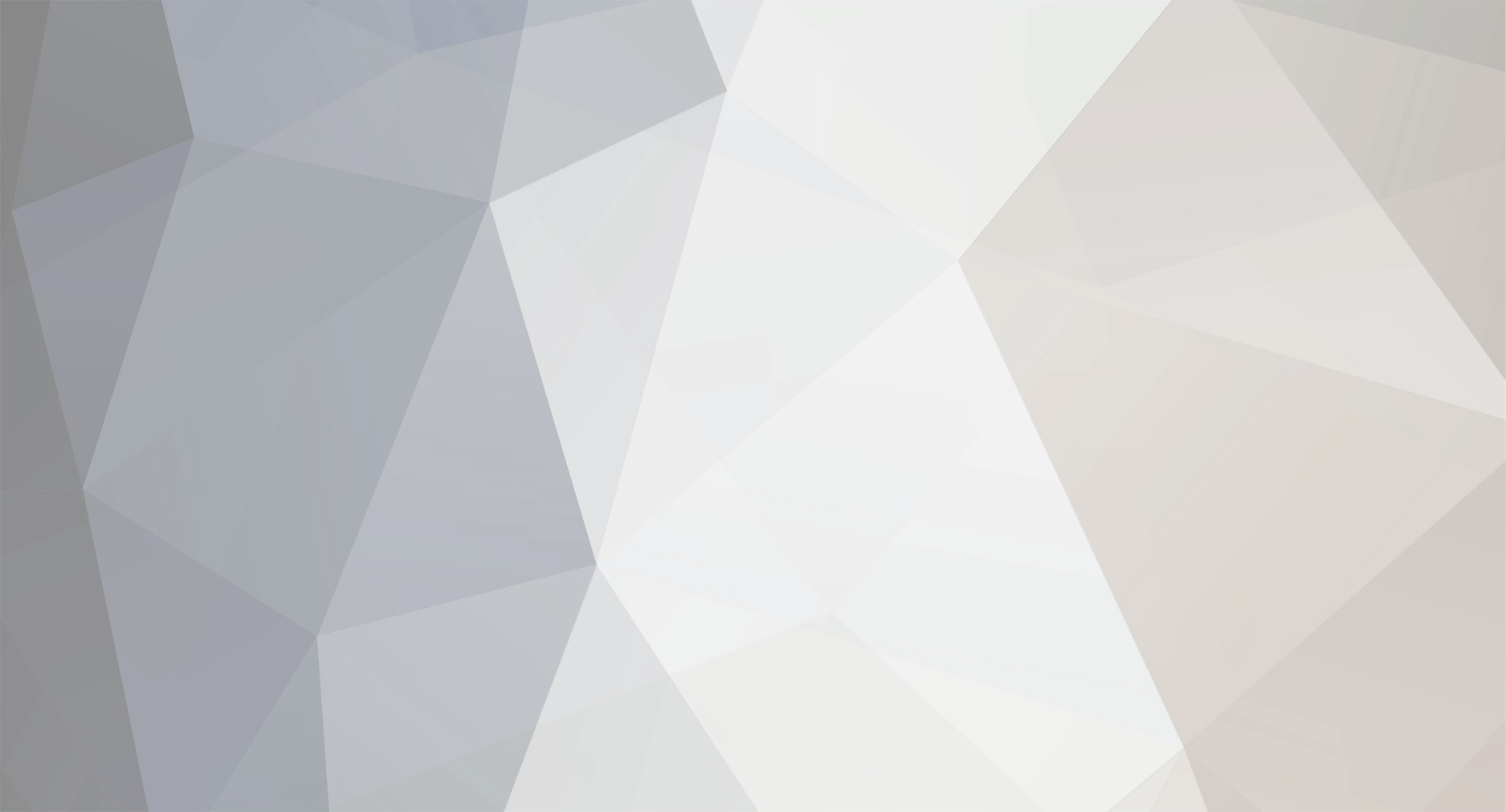
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi It looks to me like the ol' rendering-order-is-important-for-alpha-blended-blocks problem. http://www.minecraftforge.net/forum/index.php/topic,22368.msg113450.html#msg113450 Your alpha-blended pane is drawing before the water, and it is also writing to the depth buffer. So when the water is drawn afterwards, it is behind the pane and gets culled. You can turn off writing to the depth buffer while rendering your pane, using GL11.glDepthMask(false);, but that probably won't help much because then the water will render over the top of your pane which will probably look rather strange. The only way to correct this properly is to make sure that your panes are rendered last. Unfortunately this will only work when the pipe is the closest alpha-blended object to the player, so you will need to hope that your pipes are never placed behind another alpha-blended object. There may not be an easy answer unfortunately. It is for sure possible if you grasp deep into the rendering code to sort all the pass 1 rendering by depth. I'd suggest trying the depth mask fix or the render-last fix and seeing if you can live with one of them. -TGG
-
Hi This line here io.netty.handler.codec.DecoderException: java.lang.NullPointerException: Undefined message for discriminator 0 in channel zeldamod means that you haven't registered your message properly INSTANCE.registerMessage(PacketOpenZeldaInventory.class, PacketOpenZeldaInventory.class, 0, Side.SERVER); I'm guessing either you didn't call init, or you didn't register the message handler on the receiving side. -TGG
-
Error when installing Forge: The assetDir is deprecated!
TheGreyGhost replied to qpwoeiruty's topic in Modder Support
Hi Well at least it works The gradle script downloads files to a cache which you might not have noticed. When you install the second time it checks the cache and doesn't download files it already has -TGG -
Error when installing Forge: The assetDir is deprecated!
TheGreyGhost replied to qpwoeiruty's topic in Modder Support
Hi What makes you think it isn't working? My install doesn't have a root bin folder either. What installation guide are you using? This one is good I think http://www.minecraftforge.net/forum/index.php?topic=14048.0 and this one http://minalien.com/tutorial-setting-up-forgegradle-for-intellij-idea-scala/ -TGG -
[1.7.10] Making a block that you can walk through.
TheGreyGhost replied to Noodly_Doodly's topic in Modder Support
Hi Those methods you mentioned are for rendering only, not collision. Try this method from BlockTorch (you can't collide with a torch!) /** * Returns a bounding box from the pool of bounding boxes (this means this box can change after the pool has been * cleared to be reused) */ @Override public AxisAlignedBB getCollisionBoundingBoxFromPool(World world, int wx, int wy, int wz) { return null; } -TGG -
Hi I'd suggest you put a breakpoint or some System.out.println() in here to help you narrow it down, eg public void randomDisplayTick(World world, int x, int y, int z, Random random) { TileEntityCampFire tileentityCampfire = (TileEntityCampFire) world.getTileEntity(x, y, z); //here- print tileEntityCampfire if(tileentityCampfire != null) { //here - print isBurning and .burning(....) if(tileentityCampfire.isBurning() && tileentityCampfire.burning(world, x, y, z, true)) -TGG
-
Hi The easiest way is to iterate over a cube and for each [x,y,z], calculate the squared distance to the centre of the circle. If it's less than the squared radius, it's in the sphere. If more, it's outside. eg for x = centre minus radius to centre plus radius for y = centre minus radius to centre plus radius for z = centre minus radius to centre plus radius { squareDistance = (x-centre)^2 + (y-centre)^2 + (z-centre)^2 if squareDistance <= (sphere radius)^2 then set block stone } Don't worry about being efficient. Java is so fast you'll never notice the difference no matter how much you optimise it. -TGG
-
Error when installing Forge: The assetDir is deprecated!
TheGreyGhost replied to qpwoeiruty's topic in Modder Support
Hi I think you can safely ignore it, I see it too and my installed Forge work fine. -TGG -
Well I've spat the dummy and used Reflection get and set instead. After a bit more testing I'm reasonably sure it's an FML bug related to (re)obfuscation, but maybe that's just sour grapes talking. Anyway I don't need the access transformers any more. If you know what went wrong, I'm interested to hear, but don't waste time trying to figure it out for my sake:) -TGG
-
[1.7.10] Making in hand items & armor opaque?
TheGreyGhost replied to Crow's topic in Modder Support
ROFL well to be honest it might crash and burn for all I know, never tried it and I'm just making it up on the fly.. If it's any consolation I think your armour looks good opaque anyhow. -TGG -
[1.7.10] Making in hand items & armor opaque?
TheGreyGhost replied to Crow's topic in Modder Support
OK, I get it now; yeah translucent or semi-opaque or partially transparent. Anyway, I have to admit I'm not sure. The armor rendering code is based in RendererLivingEntity.doRender, in this bit for (int i = 0; i < 4; ++i) { j = this.shouldRenderPass(p_76986_1_, i, p_76986_9_); if (j > 0) { this.renderPassModel.setLivingAnimations(p_76986_1_, f7, f6, p_76986_9_); this.renderPassModel.render(p_76986_1_, f7, f6, f4, f3 - f2, f13, f5); if ((j & 240) == 16) { this.func_82408_c(p_76986_1_, i, p_76986_9_); this.renderPassModel.render(p_76986_1_, f7, f6, f4, f3 - f2, f13, f5); } if ((j & 15) == 15) // etc and in particular, shouldRenderPass calls your getArmorTexture with type set to null, and then func_82408_c calls your getArmorTexture with type set to "overlay" i.e. public String getArmorTexture(ItemStack stack, Entity entity, int slot, String type) shouldRenderPass() is misleading name by the way. It iterates through each piece of armour and returns flags for whether it's coloured, has an overlay, and whether it has a magical glint. You could perhaps try turning on the blend function GL11.glEnable(GL11.GL_BLEND); in your getArmorTexture (null). But that might make any other armor items look a bit odd, unless you turn if off again in getArmorTexture("overlay"), which means your armour type would have to be cloth (getColor returns -1). It's a bit of a hack but it might work: for (int i = 0; i < 4; ++i) { j = this.shouldRenderPass(p_76986_1_, i, p_76986_9_); // calls getArmorTexture(null) ... GL11.glEnable(GL11.GL_BLEND) in here // returns 16 or 31 because getColor returns -1 if (j > 0) { this.renderPassModel.setLivingAnimations(p_76986_1_, f7, f6, p_76986_9_); this.renderPassModel.render(p_76986_1_, f7, f6, f4, f3 - f2, f13, f5); // render your translucent armour if ((j & 240) == 16) { this.func_82408_c(p_76986_1_, i, p_76986_9_); // calls getArmorTexture("overlay") ... GL11.glDisable(GL11.GL_BLEND) in here // return a fully transparent texture so nothing is drawn this.renderPassModel.render(p_76986_1_, f7, f6, f4, f3 - f2, f13, f5); } if ((j & 15) == 15) // etc -TGG -
Issue with Loop using onBlockAdded [FORGE 1.7.10]
TheGreyGhost replied to Sethq's topic in Modder Support
Hi It looks like you're converting code from 1.6.4 or before; don't use IDs any more, compare them to the blocks instead eg Block block = world.getBlock(x + i, y + j, z + k); if (block == Blocks.flowing_water) //etc BTW it looks like you're accidentally swapping x and y sometimes int flag1=Block.getIdFromBlock(world.getBlock(i+y, j+x, k+z)); -TGG -
[1.7.10] Making in hand items & armor opaque?
TheGreyGhost replied to Crow's topic in Modder Support
Hi Could you show some screenshots - how your model should look, and how it actually looks? I'm not sure we both have the same meaning of the word "opaque" -TGG -
[1.7.10] Making in hand items & armor opaque?
TheGreyGhost replied to Crow's topic in Modder Support
Hi You mean transparent some of the time, and opaque the rest of the time? Do you know how to use blending and alpha values? Minecraft generally uses GL11.glEnable(GL11.GL_BLEND); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); and GL11.glDisable(GL11.GL_BLEND); If you're going to change these modes, it's a good idea to wrap it in try { GL11.glPushAttrib(GL11.GL_ENABLE_BIT); // save the current modes // change modes here, do your rendering } finally { GL11.glPopAttrib(); // restore the previous modes } What does your armor rendering code currently look like? -TGG -
Hi Could anyone point me towards a mod on GitHub or similar which uses Access Transformers? (private ->public for a field) I've created an access transformer config file and it worked fine for the dev workspace but when I build to a jar and load it in a release environment, the field is still private. I am about ready to explode trying to get them to work; I am using the FMLAT META-INF method, and FML is loading the rules ok but it just doesn't seem to apply them no matter what combinations I try. Based on the logs I know it's loading the three rules out of the access transformer file, and they are parsing correctly. But it seems they are silently not applying. Further information: If I create a new Forge development environment, place my released mod into the eclipse/mods folder, then debug or run, it applies the access transformation no problem. (It crashes soon after because all the names are obfuscated, but that's not the point...) Some relevant error logs -TGG
-
[Solved][1.7.10] Tile entity is not loaded on server
TheGreyGhost replied to EmperorZelos's topic in Modder Support
Hi So just to be clear: 1) when you place it, you get "is Remote" 2) after you save and quit and reload, you get no console messages at all. 3) you never get any "isn't Remote" at all Is that right? -TGG -
[1.7.10]Making an Item render like a block
TheGreyGhost replied to theOriginalByte's topic in Modder Support
Hi WIth an IItemRenderer, or (simpler) an ISimpleBlockRenderingHandler. This link might help http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html see the Item Rendering sections. -TGG -
[SOLVED][1.7.10] TileEntity var undefined?
TheGreyGhost replied to ryancpexpert's topic in Modder Support
Hi var1 and var2 are not defined because you haven't defined them. you need to use the parameters passed to the method. -TGG -
Hi Looks like - if you add a new creature type, you must only spawn it in biomes that you have created yourself. Look at BiomeGenBase.getSpawnableList() - if it can't find your enum creature value, it returns null list instead of an empty list, which causes this NPE. I think it's probably more trouble than it's worth to create a new creature type, unless you've got a really good reason for doing so? -TGG
-
gradle build for mod with library dependency
TheGreyGhost replied to TheGreyGhost's topic in ForgeGradle
OK. Thanks for the help! I think you have saved me a good few hours of stuffing around and getting very frustrated... -TGG -
gradle build for mod with library dependency
TheGreyGhost replied to TheGreyGhost's topic in ForgeGradle
Hi > So you want to include the library in your Mod? Yeah, I guess. As a last resort. I imagine neither Minecraft nor the launcher nor Forge will download the library from maven or similar, if it's not already installed? If I understand right, the shadow build will stop the library in my jar from conflicting with other jars (or if the user already has the library installed)? -TGG -
Hi My mod uses a library that doesn't come with vanilla minecraft or with forge. What's the best way to include this during gradle build so that it doesn't cause problems when folks install the mod? -TGG