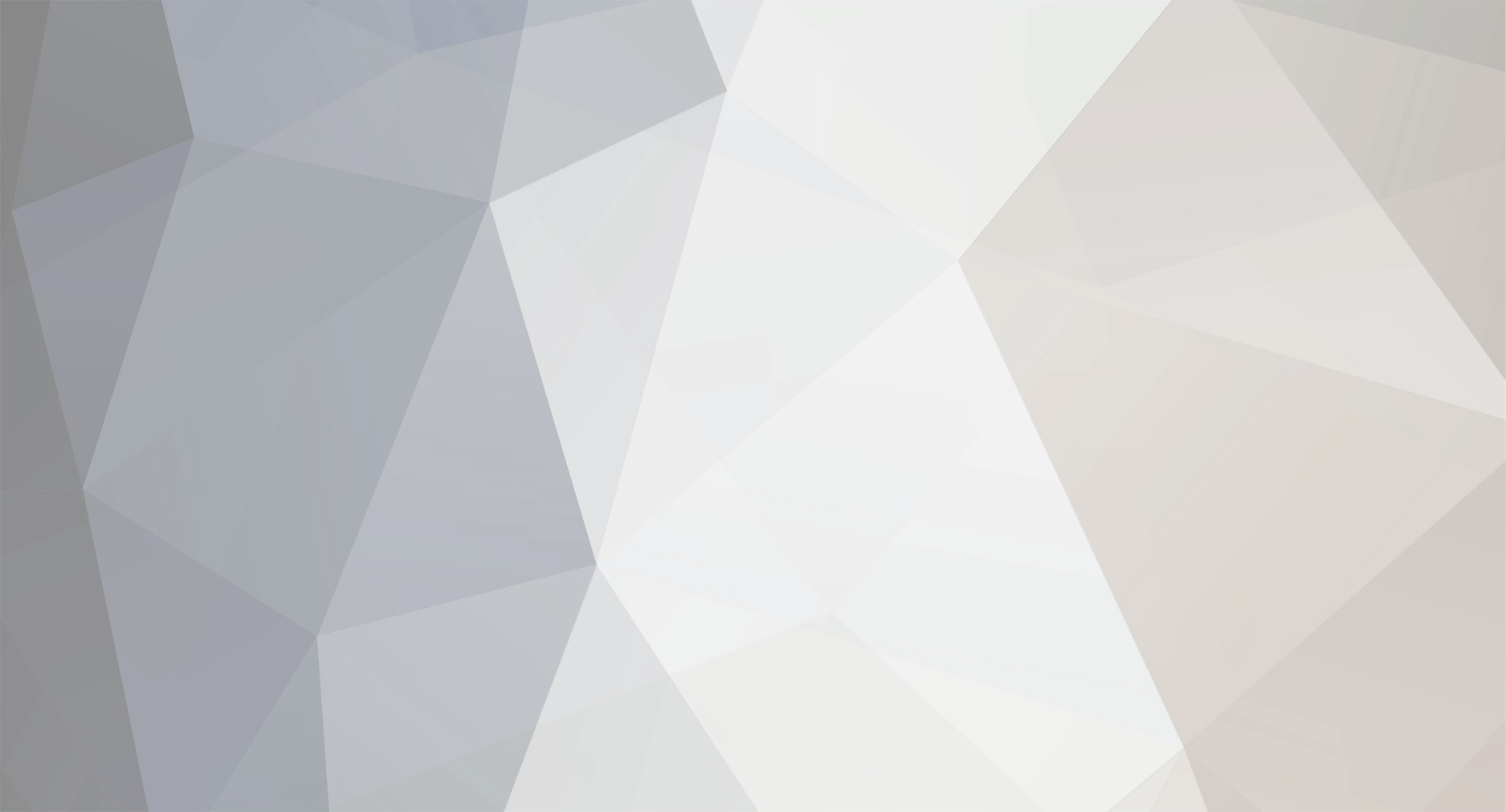
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
[SOLVED]Custom Tile Entity rendering twice while near a beacon?
TheGreyGhost replied to AlexDGr8r's topic in Modder Support
Hi Could you post some screenshots pls? I'm having trouble understanding your question -TGG -
Hi There is some information here on lighting which might help you understand lighting. http://greyminecraftcoder.blogspot.com.au/2013/08/lighting.html See World.computeLightValue(). It is possible (eg add a layer of blocks to the bottom of your island which "emit" skylight) but it will be difficult because the sky lighting is buried deep in vanilla and you will have to dig into it rather deeply using Reflection to modify the vanilla classes, for example World.computeLightValue() or Chunk.heightMap. -TGG
-
yep it's for sure possible. Look at the vanilla bed BlockBed, or BlockDoor, for inspiration. Once you understand how the bed or door keeps track of two blocks, you should be able to extend it to three without too much trouble. It is pretty complicated unless you have a good grip on some of the basic concepts already, though. -TGG
-
[1.7.10][Sloved] Help - How do I get a player's target block?
TheGreyGhost replied to LEGOlord208's topic in Modder Support
Hi There is a way, but it's secret. :-) Only kidding. I assume that you want something to happen to the block you're looking at, without clicking on it. objectMouseOver will work fine with multiplayer, so long as you only use it to do things on the client side. If you want to do something with it that needs to happen on the client side (for example, make the block change or do something that other players will see), then you need to either reproduce the objectMouseOver calculations on the server, or send a packet from the client telling the server which block the player is looking at. -TGG -
[SOLVED][1.7.x] Bug in FML, or am I just not understanding?
TheGreyGhost replied to coolAlias's topic in Modder Support
Hi I wrestled with this back in 1.6.4 for a while, where it all worked fine until I installed my jar in a dedicated server and everything broke, not the same FML classloader errors but a similar idea, i.e. the jvm found references to client-side objects in my code even when those statements were never executed, and threw some linkage errors I forget exactly what they were. Anyway the basic rules I follow now are 1) Make each class side-specific if at all possible. Server-side classes never refer to client-side at all, only server-side and common. Client-side classes never refer to server-side at all, only client-side and common. 2) If I'm writing common classes, either because they're overriding vanilla common or because I want to share the same code on both server and client, I only refer to vanilla common classes, never client or server side vanilla, even if .isRemote is available. If I need to access sided vanilla from a common class (rare), I either use a method in my proxy or more frequently I call one of my sided classes to do it instead (with isRemote to choose the correct side). For forge code which attempts to be present on both sides, in particular some tick handlers and some packet handlers, my proxy init registers a different packet handler class for each side (i.e. dedicated server doesn't register a client handler). Generally I try to keep handlers as stubs which do essentially nothing except immediately pass control to a sided class. So far it's worked fine so long as I've stuck to those rules. -TGG -
Hi I suggest you add more System.out.println(direction); to track it down- whenever you set or get it basically. That should show you pretty quickly where you're going wrong. eg public ForgeDirection getOrientation(){ System.out.println("getOrientation() - orientation:", this.orientation); return orientation; } public void setOrientation(int orientation){ // dude, name hiding is very bad practice this.orientation = ForgeDirection.getOrientation(orientation); System.out.println("setOrientation(int) - orientation:", this.orientation); } public void setOrientation(ForgeDirection direction){ this.orientation = direction; System.out.println("setOrientation(FOrgeDirection) - orientation:", this.orientation); } @Override public void readFromNBT(NBTTagCompound nbtTagCompound){ super.readFromNBT(nbtTagCompound); if (nbtTagCompound.hasKey("orientation")){ System.out.println("hfdjf"); this.orientation = ForgeDirection.getOrientation(nbtTagCompound.getInteger("orientation")); System.out.println("readFromNBT- orientation:", orientation); } if (nbtTagCompound.hasKey("customName")){ this.customName = nbtTagCompound.getString("customName"); } } @Override public void writeToNBT(NBTTagCompound nbtTagCompound){ super.writeToNBT(nbtTagCompound); nbtTagCompound.setInteger("orientation", orientation.ordinal()); System.out.println("writeToNBT - orientation:", orientation.ordinal()); if (this.hasCustomName()){ nbtTagCompound.setString("customName", this.customName); } } {etc} -TGG
-
[1.7.10] Lighting issue with custom rendered blocks
TheGreyGhost replied to Delfil's topic in Modder Support
Hi What is happening is that you're not properly setting up all the lighting for your block before rendering it. So it is being affected by the last object that was rendered. Look at a vanilla renderer to see what you're missing. Typically for TileEntityRenderers you just need GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); // full white or tessellator.setColorOpaque(1, 1, 1); You might also need RenderHelper.disableStandardItemLighting(); -TGG -
HELP! java.lang.NoSuchMethodError: WHAT DO I DO?!?!?!
TheGreyGhost replied to ckazakeich's topic in Modder Support
How did you build your jar? gradlew build? Looks to me like it might be a (de)obfuscation problem, which can happen if you don't build your jar properly, i.e. your code tries to call a deobfuscated vanilla method, which doesn't exist when you put your jar into the minecraft mods folder since the vanilla code is obfuscated there. Just a guess. -TGG -
Game crashes after adding a custom rendered block
TheGreyGhost replied to Mottbro's topic in Modder Support
Hi I'm guessing it's probably the same error again. Techne is notorious for this bug, using objects before instantiating them. Do you know how to use the debugger? It can help you solve this type of problem very quickly. http://www.vogella.com/tutorials/EclipseDebugging/article.html -TGG -
[1.7.10] Making a non-standard block
TheGreyGhost replied to Toastrackenigma's topic in Modder Support
Hi > Make a class implementing ISimpleBlockRendererHandler, and register this handler. There should be tutorials on the subject. What she said, plus this link which might help you understand what you're seeing in vanilla: http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html (See the Block Rendering topics) -TGG -
Hi Minecraft takes the image for each icon and stitches it into a single texture sheet (one for items, one for blocks). You can do this yourself I think, by overriding TextureAtlasSprite and registering that instead of a vanilla icon - overriding loadSprite and/or getFrameTextureData. (not sure of the details - try digging into TextureMap.loadTextureAtlas to watch what happens with each vanilla icon (->TextureAtlasSprite.loadSprite)). Alternatively, bind the texture yourself and use the Tessellator to render the texture. (Do you know what I mean there?) -TGG
-
Hi Ambient occlusion means that the corners of the faces have different brightnesses, i.e. the brightness on a given face varies from one corner to the others depending on what other blocks are nearby. That's not what you need. You need the different faces to have slightly different brightness depending on which way they're facing. Vanilla does this for east/west vs north/south vs up vs down, as described in that lighting link in my post. Just to be explicit- before you render each face, use Tessellator.setColorOpaque() to change the brightness. Choose a different brightness for each of the faces, probably best to try to align them with the vanilla 100% / 80% / 60%/ 40%, but it doesn't matter too much so long as they're different from each other. -TGG
-
Hi You may not need packets if you can calculate the string using information available on the client. Is there any way your client can calculate tin and copper itself, in parallel with the server? Vanilla synchronises some aspects of Inventories / Containers automatically, although I'm not clear on the details because I've never used it. If tin and copper are being altered by something else on the server that the client doesn't know about, then you will need to use Packets to tell the client what is happening on the server. In 1.6.4 you could use Packet132TileEntityData for this sort of thing (->TileEntity.onDataPacket(), TileEntity.getDescriptionPacket()); I don't know what it's called know but it's likely to be very similar. Try looking in TileEntityBeacon or TileEntitySkull for clues. -TGG
-
Hi My guess - perhaps your string is never being set correctly; or perhaps you are updating some of the components on the server side, but not on the client side. Perhaps try adding a diagnostic at the very end of updateEntity, for example System.out.println("TileEntityCrucible::updateEntity side= " + FMLcommonHandler.instance().getEffectiveSide() + ", fillResultString= " + fillResultString); -TGG
-
Hi If you want particles when the player is pushing a movement key, look in EntityPlayerClientMP.movementInput (-->EntityPlayerSP.movementInput) If you want particles when a player changes position (eg moves, falls, is pushed, etc) then either - check for motionX, motionZ as TLHPoE suggested, or - use a tick handler eg TickEvent.ClientTickEvent, and every tick check the player's position to see if it has moved by more than a certain amount -TGG
-
Hi This link might help http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html - see the Block Rendering sections, especially Lighting. You don't actually need ambient occlusion, you just need to change the intensity of the different faces (see the lighting topic). And actually, you probably don't need an IBSRH either. Just change the y upper bound and let the vanilla renderer do the rest. Look at BlockHalfSlab - in particular .setBlockBoundsBasedOnState() .setBlockBoundsForItemRender() .isOpaqueCube() -TGG
-
Looks like a whispering from the oracle to me. public interface IRecipe { /** * Used to check if a recipe matches current crafting inventory */ boolean matches(InventoryCrafting inventorycrafting, World world); /** * Returns an Item that is the result of this recipe */ ItemStack getCraftingResult(InventoryCrafting inventorycrafting); /** * Returns the size of the recipe area */ int getRecipeSize(); ItemStack getRecipeOutput(); } This interface lets you do pretty much any type of matching you want. -TGG
-
[1.7.2] Entity synchronization?
TheGreyGhost replied to Solra Bizna (Again)'s topic in Modder Support
Hi For the synchronisation of Entity information, try a search on DataWatcher. They are used to synchronise (for example) entity health on the server to the client. They are automatically sent when they change. Vanilla reserves some of them for its own use, but there are others which are free for your small bits of information. The hardest bit is to figure out which ones are unused by vanilla. -TGG -
Hi You might find some inspiration in the Minecraft package net.minecraft.pathfinding, eg PathFinder. Never used them myself but they look promising. PathFinding can be an extremely expensive operation (it has to search a very large number of possible path options) so you might need to apply some rules or short-cuts to make sure it doesn't get too high. Are you sure that "shortest distance" isn't good enough for what you want? Don't worry about whether your ArrayList of chest should be some other data structure, it will make absolutely no difference to the speed. -TGG
-
Hi Falling sand does something similar, you could look at that for inspiration (i.e. Ernio's 3rd option below). See BlockSand and EntityFallingSand. -TGG
-
[1.7.2] Texture name from NBT. Edit: Updating texture.
TheGreyGhost replied to Ernio's topic in Modder Support
steady on dude, that's harsh ! Did you actually test it, or is that just a guess? Unless things have changed recently, the server only sends item information when it's updated or moved around, and 1 KB really isn't that much. Even if you don't like that idea, you still don't need to store png files on disk. Just send the image from server to client as a BufferedImage and store it as a texture in ram. See SimpleTexture for hints on how. As far as rendering goes, I think it would be best to use an IItemRenderer - just bind your custom texture and render it using the Tessellator. See this link for information on ItemRendering (the "Item Rendering" sections) http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html -TGG -
Hi In ye olden days (1.6.4 or lower) you had to choose your own block IDs. But now (1.7.2 onwards) you don't use blockIDs any more. It gets created for you and you don't need to worry about it. This site might help explain it more. http://www.wuppy29.com/minecraft/modding-tutorials/forge-modding-1-7/#sthash.OfZ1kXYT.dpbs and http://www.wuppy29.com/minecraft/modding-tutorials/wuppys-minecraft-forge-modding-tutorials-for-1-7-basic-block/#sthash.RSt2PPo3.dpbs -TGG
-
You're welcome IntelliJ is awesome but it sure took me a while to find all the useful features & shortcuts FYI The ones I use all the time are ALT-F7 find usages of a class or variable (the one under the cursor) ctrl-B go to declaration ( of the variable name under the cursor) shift-F6 rename class or variable (under the cursor) CTRL-ALT-left arrow = move cursor to previous location (useful when browsing back and forth through code) CTRL-ALT-right arrow = move cursor to new location -TGG PS I like your avatar image
-
[1.7.10] Arrow Like Particle with keyBind and NBT??
TheGreyGhost replied to drok0920's topic in Modder Support
Hi I would suggest you take one small step at a time, eg 1) get the key binding tutorial to work so that it performs eg System.out.println("F"); every time you push the F key. Once that works 2) add the check of the player information Once that works 3) Add the EntityFX particle spawning (see RenderGlobal.spawnParticle). You don't need packets, particle effects are client-side only. -TGG -
Hi No worries, we all start knowing nothing about anything, great big parts of vanilla I know nothing about and I've been at this for a year now. Yeah if the vanilla stair doesn't exist you'll have to write your own renderer (try ISimpleBlockRenderingHandler as a keyword) and depending on how many orientations the stair can have, you might need to use multiple blocks in addition to metadata, i.e. if there are more than 16 orientations (6 directions for the "top" * 4 rotations) Sounds like you've got the right idea about the packets. I haven't used packets in 1.7.2 yet but I'm told it's not that hard and a couple of tutorials are around. -TGG