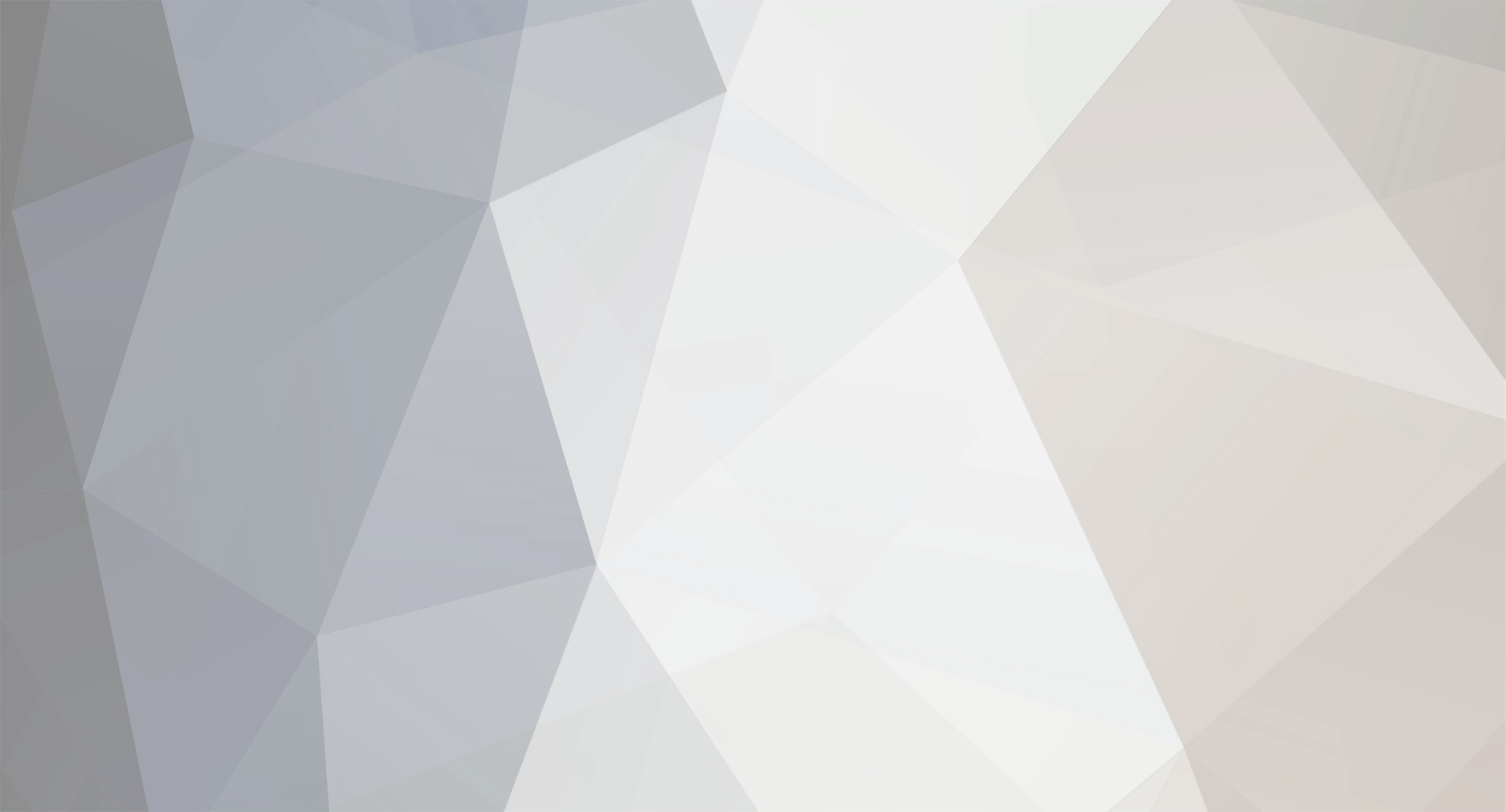
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi From following the other replies I reckon your packet handler is actually working fine. Perhaps you could try something diagnostic in your server packet handler, eg @Override public IMessage onMessage(Light1MessageHandler message, MessageContext ctx) { EntityPlayer player = ctx.getServerHandler().playerEntity; World world = ctx.getServerHandler().playerEntity.worldObj; System.out.println("onMessage:" + message.x + ", " + message.y + ", " + message.z); System.out.println("World time: " + World.getWorldTime(); world.setBlock(message.x , message.y, message.z, Blocks.stone, 0, 1 + 2); // world.setLightValue(EnumSkyBlock.Block, message.x , message.y, message.z, Amnesia.instance.getLanternLightValue()); // updateLight(world, message); return null; } -TGG
-
[SOLVED] Trying to make IMessageHandler more generic
TheGreyGhost replied to coolAlias's topic in Modder Support
Hi coolAlias (welcome back, long time no see ) Just a thought - based on another thread on this forum recently, this method might cause you grief on the dedicated server because Minecraft doesn't exist there. @Override public IMessage onMessage(T message, MessageContext ctx) { if (ctx.side == Side.CLIENT) { return handleClientMessage(Minecraft.getMinecraft().thePlayer, message, ctx); } else { return handleServerMessage(ctx.getServerHandler().playerEntity, message, ctx); } } } Not having done much with 1.7.2 so far, I haven't yet figured out whether the best way to get around that is to put the side-specific stuff into one of the proxy classes, or to register two different handlers on the different sides (is that possible with IMessageHandler?) Any thoughts? -TGG -
Hi Your up/down is because you were rotating on the z-axis? Have you tried test angles on the y-axis just to see if it rotates in the expected direction eg GL11.glRotatef(45F, 0.0F, 1.0F, 0.0F); -TGG
-
Ha ha yeah deja vu It looks like there is an easier way now, in the newer versions of Forge, I haven't tried it yet but if Lex thinks it's there, I'm sure it is. -TGG
-
Hi What doesn't work? Show your code? If you are receiving the packet on the server, why do you need to detect anything on the client side? If your packet only ever arrives on the server side, just forget about the client side totally and use ctx.getServerHandler().playerEntity.worldObj? I really don't think that will work for both sides because if I understand the code correctly getServerHandler() only works on the server side. From a recent post it looks like there might be a getHandler() now too (not in my version of Forge, but that is quite old now). http://www.minecraftforge.net/forum/index.php/topic,20689.msg104534.html#msg104534 So I'm guessing ctx.getHandler().playerEntity.worldObj ? -TGG
-
Hi I'm guessing you have an animation that you want to run at a constant speed, independent of the FPS? In order to do that, you need to synchronise your animation to something that doesn't depend on the FPS. Two ways I have used that work fine: 1) System.nanoTime() 2) MyGlobalTickCounter.getCurrentTick() where I have an event handler for World Ticks which updates the global tick counter. The major difference is whether the animation stops when the game is paused. Your animation code then uses this timer to calculate position, or frame, or whatever. For example, with a spinning wheel you might do final long DEGREES_PER_SECOND = 50; final long NANO_SECONDS_PER_SECOND = 1000 * 1000 * 1000L; long now = System.nanoTime(); long rotationAngle = (DEGREES_PER_SECOND * (now - rotationStartTime)) / NANO_SECONDS_PER_SECOND; drawMyWheel(rotationAngle); or alternatively final long FRAMES_PER_SECOND = 24; ... long frameNumber = (FRAMES_PER_SECOND * (now - rotationStartTime)) / NANO_SECONDS_PER_SECOND; etc -TGG
-
[1.7.2] onUpdate Method Not Being Called for Custom Item
TheGreyGhost replied to Malkuthe's topic in Modder Support
@Delpi Not sure about Eclipse - I use IntelliJ - but when I set a breakpoint on a line in my code or in the vanilla source, it runs to that line and stops exactly like you'd expect. IntelliJ gives the option of pausing all threads or just the one with the breakpoint, so you can choose what you want it to do (normally I suspend both threads, but doing client or server only also works). -TGG -
Hi Not sure if I've understood you correctly; Perhaps: Server side MessageContext ctx -> ctx.getServerHandler().playerEntity.worldObj; Client side Minecraft.getMinecraft().theWorld ? If your code is causing problems because the client objects don't exist on the server side, you could consider putting the side-dependent handler code into your proxies, eg along the lines of this http://www.minecraftforge.net/forum/index.php/topic,20383.msg102861.html#msg102861 -TGG
-
[Resolved] Blocks changing into other blocks
TheGreyGhost replied to altorac12's topic in Modder Support
Hmmm ok. I'd assumed your code was still for 1.6.4 (didn't read your original post properly!), but you're right you shouldn't manually allocate IDs for 1.7. I have heard rumours that the world load/save should automatically reassign IDs to the correct block names, although I don't know how that works. Probably deep in the bowels of GameData. Sorry, no ideas on how to fix it, and yes it does sound like a potential problem... -TGG -
[Resolved] Blocks changing into other blocks
TheGreyGhost replied to altorac12's topic in Modder Support
Hi My guess is that your ID allocation code (using i++) is getting out of step on save vs reload. If you change your code, the numbers allocated to your blocks change, but of course they don't change in the saved world. I'd suggest either changing to a more fixed ID allocation (at least - for debugging), or just regenerating your test world each time and don't worry about it. -TGG -
[1.7.2] Make Biome Spawn at Specific Chunks
TheGreyGhost replied to TLHPoE's topic in Modder Support
Hi These look like promising places (never tried it) GenLayerBiome (randomly chooses biomes from a list) WorldChunkManager.getBiomesForGeneration ChunkProviderGenerate.initializeNoiseField -TGG -
Hi You can get adjacent blocks if you know the [x,y,z] coordinate of your door block. The method you're overriding will give you World, call world.getBlock() with the coordinates of the adjacent block. See also here http://greyminecraftcoder.blogspot.com.au/2013/07/blocks.html My usual strategy for figuring out how something works is to google it first, check a couple of links, then if nothing promising, just look at the vanilla. For example: Pressure plate? search on pressure -> BlockBasePressurePlate looks promising browse through methods -> /** * Ticks the block if it's been scheduled */ public void updateTick(World par1World, int par2, int par3, int par4, Random par5Random) /** * Triggered whenever an entity collides with this block (enters into the block). Args: world, x, y, z, entity */ public void onEntityCollidedWithBlock(World par1World, int par2, int par3, int par4, Entity par5Entity) /** * Checks if there are mobs on the plate. If a mob is on the plate and it is off, it turns it on, and vice versa. */ protected void setStateIfMobInteractsWithPlate(World par1World, int par2, int par3, int par4, int par5) I reckon we've hit paydirt there. It's nearly always possible to get there pretty easily this way. Just think of the vanilla block / item / text command that does something, search for it in vanilla, spend a bit of time figuring out how the code works, then model your new code on it. -TGG
-
Hi That should be relatively straightforward to implement with custom packets. Your server compiles a list of the nearby world data, sends it in a packet or two to the client, which then renders it. -TGG
-
Hi There are a couple of concepts I think you're missing that might help. The first is that you're storing information about Items, not about ItemStacks. So for example there is only one Item for "Carbon", and it has six protons. There might be many ItemStacks of LumpOfCarbon lying around, and they are all Carbon, but in different amounts, one lump might be 6 moles, the other lump 12 moles. They are not the same. So if you want a HashMap to tell you how many protons are in a Carbon, you need a HashMap for Carbon, not for LumpOfCarbon. You could in principle set up a HashMap for LumpsOfCarbon which ignores the number of moles in the lump (using hashCode and equals, like DieSieben said) but that really doesn't make much sense in this case. A second thing is that, if only your new items have atomic masses, why do you need the HashMap registry at all? Why not store the information in the (eg) ItemElement class directly and override Item.addInformation(..) instead? -TGG
-
Hi The root cause of your problem is that you defined your HashMap with Object, so the compiler didn't warn you that you are mixing up Items with ItemStacks Map<Object, Integer[]>; should be better be Map<Item, Integer []> Do you know about the difference between Items and ItemStacks? If not, this might help http://greyminecraftcoder.blogspot.com.au/2013/12/items.html -TGG
-
Naming conventions and parameters in the forge code (not Java)?
TheGreyGhost replied to icecubert's topic in Modder Support
Hi FYI http://mcp.ocean-labs.de/page.php?4 http://mcpold.ocean-labs.de/index.php/MCPBot https://github.com/ModCoderPack/mcpbot http://www.minecraftforum.net/topic/2115030-mcp-mapping-viewer/ -TGG -
ha well I don't know about that, but thanks anyway glad we solved it -TGG
-
TileEntitySpecialRenderer problems (texture flickers)!
TheGreyGhost replied to AskHow1248's topic in Modder Support
Hi Block textures only update when there is a change. When rendering Blocks, minecraft uses a 'cached renderlist' that is only refreshed when the block changes. So if you try to animate your Block using the Block rendering methods above, you won't see anything until the Block is changed or updated. If you want your Block to be animated without updating it, you need to either use an animated texture for your Block, or use a TileEntity with TileEntitySpecialRender. For more info see here http://greyminecraftcoder.blogspot.com.au/2013/07/rendering-world-more-details-including.html -TGG -
Hi Some thoughts Have you registered your door block using GameRegistry? Try @Override before onDoorActivated() maybe your parameter list is wrong Your approach for onActivateMethod seems fine to me. How are you placing your door into the world? You mention an item that seems ok? -TGG
-
Hi Do you know if renderItem is being called? (i.e. breakpoint or System.out.println?) If so - something is wrong with your renderer, eg is rendering at the wrong coordinates, offscreen etc. If not - probably you haven't registered the Item renderer properly. I don't see obvious problems but there might be something subtle I'm missing. -TGG
-
[1.7.2] onUpdate Method Not Being Called for Custom Item
TheGreyGhost replied to Malkuthe's topic in Modder Support
Dude! Waste no more time! Get thee hence to debugging school posthaste! I guess you're using Eclipse? http://www.vogella.com/tutorials/EclipseDebugging/article.html or http://agile.csc.ncsu.edu/SEMaterials/tutorials/eclipse-debugger/ or many many more Seriously, it's really straightforward to learn and once you've tried it you'll wonder how on earth you ever managed without them. Step through your code line by line to see where it's going, look at the variables and see what happens to them at each step. In this case, it will let you find out in 2 minutes flat what happens when the vanilla code gets to your item and tries to call onUpdate for it. -TGG -
Change Item Texture On Right Click question
TheGreyGhost replied to Thornack's topic in Modder Support
Hi Show us the error log? It should have a line something like "texture not found". A few things to try This link shows where the files should go http://greyminecraftcoder.blogspot.com.au/2013/12/overview-of-forge-and-what-it-can-do.html If you still can't find it, this might help http://www.minecraftforge.net/forum/index.php/topic,18371.msg92948.html#msg92948 Keep in mind that the upper/lower case must match exactly. -TGG -
Hi The code in ItemRenderer.renderItemIn2D takes a part of a texture and renders it so it looks like the vanilla items, i.e. flat but with a small thickness.. You just need to provide it with the icon texture coordinates and it does the rest. Should work for blocks too. -TGG
-
Hi I just copied the entire class into an appropriate package, then called dumpAllIsEnabled(). It prints out all the openGL settings you can turn on/off. In this case I would suggest something like static int delay; // put this as a static field in your render class if (++delay % 100 == 0) { // only print once every 100 calls OpenGLdebugging.dumpAllIsEnabled(); } renderItem.renderIcon(0, 0, icon, 16, 16); Then put your item in a good slot, wait till it prints the flags, then put your item in an orange slot, wait till it prints the flags, then compare the two. No guarantees it will show a difference, but it might. My guess would be GL11.glEnable(GL11.GL_ALPHA_TEST); but perhaps also GL11.glDisable(GL11.GL_LIGHTING); -TGG
-
[1.7.2] onUpdate Method Not Being Called for Custom Item
TheGreyGhost replied to Malkuthe's topic in Modder Support
Hi I suggest you put a breakpoint into InventoryPlayer.decrementAnimations() and see directly why your item isn't getting called. -TGG