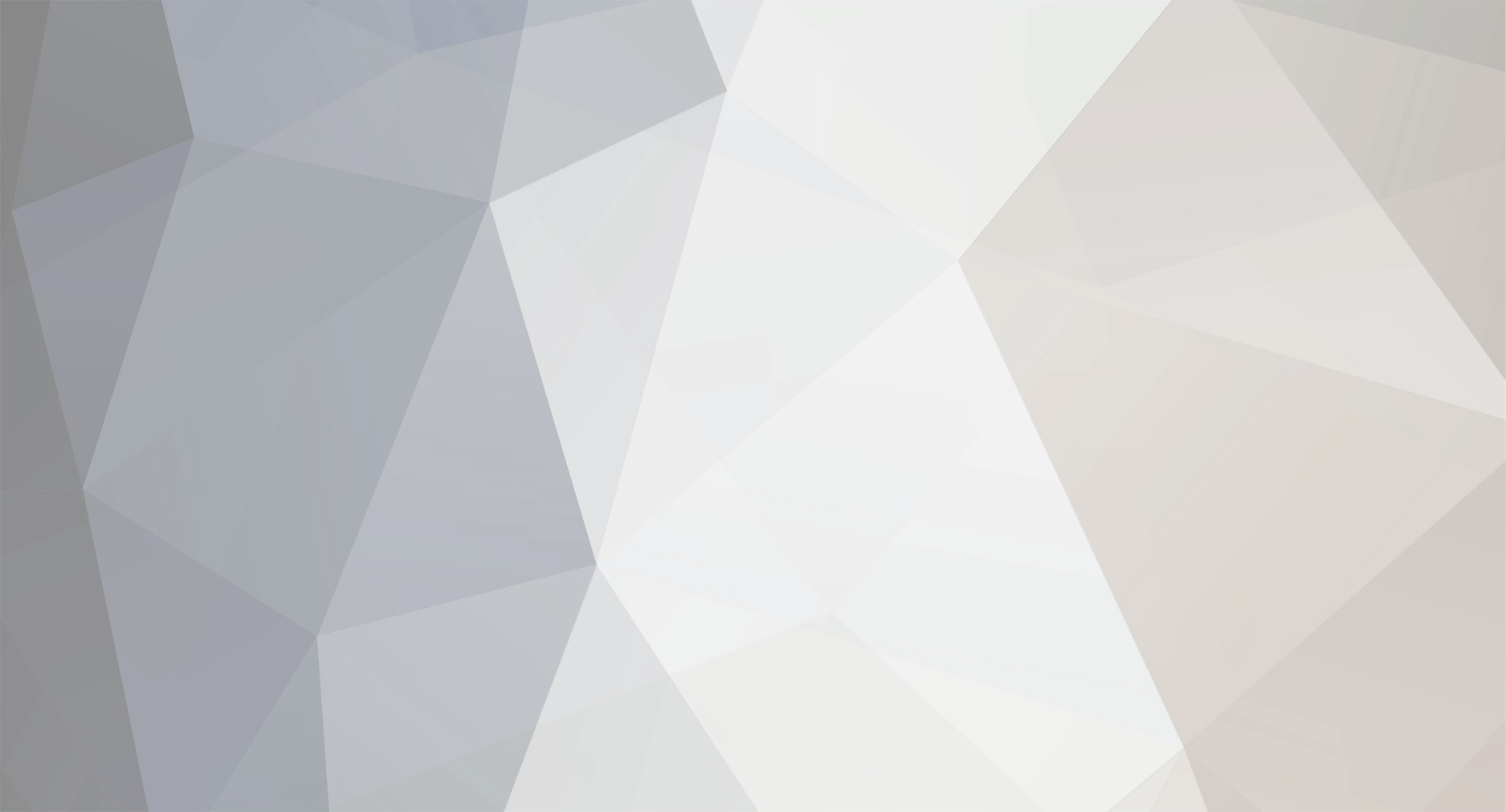
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
How do I get the block above the block I right clicked?
TheGreyGhost replied to Xcox123's topic in Modder Support
Hi This might be useful http://greyminecraftcoder.blogspot.com.au/2013/07/blocks.html -TGG -
Hmmm Screenshot or youtube? -TGG
-
[1.7] Render custom block as item in inventory
TheGreyGhost replied to Jhary's topic in Modder Support
Hi For renderFace use the coordinates from [0,0,0] to [1,1,1] because the caller has already set those coordinates for you. In 1.6.4 you needed to do an extra translate, that may have changed, but it will be obvious if so. The vanilla code is in RenderBlocks.renderBlockAsItem(), so for example this code from 1.6.4 GL11.glTranslatef(-0.5F, -0.5F, -0.5F); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, -1.0F, 0.0F); this.renderFaceYNeg(par1Block, 0.0D, 0.0D, 0.0D, this.getBlockIconFromSide(par1Block, 0)); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, 1.0F, 0.0F); this.renderFaceYPos(par1Block, 0.0D, 0.0D, 0.0D, this.getBlockIconFromSide(par1Block, 1)); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, 0.0F, -1.0F); this.renderFaceZNeg(par1Block, 0.0D, 0.0D, 0.0D, this.getBlockIconFromSide(par1Block, 2)); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, 0.0F, 1.0F); this.renderFaceZPos(par1Block, 0.0D, 0.0D, 0.0D, this.getBlockIconFromSide(par1Block, 3)); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(-1.0F, 0.0F, 0.0F); this.renderFaceXNeg(par1Block, 0.0D, 0.0D, 0.0D, this.getBlockIconFromSide(par1Block, 4)); tessellator.draw(); tessellator.startDrawingQuads(); tessellator.setNormal(1.0F, 0.0F, 0.0F); this.renderFaceXPos(par1Block, 0.0D, 0.0D, 0.0D, this.getBlockIconFromSide(par1Block, 5)); tessellator.draw(); GL11.glTranslatef(0.5F, 0.5F, 0.5F); Don't call renderBlockAsItem, because that's what called your renderer in the first place. i.e. you wind up in an infinite loop ("recursion") until the stack overflows. -TGG -
[1.6.4] Rendering Item On Block OpenGL Help
TheGreyGhost replied to Xcox123's topic in Modder Support
HI Where is this code located? Many of the rendering routines are called with the origin [0,0,0] set to the player's eye location. You need to translate the rendering location GL11.glTranslatef to take account of that. Typically the calling function provides the offset of the entity / tileentity relative to the eye location, or you can calculate it yourself. A bit of trial and error, or inspection of vanilla code, will show you the way. -TGG -
Hi Could be you are trying to render two things to exactly the same z depth? If you have depth culling turned on, the two will fight each other to be on top, which leads to a nasty stripy/ripply effect. You could try turning off depth culling, or try rendering the red one very slightly in front of the black. -TGG
-
Looking for API Documentation or Reference documentation
TheGreyGhost replied to crazycodr's topic in Modder Support
Hi There isn't much around to be honest. Some of the Forge documentation is really good, some of it... isn't. I have put together some things myself about how vanilla works, enough to help folks get a grasp of the basic concepts and how the various bits hang together, but isn't not comprehensive by any stretch (and it's currently based on 1.6.4, which is a bit different to 1.7.2 sometimes). http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html Most of the time, I find a useful strategy is 1) think of a vanilla object that does similar to what I want 2) look at the code, flip back and forth until I figure out how it works (can sometime take a while!) and then test it myself. -TGG -
Hi Kwibble is right. some more background information http://greyminecraftcoder.blogspot.com.au/2013/10/client-side-class-linkage-map.html http://greyminecraftcoder.blogspot.com.au/2013/10/server-side-class-linkage-map.html -TGG
-
Hi Yes it will work on both single player and multiplayer. client side always has only one player. server side may have lots. -TGG
-
Hi The crash is occurring on the client. Looks to me like you have populated the player field on the server but not on the client. When the client receives the packet it creates ExtendedPropertyMessage using the default constructor so everything in it is null. Presumably you then use fromBytes to copy the currentMana and maxMana, but player stays null. You can access the player on the client using Minecraft.getMinecraft().thePlayer. -TGG
-
Hi The black is probably because you haven't set up the lighting and brightness, so it is using the settings from the last thing it rendered. This is typically done using something like tessellator.setBrightness(par1Block.getMixedBrightnessForBlock(this.blockAccess, wx, wy, wz); // wx,wy,wz = world coordinates tessellator.setColorOpaque_F(red, green, blue); // from 0.0 - 1.0 If you want to rotate the faces using uvRotate, you need to call RenderBlocks.renderStandardBlock or RenderBlocks.render after setting the flags. See vanilla RenderBlocks.renderBlockLog for a good example. The rotation is a bit strange, sometimes it flips the faces. But if that doesn't bother you then you don't need the Tessellator at all. -TGG
-
Hi I don't know much about fireworks. Looking at the classes, I don't think addInformation() has anything to do with changing the properties, it just provides a "tooltip" for the existing properties of the firework. The properties of the firework are contained in its NBT information. This seems to be created in RecipeFireworks, mostly in matches() which caches the result to be later retrieved by getCraftingResult(). It builds up the NBT tag depending on the recipe and then attaches it to the Item using .setTagCompound(nbttagcompound); You could figure out what the NBT tags are from looking at the code (perhaps a breakpoint to inspect what the final nbttagcompound is). -TGG
-
Hi You need to spawn on server not client. This is the vanilla code for spawning the rocket, From ItemFirework.onItemUse(), try that: public boolean onItemUse(ItemStack par1ItemStack, EntityPlayer par2EntityPlayer, World par3World, int wx, int wy, int wz, int side, float hitVecX, float hitVecY, float hitVecZ) { if (!par3World.isRemote) { EntityFireworkRocket entityfireworkrocket = new EntityFireworkRocket(par3World, (double)((float) wx + hitVecX), (double)((float) wy + hitVecY), (double)((float) wz + hitVecZ), par1ItemStack); par3World.spawnEntityInWorld(entityfireworkrocket); wx is world x coordinate hitVecX is a number between 0 to 1 giving the position on the block where the player was looking (crosshairs) - or in other words the location where the player clicked. -TGG
-
Hi If you are doing the z = 10 positive face (south), then yes that's right, except that in an IBSRH it has already translated the coordinates for you, so you should draw between [0,0,0] and [1,1,1] instead, i.e. A: .addVertexWithUV(1, 0, 0, u, v) B: .addVertexWithUV(1, 1, 0, u, v) etc regarding u,v: if you have bound the texture yourself and the face is the whole texture, then your u,v coordinates are 0 -> 1 with the v axis pointing in the opposite direction to y, so in your case A: .addVertexWithUV(1, 0, 0, 1, 1) B: .addVertexWithUV(1, 1, 0, 1, 0) etc If each of your faces is an icon (the usual way to do it), use icon.getMinU(), icon.getMaxU(), icon.getMinV(), icon.getMaxV() -TGG
-
Forcing all events in MinecraftForge.EVENT_BUS to happen?
TheGreyGhost replied to ManIkWeet's topic in Modder Support
Hi I think events in the bus do happen right away, because most of them return a success value to the caller. Try it and see perhaps? -TGG -
Hi I'm pretty sure Entity.onUpdate() gets called every tick while the Entity is not dead. What makes you think your Entity stops receiving onUpdate() ? -TGG
-
[1.7.2] [Solved] Particle crash by custom block renderer
TheGreyGhost replied to Bektor's topic in Modder Support
Hi Some more info here http://greyminecraftcoder.blogspot.com.au/2013/07/block-rendering.html and here http://greyminecraftcoder.blogspot.com.au/2013/08/rendering-inventory-items.html -TGG -
[1.7.2] [Solved] Particle crash by custom block renderer
TheGreyGhost replied to Bektor's topic in Modder Support
Hi ISimpleBlockRenderingHandler will do the trick. IItemRenderer is also a possibility. This link might be useful to help you understand the background information - see the ItemRendering sections http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html -TGG -
Hi These links might be helpful http://greyminecraftcoder.blogspot.com.au/2013/07/rendering-standard-blocks-cubes.html and http://greyminecraftcoder.blogspot.com.au/2013/07/rendering-non-standard-blocks.html and http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html -TGG
-
Hi This link might help you understand the proxy better http://greyminecraftcoder.blogspot.com.au/2013/11/how-forge-starts-up-your-code.html It's written for 1.6.4 but the concepts are identical for 1.7.2 -TGG
-
Hi This might be what you need; DimensionManager.getCurrentSaveRootDirectory().toPath(); -TGG
-
[1.7.2] [Solved] Particle crash by custom block renderer
TheGreyGhost replied to Bektor's topic in Modder Support
Hi The problem is related to your TileEntity setup code. FYI I traced it back like this: NibbleArray:: /** * Returns the nibble of data corresponding to the passed in x, y, z. y is at most 6 bits, z is at most 4. */ public int get(int par1, int par2, int par3) { int l = par2 << this.depthBitsPlusFour | par3 << this.depthBits | par1; int i1 = l >> 1; int j1 = l & 1; return j1 == 0 ? this.data[i1] & 15 : this.data[i1] >> 4 & 15; // From Error log: error is here, i1 is -88 } So int par1, par2, and par3 are out of the expected range, and from the index it looks one or more is probably negative when they're expected to be positive Tracing this back up through the list of callers brings us eventually to public void addTileEntity(TileEntity p_150813_1_) { int i = p_150813_1_.xCoord - this.xPosition * 16; int j = p_150813_1_.yCoord; int k = p_150813_1_.zCoord - this.zPosition * 16; this.func_150812_a(i, j, k, p_150813_1_); // i, j, and/or k are out of expected range, probably smaller than the chunk [x,y,z] position //[..] } So it looks to me like your TileEntity x coordinate and/or z coordinate is wrong for the chunk that it's being added to; the vanilla assumes that the TileEntity [x,y,z] will be within the 16x256x16 block cube for that chunk. A search through the code shows that xCoord is normally set in only two places: Chunk.setChunkBlockTileEntity() and TileEntity.readFromNBT() Your TileEntity had an override of readFromNBT, so what should that look like for a vanilla TileEntity such as TileEntityChest? TileEntityChest:: /** * Reads a tile entity from NBT. */ public void readFromNBT(NBTTagCompound par1NBTTagCompound) { super.readFromNBT(par1NBTTagCompound); NBTTagList nbttaglist = par1NBTTagCompound.getTagList("Items"); --> I think this is the problem - compare with yours -TGG -
Unexpected client/server discrepancy in entity.rotationYaw
TheGreyGhost replied to MrCaracal's topic in Modder Support
Hi Looking at 1.6.4. code, the server handler for Packet10 (eg Packet12Look) uses Entity.setRotation /** * Sets the rotation of the entity */ protected void setRotation(float par1, float par2) { this.rotationYaw = par1 % 360.0F; this.rotationPitch = par2 % 360.0F; } The client frequently sends look update packets to the server, so I am surprised they get significantly out of sync (after you %360.0F, I mean). You might be seeing a slight synchronisation delay between client & server? What if you modify your code to System.out.println("CLIENT: Placing Entity Rotation: " + rotation % 360.0F); Does the error get bigger and bigger over time or does the server lag behind the client by a reasonably constant amount? @ShaneCraft: If you want entity B to look at entity A, you need to calculate the angle based on the difference in their positions, eg using Math.atan2(deltax, deltaz). The rotationYaw of A is not relevant. http://www.mathsisfun.com/geometry/unit-circle.html (Entity B is at the centre of the circle, Entity A is the point on the circle.) -TGG -
I have created an abomination (custom entity model)
TheGreyGhost replied to Zer0HD2's topic in Modder Support
Nice -
Hi. Do you mean - while the chest is closed and you're nowhere near it, or while the chest is open and you're looking inside it? -TGG