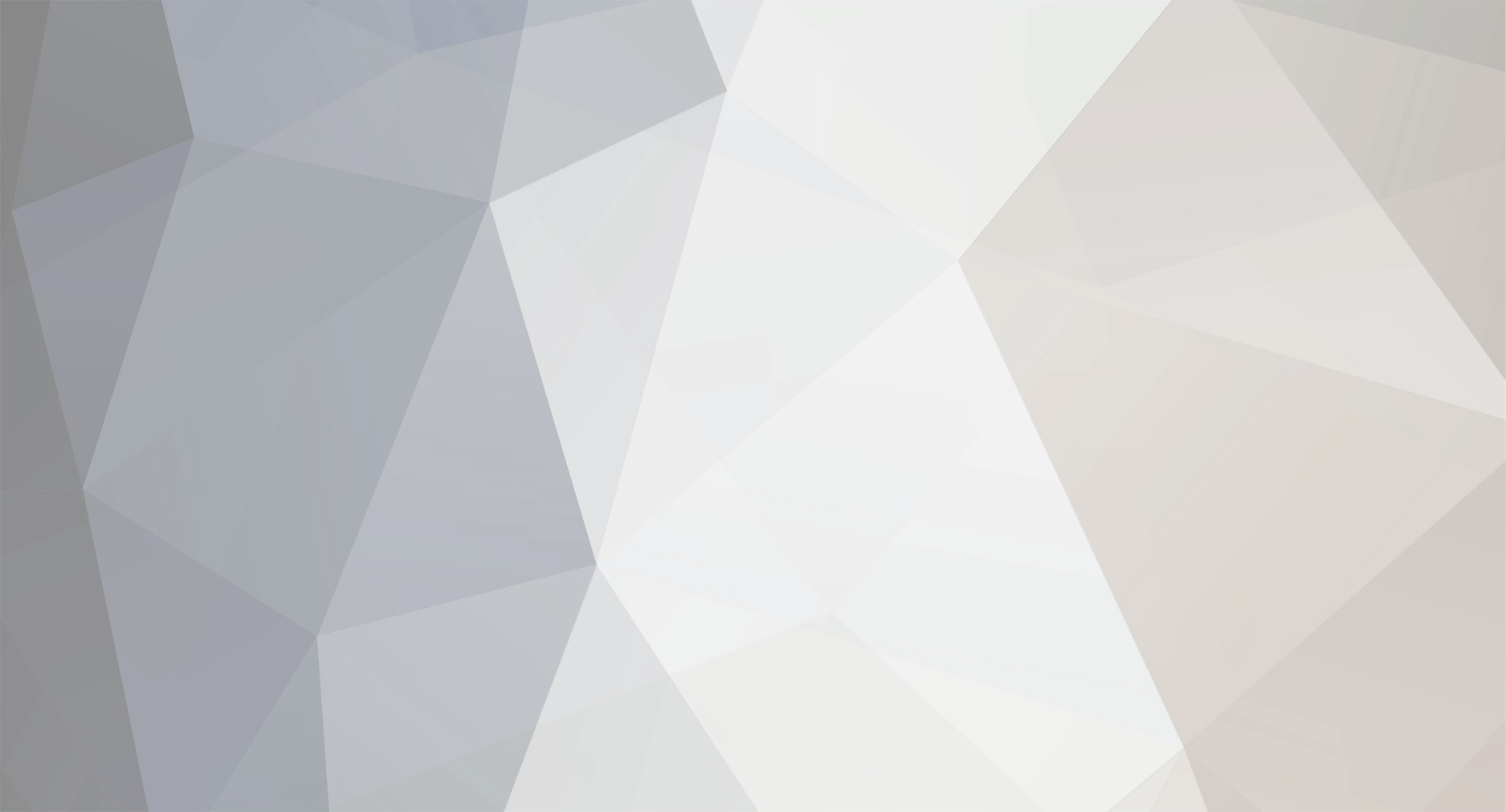
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi For these errors, it's often very helpful to look in the error log for this error "Missing texture, unable to load:" http://www.minecraftforge.net/forum/index.php/topic,18371.msg92948.html#msg92948 -TGG
-
PS re getMouseOver This is the code from 1.6.4 that updates objectMouseOver. You can copy this code, call rayTrace with a longer distance (d0) and do what you want with the result. public void getMouseOver(float par1) { if (this.mc.renderViewEntity != null) { if (this.mc.theWorld != null) { this.mc.pointedEntityLiving = null; double d0 = (double)this.mc.playerController.getBlockReachDistance(); this.mc.objectMouseOver = this.mc.renderViewEntity.rayTrace(d0, par1); double d1 = d0; Vec3 vec3 = this.mc.renderViewEntity.getPosition(par1); Alternatively, if you want to change the vanilla block reach distance, you might be able to use ASM to fiddle with PlayerControllerMP.getBlockReachDistance -TGG
-
RESOLVED [1.7.2] Multi-layered textures
TheGreyGhost replied to DiabolusNeil's topic in Modder Support
Hi I'm not sure exactly what you want to do, but if you use an ISimpleBlockRenderingHandler, you can use the Tessellator to render however you like. For example - if you want to render three textures one on top of the other, your handler render code just draws the same rectangle three times with different textures, using an appropriate blending function or transparent sections or however you want to overlay them. The hardest part is blending the images properly; you can get some strange banding patterns if you render two rectangles almost exactly in the same position. You can usually fix that by offsetting them slightly or by alpha blending. It's also possible to combine your multiple textures to a single texture, then apply this texture to your image (for example - the light map texture does this, see EntityRenderer.updateLightMap()), this is a bit more complicated but (I'm told) not too hard once you get the hang of it; some more info in here http://www.opengl-tutorial.org/intermediate-tutorials/tutorial-14-render-to-texture/ -TGG -
RESOLVED [1.7.2] Multi-layered textures
TheGreyGhost replied to DiabolusNeil's topic in Modder Support
Hi This link might help. http://greyminecraftcoder.blogspot.com.au/2013/07/rendering-transparent-blocks.html It shows you the basics, and there is a link to another tutorial on how to render a block in both pass 1 and path 2 (although - that might be the one you're talking about?) If you give your block an ISimpleBlockRenderingHandler you can render as many layers of texture as you want. -TGG -
[SOLVED][1.7.2]Recommendations for packet payload content/format
TheGreyGhost replied to jabelar's topic in Modder Support
Hi As usual, I think the most-correct answer is "it depends". You can cause a lot of bandwidth problems using Java serialisation if your class is full of stuff you don't actually need to send, or if you are sending packets multiple times a second. But if your class contains only the data that needs sending, and you don't need to send it often, there's no problem. The BitSet in my example uses an extra 76 bytes. That's not significant unless you are sending many of these each second. In many cases I think it's largely a matter of personal taste whether you initially implement the optimised hand-coding, or you just use the serialisation. Java inbuilt serialisation is for sure less efficient. It is also faster to code and reduces the chances of bugs when you refactor. Personally I prefer to keep my code simple and easy to understand/refactor, then optimise it once I have some test data that shows me where I can get significant gains. Others prefer to apply the obvious optimisations up-front. The key point is to understand the tradeoffs. -TGG -
Hi I would suggest you use atan2 instead of atan, that will give you the proper angle for all combinations of deltax and deltaz (except 0,0) , unlike atan where it only gives half the unit circle and causes division by 0 errors if deltaz is zero. Pitch is wrong because you need to use atan2 with the vertical distance to the entity divided by the horizontal distance. You've got the right idea, the vertical is deltay but the horizontal is not just x, it's the sqrt of (deltaz^2 + deltax^2). If you draw the right-angle triangles in 3D - first the "yaw" triangle with deltax and deltaz, and then the "pitch" triangle with the hypotenuse of the "yaw" triangle and the delta y, you can see why. Hard to explain without a diagram... -TGG
-
Hi The clue is here... [19:16:02] [Client thread/ERROR]: Using missing texture, unable to load minecraft:textures/blocks/omar_tutorialmod;liveOre.png.png java.io.FileNotFoundException: minecraft:textures/blocks/omar_tutorialmod;liveOre.png.png That filename looks pretty mangled up :-) -TGG
-
Associating encapsulating my class inside player?
TheGreyGhost replied to roflmuffin's topic in Modder Support
Hi I've had this issue on a server which needs to keep track of temporary information about players. I did it using a "player registry" object with a HashMap with the EntityPlayer as the key and the object as the value. WeakHashMap is good for this, to make sure that unused players are removed from the class automatically, otherwise you can use an event triggered by player login & logout to the update the map. I don't think that extending EntityPlayer is likely to bring you any significant advantages unless you start putting instanceof all throughout your code, which the Java Experts assure me is a very bad idea. -TGG -
What is better models or drawing with tesselators?
TheGreyGhost replied to Mecblader's topic in Modder Support
Hi I would personally prefer models for anything more complicated than more than 2 or three cuboid components. The tessellator just becomes major head damage to do anything complicated with, especially if it has oblique lines or non-triangular/rectangular faces in it, and I suspect it wouldn't be much faster, assuming the model is rendering efficiently. Is your model in a IBSRH or a TileEntity? One of the reasons that vanilla blocks with the tessellator are so fast, is that they are compiled once into a render list (slow), and then just rendered from the render list each time (fast). TileEntities on the other hand do a custom re-render every single frame (slow). -TGG -
[SOLVED][1.7.2]Recommendations for packet payload content/format
TheGreyGhost replied to jabelar's topic in Modder Support
Hi 1) I generally don't both economising on packet contents since unless the packet is biggish (more than say 1 K) or gets sent a lot, it's unlikely to make any noticeable difference. 2) Yes. Typical code I use is (from 1.6.4) 3) DataOutputStream has a wide range of methods for writing data, including boolean. You can even write objects if they are serialisable, eg a simple example for a BitSet 4) I haven't used those before so I can't say. To be honest, I wouldn't worry too much about finding more convenient methods, the ones you already identified are probably fine and are easy to get right. -TGG -
Hi As described in the links in my last post, your console will contain an error message along the lines of "Using missing texture: unable to load nature:textures/items/ingotTitanium.png" That will tell you what filenames it is expecting to find, if you can't be sure from your code. (Hint: the filenames are created here :-) ) for (int i =0; i < TOP.length;i++){ topIcon [ i ] = iIconRegister.registerIcon(HAT.MODID + ":Palm_Wood_Log" + "_Top" + i); sideIcon [ i ] = iIconRegister.registerIcon(HAT.MODID + ":Palm_Wood_Log" + "_Side" + i); } -TGG
-
Hi Some background information on blocks that might be of help. http://greyminecraftcoder.blogspot.com.au/2013/07/blocks.html and http://greyminecraftcoder.blogspot.com.au/2013/10/the-most-important-minecraft-classes_9.html I would suggest: use metadata. You can store 4 bits of information in it, enough for 1) door locked or unlocked 2) door open or closed 3) door facing east, west, north, or south Tile entities are harder to implement correctly, but not that hard; there are a couple of decent tutorials around that a google should show you pretty easily. -TGG
-
Hi This link might help http://www.minecraftforge.net/forum/index.php/topic,18371.msg92948.html#msg92948 -TGG
-
[1.7.2][Solved]Need Help for Temp Fix Bed Texture Bug
TheGreyGhost replied to Lylac's topic in Modder Support
Hi The bed code from 1.6.4 is the same, but I've refactored it a bit to make the logic more clear. public Icon getIcon(int side, int metadata) { if (side == 0) { return Block.planks.getBlockTextureFromSide(side); } else { int bedDirection = getDirection(metadata); int bedSide = Direction.bedDirection[bedDirection][side]; int thisIsHeadOfBed = isBlockHeadOfBed(metadata) ? 1 : 0; if (thisIsHeadOfBed == 1 && bedSide == 2) { return this.bedEndIcons[thisIsHeadOfBed]; } else { if (thisIsHeadOfBed == 0 && bedSide == 3) { return this.bedEndIcons[thisIsHeadOfBed]; } else { if (bedSide == 5 || bedSide == 4) { return this.bedSideIcons[thisIsHeadOfBed]; } else { return this.bedTopIcons[thisIsHeadOfBed]; } } } } } This link might also help understand about directions ("side") in Minecraft, assuming you don't already know http://greyminecraftcoder.blogspot.com.au/2013/07/blocks.html The bed can be placed in one of four orientations, and there are two parts. This information is encoded into the bottom three bits of metadata; bedDirectionBits and thisIsHeadOfBed extract this back out. The bedDirection[][] array is used to convert the side and the bed orientation into the "bed side", i.e. a number showing which of the six faces of the bed is being drawn. -TGG -
Hi In that case, you could create your own version of the furnace that uses its own set of recipes separate from the furnace recipes. The vanilla code for the furnace and the smelting recipes should be a good source of clues on what you need to implement, in particular FurnaceRecipes and ContainerFurnace. -TGG
-
[1.7.2][Unsolved] massive lags checkedPosition < toCheckCount
TheGreyGhost replied to TeNNoX's topic in Modder Support
Hi You get the profile dumps by issuing the command /debug start debug <(start | stop)> Starts a new debug profiling session or stops the session currently running. It notifies about potential performance bottlenecks in the console when active and creates a profiler results file in the folder debug when stopped. -TGG -
What you are effectively doing is palmlog palmlog; palmlog = new palmlog(); That is very obviously going to cause trouble because your compiler can't tell whether palmlog is the class definition, or the variable. That is why everyone names classes in CamelCase i.e. Palmlog palmlog = new Palmlog(); If you don't stick to these conventions you're going to make yourself very unpopular with anyone who has to read or debug your code. -TGG
-
Hi This link is a good one http://www.wuppy29.com/minecraft/modding-tutorials/wuppys-minecraft-forge-modding-tutorials-for-1-7-crafting-recipes/ -TGG
-
Hi If the error is on this line, I imagine it is a "Null Pointer Exception", yes? x.writeByte(animID); That probably means you are calling @Override public void encodeInto(ChannelHandlerContext paramChannelHandlerContext, ByteBuf x) { x.writeByte(animID); x.writeInt(entityID); } with an argument x that is null. If you can figure out why that is happening, you'll be a step closer... -TGG
-
[1.7.2][Unsolved] massive lags checkedPosition < toCheckCount
TheGreyGhost replied to TeNNoX's topic in Modder Support
Hi Looks to me like your mod is changing something frequently that triggers Minecraft to recalculate the sky light and block light for each block over and over. That is expensive (hence the reason that vanilla saves those values and only recalculates them when necessary). Some examples are - changing the opacity of a type of block; changing the amount of light produced by "glowing" blocks. Each change in one of those triggers a cascading recalculation of all the blocks nearby, which takes a very long time. If you're doing anything like that, try commenting it out and see if the lag disappears. I presume the lag is during game, not during world generation? -TGG PS Some bg info on lighting here... http://greyminecraftcoder.blogspot.com.au/2013/08/lighting.html -
Hi guys I have sent you a PM which hopefully is helpful. -TGG
-
Entities do not render from some rotations.
TheGreyGhost replied to david476's topic in Modder Support
Dude To be blunt, I still have absolutely no idea from your description what the symptoms of your problem are :-) I see three boxes. Are those the entities you're talking about, or is it something to do with those coloured patches on the ground? Are those boxes the ones that turn invisible, or are there other invisible ones in the screen shot that we can't see? Are they rotating on their own axes, and for some rotation positions they are invisible (if so - which positions) or - do they disappear as you move around them? or as you move away from them? or when you stop looking directly at them? If so, what positions? -TGG -
[unSOLVED] Trigonometry with connected entities
TheGreyGhost replied to david476's topic in Modder Support
Hi The equations you need are all in the posts above. Unfortunately they are quite complicated, and in order to understand them I think you would need to spend a few days reading & practicing a couple of introductory texts to 3D graphics. The matrix algebra you need isn't hard but if you don't understand what you're doing it's going to be extremely frustrating. If you don't want to do that, I can only suggest that you either - try something easier (achieve the effects you want in a different way), - look around for open-source minecraft mods which do something similar to what you want, or - try to find another modder who understands 3D transformations and is willing to collaborate with you. You might be able to find someone on this forge forum or on http://www.minecraftforum.net/forum -TGG