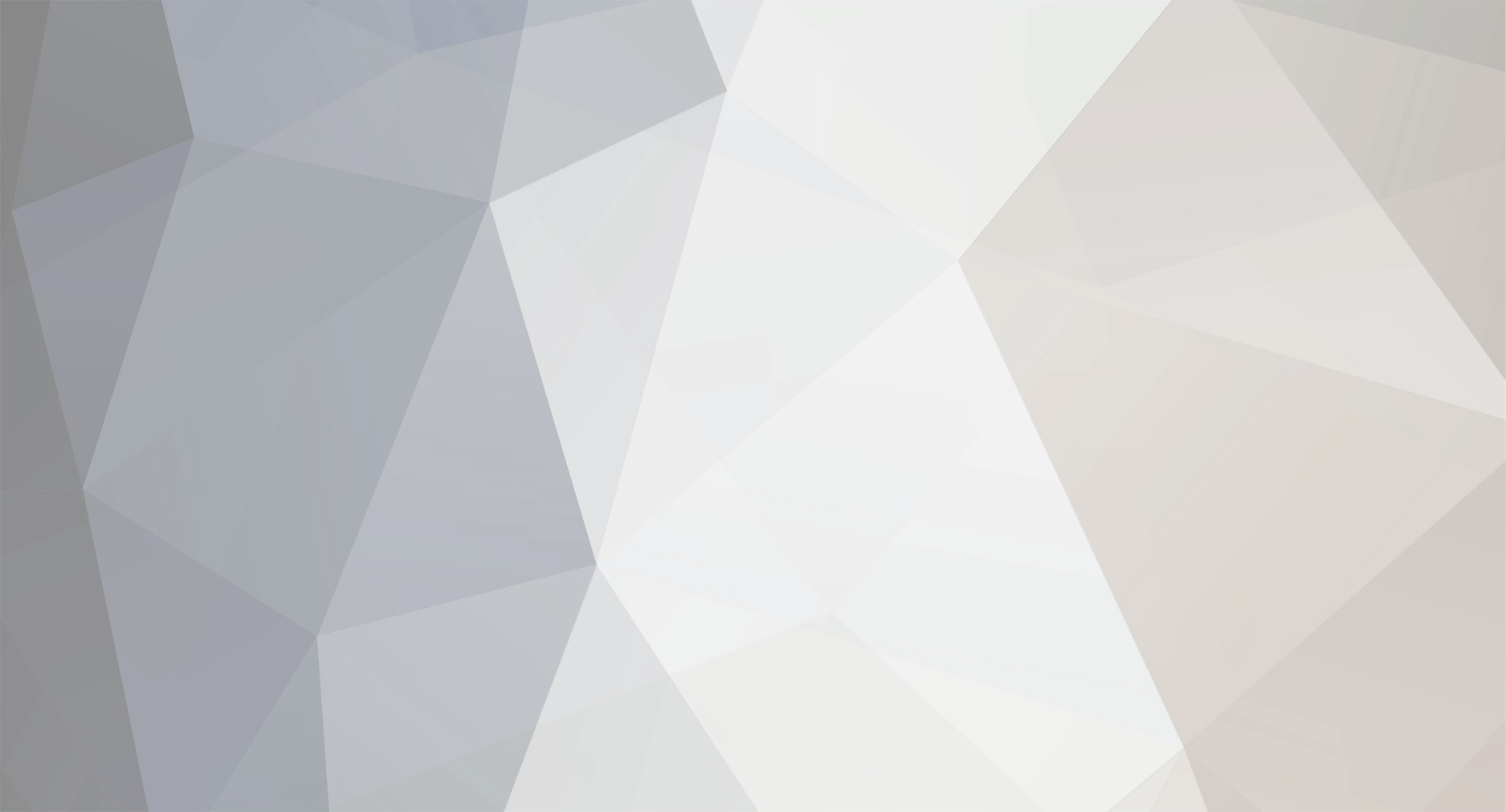
TLHPoE
Members-
Posts
638 -
Joined
-
Last visited
Everything posted by TLHPoE
-
Is there a way of making a mob spawn like a bat? There's a water creature type, but not a cave creature type.
-
Ok, I've got my entity hooked up with a hurt sound like this: protected String getHurtSound() { return ReferenceRF.RES_PRE + "mob.mummy.hurt"; } And whenever I hit my entity, this pops up in the console: [19:55:49] [Client thread/WARN]: Unable to play empty soundEvent: realmoffera:mob.mummy.hurt Here's what my json looks like: { "mob.mummy.say": { "category": "hostile", "sounds": [{ "name": "mob/mummy/say", "stream": false }] }, "mob.mummy.death": { "category": "hostile", "sounds": [{ "name": "mob/mummy/death", "stream": false }] }, "mob.mummy.hurt": { "category": "hostile", "sounds": [{ "name": "mob/mummy/hurt", "stream": false }] } } Finally here's my file tree: I'm 100% sure that my sounds are in the .ogg format.
-
How does one change the color of a biome's grass? I'm using the setColor method, but it's not affecting anything (it sets the grass to a purple color, but it looks like the plains biome color). Code: package realmoffera.world.biome; import java.util.Random; import net.minecraft.world.World; import net.minecraft.world.biome.BiomeGenBase; public class BiomeGenDreamRealmRF extends BiomeGenBase { public BiomeGenDreamRealmRF(int par1) { super(par1); this.setColor(0x7F007F); this.setBiomeName("biome.dreamRealm.name"); this.setTemperatureRainfall(0.8F, 0.4F); this.setHeight(height_LowPlains); this.theBiomeDecorator.treesPerChunk = -999; this.theBiomeDecorator.flowersPerChunk = -999; this.theBiomeDecorator.grassPerChunk = -999; this.flowers.clear(); } public void decorate(World par1World, Random par2Random, int par3, int par4) { super.decorate(par1World, par2Random, par3, par4); } public BiomeGenBase createMutation() { return null; } }
-
Found out how, just kill them with magic. this.damageEntity(DamageSource.magic, 1000);
-
Is there a method I can call that kills the entity but drops nothing? I've thought of teleporting the entity to 0, 0, 0 and killing them, but it seems impractical.
-
Is there a place where I can latch that onto the world's data?
-
I'm currently trying to go in an already existing world and generate stuff inside of a chunk. I was looking at how some others mod did it in 1.7.2 (OresPlus), and they used the ChunkDataEvent.Load event with a world tick handler to generate the stuff. I don't know if I'm doing something wrong, but the ChunkDataEvent.Load event only gets called for some chunks. My Event Class: package realmoffera.handler; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.boss.EntityDragon; import net.minecraft.entity.monster.EntityMob; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.server.MinecraftServer; import net.minecraft.util.ChatComponentText; import net.minecraft.util.EnumChatFormatting; import net.minecraft.world.World; import net.minecraft.world.chunk.Chunk; import net.minecraftforge.event.entity.living.LivingDeathEvent; import net.minecraftforge.event.entity.living.LivingDropsEvent; import net.minecraftforge.event.world.ChunkDataEvent; import realmoffera.ReferenceRF; import realmoffera.ServerProxyRF; import realmoffera.UtilRF; import realmoffera.helper.ItemHelperRF; import cpw.mods.fml.common.eventhandler.SubscribeEvent; public class ServerEventHandlerRF { public static int chunksAddedToGenerate = 0; public static int chunksLoaded = 0; @SubscribeEvent public void chunkLoad(ChunkDataEvent.Load event) { if(!event.world.isRemote) { World world = event.world; Chunk chunk = event.getChunk(); NBTTagCompound nbt = event.getData(); if(!nbt.hasKey(ReferenceRF.ID)) { nbt.setTag(ReferenceRF.ID, new NBTTagCompound()); } nbt = event.getData().getCompoundTag(ReferenceRF.ID); if(!nbt.getBoolean("postDragon") && ServerProxyRF.getWorldProperty(world.getWorldInfo().getWorldName(), "hasDefeatedEnderDragon", false).getBoolean(false)) { nbt.setBoolean("postDragon", true); ServerWorldTickHandlerRF.chunksToGenerate.add(chunk); ServerEventHandlerRF.chunksAddedToGenerate++; } ServerEventHandlerRF.chunksLoaded++; } } @SubscribeEvent public void livingDropEvent(LivingDropsEvent event) { EntityLiving entity = (EntityLiving) event.entityLiving; if(!entity.worldObj.isRemote && entity instanceof EntityMob) { if(ServerProxyRF.getWorldProperty(entity.worldObj.getWorldInfo().getWorldName(), "hasDefeatedEnderDragon", false).getBoolean(false) && UtilRF.getChance(1, 100) && !entity.worldObj.isDaytime()) entity.dropItem(ItemHelperRF.dreamCharm, 1); } } @SubscribeEvent public void livingDeathEvent(LivingDeathEvent event) { if(!event.entityLiving.worldObj.isRemote && event.entityLiving instanceof EntityDragon) { ServerProxyRF.getWorldProperty(event.entityLiving.worldObj.getWorldInfo().getWorldName(), "hasDefeatedEnderDragon", false).set(true); ServerProxyRF.saveConfigs(); MinecraftServer.getServer().getConfigurationManager().sendChatMsg(new ChatComponentText(EnumChatFormatting.DARK_RED + "You hear screams echo through out the dimensions...")); } } } And here's my world tick handler: package realmoffera.handler; import java.util.ArrayList; import net.minecraft.init.Blocks; import net.minecraft.world.chunk.Chunk; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.common.gameevent.TickEvent; public class ServerWorldTickHandlerRF { public static ArrayList<Chunk> chunksToGenerate = new ArrayList<Chunk>(); public static int chunksGenerated = 0; @SubscribeEvent public void onWorldTick(TickEvent.WorldTickEvent event) { if(!event.world.isRemote) { for(int i = 0; i < chunksToGenerate.size(); i++) { Chunk chunk = chunksToGenerate.get(i); for(int j = 0; j < 16; j++) { for(int k = 0; k < 16; k++) { int x = (chunk.xPosition * 16) + j; int z = (chunk.zPosition * 16) + k; event.world.setBlock(x, 128, z, Blocks.diamond_block); } } chunk.setChunkModified(); chunksToGenerate.remove(i); ServerWorldTickHandlerRF.chunksGenerated++; } System.err.println("Chunks Added To Generation: " + ServerEventHandlerRF.chunksAddedToGenerate); System.err.println("Chunks Generated: " + ServerWorldTickHandlerRF.chunksGenerated); System.err.println("Chunks Loaded: " + ServerEventHandlerRF.chunksLoaded); } } } Without the NBT, I don't think I can tell if the chunk has already been generated in.
-
Arghh, okay. Thanks for the information.
-
But is there a way I can get that NBT from just a Chunk instance?
-
Well is there anyway to obtain access to that NBT?
-
The ChunkDataEvent.Load event doesn't fire every time a chunk load. I'm trying to get the NBT of a chunk at any time with just a Chunk.
-
You can't really write to or read from an item's NBT, but you can write to and read from an item stack's NBT.
-
Is there anyway to get the NBTTagCompound from a chunk? I've been using ChunkDataEvent.Load and it provides access to a NBTTagCompound of the chunk.
-
Update: I found an event (ChunkWatchEvent.Watch) that gets called when a chunk is loaded, but I can't get the NBT of the chunk or even tell if I've gone through it yet.
-
Ok, I think I've found the problem. For some strange reason, the ChunkDataEvent.Load doesn't always get called.
-
I tried that, the only other thing I noticed was that if I selected the world, it would return to the world selection menu. After a couple of seconds, it spawns me in the world. During that time, it said it was generating the stuff, but when I spawned nothing was there.
-
I'm not sure what's causing the problem, but sometimes it skips out on chunks. My mod is supposed to generate new structures and ores after the Ender Dragon is killed. When I return to the overworld, only some chunks have the new ores. Chunk Load Event: @SubscribeEvent public void chunkLoad(ChunkDataEvent.Load event) { if(!event.world.isRemote) { World world = event.world; Chunk chunk = event.getChunk(); NBTTagCompound nbt = event.getData(); if(!nbt.hasKey(ReferenceRF.ID)) { nbt.setTag(ReferenceRF.ID, new NBTTagCompound()); } nbt = event.getData().getCompoundTag(ReferenceRF.ID); if(!nbt.getBoolean("postDragon") && ServerProxyRF.getWorldProperty(world.getWorldInfo().getWorldName(), "hasDefeatedEnderDragon", false).getBoolean(false)) { nbt.setBoolean("postDragon", true); ServerWorldTickHandlerRF.chunksToGenerate.add(chunk); } } } Here's my World Tick Handler: public class ServerWorldTickHandlerRF { public static ArrayList<Chunk> chunksToGenerate = new ArrayList<Chunk>(); @SubscribeEvent public void onWorldTick(TickEvent.WorldTickEvent event) { if(!event.world.isRemote) for(int i = 0; i < chunksToGenerate.size(); i++) { Chunk chunk = chunksToGenerate.get(i); for(int j = 0; j < 8; j++) { int x = (chunk.xPosition * 16) + event.world.rand.nextInt(16); int y = 128; int z = (chunk.zPosition * 16) + event.world.rand.nextInt(16); event.world.setBlock(x, y, z, Blocks.diamond_block); System.err.println("Generating at X: " + x + "|Y: " + y + "|Z: " + z); } chunksToGenerate.remove(i); System.err.println("LOADING"); } } }
-
Is there an easy way to send a message to all the players on a server? I have a working method but it seems a bit overkill. @SubscribeEvent public void livingDeathEvent(LivingDeathEvent event) { EntityLiving entity = (EntityLiving) event.entityLiving; boolean f1 = false; if(!entity.worldObj.isRemote) { if(entity instanceof EntityDragon) { ServerProxyRF.getWorldProperty(entity.worldObj.getWorldInfo().getWorldName(), "hasDefeatedEnderDragon", false).set(true); f1 = true; } } else { if(f1) { Minecraft.getMinecraft().thePlayer.addChatComponentMessage(new ChatComponentText(EnumChatFormatting.DARK_RED + "You hear screams of terror echo throughout the dimensions...")); } } }
-
Ok, I know kind of how to go about rescaling it, but I'm not sure if it will automatically change it's position every time I resize the window. Here's the gui at testing resolution: And here's the gui at fullscreen resolution: Finally, here's my Container class: package realmoffera.container; import java.util.Random; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.Slot; public class ContainerInventoryPP extends Container { private IInventory inventory; public int ingredients; public ContainerInventoryPP(IInventory inventory) { this.addSlotToContainer(new Slot(inventory, 0, -114, -26)); ingredients = new Random().nextInt(10); System.err.println("Ingredients: " + ingredients); } @Override public boolean canInteractWith(EntityPlayer var1) { return true; } }
-
Ok, I've got this method in my gui class that extends InventoryEffectRenderer: @Override protected void drawGuiContainerBackgroundLayer(float var1, int var2, int var3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(TEXTURE); int x, y; x = (int) (((double) 8 / 854) * mc.displayWidth); y = (int) (((double) 8 / 480) * mc.displayHeight); this.drawTexturedModalRect(x, y, 0, 0, 175, ySize); x = (int) (((double) 388 / 854) * mc.displayWidth); this.drawTexturedModalRect(x, y, 175, 0, 31, ySize); } For some reason, the gui doesn't properly scale itself on high resolutions, I haven't tried lower resolutions yet. Here's at testing size: And here's at a higher size:
-
In my mod, I need to cancel some of Minecraft's ore generation. I've tried cancelling the event, but soon found out that the event couldn't be canceled. Is there a way to cancel ore generation without ASM or making my own world type?
-
[SOLVED] Custom ore veins not generating?
TLHPoE replied to Captain Hillman's topic in Modder Support
You need to multiply the chunk coordinates by 16 before generating stuff. generateSurface(rand, chunkX * 16, chunkZ * 16, world, BlockStore.citrineUnref.blockID, 15, 6); generateSurface(rand, chunkX * 16, chunkZ * 16, world, BlockStore.peridotUnref.blockID, 15, 6); generateSurface(rand, chunkX * 16, chunkZ * 16, world, BlockStore.jasperUnref.blockID, 15, 6); generateSurface(rand, chunkX * 16, chunkZ * 16, world, BlockStore.aquamarineUnref.blockID, 15, 6); generateSurface(rand, chunkX * 16, chunkZ * 16, world, BlockStore.moonstoneUnref.blockID, 15, 6); -
I believe you need to cast a ray, and then render it out.
-
I'm clueless on java terms, but there was an iterator inside of itemRegistry. I loop through lists like this: for(Object o : GameData.itemRegistry) { if(o instanceof Item) { Item i = (Item) o; } }
-
GameData.itemRegistry I believe you can iterate through that.