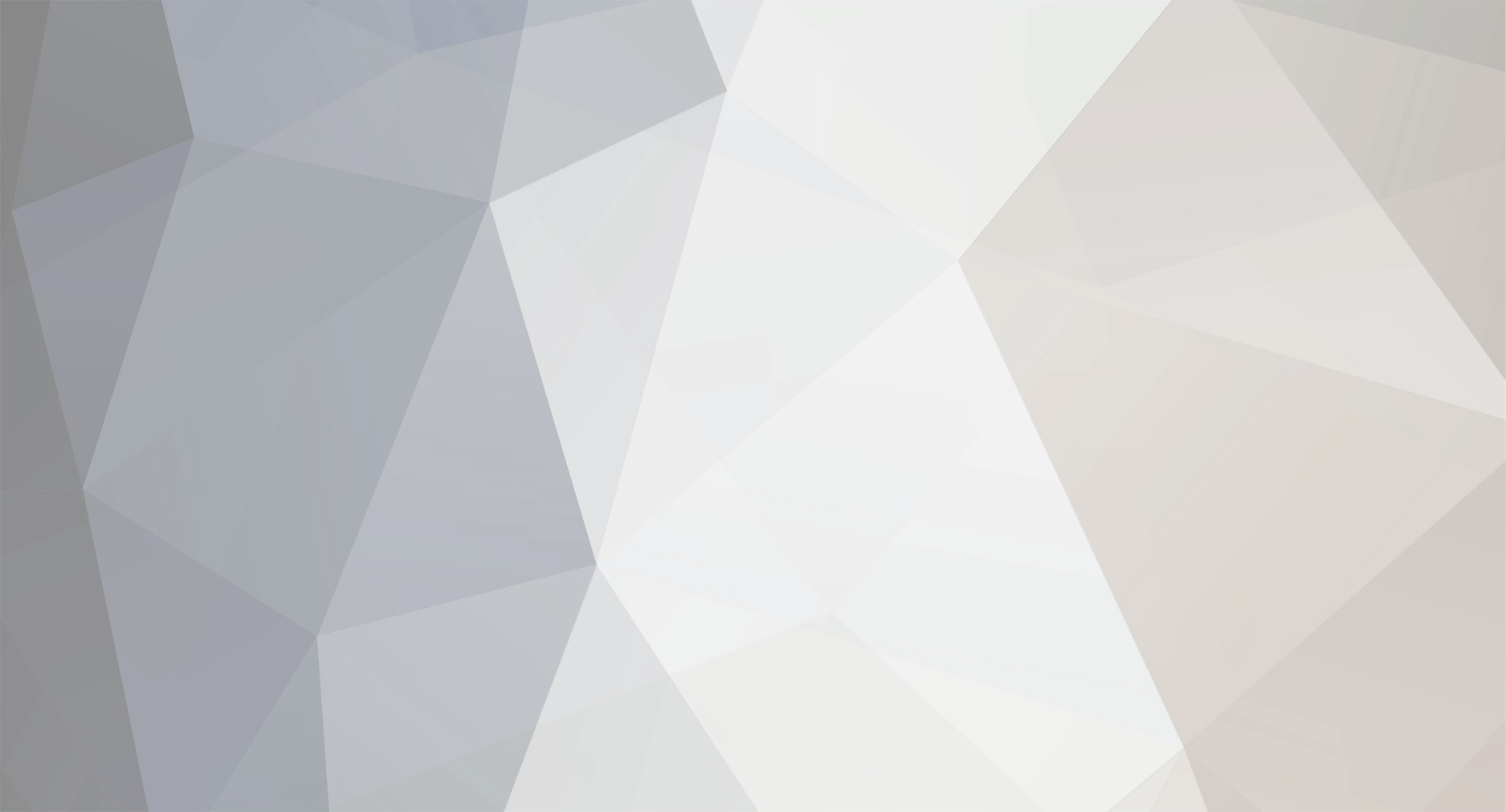
TLHPoE
Members-
Posts
638 -
Joined
-
Last visited
Everything posted by TLHPoE
-
Is there anyway of knowing which slot the player is currently mousing over? Also, what side would I be checking on? (Server or client)
-
Again, more experimentation. By extending EntityMob, it works. But I don't want it to extend EntityMob just because it's not rendering. Is there something that EntityMob or EntityLiving(Base) does that edits the rendering of entities? Even though it says it's rendering, I added a print to see where it was actually rendering. IM RENDERING THE MODEL AT X: 0.7624547|Y: -1.62|Z: -0.47452742 I don't think that's right. Entity Code: package realmoffera.entity; import net.minecraft.block.material.Material; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.DamageSource; import net.minecraft.util.MathHelper; import net.minecraft.world.World; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class EntityCoinsRF extends EntityMob { public int value = 10; public EntityCoinsRF(World world) { super(world); this.preventEntitySpawning = true; this.yOffset = 0.0F; this.setSize(0.5F, 0.5F); } public EntityCoinsRF(World world, int value, double x, double y, double z) { super(world); this.yOffset = 0.0F; this.setSize(0.5F, 0.5F); this.preventEntitySpawning = false; this.value = value; setLocationAndAngles(x, y, z, MathHelper.wrapAngleTo180_float(world.rand.nextFloat() * 360.0F), 0); } @Override protected void entityInit() { super.entityInit(); System.out.println("Initializing coins at X: " + posX + "|Y: " + posY + "|Z: " + posZ); } @Override public boolean attackEntityFrom(DamageSource par1DamageSource, float par2) { return false; } @Override public void onUpdate() { super.onUpdate(); System.out.println("Updating at X: " + posX + "|Y: " + posY + "|Z: " + posZ); } public boolean canBeCollidedWith() { return true; } @Override public void onCollideWithPlayer(EntityPlayer player) { System.out.println("Colliding with player!"); super.onCollideWithPlayer(player); NBTTagCompound nbt = player.getEntityData().getCompoundTag(EntityPlayer.PERSISTED_NBT_TAG); nbt.setInteger("Coins", nbt.getInteger("Coins") + value); setDead(); } @Override public void readEntityFromNBT(NBTTagCompound nbt) { value = nbt.getInteger("Value"); } @Override public void writeEntityToNBT(NBTTagCompound nbt) { nbt.setInteger("Value", value); } @Override public boolean canAttackWithItem() { return false; } @Override protected boolean canTriggerWalking() { return false; } @Override public boolean canBePushed() { return true; } @Override public boolean handleWaterMovement() { return this.worldObj.handleMaterialAcceleration(this.boundingBox, Material.water, this); } @Override @SideOnly(Side.CLIENT) public void performHurtAnimation() { } @Override protected void dealFireDamage(int par1) { } @SideOnly(Side.CLIENT) public float getShadowSize() { return 0.0F; } } Model Code: package realmoffera.client.model; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; import realmoffera.entity.EntityCoinsRF; public class ModelCoinsRF extends ModelBase { ModelRenderer coin1; ModelRenderer coin2; ModelRenderer coin3; ModelRenderer coin4; public ModelCoinsRF() { super(); textureWidth = 32; textureHeight = 32; coin1 = new ModelRenderer(this, 0, 0); coin1.addBox(0F, 0F, 0F, 4, 1, 4); coin1.setRotationPoint(-2F, 23F, -2F); coin1.setTextureSize(32, 32); coin1.mirror = true; setRotation(coin1, 0F, 0F, 0F); coin2 = new ModelRenderer(this, 0, 0); coin2.addBox(0F, 0F, 2F, 4, 1, 4); coin2.setRotationPoint(-1F, 23F, 7F); coin2.setTextureSize(32, 32); coin2.mirror = true; setRotation(coin2, 0.1858931F, 2.918521F, 0.0371786F); coin3 = new ModelRenderer(this, 0, 0); coin3.addBox(0F, 0F, 0F, 4, 1, 4); coin3.setRotationPoint(-2F, 23F, -5F); coin3.setTextureSize(32, 32); coin3.mirror = true; setRotation(coin3, 0.0371786F, -0.6878043F, -0.3346075F); coin4 = new ModelRenderer(this, 0, 0); coin4.addBox(0F, 0F, 0F, 4, 1, 4); coin4.setRotationPoint(4.2F, 23F, 1.4F); coin4.setTextureSize(32, 32); coin4.mirror = true; setRotation(coin4, 0F, -2.9557F, -0.2974289F); } @Override public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { System.err.println("IM RENDERING THE MODEL AT X: " + f + "|Y: " + f1 + "|Z: " + f2); EntityCoinsRF coins = (EntityCoinsRF) entity; super.render(coins, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, coins); coin1.render(f5); if(coins.value < 100) { if(coins.value >= 25) { coin2.render(f5); } if(coins.value >= 50) { coin3.render(f5); } if(coins.value >= 75) { coin4.render(5); } } else if(coins.value < 1000) { if(coins.value >= 250) { coin2.render(f5); } if(coins.value >= 500) { coin3.render(f5); } if(coins.value >= 750) { coin4.render(5); } } else if(coins.value < 10000) { if(coins.value >= 2500) { coin2.render(f5); } if(coins.value >= 5000) { coin3.render(f5); } if(coins.value >= 7500) { coin4.render(5); } } else if(coins.value < 100000) { if(coins.value >= 25000) { coin2.render(f5); } if(coins.value >= 50000) { coin3.render(f5); } if(coins.value >= 75000) { coin4.render(5); } } } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } } Render Code: package realmoffera.client.render; import net.minecraft.client.renderer.entity.Render; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; import realmoffera.UtilRF; import realmoffera.client.model.ModelCoinsRF; import realmoffera.entity.EntityCoinsRF; public class RenderCoinsRF extends Render { public static final ResourceLocation COPPER = UtilRF.newResource("textures/entity/coins/copper.png"), SILVER = UtilRF.newResource("textures/entity/coins/silver.png"), GOLD = UtilRF.newResource("textures/entity/coins/gold.png"), PLATINUM = UtilRF.newResource("textures/entity/coins/platinum.png"); public static final ModelCoinsRF model = new ModelCoinsRF(); public RenderCoinsRF() { super(); } @Override public void doRender(Entity entity, double d, double d1, double d2, float d3, float d4) { System.err.println("IM RENDERING!"); EntityCoinsRF coins = (EntityCoinsRF) entity; this.bindTexture(getEntityTexture(coins)); model.render(coins, (float) d4, (float) d1, (float) d2, (float) d3, (float) d4, 1); } @Override protected ResourceLocation getEntityTexture(Entity entity) { EntityCoinsRF coins = (EntityCoinsRF) entity; return coins.value >= 100000 ? PLATINUM : (coins.value >= 10000 ? GOLD : (coins.value >= 1000 ? SILVER : COPPER)); } }
-
I've done some more experimenting, and it seems that my entity class isn't taking any renders? I tried registering the entity with RenderXPOrb and many others, but nothing pops up as an error.
-
You could add a print in the onUpdate method that has the entity's location.
-
Ok, I'm current branching from my last problem with my entity not spawning, I've got it to work. The only problem now, is that it doesn't render. Here's my code: Entity package realmoffera.entity; import net.minecraft.block.material.Material; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.DamageSource; import net.minecraft.util.MathHelper; import net.minecraft.world.World; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class EntityCoinsRF extends Entity { public int value = 10; public EntityCoinsRF(World world) { super(world); this.yOffset = 0.0F; this.setSize(0.5F, 0.5F); } public EntityCoinsRF(World world, int value, double x, double y, double z) { this(world); this.value = value; setLocationAndAngles(x, y, z, MathHelper.wrapAngleTo180_float(world.rand.nextFloat() * 360.0F), 0); } @Override protected void entityInit() { System.out.println("Initializing coins at X: " + posX + "|Y: " + posY + "|Z: " + posZ); } @Override public boolean attackEntityFrom(DamageSource par1DamageSource, float par2) { return false; } @Override public void onUpdate() { System.out.println("Updating at X: " + posX + "|Y: " + posY + "|Z: " + posZ); super.onUpdate(); } @Override public void onCollideWithPlayer(EntityPlayer player) { System.out.println("Colliding with player!"); super.onCollideWithPlayer(player); NBTTagCompound nbt = player.getEntityData().getCompoundTag(EntityPlayer.PERSISTED_NBT_TAG); nbt.setInteger("Coins", nbt.getInteger("Coins") + value); setDead(); } @Override public void readEntityFromNBT(NBTTagCompound nbt) { value = nbt.getInteger("Value"); } @Override public void writeEntityToNBT(NBTTagCompound nbt) { nbt.setInteger("Value", value); } @Override public boolean canAttackWithItem() { return false; } @Override protected boolean canTriggerWalking() { return false; } @Override public boolean canBePushed() { return true; } @Override public boolean handleWaterMovement() { return this.worldObj.handleMaterialAcceleration(this.boundingBox, Material.water, this); } @Override @SideOnly(Side.CLIENT) public void performHurtAnimation() { } @Override protected void dealFireDamage(int par1) { } } Render package realmoffera.client.render; import net.minecraft.client.renderer.entity.Render; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; import realmoffera.UtilRF; import realmoffera.client.model.ModelCoinsRF; import realmoffera.entity.EntityCoinsRF; public class RenderCoinsRF extends Render { public static final ResourceLocation COPPER = UtilRF.newResource("textures/entity/coins/copper.png"), SILVER = UtilRF.newResource("textures/entity/coins/silver.png"), GOLD = UtilRF.newResource("textures/entity/coins/gold.png"), PLATINUM = UtilRF.newResource("textures/entity/coins/platinum.png"); public static final ModelCoinsRF model = new ModelCoinsRF(); @Override public void doRender(Entity entity, double d, double d1, double d2, float d3, float d4) { System.err.println("IM RENDERING!"); EntityCoinsRF coins = (EntityCoinsRF) entity; this.bindTexture(getEntityTexture(coins)); model.render(coins, (float) d4, (float) d1, (float) d2, (float) d3, (float) d4, 1); } @Override protected ResourceLocation getEntityTexture(Entity entity) { EntityCoinsRF coins = (EntityCoinsRF) entity; return coins.value >= 100000 ? PLATINUM : (coins.value >= 10000 ? GOLD : (coins.value >= 1000 ? SILVER : COPPER)); } } Model package realmoffera.client.model; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; import realmoffera.entity.EntityCoinsRF; public class ModelCoinsRF extends ModelBase { ModelRenderer coin1; ModelRenderer coin2; ModelRenderer coin3; ModelRenderer coin4; public ModelCoinsRF() { textureWidth = 32; textureHeight = 32; coin1 = new ModelRenderer(this, 0, 0); coin1.addBox(0F, 0F, 0F, 4, 1, 4); coin1.setRotationPoint(-2F, 23F, -2F); coin1.setTextureSize(32, 32); coin1.mirror = true; setRotation(coin1, 0F, 0F, 0F); coin2 = new ModelRenderer(this, 0, 0); coin2.addBox(0F, 0F, 2F, 4, 1, 4); coin2.setRotationPoint(-1F, 23F, 7F); coin2.setTextureSize(32, 32); coin2.mirror = true; setRotation(coin2, 0.1858931F, 2.918521F, 0.0371786F); coin3 = new ModelRenderer(this, 0, 0); coin3.addBox(0F, 0F, 0F, 4, 1, 4); coin3.setRotationPoint(-2F, 23F, -5F); coin3.setTextureSize(32, 32); coin3.mirror = true; setRotation(coin3, 0.0371786F, -0.6878043F, -0.3346075F); coin4 = new ModelRenderer(this, 0, 0); coin4.addBox(0F, 0F, 0F, 4, 1, 4); coin4.setRotationPoint(4.2F, 23F, 1.4F); coin4.setTextureSize(32, 32); coin4.mirror = true; setRotation(coin4, 0F, -2.9557F, -0.2974289F); } public void render(EntityCoinsRF coins, float f, float f1, float f2, float f3, float f4, float f5) { super.render(coins, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, coins); coin1.render(f5); if(coins.value < 100) { if(coins.value >= 25) { coin2.render(f5); } if(coins.value >= 50) { coin3.render(f5); } if(coins.value >= 75) { coin4.render(5); } } else if(coins.value < 1000) { if(coins.value >= 250) { coin2.render(f5); } if(coins.value >= 500) { coin3.render(f5); } if(coins.value >= 750) { coin4.render(5); } } else if(coins.value < 10000) { if(coins.value >= 2500) { coin2.render(f5); } if(coins.value >= 5000) { coin3.render(f5); } if(coins.value >= 7500) { coin4.render(5); } } else if(coins.value < 100000) { if(coins.value >= 25000) { coin2.render(f5); } if(coins.value >= 50000) { coin3.render(f5); } if(coins.value >= 75000) { coin4.render(5); } } } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { this.render((EntityCoinsRF) entity, f, f1, f2, f3, f4, f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } } Render Registering package realmoffera.helper; import realmoffera.UtilRF; import realmoffera.client.render.RenderBatRF; import realmoffera.client.render.RenderBipedRF; import realmoffera.client.render.RenderCoinsRF; import realmoffera.client.render.RenderMummyRF; import realmoffera.entity.EntityCaveBatRF; import realmoffera.entity.EntityCoinsRF; import realmoffera.entity.EntityGoblinArcherRF; import realmoffera.entity.EntityGoblinScoutRF; import realmoffera.entity.EntityGoblinWarriorRF; import realmoffera.entity.EntityMummyRF; import realmoffera.entity.EntityPaladinRF; import cpw.mods.fml.client.registry.RenderingRegistry; public class RenderHelperRF { public static void init() { RenderingRegistry.registerEntityRenderingHandler(EntityMummyRF.class, new RenderMummyRF(UtilRF.newResource("textures/entity/mummy.png"))); RenderingRegistry.registerEntityRenderingHandler(EntityGoblinScoutRF.class, new RenderBipedRF(UtilRF.newResource("textures/entity/goblin.png"))); RenderingRegistry.registerEntityRenderingHandler(EntityCaveBatRF.class, new RenderBatRF(UtilRF.newResource("textures/entity/caveBat.png"))); RenderingRegistry.registerEntityRenderingHandler(EntityPaladinRF.class, new RenderBipedRF(UtilRF.newResource("textures/entity/paladin.png"), 1, 1.5F)); RenderingRegistry.registerEntityRenderingHandler(EntityGoblinWarriorRF.class, new RenderBipedRF(UtilRF.newResource("textures/entity/goblin.png"))); RenderingRegistry.registerEntityRenderingHandler(EntityGoblinArcherRF.class, new RenderBipedRF(UtilRF.newResource("textures/entity/goblin.png"))); RenderingRegistry.registerEntityRenderingHandler(EntityCoinsRF.class, new RenderCoinsRF()); } } All of my other entities are spawning and rendering properly, and all the prints in the entity code is being called when they're supposed to. I have a print in my render method to see if it's rendering. It's never called.
-
Also forgot to mention, I'm using 10.12.0.1047 (Latest for 1.7.2).
-
I get this when I try using the eclipse folder as my workspace: Here are the steps I took: 1. Extract forge src into my "Minecraft" folder 2. Opened a command window inside the "Minecraft" folder 3. Typed in "gradlew setupDevWorkspace" 4. Opened Eclipse 5. Used the eclipse folder as my workspace Here's the console when it was finished:
-
I've asked friends about it, and all they did was extend EntityMob. Is there any other way besides making it a mob? I also experimented with it some more, and it seems that it initializes, never gets set dead, and is never updates. Code: package realmoffera.entity; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class EntityCoinsRF extends Entity { public int value = 10; public EntityCoinsRF(World world) { super(world); this.yOffset = 0.0F; this.setSize(0.5F, 0.5F); } public EntityCoinsRF(World world, int value) { this(world); this.value = value; } @Override public void onUpdate() { super.onUpdate(); System.err.println("UPDATING"); } @Override public void onCollideWithPlayer(EntityPlayer player) { NBTTagCompound nbt = player.getEntityData().getCompoundTag(EntityPlayer.PERSISTED_NBT_TAG); nbt.setInteger("Coins", nbt.getInteger("Coins") + value); } @Override protected void entityInit() { } @Override protected void readEntityFromNBT(NBTTagCompound nbt) { value = nbt.getInteger("Value"); } @Override protected void writeEntityToNBT(NBTTagCompound nbt) { nbt.setInteger("Value", value); } @Override public boolean canAttackWithItem() { return false; } @Override protected boolean canTriggerWalking() { return false; } @Override public boolean canBePushed() { return true; } @Override @SideOnly(Side.CLIENT) public void performHurtAnimation() { } }
-
What I would do is just store the values in the player's NBT and sync it with packets. If you're trying to make it persist through death, store the values in the persistent NBT of the player.
-
Same crash, I think this is because the equipment slots haven't been initialized yet.
-
I'm using the spawn egg. I've also registered its render class.
-
package realmoffera.entity; import net.minecraft.enchantment.Enchantment; import net.minecraft.enchantment.EnchantmentHelper; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.EnumCreatureAttribute; import net.minecraft.entity.IEntityLivingData; import net.minecraft.entity.IRangedAttackMob; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.EntityAIArrowAttack; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityArrow; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.DamageSource; import net.minecraft.world.World; import realmoffera.UtilRF; import realmoffera.world.WorldSavedDataRF; public class EntityGoblinArcherRF extends EntityMob implements IRangedAttackMob { private EntityAIArrowAttack aiArrowAttack = new EntityAIArrowAttack(this, 1.0D, 20, 60, 15.0F); private EntityAIAttackOnCollide aiAttackOnCollide = new EntityAIAttackOnCollide(this, EntityPlayer.class, 1.2D, false); public EntityGoblinArcherRF(World world) { super(world); this.tasks.addTask(1, new EntityAISwimming(this)); this.tasks.addTask(5, new EntityAIWander(this, 1.0D)); this.tasks.addTask(6, new EntityAIWatchClosest(this, EntityPlayer.class, 8.0F)); this.tasks.addTask(6, new EntityAILookIdle(this)); this.targetTasks.addTask(1, new EntityAIHurtByTarget(this, false)); this.targetTasks.addTask(2, new EntityAINearestAttackableTarget(this, EntityPlayer.class, 0, true)); if(world != null && !world.isRemote) { this.setCombatTask(); } this.setSize(0.6F, 1.8F); } @Override protected void entityInit() { super.entityInit(); this.addRandomArmor(); this.enchantEquipment(); } @Override public IEntityLivingData onSpawnWithEgg(IEntityLivingData par1EntityLivingData) { super.onSpawnWithEgg(par1EntityLivingData); this.tasks.addTask(4, this.aiArrowAttack); //this.addRandomArmor(); //this.enchantEquipment(); return par1EntityLivingData; } @Override protected void addRandomArmor() { super.addRandomArmor(); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(4, new ItemStack(Items.leather_helmet)); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(3, new ItemStack(Items.leather_chestplate)); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(2, new ItemStack(Items.leather_leggings)); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(1, new ItemStack(Items.leather_boots)); this.setCurrentItemOrArmor(0, new ItemStack(Items.bow)); } @Override protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(80.0D); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.24000000417232513D); this.getEntityAttribute(SharedMonsterAttributes.attackDamage).setBaseValue(0D); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(10.0D); } @Override protected boolean isAIEnabled() { return true; } public void setCombatTask() { this.tasks.removeTask(this.aiAttackOnCollide); this.tasks.removeTask(this.aiArrowAttack); this.tasks.addTask(4, this.aiArrowAttack); } @Override protected int getExperiencePoints(EntityPlayer player) { return super.getExperiencePoints(player); } @Override public void onLivingUpdate() { if(!worldObj.isRemote && !WorldSavedDataRF.forWorld(worldObj).isPostDragon()) { this.damageEntity(DamageSource.magic, 1000); } else if(!worldObj.isRemote && WorldSavedDataRF.forWorld(worldObj).isPostDragon()) { } super.onLivingUpdate(); } @Override public boolean attackEntityFrom(DamageSource dmgSrc, float par2) { if(this.isBurning() && UtilRF.getChance(1, 5) && dmgSrc.getEntity() instanceof EntityLiving) { dmgSrc.getEntity().setFire(UtilRF.getRandomIntegerBetween(5, 10)); } return super.attackEntityFrom(dmgSrc, par2); } @Override public EnumCreatureAttribute getCreatureAttribute() { return EnumCreatureAttribute.UNDEAD; } protected Item getDropItem() { return Items.arrow; } protected void dropFewItems(boolean par1, int par2) { int j; int k; j = this.rand.nextInt(3 + par2); for(k = 0; k < j; ++k) { this.dropItem(Items.arrow, 1); } } @Override public void attackEntityWithRangedAttack(EntityLivingBase par1EntityLivingBase, float par2) { EntityArrow entityarrow = new EntityArrow(this.worldObj, this, par1EntityLivingBase, 1.6F, (float) (14 - this.worldObj.difficultySetting.getDifficultyId() * 4)); int i = EnchantmentHelper.getEnchantmentLevel(Enchantment.power.effectId, this.getHeldItem()); int j = EnchantmentHelper.getEnchantmentLevel(Enchantment.punch.effectId, this.getHeldItem()); entityarrow.setDamage((double) (par2 * 2.0F) + this.rand.nextGaussian() * 0.25D + (double) ((float) this.worldObj.difficultySetting.getDifficultyId() * 0.11F)); if(i > 0) { entityarrow.setDamage(entityarrow.getDamage() + (double) i * 0.5D + 0.5D); } if(j > 0) { entityarrow.setKnockbackStrength(j); } if(EnchantmentHelper.getEnchantmentLevel(Enchantment.flame.effectId, this.getHeldItem()) > 0) { entityarrow.setFire(100); } this.playSound("random.bow", 1.0F, 1.0F / (this.getRNG().nextFloat() * 0.4F + 0.8F)); this.worldObj.spawnEntityInWorld(entityarrow); } public double getYOffset() { return super.getYOffset() - 0.5D; } }
-
Already tried that, error. java.lang.reflect.InvocationTargetException at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method) at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:57) at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45)[12:43:46] [server thread/WARN]: Skipping Entity with id 24 at java.lang.reflect.Constructor.newInstance(Constructor.java:526) at net.minecraft.entity.EntityList.createEntityByID(EntityList.java:233) at net.minecraft.item.ItemMonsterPlacer.spawnCreature(ItemMonsterPlacer.java:173) at net.minecraft.item.ItemMonsterPlacer.onItemUse(ItemMonsterPlacer.java:79) at net.minecraft.item.ItemStack.tryPlaceItemIntoWorld(ItemStack.java:150) at net.minecraft.server.management.ItemInWorldManager.activateBlockOrUseItem(ItemInWorldManager.java:424) at net.minecraft.network.NetHandlerPlayServer.processPlayerBlockPlacement(NetHandlerPlayServer.java:596) at net.minecraft.network.play.client.C08PacketPlayerBlockPlacement.processPacket(C08PacketPlayerBlockPlacement.java:74) at net.minecraft.network.play.client.C08PacketPlayerBlockPlacement.processPacket(C08PacketPlayerBlockPlacement.java:122) at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:242) at net.minecraft.network.NetworkSystem.networkTick(NetworkSystem.java:190) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:763) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:651) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:120) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:530) at net.minecraft.server.MinecraftServer$2.run(MinecraftServer.java:788) Caused by: java.lang.NullPointerException at net.minecraft.entity.EntityLiving.setCurrentItemOrArmor(EntityLiving.java:832) at realmoffera.entity.EntityGoblinArcherRF.addRandomArmor(EntityGoblinArcherRF.java:72) at realmoffera.entity.EntityGoblinArcherRF.entityInit(EntityGoblinArcherRF.java:51) at net.minecraft.entity.Entity.<init>(Entity.java:286) at net.minecraft.entity.EntityLivingBase.<init>(EntityLivingBase.java:203) at net.minecraft.entity.EntityLiving.<init>(EntityLiving.java:98) at net.minecraft.entity.EntityCreature.<init>(EntityCreature.java:44) at net.minecraft.entity.monster.EntityMob.<init>(EntityMob.java:21) at realmoffera.entity.EntityGoblinArcherRF.<init>(EntityGoblinArcherRF.java:35) ... 19 more java.lang.reflect.InvocationTargetException at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method) at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:57) at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45) at java.lang.reflect.Constructor.newInstance(Constructor.java:526) at net.minecraft.entity.EntityList.createEntityByID(EntityList.java:233) at net.minecraft.item.ItemMonsterPlacer.spawnCreature(ItemMonsterPlacer.java:173) at net.minecraft.item.ItemMonsterPlacer.onItemUse(ItemMonsterPlacer.java:79) at net.minecraft.item.ItemStack.tryPlaceItemIntoWorld(ItemStack.java:150) at net.minecraft.server.management.ItemInWorldManager.activateBlockOrUseItem(ItemInWorldManager.java:424) at net.minecraft.network.NetHandlerPlayServer.processPlayerBlockPlacement(NetHandlerPlayServer.java:596) at net.minecraft.network.play.client.C08PacketPlayerBlockPlacement.processPacket(C08PacketPlayerBlockPlacement.java:74) at net.minecraft.network.play.client.C08PacketPlayerBlockPlacement.processPacket(C08PacketPlayerBlockPlacement.java:122) at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:242) at net.minecraft.network.NetworkSystem.networkTick(NetworkSystem.java:190) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:763) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:651) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:120) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:530) at net.minecraft.server.MinecraftServer$2.run(MinecraftServer.java:788) Caused by: java.lang.NullPointerException at net.minecraft.entity.EntityLiving.setCurrentItemOrArmor(EntityLiving.java:832) at realmoffera.entity.EntityGoblinArcherRF.addRandomArmor(EntityGoblinArcherRF.java:75) at realmoffera.entity.EntityGoblinArcherRF.entityInit(EntityGoblinArcherRF.java:51) at net.minecraft.entity.Entity.<init>(Entity.java:286) at net.minecraft.entity.EntityLivingBase.<init>(EntityLivingBase.java:203) at net.minecraft.entity.EntityLiving.<init>(EntityLiving.java:98) at net.minecraft.entity.EntityCreature.<init>(EntityCreature.java:44) at net.minecraft.entity.monster.EntityMob.<init>(EntityMob.java:21) at realmoffera.entity.EntityGoblinArcherRF.<init>(EntityGoblinArcherRF.java:35) ... 19 more
-
I'm overriding that method and adding the armor, but it seems to only be called when I spawn with a spawn egg. If I spawn it naturally, nothing happens. @Override public IEntityLivingData onSpawnWithEgg(IEntityLivingData par1EntityLivingData) { super.onSpawnWithEgg(par1EntityLivingData); this.tasks.addTask(4, this.aiArrowAttack); this.addRandomArmor(); this.enchantEquipment(); return par1EntityLivingData; } @Override protected void addRandomArmor() { super.addRandomArmor(); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(4, new ItemStack(Items.leather_helmet)); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(3, new ItemStack(Items.leather_chestplate)); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(2, new ItemStack(Items.leather_leggings)); if(UtilRF.getChance(1, 3)) this.setCurrentItemOrArmor(1, new ItemStack(Items.leather_boots)); this.setCurrentItemOrArmor(0, new ItemStack(Items.bow)); }
-
I have this simple entity here: package realmoffera.entity; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class EntityCoinsRF extends Entity { public int value = 10; public EntityCoinsRF(World world) { super(world); } public EntityCoinsRF(World world, int value) { super(world); this.value = value; } @Override public void onCollideWithPlayer(EntityPlayer player) { NBTTagCompound nbt = player.getEntityData().getCompoundTag(EntityPlayer.PERSISTED_NBT_TAG); nbt.setInteger("Coins", nbt.getInteger("Coins") + value); } @Override protected void entityInit() { System.err.println("Initializing coins."); } @Override protected void readEntityFromNBT(NBTTagCompound nbt) { value = nbt.getInteger("Value"); } @Override protected void writeEntityToNBT(NBTTagCompound nbt) { nbt.setInteger("Value", value); } @Override public boolean canAttackWithItem() { return false; } @Override protected boolean canTriggerWalking() { return false; } @Override public boolean canBePushed() { return false; } @Override @SideOnly(Side.CLIENT) public void performHurtAnimation() { } } The problem is that it says that it has been initialized, but it never appears. I've tried overriding the setDead method and seeing if it's being called, but it isn't. Any ideas?
-
I actually want to add the random armor on my own mob.
-
I know how to render a living entity, but I have no clue how to render just an entity that has a model. Render: package realmoffera.client.render; import net.minecraft.client.renderer.entity.Render; import net.minecraft.entity.Entity; import net.minecraft.util.ResourceLocation; import realmoffera.UtilRF; import realmoffera.client.model.ModelCoinsRF; import realmoffera.entity.EntityCoinsRF; public class RenderCoinsRF extends Render { public static final ResourceLocation COPPER = UtilRF.newResource("textures/entity/coins/copper.png"), SILVER = UtilRF.newResource("textures/entity/coins/silver.png"), GOLD = UtilRF.newResource("textures/entity/coins/gold.png"), PLATINUM = UtilRF.newResource("textures/entity/coins/platinum.png"); public static final ModelCoinsRF model = new ModelCoinsRF(); @Override public void doRender(Entity entity, double d, double d1, double d2, float d3, float d4) { } protected ResourceLocation getEntityTexture(EntityCoinsRF coins) { return coins.value >= 100000 ? PLATINUM : (coins.value >= 10000 ? GOLD : (coins.value >= 1000 ? SILVER : COPPER)); } @Override protected ResourceLocation getEntityTexture(Entity e) { return getEntityTexture((EntityCoinsRF) e); } } Model: package realmoffera.client.model; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; import realmoffera.entity.EntityCoinsRF; public class ModelCoinsRF extends ModelBase { ModelRenderer coin1; ModelRenderer coin2; ModelRenderer coin3; ModelRenderer coin4; public ModelCoinsRF() { textureWidth = 32; textureHeight = 32; coin1 = new ModelRenderer(this, 0, 0); coin1.addBox(0F, 0F, 0F, 4, 1, 4); coin1.setRotationPoint(-2F, 23F, -2F); coin1.setTextureSize(32, 32); coin1.mirror = true; setRotation(coin1, 0F, 0F, 0F); coin2 = new ModelRenderer(this, 0, 0); coin2.addBox(0F, 0F, 2F, 4, 1, 4); coin2.setRotationPoint(-1F, 23F, 7F); coin2.setTextureSize(32, 32); coin2.mirror = true; setRotation(coin2, 0.1858931F, 2.918521F, 0.0371786F); coin3 = new ModelRenderer(this, 0, 0); coin3.addBox(0F, 0F, 0F, 4, 1, 4); coin3.setRotationPoint(-2F, 23F, -5F); coin3.setTextureSize(32, 32); coin3.mirror = true; setRotation(coin3, 0.0371786F, -0.6878043F, -0.3346075F); coin4 = new ModelRenderer(this, 0, 0); coin4.addBox(0F, 0F, 0F, 4, 1, 4); coin4.setRotationPoint(4.2F, 23F, 1.4F); coin4.setTextureSize(32, 32); coin4.mirror = true; setRotation(coin4, 0F, -2.9557F, -0.2974289F); } public void render(EntityCoinsRF coins, float f, float f1, float f2, float f3, float f4, float f5) { super.render(coins, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, coins); coin1.render(f5); if(coins.value < 100) { if(coins.value >= 25) { coin2.render(f5); } if(coins.value >= 50) { coin3.render(f5); } if(coins.value >= 75) { coin4.render(5); } } else if(coins.value < 1000) { if(coins.value >= 250) { coin2.render(f5); } if(coins.value >= 500) { coin3.render(f5); } if(coins.value >= 750) { coin4.render(5); } } else if(coins.value < 10000) { if(coins.value >= 2500) { coin2.render(f5); } if(coins.value >= 5000) { coin3.render(f5); } if(coins.value >= 7500) { coin4.render(5); } } else if(coins.value < 100000) { if(coins.value >= 25000) { coin2.render(f5); } if(coins.value >= 50000) { coin3.render(f5); } if(coins.value >= 75000) { coin4.render(5); } } } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { this.render((EntityCoinsRF) entity, f, f1, f2, f3, f4, f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } } Basically what I'm asking is, what do I put in the doRender method in the render. There's a render method in my model class but I'm not sure what the parameters are.
-
(1.7.2) How many ticks does the world get each second?
TLHPoE replied to TLHPoE's topic in Modder Support
It's doing the counting server-side. I'm pretty sure, I registered the event in my ServerProxy and I have a !world.isRemote check. -
I've been trying this for a while now. I've looked at skeleton and zombie code, but it calls the addArmor method on spawn with an egg though. Do I need to call the method myself outside of the entity code?
-
(1.7.2) How many ticks does the world get each second?
TLHPoE replied to TLHPoE's topic in Modder Support
Actually, thanks to the power of my key board's metronome, I've found out the world gets updated 40 times each second. -
I'm using the WorldTickEvent event if that affects the answer.
-
I had some misspelled folder names, that was about it.
-
[1.7.2] How to make a player invisible to mobs?
TLHPoE replied to SackCastellon's topic in Modder Support
I'm not sure if this would be the most efficient way, but: 1. Setup an entity tick event 2. Check if entity is a mob 3. Check if mob has a target (the player) 4. Remove if it does -
I'm not sure about this, but I think you're building your mod on a newer forge than what you're trying to play it with.
-
You need to multiply chunkX and chunkZ by 16 before passing it on to the other generate methods.