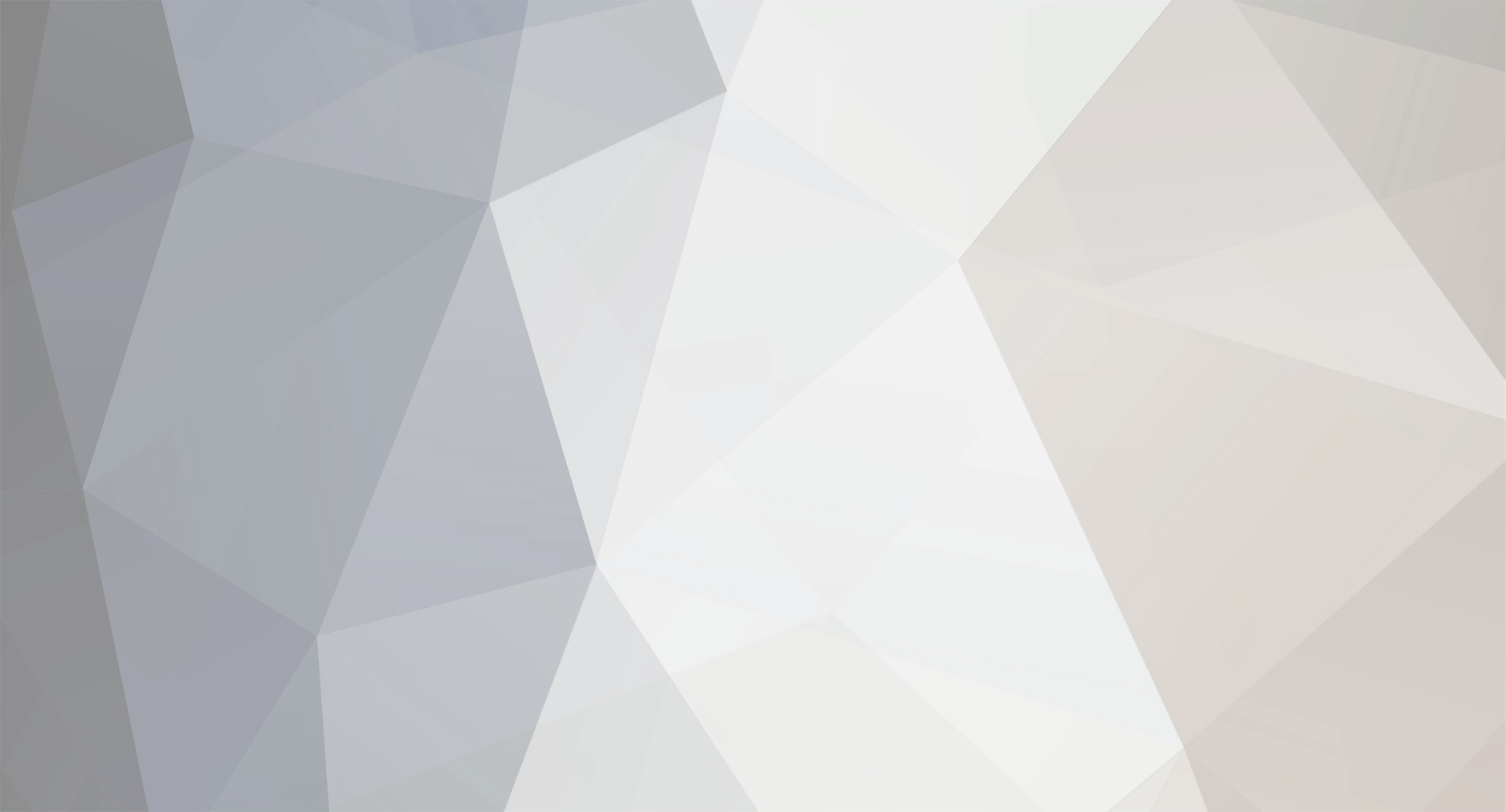
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.7.10] [SOLVED] Entity.SetSize cannot be called outside constructor?
coolAlias replied to Frag's topic in Modder Support
A possible simple solution could be to just let whatever code you have that calls #setSize run on both sides. Another solution that will allow you to avoid making your own packets is to use World#setEntityState in combination with your entity's #handleHealthUpdate method, like so: // define a couple flags: private static final int SIZE_SMALL = 5, SIZE_MEDIUM = 6, SIZE_LARGE = 7, etc.; // when your entity changes its size on the server: if (!worldObj.isRemote) { // whatever your setSize logic is, e.g. switch (currentSize) { case SIZE_SMALL: setSize(1, 1); break; case SIZE_MEDIUM: setSize(2, 2); break; // etc. } // call #setEntityState to send a flag to the client worldObj.setEntityState(currentSize); } // override #handleHealthUpdate to handle your cases @Override @SideOnly(Side.CLIENT) public void handleHealthUpdate(int flag) { switch (flag) { case SIZE_SMALL: setSize(1, 1); break; case SIZE_MEDIUM: setSize(2, 2); break; // etc. default: super.handleHealthUpdate(flag); // super class often handles various states - check for conflicts } } Just a couple ideas. Sending your own packet should work too, of course. -
Almost, and maybe, depending on what you want to do. Think of it this way: if the ItemStack doesn't have a tag compound, what should the addInformation method display about the cooldown time? In other words, the stack doesn't have any information about a cooldown yet, so what are you going to display? And if it does have that information, what do you want to display? So: if (stack.getTagCompound() == null) { // tag means no cooldown information available } else { // there is a tag, so maybe it has some information about the cooldown }
-
You do realize that ItemStack's do not have an NBTTagCompound by default, right? So the tag can be null, but you do not check for it: // itemStack.NULL.CRASH_HERE because null doesn't have any methods int coolDown = itemStack.stackTagCompound.getInteger("coolDown");
-
Well, it looks like you are trying to replace the player inventory, so have you implemented an IExtendedEntityProperties class that saves and loads the extra slots? The IInventory specification does not have any save or load methods, so any that you have would either be inherited from the parent class (which you don't have, and that's fine), or you need to make sure you call the methods yourself at an appropriate time (such as from IEEP when it saves and loads).
-
You are using the proxy incorrectly, for one. You should have an instance of the base Proxy class (e.g. 'CommonProxy') in your main class, and then use that instance when you need to call any methods in your proxy. Note that you never actually need to create the instance, i.e. you don't call 'new Proxy()', as it is created for you by FML / Forge. When you call 'proxy.method()', the correct proxy is used based on the which side is making the call, so you don't need to do any side checks in your load function area when making calls to the proxy. Also, you don't want to register mod entities in the client proxy - they need to be registered in the common area of your mod, so they are available to both sides; only the rendering, key bindings, and other client-only stuff should be isolated in that manner. I'm sure there's more, but that should give you enough to clean up for now.
-
[1.7.10] Item dissapears after reopening GUI
coolAlias replied to jackmano's topic in Modder Support
NEVER create a new TileEntity instance. Always retrieve it from the world: // in both of your IGuiHandler methods, you have something similar to this: return new ContainerElectrolyzer(player.inventory, new TileMachineElectrolyzer()); // which should instead look like this: TileEntity te = world.getTileEntity(x, y, z); if (te instanceof TileMachineElectrolyzer) { return new ContainerElectrolyzer(player.inventory, (TileMachineElectrolyzer) te); } The reason is that the data is all stored with the TileEntity in existence already; if you create a new one, obviously it has no data yet, and any data you give it will never be saved because it doesn't exist in the world, only in memory. -
These are always tricky to debug, but the first place I'd start is your read/write to NBT methods in your IInventory class; specifically, in the read you have: compound.getTagList(tagName, 0); The 2nd argument is supposed to be the type of data stored in the list, in this case NBTTagCompounds, the type of which is represented by the constant Constants.NBT.TAG_COMPOUND, or you can use the magic number 10. I prefer the constant. Now, I don't know if that is the sole cause of your issue, but it's a place to begin. Also, your use of @Override is sporadic - some methods have it, but others that should, don't. Is this simply unintentional, or did you remove it from those other methods because it 'gave you an error' ? I didn't check the method signatures very carefully, but if your answer is the second, you will want to put it back and fix the method signatures.
-
That's usually a sign that you are using something that only works on the client; since your code isn't crashing on the server, clearly it's not an @SideOnly(Side.CLIENT) method, but it could be something as innocuous as a discrepancy in eye position (very common pre-1.. As Failender mentioned, explosions should only be created server-side, as they automatically notify the client of affected block positions and such; otherwise, you can end up with 'ghost' blocks, and that's never good. To find out what's going on, I suggest you use a Logger (automatically adds Side information to each message) and start spamming the console with information about the state of every single variable you have while in multiplayer vs. single player, then try to find the difference.
-
Well, your code is kind of weird: if (distanceToAir > 1) { return false; } Why even check how long it takes to get to an air block if you are just going to return any time the block directly above the y coordinate isn't air? I suspect there are probably more logic issues like this in your code, but on to the main issue: if you want to only generate your structure in a clear space, there is only one way to do so - check if the space is clear before generating it. To do that, you need to know the length, width, and height of your structure and the location at which it will be generating, then check all* of the blocks within that area. * If you are clever, you will write your code in a way that fails as early as possible so you don't waste lots of time continuing to check when it has already failed, and you can also write it in a way that allows for some non-air blocks, depending on how strict you want to be.
-
Why are you trying to store the inventory in the gun itself, rather than on the entity? Wouldn't it make more sense for the entity to have extra inventory slots to hold the ammo, so you can give it ammo at any time? Then, if it somehow loses the gun, it would still have the extra ammo. As to your problem, you should use the Constants.NBT utility class provided by Forge when retrieving tags, and understand that when retrieving an NBTTagList, the type you pass in is the type of object stored in the list, not the list type itself. // 6 = TAG_DOUBLE, are you storing a list of doubles? No. NBTTagList nbttaglist = tagCompund.getTagList("Inventory", 6); // you are storing a list of NBTTagCompounds, so use the appropriate type NBTTagList nbttaglist = tagCompund.getTagList("Inventory", Constants.NBT.TAG_COMPOUND);
-
Technically I suppose, but what do you expect to happen with that statement? What does that statement even say? It says: set the cooldown to ZERO. Look, this really shouldn't be that difficult - all it takes is sitting down for a second and thinking about what you want your code to do, in plain English, like this: When the player right-clicks on an entity with my item, the following should happen: 1. If the entity is a mob and the item is full, a message displays for the player 2. If the entity is a mob and the item is NOT full and NOT cooling down, the mob should immediately die, the item takes 1 point of damage, and the item goes on cooldown for X ticks Something like that, anyway. When you're done writing the work-flow, compare it to your code and see if your code is doing what you wrote. Also, ItemStacks GAIN damage, so you should be adding positive 1, not -1.
-
Well, the one that I marked in bold shouldn't even compile as it is not a method. Anyway, I bet your cooldown isn't working as you expect because in most cases, you never set it. Figuring out what is wrong in cases like this is a simple game of "print the value at different points in your code". In your #itemInteractionForEntity method, put a println in each of your if statements telling you where you are at; put another at the end of the method when you set the cooldown. Now start the game and right click on a couple entities, and see if you are ever seeing the last println - with all of those early return statements, I bet you won't see it.
-
Do you mean packets? I bet Google knows...
-
Yes, that is the fix for the error mentioned earlier, and now you know how to fix them yourself So are you still having an issue? If so, what, exactly?
-
So, you want to target the closest entity to the player that presses the key? Do you want to kill it directly, or attack it? Either way, you need to send a packet to the server and let the server decide who is closest, then call #setDead on that entity to kill it directly, or #attackEntityFrom to attack it. Again, do all of the actual damaging and killing and all that stuff on the server only.
-
You'll have to be more specific about what line you are getting an error on.
-
That depends - if you are using a KeyBinding, then Minecraft.getMinecraft().thePlayer is the player pressing the key, so I don't see how that is helpful to you. At the very least, if you really do want to attack from a KeyBinding, you will need some way to send a packet to the server so it can perform the attack. There are built-in ways, but again, the solution depends on your actual code. Show your code.
-
Lol oh man - the first things I checked were those 2 methods, but I didn't pay attention to which one was doing what, just that they were symmetrical. Hooray for silly mistakes that take forever to figure out!
-
I doubt he is very concerned if the code doesn't compile as written - it was supposed to be an example to help you understand the basic logic behind ItemStack NBT-based cooldowns, not production-ready code. Also, "dead code" in programming refers to code that will never be reached due to an impossible condition, not to a line with an error on it. To fix your error, delete 'itemStack.stackTagCompound' then type in 'itemStack.' with the period and stop typing; you should get a list of options - scroll through those until you find the one that will give you the stack's tag compound, which should be pretty obvious when you see it.
-
This could be totally wrong, but I recall reading somewhere that the channel name cannot be too long, something like 12 characters. I may have totally made that up, but try shortening your channel name to "inner_power" or whatever, and see if it works.
-
1.8 vs. 1.7.10 makes no difference - you should easily be able to adapt to any changes; the code he wrote doesn't even work in 1.8 exactly as written, but you shouldn't be blindly copying and pasting anyway. If you actually type the code, sure your IDE will pop up with hints about the correct methods / fields to use, no?
-
Well, did you fix that ^^^ ? Show your updated IMessage and IMessageHandler code, as well as where you register it.
-
I hate to say it, but you seem to just be spamming code without understanding one bit what it is doing. I mean, this: NBTTagCompound nbtTagCompound = new NBTTagCompound(); int coolDown = nbtTagCompound.getInteger("coolDown"); // what do you expect this value to be? I certainly hope you don't expect anything other than zero... // how could it be otherwise with a NEW tag compound??? or this: NBTTagCompound nbtTagCompound = new NBTTagCompound(); if(nbtTagCompound == null) { // dead code - how can it be null when you just initialized it??? it can't. } or this: if (entity instanceof EntityMob){ hasEffect(itemStack); break; } I mean come on... calling #hasEffect, which is a CLIENT-side only method that simply tells the renderer whether to render the enchanted glint or not... what the heck do you expect that code to even do? Please, take some time and read your code. Really read it. Then, understand that you don't just make new NBT tag compounds for the hell of it - you only do it when the ItemStack doesn't yet have one, and then you attach it to the ItemStack. From then on, you only get the tag from the ItemStack, you never make a new one. Look again at Ernio's code example and you will see what I'm talking about.
-
When you are in Eclipse, you can check which version of Forge you have referenced under 'Referenced Libraries'. There should also be a 'build.gradle' file in the root directory of your project - that is the one you want to edit, though you don't have to do it from within Eclipse. If, after editing that file and re-running gradlew's setup, you still do not have the updated version in your referenced libraries, try refreshing the workspace (F5) or running the setup command with the '--refresh-dependencies' argument. Sometimes even just opening up a package or file from within the Forge src (Minecraft or Forge) will cause it to update with its current version number, provided it was simply that the GUI was failing to update and not that Forge itself failed to update. If both of those fail, you may have to manually set the Forge path - right click on the forgeSrc under Referenced Libraries, go to 'Build Path -> Configure Build Path', then click Edit and navigate to the build you want to use.
-
No, the way he did it will allow you to display how much time is remaining before the next use. Anything you store in ItemStack NBT is generally synchronized to the client side as well, so even if you used a non-ticking cooldown such as storing the next world time at which it can be used, you could technically still calculate the time remaining for display purposes using e.g. nextAvailableUseTime - currentWorldTime.