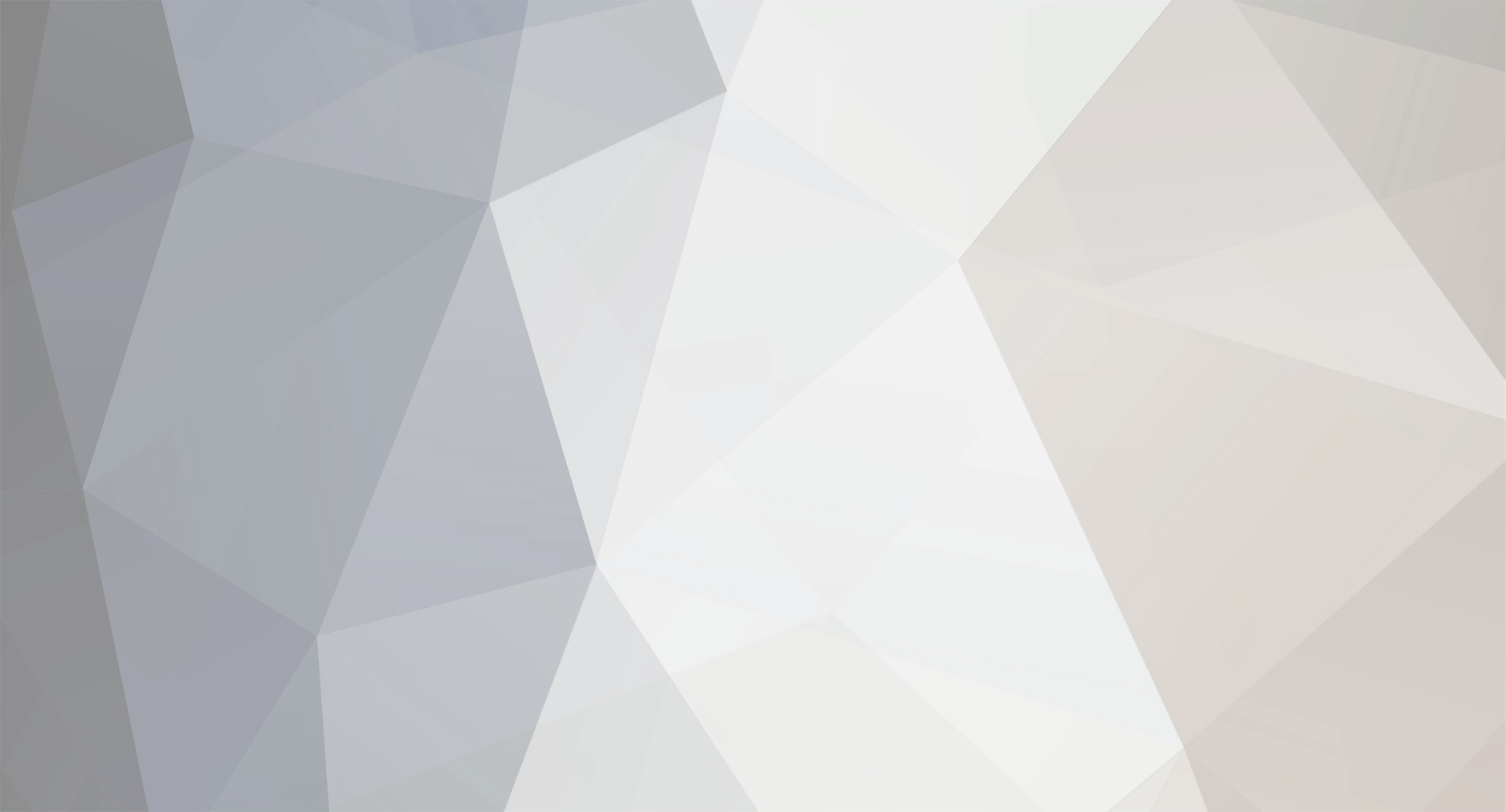
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Also, the two code snippets you have provided are very incompatible with each other: if (!worldObj.isRemote) { // true when on SERVER playSounds(); } if(isClient()){ // probably true when on CLIENT Minecraft.getMinecraft().getSoundHandler().playSound(this.startsound); } Assuming that the 2nd snippet is in your #playSounds method, there is a big problem. Otherwise, as everyone else has said, you need to post your actual code if you expect to receive help, not just tiny snippets of it. We need the full context of what's calling what where.
-
[1.7.10] Trying to scale an item with a custom IItemRenderer
coolAlias replied to Thalmias's topic in Modder Support
Do note that my code doesn't scale the item when dropped, as that wasn't really a priority for me. Shouldn't be difficult to add, though. -
[1.7.10] Trying to scale an item with a custom IItemRenderer
coolAlias replied to Thalmias's topic in Modder Support
Now that you've got it working, I'll show you mine If you don't plan to pass any arguments to your render class, you may as well just make one instance of it and pass it to each of your registrations: IItemRenderer renderer = new GreatswordRenderer(); MinecraftForgeClient.registerItemRenderer(ModItems.woodGreatsword, renderer); MinecraftForgeClient.registerItemRenderer(ModItems.stoneGreatsword, renderer); MinecraftForgeClient.registerItemRenderer(ModItems.ironGreatsword, renderer); MinecraftForgeClient.registerItemRenderer(ModItems.goldGreatsword, renderer); // etc. -
[1.7.10] Trying to scale an item with a custom IItemRenderer
coolAlias replied to Thalmias's topic in Modder Support
Do you actually call proxy.registerRenderers() from anywhere? Item#registerIcons, btw, is useful if you have more than one texture, e.g. sub-items, but is certainly redundant in your case as you said. I don't think I have ever seen anyone override #getIconIndex, though I'm sure there are use cases. -
The tutorial explains how to update to 1.8 - there is hardly anything that changed with respect to this particular tutorial, so if you can create an Item in 1.8, you can pretty much create a backpack. Also, the github link for the tutorial... there is a 1.8 branch...
-
[1.7.10] Trying to scale an item with a custom IItemRenderer
coolAlias replied to Thalmias's topic in Modder Support
Don't create a new instance of your item - use the ItemStack passed to the render method: // push matrix here // translate here // scale here IIcon icon = stack.getItem().getIcon(stack, 0); Tessellator tessellator = Tessellator.instance; ItemRenderer.renderItemIn2D(tessellator, icon.getMaxU(), icon.getMinV(), icon.getMinU(), icon.getMaxV(), icon.getIconWidth(), icon.getIconHeight(), 0.0625F); // pop matrix here With that, you don't even need to hard-code your resource location, so you can use it for any item that you want to render large. You'll have to play around a little with the translation values to get your item in the correct location, depending on the scale you use. -
How do you know it's not printing on the server? Are you checking the server output log? Also, why are you so certain it is printing on the client and not the server? "Boing" isn't exactly descriptive, especially when using System.out.println instead of a Logger.
-
Saving "entity rendering" data on the client side
coolAlias replied to jabelar's topic in Modder Support
Us developers notice a lot of things during testing - I highly doubt anyone else is going to be logging in and out as much as we do -
Saving "entity rendering" data on the client side
coolAlias replied to jabelar's topic in Modder Support
Maybe, but in all the games I've played, if something has a long dying animation, to use your example, and I quit while it is in progress, when I reload the mob is just dead and in the carcass position, rather than replaying the animation. Wouldn't that make more sense, anyway? Since the entity was dying, you quit and come back, so now it should be dead, not still dying. Even for other animations, the animation is typically just eye candy, no matter how long it is, and no one will even notice that it isn't starting in the same place when they log back in, nor would they expect it to. Just my 2 cents. -
Unless it is something that Minecraft handles for you, like sneaking, then no, there is no way to check if a key is pressed on the server side without sending packets. You're going to have to learn how to use packets someday anyway, most likely, so may as well start now. It's really not that hard once you wrap your head around it.
-
Saving "entity rendering" data on the client side
coolAlias replied to jabelar's topic in Modder Support
The thing is, animations in Minecraft are NOT saved. The server typically doesn't care about what the renderer is doing, nor should it, and what is rendering shouldn't have any effect on anything else, even though it may appear that way to the player ("oh, the boss attacks each time after it finishes this funky dance - I'd better watch carefully so I can dodge!"). In some cases, the animation may even send an 'animation complete' packet to trigger things on the server, so it is somewhat linked, but that's about the extent of it. The server says "golem is attacking now" or "mob is moving" and lets the client know, and the client then does whatever animation it wants. When the game reloads, though, the entity is neither attacking nor moving, so the animation state starts at zero (although that zero may be for a certain 'state', e.g. sitting, standing, prone, etc., sent from the server upon loading). I get what you are trying to do, but I guess I don't understand why you need the animation state to save - why not just reset it like vanilla? Will your animations really be stopping mid-way through when the game saves, and need to start at exactly the right spot when it loads? This seems unlikely, and I doubt there are many or possibly even any games that have coded animations that way. -
Saving "entity rendering" data on the client side
coolAlias replied to jabelar's topic in Modder Support
Not entirely true. You say the server initiates the animation, and the animation is predictable i.e. on a schedule, so why can't the server have the same timer counting down? Look at EntityIronGolem's attack timer - it is used on both server and client and is basically exactly what you want. Just save it to the regular entity NBT and implement IEntityAdditionalSpawnData to send the current animation point upon loading like someone else suggested. -
You already checked if the player is sneaking, so why also check the Keyboard input? Keyboard and all other input is CLIENT-side only, so not only should it NOT work on the server, it should generally crash it. Code like this: if (!world.isRemote) { // on the server if (Keyboard or Mouse or any other client-side code) { // will epically fail } } Just get rid of the Keyboard check and you should be fine without needing any packets at all. ItemStack NBT is generally sent automatically to the client, but you wouldn't need it there anyway unless you wanted to display the coordinates in the tooltip or something.
-
Saving "entity rendering" data on the client side
coolAlias replied to jabelar's topic in Modder Support
Why can't the server also run the animation counter or whatever you are using to control the stage? Similar to how Minecraft runs many methods on both sides - it's not usually application-critical that the animation is correctly timed, so you can allow a little leeway if the server and client get out of sync; if it is critical, then the only way is to send packets, but keep in mind that rendering can happen multiple times per tick, if you want to get to that level of precision. -
[1.8] Get all EntityLiving entities near a pos
coolAlias replied to ThatBenderGuy's topic in Modder Support
The arguments for AxisAlignedBB are MINIMUM x/y/z followed by MAXIMUM x/y/z; are you absolutely certain that the baseNodePos x/y/z will always be less than the connected node entry's x/y/z? That doesn't seem very likely to me... -
[1.8] Get all EntityLiving entities near a pos
coolAlias replied to ThatBenderGuy's topic in Modder Support
There isn't an analogous function, no, but you can use World#getEntitiesWithinAABB to get nearby entities, then iterate through that list to see which is closes (see World#getClosestPlayer if you need an example of that). -
Yes, the event fires any time a client player starts receiving updates from the server about any entity, e.g. in order to render that entity on the screen. You probably don't need to send anything when the player joins the world except to that 1 player (so they can render their items in 3rd person); other players will be handled by the StartTracking event. You shouldn't need an ArrayList to store any players at all - that's the whole point of the EntityTracker: use the method that gives you all tracking players for an entity. That's all you need.
-
That's the problem with syncing only from #detectAndSendChanges - you should also send the inventory update packet to all tracking players from PlayerEvent.StartTracking, and the combination should cover all your bases. As for your EDIT 2 about needing to clear the inventory, that's true because if the slot wasn't sent in the packet, that means there was nothing in it, but the client will not write to that slot in the basic NBTTagList-style loop.
-
Those network registrations should NOT have caused a crash - you're crashing because you have @SideOnly(Side.CLIENT) on your ClientHandler class, which I mentioned earlier you shouldn't. If that wasn't the cause of the crash, post the crash log. Anyway, I'm not sure about the rendering. It could be something to do with the current render view entity, as every player posts the RenderPlayer event for every other player, meaning: Player A w/ dirt block Player B w/ log block Every render tick: Player A: renders self w/ dirt block renders player B, but current GL matrix is relative to current player A, so log shows up at A's feet Player B: renders self w/ log block renders player A, but current GL matrix is relative to current player B, so log shows up at B's feet Just a guess. I haven't messed with that kind of rendering too much, and what little I did was many months ago (probably over a year).
-
You can tell which context you are in in one of several ways: 1. Experience 2. If the class or method has @SideOnly, it's pretty obvious 3. By checking if the world object (from an entity, method, or wherever else) is remote - if world.isRemote is true, that means the code is currently running on the client. 4. By using an actual Logger to output messages to the console, such as `LogManager.getLogger(ModInfo.ID)` - this will note which thread (server or client) called the log. There are more, but those are the main ones, with #3 being the most common. Note that many methods are called on both sides, which is fine. The proxy system helps to segregate code in a fashion - there's a good write-up on it somewhere around these forums; if I find it later, I'll post a link, but suffice it to say that the main use is in the ClientProxy isolating client-side only calls (Render registrations, KeyBindings, and the like) from the rest of the code so they don't crash your server. Unless an Event class has the @SideOnly(Side.CLIENT) annotation, you should NOT register it in the ClientProxy, but instead somewhere that is common to both the server and the client. This is because the code needs to run on the server, but you are preventing* that from happening. So what you are seeing is the output from YOUR client's event handler on YOUR client every time an item is picked up, but really you want to see the output on the server*. * Since you are running on LAN, however, I'm pretty sure that it still counts as single-player as far as using the integrated server instead of dedicated server, and the integrated server, being part of the client .jar, still makes calls to the ClientProxy (anyone feel free to correct me if I'm wrong, but that's what I've surmised from various tests over my time modding). That being the case, the server event is firing, but the server is running in the client host's game instance, and Minecraft.getMinecraft().thePlayer in that context will always be the host (usually it would crash, but single-player is 'special'). Anyway, that's my best crack at an explanation. Hopefully all my premises are correct
-
Pretty much, yeah, but you shouldn't send the packets to everyone all the time - only players that are currently tracking each other need to know about this stuff, so you can get by using the EntityTracker#sendToAllTrackingEntity method. Get the EntityTracker instance for the player from WorldServer.
-
Why don't you just retrieve the inventory from the client player's IEEP when rendering, instead of the convoluted and unnecessary ClientProxy static Map business? Having that map in the ClientProxy means that each player really has their own instance of the Map, despite you marking it as static - each client is a separate application that can only share information with the server via packets, which shares that information with other clients. Static client data doesn't behave as one might expect. As long as you send each player's IEEP data to any other players that begin tracking him/her, those other players will have the information they need to fetch that player's inventory from IEEP when rendering. As far as the positioning of the items, I'm not really sure what's going on with that. Maybe it will sort itself out after cleaning up. EDIT: Also, you should register your packets in a common area of the code, they don't need to be (and shouldn't be) segregated. Don't mark your message handler as @SideOnly anything, either. That is pointless and harmful. Furthermore, packets that set important data, such as your sync packet, should only ever be sent from the server to the client, never the other way around. I suggest you remove all the code in that packet class / registration that has to do with sending to the server.
-
[1.7.10] Help with status effects and items!
coolAlias replied to Dr_Derpy's topic in Modder Support
Sorry to say, but you need to brush up on your Java skills. That code is a mess.