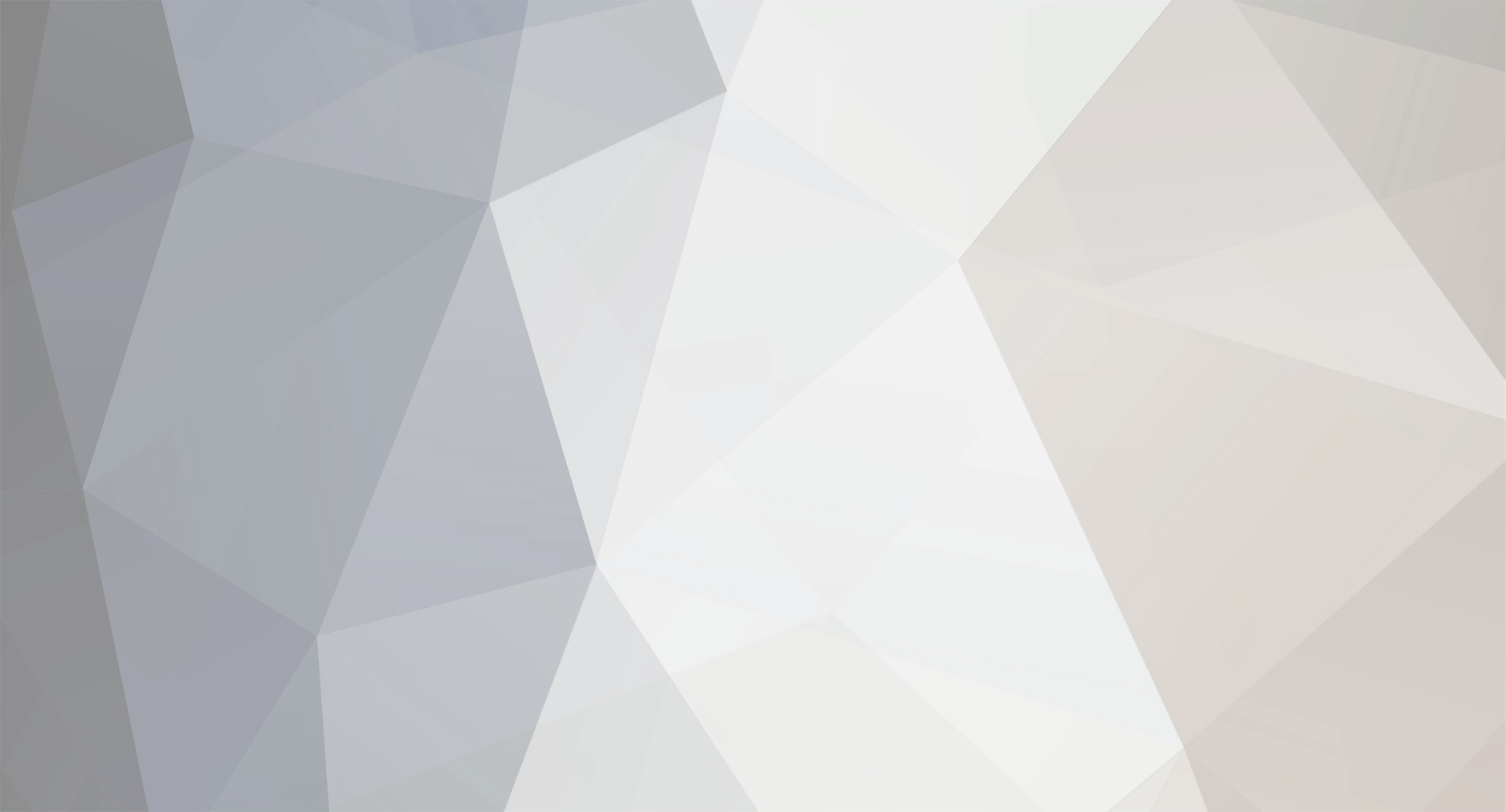
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
How is using Minecraft.getMinecraft() not crashing your server? Or are you not running it on a server? In your event handling class, you have: protected Minecraft mc = Minecraft.getMinecraft(); That WILL crash any server your mod runs on, because that is a CLIENT side only class; Item pickup event happens on the server for sure, I don't recall if it is also called on the client, but you cannot use client-side only classes in that context. Similarly, you cannot register your KeyBindings or KeyInputHandler or any other CLIENT-based code in a common context such as preInit - those should be handled via your proxy, specifically in your ClientProxy. It looks like you did that correctly, but then you are only registering your pickup event on the client side, when it should be registered on the server (or both sides, but really you only care about it on the server). If you want to store a separate list for each player, then do so. A simple way is to use a Map<EntityPlayer, Set<Item>> - a Set because it can only contain one of each, whereas a List could have 20+ of the same Item. Depends on your goal. A better way, of course, is to store the collection of unwanted items in each player's IExtendedEntityProperties - this way the unwanted items can be saved, loaded, etc., and are cleanly managed on a per-player basis.
-
[1.7.10] Help with status effects and items!
coolAlias replied to Dr_Derpy's topic in Modder Support
You can't 'give the item back' because you didn't take it away - all you did in your code was damage the stack by 1. Shouldn't you be reducing the stack size by 1 instead? If you take a look at some vanilla Item classes, such as ItemPotion or ItemFood, you will get a better idea of how to structure your method. For example, you should check if the player's current health is less than their max health before using and consuming the item, and don't consume it if the player is in Creative mode. In pseudo-code: #onItemRightClick { if (player.getCurrentHealth() < player.getMaxHealth()) { // we know for sure the item is going to be used now, but why restrict it? don't you want it to damage players if they take too many? // how will they take too many if they can't keep popping pills once they reach full health? if (player is NOT in creative mode) { --stack.stackSize; } if (the potion effect is already active on the player) { damage the player; } else { add the potion effect; heal the player; } } return stack; } For the language file, when you are in game, what does the name come up as? It should be something like 'potion.effect.name' - just take whatever that string is and put it in your en_US.lang file with a translation: potion.effect.name=My Potion Effect -
[1.7.10] Failure to Render for Other Players
coolAlias replied to HalestormXV's topic in Modder Support
That would be true if that was the method used to spawn the entity, but it is not - the entity is spawned from an Item using #onItemUse, with the whole block of code nested in if (!world.isRemote). The entity's #interact code that is responsible for taming is also only run on the server (due to nesting within if (!world.isRemote), not because the method is only called on the server [it's not]). You can see the code a few posts back. But you raise a good point about DataWatcher... the owner's UUID should be available to all clients, so calling #getOwner should, in theory, return the same value for everyone... hell, I'm stumped. There's got to be some other interaction going on somewhere that is clearing the owner for other players, or something... -
[1.7.10] Help with status effects and items!
coolAlias replied to Dr_Derpy's topic in Modder Support
The 2nd parameter of PotionEffect is duration, in ticks; 20 ticks is approximately 1 second, so 5 ticks is 1/4 second - not very long. The reason you take damage every time is that you apply your Ibp PotionEffect to the player first, and then check if it is active. Well, you just applied it, so it is going to be active very single time. Just swap the bits of code around and you'll be fine: check if active first to cause damage, THEN add the potion effect. Also, I don't see what the point of the prevHealth check is - #heal will not surpass the player's max health no matter what value you put in there, and 20 is usually the max health. What are you trying to accomplish with that bit of code? -
[1.7.10] Failure to Render for Other Players
coolAlias replied to HalestormXV's topic in Modder Support
You're absolutely right on, but not If the server side version is killed, however, the client side version is as well, so that's not the problem. The problem is (very probably) that the CLIENT-side version doesn't know about the owner, and is setting the entity to dead only on the client side. To solve that, in your #onUpdate check only set the entity to dead on the server side (i.e. when the world is NOT remote), which is usually how you should do it anyway. That would explain the entity 'disappearing' on clients while still functioning on the server, but the mystery remains why it would still render for the player that summoned it. Very strange. Anyway, let us know if that fixes it. Btw, you should not use global entity IDs or the vanilla spawn egg system when registering your entity, only EntityRegistry#registerModEntity. If you need a spawn egg, you should implement it yourself or, if you can update to 1.8, take advantage of Forge's implementation. -
[1.8] Spawning entity with item creates a "still" entity
coolAlias replied to plr123's topic in Modder Support
If you look at any of the vanilla entity spawning code, you will see that you need to call #setLocationAndAngles on the entity BEFORE you spawn it into the world, along with as much other relevant information as you can. The reason being that when you call #spawnEntityInWorld on the server, it sends a spawn packet to the client containing the entity's current information, including location - if that information is lacking, the default values are used. In your case, the entity, lacking any knowledge of its own coordinates at the time it joins the world, spawns on the client at (0, 0, 0), likely very far from where it spawned on the server and nowhere near where you will be able to see it. EDIT: And the 'still' version was, of course, because you spawned it on the client only, which doesn't run any AI logic - basically, it was a ghost, not a real entity. -
A small point, but worth mentioning: the output of Techne is not special - it's just standard model code/.JSON, i.e. a format that can be understood by Minecraft's rendering classes. Besides, many modders (such as myself) will take whatever Techne outputs and completely change it (to clean it up and/or add parent:child relationships, for example), add animations, or any number of other things that one might also find in vanilla Minecraft models. So... you literally have dozens of models at your fingertips already in the Minecraft code. What better test subjects than that?
-
Weird - I had a 64x32 texture for a custom entity using the vanilla ModelBiped and it was all sorts of f-ed up looking, but as soon as I resized it to 64x64 (just empty space for the bottom 32 pixels), it mapped perfectly to the model.
-
Ah yes, 1.8 - I had the same problem months ago when I updated and, after fixing it in a similar fashion to the above, totally forgot about it I've had the same experience - I reported one in 1.7.2 along with the fix for it, just a simple change x to y in the coordinates passed (they were passing x, x, z to getExplosionResistance). SIX months later, they close it saying it is no longer relevant because Blocks changed so much in 1.8. But in that time, they had 8 or so versions of 1.7 in which it could have been fixed -.- Anyway, I no longer report bugs to them...
-
Make sure your texture is exactly the same size as the vanilla texture (64x64, I believe), even if you don't use the same space. If it's 64x32 or some other size, things can get really funky like you are seeing, so that may be your issue.
-
Don't call #setHardness each time - there is only one instance of your Block, so each time you call that method EVERY one of your blocks in the world will change its hardness. Instead, just return the value you want based on the meta, e.g. case 0: return 3.0F; As for the crash, where is the crash report?
-
[solved][1.7.10]custom mob not attacking other mobs
coolAlias replied to [email protected]'s topic in Modder Support
In that case, you may have to override #onLivingUpdate or one of the other update methods and do a little hack like vanilla does to search for the nearest player, but instead search for the nearest of your entity and set it as the current target. I don't see anything else in your code that would prevent them from attacking each other, so perhaps it's just that the targeting AI isn't as, um, performant as one would expect -
Could be your texture is not laid out in the same format as your model expects. Does your helmet use the same model as the vanilla helmet, or is it custom? Because that's pretty weird if it renders the regular helmet texture perfectly fine, but it's a different model. Does your entity renderer extend RenderBiped? And is your mob actually wearing a helmet, as in the helmet inventory slot has a helmet item in it? Because RenderBiped will render any equipped helm automatically, though I don't recall whether how/where it fetches the model. You should take a look at that class and see, if that's the case.
-
Do you know what a List is? It's not any kind of Entity, that's for sure. I suggest you spend some time learning about Java Collections and how to use them - it will be time VERY well spent
-
You know how in your Model class there are a bunch of ModelBase fields? Each of those is a part of your model. When your model renders, you call part1.render(args), part2.render(args), etc. for every part that is not a child of another part. However, if your helmet has lots of individual parts, even something as simple as head rotation will cause it to become mangled beyond recognition if each part is not the child of a previous part of the helmet. You just need one parent part on the helmet, and every other part will be relative to that piece; when that piece renders, you can set the rotation to the head rotation and the rest of the pieces will follow.
-
That's an issue with your model rotation points and values - if you made your model in Techne, Techne doesn't support child parts, so adding all of the parts as children of the head makes everything out of whack like you are seeing. Easiest solution is to not add them as children, but just render each part separately. If you have somewhat complicated animations, however, you'll want to instead add any parts involved in that animation as children of the appropriate part, and then recalculate the rotations points/values based on the parent part (basically you just subtract the parent values from the child values to get the new child values).
-
Possible, yes, but to get every tamed entity... it's not going to be pretty. Basically, you need a way to know which entities to fetch, but there isn't any 'getAllTamedEntities' method. The way I see it, you have 2 options: 1. Use World#getLoadedEntityList and iterate through each entry checking if a. is it an EntityTameable, and b. does it belong to the player; teleport them as they are found. 2. Store a list of every entity the player tames in IExtendedEntityProperties; you'll have to write code to find out when an entity is being tamed, add it to the list, and then, since the player may log out and back in, be able to find the entities from their unique ID (which is what you should store). Both of those have a problem: if the entity is in a chunk that is not currently loaded, it will not be found. The first method, there is no way around this; the second method, it's possible, but starts to get clunky - you'd have to track the last known location of each entity, then you could try to force load that chunk (and possibly nearby chunks) if not loaded and see if the entity is still there. Even then, there is no guarantee, as the entity may have been killed, or it may have wandered / been lead off while the owner was away, and there is not really any way for you to know this. Another option, then, would be WorldSavedData with a server-tick handler that updates each tamed entities' last position, but as you can see, things have gotten far more complicated than one would like. So, you can easily teleport MOST of the player's tamed entities, but to guarantee ALL of them, you've got a lot of work ahead of you.
-
[solved][1.7.10]custom mob not attacking other mobs
coolAlias replied to [email protected]'s topic in Modder Support
#canAttackClass is called from EntityAITarget when determining if a target is suitable... if he uses your code as written, it will guarantee they don't attack each other, which is the exact opposite of what he wants. There is no reason to override the default implementation in the majority of cases. @OP I'm not sure why your mobs aren't attacking each other, but maybe they are and you just can't tell. To know for sure, override #attackEntityAsMob in each of your entity classes and put a System.out.println("Attacking " + entity.getClass().getSimpleName()) to find out a. if they are ever attacking anything and b. what they are attacking. You should still be able to get them to attack you as a player by hitting them first, so if you're not seeing any console output, try hitting one. -
Or, you could update to 1.8 and then it's simply a matter of changing the scale in the Item's .JSON model... can't get much easier than that
-
Is it YOUR block, a vanilla block, any block? If it's your block, you can override the onBlockClicked method (or whatever it's called) and you'll have the coordinates there; if it can by any block, but your Item, you can do what you did above and use onItemUse. Either way, that will give you the Block's position. How are you going to find the entity to teleport? Do you have some kind of system to track the entity, e.g. if the entity's unique ID is stored in the ItemStack NBT, the Block's TileEntity, or even the player's IEEP? Without such a system, it will be impossible to know which entity to look for and teleport to the block position. If you describe how your item/block/entity teleportation should work, not in code but from the player's perspective in game, then I could give you better advice.
-
[1.7.10]custom entitythrowable passes through entity
coolAlias replied to [email protected]'s topic in Modder Support
When isRemote is true, that means your code is running on the CLIENT side - you want the SERVER side for causing damage and such, so you should use !this.worldObj.isRemote instead. Also, you need to make sure you spawned the throwable entity correctly, i.e. on the server side. -
[1.7.10] Tessellator Textures Not Rendering
coolAlias replied to yoshiquest's topic in Modder Support
The first example renders a completely 3D block, all 6 faces. The second example showed rendering 2/6 faces of a Block and no, that will not work for anything other than a Block, a cube-shaped one at that. Techne models typically use an actual ModelBase object on which one calls model#renderAll, with the model itself handling all of the vertex rendering. Items, Blocks, and Entities are all rendered separately by Minecraft and have different methods of rendering; taking that and making a library that is general enough to handle any of them + models + animations (how do you plan on handling that, btw?) all with the same methods is going to be quite the feat. I'm not saying it's impossible, but chances are you are going to have to provide at least 3 different rendering methods, one for each of Item, Block, and Entity, as each of them are very different from each other. Blocks and Entities are especially complex; again I urge you to look through the entire RenderBlocks class as well as each of the Render{Entity} classes, and even the ItemRenderer class is no walk in the park - you can see for yourself the beast you are waking. Best of luck to you - you're going to need it -
[SOLVED] [1.8] Reducing Network Traffic
coolAlias replied to EverythingGames's topic in Modder Support
Because the server side has no concept of the Mouse or what state it is in. For the 'packetSent' variable, I might prefer to call it 'mouseClicked' or something, since it is tracking when the state of the mouse changes, but that's just a matter of how you look at it (not a big deal, obviously). Also, it would be wise to have the same logic on the server, so each time the packet is sent with the current mouse state, the server can validate it before processing anything else, this way if somehow the packet gets sent a bunch of times in a row with 'mouseClicked = true', it doesn't result in extra actions. As for clean code - that's a good thing to strive for, and if you look again at vanilla, you'll notice that, in the example I gave earlier, the packet simply calls the same code that the client called, namely EntityPlayer#attackTargetEntityWithCurrentItem, with some extra validation server side, of course. This way all of the logic is contained in that one method, and either side can call it as need be, so there is no code duplication. -
[1.7.10] Failure to Render for Other Players
coolAlias replied to HalestormXV's topic in Modder Support
Hm, all looks okay. How about your entity registration code? If it's working on single-player, I doubt that's the problem, but I'm running out of ideas :\ -
[solved][1.7.10]custom mob not attacking other mobs
coolAlias replied to [email protected]'s topic in Modder Support
1.7.10 mobs are somewhat hard-coded to look for players, as they all extend EntityCreature which every tick during #updateEntityActionState will target the nearest player if it's not already attacking something. An easy solution is to override #findPlayerToAttack in your custom mob classes to always return null.