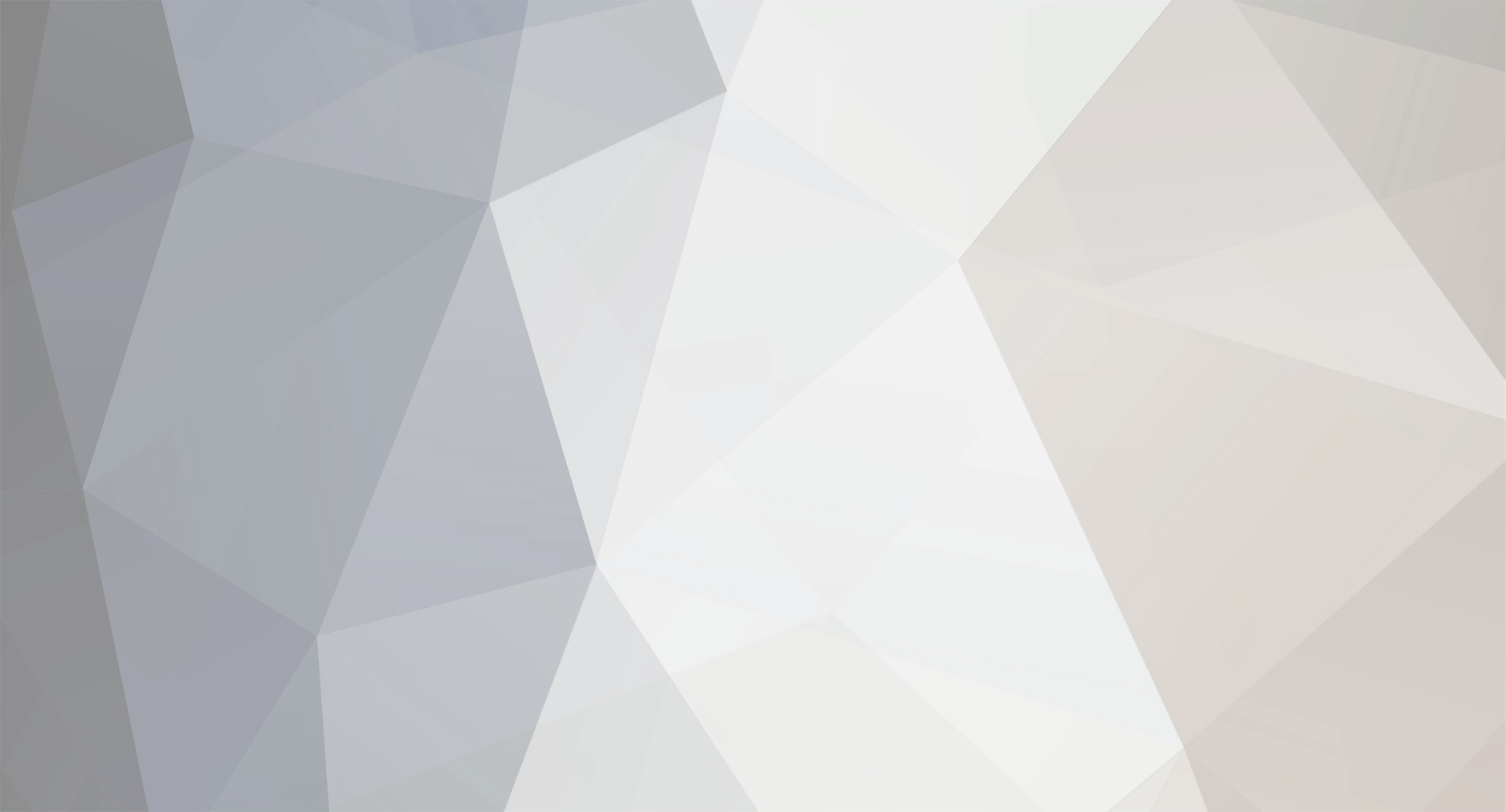
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Again: learn Java - your problem is no longer with Minecraft, it is with your own lack of knowledge. You can do whatever you want in your own constructor so long as you have everything there that the super constructor needs. You can't pass extra arguments to the super constructor, but you can have as many as you want in your own. Basic Java (sorry if it seems like I'm harping on you, but it really is very basic and you owe it to yourself to learn it).
-
This is pretty basic Java: public class YourRenderClass extends RenderWhatever { private final ModelBase[] models; public YourRenderClass(ModelBase ... models) { this.models = models; // now you have an array of models to choose from } // Or, if you don't like arrays: private final ModelBase model1, model2, ... modelN; public YourRenderClass(ModelBase model1, ModelBase model2, ... ModelBase modelN) { this.model1 = model1; // etc. } public void doRender(Entity entity, ... other args) { int modelIndex = ((YourEntity) entity).getCurrentEvolutionStage(); // this value needs to be known on the client as well as server // now you know which model to use this.mainModel = models[i]; // or make a switch if you went with option 2 } } That will work for any of your sub-entities that follow the same logic. You probably want to brush up on Object-Oriented programming if the above doesn't make sense to you. EDIT in reply to your last reply: if you are having troubles with such simple issues as how to make a boolean method, you need to take the time to properly learn Java or any other OO language. That's just a fact of programming life that you're going to have to come to grips with.
-
Is that so? I haven't actually checked to see if they get called or not, but only the 'Specials' versions of it are marked as Deprecated... I wish they would just completely remove things that no longer work, rather than leaving them in there (like IItemRenderer) causing people to think they might still function, deprecated or not. Note that 'deprecated' does not mean "No longer functions", it means "Use the newer version instead, as this one sucks and / or might be removed in the future".
-
You can change entire models within your render class just as easily as you can skins. All you do is create a reference to each model when you construct the render class, then in doRender, use the entity parameter to figure out which evolved state it is in and choose the model accordingly (this.mainModel = differentModel or something like that).
-
Do you actually need to have all of the models together, or can you just have your entity renderer switch models as needed? Combining models / rendering a transition between models is far more complicated than simply switching models, and I would only attempt it if it was really important for your entity to appear to transform, vs. if the entity just has 3 different evolution forms and the player doesn't really care about the transformation, just its current form. Even if you are going to create a transition, you don't need the models to be combined into one - see IChun's Morph mod if you are really interested in working on such a transformation.
-
[1.8] Editing day lenght - can it be unsafe? (+question)
coolAlias replied to Ernio's topic in Modder Support
I feel that way every time I play (I mean playtest my mod) Minecraft - it seems like I barely do anything and it's f-ing night time again. For some reason I thought (hoped!) they had added game rules for the day/night cycle length, in which case it would be trivial for modders to do the same... -
Ha, so they are, even though they ultimately get converted to floats. Never mind then. Well, I guess your only option then is to make your model as big as you need it to be to get the legs with the correct proportions, and then scale the model back down when you render it.
-
That's odd that Eclipse complains about it for you, because addBox takes floats: shape1 = new ModelRenderer(this, 0, 0); shape1.addBox(-3.5F, -1.25F, -4.75F, 8, 8, ; ^^^ Eclipse doesn't complain at all if I do something like that.
-
[1.8] Editing day lenght - can it be unsafe? (+question)
coolAlias replied to Ernio's topic in Modder Support
Ernio, I don't think the world time has any bearing at all on ticks. I.e. you can set world time to any value you want, even every tick set it to zero, and the world will keep ticking merrily along. I guess that's part of the problem - ticks don't care about world time, so if I have a solar panel building power by the tick, it will give me 3x the power under your system, whereas if I have another block that is usable once per hour and that uses the current time to determine if an hour has passed, if you followed the previous poster's advice that block would still be usable once per hour, but each hour would be 3x as long. -
Don't limit yourself to Techne - you've got all that Java that you can do whatever the heck you want with, or you can search for another program that lets you do decimals, or you can scale your entire model by however much you need to get the legs looking right, and then glScale the entire thing to the right size within your render class.
-
Then you'll just have to make them the right size to begin with, rather than trying to scale them dynamically.
-
Simple: call 'GL scale' before you render the legs. render stuff render stuff render stuff push scale render legs pop Or just make your legs the right size to begin with.
-
I can't think of any reason why EntityTameable specifically would cause the entity to glitch like that, which leads me to suspect you changed more than just the parent class. You will need to provide us with some EXACT details: set up your code so it works correctly (no falling through ground) and post that. Then change ONLY the parent class to EntityTameable, NOTHING else, and check if that really does cause the issue.
-
If you want your model to move smoothly, you should be converting pieces into children anyway - all you need to do is subtract the offset points up the chain to get it back into the correct place, then animations (i.e. rotating pieces) becomes far simpler. Rotating, btw, is what I would suggest rather than changing the offset, at least if what you are going for is a 'mouth opening' type of effect. Then you just say 'lowerJaw.rotationZ = 30F' or whatever and it should look pretty good if you have the rotation point in the right spot.
-
If you ever have a model piece moving in more direction than one from just changing one coordinate, it's because you have rotated that piece somehow so it is no longer aligned with the x/y/z axes. For example, if the jaw piece is rotate 45 degrees along the x axis so that it is now pointing between x and z, and you move its x coordinate, it will actually move half that distance along x, and the other half along z. There is not much to be done for it other than play with the numbers until you get it right, or sit down and do some serious math (if you know how - I don't). Perhaps others may have better suggestions, but I really think you're going to have a lot of trial and error on your hand here. Using Techne or some other modeling program can usually help you visualize what's going on, but, as you've discovered, it's not always one-to-one correspondence.
-
@Ernio He wants to change the way melons blocks behave, which is not necessarily only when placed by players, but when they grow from stems. @OP I'm not sure if this will work, but you could try BlockEvent.NeighborNotifyEvent - it fires whenever a block notifies its neighbors that it has changed, and may fire for stems when they set the melon block, or perhaps for the melon block when it is first set in the world. I'll leave digging around in the code / testing it up to you, but that's where I'd start.
-
How to make a ranged Mob? 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
Come on Boonie, READ the vanilla code! Attack with ranged is only ever called if you add the AI to do so, like I mentioned earlier Skeletons do this in setCombatTask(), but you will just move that to your constructor. -
[1.7.10] how to give player certain items?
coolAlias replied to Waabbuffet's topic in Modder Support
For some items, such as heads, you need to set NBT data. Checking the Minecraft gamepedia site is often the fastest way to find out exactly what you need, or you can pore through the code (I usually do the latter). -
How to make a ranged Mob? 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
You have to add the index (21) to data watcher before you can use it, otherwise it doesn't exist: @Override protected void entityInit() { super.entityInit(); dataWatcher.addObject(21, (byte) 0); } -
How to make a ranged Mob? 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
If you looked at Skeleton, surely you saw that it implements IRangedAttackMob and is given an EntityAIArrowAttack AI while holding a bow (added via #setCombatTask) That's pretty much all you should need, as the rest is effectively copy / paste / change the entity spawned in the ranged attack method. -
Modifying mouse clicks on the server thread
coolAlias replied to SnowyEgret's topic in Modder Support
One other thing to point out: I'm not sure how you are storing your 'key map', but you cannot reference KeyBindings on the server - the code will compile, sure, and may even work fine in single player, but it will most assuredly crash in multiplayer. This is part of the reason that vanilla does not try to reference keys, but rather state, e.g. isSneaking, isJumping, etc. all are actual fields that get toggled on or off based (mostly) on packets sent from the client. Depending on what you are trying to accomplish, you may want to follow a similar line of thought rather than trying to track the keys themselves, at least on the server. -
To clarify, this ONLY happens when you extend EntityTameable, and NOT when you extend any other Entity class?
-
I've got an item that I want to have a base enchantment that is just its 'normal' state, so I don't want the enchanted glow to render. Overriding Item#hasEffect(ItemStack) works great for the item (a helmet, btw) everywhere EXCEPT when it is worn, which I have traced down to this bizarre discrepancy: The easily-customizable Forge method Item#hasEffect(ItemStack) which is called by ItemStack#hasEffect is used everywhere except for LayorArmorBase, which instead calls the impossible-to-modify ItemStack#isItemEnchanted. Was this just missed, or is it intentional?