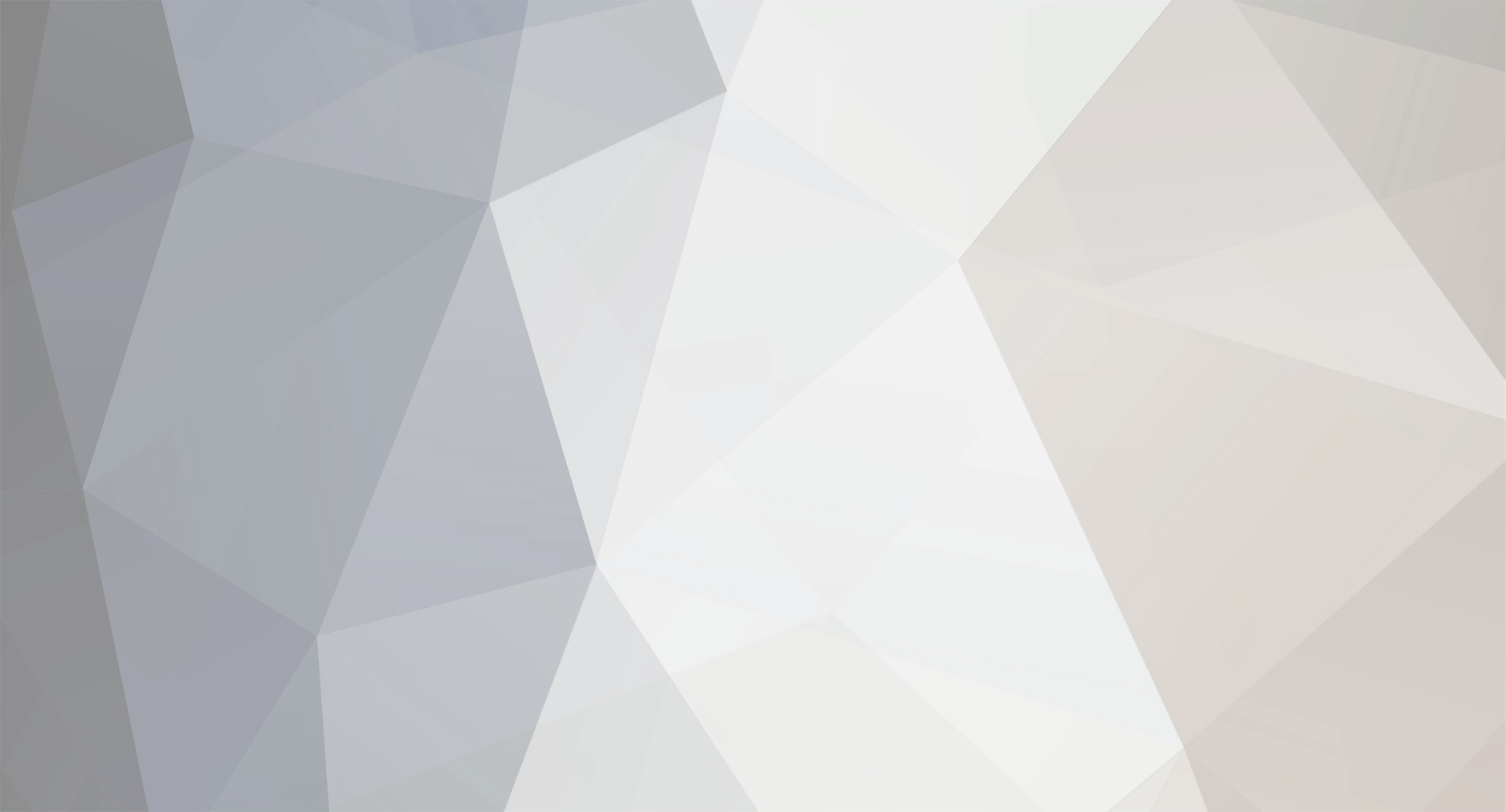
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.8] [SOLVED] Spawning Particle Trail Along a Vector
coolAlias replied to Himself12794's topic in Modder Support
If you haven't already, it is very helpful to look through the vanilla examples e.g. the critical particles trailing arrows. Gaussian values are also very nice for certain types of particles, but in this case I'd stick with rand.nextFloat() as an addition to each position value. For example: for (int i = 0; i < 4; ++i) { world.spawnParticle(EnumParticleTypes.FLAME, x + 0.5F - world.rand.nextFloat(), y + 0.5F - world.rand.nextFloat(), z + 0.5F - world.rand.nextFloat(), 0, 0, 0)); } You can place whatever you want in the motion values; the more random, the better (usually). In the example above, I get x, y, and z by iterating along the player's look vector, so that whole section of code is actually in a loop that iterates as far along that vector as I want to go. Each type of particle also has its own motion behavior, so an algorithm that works well for one particle type may not produce the same results with another. -
[SOLVED][1.8] Solid to invisible toggleable block rendering
coolAlias replied to coolAlias's topic in Modder Support
Already return null for those, same. However, TGG to the rescue as always with rendering-related questions. Solution: Create fully transparent 16x16 texture for the invisible state and change the block's render layer to CUTOUT_MIPPED. Works perfectly EXCEPT for when inside the block, where everything renders completely black. Overriding isFullCube, isOpaqueCube, and isNormalCube to all return false fixed that, in combination of course with setting the block bounds all to 0 when invisible. I'm going to experiment some more and see which, if any, of those three cube methods I can safely leave with the default value and still get the desired effect. -
[SOLVED][1.8] Solid to invisible toggleable block rendering
coolAlias replied to coolAlias's topic in Modder Support
That was my next step: seeing about creating a completely empty texture (all alpha transparency) and seeing if that would work. The problem with the ISmartBlockModel is it still requires a model to render; air does not have a model (I tried and it gives you the infamous missing model texture) as well as air is render type -1 which does not look for a model at all in the render code but instead returns false. ISmartBlockModel does not allow one to return false before anything even tries to render; I suppose that would be another alternative - change ISmartBlockModel to query whether to render a model or just return false without doing anything else. -
And when do you call registerEntitySpawns? I don't see you call that method from anywhere. It needs to be after all entities are registered, and preferably during FMLPostInitializationEvent in case you want to use any modded biomes or anything. That's where I add mob spawns, and that works for me.
-
[SOLVED][1.8] Solid to invisible toggleable block rendering
coolAlias replied to coolAlias's topic in Modder Support
If you had read my post more carefully, you'd see I already override isOpaqueCube, and isCollidable, per the Java-doc, is 'only used by Fire'. I do override canCollideCheck based on state, but my problem is not being able to walk through the block or not, it is getting the block not to render at all when it is ethereal. As it is, it always renders like it is a solid block, even when it should be invisible. The ISmart/IFlexible block model interfaces do not allow for cancelling the rendering altogether, which is what I need it to do. Maybe if I try it in the cut-out layer... EDIT: Lol, well that sorta worked - the blocks were invisible, even the solid ones! However, as soon as I entered into the block's space, even though its bounding box is supposedly all zero, the inside of the block renders and blocks out the screen. Hm. -
I'm trying to update a block to 1.8 that has a toggleable state between solid and ethereal / invisible, but I'm having some trouble with the rendering (or rather, NOT rendering). In 1.7, returning 'false' for isOpaqueCube and isNormalCube and then setting the block bounds all to zero was enough to get the block to 'disappear'; this no longer works. I tried using an ISmartBlockModel to return the default model for air which, of course, there isn't one. What I (think I) really need is to be able to dynamically return the renderType based on the block state, e.g. // Inject this method into Block class: public int getRenderType(IBlockState state) { return getRenderType(); // and deprecate this mo-fo } Then I could return -1 (do not render) or 3 (render as solid block) based on state. Barring that, does anyone have any suggestions? I've been banging around trying all sorts of things trying to get it working, to no avail. EDIT: Also, is it just me or is the block state sometimes not up to date on the client side? When first joining the world, I am occasionally able to walk through one of these blocks as though it were ethereal when it's not (and default state is not), but after a few seconds it figures out it's supposed to be solid. Are there just general clipping issues sometimes?
-
Why ask when you can simply try it in your development environment? Then you can find out immediately whether it works or not. Btw, that last argument to setBlockState is a bit flag that tells Minecraft whether or not to update the client side / notify neighboring blocks / other things, and you almost always want it set to either 2 (notify client) or 3 (notify client AND notify neighbors, IIRC).
-
Adding directly to the EntityList like that is still not a good idea - you have no idea whether the ID you chose is unique or not, and if you did still use findUniqueGlobalId but didn't register it to your entity, then it's pointless. You need to implement your own custom EntityList / spawn egg / summon command. I've brought this up before, but the Forge team is apparently not keen on making it easier to use, and that's just the way it is. I've posted links to my own code as an example somewhere around here; you can probably find it if you search. To make your entity spawn in the world, you need to add the spawn information to the EntityRegistry for each biome that you want it to spawn in: EntityRegistry.addSpawn(entity.class, spawnRate, min, max, creatureType, biome);
-
Or, if you don't want to convert all of those lines to 'new BlockPos(i+x, j+y, k+z)', just make a wrapper method and use your editor's replace function to replace all of the 'world.setBlock(...)' to 'MyHelper.setBlock(world, ', e.g.: public static void setBlock(World world, int x, int y, int z, Block block, int meta) { world.setBlockState(new BlockPos(x, y, z), block.getStateFromMeta(meta), 3); } Something like that anyway, coding from memory
-
[1.7.10][SOLVED] Get Respawn Location (Bed)
coolAlias replied to Izzy Axel's topic in Modder Support
ChunkCoordinates cc = player.getBedLocation(player.dimension); if (cc != null) { cc = EntityPlayer.verifyRespawnCoordinates(player.worldObj, cc, player.isSpawnForced(player.dimension)); } if (cc == null) { cc = player.worldObj.getSpawnPoint(); } That is the meat of the code adapted from ServerConfigurationManager#respawnPlayer. -
[1.8] Catch when player swaps item to some Item - smooth.
coolAlias replied to Ernio's topic in Modder Support
You certainly can, sort of - I do this while one of the sword skills is active to prevent changing the current item. MouseEvent has a field called 'dwheel' which indicates whether the mouse wheel is in use (+/- for up/down scrolling, zero for no input). You will also need to check the number keys using KeyInputEvent for direct changes. I haven't tried it within a GUI screen, but as long as both of those events are fired, you could probably rig up something. Checking if the open GUI is a GuiContainer, for example, and then casting would probably allow you to figure out what the current slot clicked / item is. Honestly, though, and contrary to what I usually try to promote, I think the ticking solutions are the better solution here. There are just so many ways that an item can get into a player's hotbar slot (picking up, shift-click, manually via direct inventory manipulation in code, etc.), that you can't possibly cover them all unless you actually check the slots involved and what they currently contain. -
[1.7.10] How to detect if an entity is moving?
coolAlias replied to TheRealMcrafter's topic in Modder Support
Entity.motionX/Y/Z should work fine. Are you checking on the server, the client, or both? How are you getting the player? Show some code. -
Most impressive, as always I'd say 'submit this as a bug to Mojang', but that's a lost cause. Last time I submitted a bug with an equally simple fix, it was for 1.7.2, and they dallied around the issue for 6 months and then "Oh, well it's no longer an issue because 1.8 is out now and doesn't suffer from it". Yeah, but you could have f-ing fixed it in 30 seconds 6 months ago, and then neither 1.7.2 or 1.7.10 would suffer from it either!!! Argh. Anyway, I wrote a simple helper method to use in the meantime, nothing fancy: /** * Returns a list of all entities within the given bounding box that match the class or interface provided */ @SuppressWarnings("unchecked") public static <T> List<T> getEntitiesWithinAABB(World world, Class<T> clazz, AxisAlignedBB aabb) { List<Entity> entities = world.getEntitiesWithinAABB(Entity.class, aabb); List<T> found = Lists.newArrayList(); Iterator<Entity> iterator = entities.iterator(); while (iterator.hasNext()) { Entity e = iterator.next(); if (clazz.isAssignableFrom(e.getClass())) { found.add((T) e); } } return found; } As you can see, I just piggy-backed on the vanilla method, since it's so fast and furious, to get a base list of Entities, then cherry-pick any that match the interface (or any class) out of that. It could probably be optimized better, but I didn't want to waste any more time when there is still so much of my mod that needs updating.
-
Yeah, I could see that's what it was doing, but I couldn't believe it so I had to ask. I mean, I suppose they are saving some CPU cycles, but wtf. Surely there are other areas that would improve performance more than this. Is there any way to add an interface to the mappings? Does that need to be done on a chunk-by-chunk basis as entities of that type enter the chunk, or can it be added once and be done with it? Worst case scenario is to simply write a custom method specifically for interfaces that uses standard Java instanceof comparisons on all entities found within the given area.
-
Why, Mojang, would you add something like this, when Java already knows how to test for class inheritance? // Searching for entities that implement a given interface: List entities = world.getEntitiesWithinAABB(SomeInterface.class, player.getEntityBoundingBox().expand(radius, (double) radius / 2.0D, radius)); // Oh joy... Caused by: java.lang.IllegalArgumentException: Don't know how to search for interface test.entity.SomeInterface at net.minecraft.util.ClassInheritanceMultiMap.func_180212_a(ClassInheritanceMultiMap.java:53) ~[ClassInheritanceMultiMap.class:?] at net.minecraft.util.ClassInheritanceMultiMap$1.iterator(ClassInheritanceMultiMap.java:111) ~[ClassInheritanceMultiMap$1.class:?] at net.minecraft.world.chunk.Chunk.getEntitiesOfTypeWithinAAAB(Chunk.java:1103) ~[Chunk.class:?] at net.minecraft.world.World.getEntitiesWithinAABB(World.java:3289) ~[World.class:?] at net.minecraft.world.World.getEntitiesWithinAABB(World.java:3272) ~[World.class:?] This kind of code worked perfectly fine before. I'm baffled about how to use the ClassInheritanceMultiMap class; it has a public 'add(Class)' method that is never called from anywhere, and no instance of the class is ever instantiated except for within the Chunk class, and that seems to only be used to contain a list of entity classes currently in the chunk. The method func_180212_a relies on Apache commons ClassUtils to return the class hierarchy; do we now have to register all of our custom classes / interfaces somehow in order for them to be recognized? What the hell is going on here?
-
[1.8] MinecraftByExample sample code project
coolAlias replied to TheGreyGhost's topic in Modder Support
TGG, can't thank you enough for all the work you've done with the new model-based rendering code; your block examples (#3-5) especially saved me a TON of time while working on updating one of my mods to 1.8. While the following may be out of the scope of your tutorials, I'd like to share a way to automate the swapping of models during ModelBakeEvent. I also do something similar to automate all of my variant and model mesh registrations. Instructions: Gotta love a little automation-by-Reflection -
[SOLVED][1.7.10] question about preventing entity "despawning"
coolAlias replied to jabelar's topic in Modder Support
You can also call 'func_110163_bv()' in your entity constructor to make it persistent. In 1.8, this is aptly named 'enablePersistence()'. -
[1.8][SOLVED]Custom entity : saving new fields
coolAlias replied to Shuyin76's topic in Modder Support
This is such a shame, since so many mods add entities, and they all have to write their own implementation - I keep hoping one of these days Forge will provide a way to hook into the vanilla spawn egg / summon command. That said, it's not too difficult. I wrote an implementation that involves a custom entity list and a single item to handle all of those. I just really wish that this type of compatibility layer were already available / standard. -
[1.8]Replacement for ItemRenderer.renderItemIn2D?
coolAlias replied to Renkin42's topic in Modder Support
Look at RenderSnowball, as they've told you. You should be able to COPY that, probably verbatim, into whatever render class you are using. It uses RenderItem#renderItemModel(ItemStack) in combination with GLStateManager. If you find the few obfuscated field / function names confusing, look at the type of the variable or return type of the method, if any (e.g. field_177083_e is of type RenderItem, which makes it pretty obvious what it is). Or, even better, since you are just rendering the item anyway, why not just USE RenderSnowball as your render class? Are you doing anything else in there? -
You should ask the author of Version Checker, but I presume NO. All the documentation I saw specified .json format, which basically IS a text file with a special format. Just don't save it as .txt.
-
Version Checker is very simple to use if you have an online repository, e.g. Github: // add this during FMLInitializationEvent: String link = "https://raw.githubusercontent.com/yourName/YourMod/master/src/main/resources/versionlist.json"; FMLInterModComms.sendRuntimeMessage("your_mod_id", "VersionChecker", "addVersionCheck", link); Note that it is very important that you use the 'raw' link to your json version file, otherwise it will not work. The format of the file is described in the Version Checker documentation, and it can contain multiple versions' information (e.g. both the latest 1.7.10 and 1.8 versions of your mod). The versionlist.json does not have to be in the resources folder; that just seemed like a semi-logical place to put it, so I stuck it there. You can put it wherever you want. It's really that simple Of course, you / any users will need to install Version Checker when playing in order for you to view that information in-game. You do not, however, need to have it installed in your development environment, which is pretty sweet.
-
You don't need IItemRenderer if your HookShot is an Entity (which it is, judging from your code). Just render everything, Item included, in the Entity render class, and leave the hand-held portion of the item rendered with the standard Item rendering code. If you have part of the hookshot that needs to be at the end of the chain, you should still be able to manually render a texture icon. Take a look at the fishing hook render code for an example of that.
-
Hm. Well, first step is to debug by printing out the values of the owner name and ID when loaded from NBT. Also, you should be aware that using '==' operator to compare Strings is not generally a good idea, as it compares identity, not content. You should use 'equals' method instead: if (this.dog.getOwnerName().equals(this.player.getName())) { // player viewing GUI has the same name as the owner of the dog } Furthermore, shouldn't you be using player.getDisplayNameString() instead of getName() ? That's the method that returns the nickname, if I'm not mistaken. Though it looks like getName() returns the GameProfile name... Dunno.
-
That looks about right - the problem could be in your GUI class instead of the Entity now. Can you show us your GUI code where you access the owner?
-
If you search for IItemRenderer or 'Minecraft custom item rendering' in Google or something, you are bound to come up with some tutorials on how to use them. You do need to register the renderer for your Item class in the ClientProxy (or at least called from there): MinecraftForgeClient.registerItemRenderer(YourMod.yourItem, new RenderYourItem());