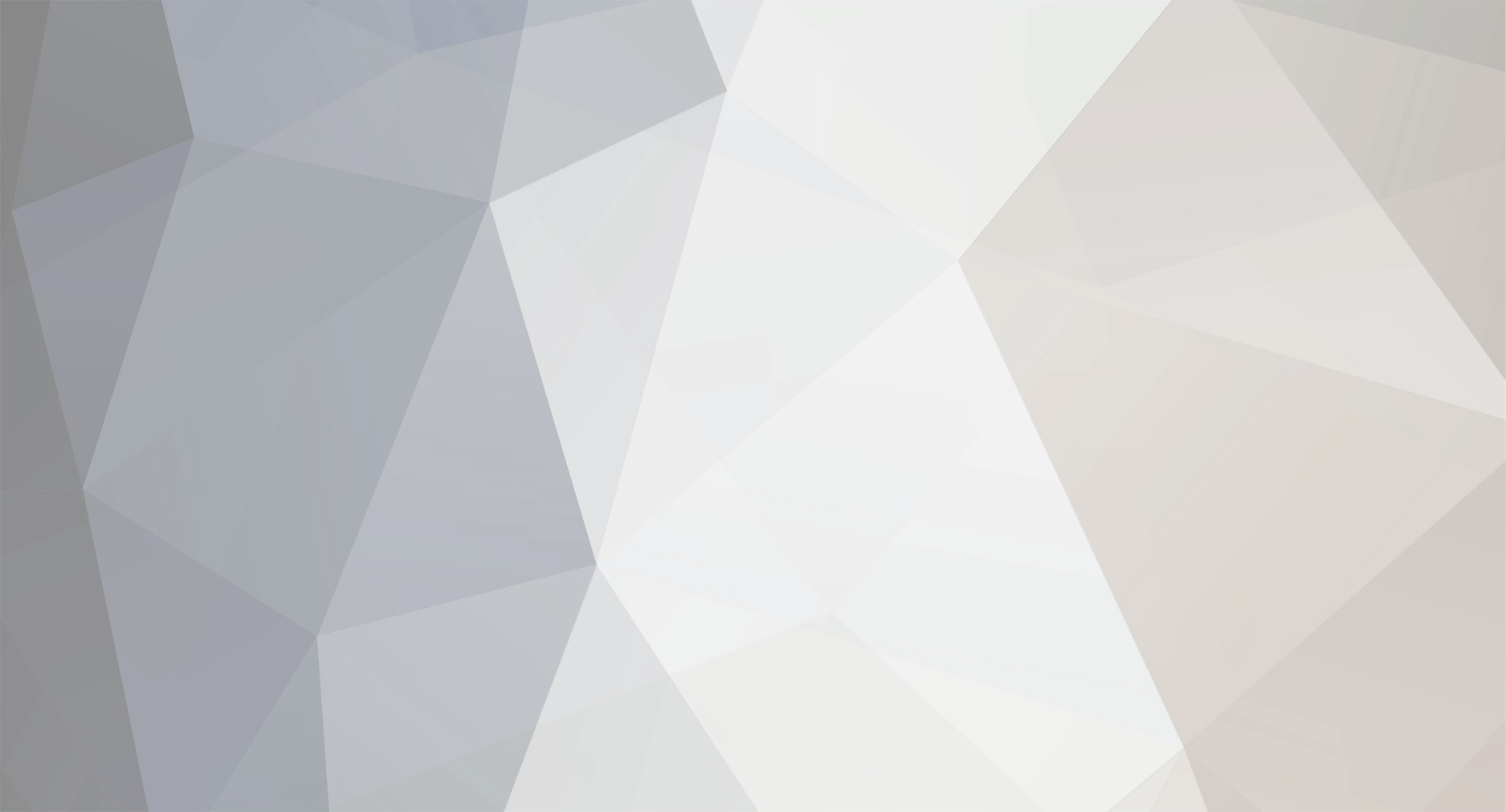
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
How to show trace/debug log entries in output console?
coolAlias replied to Two's topic in Modder Support
You can create your own Handler and Formatter for the Logger, allowing you to set what levels will be shown via logger.setLevel(Level.WHATEVER). That's with the java.util.logging.Logger, though. I'm not sure how to do it with the Apache one, but I imagine it is similar. If that's too much work for you, all logged messages (even TRACE and the like) are logged to a file on disk, such as 'eclipse/logs/fml-client-latest.txt', so you can use that for development debugging. -
[1.8] SimpleNetworkWrapper & io.netty.handler.codec.DecoderException
coolAlias replied to techstack's topic in Modder Support
It looks like you have a packet that is trying to read from an empty buffer, so I would double-check your toBytes and fromBytes implementations (the packet you linked looked fine, though). Another thing, in 1.8, the network is handled on a separate thread; see some discussion about it here. Being on a separate thread means that often the world and other objects which you would expect to be up-to-date may actually be out of sync, and odd things can happen. My own network code seems to be working fine in 1.8 and is almost directly ported from 1.7.10. Feel free to take a look. Sorry I can't tell you exactly what is wrong with your specific code -
You should use IExtendedEntityProperties. It has many advantages over getEntityData, not the least of which is that it performs better because you are not constantly reading and writing to NBT.
-
[1.7.10] ForgeDirection becoming null in packet from client to server
coolAlias replied to slugslug's topic in Modder Support
It's bad because it doesn't make any sense for your MessageHandler class to have class members or methods such as 'toBytes' - those belong to the message class and are actions a message can perform, but it is nonsensical for the handler to read itself from the byte buffer. Also, while you technically could implement it as you have, it gives you the false impression that you can use 'this.field' inside of the handler class to access your message's class members, but since the handler is not the message, this is again false and leads to errors such as yours. -
[1.7.10] ForgeDirection becoming null in packet from client to server
coolAlias replied to slugslug's topic in Modder Support
This is because the IMessage instance and the IMessageHandler instance are not the same object, even though you implement them in the same class. Thus the standard implementation being an IMessage class with a static nested class for the handler: public class SomeMessage implements IMessage { private int field; public SomeMessage() {} public SomeMessage(int something) { this.field = something; } // rest of IMessage methods // Static nested inner class which takes your message object as a parameter, meaning // that your message will be passed to it when received public static class Handler implements IMessageHandler<SomeMessage, IMessage> { @Override public final IMessage onMessage(SomeMessage msg, MessageContext ctx) { System.out.println(msg.field); // not null anymore because you got it from the IMessage instance rather than the handler } } -
Did you register your event listener? @Mod.EventHandler public void init(FMLInitializationEvent event) { // TickEvents are on the FML bus: FMLCommonHandler.instance().bus().register(new ArtifactEvent()); }
-
Yes, but you don't want it to run just on the client. Please read my response again: you can EITHER run the code ONLY on the server, in which case you may need to send that packet to update the client, OR you can try running the code ON BOTH sides, which means you wouldn't check world.isRemote at all (assuming that whatever method/event you are in does in fact run on both sides, which PlayerTickEvent does).
-
You're sending the packet from your TileEntity, right? So you should have x/y/zCoord available to create the TargetPoint: // for example, to send to all within 64 blocks: yourNetworkInstance.sendToAllAround(message, new NetworkRegistry.TargetPoint(dimension, xCoord, yCoord, zCoord, 64.0D));
-
It's been a while since I've messed with flying, but movement is usually handled on the client side, so you must inform the client of the player's changed capabilities (I don't think it happens automatically), which you can do with a vanilla packet: ((EntityPlayerMP) player).playerNetServerHandler.sendPacket(new C13PacketPlayerAbilities(player.capabilities)); Alternatively, you could let the tick logic run on both sides, rather than just the server.
-
You never write the NBT tag to the byte buffer, so the buffer index goes out of bounds when you try to read values from it. As for the nested handler class, it is simply convenient because you can then access the message class' private members without adding getter methods, and your handler is 99.9999999999999999999% likely to never be used in isolation anyways.
-
Which iterates through the inventory, but you are right, one does not have to do so manually. @OP If you want the player to be able to do other things while flying, then you have to potentially check the entire inventory every tick. It's not that terrible, but it's always better to avoid extra processing when you can. When you can't, well, computers these days are pretty dang fast.
-
You can't use the item's update method because that is not called if the item is not in your inventory - e.g. you toss it with 'Q' while flying, and then you will have permanent flying. PlayerTickEvent has a player parameter, so you can check player.getHeldItem() is your item (if you require it to be held) or iterate through the player's inventory (don't do this if you don't have to) to see if they have it.
-
[Solved] Make baby animals' model smaller than adults?
coolAlias replied to Shnupbups100's topic in Modder Support
If you look in the vanilla render classes, you will see that they set the scale for child versions, I think during the pre-render callback method: GL11.scalef(0.5F, 0.5F, 0.5F); EDIT: Actually, vanilla mobs do it inside of the model class (see ModelQuadruped#render, where model#isChild is set by the render class), but you can do the same thing inside preRenderCallback if you extend RenderLiving. -
[1.7.10] Server Client variable generation issue
coolAlias replied to Thornack's topic in Modder Support
You can send a packet immediately from just about anywhere, client or server. There should not be any queuing involved. In your particular case, you can take advantage of TileEntity#getDescriptionPacket and TileEntity#onDataPacket; any time a block updates (which you can manually trigger), the tile entity's description packet is sent to the client. -
[1.7.10] I'm trying to create a gun using extends ItemBow [SOLVED]
coolAlias replied to geoboom's topic in Modder Support
It doesn't matter what you set the texture name to if you override registerIcons - that's where the icons are registered, and you are registering a bunch as though you were going to have the bow pulling animations. If you're going to copy code, at least take the time to read it and try to figure out what each part is for. And to fix your problem, remove registerIcons entirely and remove every IIcon field / method in there - you don't need them if you are just using a single texture. -
Switch statement - read it, then USE it and experiment with it until you understand how it works. Once you do, get rid of that for loop you have and replace it with a switch statement on the random number generated for drop type, and return a specific drop for each case that you want.
-
Well, you used a 'for' loop, so if random is 3, you will drop both items '1' and '2'. Perhaps Java tutorials are in order?
-
I typically use #onSpawnWithEgg because that gives me access to real world coordinates, but if all you need to do is set a random integer, you can do so in the constructor as diesieben mentioned. Also, if you are spawning the entity, it won't have any NBT to overwrite because it didn't exist yet - can you give us more details / code on what you are doing?
-
[1.7.10] How do I disable GUI settings?
coolAlias replied to AgentCreeperHD's topic in Modder Support
What do you mean by 'do InitGuiEvent.Post'? Always post your code so people can see what you are trying and where the problem may be. All Forge event classes have various fields related to the specific event, accessed using the single Event parameter of your event listener: @SubscribeEvent public void nameItAnythingYouWant(SpecificForgeEvent event) { event.someField is now accessible for use } The specific event is the most critical component of that code, and must be the SOLE parameter for any event listener - adding any other parameters will prevent your code from being called. -
Well I'll be... I was doing it during the FMLInitializationEvent, since that's the first place it seems possible to register renderers (at least for items - mc.getRenderItem() is null during pre-init). Anyway, adding the variant names during pre-init got rid of those annoying messages. Thanks! EDIT 2: Actually, ignore the comment below. Adding variant names in the constructor is not only bad OOP, but ModelBakery is also client side only, so it would crash if run on a server. I need to go to bed EDIT: To keep the code cleaner, I decided to put it at the end of my Item class constructor and that seems to work just as well: public CustomItemClass(args[]) { super(); // assign any class fields, then add variant name(s) at end: ModelBakery.addVariantName(this, "actual_texture_name"); }
-
Torches (and many other blocks) use metadata to determine orientation, meaning you also need to set the correct metadata value for the block. For examples of how to do so, you should check out the ItemBlock class.
-
[1.8] Unknown net.minecraftforge.common.chunkio.QueuedChunk
coolAlias replied to bastetfurry's topic in Modder Support
The Decorate and Decorate.Pre/Post events are notoriously prone to throwing exceptions, though I don't recall the exact reason. One way to deal with it is to handle the exception: @SubscribeEvent public void onDecorate(DecorateBiomeEvent.Post event) { try { // Your gen code here } catch (Exception e) { Throwable cause = e.getCause(); if (e.getMessage() != null && e.getMessage().equals("Already decorating!!") || (cause != null && cause.getMessage() != null && cause.getMessage().equals("Already decorating!!"))) { ; } else { e.printStackTrace(); } } } There is very likely a better way to get around this, but I have used that and it allows the custom generation code to function without crashing. -
That's a protected method. I could use reflection to gain access to it, but there must be a better way - it's not like dyes are a unique case, certainly not amongst mods, so it would make sense that FML has some way to support this, but I just can't seem to find it. EDIT: I believe the issue stems from line 757 in GameData within the #registerItem(Item item, String name, int idHint) method, which is called by the GameRegistry when an item is registered: ((RegistryDelegate.Delegate<Item>) item.delegate).setName(name); Then in ModelBakery, the following code adds all of the delegated item names to the variantNames: for (Entry<net.minecraftforge.fml.common.registry.RegistryDelegate<Item>, Set<String>> e : customVariantNames.entrySet()) { this.variantNames.put(e.getKey().get(), Lists.newArrayList(e.getValue().iterator())); } The advantage of this is that if you register your item with the same name you will use for the texture, you don't have to do anything else, but there does not seem to be a way to avoid it, which doesn't make any sense given the existence of items such as dye.
-
Consider a vanilla example - dye. // Item.java initializes the base Item with generic names: registerItem(351, "dye", (new ItemDye()).setUnlocalizedName("dyePowder")); // Items.java gets the generic instance and assigns it a reference dye = getRegisteredItem("dye"); // ModelBakery.java attaches a list of all the subtypes, which are the 'real' items this.variantNames.put(Items.dye, Lists.newArrayList(new String[] {"dye_black", "dye_red", "dye_green", "dye_brown", "dye_blue", "dye_purple", "dye_cyan", "dye_silver", "dye_gray", "dye_pink", "dye_lime", "dye_yellow", "dye_light_blue", "dye_magenta", "dye_orange", "dye_white"})); // RenderItem.java registers each variant: this.registerItem(Items.dye, EnumDyeColor.BLACK.getDyeDamage(), "dye_black"); The result of that is exactly what I want - no need for a 'dye.json' because that generic item doesn't really exist, but is useful within the code for retrieving the base version of the item. However, there doesn't seem to be any way to specify variant names for custom items - using the ModelBakery#addVariantName method added by FML/Forge, the names are ADDED to what is already there, and whatever we register the item as to the GameRegistry seems to be automatically registered as a variant by FML, which is exactly what I don't want to happen. Am I just missing something plain as day, or is it really designed to be this way?