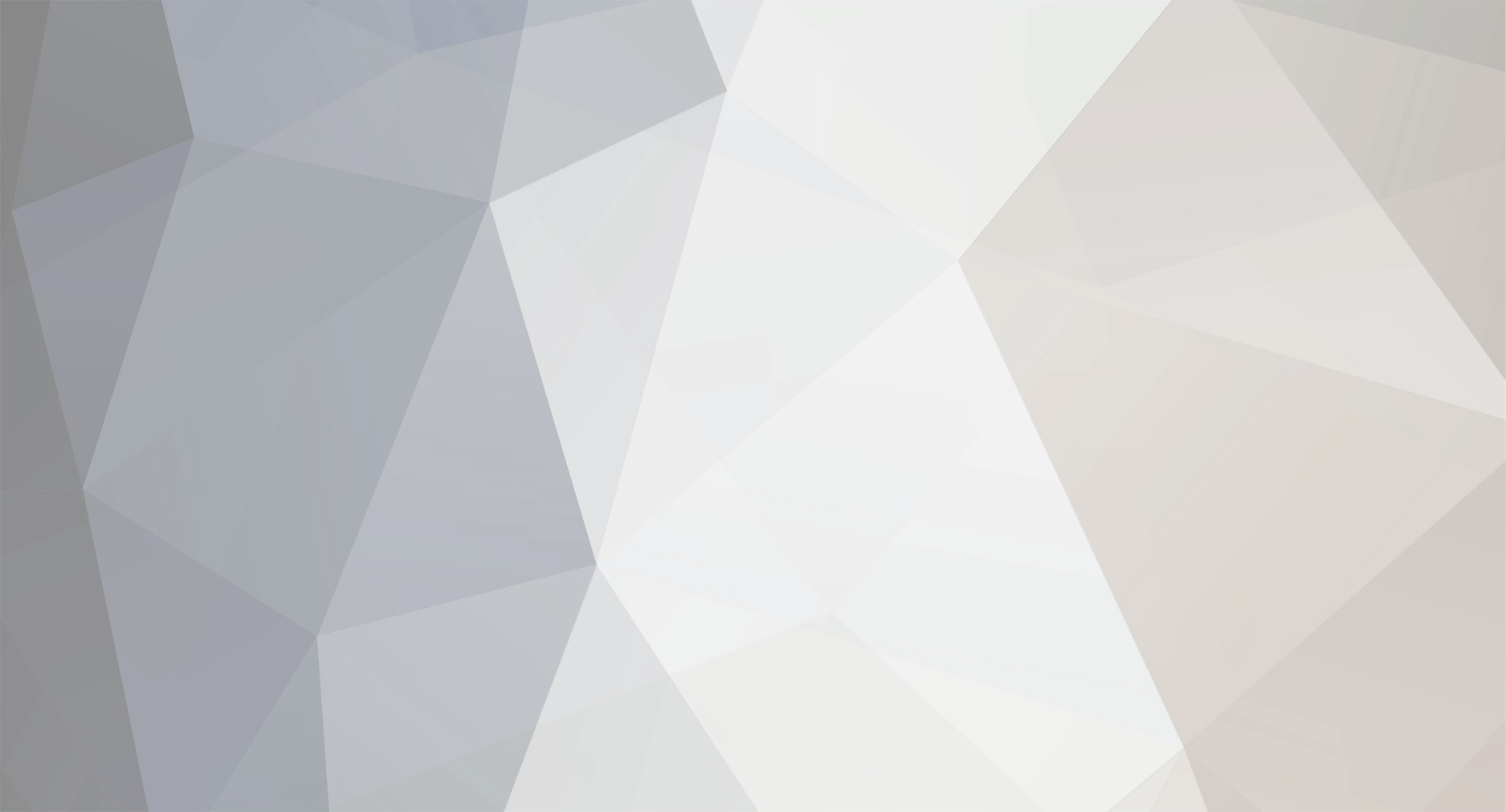
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Wait a second, if it's a custom block, how is it all vanilla? If you tell it to render a vanilla block but make it run 2 render passes on that vanilla block, that would probably explain why it's crashing - most vanilla blocks don't expect that 2nd pass. I assume you have a reason for wanting that 2nd pass on a vanilla block overlay, but I'm not sure how you can get around that. Not that you need an example, but I have a block that can render as most vanilla blocks; thing is, it only uses the first render pass. How are you managing to do so without a custom renderer?
-
You already have the logic part of it handled in your radar class, it looks like, though you shouldn't use Minecraft.getMinecraft().thePlayer - that's CLIENT side only player and will crash your game when run on a server. So how is your block supposed to work? What I mean is, if I place one of these blocks, the way you have it now it will be alerting EVERY player in the game when entities get close to that block. Shouldn't it only alert me or some other player who activates the block at least? What I would suggest is overriding onBlockPlacedBy in your Block class and storing the player who placed the block in your tile entity class, then you have a server side instance (which you can also send chat messages to, btw) and your block will only notify that one player. If you want, you could make it store a list of players and activating the block (right click) would add or remove you (the player) from the list. Anyway, if you registered your TileEntity / Block classes and your Block overrides both hasTileEntity and createTileEntity (both Forge methods), then you should be golden.
-
Nice summary there Ernio! For entities that implement IEntityAdditionalSpawnData, is that data not also sent to the client when they start tracking, since the client version of the entity must be constructed? If your data doesn't change, then, I would expect that to be sufficient - you shouldn't even need IExtendedEntityProperties unless you want the data to be applied to vanilla / other mod's entities.
-
Does this only happen right at world start up? Can you get it to crash if you place a lot of your blocks by hand, perhaps all bunched together in say a 5x5x5 or larger cube? Have you tried putting a break point in there to see what the Tessellator and renderer states are at that point? I suppose the worst case scenario is you surround the second render pass in a try/catch.
-
I understand that; my question was regarding your statement "Don't implement ITileEntityProvider" - if you don't, then your Block will not have a TileEntity regardless of what you return for hasTileEntity, so you are still stuck with either implementing ITileEntityProvider or overriding Forge's Block#createTileEntity method to return a valid TE, are you not? Is there a reason, then, NOT to implement ITileEntityProvider?
-
What about ITileEntityProvider#createNewTileEntity(World world, int metadata), though? I see Forge has added Block#createTileEntity(World, int), but if your Block doesn't implement ITileEntityProvider and return a valid TileEntity, wouldn't you always just end up with null?
-
Saving information after the game is quit?
coolAlias replied to Toastrackenigma's topic in Modder Support
Use WorldSavedData to store data on a per-world basis. -
The problem lies in the TileEntityFurnace having hard-coded references to the vanilla active/idle furnace blocks, as well as using a static method 'keepInventory' - if this weren't the case, every modder could easily extend this one class, make a few minor changes and be done with it. This goes for any type of machine - I've seen (and coded some myself... derp) many machine variations, grinders, furnaces, etc. that all follow the vanilla 'model' and make the same poor design decisions, but even if you were to design a more flexible class, one that say takes two Blocks in a non-static keepInventory method and by default switches between the two, what if a mod wanted 3 states? Or 4? Also, the logic within the TE class itself is always going to be far too specific, whereas the fact that it implements one of the Inventory interfaces already makes it generic enough for any mod to interact with it (as Draco mentioned). The best I've come up with, not really related to furnaces per se, is to make a generic TileEntity with IInventory implementation, since I found myself repeating that code a lot. If I was making lots of furnaces and other machines, I might be inspired to do something similar, but I don't think it would turn out to be as widely applicable as one might hope.
-
[SOLVED][1.7.10+] Check player starts tracking himself.
coolAlias replied to Ernio's topic in Modder Support
Sending from EntityJoinWorldEvent does work, unless you are you talking about 1.8? In that case, yes, you have to make sure you only process the packet on the main thread, otherwise you are right, the client player will not have been constructed yet. I've used it plenty and it works fine for me, but use whatever works mate. -
[SOLVED][1.7.10+] Check player starts tracking himself.
coolAlias replied to Ernio's topic in Modder Support
EntityJoinWorldEvent works perfectly for this, too, if you want to catch both login and respawn in one event, but it fires for all entities so you have to check if it's a player so it's probably less efficient than using the two specific events combined. Still, if you are doing other stuff there already, it's handy. -
For starters, making an instance of a TileEntity inside of an Item. I don't know what you expect it to do, but that TileEntity instance won't be doing much of anything since it doesn't exist in the World.
-
[1.7.10] Adding trade to "new" villager type mob
coolAlias replied to DonKresenko's topic in Modder Support
Your problem is that you are extending EntityVillager, but buyingList and addDefaultEquipment are both PRIVATE members of EntityVillager - you cannot use or override them without Reflection or ASM. What you can do, however, is override getRecipes to return your own list, but because you override EntityVillager, your mob is still using the villager's buyingList (which is NULL) when useRecipe or any other villager method is called. You either have to override every method from EntityVillager which interacts with buyingList and make it use your own list, or you need to not extend EntityVillager and just implement IMerchant instead. -
No, you use it when registering your TickEvent handling class. Look at the method requirements for FMLCommonHandler's 'register' method - it requires an Object instance be passed in, i.e. the instance of your class.
-
Yeah, it got pasted twice. Fixed. That's not an instance of your class, so of course it wouldn't work. The argument is an Object, not a Class: new TickHandler() // that creates an instance of your class, i.e. a real object that exists
-
... sounds like you need to learn Java.
-
Why don't you make the leaves children of the head? Then whenever the head moves, you don't have to do anything and they will automatically rotate / move with it.
-
That's because the MinecraftForge.EVENT_BUS is NOT the FML bus. FMLCommonHandler.instance().bus().register(yourEvents);
-
If you didn't register it, then yes, you do, just like any other event-watching class. TickEvents are fired on the FML bus, so register your TickHandler class on that one.
-
[1.7.10] Adding trade to "new" villager type mob
coolAlias replied to DonKresenko's topic in Modder Support
You made a 'new MerchantRecipeList' but only assign it to a local variable within the method - you never assign anything to your 'buyingList', thus it is always empty (null). -
[1.7.10]How to check actual entity type with Check Spawn event
coolAlias replied to jabelar's topic in Modder Support
You're right, it seems that CheckSpawn is not fired at all for animals: @SubscribeEvent public void onSpawn(LivingSpawnEvent.CheckSpawn event) { if (event.entity instanceof EntityAnimal) { // never prints... System.out.println("Spawning animal class: " + event.entity.getClass().getSimpleName()); } } I traced it back to the WorldServer#tick, where it is initially called from here: if (this.getGameRules().getGameRuleBooleanValue("doMobSpawning")) { this.animalSpawner.findChunksForSpawning(this, this.spawnHostileMobs, this.spawnPeacefulMobs, this.worldInfo.getWorldTotalTime() % 400L == 0L); } If you look at that method in AnimalSpawner(), it turns out the final parameter determines whether animals should spawn: (!enumcreaturetype.getAnimal() || p_77192_4_) <== last parameter, which is every 400 ticks apparently Still, animals obviously spawn, but the event never seems to be posted for them despite having the same code as other entity types. Maybe they are actually being spawned from somewhere else in the vanilla code? -
[Solved][1.7.10]Rendering Nametag Above Custom Mob?
coolAlias replied to skullcrusher1005's topic in Modder Support
No, don't use events. Put that code in your entity's constructor, like I said earlier. -
[1.7.10]How to check actual entity type with Check Spawn event
coolAlias replied to jabelar's topic in Modder Support
I already mentioned that, but yes. Should I have put it in bold? -
[1.7.10] detect if specific player enters/leaves area.
coolAlias replied to denbukki's topic in Modder Support
Then you'll have to store which players have been found, e.g. Set<EntityPlayer> playersInArea; if the player is found by getEntitiesWithinAABB but is not yet in the set, add them to it and do whatever you want for when they enter the area; if the player is in the set but not found nearby, remove them from the set and do whatever you want to the player for when they leave. -
[1.7.10]How to check actual entity type with Check Spawn event
coolAlias replied to jabelar's topic in Modder Support
EntityJoinWorldEvent fires once for each individual entity as it spawns, not once per type or only sometimes, but every single time. A class instance in Java (and most other OOP languages) will always check true for every class from which it inherits, all the way up to Object, so EntitySheep will return true for instanceof in all of the following cases (in addition to any interfaces any of those types implement): EntitySheep->EntityAnimal->EntityAgeable->EntityCreature->EntityLiving->EntityLivingBase->Entity->Object What you can do to see the current class that is spawning: // prints the actual class, i.e. the most specific type, e.g. EntitySheep System.out.println("Spawning: " + event.entity.getClass().getSimpleName()); EDIT: When I print that out, I literally get spammed with console messages. Perhaps yours are buried amidst other entities?