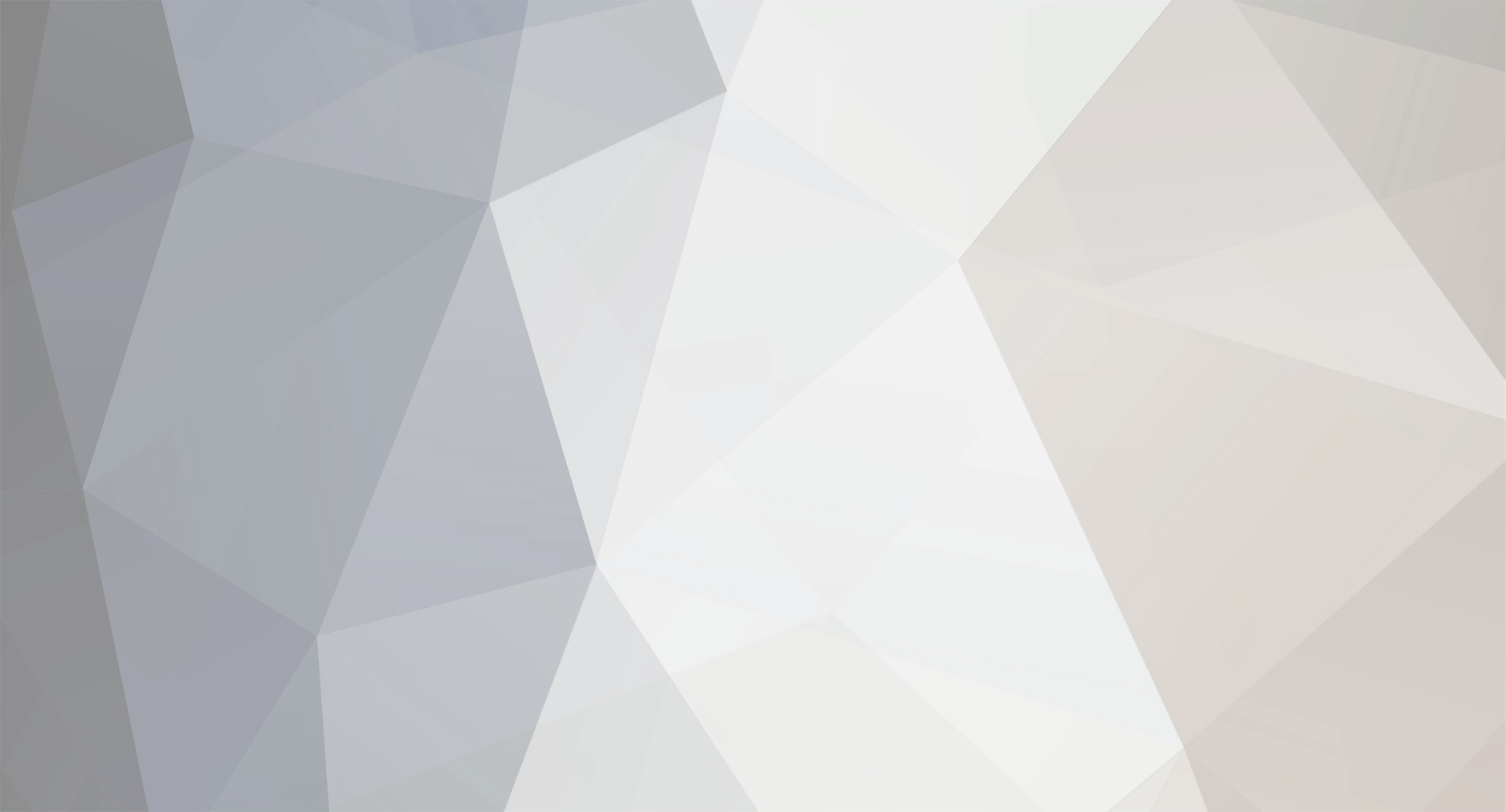
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
how to detect if a class implements an interface
coolAlias replied to memcallen's topic in Modder Support
Assuming you have an instance of your class (or any instance of any class, really), such as 'Battery battery = new Battery()', then to check if it implements an interface and then use those interface methods, you use the following, which is what everyone else has been trying to say: // checking if the object implements the interface if (battery instanceof IPowerProvider) { // now casting the object to the interface class so you can use the interface methods IPowerProvider power = (IPowerProvider) battery; power.someInterfaceMethod(); // which you can also write as one line (after checking instanceof) if you only need to call one method: ((IPowerProvider) battery).someInterfaceMethod(); } -
[SOLVED] How are rendering parameters determined?
coolAlias replied to coolAlias's topic in Modder Support
That's the strange part - both the body and head rotated normally on the screen, not just one or the other, and none of the yaw/renderYaw or any of those variables seemed to be working. I probably just screwed something up in the model, but nothing stood out at me. A problem for another day, perhaps -
[SOLVED] How are rendering parameters determined?
coolAlias replied to coolAlias's topic in Modder Support
@TGG Yes, I looked at the renderYawOffset and a host of other fields as well. No dice Anyway, shortly after realizing what the dx/dy/dz parameters were, I was able to come up with a solution: Vec3 vec3 = Vec3.createVectorHelper(dx, dy, dz).normalize(); GL11.glTranslated(dx - vec3.xCoord, dy + caster.getEyeHeight(), dz - vec3.zCoord); This renders the spell perfectly in front of the caster's eyes, though I am not yet sure how well it will work if there are a bunch of players all looking at the entity from different angles... whatever, it looks great in single player, at least! -
[SOLVED] How are rendering parameters determined?
coolAlias replied to coolAlias's topic in Modder Support
I've been doing some debugging and here's what I've discovered: The parameters to RenderLiving#doRender(dx, dy, dz, yaw, partialTick) are apparently all relative to the player. dy is usually -1.6200..., becoming more negative if you jump, and both dx and dz appear to be the difference between the entity and the player's position (which implies that dy is also the difference in y position). I'm not sure yet how yaw is determined, but it is not affected by the player's rotation at all - the player spinning around in circles did not change the yaw parameter in the entity's doRender call. In fact, during my last test it stayed exactly at -21.09375 the entire game session... no matter where I stood, which way the spell-casting entity was facing, nothing changed it. I'm at a loss with this one. I still have no idea why the entity's rotationYaw and look vector remain the same for such extended periods of time, either. I can see the entity turning this way and that on the screen, but apparently the rotationYaw is somehow disconnected from it. Needless to say, what I've found is not at all what I expected. Am I missing something glaringly obvious? As it is, I don't see how any object could possibly be rendered in front of an entity, if the parameters are always relative to the on-looking player(s), but clearly it IS possible. -
While working on my spell-casting entity, I got the spell to render in front of the main entity, but I noticed that its position was not always correct, nor would it ever change position as the main entity turned or moved. This got me thinking and printing stuff to the screen, and I discovered that for the entire time while my spell was rendering (about 20 ticks), the entity's look vector, rotationYaw, and the yaw parameter passed to the doRender method did not ever change. Here's the relevant sections of my entity's render code: Here is some sample output from my logging: So I'm curious: if the caster turns, should not his look vector change to match, or at least the caster's rotationYaw as I clearly can see the caster rotating on the screen, so the client is obviously aware of the current rotationYaw, and how is the 'yaw' parameter from the doRender method determined / why would it be identical for over 20 ticks straight? Thanks for any tips, coolAlias
-
[1.7.x] Adding attributes to vanilla mobs
coolAlias replied to Major_Mints's topic in Modder Support
Exactly as jabelar said, but if you wait for 1.8, one of the major points of the update was to get ALL of the entities using the AI system (it's shocking how many don't use it in 1.7, actually). In the meantime, his solution is viable - I've done similar things in the past and they work fine. -
The annotation is @SubscribeEvent.
-
Rendering an entity within another entity's render
coolAlias replied to coolAlias's topic in Modder Support
Ha, you are probably right TGG. Instantiating a render class instance probably doesn't initialize the render manager/engine in any way... hmm. Sometimes it just takes another pair of eyes -
I'm working on a spell-casting mob and was thinking I would be clever and just create a fake spell entity within the mob's render class, and render that as appropriate, since I don't actually want to spawn the spell into the world just yet. Anyway, Minecraft doesn't seem to like that so much: Here is what I tried in the mob's render class: Here is the RenderEntityMagicSpell class - works fine for real spell entities rendering in the world: Clearly the problem is that Render#bindEntityTexture is not able to get the correct texture, but after going through the stack trace and trying to trace the method calls, I still cannot fathom why. Does anyone have experience doing something like this?
-
[SOLVED] Set Direction of Block (chest) during generate()
coolAlias replied to gottsch's topic in Modder Support
The problem is that chests automatically set their own metadata once placed in the world, so the value you use in the initial setBlock is actually ignored - to get around the problem, you just have to set the metadata after the block is placed, as memcallen mentioned earlier. -
With coding, there is always more to learn, and after having coded for a while, you may be astonished at what you can still learn by going back over the basics
-
You are confusing rendering with functionality. You could render a 1x1x1 cube as a giant whale if you wanted, but 'physically' it would still just be a 1x1x1 cube. Same goes for your slab and adjacent block examples - it doesn't matter what is next to your block, or what any block is rendering as, for it to function in a certain way, unless you code it that way. It would probably be a good idea to spend some time learning more Java, and the answers to your questions will become obvious to you.
-
There is no built-in way to achieve attack delay, at least that I have found. What I did is hijack vanilla's EntityLivingBase#attackTime field, since it is not used at all for players, and, using MouseEvent, whenever the player left-clicks with your weapon, you set this timer or, if the timer is already greater than zero, cancel the event, thus preventing any attack from processing. The timer is automatically decremented for you during the player's update tick, so you don't have to worry about managing it. As for getting the hammer to swing slowly without the potion effect... The only way I can think of would be to cancel the normal player rendering from RenderPlayerEvent (only while swinging your hammer, of course) and do the rendering yourself based on current swing time. RenderHandEvent may actually be enough; if so, it is the better option. For custom events, I would post to the same one that the parent event does.
-
[Solved] [1.6.4] Creating a scope for a weapon?
coolAlias replied to adusut's topic in Modder Support
Use FOVUpdateEvent - you can see my implementation here, which is flexible enough to allow different levels of zoom. -
See my reply here for a solution.
-
You could try mine, but it might be a bit too complicated if you're not even familiar with packets yet. A better option to start with would be diesieben07's tutorial.
-
mc.thePlayer, but you'll have to make sure that the client-side data is updated from the server via packets.
-
Players extend from EntityLivingBase as well, so have the same methods available to them.
-
The rotation problem is a simple fix: put "setFull3D();" in your bow's constructor. It's used by swords, bows, and similar things to flag the renderer to rotate the item in your hand, so it looks like you are wielding it rather than simply holding it.
-
The methods are pretty unintuitive and mixed up; what happens when an entity is attacked (i.e. attackEntityFrom is called, which happens almost always when an entity is damaged), the entity calls setRevengeTarget, which sets the field entityLivingToAttack which can be retrieved via getAITarget(); if the entity is a player, it also sets the attackingPlayer field, which does not have a specific getter, but you can use EntityLivingBase#func_94060_bK(), which returns either the combat tracker target, the attackingPlayer, or the entityLivingToAttack (i.e. AI target). It's not a perfect solution, as your mob may have a target without being attacked, in which case it would apply the effect in error, so you could use getAITarget first then, if that returns null, call func_94060_bK and check if it is an EntityPlayer - this way may avoid some of the incorrect triggers, but it's difficult to say for sure how well it would work. Depending on how your code is supposed to work, it may be better to use LivingHurtEvent and cause the effect to the hurt entity's attacker using event.source.getEntity(), or to put the code in your mob's attackEntityFrom method, but it looks like that may not be an option for you.
-
In 1.7, you no longer use any events at all, but a json file for sounds. Put it in your resources/assets directory (the base directory, not the sounds folder). It should look something like this: { "levelup": {"category": "player","sounds":["special/levelup"]}, "master_sword": {"category": "ambient","sounds":["special/master_sword"]}, "secret_medley": {"category": "ambient","sounds":["special/secret_medley"]} } Note that the final entry does not have a comma. The directory for those sounds is "assets/sounds/sound | subfolder" EDIT: Btw, I have only used .ogg format in 1.7; I'm not sure if .wav works or not, so you'll have to try it.
-
armorInventory[] slots on the EntityPlayer [1.7.10]
coolAlias replied to LordMastodonFTW's topic in Modder Support
It is 3 if you use player.getEquipmentInSlot(i), as slot 0 is the held item and 1-4 are the armor slots, with the same indexes as diesieben07 mentioned but all incremented by 1. You don't have to shift the index if you use player.getCurrentArmor(i). -
Since your code will be client side for the rendering, you can use Minecraft.getMinecraft().objectMouseOver, which is the current MovingObjectPosition as determined by the EntityRenderer. Use it during RenderGameOverlayEvent.Pre for event.type==ElementType.CROSSHAIRS, and you won't have to do any calculations yourself.
-
armorInventory[] slots on the EntityPlayer [1.7.10]
coolAlias replied to LordMastodonFTW's topic in Modder Support
Also, if you look in ItemArmor, you will see that those are the opposite indexes of what you would use when creating armor: /** * Stores the armor type: 0 is helmet, 1 is plate, 2 is legs and 3 is boots */ public final int armorType; Confusing that they don't match, eh? But the indexes you want for your question are the ones diesieben07 pointed out.