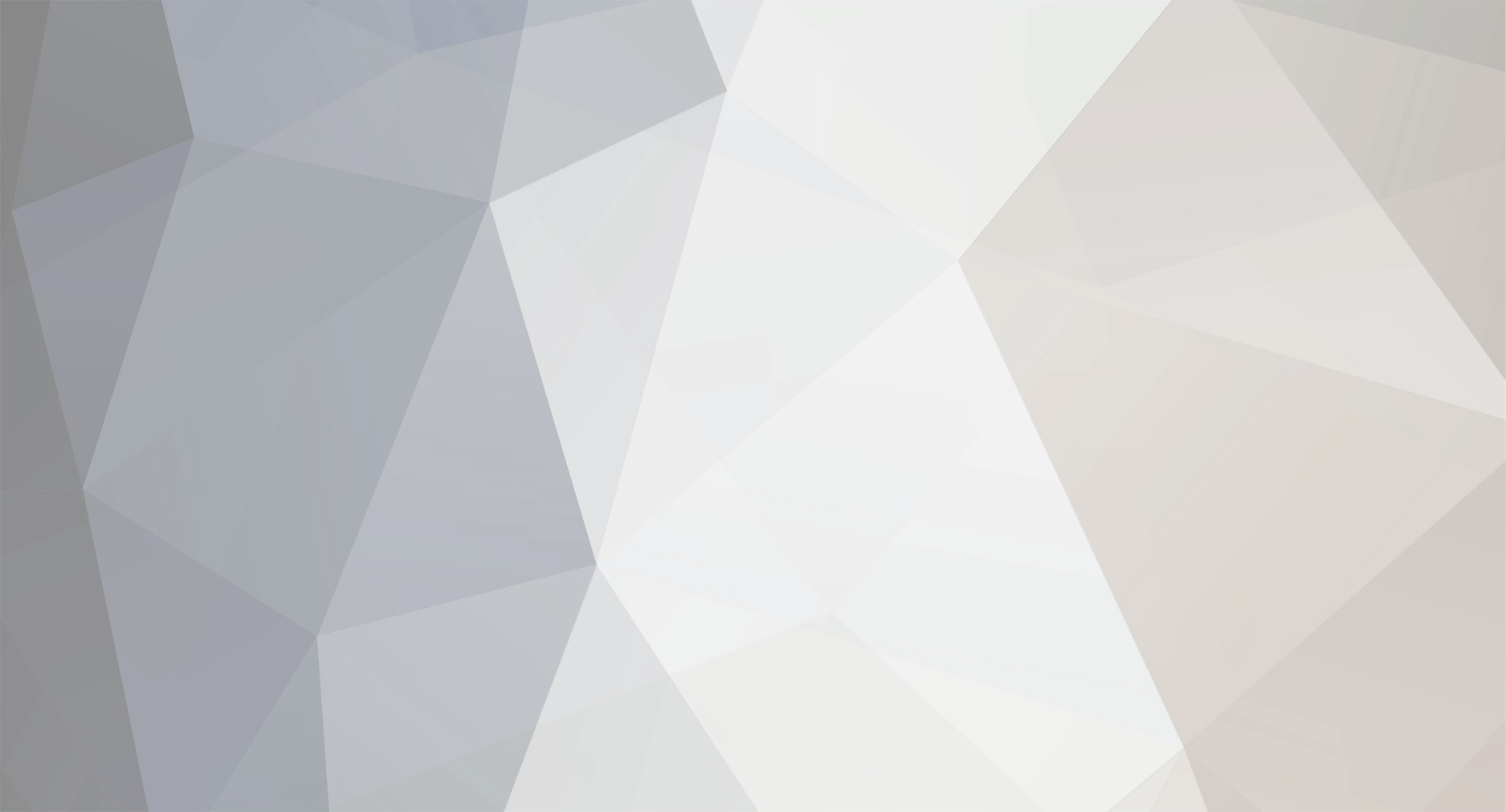
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
You could make your own spawner TileEntity and just re-use the code for the model / animation of the block in the world. That would give you all the control you need over which entity to spawn and what to equip it with, for example by setting a "level" variable in the tile entity, you could use that "level" to retrieve equipment level, enchantments, or anything else you wanted, either by linking it to some kind of Map or just using a good old switch statement.
-
True, but that not only adds an extra step in the process, it is also likely to not work in 1.8. Might as well switch now
-
Change the return type of getValidSpawnBlocks from int[] to Block[] and return a Block array; iterate through that instead. Likewise change all the "int someId" to "Block someBlock" in your code, as world.getBlock(x, y, z) returns a Block, not an int.
-
Hi, I was indeed able to get the camera angle to a higher position using some code that Noppes shared with me. You can read the full thread about it here. The solution I posted there worked for 1.6.4, but I had to change a few things to get the mouse over position working correctly for 1.7.2. I'll put the highlights of it here: It's not perfect, as you can see, but it works for the most part and is a lot simpler than what I could find in iChun's Morph mod. Whether iChun's works any better or worse I can't say, as I've never tried his mod, but you it's open source so you can check it out for yourself: https://github.com/iChun/Morph Good luck!
-
You're on the right track - it works for most entities because it is handled on the server, but for players, their motion is actually handled on the client. You need to send a packet telling the player client about its new motion for it to work. After making sure the entity hit is a player and you are on the server, you can use this vanilla packet: ((EntityPlayerMP) mop.entityHit).playerNetServerHandler.sendPacket(new S12PacketEntityVelocity(mop.entityHit)); Just be sure you set the player's new motion values first
-
[1.7.2] armor material in configuration file
coolAlias replied to thomassu's topic in Modder Support
You should learn Java before you start modding... Just because you put that now at the bottom doesn't mean it gets called after all methods in the class. Aside: static initializers actually do get called in the order they appear in the code, according to Java docs: "The runtime system guarantees that static initialization blocks are called in the order that they appear in the source code." While that refers specifically to static blocks, it's true for all static initializations. More info. @OP That doesn't negate the fact that you are doing it incorrectly. Your static PeanutPartC fields are all unitialized when you attempt to use them, which is something you should know as part of fundamental Java basics. As SanAndreasP suggests, I recommend you start there. Your values need to be read from the config, correct? Whereever your config sets those values (i.e. in a method body) is where you need to put your ArmorMaterial initialization. public static ArmorMaterial PEANUT = EnumHelper.OOPS. Config values have not been read here!!! // declare: public static ArmorMaterial PEANUT; @EventHandler public void preInit(FMLPreInitializationEvent event) { // Config is read here // Sweet, now the values should be set, and it is safe to initialize PEANUT = EnumHelper.SUCCESS! } -
[1.6.4] Best practices for organizing code for a mod?
coolAlias replied to keybounce's topic in Modder Support
If you are using Gradle, you can easily set up multiple projects in the same workspace; check Lex's tutorial: Also, after getting tons of help from GotoLink on using one of his APIs and then writing one of my own, I put together this little piece that you may or may not find helpful: http://www.minecraftforum.net/topic/2496232-tutorial-modding-with-apis/ Hope that helps! -
I don't suppose you could inform me of any examples (either vanilla or open-source mod?) Not quite sure I follow, I've always been a bit confused by redstone for some reason =/ Yeah, all the vanilla redstone blocks, from wire to torches to levers etc., all provide excellent examples. I recommend looking through those respective block classes. As a more immediate example, these are the two methods I needed to add to one of my blocks to get it to provide power. Note that it's just a simple on/off based on whether the master sword is in the pedestal, but you could return any amount based on any variable stored in your tile entity: @Override public boolean canProvidePower() { return true; } @Override public int isProvidingWeakPower(IBlockAccess world, int x, int y, int z, int side) { EDIT: oops, that's 1.7: TileEntity te = world.getTileEntity(x, y, z); TileEntity te = world.getBlockTileEntity(x, y, z); if (te instanceof TileEntityPedestal) { ItemStack sword = ((TileEntityPedestal) te).getSword(); if (sword != null && sword.getItem() == ZSSItems.swordMaster) { return 15; } } return 0; }
-
A lot of redstone logic is handled via notification of neighbors rather than update ticks, as well as using the isProvidingStrongPower and isProvidingWeakPower methods. Those both have x/y/z coordinates, so you can check your tile entity's data to return whatever power level you want, all without using update ticks. This way you provide real-time signal strength but only when requested.
-
No, i'm spawning it in both sides. I only spawn effects on client. That's the point: don't spawn it on the client, that happens automatically. Same for explosions and destroying blocks - only do that on the server side or you will encounter these "ghost" blocks. The client is updated automatically when blocks are destroyed.
-
This is the simplest way I know of, though that's not to say there isn't any other way. It's basically two steps: 1. Store the data when the player dies 2. Load the data when the player respawns Maybe you can automate those steps by storing the data directly in the player's PERSISTED_NBT_TAG tag, but I have never had any luck with that one. I didn't try too hard, since I already had everything working, so maybe you can get it to work. EDIT: Here's the relevant code from EntityPlayer#clonePlayer: //Copy over a section of the Entity Data from the old player. //Allows mods to specify data that persists after players respawn. NBTTagCompound old = par1EntityPlayer.getEntityData(); if (old.hasKey(PERSISTED_NBT_TAG)) { getEntityData().setTag(PERSISTED_NBT_TAG, old.getCompoundTag(PERSISTED_NBT_TAG)); }
-
Sorry I guess that part was a little unclear: PlayerInformation.saveProxyData((EntityPlayer) event.entity); is a replacement for, not an addition to, all the code in the living death event. See section 6 for the exact details, or you can see a working example here: https://github.com/coolAlias/Tutorial-1.7.2/tree/master/src/main/java/tutorial
-
username cannot be accessed in 1.7.2, change it to getCommandSenderName() or whatever. For the rest, you have to add the methods, otherwise of course they are undefined.
-
Extended property data doesn't load by default when the player respawns, but you can do so manually using the PlayerRespawnEvent from FML. Be sure to save the data first when the player dies! Also, this may interest you, as it discusses the issue of persisting your data across death and respawn. Note that I didn't use the PlayerRespawnEvent, but that would be preferable as it is only posted for players.
-
Take a look at some flying entities to see how they use flight paths (such as the Ghast or Bat); you should be able to adapt that to your needs, taking into account the new position's height above the ground and such as needed, possibly even giving your mob some leeway so it can fly anywhere between 1-4 blocks above the ground, rather than always 4. One advantage of using a flight path is you can calculate a target coordinate and then let the mob move without re-checking the flight path until it arrives or gets close to the destination; this is nice if your flight calculations are complicated, but you may also want to allow the path to be interrupted say if the mob is attacked or a player comes near (like with bats).
-
registerModEntity doesn't add entities to the ID mapping in EntityList, but it does add them by name and class. The following is a snippet of the registration code: String entityModName = String.format("%s.%s", mc.getModId(), entityName); EntityList.classToStringMapping.put(entityClass, entityModName); EntityList.stringToClassMapping.put(entityModName, entityClass); If you print out the entity list using the string to class map, with "modid.entityName", you will surely see your entities. The ID to class mapping is only used by spawn eggs (which is why the mod entity index and global ID can be different). My question is why haven't either Mojang or Forge team altered the vanilla ID to class map to allow everyone to use the ItemMonsterPlacer? Sure, it may be lazy to not make our own, but it's also good coding practice to not reinvent the wheel. Why copy/paste when you should be able to use something directly, or even through inheritance? It just seems bizarre to be moving toward a modding API but leave something as widely used as this in such a state.
-
Yeah, you didn't use it right :\ Any conditional statements you want to use have to be involved in the logic of the result, otherwise it is totally wasted. Look: if (can player harvest and player has a pickaxe) { // here's your result: add level; } if (material doesn't require tool) { do nothing... // but your result has already finished... } Does that make sense? You have to use the if statement as a pre-condition for your result, i.e. put it INSIDE of the other if statement. You could also check if the material does not NOT need a tool, meaning it does need a tool... if (can harvest and pickaxe stuff) { if (!block.getMaterial().isToolNotRequired()) { // it doesn't not not need a tool: double-negative is positive, so it DOES need a tool // great! add your xp } }
-
No, don't create a compound. @Override public void saveNBTData(NBTTagCompound compound) { // see that NBTTagCompound compound ^^^^^^^ right there? use that: compound.setInteger("someData", value); } Same for reading from NBT. How do you expect the data to persist if you create new data every single time?
-
You have to store your data in the tag compound that you are passed. Don't create a new NBTTagCompound unless you are adding that tag to the parameter compound. That goes for reading, too - use the parameter.
-
Some blocks can be harvested by anything, including a player's hands, so those will return true. You can check first to make sure a tool is required to harvest the block, thus ruling out those that can be harvested with anything (or nothing ) if (block.getMaterial().isToolNotRequired()) { // don't get xp for this one! } // this will crash if the player's not holding anything: player.inventory.getCurrentItem().getItem() // be sure to null check first, and I use getHeldItem() because it's shorter to write: player.getHeldItem() != null && player.getHeldItem().getItem()
-
Event methods can only have a single parameter: the event to which you are subscribing. All other stuff (player, block, etc) must be retrieved some other way, typically they are fields in the event class. In this case, BreakEvent has public fields for the block and metadata, and a getter method to retrieve the player. Ctrl-click on "BreakEvent" to open up the class in Eclipse, or even just expand it in the package explorer to see what variables are available to you and how to get them.
-
Do you want it only for your custom pickaxe, or any pickaxe breaking any pickaxe-breakable block? If it's the latter, then you do want to use an event: BreakEvent. If you check out the BreakEvent code, you'll see how to check if the block is harvestable by the player (i.e. returns true when the player is using the correct tool for the job). You can also check if the player is holding a pickaxe before awarding your xp. These two checks in combination assure you that a) you have a pickaxe and b) the player's pickaxe can harvest the current block that just broke. You may also want to check if the player is silk-harvesting the block; again, look at the BreakEvent's code for an example. In pseudo-code: @SubscribeEvent public void onBreakBlock(BreakEvent event) { if (ForgeHooks.canHarvestBlock(block, player, meta) and player is holding an item and that item is a pickaxe) { add experience to the player } }
-
[1.7.2]Any key holding method? or any suggestion on how to do this?
coolAlias replied to lorizz's topic in Modder Support
If you need to check that a key is pressed constantly, don't do that inside the key input event, and not with a while loop. I'm pretty sure that the key input event is called when a key is pressed, or released, but not any time in between. It is not a tick event. I suggest using some kind of onUpdate method, be it in a custom item, onLivingUpdate for players, a tick event for clients, or whatever, then you can check if your key is pressed just like you did, and perform the action every tick: public void someMethodCalledEveryTick(EntityPlayer player) { // make sure you are on the client! if (player.worldObj.isRemote && YourKeyClass.keys[PROTOJUMP].getIsKeyPressed()) { // jump code } } -
Hi again, This issue was quite confusing to me as well, to be honest. In fact, I was under the impression that global entity IDs were not supposed to be used at all, and only to use registerModEntity. registerModEntity can take any ID, as the IDs are associated with your mod specifically, so I just start at zero and iterate up. This is what is used to track your entity. All mod entities should be registered this way, including projectiles, mobs, item entities, etc. Global entity ID is only necessary when registering an entity with a spawn egg, and needs to get a unique global entity ID from the EntityRegistry, otherwise your spawn eggs may conflict with those added by other mods. So if your entity is going to have a spawn egg, you need to register it globally, in addition to the registerModEntity. That's at least how I've come to understand it after working with it some, but the issue is far from 100% clear even after looking through Forge and what little documentation is available. If anyone who knows with absolute certainty can corroborate or correct my experiences, that would be wonderful. Cheers, coolAlias