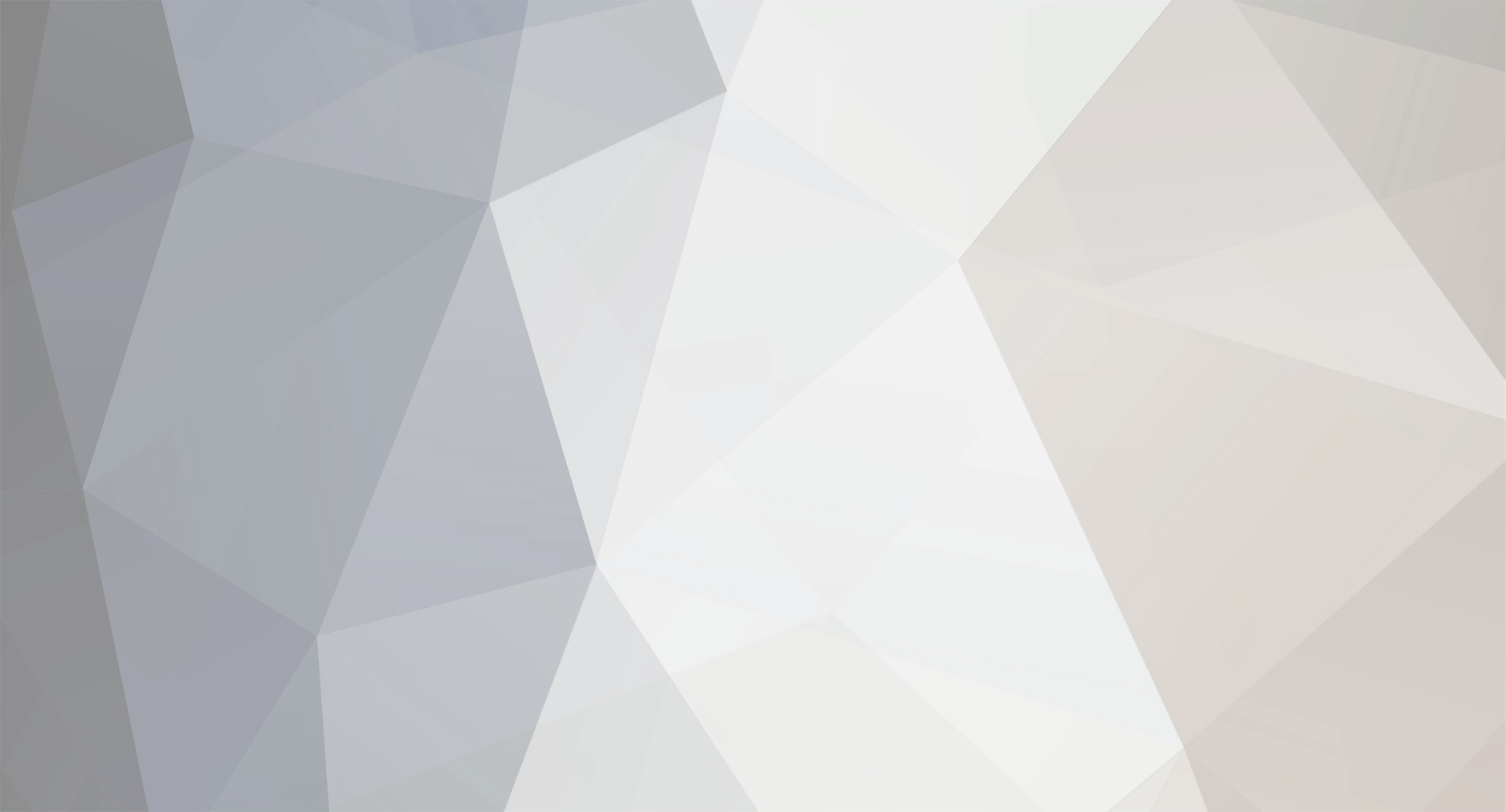
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[SOLVED] [1.7.2] Rendering bow in 3rd person
coolAlias replied to JimiIT92's topic in Modder Support
EDIT: Damn, beaten to it by SanAndreasP EDIT 2: Here's my FOVUpdateEvent that I used for my own custom bow, as well as various FOV-modifying gear: Hope that helps. FOV zoom is handled in EntityPlayerSP (so you can snag the code from there for the appropriate algorithm), and there is an FOVUpdateEvent from Forge that you can use to calculate FOV when using your custom bow. -
Make something happen when the game ticks (ITickHandler Replacement)
coolAlias replied to dude22072's topic in Modder Support
Better yet, remove your tick event / handler altogether and use the Item onArmorTick method, which is called every tick for custom armor while worn. @Override public void onArmorTick(World world, EntityPlayer player, ItemStack stack) { if (this == LOZmod.healingStone && stack.getItemDamage() > 0 etc.) { // heal } } -
It needs to be in a "sound" directory: forge/mcp/src/assets/modmud/sound/mud_chucker/hurt.ogg
-
Same way you spawn an entity anywhere else. AbstractPacket's handleServerSide method has an EntityPlayer parameter, so you can use that to get the world object, create your entity as normal, then player.worldObj.spawnEntityInWorld(yourEntity).
-
Verify Renderer, Tessalator and RenderEntity question
coolAlias replied to navybofus's topic in Modder Support
Whenever you're not sure if a method is being called, that's an excellent time to use either break points or a simple println. If it doesn't print, it's not getting called and you should check that your block implements ITileEntityProvider (or extends BlockContainer) and returns your tile entity for the create new tile entity method. If it does print, then the problem is with your rendering. Btw, if you want an item to float and rotate above the block, why not use an EntityItem directly in the render class (not for spawning in the world, but only for rendering) ? I think that could greatly simplify the work you need to do. -
Look at your error log, it tells you exactly what and where the problem is, null pointer on line 59 of your model class. Shape7.mirror = true; Shape7 = new ModelRenderer(this, 0, 20); How can you set Shape7's mirror state when it does not yet exist?
-
Have you looked at TileEntitySkullRenderer in net.minecraft.client.renderer.tileentity? You could probably use nearly that exact code for rendering a model head in your Gui.
-
Keep in mind that keys / mouse are only available CLIENT side, so you must send a packet to the server letting it know what the key states are or what to do, and then let your tick handler go from there. One option to keep the code in your Item class is to use the onUpdate method that maps use, as that's called every tick while the item is in inventory whether or not it is in use or held. You will still need to send packets if you don't use the vanilla system for using items, and set a custom using item flag either in your player's IExtendedEntityProperties or the ItemStack's NBT. The former is probably best, especially if you plan on having other items with a similar mechanic. @Override public void onUpdate(ItemStack stack, World world, Entity entity, int slot, boolean isHeld) { // check if the item is held, if entity is a player and "isCustomItemInUse" or whatever // then do what you want with no move slowdown, since the item is not in use as far as vanilla is concerned }
-
How to make a weapon [gun] fire faster than default speed?
coolAlias replied to HappleAcks's topic in Modder Support
Note that you cannot check mouse and keyboard states on the server, and they are thus not suitable for spawning entities without sending packets, since entities must spawn on the server. Besides, the using item method already IS a tick handler, being called every single tick while the item is in use, and should be the preferred method if you want a fast rate of fire (20 bullets per second, about). On right click, you need to set your item in use and make sure to give it a max item use duration, then in the update tick spawn an entity every tick, every other tick, or however many ticks you want. @Override public void onUsingTick(ItemStack stack, EntityPlayer player, int count) { // count starts at the max duration and counts down int ticksInUse = getMaxItemUseDuration(stack) - count; // this will fire every 2 ticks; change or remove to suit your needs if (ticksInUse % 2 == 0) { // consume bullet and spawn projectile on server } } If you want faster than one bullet per tick, you can use a render tick handler/event and send a packet to the server each render tick to consume and spawn the bullet, but I think 20 per second should be plenty fast. -
We need more information to help you: what is your full file path to the sound? Do you get an error message in the console or a crash when you try to play the sound and it cannot be found, and what does that say? Are you using only one sound file, or are there multiple "hurt" sounds? If you have multiple sound files named "hurt1.ogg", "hurt2.ogg", etc., you must add them all during the sound load event: for (int i = 1; i <= number_of_sounds; ++i) { event.manager.soundPoolSound.addSound("modmud:hurt" + i + ".ogg"); } And play it normally, using "modmud.hurt" without any number on it.
-
Er, heh, totally derped on that one, sorry. Yeah, the slash was in the right direction originally. Well, I don't know how to register sub-foldered sounds in 1.6.4, but in 1.7.2, I use the sound names without specifying directory (note that 1.7.2 doesn't register sounds, but I was using the same format in 1.6.4 without subfolders): event.manager.addSound("modmud:hurt.ogg"); // use the same string for playing the sound Sorry if that's a bit confusing. If that doesn't work, I suggest trying to get your sound to work just in the base "assets/modid/sounds/" directory first, and then go from there. I never used subfolders for my sounds in 1.6.4, but they worked fine using the exact same format as I showed above when I ported to 1.7.2 and DID put them in subfolders.
-
It looks to me like your slash is backwards, based on how texture paths and such are written. Try: "modmud:mud_chucker\hurt.ogg"
-
Firstly, you should always check if the stack in the slot is null before doing anything with it, or you will get a null pointer exception. Secondly, an ItemStack will never return true when comparing it to a Block with equals; instead, you need to get the Item and compare that to the ItemBlock: ItemStack stack = getStackInSlot(2); // or whatever slot if (stack != null && stack.getItem() == Item.getItemFromBlock(Blocks.cobblestone)) { // now you know for sure you've got a stack with cobblestone } Finally, you should look at the Slot class for methods you can use to limit what goes into a slot; you will need to make your own class that extends Slot and add that instead of the generic Slot to your container.
-
What Godis_apan said, and also if you setFull3D() in your Item class, it should do all the rotating for you automatically, at least how I've set it up in my render class that I linked earlier.
-
Render type EQUIPPED is for 3rd person, and EQUIPPED_FIRST_PERSON is for 1st person (which I'm guessing is where you noticed it's invisible). You need to account for both cases in your render class. If you need a further example, take a look at my RenderBigItem class that I used for my hammers and big swords.
-
How to tell when a piece of armor is unequipped?
coolAlias replied to AskHow1248's topic in Modder Support
Yes, that's true, unless you also check for that other method. It gets tricky when a bunch of mods all add flying stuff, and then disable it, which is why you should enable flying every tick while your conditions are true, and only set it to false once if you can. An example would be to set a flag in your extended properties that says "something is letting me fly" each tick while you are flying, then check that flag is true and your flying conditions are false to disable flying, and set that flag to false. This way, you only disable your particular flying that one time, so if another mod is adding flying each tick, you are not constantly disabling their flying, too. -
How to tell when a piece of armor is unequipped?
coolAlias replied to AskHow1248's topic in Modder Support
What he means is that the else statement cannot be called if you are not wearing the armor, because the armor is no longer ticking. @OP The only way I know of to do this is to check in one of the player on update methods if they can fly and are no longer wearing the correct armor, then set allow flying and is flying to false (if not in creative ); I typically use LivingUpdateEvent since I have various extended properties to update anyway. -
That's because it takes an Item, not a Class. Just look at the method arguments and you will see that.
-
[1.6.4] Best practices for organizing code for a mod?
coolAlias replied to keybounce's topic in Modder Support
Yes, you can switch between 965 and 964 without any problems. In fact, they are exactly the same except for the fact that 964 supports Gradle, whereas 965 does not. Whether you do or do not use Gradle has absolutely no impact on your end users. -
[1.6.4] Best practices for organizing code for a mod?
coolAlias replied to keybounce's topic in Modder Support
Gradle has nothing to do with the mods per se, it's just a more convenient way of organizing / setting up your workspace. For 1.6.4, you need Forge 9.11.1.964 in order to use Gradle (which I highly recommend). If you have an existing mod, the easiest way to switch to Gradle is to follow Lex's tutorial to create an empty example mod folder, then delete the example mod and just copy your existing source code into the /src/main/java/YourCode folder, and assets into /src/main/resources/assets/your_mod_id/Folders folder. Once you've done that, import it into Eclipse as an existing project and it should work great (make sure to change the values in the build.gradle file for when you export your mod). Rinse and repeat for however many mods you have, and there you go, you've got them all in the same workspace, but as independent projects that you can run one at a time in your debug client - that's a huge step above the mess that was working with MCP and having all your projects in the same source directory, at least in my opinion -
private float scale; // default value is zero... // uh oh... GL11.glScalef(scale * 0.5F, scale * 0.5F, scale * 0.5F); You need to initialize your variable.
-
In 1.6.4, you can use either getCurrentArmor(armor slot) or getCurrentItemOrArmor(armor slot minus 1), with the first method available only to EntityPlayer, and the second to all EntityLivingBase. Otherwise, the previous answer is correct. I suggest you also to check first if the stack is not null, otherwise accessing even .itemID will crash; and second I suggest using getItem() == YourMod.yourItem rather than itemID, as then your mod will be easier to update to 1.7.2 if you ever decide to do so. ItemStack boots = player.getCurrentArmor(0); // legs = 1, chest = 2, helm = 3 if (boots != null && boots.getItem() == YourMod.yourBootsItem && (same checks for legs, chest, helm)) { // you've got your full set }
-
MinecraftServer.getServer() returns null! [Help]
coolAlias replied to Nimolo's topic in Modder Support
Keys are handled client side, thus getting the server will be null. -
As far as I am aware, you get that "bouncing" animation every time the damage value changes. I personally don't know any way around that, which is why I always use NBT to track these kinds of values, as NBT can update without triggering the animation.