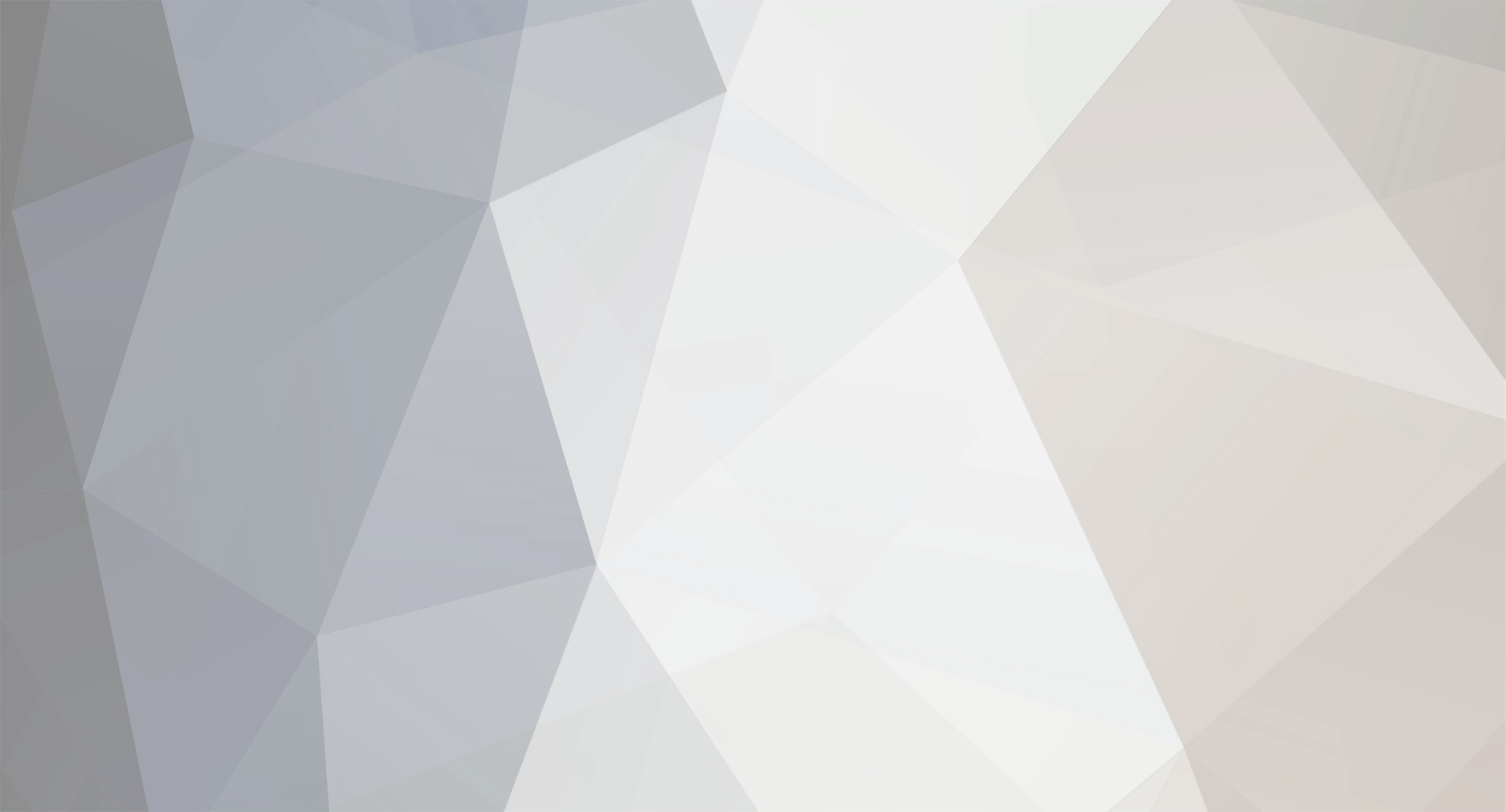
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Java "pass-by-reference" and private variables question
coolAlias replied to jabelar's topic in Modder Support
Heh, that is indeed interesting I thought you were concerned about someone else changing your mod info or something, rather than just looking for a way to get rid of the mcmod.info file. Thanks for sharing your findings! -
Java "pass-by-reference" and private variables question
coolAlias replied to jabelar's topic in Modder Support
Pointers are equally as dangerous, since they give you access to the same memory location you can just as readily alter supposedly private data. In C++, if you give me a pointer to a List, whether by reference or value, I can now change your List just the same as the Java example I showed you. If you pass me the pointer by reference, I can not only change your list, but I can change where your private field points! In Java, the second (and far more dangerous of the two), is not possible. In Java, therefore, you can not actually change the private field, you can only change data that it contains if the field itself has methods or public fields that can be changed (like in the case of List). Anyway, you are probably overthinking this; why are you so concerned about the mod info? -
Java "pass-by-reference" and private variables question
coolAlias replied to jabelar's topic in Modder Support
The access modifier of the variable in question is irrelevant as soon as you return it. For example: public class A { private List list; public getList() { return list; } } public class B { A a = new A(); public void someMethod() { List l = a.getList(); // I can now modify the list to my heart's content, and all changes // will be reflected in the instance of "A"'s list as well (other than reassignment) l.add("I'm in a's list, too!!!"); l = new List(); l.add("I'm no longer modifying a's list"); } Now the A object 'a' has a list with one String, and the B class has a list with one String, but both strings are different. Whenever you return a field from a class, if that field is an Object with modifiable fields, you have just opened up your private field to modification of this sort. -
Java "pass-by-reference" and private variables question
coolAlias replied to jabelar's topic in Modder Support
Hi, The answer is both yes and no See http://stackoverflow.com/questions/40480/is-java-pass-by-reference for a good description of how Java passes even references by value. While you cannot change what the variable in the other location refers to (you cannot reassign it), as long it is not a primitive type you CAN change the values that it holds. For example, if the method returned a private list, I can clear the list and it will be cleared in the other class as well, but if I reassign the list to a new list, the old list and variable holding the list in the other class are not affected. Hope that helps, coolAlias -
[1.7.2]Any key holding method? or any suggestion on how to do this?
coolAlias replied to lorizz's topic in Modder Support
Here ya go: https://github.com/coolAlias/Tutorial-1.7.2/blob/master/src/main/java/tutorial/client/KeyHandler.java There is now KeyInputEvent, and you can check if your KeyBinding or any vanilla KeyBinding is pressed or not. -
[SOLVED][1.7.2]Registering event handlers for server versus client
coolAlias replied to jabelar's topic in Modder Support
Hi, I think what the OP is getting at is that when you use proxies with FML, the ClientProxy methods ONLY run on the client side, but the CommonProxy methods are run on both. So if you have a GuiEvent class, it is easiest and trouble-free to register it in the ClientProxy, whereas if you put that in the CommonProxy or your main class, you will have to check if you are on the client side before registering it if you wish to avoid a crash in the future. There could of course be exceptions to this, such as if you create an instance of your ClientProxy or a static method and call it yourself from some random point in your code, it could very well be called on the server, but using "proxy.someMethod" during the FML events does, I believe, make that distinction for you. Please correct me if I'm wrong, but that has been my experience using proxies and what I have come to understand as their main purpose. EDIT: @jabelar A lot of things are just stylistic preference; for example, I have all my entity registration in a separate Entities class, same for Items and Blocks. You can see my main class here, if you want, but I would just do it however you like: https://github.com/coolAlias/ZeldaSwordSkills-1.6.4/blob/master/src/main/java/zeldaswordskills/ZSSMain.java -
[Solved] [1.7.4] Item positioning and Particle Questions???~~
coolAlias replied to Pandassaurus's topic in Modder Support
Excellent report there, SanAndreasP. Seems like registering the entity is required for it to spawn correctly on the client side, then, like all other entities. Thanks for sharing! Ah, I thought the OP meant when the item was dropped, not while held in the player's hand. While held in the player's hand, you can spawn particles using the Item onUpdate method; the last parameter is true when the item is held. Since you know that the item is in the player's hand, you can use the player's position as the base for spawning whatever particles you want, and play around with the position and motion until it looks right; if it's important to have the particles trail the item's swing motion, you can get the swing progress from the player as well (note this stuff should all be client-side only). If you want other players to be able to see the particles, too, you will have to figure out a way of sending a packet to nearby players with the appropriate information. One way I did this was to create an interface for Items that can spawn particles, and a custom packet that calls that method with the information provided: Interface: https://github.com/coolAlias/ZeldaSwordSkills-1.6.4/blob/master/src/main/java/zeldaswordskills/item/ISpawnParticles.java Packet: https://github.com/coolAlias/ZeldaSwordSkills-1.6.4/blob/master/src/main/java/zeldaswordskills/network/PacketISpawnParticles.java You could easily adapt that to include swing progress or other information you may need. -
[Solved] [1.7.4] Item positioning and Particle Questions???~~
coolAlias replied to Pandassaurus's topic in Modder Support
This is really interesting. So why is it that other custom entities then have the correct class on the client side? Would it work if the custom EntityItem was registered as a mod entity, like custom mobs, projectiles, etc? Or is there something else at work here? -
[Solved] [1.7.4] Item positioning and Particle Questions???~~
coolAlias replied to Pandassaurus's topic in Modder Support
I haven't personally tried it with EntityItem, but I thought every subclass of Entity has a client-side version that spawns and gets updates client-side - this is how arrows and such spawn particles when critical; are EntityItems an exception to this? Though the method you mentioned will do the trick without creating a new class, so that's a better solution anyway -
[Solved] [1.7.4] Item positioning and Particle Questions???~~
coolAlias replied to Pandassaurus's topic in Modder Support
All items turn into EntityItems when dropped; you can have your special item return a custom EntityItem (read "SomeClass extends EntityItem"). Just like every other entity, EntityItem has its onUpdate method called every tick, so you can then use to check for fire and spawn particles based on its current position and motion. Method in Item class: /** * This function should return a new entity to replace the dropped item. * Returning null here will not kill the EntityItem and will leave it to function normally. * Called when the item it placed in a world. * * @param world The world object * @param location The EntityItem object, useful for getting the position of the entity * @param itemstack The current item stack * @return A new Entity object to spawn or null */ public Entity createEntity(World world, Entity location, ItemStack itemstack) { return null; } Basically you spawn your custom EntityItem class at the same location and with the same ItemStack as the original EntityItem (somewhat poorly named as "location"), making sure to kill the original EntityItem so you don't end up spawning two. -
Did you pass "YourMod.instance" when opening the GUI? Did you make an IGuiHandler class? Does your IGuiHandler return the correct Gui Elements on both the client and the server? Did you put the @NetworkMod and all other necessary annotations in your main class? @Mod(modid = "your_mod_id", name = "Readable Mod Name", version = "1.0") @NetworkMod(clientSideRequired=true, serverSideRequired=true) public class YourMod { @Instance("your_mod_id") public static YourMod instance; @SidedProxy(clientSide = "client_proxy_package", serverSide = "common_proxy_package") public static CommonProxy proxy; // Initialization events } If none of those help you, please post your code.
-
[SOLVED] Changing eye height / camera render viewpoint
coolAlias replied to coolAlias's topic in Modder Support
Alright, I've gotten a solution from Noppes that I've modified to suit my needs. It involves extending EntityRenderer and switching to the alternate one when needed, and after changing some stuff I got it to return a correct mouse over object as well. For those who may be interested in this particular solution, here is the current code. Lots of hard-coded stuff at the moment, but it works fairly well. EntityRendererAlt RenderTickEvent / Handler RenderPlayerEvent -
[1.7.2] Teleporting to where your cursor is at?
coolAlias replied to MegaGEN50's topic in Modder Support
Not to be that guy again, but you should be aware that EntityLivingBase#getPosition is CLIENT side only - you can not use this method when on a server or you will crash. You need to create your own Vec3 using the player's position coordinates: // you can add 1 to the player's posY directly while creating the vector if you want: Vec3 positionVector = world.getWorldVec3Pool().getVecFromPool(player.posX, player.posY + 1, player.posZ); Anyway, the rest of the code should work, just thought it worth mentioning (I learned this one the hard way ) -
[SOLVED] Changing eye height / camera render viewpoint
coolAlias replied to coolAlias's topic in Modder Support
@jabelar You're correct that orientCamera is where F5 (i.e. 3rd person mode) and other camera-related effects are handled, but it's useless to me without using ASM as there is no Forge hook called from within that method (other than one for sleeping, for some reason). What this means is that trying to change any of the camera values from anywhere else will not have any effect since they are all handled locally within that method. Changing the player / render view entity's actual position or yOffset does have an effect, since that can be updated at any time before the camera orientation is determined, as has been mentioned and attempted. @coolboy4531 Indeed he did, and I still need to do a more thorough study of his code and see if it's a simple solution or not. iChun's Morph mod is a real work of coding genius, to say the least I'm hoping it's not over my head lol. In other news, I found out why using a dummy render view entity screws up object mouse over / left-click: List list = this.mc.theWorld.getEntitiesWithinAABBExcludingEntity(this.mc.renderViewEntity, this.mc.renderViewEntity.boundingBox.addCoord(vec31.xCoord * d0, vec31.yCoord * d0, vec31.zCoord * d0).expand((double)f1, (double)f1, (double)f1)); Since the renderViewEntity is supposed to be the player, normally the player is excluded from the list of potential targets, but with the dummy entity, the player is included. Now it makes sense why I was getting "Invalid entity" errors - the player can't attack himself! -
It would help us help you if you posted your code. Also, by "max", I meant the maximum value your xp bar should hold, so if you need 100 kills to gain a level, max = 100, and kills is obviously the number of kills the player currently has. ((float) current_player_kills / 100F) * 50F would give you a scale of 2 kills per pixel.
-
Like SanAndreas and diesieben07 pointed out, and I forgot to mention explicitly, cast to float during division, and back to int for your value: int xp_width = (int) ((float) xp / (float) max) * 50);
-
Assuming maxXP is a float/double. If it isn't, you do an integer division and thus only return 0 until currentXP (which can be an integer) reaches maxXP. Right. I forgot to mention you need to cast your int to float when doing the division, in case you weren't already aware of that.
-
First, you need a texture to represent the full bar that will render over top of the background image, if any. Let's assume your full xp bar texture is 50 pixels in width, then you can calculate the amount to draw as: // currentXP is the current amount the player has // maxXP is the maximum amount the player can have before increasing in level int xp_width = (currentXP / maxXP) * 50; drawTexturedModalRect(xPos, yPos, texturePosX, texturePosY, xp_width, textureHeight); Basically you are drawing your texture as normal, but restricting the width to a proportional amount.
-
You shouldn't need to cut the number of sounds down to one; try removing "name", as that may be setting some flag that indicates there is only one sound. EDIT: Curly brackets within the array brackets is neither required nor correct: [ -sounds- ] is just fine, [{ -sounds- }] breaks the json file format for multiple sound names, though it seemingly works for single sounds. Here is what part of my sounds.json file looks like: "break_jar": {"category": "block","sounds":["block/ceramic1","block/ceramic2","block/ceramic3","block/ceramic4"]}, "hit_peg": {"category": "block","sounds":["block/hit_peg1","block/hit_peg2","block/hit_peg3","block/hit_peg4"]}, "hit_rusty": {"category": "block","sounds":["block/hit_rusty1","block/hit_rusty2","block/hit_rusty3"]}, "lock_chest": {"category": "block","sounds":["block/lock_chest"]}, "lock_door": {"category": "block","sounds":["block/lock_door"]}, etc.
-
Hi, The bow stance is rendered in ModelBiped, called from RenderPlayer; when the item in use has EnumAction.bow, it sets the "aimedBow" field in the player's armor, chest armor, and main biped models to true. You may be able to get away with simply doing the same (i.e. setting the value) in a Pre RenderPlayerEvent if the player is holding your item, otherwise you will probably have to snatch the actual rendering code out of ModelBiped and the armor models and try rendering those at the Post RenderPlayerEvent - on second thought, that will likely give you two models overlaying each other, in which case you may need to completely cancel the player render event and substitute it with your own rendering. Another alternative, if you are able, would be to use ASM to insert a check for your item into the RenderPlayer code - to minimize potential conflicts, you can just add it as an additional check, rather than modifying any of the currently existing code. Those are all the options I can think of, anyway
-
[RESOLVED] Alternative to TileEntity for chameleon Block?
coolAlias replied to coolAlias's topic in Modder Support
You are just too damn smart I'm actually coding the same thing for both 1.6.4 and 1.7.2 (for some masochistic reason I've been keeping both versions of my mod updated...), but otherwise this sounds like an extremely viable and efficient solution. Thanks for this!