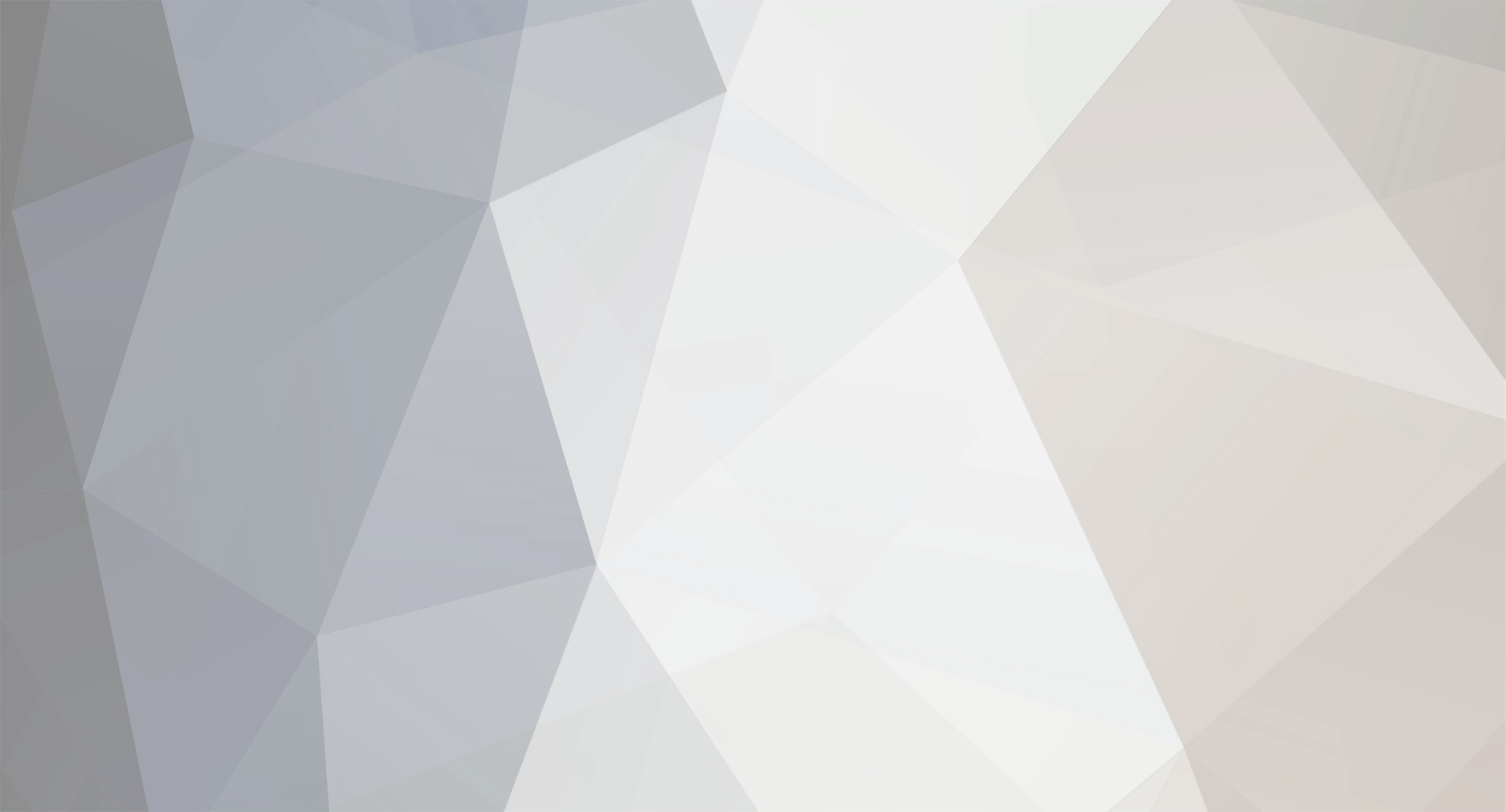
PeterRDevries
Forge Modder-
Posts
169 -
Joined
-
Last visited
Everything posted by PeterRDevries
-
Hi Somehow my Custom furnace like block isn't working i probably made a noob error or something :3 sorry container package molecularscience.electrolyzer; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.ICrafting; import net.minecraft.inventory.Slot; import net.minecraft.item.ItemStack; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class ContainerElectrolyzer extends Container { protected TileEntityElectrolyzer tileEntity; private int progress; public ContainerElectrolyzer (InventoryPlayer inventoryPlayer, TileEntityElectrolyzer te){ tileEntity = te; addSlotToContainer(new Slot(tileEntity, 0, 22, ); addSlotToContainer(new Slot(tileEntity, 1, 76, ); addSlotToContainer(new Slot(tileEntity, 2, 49, 50)); addSlotToContainer(new Slot(tileEntity, 3, 128, 29)); bindPlayerInventory(inventoryPlayer); } @Override public boolean canInteractWith(EntityPlayer player) { return tileEntity.isUseableByPlayer(player); } protected void bindPlayerInventory(InventoryPlayer inventoryPlayer) { for (int i = 0; i < 3; i++) { for (int j = 0; j < 9; j++) { addSlotToContainer(new Slot(inventoryPlayer, j + i * 9 + 9, 8 + j * 18, 99 + i * 18)); } } for (int i = 0; i < 9; i++) { addSlotToContainer(new Slot(inventoryPlayer, i, 8 + i * 18, 157)); } } @Override public ItemStack transferStackInSlot(EntityPlayer player, int slot) { ItemStack stack = null; Slot slotObject = (Slot) inventorySlots.get(slot); if (slotObject != null && slotObject.getHasStack()) { ItemStack stackInSlot = slotObject.getStack(); stack = stackInSlot.copy(); if (slot < 9) { if (!this.mergeItemStack(stackInSlot, 0, 35, true)) { return null; } } else if (!this.mergeItemStack(stackInSlot, 0, 9, false)) { return null; } if (stackInSlot.stackSize == 0) { slotObject.putStack(null); } else { slotObject.onSlotChanged(); } if (stackInSlot.stackSize == stack.stackSize) { return null; } slotObject.onPickupFromSlot(player, stackInSlot); } return stack; } public void addCraftingToCrafters(ICrafting crafting){ super.addCraftingToCrafters(crafting); crafting.sendProgressBarUpdate(this, 0, this.tileEntity.progress); } public void detectAndSendChanges(){ super.detectAndSendChanges(); for (int i = 0; i < this.crafters.size(); ++i){ ICrafting icrafting = (ICrafting)this.crafters.get(i); if (this.progress != this.tileEntity.progress){ icrafting.sendProgressBarUpdate(this, 0, this.tileEntity.progress); } } this.progress = this.tileEntity.progress; } @SideOnly(Side.CLIENT) public void updateProgressBar(int par1, int par2){ if (par1 == 0){ this.tileEntity.progress = par2; } } } TileEntity package molecularscience.electrolyzer; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.tileentity.TileEntity; public class TileEntityElectrolyzer extends TileEntity implements IInventory { private ItemStack[] inv; private int time; public int progress; private int resultItem = 3; public TileEntityElectrolyzer(){ inv = new ItemStack[4]; } @Override public int getSizeInventory() { return inv.length; } @Override public ItemStack getStackInSlot(int slot) { return inv[slot]; } @Override public void setInventorySlotContents(int slot, ItemStack stack) { inv[slot] = stack; if (stack != null && stack.stackSize > getInventoryStackLimit()) { stack.stackSize = getInventoryStackLimit(); } } @Override public ItemStack decrStackSize(int slot, int amt) { ItemStack stack = getStackInSlot(slot); if (stack != null) { if (stack.stackSize <= amt) { setInventorySlotContents(slot, null); } else { stack = stack.splitStack(amt); if (stack.stackSize == 0) { setInventorySlotContents(slot, null); } } } return stack; } @Override public void updateEntity(){ if(!worldObj.isRemote){ int item = getAvailableSlot(); if(inv[item] != null){ Item type = inv[item].getItem(); ItemStack result = ElectrolyzerResults.getResult(item, type); time = ElectrolyzerResults.getMaxTime(item, type); if(result != null){ if(progress > time){ if (inv[resultItem] == null){ inv[resultItem] = result.copy(); } else if (inv[resultItem].isItemEqual(result)){ if(inv[resultItem].stackSize + result.stackSize <= getInventoryStackLimit()){ inv[resultItem].stackSize += result.stackSize; } } if(inv[item] != null){ if(!((inv[item].stackSize - ElectrolyzerResults.getReduceItem(item, type)) < 0)){ inv[item].stackSize -= ElectrolyzerResults.getReduceItem(item, type); } if(inv[item].stackSize <= 0){ inv[item] = null; } } progress = 0; } if(inv[resultItem] != null){ if(inv[resultItem].isItemEqual(result)){ if(inv[resultItem].stackSize < getInventoryStackLimit() || inv[resultItem].stackSize < result.getItem().getItemStackLimit()){ if(inv[item] != null){ if(!((inv[item].stackSize - ElectrolyzerResults.getReduceItem(item, type)) < 0)){ if(inv[resultItem].stackSize + result.stackSize <= getInventoryStackLimit()){ progress++; } else{ progress = 0; } } else{ progress = 0; } } else{ progress = 0; } } else{ progress = 0; } } else{ progress = 0; } } else{ progress = 0; } } else{ if(inv[item] != null){ if(!((inv[item].stackSize - ElectrolyzerResults.getReduceItem(item, type)) < 0)){ progress++; } else{ progress = 0; } } else{ progress = 0; } } } else{ progress = 0; } } } @Override public ItemStack getStackInSlotOnClosing(int slot) { ItemStack stack = getStackInSlot(slot); if (stack != null) { setInventorySlotContents(slot, null); } return stack; } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return worldObj.getTileEntity(xCoord, yCoord, zCoord) == this && player.getDistanceSq(xCoord + 0.5, yCoord + 0.5, zCoord + 0.5) < 64; } public void openChest() {} public void closeChest() {} @Override public void readFromNBT(NBTTagCompound tagCompound) { super.readFromNBT(tagCompound); progress = tagCompound.getShort("progressTime"); } @Override public void writeToNBT(NBTTagCompound tagCompound) { super.writeToNBT(tagCompound); tagCompound.setShort("progressTime", (short)progress); NBTTagList itemList = new NBTTagList(); for (int i = 0; i < inv.length; i++) { ItemStack stack = inv[i]; if (stack != null) { NBTTagCompound tag = new NBTTagCompound(); tag.setByte("Slot", (byte) i); stack.writeToNBT(tag); itemList.appendTag(tag); } } tagCompound.setTag("Inventory", itemList); } @Override public String getInventoryName() { return "molecularscience.tileentityelectrolyzer"; } @SideOnly(Side.CLIENT) public int getCraftingProgressScaled(int par1){ //return this.progress * par1 / ElectrolyzerResults.getMaxTime(0, 0); return 0; } public int getAvailableSlot(){ for(int i = 0; i < inv.length - 1; i++){ if(inv[i] != null){ return i; } } return 0; } public boolean isBusy(){ return progress >= 0; } @Override public boolean hasCustomInventoryName() { // TODO Auto-generated method stub return false; } @Override public void openInventory() { // TODO Auto-generated method stub } @Override public void closeInventory() { // TODO Auto-generated method stub } @Override public boolean isItemValidForSlot(int var1, ItemStack var2) { // TODO Auto-generated method stub return false; } } Gui package molecularscience.electrolyzer; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.util.ResourceLocation; import net.minecraft.util.StatCollector; import org.lwjgl.opengl.GL11; public class GuiElectrolyzer extends GuiContainer { private static final ResourceLocation Guitexture = new ResourceLocation("molecularscience","textures/gui/container/guielectrolyzer.png"); public GuiElectrolyzer (InventoryPlayer inventoryPlayer, TileEntityElectrolyzer tileEntity) { super(new ContainerElectrolyzer(inventoryPlayer, tileEntity)); } @Override protected void drawGuiContainerForegroundLayer(int param1, int param2) { } @Override protected void drawGuiContainerBackgroundLayer(float par1, int par2, int par3) { this.mc.getTextureManager().bindTexture(Guitexture); int x = (width - xSize) / 2; int y = (height - (ySize+27)) / 2; this.drawTexturedModalRect(x, y, 0, 0, xSize, ySize+27); } } results package molecularscience.electrolyzer; import java.util.HashMap; import java.util.Map; import molecularscience.moditems.BlocksItems; import net.minecraft.block.Block; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class ElectrolyzerResults { private static Map<Integer, Object[]> results = new HashMap<Integer, Object[]>(); public static void initResult(){ addResult(0, 0, BlocksItems.Emptyjar, 1, BlocksItems.Sample, 1); } public static ItemStack getResult(int slot, Item type){ for(int i = 0; i < results.size(); i++){ if((int)results.get(i)[0] == slot){ if(results.get(i)[1] == type){ return new ItemStack((Item) results.get(i)[4], 1); } } } return null; } public static int getMaxTime(int slot, Item type){ return 50; } public static int getReduceItem(int slot, Item type){ for(int i = 0; i < results.size(); i++){ if((int)results.get(i)[0] == slot){ if(results.get(i)[1] == type){ return (int)results.get(i)[2]; } } } return 1; } private static void addResult(int id, int slot, Item type, int ammount, Item result, int size){ results.put(id, new Object[]{slot, type, ammount, result, size}); } } slot package molecularscience.electrolyzer; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.Slot; import net.minecraft.item.ItemStack; public class SlotElectrolyzer extends Slot { private TileEntityElectrolyzer teSmithingAnvil; public SlotElectrolyzer(IInventory inventory, int id, int x, int y) { super(inventory, id, x, y); this.teSmithingAnvil = (TileEntityElectrolyzer) inventory; } @Override public boolean isItemValid(ItemStack itemstack) { return teSmithingAnvil.isItemValidForSlot(slotNumber, itemstack); } } Thanks for your help
-
Items not registering - Likely a Rookie mistake
PeterRDevries replied to Minothor's topic in Modder Support
Damn diesieben i was just about to say that -
I added those 2 methods and BOOM it worked thanks alot!
-
Hmm yes i didn't do that but where would I place that percisely?
-
[1.7.2] Buckets yo!... and now an Init discussion.
PeterRDevries replied to Pandassaurus's topic in Modder Support
Doesn't your empty bucket need an onitemuse method? -
did your run Gradlew.bat eclipse?
-
Minecraft 1.7.2 does NOT have id's
-
derp lol i thought it was x y z.. but yeah............ a gui is like 2D....
-
Yep i figured that out but i don't know where
-
[18:01:04] [Client thread/FATAL]: Reported exception thrown! net.minecraft.util.ReportedException: Rendering screen at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1239) ~[EntityRenderer.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1064) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:951) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_51] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_51] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_51] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_51] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] Caused by: java.lang.ArrayIndexOutOfBoundsException: 10 at molecularscience.electrolyzer.TileEntityElectrolyzer.getStackInSlot(TileEntityElectrolyzer.java:25) ~[TileEntityElectrolyzer.class:?] at net.minecraft.inventory.Slot.getStack(Slot.java:98) ~[slot.class:?] at net.minecraft.client.gui.inventory.GuiContainer.func_146977_a(GuiContainer.java:237) ~[GuiContainer.class:?] at net.minecraft.client.gui.inventory.GuiContainer.drawScreen(GuiContainer.java:132) ~[GuiContainer.class:?] at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1209) ~[EntityRenderer.class:?] ... 9 more ---- Minecraft Crash Report ---- // Shall we play a game? Time: 3/12/14 6:01 PM Description: Rendering screen java.lang.ArrayIndexOutOfBoundsException: 10 at molecularscience.electrolyzer.TileEntityElectrolyzer.getStackInSlot(TileEntityElectrolyzer.java:25) at net.minecraft.inventory.Slot.getStack(Slot.java:98) at net.minecraft.client.gui.inventory.GuiContainer.func_146977_a(GuiContainer.java:237) at net.minecraft.client.gui.inventory.GuiContainer.drawScreen(GuiContainer.java:132) at net.minecraft.client.renderer.EntityRenderer.updateCameraAndRender(EntityRenderer.java:1209) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1064) at net.minecraft.client.Minecraft.run(Minecraft.java:951) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at molecularscience.electrolyzer.TileEntityElectrolyzer.getStackInSlot(TileEntityElectrolyzer.java:25) at net.minecraft.inventory.Slot.getStack(Slot.java:98) at net.minecraft.client.gui.inventory.GuiContainer.func_146977_a(GuiContainer.java:237) at net.minecraft.client.gui.inventory.GuiContainer.drawScreen(GuiContainer.java:132) -- Screen render details -- Details: Screen name: molecularscience.electrolyzer.GuiElectrolyzer Mouse location: Scaled: (213, 119). Absolute: (427, 240) Screen size: Scaled: (427, 240). Absolute: (854, 480). Scale factor of 2 -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityClientPlayerMP['Player393'/197, l='MpServer', x=-1741.10, y=6.05, z=-1195.09]] Chunk stats: MultiplayerChunkCache: 140, 140 Level seed: 0 Level generator: ID 01 - flat, ver 0. Features enabled: false Level generator options: Level spawn location: World: (-1630,4,-1107), Chunk: (at 2,0,13 in -102,-70; contains blocks -1632,0,-1120 to -1617,255,-1105), Region: (-4,-3; contains chunks -128,-96 to -97,-65, blocks -2048,0,-1536 to -1537,255,-1025) Level time: 130000 game time, 8189 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 93 total; [EntitySlime['Slime'/0, l='MpServer', x=-1816.11, y=4.02, z=-1243.06], EntitySlime['Slime'/1, l='MpServer', x=-1809.90, y=4.59, z=-1190.23], EntitySlime['Slime'/2, l='MpServer', x=-1815.31, y=4.00, z=-1170.22], EntityPig['Pig'/139, l='MpServer', x=-1668.97, y=4.00, z=-1234.94], EntitySlime['Slime'/138, l='MpServer', x=-1668.70, y=4.74, z=-1248.09], EntityChicken['Chicken'/4, l='MpServer', x=-1792.19, y=4.00, z=-1173.59], EntitySlime['Slime'/5, l='MpServer', x=-1805.70, y=4.83, z=-1177.90], EntityCow['Cow'/140, l='MpServer', x=-1678.69, y=4.00, z=-1241.25], EntitySlime['Slime'/6, l='MpServer', x=-1803.29, y=4.02, z=-1143.04], EntityHorse['Horse'/143, l='MpServer', x=-1671.22, y=4.00, z=-1200.91], EntitySlime['Slime'/7, l='MpServer', x=-1804.19, y=4.74, z=-1136.16], EntityCow['Cow'/142, l='MpServer', x=-1667.72, y=4.00, z=-1202.56], EntityPig['Pig'/129, l='MpServer', x=-1681.75, y=4.00, z=-1166.53], EntitySlime['Slime'/9, l='MpServer', x=-1778.76, y=4.20, z=-1260.68], EntityCow['Cow'/128, l='MpServer', x=-1688.19, y=4.00, z=-1155.13], EntitySlime['Slime'/10, l='MpServer', x=-1777.25, y=4.83, z=-1256.93], EntityCow['Cow'/130, l='MpServer', x=-1684.84, y=4.00, z=-1126.81], EntitySlime['Slime'/11, l='MpServer', x=-1791.86, y=4.00, z=-1222.87], EntitySheep['Sheep'/12, l='MpServer', x=-1778.68, y=4.00, z=-1166.67], EntitySlime['Slime'/13, l='MpServer', x=-1778.42, y=5.08, z=-1167.87], EntitySlime['Slime'/14, l='MpServer', x=-1789.78, y=4.50, z=-1143.48], EntitySlime['Slime'/15, l='MpServer', x=-1778.33, y=4.34, z=-1144.61], EntitySlime['Slime'/152, l='MpServer', x=-1672.47, y=4.78, z=-1160.82], EntitySlime['Slime'/153, l='MpServer', x=-1667.57, y=4.00, z=-1133.91], EntitySlime['Slime'/21, l='MpServer', x=-1761.77, y=4.78, z=-1227.50], EntitySlime['Slime'/158, l='MpServer', x=-1662.43, y=4.59, z=-1251.83], EntitySheep['Sheep'/23, l='MpServer', x=-1772.94, y=4.00, z=-1131.06], EntityChicken['Chicken'/22, l='MpServer', x=-1770.53, y=4.00, z=-1205.53], EntitySheep['Sheep'/144, l='MpServer', x=-1664.63, y=4.00, z=-1202.56], EntitySlime['Slime'/24, l='MpServer', x=-1768.59, y=4.20, z=-1118.56], EntityPig['Pig'/145, l='MpServer', x=-1666.28, y=4.00, z=-1202.41], EntitySlime['Slime'/27, l='MpServer', x=-1745.31, y=4.00, z=-1275.12], EntitySheep['Sheep'/146, l='MpServer', x=-1671.69, y=4.00, z=-1186.16], EntitySheep['Sheep'/147, l='MpServer', x=-1675.75, y=4.00, z=-1189.53], EntitySlime['Slime'/29, l='MpServer', x=-1751.31, y=4.50, z=-1251.57], EntitySheep['Sheep'/148, l='MpServer', x=-1665.09, y=4.00, z=-1184.97], EntitySlime['Slime'/28, l='MpServer', x=-1746.43, y=4.37, z=-1271.83], EntitySlime['Slime'/149, l='MpServer', x=-1679.94, y=4.00, z=-1189.75], EntitySlime['Slime'/150, l='MpServer', x=-1670.95, y=4.00, z=-1196.79], EntitySlime['Slime'/31, l='MpServer', x=-1749.66, y=4.50, z=-1243.72], EntitySlime['Slime'/30, l='MpServer', x=-1749.24, y=4.83, z=-1254.54], EntityChicken['Chicken'/151, l='MpServer', x=-1677.59, y=4.00, z=-1171.56], EntitySlime['Slime'/34, l='MpServer', x=-1750.59, y=4.00, z=-1227.43], EntitySlime['Slime'/35, l='MpServer', x=-1749.79, y=4.00, z=-1195.72], EntitySlime['Slime'/32, l='MpServer', x=-1754.95, y=4.20, z=-1238.79], EntityHorse['Horse'/33, l='MpServer', x=-1750.19, y=4.00, z=-1229.88], EntitySlime['Slime'/36, l='MpServer', x=-1746.82, y=4.00, z=-1184.41], EntityItem['item.item.slimeball'/37, l='MpServer', x=-1745.47, y=4.13, z=-1161.63], EntityPig['Pig'/51, l='MpServer', x=-1731.81, y=4.00, z=-1139.13], EntityItem['item.item.slimeball'/50, l='MpServer', x=-1734.69, y=4.13, z=-1164.88], EntityItem['item.item.slimeball'/49, l='MpServer', x=-1741.34, y=4.13, z=-1166.47], EntityHorse['Horse'/48, l='MpServer', x=-1733.72, y=4.00, z=-1215.91], EntityHorse['Horse'/55, l='MpServer', x=-1732.06, y=4.00, z=-1135.59], EntitySlime['Slime'/54, l='MpServer', x=-1737.40, y=4.00, z=-1146.44], EntitySlime['Slime'/53, l='MpServer', x=-1736.31, y=4.00, z=-1144.22], EntityCow['Cow'/52, l='MpServer', x=-1731.06, y=4.00, z=-1137.84], EntitySlime['Slime'/57, l='MpServer', x=-1739.74, y=4.00, z=-1136.49], EntityPig['Pig'/56, l='MpServer', x=-1738.19, y=4.00, z=-1129.84], EntitySlime['Slime'/68, l='MpServer', x=-1713.61, y=4.00, z=-1237.53], EntitySlime['Slime'/69, l='MpServer', x=-1714.09, y=4.59, z=-1223.47], EntityHorse['Horse'/70, l='MpServer', x=-1715.16, y=4.00, z=-1213.97], EntityCow['Cow'/71, l='MpServer', x=-1717.22, y=4.00, z=-1192.75], EntitySlime['Slime'/7945, l='MpServer', x=-1705.50, y=4.00, z=-1247.50], EntitySlime['Slime'/66, l='MpServer', x=-1724.59, y=4.00, z=-1268.14], EntityHorse['Horse'/67, l='MpServer', x=-1712.53, y=4.00, z=-1245.22], EntitySlime['Slime'/76, l='MpServer', x=-1714.65, y=4.00, z=-1169.78], EntityClientPlayerMP['Player393'/197, l='MpServer', x=-1741.10, y=6.05, z=-1195.09], EntitySlime['Slime'/77, l='MpServer', x=-1726.90, y=4.02, z=-1180.52], EntityChicken['Chicken'/78, l='MpServer', x=-1718.53, y=4.00, z=-1163.44], EntityHorse['Horse'/79, l='MpServer', x=-1722.94, y=4.00, z=-1128.00], EntityPig['Pig'/72, l='MpServer', x=-1723.25, y=4.00, z=-1199.16], EntityHorse['Horse'/73, l='MpServer', x=-1723.94, y=4.00, z=-1169.94], EntityPig['Pig'/74, l='MpServer', x=-1719.92, y=4.00, z=-1182.04], EntitySlime['Slime'/75, l='MpServer', x=-1721.21, y=4.00, z=-1179.60], EntitySlime['Slime'/80, l='MpServer', x=-1721.24, y=4.00, z=-1134.90], EntityCow['Cow'/102, l='MpServer', x=-1699.25, y=4.00, z=-1190.31], EntityPig['Pig'/103, l='MpServer', x=-1703.06, y=4.00, z=-1186.41], EntitySlime['Slime'/100, l='MpServer', x=-1705.93, y=4.81, z=-1232.20], EntityPig['Pig'/101, l='MpServer', x=-1699.13, y=4.00, z=-1191.81], EntitySlime['Slime'/99, l='MpServer', x=-1697.74, y=4.20, z=-1250.48], EntityChicken['Chicken'/106, l='MpServer', x=-1701.56, y=4.00, z=-1123.56], EntityHorse['Horse'/107, l='MpServer', x=-1706.88, y=4.00, z=-1122.94], EntitySlime['Slime'/104, l='MpServer', x=-1709.13, y=5.20, z=-1175.77], EntityHorse['Horse'/105, l='MpServer', x=-1711.16, y=4.00, z=-1179.16], EntitySlime['Slime'/119, l='MpServer', x=-1680.57, y=4.00, z=-1230.52], EntityHorse['Horse'/127, l='MpServer', x=-1685.97, y=4.00, z=-1182.81], EntitySlime['Slime'/126, l='MpServer', x=-1686.38, y=2.00, z=-1173.38], EntitySlime['Slime'/125, l='MpServer', x=-1687.98, y=2.00, z=-1171.86], EntityCow['Cow'/124, l='MpServer', x=-1693.09, y=4.00, z=-1188.03], EntityHorse['Horse'/123, l='MpServer', x=-1681.16, y=4.00, z=-1188.44], EntityCow['Cow'/122, l='MpServer', x=-1695.34, y=4.00, z=-1211.44], EntitySlime['Slime'/121, l='MpServer', x=-1695.15, y=4.00, z=-1215.65], EntitySlime['Slime'/120, l='MpServer', x=-1695.42, y=4.00, z=-1232.33]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:418) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2557) at net.minecraft.client.Minecraft.run(Minecraft.java:972) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) -- System Details -- Details: Minecraft Version: 1.7.2 Operating System: Windows 8 (amd64) version 6.2 Java Version: 1.7.0_51, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 677886104 bytes (646 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M AABB Pool Size: 20006 (1120336 bytes; 1 MB) allocated, 1862 (104272 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v9.01-pre FML v7.2.116.1024 Minecraft Forge 10.12.0.1024 4 mods loaded, 4 mods active mcp{8.09} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available FML{7.2.116.1024} [Forge Mod Loader] (forgeSrc-1.7.2-10.12.0.1024.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Forge{10.12.0.1024} [Minecraft Forge] (forgeSrc-1.7.2-10.12.0.1024.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available MolecularScience{1.0.0} [MolecularScience] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Launched Version: 1.6 LWJGL: 2.9.0 OpenGL: AMD Radeon HD 6670 GL version 4.3.12618 Compatibility Profile Context 13.251.0.0, ATI Technologies Inc. Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: English (US) Profiler Position: N/A (disabled) Vec3 Pool Size: 1906 (106736 bytes; 0 MB) allocated, 776 (43456 bytes; 0 MB) used Anisotropic Filtering: Off (1) #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\Stijn\Desktop\forge\eclipse\.\crash-reports\crash-2014-03-12_18.01.04-client.txt AL lib: (EE) alc_cleanup: 1 device not closed
-
Hello forge, I'm sorry but I have 00000000.1% experience in containers could anyone help me? error: -- Head -- Stacktrace: at molecularscience.electrolyzer.TileEntityElectrolyzer.getStackInSlot(TileEntityElectrolyzer.java:25) at net.minecraft.inventory.Slot.getStack(Slot.java:98) at net.minecraft.client.gui.inventory.GuiContainer.func_146977_a(GuiContainer.java:237) at net.minecraft.client.gui.inventory.GuiContainer.drawScreen(GuiContainer.java:132) tilentity package molecularscience.electrolyzer; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.tileentity.TileEntity; public class TileEntityElectrolyzer extends TileEntity implements IInventory { private ItemStack[] inv; public TileEntityElectrolyzer(){ inv = new ItemStack[1]; } @Override public int getSizeInventory() { return inv.length; } @Override public ItemStack getStackInSlot(int slot) { return inv[slot]; } @Override public void setInventorySlotContents(int slot, ItemStack stack) { inv[slot] = stack; if (stack != null && stack.stackSize > getInventoryStackLimit()) { stack.stackSize = getInventoryStackLimit(); } } @Override public ItemStack decrStackSize(int slot, int amt) { ItemStack stack = getStackInSlot(slot); if (stack != null) { if (stack.stackSize <= amt) { setInventorySlotContents(slot, null); } else { stack = stack.splitStack(amt); if (stack.stackSize == 0) { setInventorySlotContents(slot, null); } } } return stack; } @Override public ItemStack getStackInSlotOnClosing(int slot) { ItemStack stack = getStackInSlot(slot); if (stack != null) { setInventorySlotContents(slot, null); } return stack; } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return worldObj.getTileEntity(xCoord, yCoord, zCoord) == this && player.getDistanceSq(xCoord + 0.5, yCoord + 0.5, zCoord + 0.5) < 64; } public void openChest() {} public void closeChest() {} @Override public void readFromNBT(NBTTagCompound tagCompound) { super.readFromNBT(tagCompound); } @Override public void writeToNBT(NBTTagCompound tagCompound) { super.writeToNBT(tagCompound); NBTTagList itemList = new NBTTagList(); for (int i = 0; i < inv.length; i++) { ItemStack stack = inv[i]; if (stack != null) { NBTTagCompound tag = new NBTTagCompound(); tag.setByte("Slot", (byte) i); stack.writeToNBT(tag); itemList.appendTag(tag); } } tagCompound.setTag("Inventory", itemList); } @Override public String getInventoryName() { return "molecularscience.tileentityelectrolyzer"; } @Override public boolean hasCustomInventoryName() { // TODO Auto-generated method stub return false; } @Override public void openInventory() { // TODO Auto-generated method stub } @Override public void closeInventory() { // TODO Auto-generated method stub } @Override public boolean isItemValidForSlot(int var1, ItemStack var2) { // TODO Auto-generated method stub return false; } } container package molecularscience.electrolyzer; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.Slot; import net.minecraft.item.ItemStack; public class ContainerElectrolyzer extends Container { protected TileEntityElectrolyzer tileEntity; public ContainerElectrolyzer (InventoryPlayer inventoryPlayer, TileEntityElectrolyzer te){ tileEntity = te; addSlotToContainer(new Slot(tileEntity, 10, 10, 10)); bindPlayerInventory(inventoryPlayer); } @Override public boolean canInteractWith(EntityPlayer player) { return tileEntity.isUseableByPlayer(player); } protected void bindPlayerInventory(InventoryPlayer inventoryPlayer) { for (int i = 0; i < 3; i++) { for (int j = 0; j < 9; j++) { addSlotToContainer(new Slot(inventoryPlayer, j + i * 9 + 9, 8 + j * 18, 84 + i * 18)); } } for (int i = 0; i < 9; i++) { addSlotToContainer(new Slot(inventoryPlayer, i, 8 + i * 18, 142)); } } @Override public ItemStack transferStackInSlot(EntityPlayer player, int slot) { ItemStack stack = null; Slot slotObject = (Slot) inventorySlots.get(slot); if (slotObject != null && slotObject.getHasStack()) { ItemStack stackInSlot = slotObject.getStack(); stack = stackInSlot.copy(); if (slot < 9) { if (!this.mergeItemStack(stackInSlot, 0, 35, true)) { return null; } } else if (!this.mergeItemStack(stackInSlot, 0, 9, false)) { return null; } if (stackInSlot.stackSize == 0) { slotObject.putStack(null); } else { slotObject.onSlotChanged(); } if (stackInSlot.stackSize == stack.stackSize) { return null; } slotObject.onPickupFromSlot(player, stackInSlot); } return stack; } } Block package molecularscience.electrolyzer; import java.util.Random; import molecularscience.MolecularScience; import net.minecraft.block.Block; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.item.EntityItem; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; public class BlockElectrolyzer extends BlockContainer { public BlockElectrolyzer () { super(Material.wood); setHardness(2.0F); setResistance(5.0F); setBlockName("blockTiny"); setCreativeTab(CreativeTabs.tabDecorations); } @Override public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int metadata, float what, float these, float are) { TileEntity tileEntity = world.getTileEntity(x, y, z); if (tileEntity == null || player.isSneaking()) { return false; } //code to open gui explained later player.openGui(MolecularScience.instance, 0, world, x, y, z); return true; } public void breakBlock(World world, int x, int y, int z, Block par5, int par6) { dropItems(world, x, y, z); super.breakBlock(world, x, y, z, par5, par6); } private void dropItems(World world, int x, int y, int z){ Random rand = new Random(); TileEntity tileEntity = world.getTileEntity(x, y, z); if (!(tileEntity instanceof IInventory)) { return; } IInventory inventory = (IInventory) tileEntity; for (int i = 0; i < inventory.getSizeInventory(); i++) { ItemStack item = inventory.getStackInSlot(i); if (item != null && item.stackSize > 0) { float rx = rand.nextFloat() * 0.8F + 0.1F; float ry = rand.nextFloat() * 0.8F + 0.1F; float rz = rand.nextFloat() * 0.8F + 0.1F; EntityItem entityItem = new EntityItem(world, x + rx, y + ry, z + rz, new ItemStack(item.getItem(), item.stackSize, item.getItemDamage())); if (item.hasTagCompound()) { entityItem.getEntityItem().setTagCompound((NBTTagCompound) item.getTagCompound().copy()); } float factor = 0.05F; entityItem.motionX = rand.nextGaussian() * factor; entityItem.motionY = rand.nextGaussian() * factor + 0.2F; entityItem.motionZ = rand.nextGaussian() * factor; world.spawnEntityInWorld(entityItem); item.stackSize = 0; } } } @Override public TileEntity createNewTileEntity(World var1, int var2) { return new TileEntityElectrolyzer(); } @Override public int getRenderType() { return -1; } //It's not an opaque cube, so you need this. @Override public boolean isOpaqueCube() { return false; } //It's not a normal block, so you need this too. public boolean renderAsNormalBlock() { return false; } } I know why this error is there but I don't know how to fix it
-
really? public boolean canPlaceBlockAt(World par1World, int par2, int par3, int par4) { return true; }
-
[1.7.2] cancel crafting event when crafting specefic item
PeterRDevries replied to dark2222's topic in Modder Support
love you for this post +1000 -
hmm somehow the nbt data is still lost this is my tile entity public class TileEntityHeatConductant extends TileEntity{ double Temperature = 21; int color = 0x555555; @Override public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); nbt.setDouble("Temperature", Temperature); nbt.setInteger("Color", color); } @Override public void readFromNBT(NBTTagCompound nbt) { super.readFromNBT(nbt); this.Temperature = nbt.getDouble("Temperature"); this.color = nbt.getInteger("Color"); } @Override public boolean canUpdate(){ return true; } public int getColor(){ int intcolor = 0; int green = (int) ((64.0 / 700.0) * this.Temperature); int red = (int) ((255.0 / 700.0) * this.Temperature); return ((green*256) + (red*65536)); } @Override public void updateEntity() { checkblocks(worldObj.getBlock(xCoord+1, yCoord, zCoord).getLocalizedName(), "1 0 0"); checkblocks(worldObj.getBlock(xCoord-1, yCoord, zCoord).getLocalizedName(), "-1 0 0"); checkblocks(worldObj.getBlock(xCoord, yCoord+1, zCoord).getLocalizedName(), "0 1 0"); checkblocks(worldObj.getBlock(xCoord, yCoord-1, zCoord).getLocalizedName(), "0 -1 0"); checkblocks(worldObj.getBlock(xCoord, yCoord, zCoord+1).getLocalizedName(), "0 0 1"); checkblocks(worldObj.getBlock(xCoord, yCoord, zCoord-1).getLocalizedName(), "0 0 -1"); worldObj.scheduleBlockUpdate(xCoord, yCoord, zCoord, this.getBlockType(), 1); this.color = getColor(); this.worldObj.markBlockForUpdate(xCoord, yCoord, zCoord); } public void checkblocks(String block, String coord){ boolean aircool = true; String[] coords = coord.split(" "); if(block.equals("Fire")){ if(Temperature <= 600.0){ Temperature = Temperature + 0.1; } aircool = false; } if(block.equals("Lava")){ if(Temperature <= 700.0){ Temperature = Temperature + 0.5; }else{ } aircool = false; } if(block.equals("Water")){ if(Temperature >= 10.0){ Temperature = Temperature - 20; if(Temperature >= 100){ //worldObj.setBlockToAir(xCoord + Integer.parseInt(coords[0]), yCoord + Integer.parseInt(coords[1]), zCoord + Integer.parseInt(coords[2])); } } aircool = false; } if(block.contains("HeatConductant")){ int gettiletemp = 0; TileEntityHeatConductant tile = (TileEntityHeatConductant) worldObj.getTileEntity(xCoord + Integer.parseInt(coords[0]), yCoord + Integer.parseInt(coords[1]), zCoord + Integer.parseInt(coords[2])); if (tile != null) { gettiletemp = (int) tile.Temperature; } if(gettiletemp >= this.Temperature){ int conductiveness = 0; String[] test = this.getBlockType().getLocalizedName().split(" "); if(test[0].equals("Fast")){ conductiveness = 5; } double add = gettiletemp - this.Temperature; add = add / conductiveness; Temperature = Temperature + add; } aircool = false; } if(aircool == true){ if(Temperature >= 20){ Temperature = Temperature - 0.3; } } }
-
Ah well thats wierd atleast thanks for the help I would have never figured that out. Would you know how I can get the block the tileentity is connected to? EDIT: Stupid me this.getblocktype(); :3
-
Even just 1 int?
-
[1.7.2] cancel crafting event when crafting specefic item
PeterRDevries replied to dark2222's topic in Modder Support
Removing a recipe is easy CraftingManager.getInstance().getRecipeList().removeAt(); only removing a recipe per player is hard. -
[1.7.2] cancel crafting event when crafting specefic item
PeterRDevries replied to dark2222's topic in Modder Support
https://github.com/riking/neiplugin-thaumcraft/blob/master/src/minecraft/thaumcraft/common/ThaumcraftCraftingManager.java -
How to find an empty place in the nether?
PeterRDevries replied to Bedrock_Miner's topic in Modder Support
yep youre right it i mistook the y for z coord -
How to find an empty place in the nether?
PeterRDevries replied to Bedrock_Miner's topic in Modder Support
try and use a forloop to check if getblock(xcoord ycoord zcoord+var) = air -
unassigned blocks and items in save
PeterRDevries replied to OniNoSeishin's topic in Support & Bug Reports
let "it deal with it himself" Your'e getting this error because there where items with ids of the mod your removed. The id's are still present in that world but have no use so youd have to remove them -
great