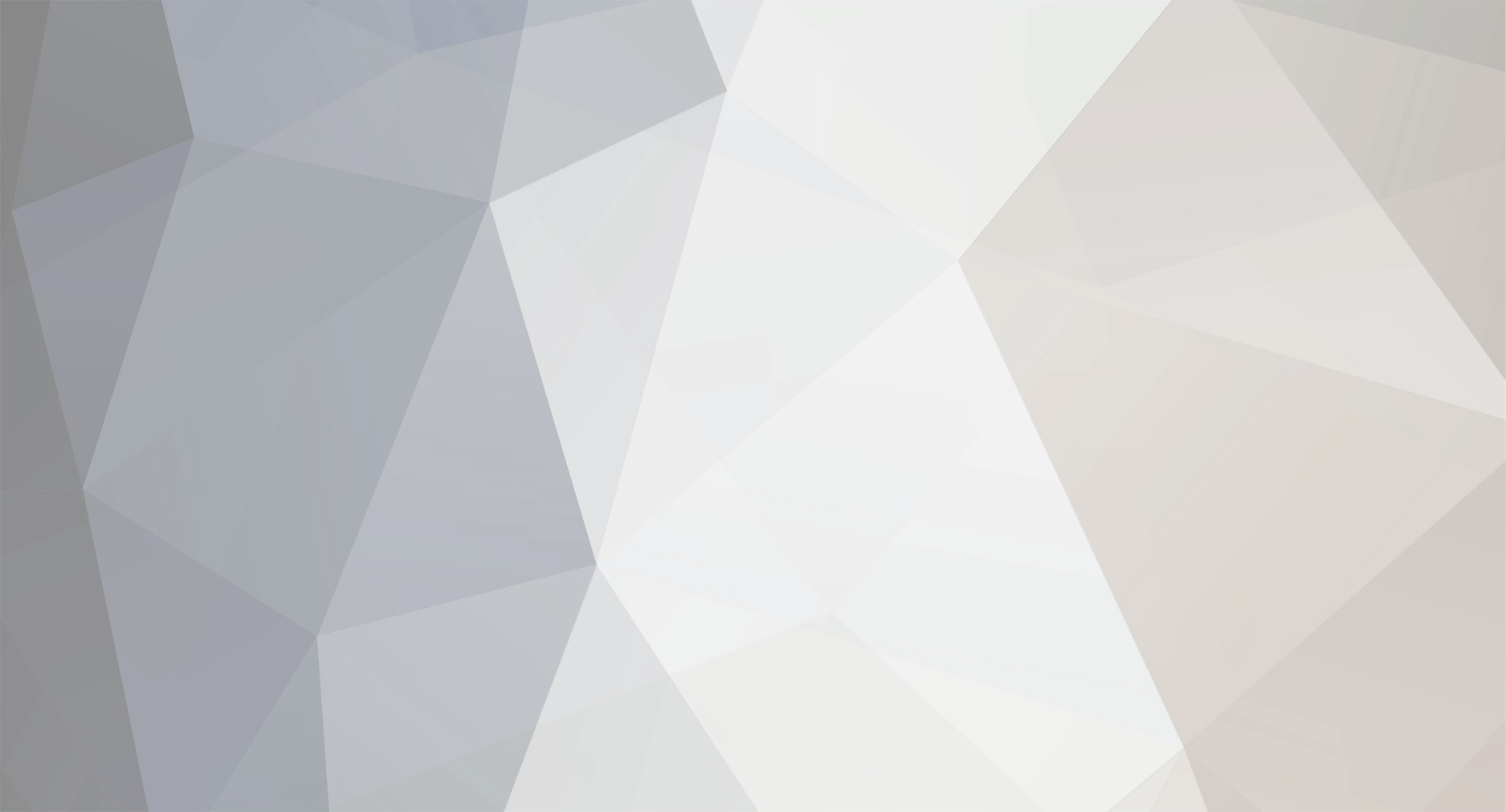
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
Well again usually in programming the problem is very specific mistake, like maybe you didn't call exactly the right method. So you really need to check absolutely everything. Like after you add velocity did the motion values change, and so forth. If you're really stuck on this method, then I suggest you manage the movement yourself. You can use your entity's update methods and move the entity how you want it.
-
How to make the player do something when the held item breaks
jabelar replied to FireDragon033632's topic in Modder Support
That topic is a bit old. I've got most of a tutorial on events drafted as well, updated for 1.7.2 which may help some people. FireDragon, you can check it out here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html -
qpwoeiruty, all of your questions in this thread seem to be from not understanding Java fully. It is okay that you're a beginning programmer (everyone has to start somewhere), but your questions really aren't about modding they are about Java. You didn't know how to call a method, you didn't know about static methods, you didn't know about casting, and now you don't know about return values. You should really just learn more Java. There are plenty of online references, but I also suggest having a very good book that is easy to read called: Java In Easy Steps.
-
How to make the player do something when the held item breaks
jabelar replied to FireDragon033632's topic in Modder Support
Not sure if this would work for you, but there is an event called PlayerDestroyItemEvent that might be what you need. -
To debug this sort of issue, I add a lot of System.out.println() statements to help trace the code to confirm if it is doing what I expect. For example, if you change the code to the following: @Override public boolean canHarvestBlock(Block par1, ItemStack itemStack) { System.out.println("Executing canHarvestBlock() method, block parameter = "+par1.getUnlocalizedName()); boolean isHarvestable; isHarvestable= (par1 == Blocks.bedrock || par1 == Blocks.log || par1 == Blocks.log2); System.out.println("isHarvestable ="+isHarvestable); return !(isHarvestable); } It should tell you whether the method is being called at all, tell you what block was passed to the method, and what the isHarvestable value got set to. That sort of information usually helps you figure out where the execution is going wrong.
-
The disco ball is a TileEntity which is just fancy way of saying a block that executes logic. All that codes seems to obviously be calculating the positions of the little lights. I suppose that those could positions could be used to control particles but it isn't clear since there is no mention of particles in the code you showed. It could still be some sort of render effect. Anyway, let's go back to what you want to do -- make some ghostly smoke come from some blocks. The main point is that you should have a TileEntity somewhere (I assume that would be your custom block you mentioned that already has a guy) that searches for the blocks you care about (the walls) and then creates the effect with your particles. There are probably several ways to do this, but basically your TileEntity just needs to do a "search" around itself to find where the blocks that form the walls are, and then you just control the particle position according to what you find.
-
Block that hurts hostile mobs but not the player or passive mobs?
jabelar replied to MikeZ's topic in Modder Support
I actually think that onEntityCollidedWithBlock() method doesn't work for the idea of an entity "bumping" into a block. Rather it is intended for those types of blocks that an entity can actually "enter" into. Like a portal or a web. And arrows are allowed to "enter" blocks to trigger this method. If you think about it, most entities can't "collide" with a regular block because the path finding won't let that happen -- the movement is prevented before an actual collision occurs. It is a poorly named method, but from some other debugging I've done recently I came to the conclusion that you have to be inside the block to trigger this method. I could be wrong, but that was something I thought I previously found to be true. Anyway, it is easy enough to prove. Just put a System.out.println() statement in the method to see whether it is ever being called. -
SimpleNetworkWrapper return packet problem
jabelar replied to octarine-noise's topic in Modder Support
That does seem like the reply mechanism is "borked". As you did do, you can of course create your own reply message easily enough, but it is a shame if the standard mechanism isn't working. If none of the experts here can point out an obvious flaw in your approach you should probably submit a bug report. -
Yeah, I was a bit worried about that part (converting multiple levels). I think we'll have to keep track of the previous absolute position for each child as well. Probably easiest to add that as fields in an extended ModelRenderer class, but could also just be some sort of array in the Model class. So the convertToChild() would look at the parent's originalRotationPointX, originalRotationPointY, originalRotationPointZ for the purpose of the conversion. That should solve it, right?
-
If you look at the constructor it sets the initial motion and the life of the particles and then the onUpdate() updates the position. So you would put in what you want -- do you want them to start out in specific directions, move fast or slow, move randomly, move straight or in circles, etc. And to spawn on the walls you just need to set their initial position to be where the wall is. Whether or not the wall is easy to detect would depend on whether the size and shape of the room is already expected to be a certain way or whether you want your particles to be smart enough to go around any shape and size of room. However, I looked at that video you posted again and it is possible that they did not use particles. They may just be doing some sort of render effect. Anyway, I think it would be possible for the disco ball in that example to have a TileEntity associated which is controlling the effect. In fact, if you keep track of the particles that are created, the TileEntity itself should be able to update the position.
-
In terms of Netty (or packet handling generally) the key thing is to understand the concepts they all have in common, namely: The Steps Needed For A Packet Handling System Basically, you’ll need a packet system that: a. Registers a networking channel specific for your mod (this allows you to ignore packets that may come from other mods). You could theoretically use an existing channel but then there would be chance that your payload format conflicts with other mods or vanilla packets. b. Has packet handler classes on each side that is called on packet events. Depending on the packet system, you may need to subscribe to an event or simply use an onMessage() method or similar. c. Has a class on each side to create packets. The creation mostly involves creating a payload which is usually a ByteBuf. Most systems make the first int as an identifier of the packet type, often called the "discriminator". You can make up your own types. The rest of the creation of the payload is often called "encoding" but really just means you put the values you care about into the buffer in an order of your choosing. d. Has a class on each side to process received packets, distinguish packet type and what side it is on and process the packet accordingly. You could actually put this code in the handler, but as your mod grows the number of packet types and complexity of their payloads will grow so most systems put these in separate message classes. Again most of the work is related to the payload, with the first int usually being a discriminator that represents the packet type, then remaining payload which needs to be "decoded" into retrieved values in the order you expect them for that packet type. d. Sends the packets as needed (ideally just when there are changes that require syncing of a value between client and server or vice versa, which is often in a setter method). I have a modpack with about 20 mods. After some time the game uses much more memory and freezes when i switch to another window. When I read about the memory leak in the tutorial Packet Handling, which I guess tons of modders are using, I was wondering if this could be the problem. Any ideas on that? A memory leak would do something like that -- increasing memory use until system has trouble.
-
Yes, it is worth understanding inside and out. The only problem for you is you made a complicated model before fully understanding it, now the rework is extensive. I was trying to fix just the teeth and it is a lot of work, after about half an hour on it I was only halfway there.
-
I've been fretting over this topic for a while too. This link shows working example of the SimpleNetworkWrapper system: https://github.com/pahimar/Equivalent-Exchange-3/tree/master/src/main/java/com/pahimar/ee3/network It is meant to be "simple" so perhaps loses some power and flexibility that some modders would like. But if cpw and pahimar both recommend it I've got to believe it is worth checking out.
-
Okay, while I do want people to understand the concepts, I've come up with the (simple) convertToChild() method that will make taking the Techne Java output and creating hierarchy extremely easy. The important thing is to first in Techne get the rotation points in right place (see the video I linked above). Then export the Java and put it into your custom model class in Eclipse. Then add the following method to your model class: // This is really useful for converting the source from a Techne model export // which will have absolute rotation points that need to be converted before // creating the addChild() relationship protected void convertToChild(ModelRenderer parParent, ModelRenderer parChild) { // move child rotation point to be relative to parent parChild.rotationPointX -= parParent.rotationPointX; parChild.rotationPointY -= parParent.rotationPointY; parChild.rotationPointZ -= parParent.rotationPointZ; // make rotations relative to parent parChild.rotateAngleX -= parParent.rotateAngleX; parChild.rotateAngleY -= parParent.rotateAngleY; parChild.rotateAngleZ -= parParent.rotateAngleZ; // create relationship parParent.addChild(parChild); } Then call this method to create the hierarchy. For example, I took the Techne default ModelBiped and output the Java then added this method, with the result being: head = new ModelRenderer(this, 0, 0); head.addBox(-4F, -8F, -4F, 8, 8, ; head.setRotationPoint(0F, 0F, 0F); head.setTextureSize(64, 32); head.mirror = true; setRotation(head, 0F, 0F, 0F); body = new ModelRenderer(this, 16, 16); body.addBox(-4F, 0F, -2F, 8, 12, 4); body.setRotationPoint(0F, 0F, 0F); body.setTextureSize(64, 32); body.mirror = true; setRotation(body, 0F, 0F, 0F); [color=blue]convertToChild(body, head);[/color] rightarm = new ModelRenderer(this, 40, 16); rightarm.addBox(-3F, -2F, -2F, 4, 12, 4); rightarm.setRotationPoint(-5F, 2F, 0F); rightarm.setTextureSize(64, 32); rightarm.mirror = true; setRotation(rightarm, 0F, 0F, 0F); [color=blue]convertToChild(body, rightarm);[/color] leftarm = new ModelRenderer(this, 40, 16); leftarm.addBox(-1F, -2F, -2F, 4, 12, 4); leftarm.setRotationPoint(5F, 2F, 0F); leftarm.setTextureSize(64, 32); leftarm.mirror = true; setRotation(leftarm, 0F, 0F, 0F); [color=blue]convertToChild(body, leftarm);[/color] rightleg = new ModelRenderer(this, 0, 16); rightleg.addBox(-2F, 0F, -2F, 4, 12, 4); rightleg.setRotationPoint(-2F, 12F, 0F); rightleg.setTextureSize(64, 32); rightleg.mirror = true; setRotation(rightleg, 0F, 0F, 0F); [color=blue]convertToChild(body, rightleg);[/color] leftleg = new ModelRenderer(this, 0, 16); leftleg.addBox(-2F, 0F, -2F, 4, 12, 4); leftleg.setRotationPoint(2F, 12F, 0F); leftleg.setTextureSize(64, 32); leftleg.mirror = true; setRotation(leftleg, 0F, 0F, 0F); [color=blue]convertToChild(body, leftleg);[/color] Lastly, I got rid of the render calls for each child, and only called body.render(f5). Hope this helps. If you're getting tired of reworking your model, feel free to send me the Techne file and I'll be able to get it going pretty quickly.
-
People run into trouble because the placement can look correct even if the rotation point is wrong. This is because the offsets can hide the problem. I just made a quick vid ( ) to show you this -- I move the rotation point and offset of the arm and the arm still seems to be in correct place -- but you can see the problem when you try to rotate it. This is what you've done with the teeth. The child will automatically rotate around the parent's rotation point. You don't need to align the child and parents rotation points in any way (you only have to align them if you don't have hierarchy). Any further rotation on the child itself will be around its own rotation point. This is only really critical for child pieces you plan to dynamically rotate. If you've already figured out a combination of rotation points, offsets and rotations for the teeth that work, I would just leave them. But for anything that will move dynamically (legs, tail, etc.) you need to ensure you get it right. In my tutorial, I recommend adding spinX(), spinY() and spinZ() function to use for debugging. You can call this right before the render and can pass a child piece to it. The child piece will then rotate and you will quickly understand whether the rotation point is correct. // spin methods are good for testing and debug rotation points and offsets in the model protected void spinX(ModelRenderer model) { model.rotateAngleX += degToRad(0.5F); } protected void spinY(ModelRenderer model) { model.rotateAngleY += degToRad(0.5F); } protected void spinZ(ModelRenderer model) { model.rotateAngleZ += degToRad(0.5F); }
-
No, there shouldn't be any math. I haven't looked at your model class yet, but I suspect you didn't entirely adjust all the rotation points properly. The rotation points should be at the point of connection between the parts (in most cases). So for the teeth it could be the center of each tooth, and then each tooth is attached at different point to the jaw. If in Techne you already put those rotation points in proper place, there shouldn't be any issue. They were all just rotated 45degrees, and it doesn't matter whether the jaw is also rotated relative to the head and the head is relative to the neck and the neck relative to the body and the body relative to the world -- shouldn't be any math except to set rotation to 45degrees.
-
Awesome. I suggest that the setRotation() method be converted to use degrees as well (just takes a few minutes to update all the calls) -- as always just trying to protect your sanity! A formula is a series of steps - I was just using the concept of steps to explain why your formula should work. In other words, your formula of: 1.5 - (1.5 * shrink) is exactly equivalent to me saying "take the steps to translate 1.5 then translate back by 1.5 times the scale factor." It is great to have figured this out together. I think now you can understand the powerful animation possibilities once you have the rotation points and hierarchy in proper place. The only thing I still want to work on is to make the convertToChild() method so I can just take a Techne Java output as is and directly create the hierarchy without having to think much. It should be pretty easy, provided you put the rotation points in the proper places in the Techne model.
-
Is you file jpg or PNG? You have mismatched them in what you wrote ...
-
I think you need to override the constructor and the onUpdate() method in the EntityFX class for your particle. That is, I believe, where the motions are intialized and ujpdated.
-
You mean a texture for the item itself? There is a potion_bottle_splash.png which I think is set by the getIconFromDamage() method in ItemPotion.
-
It matters because any point away from the origin of the scale will also get scaled. If you have a model that is 1 pixel offset from the origin and call the scale for 2x, the model will then be 2 pixels away (in addition to being bigger). If you want to scale something but keep it in its current postion you need to translate to origin of the scaling reference, scale, then translate back. Otherwise the model's relative origin will move during the scaling. Basically, scaling something that isn't at the origin of the scaling system will also cause translation. Not sure if that was clear ... Note that we have an additional problem to consider in our case -- we want the feet/base of our model to still touch the ground after the scaling. So we can't just move the model back to original translation, but rather we need to do the scaled translation. This is what you found in your formula -- i.e. you had to scale the second translation. So what I think we need to do: - original model starts at 1.5blocks above ground. - need to move model down 1.5blocks such that origin is at the ground - scale the model - move the model up by 1.5*scaleFactor (since you're trying to get the feet of the scaled model back up to the ground level) That is pretty much what you found out, and so I'm just explaining why it makes sense. I think.
-
Delpi, do you agree that the 1.5 probably is explained by the explanation I proposed? (1.5 is due to 0, 0, 0 point of model being at 24 pixels above ground.) The thing we need to think through is where this offset is in the order of the actual operations. Does the scale happen around the 0, 0, 0 point of the model? I kind of expect that it does not because otherwise the model wouldn't "move" so much when scaled. So is the scaling actually happening around the point on the ground (1.5 down in Y direction)? In other words, should you scale then translate, or translate then scale? I've always just mucked around to correct the offset manually ... What's your understanding? I'm also wondering if doing the scaling in the preRenderCallback() behaves any differently. It's possible (I haven't had time to trace the implementation) that that is called inside the translation.
-
How to create an OBJ Importer for Entities, Items, and Blocks
jabelar replied to Brinith's topic in Modder Support
Not sure how easy it is to understand by just looking at the code, but there is already a WavefrontObject class that handles the importing of obj files. I could be wrong but I think you just extend that class, point it to your obj file and register the call with a renderer. -
Note that Eclipse needs to "see" your assets. Moving them into the folder isn't always enough (sometimes it is depending on how it was set up). You should go into the package explorer and confirm that the asset packages are there and furthermore see if the texture assets show up. If not you need to Import them (right click on the package). Note that annoyingly if you try to import them while they're already in the folder, Eclipse will complain that it is already there so I drag them out of the folders then import them through Eclipse. Not sure if that is what is causing your trouble, but most times an asset isn't recognized for me it is related to this.