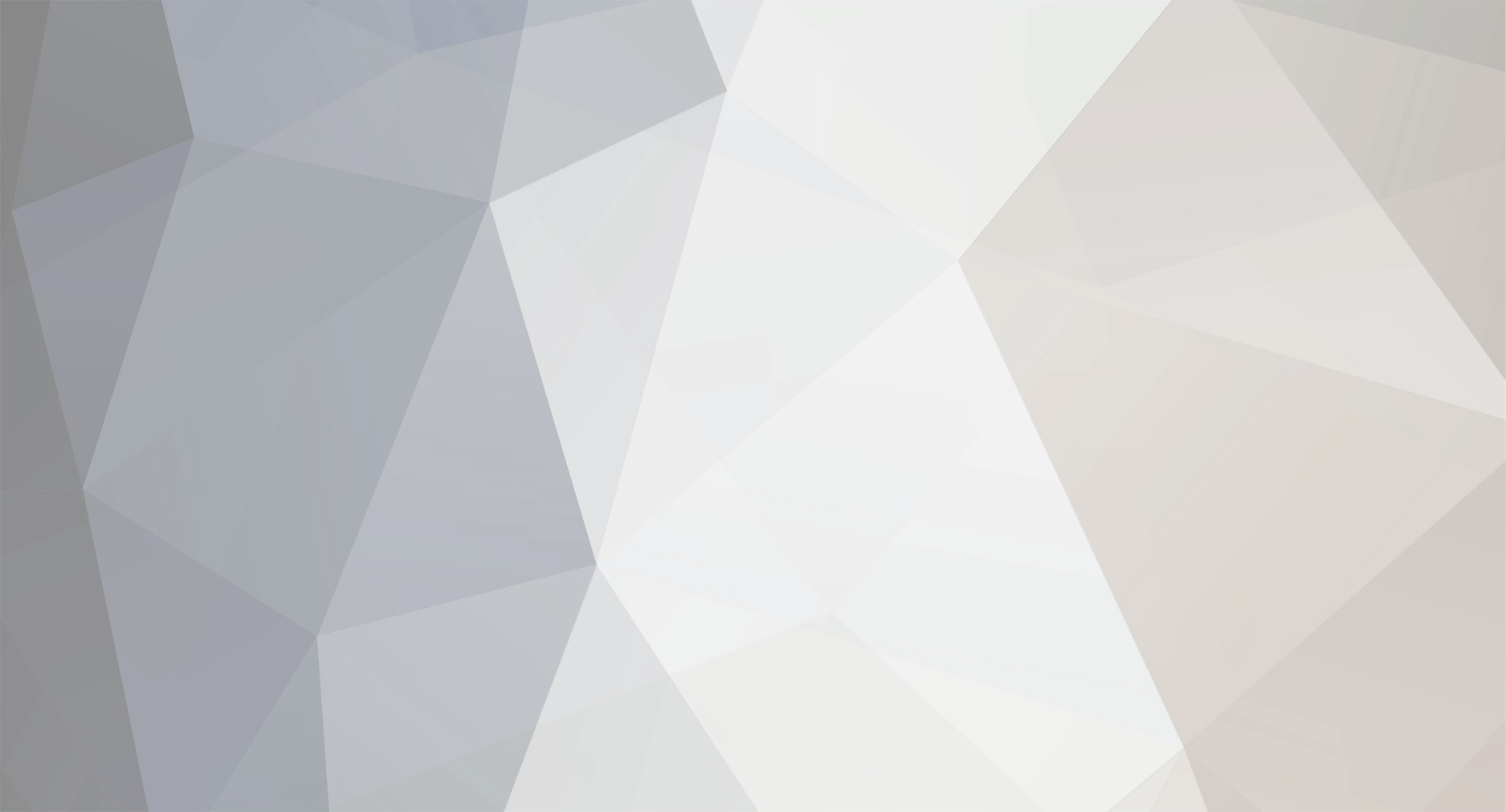
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
[1.12.2] Hostile mob can't attack, only pushes aggressively
jabelar replied to That_Martin_Guy's topic in Modder Support
I have a tutorial on this. http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-custom-entity.html?m=1 The problem is usually you haven't properly overridden the attack as mob method, depending on what class you extended.- 1 reply
-
- 1
-
-
[1.12.2] Stop rain splash particles from spawning
jabelar replied to Daeruin's topic in Modder Support
Hey, I think there is an easy way. There is a set alpha method I think in the particle fx class. You can probably just set that to make fully transparent and then back when you want them visible. -
[1.12.2] Stop rain splash particles from spawning
jabelar replied to Daeruin's topic in Modder Support
I'm traveling at the moment so won't have chance to helpmuch this weekend. I would not worry about the other resource packs at the moment, but just put in your own modified texture file for the vanilla particles edited so the splash is transparent. Confirm that works. Then if the texture field is accessible, even if you have to use reflection, you could swap it dynamically. Otherwise you need to create the custom particle. I'm sure it is a bit tricky as vanilla uses indexes to pick out the texture. -
Help With Ore Gen With Custom BiomeDecorator
jabelar replied to Emerald_Galaxy's topic in Modder Support
When you run into a problem like this, I don't know why people ask for help without simply trying to observe what is happening. Programmers don't debug by simply looking at code, rather they "trace" the execution. You have two ways of doing that (1) use debug mode in Eclipse (or whatever IDE you're using) and set breakpoints in interesting parts of the code and see what path the code takes, or (2) use console or logger print statements to print out what is happening. For example, how come you haven't put any print statements in your decorator methods? If you put some in you can confirm which methods are being called. For example, do you even know if your generateOres() method is being called? Just put in a print statement! And your print statement can have extra information in it like values of important variables. If you don't like print statements you can do same thing by setting the breakpoint for the method and then stepping through the execution. Please do that and it should be obvious what is happening. But if you still can't figure it out post what you find and we can help. -
I looked through the call hierarchy and technically in some cases it is in fact clamped. The LootingEnchantBonus.apply() method clamps the loot level to the "limit" which is set by deserializing the loot JSON for the looting_echant limit value. Now I don't think the vanilla looting tables specify a limit so I think they should deserialize to 0 in which case the clamping won't happen. But just saying there are cases of code that might impact the looting after you process your event so you need to look at that. I think you should just use standard debugging and set breakpoints and trace the value along the way. Computers are pretty much perfectly logical so if you trace every step you're sure to see where it does something you didn't expect, and then the answer will be clear.
-
[1.12.2] Stop rain splash particles from spawning
jabelar replied to Daeruin's topic in Modder Support
I think it would be compatible with texture packs. Your replacement particle would use whatever texture the rain would have been, which would include any texture pack replacement. At least you should be able to do so depending on when you assign the texture to your particle. -
[1.12.2] Stop rain splash particles from spawning
jabelar replied to Daeruin's topic in Modder Support
Probably. But you could also create a custom particle that has the original texture and explicitly generate it yourself. So basically let the original spawn since it is a bit tricky to intercept but have it invisible, and then spawn your own when appropriate. -
Back to the original problem. I don't have my workspace on this computer, but maybe the setLootingLevel() or getLootingLevel() is validating the value and clamping it to a certain range. For example, your math in your event would set looting level to 21 higher than what it already is. Is that a valid level? I thought looting levels go to 3 or something....
-
Okay, yeah the block colors are limited but there is space for the overall colors array. Just use Reflection to make it accessible, which I'm assuming is the solution you found.
-
Do you mean to use it on actual maps? And you want to add an additional color? I don't think you can because the way the map information is stored, there is only 4 bits for each location to represent color and there are already 16 colors defined.
-
Hook like LivingEntityUseItemEvent but for left click
jabelar replied to wolfboyft's topic in Modder Support
There is also event for clicking on nothing. So I think pretty much every type is covered. But there are also mouse events you can directly handle. -
Back to the original problem, it says that the slot is not in valid range. I don't think that has anything to do with the size of the itemstack, I think you're trying to access a slot that doesn't "exist" based on the slot numbers. I usually find these errors have to do with some mismatch in how I add my own slots and then access them later. As mentioned, use standard debugging methods but I think focus should be on your slot numbering not the itemstack size.
-
Getting a block's smelting recipe? (1.12)
jabelar replied to ThatBenderGuy's topic in Modder Support
Yeah, Draco18s is right as usual -- best to compare the Item not the stack. To original poster, if you do want to compare item stacks the ItemStack class has a number of methods for comparing, some that include NBT, some don't etc. There is areItemsEqual(), areItemsEqualIgnoreDurability(), areItemEqualUsingNBT() and so forth. -
A fake world is the proper way to do it, but you could probably be sneaky and place the block somewhere very, very unlikely to cause trouble, like a bedrock location, temporarily and then put it back to bedrock. So basically, set hardness and stuff to some default fixed value in the constructor. Then during world generation run a loop once that takes a bedrock location, cycles through the list of blocks you want to create slabs for, place the block, grab the properties, replace with next block, grab the properties, and so on then put back the bedrock when done. Also, if you only want to handle vanilla blocks you could probably just hardcode the values. A bit of a pain, but you figure out the values (maybe even algorithmically with a simple mod/program) and then read them in.
-
Hook like LivingEntityUseItemEvent but for left click
jabelar replied to wolfboyft's topic in Modder Support
The PlayerInteractEvent has a number of sub-events like LeftClickBlock, RightClickBlock and so forth. I also think maybe the LivingEntityAttackEvent might trigger on left click. -
Are you talking about the player UUID? You should be able to run with same player in development environment by adding the --username and --password arguments to your run configuration. I'm not sure in IntelliJ where that is done, but in Eclipse you edit the run configuration arguments. This thread made me chuckle. NBT is theoretically really simple, but I admit it can hurt your head, mostly because you can combine things in multiple ways -- lists in compounds, compounds in lists, lists of tags, lists of primitives and so forth. Also, the order of construction/deconstruction has to be matched and any typo will kill you. I highly recommend when working with NBT that you print the contents out to console/logger at each step to get a sense of what is really happening. Seems like you got NBT working now? EDIT: I wish Forge would have provided methods for syncing capabilities to client. While not that hard to implement it is such a common modding need. Someday maybe I'll suggest a pull request for that, although maybe there was a reason they chose not to in the first place.
-
@GameAndDevNote that your registration style is outdated. While it can be made to work, you should probably convert to the event based method. See http://mcforge.readthedocs.io/en/latest/concepts/registries/. Also I have example code here: https://github.com/jabelar/ExampleMod-1.12/blob/master/src/main/java/com/blogspot/jabelarminecraft/examplemod/init/ModBlocks.java Secondly, your console should be warning you about any problem with models or textures. What error is it saying? To get textures right, you have to be extremely careful to make sure the names match up exactly including capitalization. The name of the blockstate JSON filename must match exactly the registry name of the block. Then that file needs to reference any variants with exactly matching names, and point to models who in turn have to have file names that match exactly. Those model JSONs then will point to textures that need to have names that match exactly. So basically look at the console error and see if it is a variant model missing or a texture missing then look carefully at your asset files to ensure they all match up.
-
Getting a block's smelting recipe? (1.12)
jabelar replied to ThatBenderGuy's topic in Modder Support
Yeah, the general idea looks correct. You might need to trace through to make sure there aren't any detailed bugs, but conceptually yes -- you take the smelting list, you grab the block from the block recipe, and iterate through the smelting list to find a match. For storing in NBT, store the registry name (and anything additional you care about like metadata) then read the NBT to restore it from registry (and restore any additional info you care about). -
That isn't a breakpoint, it is obviously a null pointer exception. Not sure why you'd hit it in debug mode and not in normal mode. But it says you have a null pointer problem with your ContainerDragon class.
-
Good point. Not only is vanilla hard-coded to the leaves for the init() method of Minecraft class, but for any other change in settings. Personally I don't care about that as I feel that very few people must have computers which need to have fancy turned off any more, so I just forced it to be "fancy all the time". But logically yes you would fully handle the game setting directly if you wanted to support fast level.
-
Getting a block's smelting recipe? (1.12)
jabelar replied to ThatBenderGuy's topic in Modder Support
I think so. That should include the modid automatically. When you read it back, just pass the string to a new ResourceLocation() constructor. -
[1.12.2] Stop rain splash particles from spawning
jabelar replied to Daeruin's topic in Modder Support
the entityRenderer field is public in Minecraft class. I think you might be able to replace it with your own version (that copies that class and changes the code related to the particles). Another thing you could try is to make the particles invisible. Like maybe replace their texture with something fully transparent. You should also log an issue at the github MinecraftForge project requesting an event hook for this. -
Getting a block's smelting recipe? (1.12)
jabelar replied to ThatBenderGuy's topic in Modder Support
Yes, other mods are supposed to add their recipes using FurnaceRecipes#addSmeltingRecipe() method, so I think the list should contain them all. You should store the registry name not the unlocalized name (there is no guarantee they are the same). Then you can just read in the registry name and look it up in the ForgeRegistries.BLOCKS. -
What exactly are you trying to do? The server already knows who attacked you so why not just have the server send the info directly? Or is it because you want this to be a client-side-only mod? What specifically are you trying to do? Are you doing this for projectiles like arrows? In that case I think you could simply detect when arrow are created and tag them with information about the entity at that location that probably is shooting it.
-
Getting a block's smelting recipe? (1.12)
jabelar replied to ThatBenderGuy's topic in Modder Support
Can't you use FurnaceRecipes.getSmeltingList() which actually returns a Map and then just look for a key that is same as your block?