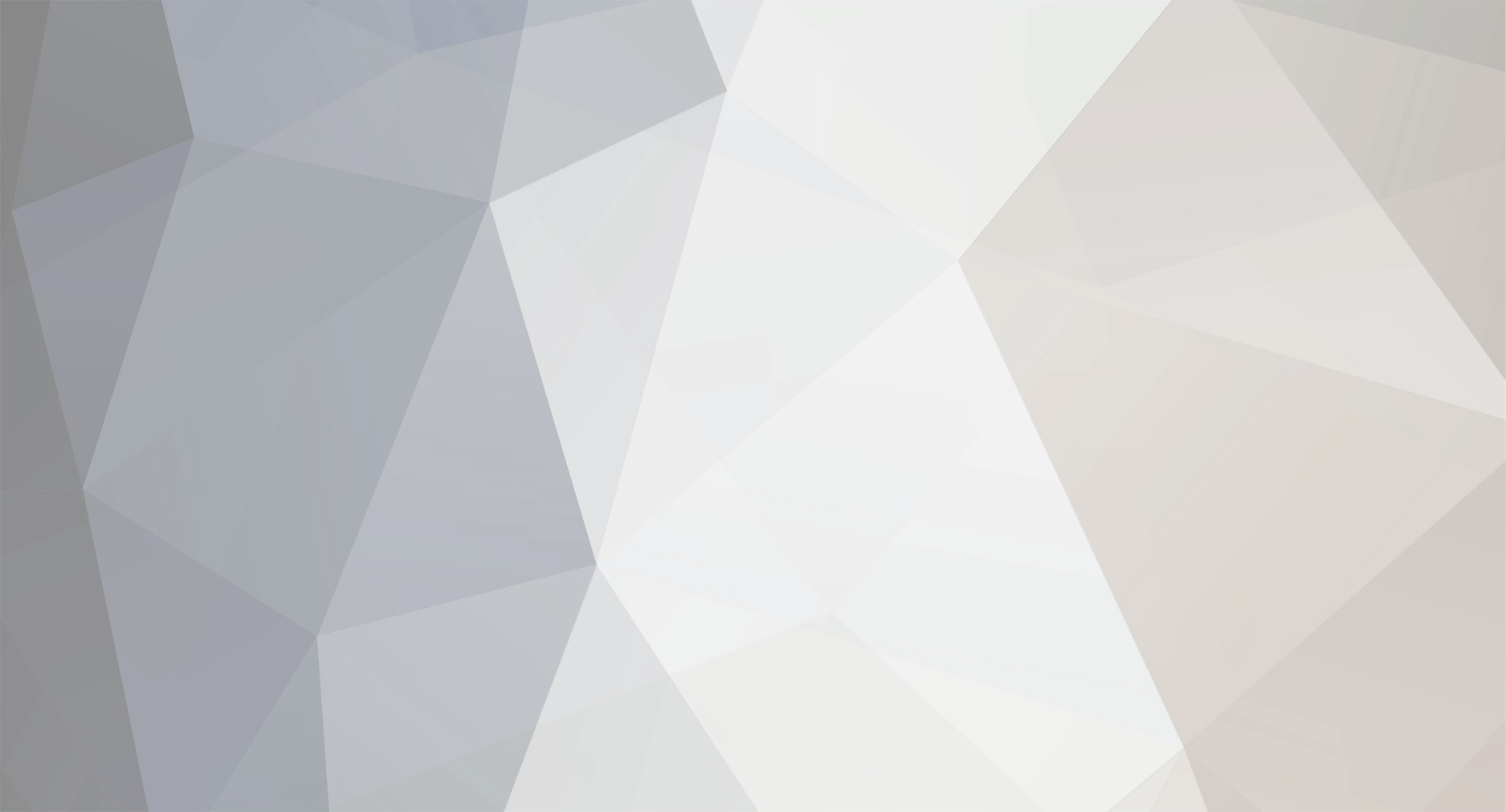
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
I think your registrations aren't in the proper FML event and possibly you're creating multiple instances of the item. In your pre-init handling method you are creating recipes using stack of an instance from your Items class (which you didn't provide code for us to see) but this is before you even register your item (which you do in your init handling method). Furthermore, I thought the model needs to be loaded on the client side, although I used to register my mesher directly and I suppose the model loader class might take care of that. I personally would do things in following order: Pre-Init: Register your item. Init: Register your recipe Register your model (only on client side). Also, I would check to make sure that your Items class isn't making extra instances of the item (since you also create an instance in your main mod class).
-
It also depends on how you want the inter-mod interactions to work. While you can't combine other mods generally into single JAR you can make the other mod a dependency so that your mod requires the other one to work. Next you need to make sure your code that depends on the other mod loads or executes after the other mod is loaded -- for example if you want to use an item from another mod you'd need it registered before you try to use it. For things that get registered, you can generally just access them by knowing the modid of the other mod and referencing the related registry. if the other mod is designed to provide an API then you can call those methods from the other mod and they should work.
-
Okay, fair enough. Generally looping through enums in Java is kinda annoying, but I guess EnumFacing class provides a couple of arrays that can help looping.
-
Not that easy though because it isn't just the conditions that cycle through the directions but also the action -- the positions are different in each case. So if he had an iterator for the direction he'd still have to look up what to do, probably using a bunch of if statements, when the condition is met. I really don't think it would save many lines of code. If he was doing the SAME thing in each case then definitely it could be compacted, but harder when it does different things.
-
Yeah, but you'll note that none of your code is really repeated -- you're checking different blocks / locations and placing blocks in different locations. You've already shortened the amount of code by assigning things like north, east, south, west. Now the code you posted seems to be about checking specific location. Do you have other code that loops through lots of locations? Or maybe you're just started and will add a lot more lines like the ones you show? In those cases, yes you might be able to compact it. But there is nothing wrong with "brute" force approaches to programming. It is often less buggy to do it the way you're doing it, because if you try to get clever you can end up doing something wrong in a loop that creates very strange results. It also doesn't save much typing because you can cut and paste easily enough. So my recommendation is to only look at compacting code if you have a lot of repetition or if you feel performance is being hampered due to inefficiency. Otherwise, as long as it is short and readable it is good code.
-
I don't think there is any reason to "compact" it. It is already short, doesn't have any performance impact, and is fairly readable.
-
What errors do you get in the console? Is it complaining about a missing model? Is it warning about a malformed JSON? Also you need to have model files with the right names in the right folder in the assets. Can you show what your file tree looks like?
-
I know what the problem is but let me mention a couple things before I get to that. Looking at your code I think you probably based your effort off of one of my tutorials or github examples. For an item model to show up, it needs to be 1) instantiated, 2) registered with a registry name 3) have a model JSON file with matching name in proper asset location, 4) have a texture with name pointed to by the model JSON. Recently I've been improving a few things. I don't think they're causing you trouble in your case, but you should still probably update as follows. In the JSON, the one you posted was copied from some vanilla and the scaling was modified because I think I wanted to make a bigger item than normal. If you just want a regular sized model you can use the following instead: { "parent": "item/generated", "textures": { "layer0": "extendedemeralds:items/emerald_nugget" } } The second thing is to do what Ugdhar suggested and register using the ModelLoader instead of the item mesher. However, I don't your error is caused by that in your case it is explicitly telling you that the model is not found. That means it knows there is supposed to be a model therefore it means your item is registered at least as an item. Almost every time this happens to me it means that I have a typographical error somewhere and the names don't match. Now one of the things that changed around 1.8 is they introduced the registry name as being separate from the unlocalized name. The registry name is the string used as the key for the registry and the unlocalized name is used for looking up localization in the lang file. There is no reason for them to be different but they can be so most people keep them the same. Your problem is that when you register in the GameRegistry you use the wrong name -- it doesn't match your model file name. You put "EmeraldNugget" when it should be "emerald_nugget". Generally to avoid having to type the name twice and have chance for errors, people instead now put a setRegistryName() call in the constructor and when using the GameRegistry register method you don't put any string at all. Be careful if you try to get fancy by trying to get the unlocalized name to set the registry name because the setUnlocalizedName() and getUnlocalizedName() are not symmetric -- you get a different thing back because it adds "item." to the front of the name. I get around this by creating a method for setting both names at once like this: // Need to call this on item instance prior to registering the item // chainable public static Item setItemName(Item parItem, String parItemName) { parItem.setRegistryName(parItemName); parItem.setUnlocalizedName(parItemName); return parItem; } Note I personally think it is a very weird thing to have the registry name in the item (or block which works the same way) but that's the way it works.
-
[1.12] Butcher Harvesting Meat Doesn't do Anything
jabelar replied to OrangeVillager61's topic in Modder Support
In your code posted above, in the constructor of your AI class you call the setMutexBits(3) method. Which means you set the mutex bits to value of 3. "Mutex" is short for "mutually exclusive" and works sort of like a mask. I have a tutorial on making entity AI which discusses mutex bits about 1/3rd way down the page: http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-custom-entity-ai.html -
How do you make your mod able to run in multiple versions in mcmod.info?
jabelar replied to Arkyo's topic in Modder Support
Generally you can't make a single mod support multiple versions because the codebase and Forge change with each version. For example, the way you register things in 1.12 is very different than in 1.7.10, newer versions use block states, recipes now use JSON, there are no longer Achievement but now there are Advancements, extended entity properties have been replaced with Capabilities and so for. Even for things that are functionally the same many of the important methods and fields have changed names in the SRG mappings. So it would be very difficult and unlikely to be able to make a mod that can be applied to many different versions. Instead what you should do is port the code over to each version as separate project, build each one and then provide them to people to use together with the appropriate version. In other words, to make it work with multiple versions you need to do a lot more than just change the mcversion value. You have to actually port your code and for complex mods each port to a new version can take weeks of effort. -
[1.12] Butcher Harvesting Meat Doesn't do Anything
jabelar replied to OrangeVillager61's topic in Modder Support
You should keep going with the print statements. For example are you sure that the add task is even being called? Put a print statement there. If you're confident that that the AI task is actually being properly added to the entity, then it may be that the mutex bits are set wrong, or maybe if they are set right but another AI task is already running. The mutex bits prevent two AI from running at the same time that might conflict with each other. So if a higher priority AI task is already running, the shouldExecute() for your AI task won't ever be called. -
Doh, that would do it. I guess 1.12.1 got going a couple weeks ago and I missed that transition. Thanks for point it out.
-
Actually, I usually update from the Forge download site. The "latest" there is still 14.21.1.2443. Do you really recommend downloading from github instead or weiting until it is posted at the official download site?
-
Okay, cool. I update almost weekly, so looks like that just came in. Nice.
-
I spent some time to figure out what Choonster is talking about but couldn't follow the code in a meaningful way. As mentioned, there is no ItemPredicates class and ItemPredicate doesn't have a register method. So I'm assuming that he's created his own custom factory or registry class that he calls ItemPredicates but for the life of me I can't see where the various JSON derserializer methods will look for custom ItemPredicates. So I guess we'll have to wait for Choonster to clarify what he's talking about -- he usually knows what he's talking about!
-
What Draco means is that there is no specific event related to that method, but there are several tick and update events that you could use to have your own code to check the block the entity is on. For example, you can using the living entity update event and check which block is under the entity.
-
[1.12] Butcher Harvesting Meat Doesn't do Anything
jabelar replied to OrangeVillager61's topic in Modder Support
Why did you only put one print statement? If you want to debug you should put a print in every if statement to figure out which path is executing. Without thinking too much about it, I expect your shouldExecute() is always returning false. So add print statements in each if within that method and check if any of those are triggering and returning false. -
Okay, so you want a block that is a source of a gas that spreads an effect and spreads out further over time? In that case you would make a gas source block that has a tile entity. The tile entity could search the surrounding area over time and put an effect on any entities it finds, with the range growing. Look up tutorials on making tile entities. Try to make one, then post your code if you need more help.
-
This type of problem is usually very specific to your code and not everyone has time to debug other peoples code. So I suggest you just debug the problem. The way I debug a problem is to add a lot of console statements (either System.out.println() statements or Logger) at all the key points in the code. I usually put one before each if statement and inside the if statement to prove which path of the code gets executed. I'm pretty sure if you just print out the energy transfer values at all the points where they are supposed to change, you'll quickly identify which part isn't changing as expected and then you can narrow in to solve the problem.
-
What have you tried so far? And what exactly do you mean by a block having "gas physics"? Like it would spread out if it has space?
-
HashMap is a common Java collection. It is just an implementation of the Map interface. A map just means there is a key and a value so you can look them up. So in this case you would add each entity as a "key" and the initial wait value as the "value". Then each tick you would iterate (loop) through the list of keys and for each on you would read the value and then set the value to one less (count down) and check if it hit zero (in which case you would remove the entry from the map). Whenever a butcher tries to harvest an entity you would just check if the entity exists as a key in the map, if it does it means it is not yet harvestable. Just search Google for Java HashMap examples.
-
You can create a world data extension with a HashMap or similar collection where you map the entities to the time since last harvested, and update using the server tick event or world tick event. You would only need to add to the map when a successful harvest attempt happens (you don't have to have all the cows in the world in the map for example), and you could delete entries when the timer counts to zero or the entity dies. So it could be a fairly small and efficient map.
-
[1.12] Get EntityCow and other animals out of EntityAnimal
jabelar replied to OrangeVillager61's topic in Modder Support
As an alternative, in Java you can use the instanceof operator instead. So you could have put if (entity instanceof EntityCow) -
So for all these years, my posts have looked very clean to me -- like the following: https://drive.google.com/file/d/0B4i6U-szMzMYTHh0WVBiMnlVMEU/view?usp=sharing Meanwhile, apparently I've had an old signature running that blasts people with full size videos on all my posts! I'm sorry for looking like an idiot all this time but only just now someone took the time to write me and tell me the havoc I was creating. (You know who your true friends are -- they are the ones that tell you have something stuck between your teeth or that your zipper is down.) But I wanted to warn people that you may be running a signature that the forum isn't showing to you when you're logged in. Worth checking occasionally.
-
Well I do see the comment about not being fully implemented, but if you look at the Explosion class the doExplosionA() method does seem to get all entities in the area, check if they are immune to explosion, then damages all that are not immune. You're saying you tried this and it didn't work? If so, just trace through the execution and see what is going on. Another way is to make your own explosion class and copy the code from the vanilla explosion class and debug and modify to your heart's content.