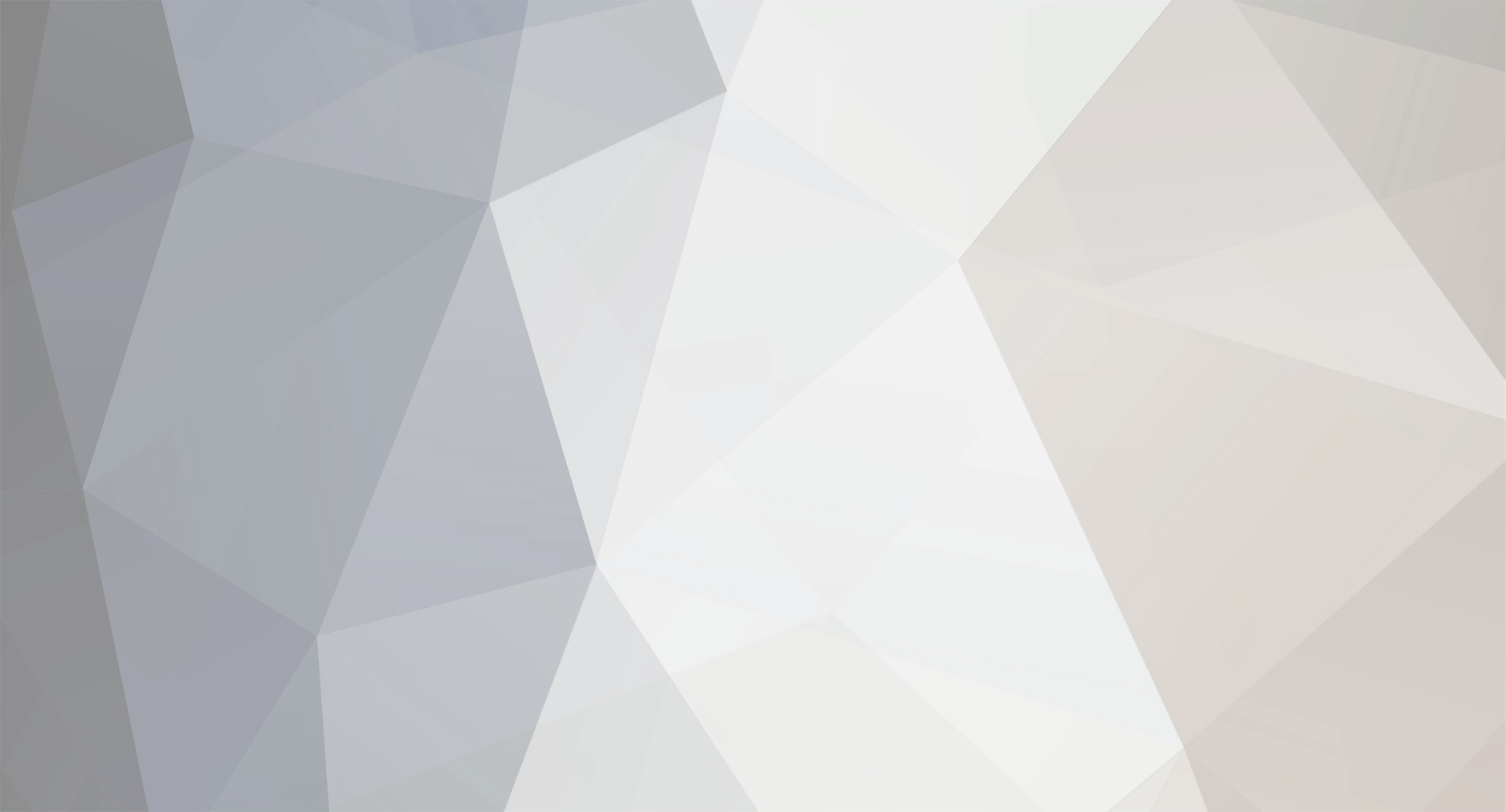
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
I think an important option you've failed to consider is you don't need to use the vanilla mob spawner, rather you can create your own custom ones that extend the vanilla one , and have those placed during structure generation, and have them do the initial logic you want (or otherwise expose the private fields through setter and getter methods), etc. I'm not exactly sure why you need to do the reflection part.
-
Keep in mind that part of the reason there is only 20 ticks per second is that on many computers, at least historically, the game code could barely execute fully in that time and in fact you can sometimes see lag where the game can't in fact complete all the code in time. Now perhaps 1.12 is getting more efficient, and everyone's computers are getting better, so perhaps it is not an issue. But I suspect that running "much" faster could still be an issue even if you could modify the code to try to run faster.
-
If you get malformed JSON it means that you have something syntactically wrong, like an extra comma or missing brace somewhere. There are websites that can verify that your JSON is valid, and I use a JSON formatter plugin for Eclipse to help me make sure I did it all right. Basically you have a typo, which is easy to do in JSON files. Use a verifier and it will quickly be obvious.
-
[1.12] Porting from 1.11.2 registry assistance needed
jabelar replied to OrangeVillager61's topic in Modder Support
I think this line might cause trouble because you're setting the registry name from getting the registry name: event.getRegistry().register(new ItemBlock(light_blue_stairs).setRegistryName(light_blue_stairs.getRegistryName())); Also, I think that uou need to register the item model mesher in your client proxy. But you didn't really say what is going wrong -- is it not showing up at all? is it showing up but with the wrong model or texture? -
Generally the only thing that can take a lot of time are loops, especially ones like while loops which are waiting for a condition that might not be there. So I would suspect it is your while loop in your cable class. When looking at performance problems like this, it can be useful to printout actual timestamps or use a real profiler to time each part of your code. It is usually pretty easy at that point to determine what is taking the most time and then inspection of that code usually quickly leads to realizing what the problem is. Once you isolate the problem, you need to think about alternative techniques that might be more efficient. For example, imagine if you have a bunch of nested if statements you should order them so the least likely thing is tested first. For example, imagine if you wanted something to happen if the player was holding a certain item at night time -- you could first check for holding the item but that would be wasteful because daytime happens half the time in a game whereas holding a specific item might never happen for some players. Sometimes algorithms just don't scale well. In math there are a lot of cases, particularly in networking (which is basically what your energy network is implementing) that don't scale well. In other words there is usually a max size for networks. In fact I think that is why redstone has limited ability to propagate and why entity pathfinding is limited in total area that it checks. In cases where the overall size of the network is limited by the algorithm, you can still improve performance by reducing the frequency of the update operations. That is why "random ticking" is useful -- you don't need leaves on trees to get updated every tick, but you still want them updated sometimes. So you could do the same with your network. For example, you could randomly update each tile entity. Or you could update cables on a different tick than the solar panels which are on a different tick from the machines. Overall though, pretty much every network will be hit a limit where performance doesn't scale well after a certain size.
-
By "craft" do you mean on a crafting table? For that you could just create a custom recipe. Since you said "force" I think you meant that just by holding something it would "craft" into something else? In that case you would just check the ItemStack in the player's hand, compare it to the things you want to be ingredients and then replace it with the output of the "recipe".
-
If you're considering advancements that are triggered similarly to vanilla ones, and for replacing vanilla ones you might want to read up on the JSON capabilities: http://www.minecraftforum.net/forums/minecraft-discussion/redstone-discussion-and/commands-command-blocks-and/2809368-1-12-custom-advancements-aka-achievements
-
Do you want them to also not be available in the creative tabs? Also, if the configuration changes, what will you do with any items already existing in the game? Just making sure you think through all the impacts.
-
You might want to check out my tutorial where I create a weapon with extended reach. In that case I had to find the entity that mouse was pointing at and tell server to process an attack. As mentioned above, the key is to have client send the information to server with custom packet message. My tutorial is here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-extending-reach-of.html
-
The problem with updating to new versions is that there are multiple types of changes. Some are simply changes of names, but some are changes in prototype (like methods now take additional parameters), and some are major changes in functionality. You need to be good at Java and also experienced with modding to be able to clear out all the issues.
-
For the list of items, I think I tend to use: List<Item> list = ImmutableList.copyOf(Item.itemRegistry);
-
I'm not sure specifically about 1.11 but in previous versions you need to make sure that when you add the block to world that the lighting is updated. So you should check what your add block to world function is doing. However, having lighting updated after every block placed can slow the generation of the structure, so maybe you should look for a way to update the chunk lighting once at the end of the structure generation.
-
I missed the memo as well, but darn that is going to really make things nicer (especially for new modders).
-
I've always wondered if you could sort of fake it by using a "dynamic" entity model. Imagine you had an entity that doesn't move that is created to coexist at the light source block position. Then in the model for the entity you scan the surrounding area and create model cubes for each block in the vicinity and simply put a texture on them that is transparent with light tint of the color you want. So basically the entity would be creating a colored filter over the surrounding area. You would probably need to make each block in the dynamic model to be a tiny bit bigger than the blocks to ensure it rendered on top of them. If the updating of the model caused a performance issue you could simply only update it when a block changed. Basically imagine draping a colored transparent blanket over the surrounding blocks. Just a thought -- seems like an approach that could work, would be easy for most of us to program, and not too performance heavy.
-
[1.10.2] Converting my IEEPs [1.8.X] to Capabilities
jabelar replied to hugo_the_dwarf's topic in Modder Support
-
[1.10.2] Converting my IEEPs [1.8.X] to Capabilities
jabelar replied to hugo_the_dwarf's topic in Modder Support
Also, where in your packet to you indicate which entity should get the synced information? Maybe your approach works (to sync capabilities directly) but I personally would do the syncing as follows. 1) When an entity capability value changes, send a packet indicating containing the entity ID and the VALUES of the capability (e.g. the same NBT you'd have from the writeNBT()). 2) When an the packet is received I'd go through the received NBT and then take the entity indicated by the ID and one-by-one I'd update all the attributes one-by-one by calling their setter methods. Pet peeve: why doesn't Forge go ahead and add the syncing functions for these interfaces (it was a problem with IEEP and Capabilities)? It is pretty standard problem to have to sync them, and only a few of us are sufficiently familiar with custom packets to do it right, so would probably save 10% of this forum's time by providing a sync interface that eliminates the need for every modder to redo it... -
[1.10.2] Converting my IEEPs [1.8.X] to Capabilities
jabelar replied to hugo_the_dwarf's topic in Modder Support
Aren't those just String constants he's using to name the NBT fields? Not sure how that could cause any trouble... Hugo, in these situations I usually start again more simply. Right now you have a fairly large number of attributes and are debugging all of generating / save & loading / syncing with packets. Break it down. At the time of generation are you getting expected different random stats? At the time of writing NBT are you getting expected stats? At time of reading NBT are you getting expected stats? At the time of sending a sync packet are you getting expected stats? At the time of receiving the packet are you getting expected stats? So I'd write console statements in all of the above points in the code and trace through to see where the problem is occurring. -
While it is nice if someone has made a nice tutorial, the reality is that the people who write the tutorials have to figure it out themselves so why not just learn to figure things out yourself. As you mentioned, between versions things changes. Sometimes it is very simple to figure out yourself what is the new way. I give some tips on how to update your mods here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-updating-your-mod.html I don't do a lot with biomes so my lists won't cover that, but the stuff with blocks should be useful. But beyond that I explain how to figure things out yourself here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-general-tips-for.html Mainly you should look at the vanilla code and see what has changed. In Eclipse you can open a project in both versions (the old one and new one) and then open up the same classes and go through and compare them. Usually methods and fields that have changed will be obvious (simple renames, added parameters, etc.) and if not totally obvious you can figure it out by looking for new methods that might do the same thing. Occasionally some aspects of the code change drastically -- like extended entity parameters now need to be capabilities, and those things usually get a lot of tutorials. Since there is not a lot of biome tutorials it probably means that not much has changed since 1.7 and you should be able to figure it out.
-
FYI, for detecting biome at a location I think I've used this in the past: worldObj.getChunkFromBlockCoords(blockpos).getBiome(blockpos, worldObj.getWorldChunkManager()).biomeName I haven't tried it in 1.10.2 but I assume it would still work.
-
[1.10.2] Any real need to convert working NBT system to Capabilities?
jabelar replied to jabelar's topic in Modder Support
Okay, that is cool. I knew how to do that, but every time I embarked on an update I never made time to figure it out once and for all (plus the idea of a home-brew regex tearing through my source code was daunting). -
[1.10.2] Any real need to convert working NBT system to Capabilities?
jabelar replied to jabelar's topic in Modder Support
I do do that, but I mean lets say I have recipes that use Items.leather, Items.iron_ingot, etc. and have almost all the items in the game somewhere in my code. I refactor each one (Items.leather to Items.LEATHER) and so forth but it is still quite a bit of work -- select it, call up refactor window, type in the new one, ignore the warning about refactoring when there are errors (when updating you have a lot of errors that can impact easy refactoring), then repeat another 50 times. It literally can take hours. Or do you mean there is some easy way to change all the Items fields to refactor at once? -
Converting power from RF to mod power system
jabelar replied to darthvader45's topic in Modder Support
Yes, we're telling him he would need to make an equivalent API for the second power system. -
I think a more correct way would be to check the "celestial angle" -- i.e. the position of the sun/moon in the sky.
-
[1.10.2] Any real need to convert working NBT system to Capabilities?
jabelar replied to jabelar's topic in Modder Support
Thanks, I guess I'll try. I am of course planning to use Capability for new mods, but I just find updating mods quite a chore in general. Even the simplest things, like having constant fields renamed in all-caps can take quite a while to update if you used them extensively, and the more fundamental changes (like moving events to common bus) take a while to work through. Takes me about a week of evenings after work to update each mod and I've got half a dozen mods so it is usually a couple months duration to get everything working. So I'm interested in minimizing updating unless forced to do it... -
This question is about updating my mods from older versions. In most of my mods, I essentially created my own "capability" interfaces that directly worked with the NBT data (for entities, itemstacks, tileentities, etc.). The important point is I typically did this only for my own custom stuff -- I was not trying to add data to vanilla or other mod objects. My interface included saving and loading as well as syncing with client via custom packets. So it is a working system. Now I'm going through the chore of upgrading all my mods to 1.10.2. At first I read a lot about the capability system and then realized it pretty much does the same thing as my NBT-based custom interfaces. Is there any good reason that when upgrading I should convert my system to the new capability system? It seems like a lot of work that is likely unnecessary if I have a working NBT system already...