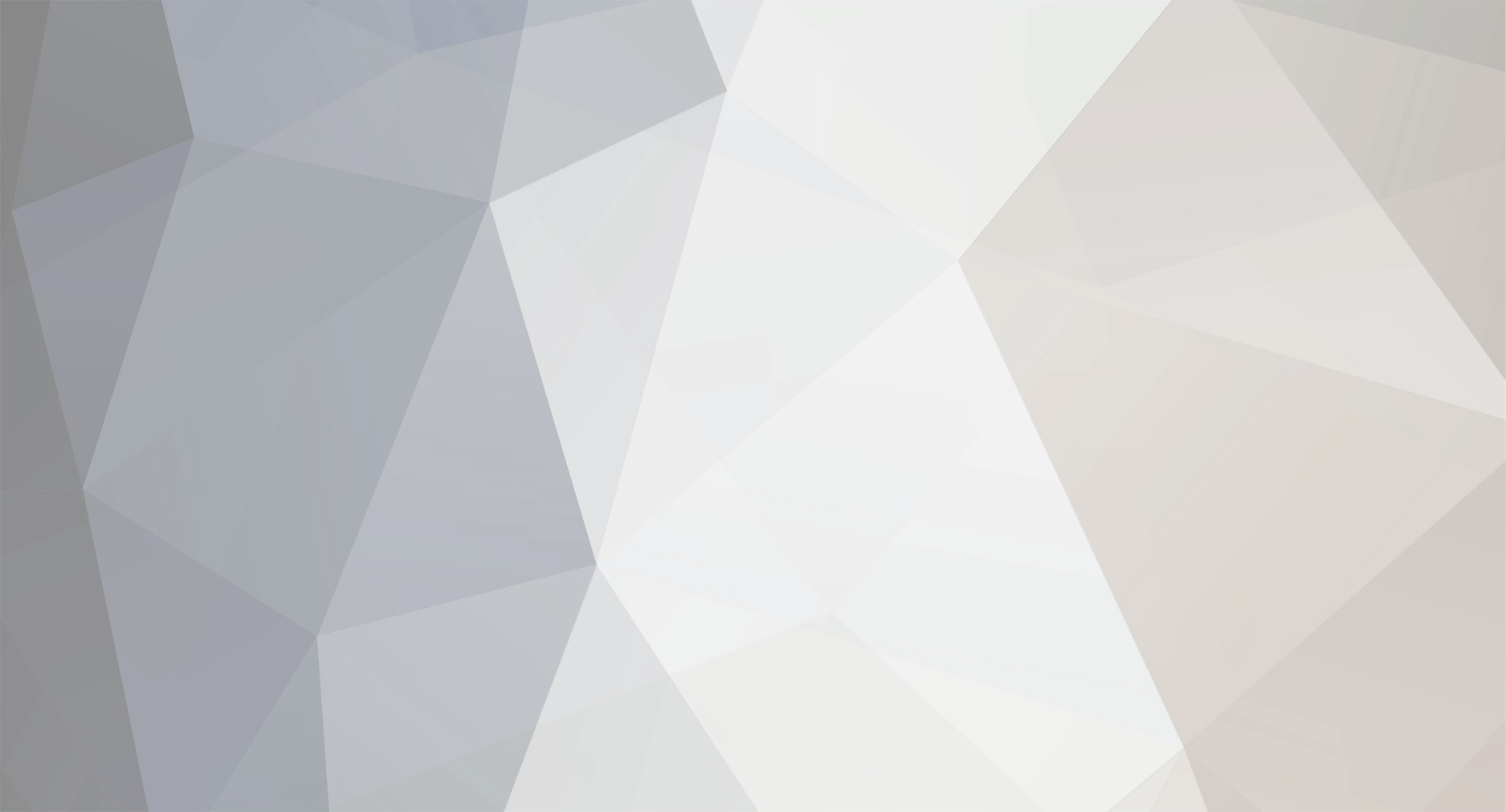
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
You're not really supposed to modify the actual Minecraft source. That is technically against the EULA (if you distribute the results) and also can cause incompatibilities with other mods. If you're into modding, I suggest you use Forge properly. Basically it provides "hooks" that allow you to modify most of the common things you want to change. If you want to change recipes, add items, blocks, or entities, change the AI of vanilla mobs, and so on, there are easy ways of doing it with Forge. And you're allowed to share the result and can mix it with other mods more easily. What are you trying to accomplish with your modification anyway?
-
[Solved] Entities moved in same loop don't update at the same time.
jabelar replied to don_bruce's topic in Modder Support
I think he probably is interested in more than the rendering. He probably wants the multiple collision boxes as well. If he doesn't care about the collision boxes, then yes can probably handle this all as one complex model (with parts that are enabled as appropriate). But if he cares about collision boxes, then he needs to do it like EntityDragon -- associate child parts and sync them all up in the parent's update. -
It is a common mistake that people think they need to create an event class, but actually you just subscribe to existing events. (Theoretically you can create a new event type but then you also have to take care of posting it to the bus, and in the case of what you're doing I don't think you need a custom event.) And there are two types of subscriptions. The @EventHandler annotation is for FML lifestyle events (not what you want). The @Subscribe annotation is for in-game events, and further you need to make sure your event handling class is registered to the appropriate bus -- there are several buses so that single event buses don't get overwhelmed with other event types.
-
What are you trying to do? Are you actually trying to handle an event? You can't handle an event the way you wrote it. Just calling the method "event" doesn't make it an event. You have to use the @Subscribe annotation to indicate that the method might be an event handler and then the parameter passed must be an event (like LivingHurtEvent). I have a tutorial on event handling here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html
-
[Solved] Entities moved in same loop don't update at the same time.
jabelar replied to don_bruce's topic in Modder Support
I honestly didn't go through all your code. But a couple things come to mind. First of all, why are you sending your own packets to sync positions? Why can't you rely on the built-in movement syncing? If you're sending your own packets, I could understand where they might conflict with the packets the server is already sending. Are you sending packets for all the parts, or only the parent? Because maybe you already did it this way, but instead of having the children try to catch up with the parent, you should have the parent re-position the children. If such code runs on the client I would be very surprised if they would get out of sync. Like in all cases in modding, it is best to examine how a similar vanilla mechanism works. So if you look at EntityDragon, you'll see that the EntityDragonPart class is really quite dumb and all the movement of the parts is done in the parent EntityDragon's onLivingUpdate() method, with the following code: this.dragonPartBody.onUpdate(); this.dragonPartBody.setLocationAndAngles(this.posX + (double)(f16 * 0.5F), this.posY, this.posZ - (double)(f4 * 0.5F), 0.0F, 0.0F); this.dragonPartWing1.onUpdate(); this.dragonPartWing1.setLocationAndAngles(this.posX + (double)(f4 * 4.5F), this.posY + 2.0D, this.posZ + (double)(f16 * 4.5F), 0.0F, 0.0F); this.dragonPartWing2.onUpdate(); this.dragonPartWing2.setLocationAndAngles(this.posX - (double)(f4 * 4.5F), this.posY + 2.0D, this.posZ - (double)(f16 * 4.5F), 0.0F, 0.0F); -
[Solved] - Detecting asymmetrical multiblock pattern
jabelar replied to xilef11's topic in Modder Support
Since all the blocks in the patterns will be specific blocks from my mod, I was thinking I could define the patterns in a way that all blocks in it need to be "connected", and using some sort of search algorithm (breadth-first seems logical?) around the clicked block. Not sure how well taht would work though. Yes, if there is some additional "rules" about the patterns besides simple matching, then yes there may be opportunities for more efficient searching. Like if the pattern has to be connected, then you start by just finding the connected parts and that would help define the area of the full pattern. That would be a big efficiency because you wouldn't have to check every shift of the pattern. Note however you still have the possible issue of two connected patterns right next to each other that look connected. That would be extremely hard to figure out logically which part is the pattern area. A 2D array is certainly a good way to represent the pattern, and the pattern to be matched. If you create a couple functions to rotate the array and check symmetry or check pattern, then you could have some very clean code. For this sort of problem, the simpler and more understandable your code is the more likely you are to not introduce bugs. Doing this in a convoluted way could be extremely buggy... So I would define 2d array fields for all the patterns you want to detect. I would make a method for rotating by 90 degrees (you could use that multiple times to rotate more. I would create a method to detect the pattern area (which would be much easier if all patterns were connected, as discussed above) and then create a method for detecting the pattern that simply loops through the patterns you want to detect with all rotations (and for good performance escapes from the loop as soon as it finds a pattern). -
[1.7.10] Change damage type of lightning for all entities?
jabelar replied to IceMetalPunk's topic in Modder Support
In 1.8 they added a lightingBolt damage source. So doing this mod would be much easier in 1.8 because as diesieben07 mentions then you could just handle the living hurt event and check for that damage source. I think in 1.7.10 you might get the most control by actually replacing the vanilla lightning bolt entity with your own, but that might require a lot of coding (or at least copying). You're also right that it is bad that the fire field in Entity doesn't have a getter function (someone should submit a PR for this), while it does have a setter function. As diesieben07 mentions you should probably use reflection, but theoretically since there is a setter function you could create your own field to keep track of what it should be and call the setter function. -
[Solved] - Detecting asymmetrical multiblock pattern
jabelar replied to xilef11's topic in Modder Support
You still have the issue of defining the "search area". Like if you find one block of the type for the pattern, how would you know if that block was from the corner, and edge, or somewhere in the middle of the pattern? So your problem isn't just rotation, but also matter of shifting in each direction. Anyway, assuming you don't have pre-defined area where the structure might be built, then logically there is no more efficient way than checking for every pattern in every shift and rotation. If you think about the logic, the only way to prove that any of the patterns exists is to check for them all. Again though, in terms of performance, you can keep things a bit more reasonable by only checking for patterns when a block is added to the world, rather than every tick. And furthermore, you can spread the checking out over several ticks after that event. Lastly, the logic should be that you assume the pattern is there and then escape the check as soon as you determine the pattern isn't there. For most patterns you'll only have to check two positions per shift-rotation combination because most of the time there won't be a pattern. But my main point is that you can't get away from the logic that you have to check for presence of every pattern shift and rotation. -
Note I have a tutorial that explains a bit more about the localization keys and a couple of mistakes to avoid and tips (like if you want special characters) here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-localization.html
-
You can't just make up your own method and hope it gets called by Minecraft. You need to @Override an existing method. There is no such thing as public ItemStack onItemUse(ItemStack itemstack, World world, EntityPlayer player) method in the item class. There is a method public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) which you can override though. You must get in the habit of using the @Override annotation on every method that is supposed to replace a method from the super class. Then your IDE (like Eclipse) can warn you if you didn't define a method that exists.
-
[1.7.10] Cannot get this hanging entity to work for the life of me
jabelar replied to PhantomTiger's topic in Modder Support
You need to learn to use the debugging mode in Eclipse. You can create breakpoints to stop the execution, or step slowly through the code and give you time to examine the value of the fields. You can then work backwards to understand why a certain value happened. In this case you want to know why it is null. You must expect the value to be set somewhere, so you should set a breakpoint just after the code you think is supposed to set the value and set another breakpoint just before the null pointer exception. If your first breakpoint doesn't happen before the second breakpoint, then it confirms that the value isn't getting set. You can then step through and figure out why. -
[Solved] - Detecting asymmetrical multiblock pattern
jabelar replied to xilef11's topic in Modder Support
There are a lot of further clarifications you need to give about what you are trying to achieve: - What do you consider symmetrical? I would consider the diagram you drew in your post as being symmetrical (since it is mirrored across). Do you mean symmetrical in both x and y together? So a circle would be symmetrical but a triangle would not? (Just pointing out that the mathematical definition of symmetry means that there only has to be one axis of symmetry to declare something symmetrical...) - Are you also looking for specific patterns? Or just looking for symmetry in any arbitrary pattern? - Is the pattern expected to be in a specific location or do you have to detect the symmetrical structure anywhere in the world? - Is there a size limit to the structure (performance will be greatly impacted if you're trying to check for arbitrary structures more than a few blocks per side)? What if structures overlap or are next to each other, how do you define where one begins and next one ends? - Do you consider a single block symmetrical? I think the first performance tip you should consider is you should only check for the pattern / symmetry when a block is placed, rather than always searching for it. So I would do this in detection code in an event handler for block placement. I think the big challenge is not the actual symmetry part (I'll explain how to do that more efficiently) but figuring out where the structure is. For example, if your maximum size of the structure was 5 x 5, let me show you the problem if you had only a couple blocks in the structure. Here is some block placement: ---------- ---------- -X--X----- ---------- -X--X----- ---------- ---------- ---------- -------X-- ---------- Is the pattern above a symmetrical 4 x 4 pattern with some other block that isn't counted, or is it an asymmetrical pattern? Do you understand my point? It is hard to define exactly what area you should be looking for symmetry on, because you can find symmetry in the smaller area. Anyway, once you have figured out the area to check, I think there are more efficient ways to determine symmetry. The first thing you need to figure out is the axis of symmetry: I would do it by checking "across" the area for symmetries. Like if you found a block at position 3 , 1 in a 5 x 5 area, then you should check if there is a block at position 3 , 4 and if not then there cannot be symmetry around the x-axis, and you would also check if there is a block 2, 1 and if not then there cannot be symmetry around the y-axis. If you find there is no symmetry around either axis, then you're done -- just checking for symmetry on that one block proved there is no symmetry. If you find there is symmetry around one or both axes, you continue but now adhere to the axis of symmetry: find another block (if there is one) and check across the known axes of symmetry. If there is not matching block across, then you know there is no symmetry for that axis. I believe this would be very efficient. In most cases just checking two block positions would prove there is no symmetry and you're done. The worst case is you have to check all the blocks across the axis of symmetry, but that still isn't that much and wouldn't happen too often. Did you understand what I'm suggesting? Now if you wanted to get fancy and also consider symmetry around diagonal axes, you would just extend the concept. Hope that helps. I greatly suggest that you only look for symmetry in pre-defined areas that are relatively small. Otherwise, you will have to do all the above multiple times around any block because you won't know where the possible axes are. Think of this: if you don't know the size of the area, how can you know where to check for symmetry? You can't because symmetry has to be across the half-way, and you don't know where half-way is without knowing the size and boundaries of the area you're checking. -
[1.8.8] ResourceLocation as a directory [SOLVED]
jabelar replied to RANKSHANK's topic in Modder Support
I didn't say to look at IResourcePack. I said to look at the resource manager, which works on resource locations. It is the same thing that Minecraft uses to find all the block models, entity models, textures, etc. It looks in a folder and gives a List of all the resources in it. -
I'm not into that sort of thing. Not sure Fred is either.
-
[1.8.8] ResourceLocation as a directory [SOLVED]
jabelar replied to RANKSHANK's topic in Modder Support
I have some explanation of ways to access resources here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-modding-tips_9.html The key point is that Minecraft has a resource manager class that provides various methods. One of the methods gets all the resources: Minecraft.getMinecraft().getResourceManager().getAllResources(). I think it maybe can help do what you want. -
[PARTIALLY SOLVED] [1.8] See who added a block in the world
jabelar replied to JimiIT92's topic in Modder Support
To figure out if redstone is connected, you should look at the vanilla code for redstone and understand how it calculates what is connected. -
[PARTIALLY SOLVED] [1.8] See who added a block in the world
jabelar replied to JimiIT92's topic in Modder Support
I think first of all I want to make sure you understand some important concepts. The reason your first idea of recording the player in a player field wouldn't work is that you need to understand that there is only one instance (a singleton) of the block's class used, so any fields in the block itself will act globally (affect all blocks of that type in the game). This is because there are so many blocks in the game -- there are 65,000 blocks just in a single chunk -- that the computer would not have enough memory to instantiate them all as separate instances. So instead, there is a map of block types to locations in the chunk, and chunks in the world. They have also allowed a tiny bit of data (just four bits) to be stored per block location -- this is often called metadata. So then something like the color of clay block doesn't need a TileEntity, but rather can just use metadata. So for blocks that don't do any processing (i.e. unique data per block location) that is all that is needed. But in your case, you want unique player information associated with each block location, and this is too much for 4 bit metadata. So they have provided TileEntities which are designed to allow you to make some blocks unique. You still can't use a lot of them, otherwise they would cause same problem as creating multiple block instances. Note that a TileEntity can just store data, or it can do some actual processing (like do things each tick). In your case you probably don't need them to tick, just store the player UUID, which is good because ticking a lot of TileEntities can cause performance problems. There is an alternate way of storing data per location though. You could create a map in the world data that associates block positions with player UUIDs. Note that in both approaches (TileEntity or world data) you will want to make sure you save and load the information as part of the NBT save / load processes. Now regarding the redstone part, it actually is possible, but it would require quite a bit of programming. One approach is to substitute custom blocks for each redstone and redstone activated element and have them either have TileEntities or have them interact with world data. For example, you could have a world data map that represents who activated each pressure plate, and in your block that substitutes for the pressure plate you could update that world data map. It would take a lot of programming though to achieve. Also you have to consider that redstone circuits can actually be activated by multiple things simultaneously -- imagine two pressure plates where one player gets on, then another player gets on, and then the first player gets off. Which player would you consider as the one that activated the circuit. So not impossible, but really probably an advanced mod to make. -
[1.7.10] Cannot get this hanging entity to work for the life of me
jabelar replied to PhantomTiger's topic in Modder Support
Glad you made progress. Yeah, I find it is best to not get to fancy with self-registering classes but instead do the registering in the proxy where you can control the order and be sure it is being called. -
Making mod not show up in Minecrafts mod list?
jabelar replied to CacaHuate's topic in Modder Support
Why? I feel that any mucking around with the main menus is not something most users would like. -
Unfortunately, I don't think you can do this without severe, crippling lag. Basically what you're making is like a climate model and that is best done with massively parallel supercomputers. What you have to realize is how many blocks of air there actually are. In a single chunk there is 65,536 blocks, with probably three quarters of those air blocks! If you want those blocks to be actively processed (like calculating spreading out, calculating mixing, etc.) it would be crazy. That is why Minecraft has TileEntities, because it simply can't make all blocks actively processing. (And TileEntity won't really help you because once you have enough of those it would be same performance issue). I think if you really wanted to try to do this, you shouldn't do it block by block but rather much larger groups like per chunk. Then you might have a chance. Like you could have a map in your world data where the atmosphere of each chunk is stored, and you could do some simple calculations, like mixing with neighbors.
-
I think you missed the point I don't want to create a wand, what i really want is to make a block (ore) that has a charging effect and it can electrify you when you try to mine it and I just want the shocking effect from thaumcraft Okay, well I'd give the same advice. Start with the basics. Okay, I'll assume you want something like the charging effect from the "node" in Thaumcraft. A node has a certain amount of charge and certain type of "focus" (type of charge). Also, the model / texture are animated. If you want that sort of thing (i.e. the charge can drain and there are different types of charge) then you will need to make it a TileEntity. So go ahead and create the custom block and tile entity, along with the fields you want (charge level, focus type and animation step), make sure those are saved and loaded via NBT, and make sure the tile entity is enabled to tick. You'll also need a field to identity the current target of the charge (i.e. which player it is charging in your case) Then in the update() method of the tile entity you can check for whether a player is close enough, set it as the charge target and start transfering charge. If you want the player itself to accumulate charge (instead of a staff or item that the player is carrying), then you would need to use IExtendedEntityProperties on the player to save the charge level. If you want it to look like a block (rather than floating pulsating energy like in Thaumcraft) then you'd just use the regular block state and block model system. If you want to animate it, just create a block state for the animation and a bunch of madels / textures and cycle through them. If you want to draw things that are more interesting, like the lightning that shoots out or the sphere that protects nodes, then you'll have to use GL code in the rendering events. I have an example of how to render a sphere here (look down the page to the "render a sphere" section: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-quick-tips-gl11-and.html For a lightning effect, lightning is just a set of small connected lines that are a bit random. So do web search on how to draw a line (or laser, a lot of people ask about lasers and it is similar idea). Then when you want to shoot lightning, you would create a list of coordinates that are a bit random along the line between the player and the ore, and draw lines connecting those up. You can alternatively create textures of lightning and apply those to a long skinny plane between the block and player.
-
[1.7.10] Cannot get this hanging entity to work for the life of me
jabelar replied to PhantomTiger's topic in Modder Support
Yeah, that is what I meant. I'm quite sure that you need the rendering registering to happen in the init phase, so I think it is good you moved it. Entity registering should happen in the pre-init phase. You're now talking about TigerEntity but in your previous posts it was something called PapPapEntity. In your first post you show some code for a createEntity() method that does the registering, but it is not clear to me where you're calling that from. Anyway, there is another thing I'm a bit concerned with. You have a @Instance annotation without giving the mod id. I usually use @Instance(MODID) where MODID is constant containing my mod id string. I'm not sure what happens if you leave it blank. The default is is "" so perhaps your instance field is not being set properly which might be screwing up your registration? Just a wild idea really...but worth a try. -
Yeah, same here. I'm bit myopic about this -- mostly I mod considering my own mod(s) but the ore dictionary is a nice future-proofing mechanism.
-
[1.7.10] Correct way to get block under the player?
jabelar replied to American2050's topic in Modder Support
That is the code I already gave him. Copied from the vanilla code. The only issue, like I mentioned, is that for some custom entities you need to make sure you factor in the bottom of the bounding box better. -
[1.7.10] Correct way to get block under the player?
jabelar replied to American2050's topic in Modder Support
If you want to consider jumping as well, you might want to try ray-tracing instead. Basically you start at the player and keep checking downwards until you find a block that isn't air.