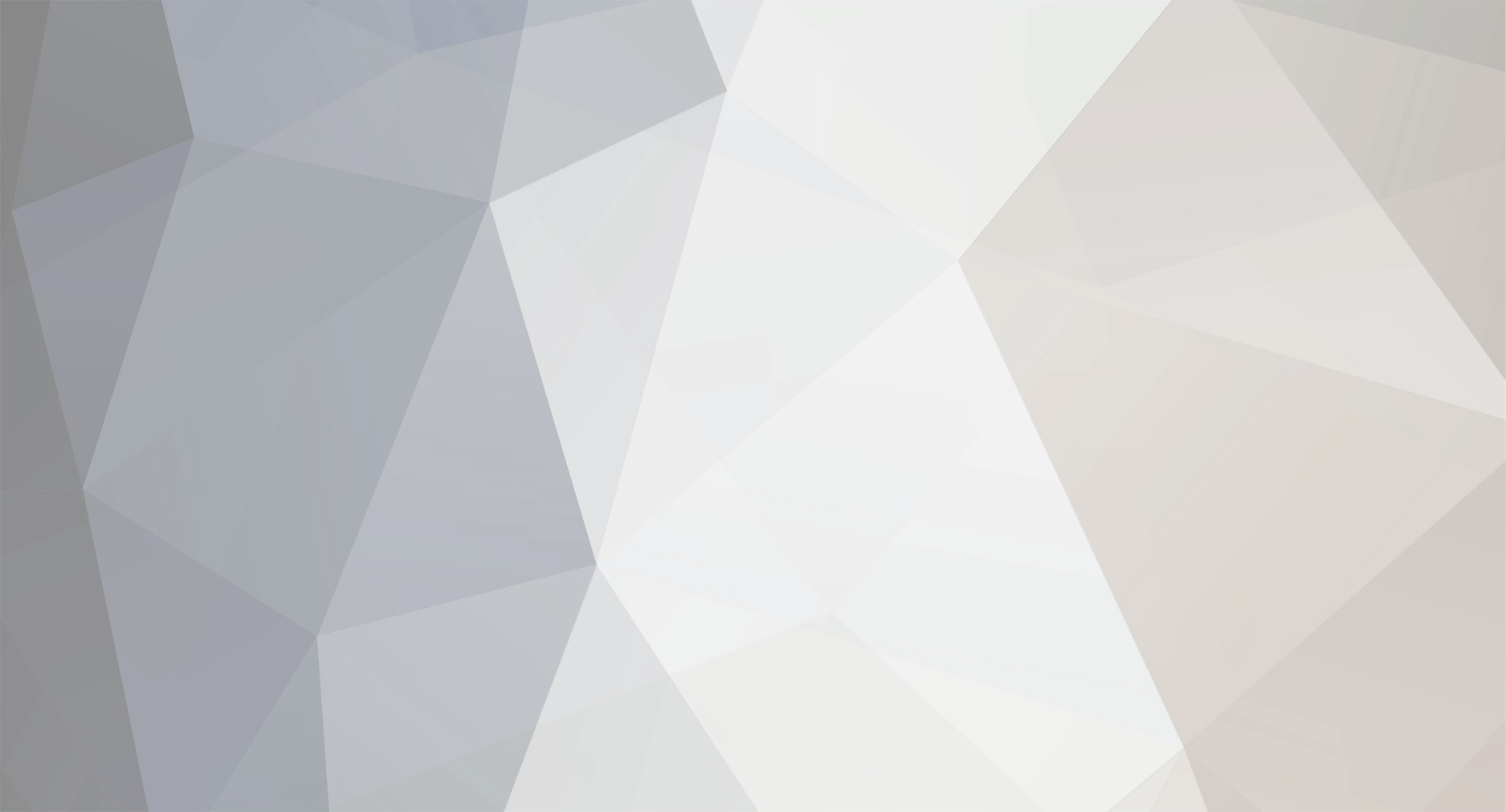
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
[1.7.10] [Newbie] Is it possible to disable/enable other mods
jabelar replied to Loke's topic in Modder Support
It really depends on the mods that you're considering. If it is mostly about making items, entities, and recipes available over time then you can generally do that using the various registries. The reason why there is a "post-init" phase of the mod loading is to allow mods to interact with each other with confidence that they have been loaded already. You can also check whether a mod is loaded and if it is you can start adjusting the registries to look for things from that mod and edit them according to your need. But if the mod affects gameplay directly, you probably won't be able to simply turn it on or off unless you're lucky (like if they use events you could possibly make your event handler higher priority and intercept the event before it gets to their mod). But in the more complicated case you'd probably have to work with the other mod's author to support your need if they choose to. By the way I think your idea is extremely neat idea. -
Your configuration code looks correct to me. What do you mean you want the entity to disappear? Do you mean you create a world with the entity in it and then change the config and load the same world? Or do you mean you create a new world but still get the spawn egg for the entity even though the config is false? I'm not sure that not registering would delete an entity that is already existing. In other words if the entity is created in the world and then you change the config and reload the world I think it would still be there. If you want it to go away, I think you might want to use an event to get rid of the entity when it is trying to be constructed or join the world.
-
Yes, that's what I mean. Yes to keep it connected you would need to ensure that the head can't move more in a tick than half the length of a segment. The problem with the alternative is the issue of colliding with blocks. The head will do actual pathfinding, but you don't want the next segment to move straight to the head position because that might go through a block. The nice thing about the head's previous position is you know it is the actual path the head took. The video link you shared didn't have the same problem because the parts were just arcing in the air. But imagine a snake slithering through some bumpy terrain. I suppose another way would be to have each part do some path finding. So the first part does its own thing, then next part finds a path to the head, etc. However, I think that the pathfinding may be difficult if it thinks the parts are going to collide and it is not clear to me that you could really do it properly. For example, you don't want the next segment to go to the middle of the head, but rather connect to the base of the head. Also, it would be difficult to know the rotation angles. I guess the ultimate is to make your own pathfinding algorithm for the head that saves the path (not necessarily the last tick position, but also all the points in between) and then places the body segments along that path. It is actually a pretty tricky problem. That is why the multi-part vanilla dragon is flying and goes through blocks -- then it doesn't have to worry about parts navigating a complicated path. Ultimately this is Minecraft though and the imperfection is the charm of the game. So if you have a blocky snake that doesn't perfectly connect most people would still appreciate it.
-
I would move the head entity using regular navigation and if the head moves, then move all the pieces to the position that the preceding part just had. Note that you only want to update the positions if the head moves otherwise the whole thing will compress -- the head will stop then all the other parts will keep moving until they're in same position. And then just rotate each part to line up between the part in front and the part behind. I think that should be all that is needed.
-
How to get list of all registered mod entities?
jabelar replied to jabelar's topic in Modder Support
Hmmm. You're right. For some reason I was looking at the code and thinking EntityRegistry was contained within itself. But you're right it also uses EntityList. EntityList also directly registers all the vanilla entities so I guess that is what got me thinking in the wrong path. Anyway, yes it looks like the mappings are public so I can iterate through them. -
So I want to remove some spawns but would like to do it with a loop instead of hard-coding every entity. So I'd like to loop through all the entities and remove the spawns. But my difficulty is in getting a list of all registered entities (vanilla and other mods). I think EntityList is only for vanilla entities now (and any mods that still use global ids). But it seems that the list for mod entities is kept in a private map called entityRegistrations in EntityRegistry class. I can use reflection I guess but I like to know if there is a more proper way to get the list of all registered entities. Anyone know? After looking at it I think I actually should approach this the other way around: instead of cycling through entities, instead cycle through all biomes and creature types? Seems that those have public methods and fields I can work with... But I still am interested in the general answer -- is there a public way to get list of all registered mod entities?
-
And networking also runs on separate thread now.
-
[1.7.10] Rendering many mobs ... they are all rendered synched?
jabelar replied to Frag's topic in Modder Support
With animation you really don't need to do math. You can just make an array containing all the angles in the cycle then step through them. I find that is a lot easier to "visualize" and also works for irregular movements whereas math mostly works for very regular movement. This is also the way that real animators work -- they create a series of poses they like and cycle through them (like one of those books that you flip to animate a cartoon). A fish is fairly regular in its motion so math works. But I bet it will take you more time to figure out the sine and cosine stuff than just having a cycle hard-coded. I have a tutorial using the array-based pose method: http://jabelarminecraft.blogspot.com/p/introduction-first-of-all-to-understand.html -
Questions About Copyrighted Content in a Mod.
jabelar replied to CausticLasagne's topic in Modder Support
I agree with most of what is said above. In addition to general copyright you need to look at the EULA license with which you obtained the content as it could (likely does) contain additional restrictions. Like music downloads might explicitly say to not use it for incorporation into software/games. Realistically though, unless your mod is distributed widely, the copyright violation is notable (e.g. Star Wars mod that uses all the music and characters from the movies), and causes actual damages the worst case is likely to just get cease and desist order. But any interaction with legal issues is worth while trying to avoid. I'd suggest that there are lots of musicians and artists who are interested in contributing to mods under a free license so why not reach out to some. It can be fun to collaborate. -
I have a tutorial on using events to change drops of vanilla mobs: http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-changing-drops.html
-
You can only use a loop if there is something "regular" (i.e. predictable or possible to calculate) about the sequence, or if all the information is already stored in a collection/array. But if you just had the items in the array but not the egg color then a loop won't help because it wouldn't be able to figure out the egg color. In other words, you can't save much typing with a loop for spawn eggs because for every item you'll still need to type somewhere the unlocalized name and the egg color and associate that with each actual item. Whether you type that in the preinit handler, or within the item constructor won't avoid the fact you have to type it. Now if the colors could be calculated -- like you could randomize them from a fixed seed so they always turned out the same -- then a loop makes sense.
-
I agree that material should work with vanilla liquids but I think there is more chance that other mods create new materials for custom fluids whereas it is unlikely that they wouldn't extend or implement fluids. So I think maximum compatibility would be using instanceof.
-
Actually, CheckSpawn is still giving me some trouble -- it seems to be firing and setting result to DENY but when I make new world it is still full of chickens, pigs, etc. I noticed CheckSpawn is not fired when the "preparing spawn area" messages are happening, but only later when there is a tick. If you look at the call hierarchy it seems that CheckSpawn isn't fired during world gen. My code for the event handler: @SubscribeEvent(priority=EventPriority.HIGHEST, receiveCanceled=true) public void onCheckSpawn(CheckSpawn event) { if (event.entityLiving instanceof EntityDinosaur) { if (MyMod.spawnDinosNaturally) { event.setResult(Result.ALLOW); } else { event.setResult(Result.DENY); } } else { if (MyMod.spawnNonDinoMobsNaturally) { MyMod.instance.getLogger().debug("Allowing spawn for non-dino"); event.setResult(Result.ALLOW); } else { MyMod.instance.getLogger().debug("Denying spawn for non-dino"); event.setResult(Result.DENY); } } } And it is definitely getting called in game (although not during "preparing spawn area" as I mentioned). I know this because the logger sptis out continuos stream of "Denying spawn for non-dino". So what should I do to prevent generation in the first place? I'm thinking using the removeSpawn from EntityRegistry is cleanest way...
-
I would use instanceof checks instead of material checks. I would check for both BlockLiquid and BlockFluidBase. That should cover most vanilla and mod "liquids". If you want an exception (like if there is some BlockLiquid you don't want to act the same way, then additionally check for whatever you want.
-
Okay basically I just want to make a configuration where either only my custom mobs spawn naturally or every mob spawns naturally. I always think that the entity join event will do the trick, but then realize that it seems to be fired not just for spawns but also whenever chunk loads -- right? So if a player spawns a mob with a spawn egg then if that chunk has to load later it would disappear. Right? Sorry but I always get confused on what "join" means exactly. The entity constructing event doesn't help because it isn't cancelable, although I suppose you could do something like set it dead right away? Anyway, I think the best way is to actually use the removeSpawn method in the EntityRegistry, right? That isn't too hard, so I'll probably do that. However, while looking at EntityRegistry I see a method called setCustomSpawn() which seems to allow you to fully override the spawning functionality. Has anyone used that before? It seems like it could be pretty powerful if you could fully take over the spawning decisions...
-
[1.7.2] Replacing vanilla abstract method in EntityLivingBase
jabelar replied to Sokaya's topic in Modder Support
I think a different approach would be to cancel the regular potions use item functionality (i.e. with the PlayerUseItem event) and replace it with your own. You wouldn't need to change any vanilla functions, just they'll never get called but yours will. -
[1.7.10] Another setsize weird behavior question ...
jabelar replied to Frag's topic in Modder Support
It has nothing to do with your render scaling. There is no real interaction between bounding box and rendered model. There may well be a limit on how small the bounding box can be. There certainly is limit to how big you can make it. The thing is that the bounding box is used for path-finding and to make the path-finding code efficient they can't allow too extreme of size and also don't rotate the bounding box and lastly make the X and Z to always be same. So there is probably some pathfinding that is messing up and then moving the entity. Anyway, you should test whether it is the height or the width of the bounding box that is causing the problem. Because in worst case you can at least make some of the dimensions match more closely. -
First of all, what kind of entity are you making? Or are you trying to do this to all entities? You're extending entity, but if you really wanted to do this for a mob or living entity you need to extend the proper subclass, like EntityMob or whatever. But extending is for making your own entity -- is that what you want to do, or do you want behavior of vanilla entities to change? Basically I'm saying that extending a class is used for making something new, in this case a new entity.
-
How to enable "debug" logging in eclipse?
jabelar replied to Bedrock_Miner's topic in Modder Support
Yeah, that is the way I thought I tried a few times. Basically supercede the xml with my own. However I never quite got it to work. Although I'm pretty sure I tried each of the things you mention, I may have missed putting them together in combination correctly. I'll try it out. -
Looking for some guidance regarding hierarchy and code organization
jabelar replied to DavidTriphon's topic in Modder Support
Classes that have @SideOnly annotation for Side.CLIENT should go in client. All others in common. Examples of client-only classes are models, renderers, GUI, key handlers. Basically things that either affect the display or process input are usually client side. So for example, the entities folder under client would have the models and renderer classes under it, whereas the entities folder under common would have the entity class itself. Regarding your question about how the proxy works, I have a information on the subject here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-17217x-quick-tips-for.html -
Looking for some guidance regarding hierarchy and code organization
jabelar replied to DavidTriphon's topic in Modder Support
Well first of all, most programmers end up with a distinctive style. Some styles can have negative consequences (like being prone to errors, or being hard to maintain) while others are simply choices that are equally good. If you're the only coder on your mods, then usually you can express your own style, but when doing a joint project it is important to establish an agreed style / organization for everyone to follow. In fact in multi-coder projects you usually have to make sure your IDE has same code style settings as well because all the automatic indenting and such can make the version control system think changes are being made when they aren't. Anyway, beyond that I'd say the next question is whether it will be a complicated mod or a simple mod. Mostly complexity can be measured in the number of classes. So if you're just adding a single item or something you probably can use a flat hierarchy. However, in my experience even simple mods will get added to so it is best to organize like a big mod even if you think it will only be small. So, assuming you're organizing for a big mod, most teams I've seen do the class hierarchy as follows: 1) top level has mod class and package folders for client and common 2) under each of client and common you have package folders for each type of thing. Like Entities, Blocks, Items, Packets, Particles, GUIs, etc. 3) if you have a lot of any particular thing you might have one more level of package folders. Like under entities you might have a birds, big cats, serpents, etc. That's pretty much it. Note that from a pure coding perspective, package organization is usually more important because there is usually "package private" scope information that allows classes in same package to access each other. But in Minecraft modding people seem to freely make public scope access so this isn't really something needing thought for modding. There are lots of other style questions. Like some people like to register their things from within the constructors. Some people like to create registration methods within each thing, then call those. Mostly these are all valid choices, but sometimes they have impact. Like I like having explicit control of registering because sometimes the order matters (like crops need the block registered before the seed item). But frankly you sort of need to encounter these issues yourself to really figure out how you prefer to tackle the problems. -
You should always post the error output when asking a question. The error usually says exactly what's wrong. Usually the problem with checking items is that it is possible that the itemstack is null. So when you call .getItem() you'll get a null pointer exception. So generally you should first check if itemstack is not null and then do the rest of your code.
-
Yep, that is all right. Regarding the message payload:The bytes in the packet are in a ByteBuf which has methods for writing and reading various data types and there is a class called ByteBufUtils which allows you to write and read NBT and such. So you handler takes the ByteBuf and reads out the data types in the same order you wrote them in and stores them in custom fields that you can then work with.
-
How to enable the mod Disable button in the mod list
jabelar replied to colinvella's topic in Modder Support
True but when programming a large project it is often the case that one feature isn't fully implemented but it is good practice to handle or hook into it anyway so it works properly when one day someone does implement it. So if you wanted your mod to have disable functionality, you might as well hook into this (e.g. in this case put in the mod annotation). Furthermore, I'm thinking you should be able to actually make the disable do something, assuming disable just meant "do nothing" rather than a something like fully unloading the mod. Can't you check for gui screen open event and then for button click events?