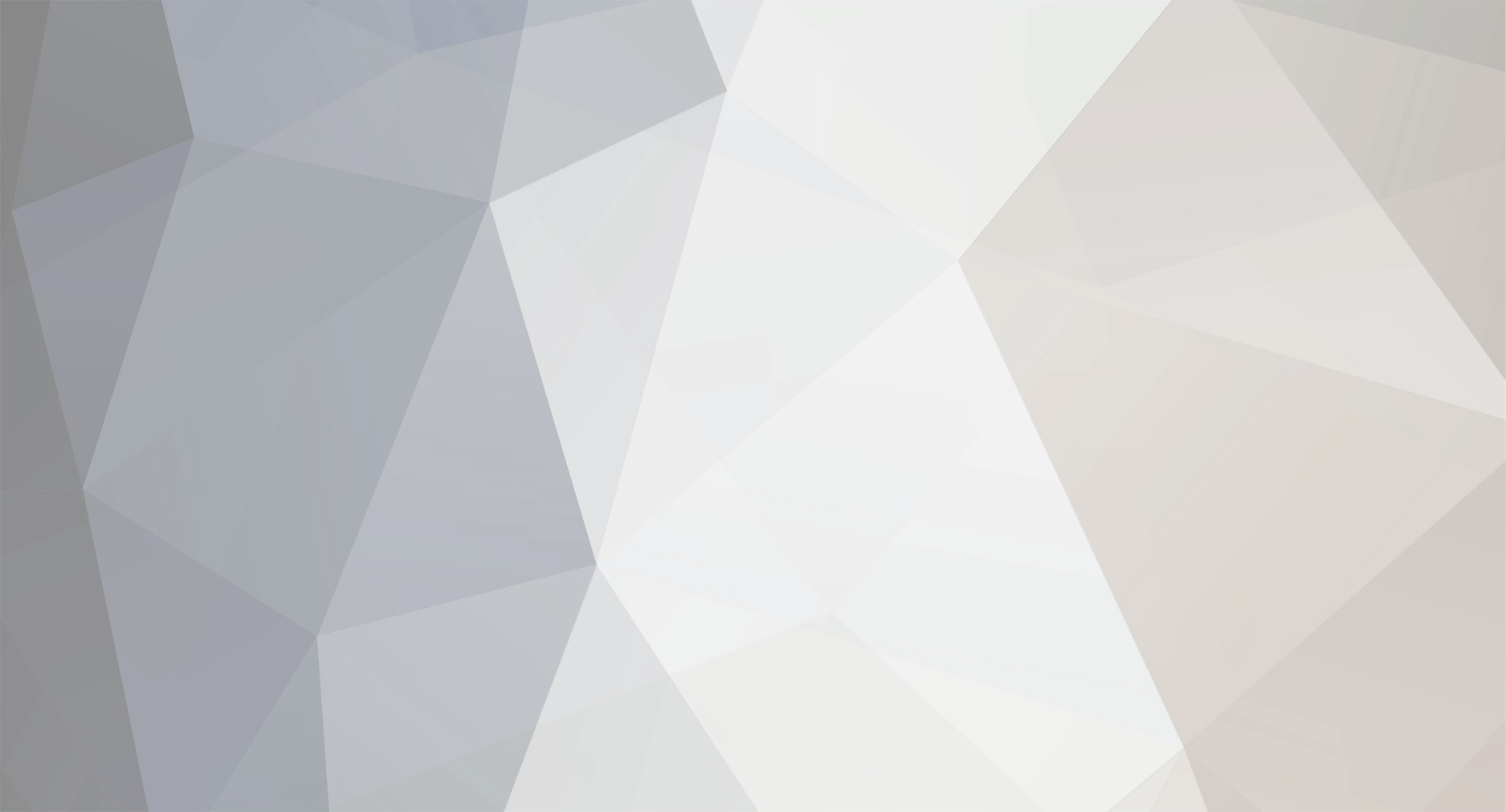
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
The growable animal mobs scale the body and head differently. If you look at ModelWolf, it has this code: if (this.isChild) { float f = 2.0F; GlStateManager.pushMatrix(); GlStateManager.translate(0.0F, 5.0F * scale, 2.0F * scale); this.wolfHeadMain.renderWithRotation(scale); GlStateManager.popMatrix(); GlStateManager.pushMatrix(); GlStateManager.scale(1.0F / f, 1.0F / f, 1.0F / f); GlStateManager.translate(0.0F, 24.0F * scale, 0.0F); this.wolfBody.render(scale); this.wolfLeg1.render(scale); this.wolfLeg2.render(scale); this.wolfLeg3.render(scale); this.wolfLeg4.render(scale); this.wolfTail.renderWithRotation(scale); this.wolfMane.render(scale); GlStateManager.popMatrix(); } else { this.wolfHeadMain.renderWithRotation(scale); this.wolfBody.render(scale); this.wolfLeg1.render(scale); this.wolfLeg2.render(scale); this.wolfLeg3.render(scale); this.wolfLeg4.render(scale); this.wolfTail.renderWithRotation(scale); this.wolfMane.render(scale); } } Notice one of the key points is that there is a push and pop of a matrix. In GL11 there is a "stack" of transformations and if you just want to operate on one part you will want to create a new matrix to perform that transformation. In other words, you push the matrix and scale and render the part then pop the matrix and render the parts you don't want to scale.
-
[1.8.0] How to allow EXISTING in game items to be used as fuels
jabelar replied to boredherobrine13's topic in Modder Support
Fuel for what? You want it to fuel existing furnaces, or you've created your own machine/furnace that needs fuel? -
Vec3 does have a lot of math methods though. Are you sure none of them work for your case? Looking at the type hierarchy there are subtractions, additions, dot products, multiply, get intermediate, normalize, etc.
-
I do exactly this -- switch from laptop to desktop. The best way is you should use Git or similar online revision control method. Basically, your code (and other files needed to build) are stored on github or bitbucket and then git keeps each of your computers in sync. It is the same way multiple programmers can work on same project from different computers. I have a tutorial on setting this up. Instead of using git directly I recommend using a graphical interface that has embedded git called SourceTree. This is free, as is github. It isn't so hard to set up and is really useful. It also provides full revision control that allows you to revert mistakes, selectively merge, maintain common code for multiple versions of Minecraft, and so forth. Here is the link to my tutorial: http://jabelarminecraft.blogspot.com/p/minecraft-forge-publishing-to-github.html
-
I have a tutorial tip where it mentions some further details about setting up the run configurations. There are usually some additional arguments and such you want. Look halfway down this page: http://jabelarminecraft.blogspot.com/p/quick-tips-eclipse.html
-
Hey, good to hear from another "old guy" who is into Minecraft modding! In terms of credits, I don't think you need to go too far with it. You don't need to thank the people who developed the Java language and stuff of course, and I don't think the creators of Minecraft really need it either (they're a large company and the creative people already reaped significant reward through the commercial success). But FML and Forge are actually done by a small set of people in the community for no commercial reward, so it would be nice to thank them. Regarding your questions about how the FML/Forge API came to be, diesieben07 and LexManos can give much better explanation, but here is what I understand: - MCP: Even after deobfuscating the Minecraft JAR the method and field names are cryptic (you see things like p_23435_a) so the community has created a mapping called MCP to make the vanilla code more readable. MCP is the mapping of "SRG names" to readable names. Every time there is new version they have to do it again, so at the early part of adopting a new version there is a lot of stuff unmapped. (Note for this reason, it is important to specify the newest mapping you can in your build.gradle file.) - FML: The whole point of this system is that multiple mods can be "loaded" at the same time. It is possible to make different mod loaders, and some people argue that they prefer others, but FML seems to work well. The loader adds dependency helps interleave the pre-init, init, and post-init FML lifecycle events so that you can control interactions between mods. - Forge: This provides all the "hooks" that allow modders to do common things and for the most part play nicely with other mods. Some of the most important parts are the various registries, ore dictionaries and events. There is probably a lot more details and history others can tell us. But hopefully that gives you a working knowledge.
-
Does the entity have to move (by itself)? If not, then maybe a TileEntity is a better approach. Otherwise, you need to look at vanilla entities that resist pushing. Maybe minecart would be interesting. It looks like the applyEntityCollisions() is the method you need to look at.
-
[1.8] [SOLVED] Set maximum number of entities
jabelar replied to colossali's topic in Modder Support
That seems correct. Are you sure the event is firing? You should either trace execution in debug mode of Eclipse, or you should add a console or logger print statement to say something when the code is executed. If it doesn't execute, then it means you registered the event handler wrong. However, is EntityNessie your own class? If it is, then you don't need an event to control this. Instead in the EntityNessie class you should @Override the getMaxSpawnedInChunk() method to return 1. -
[1.8] Natural vs. Manufactured block detection
jabelar replied to tool_user's topic in Modder Support
Well compatibility with other mods would take work, but wouldn't necessarily be impossible. For mods that modify or add to the existing recipe systems, you can still just search the recipes. For mods that use their own recipe system, it would be "impossible" to make a general solution, but if you wanted to cover the major mods that people are using you can handle each one -- basically just detect whether the mod is loaded, and if it is proceed accordingly. Things like uncrafting tables, grinders, etc. usually reduce things to natural ingredients so those might not be an issue. But certainly any custom crafting / smelting that uses a separate recipe system would need you to code for it. But really it wouldn't be that much work. If a certain mod is loaded, you can just add to your list of "manufactured" blocks based on what you know about the mod's functionality. -
[1.8] Natural vs. Manufactured block detection
jabelar replied to tool_user's topic in Modder Support
The important question I have is do you mean to detect something that is manufacturable, or do you want to detect things that were specifically manufactured by a player? Because some manufacturable blocks will spawn naturally in structures and villages. If you just want to check if a block can be manufactured, I think you can do it by just looking to see if there is a crafting recipe that creates it. -
Do you mean generation like biome decoration? Or do you mean a crop plant that you can plant, gross and harvest? If you mean a crop, I have a tutorial herehttp://jabelarminecraft.blogspot.com/p/minecraft-forge-172-creating-custom.html?m=1
-
[1.7.10] Easy way to check if block can stand alone?
jabelar replied to IceMetalPunk's topic in Modder Support
I think the canPlaceBlockAt() method should contain the logic to check whether a neighboring block is required and present. Then when you actually place it, it will convert to the right metadata. You shouldn't have to know the metadata to determine if it a standalone block. -
Agreed. Up to the community to make the documentation better. Thanks for the other links!
-
Sure, the comments on the code is something most of us have read. But that really isn't the same as a definitive guide. While a close inspection now makes the relevance of the deobfuscation clear, it wasn't something that was immediately apparent when I read it in the past because at the time I wasn't thinking specifically ("I wonder if it can deobfuscate"). So then later when I want to do deobfuscate I may not have confidence that Forge Gradle has that capability. If I know that something should be possible, then I'm capable of researching the answer because I know it must be there. So if it was common knowledge that Forge Gradle could deobfuscate we'd have investigated by now. I'm not lazy, I'll do the research and trial-and-error to figure something out, but I still sometimes need to know the initial direction to concentrate on. Anyway, I still think it would be cool to have a solid list of all the Forge Gradle capabilities. I'm certain there are still many things it can do for me that I'm not even aware of... For example, there is also the documentation here: http://forgegradle.readthedocs.org/en/FG_1.2/ but that doesn't mention this topic at all. It would be a great addition to the "cookbook" examples, and furthermore a general capabilities list would be useful there too. To emphasize my point, it took Lex to mention it on this thread -- no one else who responded knew, and I expect most threads on the topic have not mentioned it either. Instead everyone is suggesting getting deobfuscated versions, using chicken core stuff, etc. Yet there was a beautiful way to do it right in front of us!
-
Simply adding the mod as a dependency does that? Awesome! I thought the dependency was more of a check rather than an action. Like Draco said, what is the definitive guide to build.gradle syntax?
-
I also think that the collideWithEntity() method might be able to be used (also with canBeCollidedWith returning true) to adjust the position and keep it fixed and bounce the entity back. You could probably copy the code for how an entity collides with a block. Another, different approach might be to create an invisible block at the same location.
-
Block.isAir method - why does it have parameters?
jabelar replied to BinaryCrafter's topic in Modder Support
I think JeffryFisher's answer is the main reason. I also suspect that some parameters are just left-over from some coding change. I'm sure you have experience of coding a method thinking you'll need certain parameters, then later you revise your code and eventually some parameters become unused. Or maybe that is just me... -
[1.7.x] I want create system my friend in minecraft.
jabelar replied to zlappedx3's topic in Modder Support
Well he speaks better English than I speak other languages, so I won't criticize. It seems clear that he is saying he wants to create a system of keeping track of a player's friends, and is asking if NBT is a suitable data structure. CoolAlias' answer seems correct -- each player could have extended property with a list of friends. Furthermore, it seems that he wants a GUI. So yes, you would just make a GUI that lists people that could be added as a friend, and the GUI could update the player's NBT. If you want to alert the other player that they have been asked to be a friend, then you'd have to use a custom packet. You'd probably need a packet to handle requesting friendship, another for approving or denying friendship, and another for removing a friendship. You could also have a GUI that overlays the game, showing when friends are on the server. -
[1.8] [SOLVED] Confused on creating a custom entity
jabelar replied to Max Noodles's topic in Modder Support
For rendering problems it is also really useful to use the F3+B key combo to enable entity bounding boxes to be highlighted. If you see your entity's bounding box, but not the entity, then you know it is a rendering problem. And to find the location of your entity (a lot of people accidentally spawn them at 0, 0, 0) like Choonster says you should use console statements to print out the position of the entity, then you know where to look. If you never get those print statements, then presumably the entity didn't even spawn. -
[1.8] [SOLVED] Confused on creating a custom entity
jabelar replied to Max Noodles's topic in Modder Support
I have a tutorial that covers a lot of the topics related to custom entities here: http://jabelarminecraft.blogspot.com/p/creating-custom-entities.html There is a lot of things that actually have to come together to make a successful entity, so probably worthwhile to go through the points in my tutorial. Registering the entity, registering the renderers, animating the entity, creating a spawn egg, etc. is all described. -
[Solved] Entities moved in same loop don't update at the same time.
jabelar replied to don_bruce's topic in Modder Support
Okay, but it that is the case you could still spawn all the possible parts during the parent constructor and then enable rendering and collisions selectively as the parts are added. I know that is a bit of extra work, but theoretically should provide a solid result. -
[Solved] Entities moved in same loop don't update at the same time.
jabelar replied to don_bruce's topic in Modder Support
I think it is possible that the dragon has some mismatch that isn't noticeable due to overlap and "organic" shape -- it is much harder to notice a flapping wing is lagging than a fixed airplane wing. However, I don't understand your point about creating the child parts in the constructor. I suppose you're saying that the order of entity registration seems to be affecting which tick the update occurs in, although that still seems very odd to me. Anyway, there is a difference between "constructing" and "registration". You can always go ahead and register all the possible parts at the same time, and then just spawn them (which would call the constructor for the part) when needed. Theoretically you could probably actually spawn them all too, but make them invisible until needed. So if it is really a matter of registration order or constructor order, I think you could still manage it. Another approach is to do all the rendering from the parent (so it will look solidly connected) and have the other parts as invisible entities. The collision boxes of those entities would still be out of sync a bit, but perhaps it would be tolerable. So you could still get fairly good collision processing, while making sure that the visuals are perfect. -
Is there a Forge hook to modify base classes?
jabelar replied to MagnusCaligo's topic in Modder Support
For those who think they need to edit base classes, they need to check out the following first -- i.e. tons of ways to create mod without editing base classes. I usually look for approaches in the following order: 1) Public fields and methods already available. There are tons of vanilla fields and methods that are already public. For example, the AI task lists for all vanilla mods are fully modifiable directly. 2) Registries, Ore Dictionary, substitution aliases. There are multiple Forge mechanisms which allow you to access the available items, blocks and entities and replace them or delete them. 3) Events. There are more and more great events that allow you to intercept the vanilla processing and replace with your own code. 4) Reflection. Java allows exposing the private fields and methods of vanilla classes through reflection. Although theoretically there is a performance impact to this, it is in most cases insignificant. Once you can access the private fields and methods of a class, you certainly have a lot of control. 5) Access transformers, ASM, other advanced methods. Honestly I have never had much reasons to get to the point where i needed these. So, to the OP the question is really: what are you trying to achieve with your edits? -
[1.8 Forge] How to change the player's eye height?
jabelar replied to JelloMOO's topic in Modder Support
Also, 1.8 now has additional events for the view rendering camera. Furthermore, you need to be specific about what you mean by "eye height". There is the camera view that the player sees, and then there is the actual eye height used for look vectors and detecting blocks under the player. -
[Solved] Entities moved in same loop don't update at the same time.
jabelar replied to don_bruce's topic in Modder Support
Okay, well as mentioned before, when modding it is best to check out the vanilla source code for any similar logic in Minecraft. The EntityDragon seems to do something very similar to what you want to do, so I would check out its code.