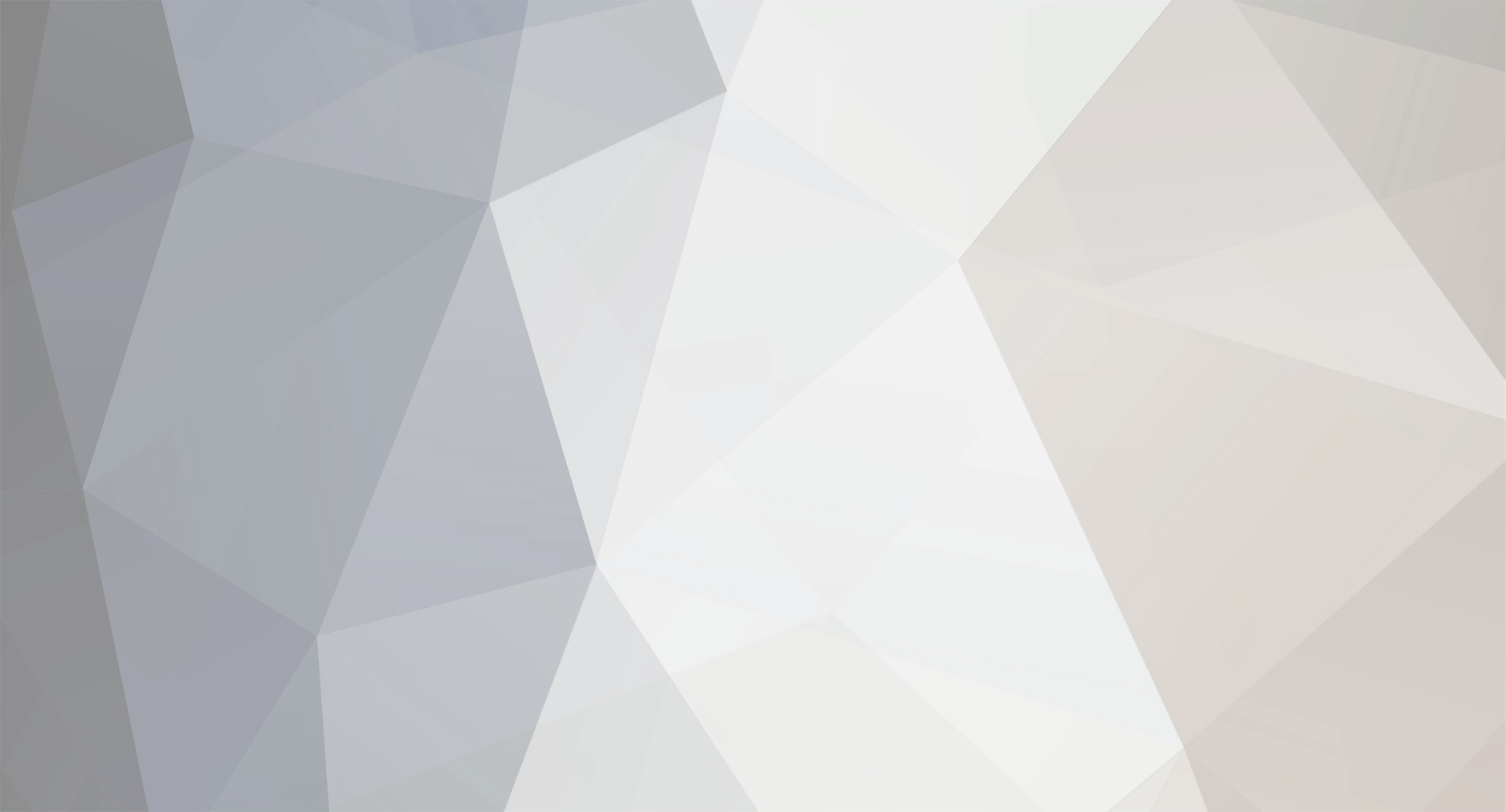
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
[1.7.10] Correct way to get block under the player?
jabelar replied to American2050's topic in Modder Support
I'm not sure you're properly factoring in the Y-offset of the entity. I basically follow what the vanilla code does for this: It is pretty simple really, but I see this question asked and some people get confused because the block position uses int versus the double used by entity position, plus entities have some y offsets that need to be considered. public Block findBlockUnderEntity(Entity parEntity) { int blockX = MathHelper.floor_double(parEntity.posX); int blockY = MathHelper.floor_double(parEntity.posY-0.2D - (double)parEntity.yOffset); int blockZ = MathHelper.floor_double(parEntity.posZ); return parEntity.worldObj.getBlock(blockX, blockY, blockZ); } However, for custom entities it is possible that the yOffset isn't properly set. It may be preferable (suggested by CoolAlias) to use the bounding box instead. So this version is probably the more trustworthy: public Block findBlockUnderEntity(Entity parEntity) { int blockX = MathHelper.floor_double(parEntity.posX); int blockY = MathHelper.floor_double(parEntity.boundingBox.minY)-1; int blockZ = MathHelper.floor_double(parEntity.posZ); return parEntity.worldObj.getBlock(blockX, blockY, blockZ); } -
If you're just starting learning to mod with Forge, I suggest you start by creating the staff item first. Create a new item, register it, get it a good model and good texture, get the crafting recipe working. Thaumcraft is complicated because there is also "focus", "charging" and levels of the staff to deal with. If you want to implement each of those, then you'd make an instance int field for each and store that in the NBT. After that, then it is a matter of just adding functionality when right-clicked, which would include testing the focus, level and charge, and adding functionality to change focus and recharge (this would simply be an update each tick to increase the value of the charge until it hits its limit). But anyway, start simply -- just create a custom staff. Then you can make it fancy.
-
[1.7.10] Cannot get this hanging entity to work for the life of me
jabelar replied to PhantomTiger's topic in Modder Support
Okay, I think I might know what is going wrong. You're registering the renderers in the preinit phase, but I always do it in the init phase. I suspect that it is not getting registered, and an interesting thing is that if you look at the getEntityClassRenderObject() method in the RenderManager, if it doesn't find an entry it will use the entry for the super-class. Since your class extends EntityPainting, its renderer would be called. If you want to know whether to use pre-init or init or post-init for something you can look through the startGame() method in the Minecraft class. If you check it out, you'll see that the entityRenderer isn't created at the time of pre-init, and is created just a bit before the init event. Anyway, try moving the registration to the init and see if you have the same problem. Also, another thing to look at -- I don't see you actually registering the mod entity. I see you registering the renderer for the entity, but where do you register the entity itself? Maybe entities like this type don't need to be registered, but I'd double check. -
[1.7.10] Cannot get this hanging entity to work for the life of me
jabelar replied to PhantomTiger's topic in Modder Support
I would suggest that if you can't figure it out you should try a different approach. Instead of extending EntityPainting you should copy it instead (ie as a class that extends EntityHanging). Then if their is any vanilla choose that is somehow doing something weird based on an instance of EntityPainting it won't trigger for your entity. -
I want to update my eclipse from 1.6.4 to 1.8 but I don't know how.
jabelar replied to War Crime's topic in Modder Support
Yes, that is what I said to do. Create a new 1.8 workspace and import your java and resource files from the 1.6.4 project. However, like I said: after doing that, Eclipse will complain about a lot of errors because the code will no longer be correct (for all the reasons I already listed above). -
[1.7.10] Cannot get this hanging entity to work for the life of me
jabelar replied to PhantomTiger's topic in Modder Support
Can you explain more about how you're using the enums? I think what you're saying is that you have a texture that is just like the vanilla and you want to pick up the same coordinates. However, I don't understand where you set the enum for your entity. And out of curiosity, which vanilla texture is it displaying? -
I want to update my eclipse from 1.6.4 to 1.8 but I don't know how.
jabelar replied to War Crime's topic in Modder Support
This is actually fairly tricky. But here's what you do. First of all, save a copy of your 1.6.4 cause you don't want to lose it while you muck around with conversion. Second, I suggest creating a new 1.8 workspace (follow any tutorial for this) and then import/copy your java files and resources into it. Now the problem is that many things have changed between these versions. Many package names have changed (so your imports will have to change), many methods have changed names, the way some things are done (like block states and models) is now very different, some things like sound events have been deprecated. So depending on what your mod had in it, Eclipse will tell you you have a LOT of errors. You then just start carefully going through them and figuring out what is wrong. The first thing is you'll need to make sure all the imports are right -- they changed many of the packages so that they are not under cpw anymore. That should clear up a lot of errors. Then you need to look at each method and figure out what might have changed. Sometimes the names have changed, sometimes the parameters used have changed. The best way to figure it out is to look at similar vanilla classes' code to see how they do it. Then there are the things that have changes a lot. If your mod is mostly about entities, then you'll be in good shape. But if it has a lot of blocks in it, then you're probably in for quite a bit of work. You will need to look at tutorials for converting your mod. I wrote some tips for converting from 1.7 to 1.8 here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-converting-your-mod.html You'll have to look at other tutorials about converting from 1.6.4 to 1.7.x. There are some cases where it is actually easier to start over (sorry). -
The box is positioned relative to the rotation point, and the rotation point is relative to the origin of the overall model. So if you have a rotation point for a box at 2, 3, 5 and have a box that is size 1, 1, 1, then one corner will be at 2, 3, 5 and other corner at 3, 4, 6. Now, if you want the rotation point to end up somewhere other than the corner of the box, that is when you use the offset. So if you wanted the rotation point to be in the middle of the box above, you would set the offset to be -0.5, -0.5, -0.5. So it is best to think in this order (but you don't code in this order, I'm just talking about how to figure out the numbers) : 1) specify the rotation point 2) specify the size 3) specify the offset 4) specify the rotation around the rotation point Hope that helps.
-
Is your ship an entity? Or does it have a tile entity controlling it? The best way to think about AI is to think in terms of "states" and "stimulus" (conditions that can cause a change of state). For example, your ship will spawn with a state that is "Just spawned and going up". Then at some point it will get to the height (this is a stimulus) and you would change the state to "Going down after first ascent". Then at some point it will hit the ground, where you would change the state to "On the ground and haven't spawned entity yet". Then you would spawn the entity and change the state to "On the ground and already spawned entity". So all you need to do is check each tick (like in the update() method) what the "state", and then check for stimuli that might cause it to change state. I tend to manage the states as integer constants. I think this is preferable to enumerated type because it is easier to map into packets and NBT if needed. I find it better than strings because strings can have spelling mistakes, whereas your IDE will warn you if you try to compare with an undefined constant. So you do something like: private static int SPAWNED_GOING_UP = 0; private static int SPAWNED_GOING_DOWN = 1; private static int GROUND_NOT_YET_ENTITY = 2; private static int GROUND_ALREADY_ENTITY = 3; private int state = SPAWNED_GOING_UP; Then in the update() method of your entity, you process the state then check the stimulus for changes in state. Something like if (state == SPAWNED_GOING_UP) { // move up this.posY += 0.5D; // check if reached maximum height if (this.posY > 150) { state = SPAWNED_GOING_DOWN) { } else if (state == SPAWNED_GOING_DOWN) { . . . and so on If you organize your code this way, it is really easy to make sure it all works correctly, even when complicated things are going on.
-
It looks like they may have made a fix for this in Build 1.8-11.14.3.1559. The changelog says "Fix substitutions for recipes and oredict recipes. Should mean that substitutions start working properly."
-
The rotation is controlled the the EntityLookHelper associated with the EntityLiving. So you can @Override the getEntityLookHelper() method in your cow to return a custom class that extends EntityLookHelper. In that custom EntityLookHelper you would @Override the onUpdateLook() method to do nothing in the cases where you want the cow to be frozen, otherwise let it act normally.
-
You shouldn't have to move the player. There is a separate camera view based on the Minecraft#getRenderViewEntity(). You can move that around to get different camera views. For example, this is how they do 3rd person view. I think what you'd need to do is have the server send a packet to the client to tell it it is in forced view mode and the position and rotation of that view. Then you'd have to update the renderViewEntity with that information, and prevent other modification of the renderViewEntity. Then later you'd send a packet that releases the forced view and allows it to go back to the player following mode. To change these, I think you would handle the new RenderViewEntity event.
-
I don't quite understand this. I thought the event handler method was called based on the method prototype. Doesn't the forge bus only look for methods that take event parameters for that bus, and similarly for the FML bus? Why would forge bus fire the LivingDropsEvent for example?
-
You should probably file a bug report ("pull request) to Forge team. This is something that really should work as it is a powerful way to mod.
-
Does forge have a way to load models from MrCrayFish model creator?
jabelar replied to ciroreed's topic in Modder Support
Actually it is really easy to just code it directly in Java. Basically it is just a bunch of cubes that you specify that have positions, offsets and rotation (if necessary), plus some texture binding. Just look at the models for entities and it should be pretty obvious how it works. I just sketch my model on graph paper, and then it is easy to code in Java. -
Once an item is dropped it become an EntityItem. An EntityItem extends Entity so has access to the isInWater() method to tell you it is in water. If you also want it to explode in rain, use the isWet() method instead. So like diesieben07 says, you should make your item drop your own custom EntityItem that explodes if isInWater() is true (check in the onUpdate() method). For explosions, look at TNT or EntityCreeper.
-
[1.7.10] Two mods fighting over which chunk manager to use?
jabelar replied to WhichOnesPink's topic in Modder Support
Well, if they both extend a common class then maybe you can rely on that so then you wont get the cast error. The only time you should need to differentiate between the two chunk managers in code is if you're calling a method that doesn't @override the superclass. -
Instant Structure is that possible? (PAYED IF HELPED)
jabelar replied to chrisknoop's topic in Modder Support
This is a good example why it is good to work through a problem yourself (instead of paying people). You actually learned something. -
Except that also stops them from spawning naturally, later. Which as I understood is exactly not what OP wants. The OP said he wanted them to spawn later "through other means". I understood that to mean he would spawn them explicitly. Basically nothing auto-spawned. But you're right he might have meant something else.
-
The events are not really "nested" in the sense you think. An event is simply an event, meaning a singular point in time/execution of the code. The PRE event is basically saying I'm starting something, and the POST is saying its finishing something, but there is no real relationship except hopefully the code fires each at the right point. There is nothing weird about starting a bunch of things before finishing the first thing.
-
I vote for SourceTree. I have tutorial on setting it up here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-publishing-to-github.html I know using command line is more "manly" but in the modern world you might as well take advantage of a graphical interface.
-
How can I make 24 rotation states of block across blockstate?
jabelar replied to alexfadeev's topic in Modder Support
One other solution is to actually have two different blocks with the various states distributed between them. When someone performs certain rotations then you'd replace with the other block (with corresponding rotation state). If both blocks extend the same base block, then you can test for the base block anywhere your code needs the block. But tile entity is better way to go. Just wanted to say that there are usually multiple options to solve problems in programming.