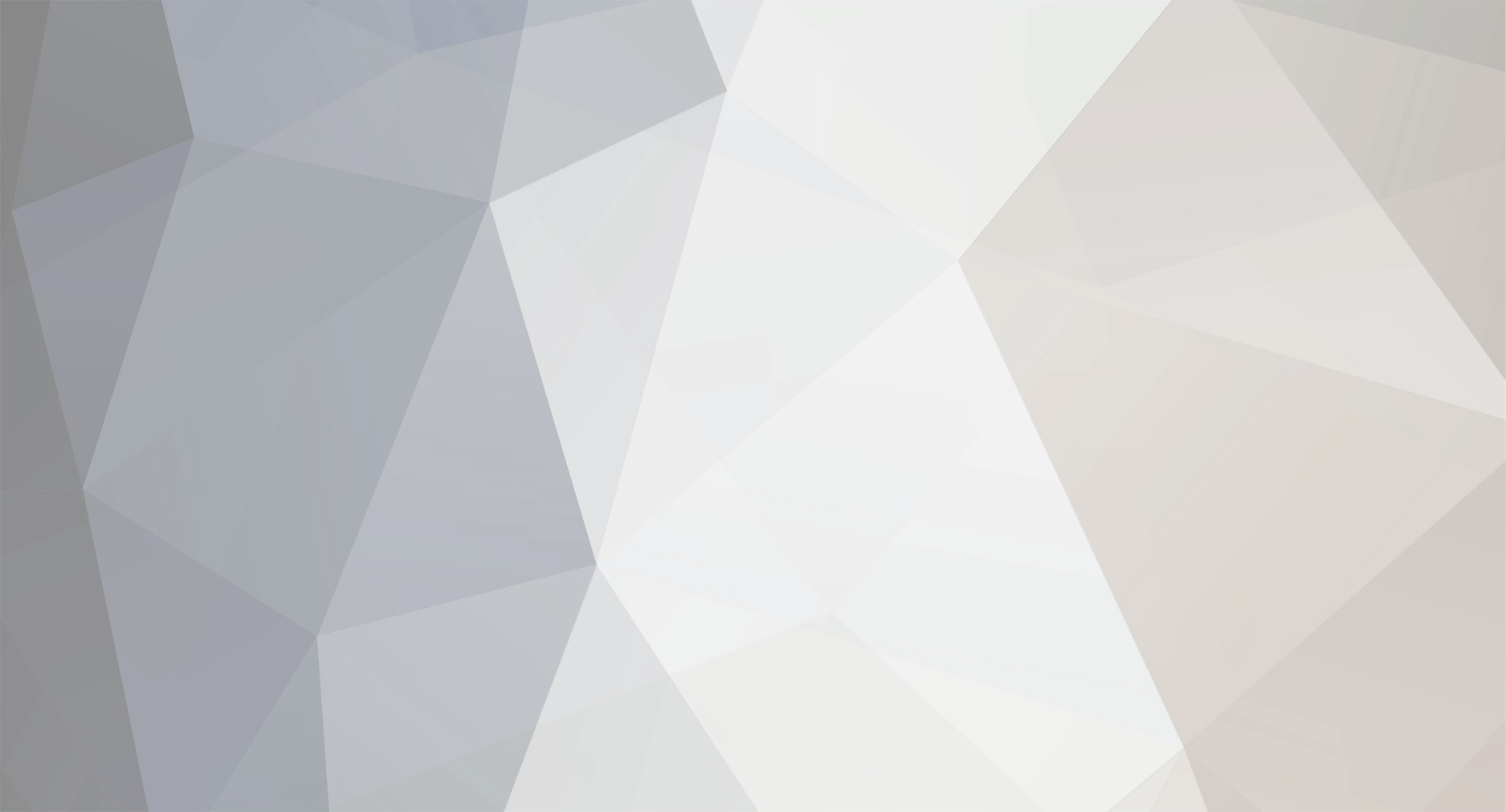
The_SlayerMC
Members-
Posts
209 -
Joined
-
Last visited
Everything posted by The_SlayerMC
-
Thats what i have all my other GUI's set too, i also thought this would be a problem so i casted the players pos to an int and set the coords like that, but it still did nothing.
-
[spoiler=Gui Handler] package net.essence.client; import net.essence.blocks.tileentity.*; import net.essence.blocks.tileentity.container.*; import net.essence.client.render.gui.*; import net.essence.items.tileentity.*; import net.essence.items.tileentity.container.*; import net.essence.util.ContainerEmpty; import net.minecraft.entity.Entity; import net.minecraft.entity.IMerchant; import net.minecraft.entity.player.*; import net.minecraft.tileentity.*; import net.minecraft.util.*; import net.minecraft.world.*; import net.minecraftforge.fml.common.network.*; import net.slayer.api.entity.tileentity.container.*; public class GuiHandler implements IGuiHandler { public enum GuiIDs { ENRICHMENT_TABLE, INCUBATOR, BACKPACK, MAGE, BLACKSMITH, GREEN_ELF, RED_ELF, KNOWLEDGE; } @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { TileEntity entity = world.getTileEntity(new BlockPos(x, y, z)); if(ID == GuiIDs.ENRICHMENT_TABLE.ordinal()) return new ContainerEnrichedTable(player.inventory, world, new BlockPos(x, y, z), player); if(ID == GuiIDs.INCUBATOR.ordinal()) return new ContainerIncubator(player.inventory, (TileEntityIncubator)entity); if(ID == GuiIDs.BACKPACK.ordinal()) return new ContainerBackpack(player.inventory, (TileEntityBackpack)entity); if(ID == GuiIDs.MAGE.ordinal()) return new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world); if(ID == GuiIDs.BLACKSMITH.ordinal()) return new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world); if(ID == GuiIDs.GREEN_ELF.ordinal()) return new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world); if(ID == GuiIDs.RED_ELF.ordinal()) return new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world); if(ID == GuiIDs.KNOWLEDGE.ordinal()) return new ContainerKnowledgeTable(player.inventory, (TileEntityKnowledgeTable)entity, world); return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { TileEntity entity = world.getTileEntity(new BlockPos(x, y, z)); if(ID == GuiIDs.ENRICHMENT_TABLE.ordinal()) return new GuiEnrichedEnchantmentTable(player.inventory, world, x, y, z, player); if(ID == GuiIDs.INCUBATOR.ordinal()) return new GuiIncubator(player.inventory, (TileEntityIncubator)entity); if(ID == GuiIDs.BACKPACK.ordinal()) return new GuiBackpack(player.inventory, (TileEntityBackpack)entity); if(ID == GuiIDs.MAGE.ordinal()) return new GuiMage(new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world), (IMerchant)getEntityByID(x, world)); if(ID == GuiIDs.BLACKSMITH.ordinal()) return new GuiBlacksmith(new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world), (IMerchant)getEntityByID(x, world)); if(ID == GuiIDs.GREEN_ELF.ordinal()) return new GuiGreenElf(new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world), (IMerchant)getEntityByID(x, world)); if(ID == GuiIDs.RED_ELF.ordinal()) return new GuiRedElf(new ContainerModVillager(player.inventory, (IMerchant)getEntityByID(x, world), world), (IMerchant)getEntityByID(x, world)); if(ID == GuiIDs.KNOWLEDGE.ordinal()) return new GuiKnowledgeTable(player.inventory, (TileEntityKnowledgeTable)entity, world); return null; } private Entity getEntityByID(int entityID, World world) { for(int i = 0; i < world.getLoadedEntityList().size(); i++) { if(((Entity)world.getLoadedEntityList().get(i)).getEntityId() == entityID) { return ((Entity)world.getLoadedEntityList().get(i)); } } return null; } } [spoiler=Block Code] @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumFacing side, float hitX, float hitY, float hitZ) { TileEntityKnowledgeTable tile = (TileEntityKnowledgeTable)worldIn.getTileEntity(pos); if(!worldIn.isRemote) { if(tile != null) { playerIn.openGui(Essence.instance, GuiIDs.KNOWLEDGE.ordinal(), worldIn, 0, 0, 0); } return true; } return false; } @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new TileEntityKnowledgeTable(); } }
-
Hello, i'm getting a really strange crash for a slot not getting the stack... I've tried to change things such as you would for a TE but if i comment the slot for the TE then it works fine... [spoiler=Container] package net.essence.blocks.tileentity.container; import net.essence.blocks.tileentity.TileEntityKnowledgeTable; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.InventoryBasic; import net.minecraft.inventory.Slot; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public class ContainerKnowledgeTable extends Container { public IInventory tableInventory; private World world; public ContainerKnowledgeTable(InventoryPlayer inventory, TileEntityKnowledgeTable entity, World w) { world = w; tableInventory = entity; this.addSlotToContainer(new Slot(entity, 0, 80, 35)); for(int i = 0; i < 3; ++i) for(int j = 0; j < 9; ++j) this.addSlotToContainer(new Slot(inventory, j + i * 9 + 9, 8 + j * 18, 84 + i * 18)); for(int i = 0; i < 9; ++i) this.addSlotToContainer(new Slot(inventory, i, 8 + i * 18, 142)); } @Override public void onContainerClosed(EntityPlayer playerIn) { super.onContainerClosed(playerIn); if(!world.isRemote) { ItemStack itemstack = this.tableInventory.getStackInSlotOnClosing(0); if (itemstack != null) { playerIn.dropPlayerItemWithRandomChoice(itemstack, false); } } } @Override public boolean canInteractWith(EntityPlayer playerIn) { return true; } } [spoiler=Tile Entity] package net.essence.blocks.tileentity; import net.essence.EssenceItems; import net.essence.items.ItemKnowledge; import net.minecraft.entity.item.EntityItem; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.server.gui.IUpdatePlayerListBox; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.IChatComponent; public class TileEntityKnowledgeTable extends TileEntity implements IUpdatePlayerListBox, IInventory { private float rotate = 0.0F; private ItemStack inventory = new ItemStack(EssenceItems.blankKnowledge); @Override public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); nbt.setFloat("rotation", 0.0F); } @Override public void readFromNBT(NBTTagCompound nbt) { super.readFromNBT(nbt); rotate = nbt.getFloat("rotation"); } public float getRotation() { return rotate; } @Override public void update() { rotate += 5.0F; if(rotate >= 360F) rotate = 0.0F; } @Override public int getSizeInventory() { return 1; } @Override public ItemStack getStackInSlot(int i) { return i == 0 ? inventory : null; } @Override public String getName() { return "knowledge Table"; } @Override public boolean hasCustomName() { return true; } @Override public IChatComponent getDisplayName() { return null; } @Override public ItemStack decrStackSize(int i, int j) { if(i == 0 && inventory != null) { if(inventory.stackSize <= j) { ItemStack itemstack = inventory; inventory = null; return itemstack; } else { inventory.stackSize -= j; return new ItemStack(inventory.getItem(), j, inventory.getMetadata()); } } else { return null; } } @Override public ItemStack getStackInSlotOnClosing(int index) { if(index == 0 && this.inventory != null) { ItemStack itemstack = this.inventory; this.inventory = null; return itemstack; } else { return null; } } @Override public void setInventorySlotContents(int i, ItemStack stack) { if(i == 0) this.inventory = stack; } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer player) { return true; } @Override public void openInventory(EntityPlayer player) { } @Override public void closeInventory(EntityPlayer player) { } @Override public boolean isItemValidForSlot(int index, ItemStack stack) { return stack.getItem() != null && stack.getItem() instanceof ItemKnowledge; } @Override public int getField(int id) { return 0; } @Override public void setField(int id, int value) { } @Override public int getFieldCount() { return 0; } @Override public void clear() { inventory = null; } } Here is the crash: ---- Minecraft Crash Report ---- // Oh - I know what I did wrong! Time: 20/03/15 3:26 PM Description: Ticking entity java.lang.NullPointerException: Ticking entity at net.minecraft.inventory.Slot.getStack(Slot.java:58) at net.minecraft.inventory.Container.detectAndSendChanges(Container.java:76) at net.minecraft.entity.player.EntityPlayerMP.onUpdate(EntityPlayerMP.java:229) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:1865) at net.minecraft.world.WorldServer.updateEntityWithOptionalForce(WorldServer.java:732) at net.minecraft.world.World.updateEntity(World.java:1835) at net.minecraft.world.World.updateEntities(World.java:1664) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:571) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:703) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:598) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:164) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:478) at java.lang.Thread.run(Unknown Source) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at net.minecraft.inventory.Slot.getStack(Slot.java:58) at net.minecraft.inventory.Container.detectAndSendChanges(Container.java:76) at net.minecraft.entity.player.EntityPlayerMP.onUpdate(EntityPlayerMP.java:229) at net.minecraft.world.World.updateEntityWithOptionalForce(World.java:1865) at net.minecraft.world.WorldServer.updateEntityWithOptionalForce(WorldServer.java:732) at net.minecraft.world.World.updateEntity(World.java:1835) -- Entity being ticked -- Details: Entity Type: null (net.minecraft.entity.player.EntityPlayerMP) Entity ID: 78 Entity Name: Player155 Entity's Exact location: 593.97, 4.00, 693.67 Entity's Block location: 593.00,4.00,693.00 - World: (593,4,693), Chunk: (at 1,0,5 in 37,43; contains blocks 592,0,688 to 607,255,703), Region: (1,1; contains chunks 32,32 to 63,63, blocks 512,0,512 to 1023,255,1023) Entity's Momentum: 0.00, -0.08, 0.00 Entity's Rider: ~~ERROR~~ NullPointerException: null Entity's Vehicle: ~~ERROR~~ NullPointerException: null Stacktrace: at net.minecraft.world.World.updateEntities(World.java:1664) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:571) -- Affected level -- Details: Level name: New World All players: 1 total; [EntityPlayerMP['Player155'/78, l='New World', x=593.97, y=4.00, z=693.67]] Chunk stats: ServerChunkCache: 1286 Drop: 0 Level seed: 2209123573195456436 Level generator: ID 01 - flat, ver 0. Features enabled: true Level generator options: Level spawn location: 601.00,4.00,701.00 - World: (601,4,701), Chunk: (at 9,0,13 in 37,43; contains blocks 592,0,688 to 607,255,703), Region: (1,1; contains chunks 32,32 to 63,63, blocks 512,0,512 to 1023,255,1023) Level time: 27434 game time, 27434 day time Level dimension: 0 Level storage version: 0x04ABD - Anvil Level weather: Rain time: 72120 (now: false), thunder time: 64136 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: true Stacktrace: at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:703) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:598) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:164) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:478) at java.lang.Thread.run(Unknown Source) -- System Details -- Details: Minecraft Version: 1.8 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.8.0_20, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 624873872 bytes (595 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP v9.10 FML v8.0.26.1305 Minecraft Forge 11.14.1.1305 4 mods loaded, 4 mods active mcp{9.05} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available FML{8.0.26.1305} [Forge Mod Loader] (forgeSrc-1.8-11.14.1.1305.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Forge{11.14.1.1305} [Minecraft Forge] (forgeSrc-1.8-11.14.1.1305.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available essence{1.1.0} [Essence of the Gods] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Profiler Position: N/A (disabled) Player Count: 1 / 8; [EntityPlayerMP['Player155'/78, l='New World', x=593.97, y=4.00, z=693.67]] Type: Integrated Server (map_client.txt) Is Modded: Definitely; Client brand changed to 'fml,forge'
-
Okay, nevermind... I'm so stupid I removed the ScaledResolution and replaced that with a 0 It now renders on the left side and changes when you change the screen size. Thanks anyway
-
@SubscribeEvent public void tickEvent(RenderTickEvent event) { GuiIngame gig = mc.ingameGUI; FontRenderer font = mc.fontRendererObj; EntityPlayer player = mc.thePlayer; if(mc.currentScreen == null || mc.currentScreen instanceof GuiChat) { if(!mc.gameSettings.showDebugInfo) { if(SlayerAPI.BETA) mc.fontRendererObj.drawString(EnumChatFormatting.DARK_GREEN + "Essence of the Gods: " + EnumChatFormatting.DARK_RED + SlayerAPI.MOD_VERSION, 5, 5, 0); if(!player.capabilities.isCreativeMode) { mc.getTextureManager().bindTexture(new ResourceLocation(SlayerAPI.PREFIX + "textures/gui/playerStats.png")); float health = player.getHealth() * 5F; float hunger = player.getFoodStats().getFoodLevel() * 5F; float experience = (int)(mc.thePlayer.experience * 150F); float armor = player.getTotalArmorValue() * 5; float air = (float)player.getAir() / 1.5F / 2F; ScaledResolution res = new ScaledResolution(mc, mc.displayWidth, mc.displayHeight); int w = res.getScaledWidth() - 160, h = 10; GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); GL11.glEnable(GL11.GL_BLEND); int lengthOfBar = 151; gig.drawTexturedModalRect(w - 10, h, 0, 0, lengthOfBar, 108 - 18); gig.drawTexturedModalRect(w - 10, h + 108 - 18, 0, 90, lengthOfBar, 18); gig.drawTexturedModalRect(w - 10, h + 108, 0, 235, 182, 18); gig.drawTexturedModalRect(w - 10, h, 0, 109, (int)(health * 1.5F), 18); gig.drawTexturedModalRect(w - 10 + 3, h + 3, 0, 199, 12, 11); gig.drawTexturedModalRect(w - 10, h + 18, 0, 127, (int)(hunger * 1.5F), 18); gig.drawTexturedModalRect(w - 10 + 3, h + 21, 0, 210, 12, 11); gig.drawTexturedModalRect(w - 10, h + 36 + 18 + 17, 0, 180, (int)(armor * 1.5F), 18); gig.drawTexturedModalRect(w - 10 + 3, h + 36 + 40, 17, 210, 9, 9); gig.drawTexturedModalRect(w - 10, h + 36 + 36 - 18, 0, 163, (int)(air * 2), 18); gig.drawTexturedModalRect(w - 10 + 3, h + 36 + 40 - 18, 17, 200, 9, 9); gig.drawTexturedModalRect(w - 10, h + 36, 0, 145, (int)experience, 18); GL11.glPushMatrix(); GL11.glColor4f(0.4F, 1.0F, 0.3F, 1.0F); gig.drawTexturedModalRect(w - 10 + 3, h + 38, 0, 221, 14, 14); GL11.glPopMatrix(); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); font.drawString(I18n.format("essence.healthbar", new Object[0]) + " " + health + "%", w - 10 + 20, h + 5, 0xFFFFFF); font.drawString(I18n.format("essence.hungerbar", new Object[0]) + " " + hunger + "%" , w - 10 + 20, h + 23, 0xFFFFFF); font.drawString(I18n.format("essence.armorbar", new Object[0]) + " " + armor + "%", w - 10 + 20, h + 36 + 23 + 18, 0xFFFFFF); if(player.getAir() <= 0) font.drawString(I18n.format("essence.airbar", new Object[0]) + " " + "0%" , w - 10 + 20, h + 36 - 18 + 42, 0xFFFFFF); else font.drawString(I18n.format("essence.airbar", new Object[0]) + " " + (int)air + "%" , w - 10 + 20, h + 36 - 18 + 42, 0xFFFFFF); font.drawString(I18n.format("essence.xpbar", new Object[0]) + " " + mc.thePlayer.experienceLevel , w - 10 + 20, h + 41, 0xFFFFFF); String st = I18n.format("essence.time", new Object[0]) + " " + formatTime(getWorldTime(mc)); font.drawString(st, w - 10 + 3, h + 41 + 36 + 18, 0xFFFFFF); font.drawString(I18n.format("essence.coords", new Object[0]) + " X: " + (int)player.posX + ", Y: " + (int)(player.posY - 1) + ", Z: " + (int)player.posZ, w - 10 + 5, h + 36 + 78, 0xFFFFFF); GL11.glDisable(GL11.GL_BLEND); } } } }
-
As all the people that mess around with "Gui InGame" would know, that you can only get things to render to the right side of the screen easily (so when the player changes the screen size it stays in the same place) and I'm wondering if you're able to do the same thing but on the left side instead. I mean so when you set a spot for the overlay on the left, it corresponds with the screen size so you don't just have a random overlay sitting in the middle of the screen when you're going in full screen. Any help?
-
Okay! it worked!! Thanks a bunch. But how would i go around getting it to be compatable with Morph and mods like that?
-
I've never really even used packets, it sounds noobish but i haven't ever had a need for it
-
Well using if (event.player instanceof EntityPlayerMP) { EntityPlayerMP playerMP = (EntityPlayerMP)event.player; if(playerMP.theItemInWorldManager.getGameType() != GameType.CREATIVE || playerMP.theItemInWorldManager.getGameType() != GameType.SPECTATOR) { if(helmet == item.flairiumHelmet && body == item.flairiumChest && legs == item.flairiumLegs && boots == item.flairiumBoots) { event.player.capabilities.allowFlying = true; } else { event.player.capabilities.allowFlying = false; event.player.capabilities.isFlying = false; } } } Doesn't let me fly when using the flairium armour set anymore. Doesn't matter what game mode I'm in
-
Okay, so how would i go for being in single player and checking the game mode?
-
Oh my god... that was so fucking stupid of me hahaha. Thanks, ill try it out.
-
private EssenceItems item = new EssenceItems(); private Item boots = null, body = null, legs = null, helmet = null; @SubscribeEvent public void playerTick(PlayerTickEvent event) { ItemStack stackBoots = event.player.inventory.armorItemInSlot(0); ItemStack stackLegs = event.player.inventory.armorItemInSlot(1); ItemStack stackBody = event.player.inventory.armorItemInSlot(2); ItemStack stackHelmet = event.player.inventory.armorItemInSlot(3); if(stackBoots != null) boots = stackBoots.getItem(); else boots = null; if(stackBody != null) body = stackBody.getItem(); else body = null; if(stackLegs != null) legs = stackLegs.getItem(); else legs = null; if(stackHelmet != null) helmet = stackHelmet.getItem(); else helmet = null; EntityPlayerMP playerMP = (EntityPlayerMP)event.player; if(playerMP.theItemInWorldManager.getGameType() != GameType.CREATIVE || playerMP.theItemInWorldManager.getGameType() != GameType.SPECTATOR) { if(helmet == item.flairiumHelmet && body == item.flairiumChest && legs == item.flairiumLegs && boots == item.flairiumBoots){ event.player.capabilities.allowFlying = true; } else { event.player.capabilities.allowFlying = false; event.player.capabilities.isFlying = false; } } } That gives me a crash that leads to this when trying to enter a server with my mod and also when i use the EntityPlayerMP code in sp:
-
I also want to state that im using the tick event for this code, aswell as @SideOnly(Side.CLIENT) annotation because on servers it crashes without it... I know it's not supposed to be used that but i don't think that client and EntityPlayerMP is compatible (Well duhhh)
-
Then what should i do? I want access to all the game modes not just creative
-
Hello, I'm trying to get the players game mode is single player. But the only way i can figure it out is using this: EntityPlayerMP playerMP = (EntityPlayerMP)event.player; if(playerMP.theItemInWorldManager.getGameType() != GameType.CREATIVE || playerMP.theItemInWorldManager.getGameType() != GameType.SPECTATOR) { Is there any other way to do it?
-
What does the "modgenerationweight"? (world generation)
The_SlayerMC replied to Kander16's topic in Modder Support
The generation weight is to tell minecraft when to run your code. So if you set it to 10 it would go last, if it is 0 it will go first. It's the priority of the generation. -
If it is making a Nether Portal instead of your custom one, then you're obviously not overriding "makePortal()" or whatever inside the teleporter you're using. Edit: Scratch that... You're hardly even overriding any methods at all... If you want a custom portal, then actually have the code there to make it. Your portal block has bad syntax by the way. "teleporter.java" is not a good portal block name, atleast call it "BlockTeleporter.java" or something. You also have no code in your actual Teleporter class besides the constructor. So ofcourse your dimension travel isn't going to work as expected. So if I were you, I would look up some tutorials or look at the vanilla code to help you.
-
[1.6.4][SOLVED] My Custom Grass Textures
The_SlayerMC replied to jkorn2324swagg's topic in Modder Support
Side note, why do your textures/code have a terrible syntax?! this.Dirt_2 = par1IconRegister.registerIcon(this.getTextureName() + "Side of Grass (Dirt_2)"); Does the course you're doing also teach how to make your textures/code looks nice? If so, they're doing it wrong. -
Try looking at "Loader.java"
-
Add Enchantment.addToBookList(this); to your constructor
-
I wont give you the code to do it with the event. But what it is, is getting the event block arraylist and remove the block it drops and add the furnace recipe like this as example: ItemStack stack = FurnaceRecipes.instance().getSmeltingResult(new ItemStack(event.state.getBlock()));
-
If you want to use a vanilla particle, such as the flame: world.spawnParticle("flame", x, y, z, 0.0D, 0.0D, 0.0D);
-
well you can have a look at "EntityReddustFX.java" and do magic by c&p that, then add this into your block.java: @Override @SideOnly(Side.CLIENT) public void randomDisplayTick(World w, int x, int y, int z, Random rand) { renderParticle(w, x, y, z); } private void renderParticle(World w, int x, int y, int z) { Random random = w.rand; double d0 = 0.0625D; for(int l = 0; l < 6; ++l) { double d1 = (double)((float)x + random.nextFloat()); double d2 = (double)((float)y + random.nextFloat()); double d3 = (double)((float)z + random.nextFloat()); if(l == 0 && !w.getBlock(x, y + 1, z).isOpaqueCube()) d2 = (double)(y + 1) + d0; if(l == 1 && !w.getBlock(x, y - 1, z).isOpaqueCube()) d2 = (double)(y + 0) - d0; if(l == 2 && !w.getBlock(x, y, z + 1).isOpaqueCube()) d3 = (double)(z + 1) + d0; if(l == 3 && !w.getBlock(x, y, z - 1).isOpaqueCube()) d3 = (double)(z + 0) - d0; if(l == 4 && !w.getBlock(x + 1, y, z).isOpaqueCube()) d1 = (double)(x + 1) + d0; if(l == 5 && !w.getBlock(x - 1, y, z).isOpaqueCube()) d1 = (double)(x + 0) - d0; if(d1 < (double)x || d1 > (double)(x + 1) || d2 < 0.0D || d2 > (double)(y + 1) || d3 < (double)z || d3 > (double)(z + 1)) { OreParticleFX fx= new OreParticleFX(w, d1, d2, d3); FMLClientHandler.instance().getClient().effectRenderer.addEffect(fx); } } } And thats basically it
-
Not really... And there is nothing "special" to what my ores are doing really... Aha
-
Well you can have a look at my ore class to see if it will help!