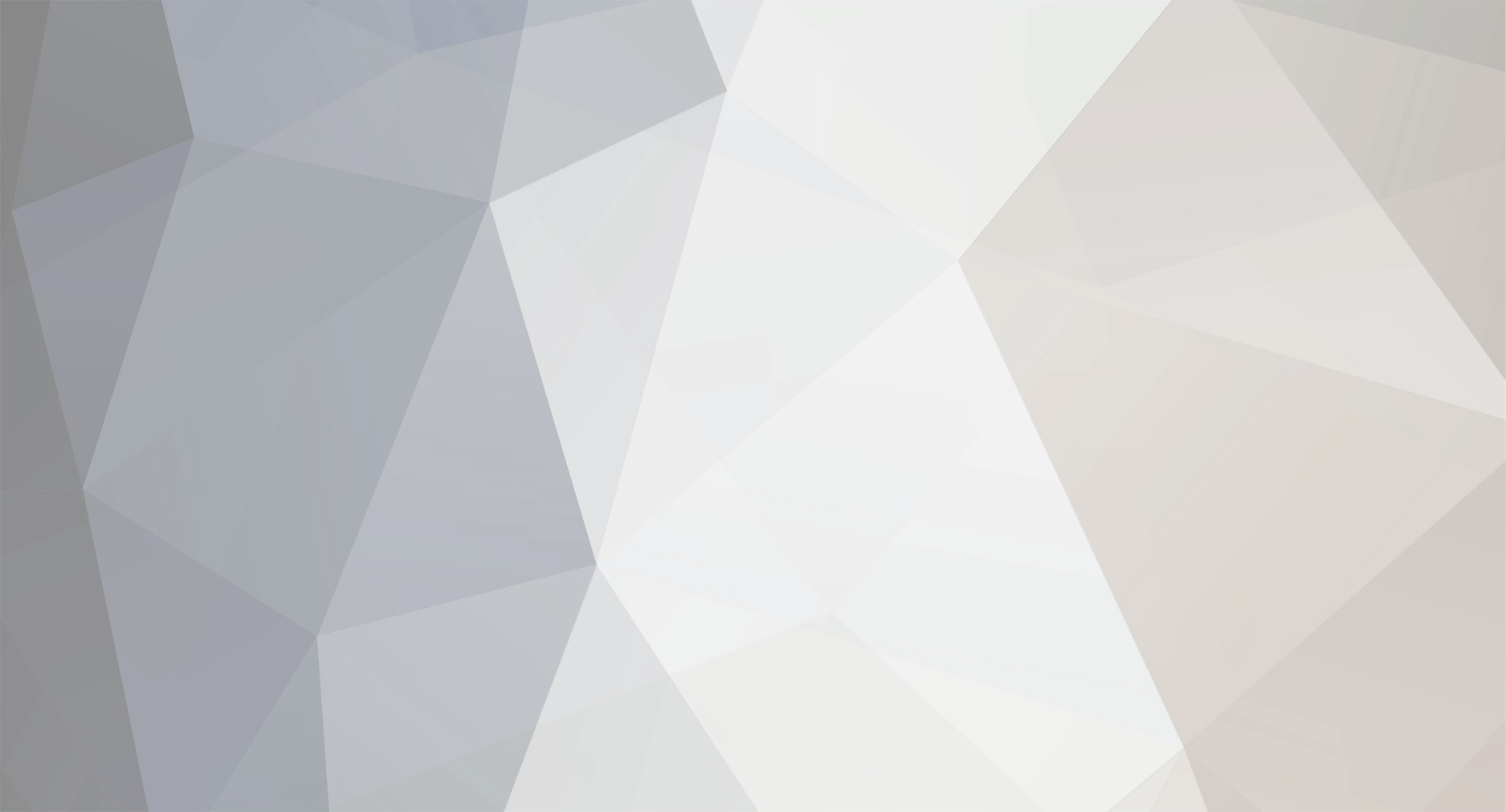
Briggybros
Members-
Posts
155 -
Joined
-
Last visited
Everything posted by Briggybros
-
Possibly something like this, using reflect. Where ChestGenHooks.EnumType is passed through private ItemStack[] getDungeonLoot(ChestGenHooks cgh) { try { Field field = cgh.getClass().getDeclaredField("contents"); if (!Modifier.isPublic(field.getModifiers()) || !Modifier.isPublic(field.getDeclaringClass().getModifiers())) { field.setAccessible(true); ArrayList<WeightedRandomChestContent> list = (ArrayList<WeightedRandomChestContent>) field.get(cgh); ArrayList<ItemStack> isl = new ArrayList<ItemStack>(); for (WeightedRandomChestContent wrcc: list) { isl.add(wrcc.theItemId); } return isl.toArray(new ItemStack[isl.size()]); } } catch (NoSuchFieldException e) { e.printStackTrace(); } catch (SecurityException e) { e.printStackTrace(); } catch (IllegalArgumentException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } return null; } NOTE: I've never used reflect in Minecraft and I'm not sure if it'll work. Also, I'm not the best at using it. Just putting it up as a suggestion.
-
@SideOnly(Side.CLIENT) public class RenderMyNewOrb extends Render { private static final ResourceLocation field_110785_a = new ResourceLocation("textures/entity/experience_orb.png"); public RenderMyNewOrb() { this.shadowSize = 0.15F; this.shadowOpaque = 0.75F; } /** * Renders the Orb. */ public void renderTheOrb(EntityMyNewOrb par1EntityMyNewOrb, double par2, double par4, double par6, float par8, float par9) { GL11.glPushMatrix(); GL11.glTranslatef((float)par2, (float)par4, (float)par6); this.func_110777_b(par1EntityMyNewOrb); int i = par1EntityMyNewOrb.getTextureByValue(); float f2 = (float)(i % 4 * 16 + 0) / 64.0F; float f3 = (float)(i % 4 * 16 + 16) / 64.0F; float f4 = (float)(i / 4 * 16 + 0) / 64.0F; float f5 = (float)(i / 4 * 16 + 16) / 64.0F; float f6 = 1.0F; float f7 = 0.5F; float f8 = 0.25F; int j = par1EntityMyNewOrb.getBrightnessForRender(par9); int k = j % 65536; int l = j / 65536; OpenGlHelper.setLightmapTextureCoords(OpenGlHelper.lightmapTexUnit, (float)k / 1.0F, (float)l / 1.0F); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); float f9 = 255.0F; int i1 = (int)f9; GL11.glRotatef(180.0F - this.renderManager.playerViewY, 0.0F, 1.0F, 0.0F); GL11.glRotatef(-this.renderManager.playerViewX, 1.0F, 0.0F, 0.0F); float f11 = 0.3F; GL11.glScalef(f11, f11, f11); Tessellator tessellator = Tessellator.instance; tessellator.startDrawingQuads(); int r = 188; int g = 94; int b = 94; if (par1EntityMyNewOrb.isLight) { r = 131; g = 227; b = 227; } tessellator.setColorRGBA(r,g,b, 128); tessellator.setNormal(0.0F, 1.0F, 0.0F); tessellator.addVertexWithUV((double)(0.0F - f7), (double)(0.0F - f8), 0.0D, (double)f2, (double)f5); tessellator.addVertexWithUV((double)(f6 - f7), (double)(0.0F - f8), 0.0D, (double)f3, (double)f5); tessellator.addVertexWithUV((double)(f6 - f7), (double)(1.0F - f8), 0.0D, (double)f3, (double)f4); tessellator.addVertexWithUV((double)(0.0F - f7), (double)(1.0F - f8), 0.0D, (double)f2, (double)f4); tessellator.draw(); GL11.glDisable(GL11.GL_BLEND); GL11.glDisable(GL12.GL_RESCALE_NORMAL); GL11.glPopMatrix(); } protected ResourceLocation func_110784_a(EntityMyNewOrb par1EntityMyNewOrb) { return field_110785_a; } protected ResourceLocation func_110775_a(Entity par1Entity) { return this.func_110784_a((EntityMyNewOrb)par1Entity); } /** * Actually renders the given argument. This is a synthetic bridge method, always casting down its argument and then * handing it off to a worker function which does the actual work. In all probabilty, the class Render is generic * (Render<T extends Entity) and this method has signature public void doRender(T entity, double d, double d1, * double d2, float f, float f1). But JAD is pre 1.5 so doesn't do that. */ public void doRender(Entity par1Entity, double par2, double par4, double par6, float par8, float par9) { this.renderTheOrb((EntityMyNewOrb)par1Entity, par2, par4, par6, par8, par9); } } Updated renderer to not use bitwise logic, still no orbs are rendered.
-
Hello, I'm trying to create a new type of xp orb. To do this I've basically copied the original xp orb, and added a few modifications. The entity works fine as I can see its effects, however, I cannot get it to render at all. I've tried adding this to my initialization method: EntityRegistry.registerGlobalEntityID(MyNewOrb.class, "myNewOrb", EntityRegistry.findGlobalUniqueEntityId()); and then this at the same point, only in my ClientProxy: RenderingRegistry.registerEntityRenderingHandler(MyNewOrb.class, new RenderMyNewOrb()); and finally, here is my code for rendering it: @SideOnly(Side.CLIENT) public class RenderMyNewOrb extends Render { private static final ResourceLocation field_110785_a = new ResourceLocation("textures/entity/experience_orb.png"); public RenderMyNewOrb() { this.shadowSize = 0.15F; this.shadowOpaque = 0.75F; } /** * Renders the Orb. */ public void renderTheOrb(EntityMyNewOrb par1EntityMyNewOrb, double par2, double par4, double par6, float par8, float par9) { GL11.glPushMatrix(); GL11.glTranslatef((float)par2, (float)par4, (float)par6); this.func_110777_b(par1EntityMyNewOrb); int i = par1EntityMyNewOrb.getTextureByValue(); float f2 = (float)(i % 4 * 16 + 0) / 64.0F; float f3 = (float)(i % 4 * 16 + 16) / 64.0F; float f4 = (float)(i / 4 * 16 + 0) / 64.0F; float f5 = (float)(i / 4 * 16 + 16) / 64.0F; float f6 = 1.0F; float f7 = 0.5F; float f8 = 0.25F; int j = par1EntityMyNewOrb.getBrightnessForRender(par9); int k = j % 65536; int l = j / 65536; OpenGlHelper.setLightmapTextureCoords(OpenGlHelper.lightmapTexUnit, (float)k / 1.0F, (float)l / 1.0F); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); float f9 = 255.0F; int i1 = (int)f9; int k1 = 0x00BC5E5E; if (par1EntityMyNewOrb.isBlue) k1 = 0x0083E3E3; GL11.glRotatef(180.0F - this.renderManager.playerViewY, 0.0F, 1.0F, 0.0F); GL11.glRotatef(-this.renderManager.playerViewX, 1.0F, 0.0F, 0.0F); float f11 = 0.3F; GL11.glScalef(f11, f11, f11); Tessellator tessellator = Tessellator.instance; tessellator.startDrawingQuads(); tessellator.setColorRGBA_I(k1, 128); tessellator.setNormal(0.0F, 1.0F, 0.0F); tessellator.addVertexWithUV((double)(0.0F - f7), (double)(0.0F - f8), 0.0D, (double)f2, (double)f5); tessellator.addVertexWithUV((double)(f6 - f7), (double)(0.0F - f8), 0.0D, (double)f3, (double)f5); tessellator.addVertexWithUV((double)(f6 - f7), (double)(1.0F - f8), 0.0D, (double)f3, (double)f4); tessellator.addVertexWithUV((double)(0.0F - f7), (double)(1.0F - f8), 0.0D, (double)f2, (double)f4); tessellator.draw(); GL11.glDisable(GL11.GL_BLEND); GL11.glDisable(GL12.GL_RESCALE_NORMAL); GL11.glPopMatrix(); } protected ResourceLocation func_110784_a(EntityMyNewOrb par1EntityMyNewOrb) { return field_110785_a; } protected ResourceLocation func_110775_a(Entity par1Entity) { return this.func_110784_a((EntityMyNewOrb)par1Entity); } /** * Actually renders the given argument. This is a synthetic bridge method, always casting down its argument and then * handing it off to a worker function which does the actual work. In all probabilty, the class Render is generic * (Render<T extends Entity) and this method has signature public void doRender(T entity, double d, double d1, * double d2, float f, float f1). But JAD is pre 1.5 so doesn't do that. */ public void doRender(Entity par1Entity, double par2, double par4, double par6, float par8, float par9) { this.renderTheOrb((EntityMyNewOrb)par1Entity, par2, par4, par6, par8, par9); } } The only thing changed is the colour section, which may be wrong, but I'm not the best at bitwise logic.
-
My PacketHandler does work because the first channel is working
-
won't isRemote always return false on the serverside though?
-
This is my packet handler, the System.out.println isn't called when on a singleplayer game package mods.SaberMod; import java.io.ByteArrayInputStream; import java.io.DataInputStream; import java.io.IOException; import mods.SaberMod.client.ClientProxy; import net.minecraft.client.Minecraft; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.item.ItemStack; import net.minecraft.network.INetworkManager; import net.minecraft.network.packet.Packet250CustomPayload; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.Player; import cpw.mods.fml.relauncher.Side; public class PacketHandler implements IPacketHandler { @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player playerEntity) { Side side = FMLCommonHandler.instance().getEffectiveSide(); if (packet.channel.equals("SaberExtend") && side == Side.SERVER) { handleExtend(packet, playerEntity); } if (packet.channel.equals("SaberVaules") && side == Side.CLIENT) { handleValues(packet); } } private void handleExtend(Packet250CustomPayload packet, Player playerEntity) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int stage = 0; int damage = 0; try { stage = inputStream.readInt(); damage = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } stage = (stage == 0) ? 1 : 0; EntityPlayerMP player = (EntityPlayerMP) playerEntity; player.inventory.setInventorySlotContents(player.inventory.currentItem, new ItemStack((256 + SaberMod.BASE_ITEM_ID + stage), 1, damage)); player.updateHeldItem(); } private void handleValues(Packet250CustomPayload packet) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); Minecraft mc; mc = Minecraft.getMinecraft(); mc.getLogAgent().logFine("Saber Values packet recieved."); int force = 0; int credits = 0; try { force = inputStream.readInt(); credits = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } ClientProxy.credits = credits; ClientProxy.force = force; } }
-
How would I do an ifRemote check on serverside without an instance of the player or world?
-
If you check for instanceof EntityPlayer in the EntityConstructing event a dummy of your data will also be present on the client. Use packets to sync the data between the client & server version. I've basically done just that.. My main data class now looks like: package mods.XXXX; import java.io.ByteArrayOutputStream; import java.io.DataOutputStream; import cpw.mods.fml.common.network.PacketDispatcher; import cpw.mods.fml.common.network.Player; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.packet.Packet250CustomPayload; import net.minecraft.world.World; import net.minecraftforge.common.IExtendedEntityProperties; public class PlayerVariables implements IExtendedEntityProperties { public static final String IDENTIFIER = "xxxxvalues"; public static PlayerVariables fromPlayer(EntityPlayerMP player) { return (PlayerVariables)player.getExtendedProperties(IDENTIFIER); } int xxx = 0; int yyy = 68; EntityPlayerMP player; @Override public void saveNBTData(NBTTagCompound compound) { compound.setInteger("xxx", xxx); compound.setInteger("yyy", yyy); } @Override public void loadNBTData(NBTTagCompound compound) { if (compound.hasKey("xxx")) { this.addXxx(compound.getInteger("xxx")); } else { this.addXxx(30); } if (compound.hasKey("yyy")) { this.useYyy(68 - (68 - compound.getInteger("yyy"))); } else { this.useYyy(0); } } @Override public void init(Entity entity, World world) { player = (EntityPlayerMP) entity; } public void addXxx(int value) { xxx += value; sendPacket(); } public boolean removeXxx(int value) { if (xxx > value) { xxx -= value; sendPacket(); return true; } else { return false; } } public boolean useYyy(int value) { if (yyy > value) { yyy -= value; sendPacket(); return true; } else { return false; } } private void sendPacket() { ByteArrayOutputStream bos = new ByteArrayOutputStream(; DataOutputStream outputStream = new DataOutputStream(bos); try { outputStream.writeInt(yyy); outputStream.writeInt(xxx); } catch (Exception ex) { ex.printStackTrace(); } Packet250CustomPayload packet = new Packet250CustomPayload(); packet.channel = "XxxxValues"; packet.data = bos.toByteArray(); packet.length = bos.size(); PacketDispatcher.sendPacketToPlayer(packet, (Player)player); } } The entity constructor code is.. package mods.XXXX; import java.io.ByteArrayOutputStream; import java.io.DataOutputStream; import cpw.mods.fml.common.network.PacketDispatcher; import cpw.mods.fml.common.network.Player; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.network.packet.Packet250CustomPayload; import net.minecraftforge.event.EventPriority; import net.minecraftforge.event.ForgeSubscribe; import net.minecraftforge.event.entity.EntityEvent.EntityConstructing; public class EntityConstructListener { @ForgeSubscribe(priority = EventPriority.NORMAL) public void onEntityConstuct(EntityConstructing event) { if (event.entity instanceof EntityPlayerMP) { EntityPlayerMP ep = (EntityPlayerMP) event.entity; ep.registerExtendedProperties(PlayerVariables.IDENTIFIER, new PlayerVariables()); } } } My packet handler is as so: package mods.XXXX; import java.io.ByteArrayInputStream; import java.io.DataInputStream; import java.io.IOException; import mods.XXXX.client.ClientProxy; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.item.ItemStack; import net.minecraft.network.INetworkManager; import net.minecraft.network.packet.Packet250CustomPayload; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.Player; import cpw.mods.fml.relauncher.Side; public class PacketHandler implements IPacketHandler { @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player playerEntity) { Side side = FMLCommonHandler.instance().getEffectiveSide(); if (packet.channel.equals("Some other packet") && side == Side.SERVER) { handleXxxx(packet, playerEntity); // method is hidden <- this one already works } if (packet.channel.equals("XxxVaules") && side == Side.CLIENT) { handleValues(packet); } } private void handleValues(Packet250CustomPayload packet) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int yyy = 0; int xxx = 0; try { yyy = inputStream.readInt(); xxx = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } ClientProxy.xxx = xxx; ClientProxy.yyy = yyy; } } I then use ClientProxy.xxx and ClientProxy.yyy as variables in calculations for a graphical overlay. However, the variables do not set to default when testing on singleplayer, but both are at 0. Why are they 0, they should be send from the data.
-
How is this used properly for both client and server?
-
Thanks, I'll check that out now
-
Nobody?
-
I'm trying to give every player a money variable, but I'm having some trouble. I have created a connection handler like so; @EventHandler public void load(FMLInitializationEvent event) { proxy.init(); NetworkRegistry.instance().registerConnectionHandler(new ConnectionHandler()); } In the playerLoggedIn method I then do this: @Override public void playerLoggedIn(Player player, NetHandler netHandler, INetworkManager manager) { EntityPlayer entityPlayer = (EntityPlayer) player; System.out.println("Player joined!"); NBTTagCompound tag = entityPlayer.getEntityData(); NBTBase modeTag = tag.getTag("credits"); if(modeTag == null) { XXXX.credits.put(entityPlayer.username, 30); tag.setInteger("credits", 30); } else { XXXX.credits.put(entityPlayer.username, ((NBTTagInt)modeTag).data); } ByteArrayOutputStream bos = new ByteArrayOutputStream(4); DataOutputStream outputStream = new DataOutputStream(bos); int credits = 0; for (String key : XXXX.credits.keySet()) { if(key.equalsIgnoreCase(((EntityPlayer)player).username))credits = XXXX.credits.get(key); } try { outputStream.writeInt(credits); } catch (Exception ex) { ex.printStackTrace(); } Packet250CustomPayload packet = new Packet250CustomPayload(); packet.channel = "CreditValues"; packet.data = bos.toByteArray(); packet.length = bos.size(); PacketDispatcher.sendPacketToPlayer(packet, player); } Where XXXX.credits is a HashMap like so: public static HashMap<String, Integer> credits = new HashMap<String, Integer>(); The packet is then handled like so: @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player playerEntity) { if (packet.channel.equals("CreditVaules")) { handleValues(packet); } private void handleValues(Packet250CustomPayload packet) { Side side = FMLCommonHandler.instance().getEffectiveSide(); if (side.isClient()) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int credits = 0; try { credits = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } XXXX.myCredits = credits; } } } Where myCredits is an integer to hold the individual client's credits. I then print this number onto the screen, However, it is always 0. I don't know why this is the case. Can someone help? NOTE: I'm drunk when writing this so if something doesn't make sense just ask.
-
Thanks
-
Thanks
-
Hello, I am interested in using .obj model files in my Minecraft mod. I found this tutorial to load it in. However, I don't seem to have net.minecraftforge.client.model.IModelCustom or net.minecraftforge.client.model.AdvancedModelLoader in my workspace. I am using forge 7.7.1.611. Is this still possible?
-
Hello, I am interested in using .obj model files in my Minecraft mod. I found this tutorial to load it in. However, I don't seem to have net.minecraftforge.client.model.IModelCustom or net.minecraftforge.client.model.AdvancedModelLoader in my workspace. I am using forge 7.7.1.611. Is this still possible?
-
This sounds rather interesting, do you know how it is done, and what dimensions and complexity the model needs to be? Also can they be used for entities? If so, how would texture mapping work? I haven't tried it yet, but it's used in EE3 (it's open source, code is available on github) or you can read this tutorial - looks nice. It can definitely be used for entities (not sure about blocks though, maybe just TE). In 1.5, both net.minecraftforge.client.model.AdvancedModelLoader and net.minecraftforge.client.model.IModelCustom no longer exist. Is it still possible to import .obj files into minecraft?
-
This sounds rather interesting, do you know how it is done, and what dimensions and complexity the model needs to be? Also can they be used for entities? If so, how would texture mapping work? I haven't tried it yet, but it's used in EE3 (it's open source, code is available on github) or you can read this tutorial - looks nice. It can definitely be used for entities (not sure about blocks though, maybe just TE). In 1.5, both net.minecraftforge.client.model.AdvancedModelLoader and net.minecraftforge.client.model.IModelCustom no longer exist. Is it still possible to import .obj files into minecraft?
-
Making block harvest-able only with enchantment
Briggybros replied to Briggybros's topic in Modder Support
Found the problem, the if statement was invalid because there was a semi colon after it. herpy derp derp! -
Making block harvest-able only with enchantment
Briggybros replied to Briggybros's topic in Modder Support
Found the problem, the if statement was invalid because there was a semi colon after it. herpy derp derp! -
This sounds rather interesting, do you know how it is done, and what dimensions and complexity the model needs to be? Also can they be used for entities? If so, how would texture mapping work?
-
Nobody?
-
The code now works, how can I make it forge dependant rather than ModLoader dependant? also, how can I improve the random location picker to choose a random location anywhere in the generated world? it is, at the moment, like this; int x = world.getSpawnPoint().posX + (rand.nextInt(1000) - 500); int z = world.getSpawnPoint().posZ + (rand.nextInt(1000) - 500); int totalY = world.getHeightValue(x, z); int y = totalY - rand.nextInt(totalY - 5);
-
Making block harvest-able only with enchantment
Briggybros replied to Briggybros's topic in Modder Support
Still drops without the enchantment